#include <Store.h>
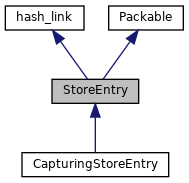
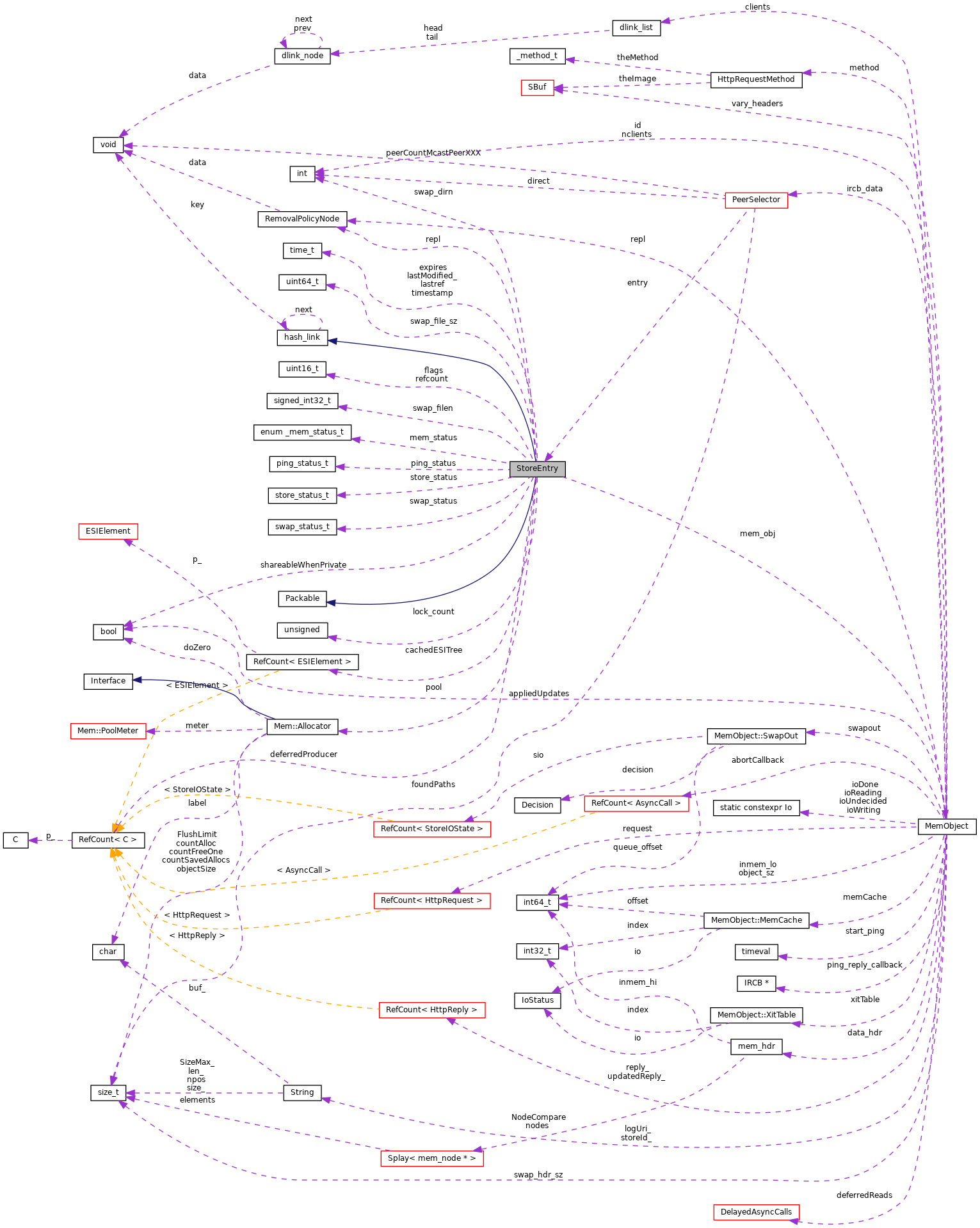
Public Member Functions | |
bool | checkDeferRead (int fd) const |
const char * | getMD5Text () const |
StoreEntry () | |
~StoreEntry () override | |
MemObject & | mem () |
const MemObject & | mem () const |
const HttpReply * | hasFreshestReply () const |
bool | hasParsedReplyHeader () const |
whether this entry has access to [deserialized] [HTTP] response headers More... | |
void | write (StoreIOBuffer) |
bool | isEmpty () const |
bool | isAccepting () const |
size_t | bytesWanted (Range< size_t > const aRange, bool ignoreDelayPool=false) const |
void | completeSuccessfully (const char *whyWeAreSureWeStoredTheWholeReply) |
void | completeTruncated (const char *whyWeConsiderTheReplyTruncated) |
void | complete () |
store_client_t | storeClientType () const |
const char * | getSerialisedMetaData (size_t &length) const |
void | storeErrorResponse (HttpReply *reply) |
Store a prepared error response. MemObject locks the reply object. More... | |
void | replaceHttpReply (const HttpReplyPointer &, const bool andStartWriting=true) |
void | startWriting () |
bool | mayStartSwapOut () |
whether we may start writing to disk (now or in the future) More... | |
void | trimMemory (const bool preserveSwappable) |
void | memOutDecision (const bool willCacheInRam) |
void | swapOutDecision (const MemObject::SwapOut::Decision &decision) |
void | storeWriterDone () |
called when a store writer ends its work (successfully or not) More... | |
void | abort () |
bool | makePublic (const KeyScope keyScope=ksDefault) |
void | makePrivate (const bool shareable) |
void | clearPrivate () |
bool | setPublicKey (const KeyScope keyScope=ksDefault) |
void | clearPublicKeyScope () |
const cache_key * | publicKey () const |
void | setPrivateKey (const bool shareable, const bool permanent) |
void | expireNow () |
void | releaseRequest (const bool shareable=false) |
void | negativeCache () |
bool | cacheNegatively () |
void | invokeHandlers () |
void | cacheInMemory () |
start or continue storing in memory cache More... | |
void | swapOut () |
bool | swappingOut () const |
whether we are in the process of writing this entry to disk More... | |
bool | swappedOut () const |
whether the entire entry is now on disk (possibly marked for deletion) More... | |
bool | swapoutFailed () const |
whether we failed to write this entry to disk More... | |
void | swapOutFileClose (int how) |
const char * | url () const |
bool | checkCachable () |
int | checkNegativeHit () const |
int | locked () const |
int | validToSend () const |
bool | memoryCachable () |
checkCachable() and can be cached in memory More... | |
void | createMemObject () |
void | createMemObject (const char *storeId, const char *logUri, const HttpRequestMethod &aMethod) |
initialize mem_obj with URIs/method; assert if mem_obj already exists More... | |
void | ensureMemObject (const char *storeId, const char *logUri, const HttpRequestMethod &aMethod) |
initialize mem_obj (if needed) and set URIs/method (if missing) More... | |
void | dump (int debug_lvl) const |
void | hashDelete () |
void | hashInsert (const cache_key *) |
void | registerAbortCallback (const AsyncCall::Pointer &) |
notify the StoreEntry writer of a 3rd-party initiated StoreEntry abort More... | |
void | reset () |
void | setMemStatus (mem_status_t) |
bool | timestampsSet () |
void | unregisterAbortCallback (const char *reason) |
void | destroyMemObject () |
int | checkTooSmall () |
void | setNoDelay (bool const) |
void | lastModified (const time_t when) |
time_t | lastModified () const |
const char * | describeTimestamps () const |
bool | modifiedSince (const time_t ims, const int imslen=-1) const |
bool | hasIfMatchEtag (const HttpRequest &request) const |
has ETag matching at least one of the If-Match etags More... | |
bool | hasIfNoneMatchEtag (const HttpRequest &request) const |
has ETag matching at least one of the If-None-Match etags More... | |
bool | hasEtag (ETag &etag) const |
whether this entry has an ETag; if yes, puts ETag value into parameter More... | |
bool | updateOnNotModified (const StoreEntry &e304) |
Store::Disk & | disk () const |
the disk this entry is [being] cached on; asserts for entries w/o a disk More... | |
bool | hasDisk (const sdirno dirn=-1, const sfileno filen=-1) const |
void | attachToDisk (const sdirno, const sfileno, const swap_status_t) |
void | detachFromDisk () |
bool | hasTransients () const |
whether there is a corresponding locked transients table entry More... | |
bool | hasMemStore () const |
whether there is a corresponding locked shared memory table entry More... | |
bool | hittingRequiresCollapsing () const |
whether this entry can feed collapsed requests and only them More... | |
void | setCollapsingRequirement (const bool required) |
allow or forbid collapsed requests feeding More... | |
void * | operator new (size_t byteCount) |
void | operator delete (void *address) |
int64_t | objectLen () const |
int64_t | contentLen () const |
void | lock (const char *context) |
int | unlock (const char *context) |
int | locks () const |
returns a local concurrent use counter, for debugging More... | |
void | touch () |
update last reference timestamp and related Store metadata More... | |
void | release (const bool shareable=false) |
void | abandon (const char *context) |
bool | mayStartHitting () const |
void | deferProducer (const AsyncCall::Pointer &producer) |
call back producer when more buffer space is available More... | |
void | kickProducer () |
calls back producer registered with deferProducer More... | |
void | append (char const *, int) override |
Appends a c-string to existing packed data. More... | |
void | vappendf (const char *, va_list) override |
void | buffer () override |
void | flush () override |
void | appendf (const char *fmt,...) PRINTF_FORMAT_ARG2 |
Append operation with printf-style arguments. More... | |
Static Public Member Functions | |
static size_t | inUseCount () |
Public Attributes | |
MemObject * | mem_obj |
RemovalPolicyNode | repl |
time_t | timestamp |
time_t | lastref |
time_t | expires |
uint64_t | swap_file_sz |
uint16_t | refcount |
uint16_t | flags |
sfileno | swap_filen:25 |
unique ID inside a cache_dir for swapped out entries; -1 for others More... | |
sdirno | swap_dirn:7 |
mem_status_t | mem_status:3 |
ping_status_t | ping_status:3 |
store_status_t | store_status:3 |
swap_status_t | swap_status:3 |
void * | key |
hash_link * | next |
Protected Types | |
typedef Store::EntryGuard | EntryGuard |
Protected Member Functions | |
void | storeWritingCheckpoint () |
void | checkDisk () const |
does nothing except throwing if disk-associated data members are inconsistent More... | |
Private Member Functions | |
void | doAbandon (const char *context) |
bool | checkTooBig () const |
void | forcePublicKey (const cache_key *newkey) |
StoreEntry * | adjustVary () |
const cache_key * | calcPublicKey (const KeyScope keyScope) |
void | lengthWentBad (const char *reason) |
flags [truncated or too big] entry with ENTRY_BAD_LENGTH and releases it More... | |
bool | validLength () const |
bool | hasOneOfEtags (const String &reqETags, const bool allowWeakMatch) const |
whether at least one of the request ETags matches entity ETag More... | |
Private Attributes | |
time_t | lastModified_ |
received Last-Modified value or -1; use lastModified() More... | |
unsigned short | lock_count |
bool | shareableWhenPrivate |
AsyncCall::Pointer | deferredProducer |
producer callback registered with deferProducer More... | |
Static Private Attributes | |
static Mem::Allocator * | pool = nullptr |
Friends | |
std::ostream & | operator<< (std::ostream &os, const StoreEntry &e) |
Detailed Description
Member Typedef Documentation
◆ EntryGuard
|
protected |
Constructor & Destructor Documentation
◆ StoreEntry()
◆ ~StoreEntry()
Member Function Documentation
◆ abandon()
|
inline |
One of the three methods to get rid of an unlocked StoreEntry object. May destroy this object if it is unlocked; does nothing otherwise. Unlike release(), may not trigger eviction of underlying store entries, but, unlike destroyStoreEntry(), does honor an earlier release request.
Definition at line 280 of file Store.h.
References doAbandon(), and locked().
Referenced by unlock().
◆ abort()
void StoreEntry::abort | ( | ) |
Definition at line 1077 of file store.cc.
References MemObject::abortCallback, StatCounters::aborted_requests, assert, debugs, EBIT_CLR, EBIT_SET, ENTRY_ABORTED, ENTRY_FWD_HDR_WAIT, flags, getMD5Text(), invokeHandlers(), lock(), mem_obj, negativeCache(), NOT_IN_MEMORY, releaseRequest(), ScheduleCallHere, setMemStatus(), statCounter, STORE_OK, STORE_PENDING, store_status, swapOutFileClose(), unlock(), and StoreIOState::writerGone.
Referenced by FwdState::connectedToPeer(), FwdState::dispatch(), peerCountMcastPeersAbort(), storeUnregister(), swapOut(), and Store::Controller::syncCollapsed().
◆ adjustVary()
|
private |
Updates mem_obj->request->vary_headers to reflect the current Vary. The vary_headers field is used to calculate the Vary marker key. Releases the old Vary marker with an outdated key (if any).
- Returns
- new (locked) Vary marker StoreEntry or, if none was needed, nil
- Exceptions
-
std::exception on failures
Definition at line 665 of file store.cc.
References assert, SBuf::clear(), SBuf::cmp(), completeSuccessfully(), HttpRequest::flags, MemObject::freshestReply(), RefCount< C >::getRaw(), Http::HDR_X_ACCELERATOR_VARY, Http::Message::header, httpMakeVaryMark(), SBuf::isEmpty(), MemObject::logUri(), makePublic(), mem_obj, HttpRequest::method, MemObject::method, HttpHeader::putStr(), replaceHttpReply(), MemObject::request, Http::scOkay, HttpReply::setHeaders(), squid_curtime, startWriting(), storeCreateEntry(), storeGetPublic(), MemObject::storeId(), TexcHere, timestampsSet(), unlock(), Http::VARY, HttpRequest::vary_headers, and MemObject::vary_headers.
Referenced by setPublicKey().
◆ append()
|
overridevirtual |
Implements Packable.
Reimplemented in CapturingStoreEntry.
Definition at line 803 of file store.cc.
References assert, MemObject::baseReply(), StoreIOBuffer::data, MemObject::endOffset(), Http::Message::hdr_sz, StoreIOBuffer::length, mem_obj, StoreIOBuffer::offset, STORE_PENDING, store_status, and write().
Referenced by Format::Format::dump(), dump_cache_log_message(), dump_SBufList(), DumpDirective(), DumpInfo(), internalStart(), MimeIcon::load(), netdbBinaryExchange(), WhoisState::readReply(), statObjects(), storeDigestCBlockSwapOut(), storeDigestSwapOutStep(), and vappendf().
◆ appendf()
|
inlineinherited |
Definition at line 61 of file Packable.h.
References Packable::vappendf().
Referenced by Ftp::Server::acceptDataConnection(), Format::Format::assemble(), HttpStateData::buildRequestPrefix(), clientPackRangeHdr(), clientPackTermBound(), ErrorState::compileLegacyCode(), EventScheduler::dump(), dump_cachemgrpasswd(), store_client::dumpStats(), Adaptation::Icap::ModXact::encapsulateHead(), errorInitialize(), Adaptation::Icap::Xaction::fillDoneStatus(), Adaptation::Icap::ModXact::fillDoneStatus(), Adaptation::Icap::Xaction::fillPendingStatus(), Adaptation::Icap::ModXact::fillPendingStatus(), HttpStateData::getMoreRequestBody(), Ftp::Server::handleEpsvReply(), Ftp::Server::handlePasvReply(), helperDispatch(), httpHdrContRangePackInto(), httpHdrRangeRespSpecPackInto(), internalRemoteUri(), Adaptation::Icap::ModXact::makeAllowHeader(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Icap::ModXact::makeUsernameHeader(), memBufReport(), Adaptation::Icap::ModXact::openChunk(), HttpRequest::pack(), Http::Stream::packChunk(), HttpRequest::packFirstLineInto(), HttpHdrRangeSpec::packInto(), Http::StatusLine::packInto(), HttpHdrScTarget::packInto(), HttpHdrCc::packInto(), Helper::Client::packStatsInto(), Ftp::PrintReply(), Ftp::Client::sendEprt(), Ftp::Client::sendPassive(), ACLExternal::startLookup(), MemObject::stat(), statStoreEntry(), Ipc::Inquirer::status(), Comm::TcpAcceptor::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), Adaptation::Icap::ServiceRep::status(), wordlistCat(), Ftp::Server::writeCustomReply(), Ftp::Server::writeEarlyReply(), Ftp::Server::writeErrorReply(), and Http::Tunneler::writeRequest().
◆ attachToDisk()
void StoreEntry::attachToDisk | ( | const sdirno | dirn, |
const sfileno | fno, | ||
const swap_status_t | status | ||
) |
Makes hasDisk(dirn, filn) true. The caller should have locked the corresponding disk store entry for reading or writing.
Definition at line 1940 of file store.cc.
References asHex(), checkDisk(), debugs, getMD5Text(), swap_dirn, swap_filen, swap_status, and swapStatusStr.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), Rock::SwapDir::anchorEntry(), and storeSwapOutStart().
◆ buffer()
|
overridevirtual |
disable sending content to the clients.
This just sets DELAY_SENDING.
Reimplemented from Packable.
Reimplemented in CapturingStoreEntry.
Definition at line 1601 of file store.cc.
References DELAY_SENDING, EBIT_SET, and flags.
Referenced by TestRock::addEntry(), Mgr::Action::fillEntry(), MimeIcon::load(), netdbBinaryExchange(), WhoisState::setReplyToOK(), startWriting(), statObjects(), storeDigestRewriteResume(), storeErrorResponse(), HttpRequest::swapOut(), TestUfs::testUfsSearch(), clientReplyContext::traceReply(), and urnHandleReply().
◆ bytesWanted()
Definition at line 213 of file store.cc.
References Range< C, S >::end, mem_obj, MemObject::mostBytesWanted(), and MemObject::readAheadPolicyCanRead().
Referenced by checkDeferRead(), Client::handleMoreAdaptedBodyAvailable(), ClientHttpRequest::noteMoreBodyDataAvailable(), and HttpStateData::readReply().
◆ cacheInMemory()
void StoreEntry::cacheInMemory | ( | ) |
◆ cacheNegatively()
bool StoreEntry::cacheNegatively | ( | ) |
Definition at line 188 of file store.cc.
References makePublic(), and negativeCache().
Referenced by HttpStateData::haveParsedReplyHeaders().
◆ calcPublicKey()
Calculates correct public key for feeding forcePublicKey(). Assumes adjustVary() has been called for this entry already.
Definition at line 652 of file store.cc.
References assert, RefCount< C >::getRaw(), mem_obj, MemObject::method, MemObject::request, MemObject::storeId(), storeKeyPublic(), and storeKeyPublicByRequest().
Referenced by clearPublicKeyScope(), and setPublicKey().
◆ checkCachable()
bool StoreEntry::checkCachable | ( | ) |
Satisfies cachability requirements shared among disk and RAM caches. Encapsulates common checks of mayStartSwapOut() and memoryCachable(). TODO: Rename and make private so only those two methods can call this.
Definition at line 929 of file store.cc.
References checkTooBig(), checkTooSmall(), debugs, _store_check_cachable_hist::Default, EBIT_TEST, ENTRY_BAD_LENGTH, fdNFree(), flags, hasDisk(), KEY_PRIVATE, mem_obj, _store_check_cachable_hist::missing_parts, _store_check_cachable_hist::no, _store_check_cachable_hist::not_entry_cachable, _store_check_cachable_hist::private_key, RELEASE_REQUEST, releaseRequest(), RESERVED_FD, store_check_cachable_hist, storeTooManyDiskFilesOpen(), _store_check_cachable_hist::too_big, _store_check_cachable_hist::too_many_open_fds, _store_check_cachable_hist::too_many_open_files, _store_check_cachable_hist::too_small, _store_check_cachable_hist::wrong_content_length, and _store_check_cachable_hist::yes.
Referenced by mayStartSwapOut(), memoryCachable(), and storeSwapOutFileClosed().
◆ checkDeferRead()
bool StoreEntry::checkDeferRead | ( | int | fd | ) | const |
Definition at line 244 of file store.cc.
References bytesWanted(), and INT_MAX.
Referenced by statObjects(), and swapOut().
◆ checkDisk()
|
protected |
Definition at line 1961 of file store.cc.
References SquidConfig::cacheSwap, Config, CurrentException(), DBG_IMPORTANT, debugs, EBIT_TEST, flags, Must, Store::DiskConfig::n_configured, RELEASE_REQUEST, swap_dirn, swap_filen, swap_status, SWAPOUT_NONE, swapoutFailed(), swappedOut(), and swappingOut().
Referenced by attachToDisk(), and hasDisk().
◆ checkNegativeHit()
int StoreEntry::checkNegativeHit | ( | ) | const |
Definition at line 1300 of file store.cc.
References EBIT_TEST, ENTRY_NEGCACHED, expires, flags, squid_curtime, STORE_OK, and store_status.
Referenced by clientReplyContext::cacheHit().
◆ checkTooBig()
|
private |
Definition at line 918 of file store.cc.
References MemObject::baseReply(), Http::Message::content_length, MemObject::endOffset(), mem_obj, and store_maxobjsize.
Referenced by checkCachable().
◆ checkTooSmall()
int StoreEntry::checkTooSmall | ( | ) |
Definition at line 901 of file store.cc.
References MemObject::baseReply(), Config, Http::Message::content_length, EBIT_TEST, ENTRY_SPECIAL, flags, mem(), mem_obj, SquidConfig::minObjectSize, MemObject::object_sz, SquidConfig::Store, STORE_OK, and store_status.
Referenced by checkCachable().
◆ clearPrivate()
void StoreEntry::clearPrivate | ( | ) |
A low-level method just resetting "private key" flags. To avoid key inconsistency please use forcePublicKey() or similar instead.
Definition at line 180 of file store.cc.
References assert, EBIT_CLR, EBIT_TEST, flags, KEY_PRIVATE, RELEASE_REQUEST, and shareableWhenPrivate.
Referenced by forcePublicKey().
◆ clearPublicKeyScope()
void StoreEntry::clearPublicKeyScope | ( | ) |
Resets existing public key to a public key with default scope, releasing the old default-scope entry (if any). Does nothing if the existing public key already has default scope.
Definition at line 609 of file store.cc.
References calcPublicKey(), EBIT_TEST, flags, forcePublicKey(), hash_link::key, KEY_PRIVATE, ksDefault, and storeKeyHashCmp.
Referenced by clientReplyContext::sendClientUpstreamResponse().
◆ complete()
void StoreEntry::complete | ( | ) |
- Deprecated:
- use either completeSuccessfully() or completeTruncated() instead
Definition at line 1031 of file store.cc.
References assert, current_time, debugs, EBIT_CLR, EBIT_TEST, MemObject::endOffset(), ENTRY_ABORTED, ENTRY_BAD_LENGTH, ENTRY_FWD_HDR_WAIT, flags, getMD5Text(), HttpRequest::hier, invokeHandlers(), lengthWentBad(), mem_obj, mem_status, MemObject::nclients, NOT_IN_MEMORY, MemObject::object_sz, MemObject::request, HierarchyLogEntry::store_complete_stop, STORE_OK, STORE_PENDING, store_status, and validLength().
Referenced by TestRock::addEntry(), completeSuccessfully(), completeTruncated(), Mgr::Action::fillEntry(), ClientHttpRequest::handleAdaptedHeader(), internalStart(), MimeIcon::load(), netdbBinaryExchange(), clientReplyContext::processMiss(), clientReplyContext::purgeDoPurge(), clientReplyContext::sendNotModified(), CacheManager::start(), statObjects(), storeDigestRewriteFinish(), TestUfs::testUfsSearch(), clientReplyContext::traceReply(), and urnHandleReply().
◆ completeSuccessfully()
void StoreEntry::completeSuccessfully | ( | const char * | whyWeAreSureWeStoredTheWholeReply | ) |
Signals that the entire response has been stored and no more append() calls should be expected; cf. completeTruncated().
Definition at line 1017 of file store.cc.
References complete(), and debugs.
Referenced by adjustVary(), FwdState::completed(), ClientHttpRequest::endRequestSatisfaction(), and storeErrorResponse().
◆ completeTruncated()
void StoreEntry::completeTruncated | ( | const char * | whyWeConsiderTheReplyTruncated | ) |
Signals that a partial response (if any) has been stored but no more append() calls should be expected; cf. completeSuccessfully().
Definition at line 1024 of file store.cc.
References complete(), and lengthWentBad().
Referenced by FwdState::completed(), and ClientHttpRequest::endRequestSatisfaction().
◆ contentLen()
|
inline |
Definition at line 254 of file Store.h.
References MemObject::baseReply(), Http::Message::hdr_sz, mem(), and objectLen().
Referenced by ClientHttpRequest::logRequest(), store_client::moreToRead(), and storeLog().
◆ createMemObject() [1/2]
void StoreEntry::createMemObject | ( | ) |
initialize mem_obj; assert if mem_obj already exists avoid this method in favor of createMemObject(trio)!
Definition at line 1575 of file store.cc.
References assert, and mem_obj.
Referenced by Rock::SwapDir::get(), MemStore::get(), Transients::get(), storeCreatePureEntry(), TestStoreHashIndex::testMaxSize(), TestStoreController::testMaxSize(), TestCacheManager::testRegister(), TestStoreController::testStats(), and TestStoreHashIndex::testStats().
◆ createMemObject() [2/2]
void StoreEntry::createMemObject | ( | const char * | storeId, |
const char * | logUri, | ||
const HttpRequestMethod & | aMethod | ||
) |
Definition at line 1582 of file store.cc.
References assert, ensureMemObject(), and mem_obj.
◆ deferProducer()
void StoreEntry::deferProducer | ( | const AsyncCall::Pointer & | producer | ) |
Definition at line 366 of file store.cc.
References debugs, and deferredProducer.
Referenced by Client::handleMoreAdaptedBodyAvailable(), and ClientHttpRequest::noteMoreBodyDataAvailable().
◆ describeTimestamps()
const char * StoreEntry::describeTimestamps | ( | ) | const |
- Returns
- a formatted string with entry's timestamps
Definition at line 2000 of file store.cc.
References expires, lastModified_, lastref, LOCAL_ARRAY, and timestamp.
Referenced by statStoreEntry(), and timestampsSet().
◆ destroyMemObject()
void StoreEntry::destroyMemObject | ( | ) |
Definition at line 386 of file store.cc.
References debugs, hasMemStore(), hasTransients(), mem_obj, Store::Controller::memoryDisconnect(), NOT_IN_MEMORY, Store::Root(), setMemStatus(), and Store::Controller::transientsDisconnect().
Referenced by destroyStoreEntry(), MemStore::evictCached(), and Store::Controller::memoryEvictCached().
◆ detachFromDisk()
void StoreEntry::detachFromDisk | ( | ) |
Makes hasDisk() false. The caller should have unlocked the corresponding disk store entry.
Definition at line 1953 of file store.cc.
References swap_dirn, swap_filen, swap_status, and SWAPOUT_NONE.
Referenced by Rock::SwapDir::disconnect(), and Fs::Ufs::UFSSwapDir::evictCached().
◆ disk()
Store::Disk & StoreEntry::disk | ( | ) | const |
Definition at line 1920 of file store.cc.
References assert, hasDisk(), INDEXSD, and swap_dirn.
Referenced by Store::Disks::dereference(), destroyStoreEntry(), Store::Disks::evictCached(), Store::Disks::reference(), storeCleanup(), storeDirSwapLog(), storeOpen(), storeSwapOutFileClosed(), Store::Disks::updateAnchored(), and Store::Disks::updateHeaders().
◆ doAbandon()
|
private |
keep the unlocked StoreEntry object in the local store_table (if needed) or delete it (otherwise)
Definition at line 486 of file store.cc.
References assert, debugs, EBIT_TEST, flags, Store::Controller::handleIdleEntry(), locked(), release(), RELEASE_REQUEST, Store::Root(), STORE_PENDING, store_status, and storePendingNClients().
Referenced by abandon().
◆ dump()
void StoreEntry::dump | ( | int | debug_lvl | ) | const |
Definition at line 1499 of file store.cc.
References debugs, expires, getMD5Text(), lastModified_, lastref, lock_count, mem_obj, mem_status, hash_link::next, ping_status, refcount, store_status, storeEntryFlags(), swap_dirn, swap_file_sz, swap_filen, swap_status, and timestamp.
Referenced by clientReplyContext::buildReplyHeader(), Fs::Ufs::UFSSwapDir::dumpEntry(), and clientReplyContext::processMiss().
◆ ensureMemObject()
void StoreEntry::ensureMemObject | ( | const char * | storeId, |
const char * | logUri, | ||
const HttpRequestMethod & | aMethod | ||
) |
Definition at line 1589 of file store.cc.
References mem_obj, and MemObject::setUris().
Referenced by createMemObject(), clientReplyContext::doGetMoreData(), peerDigestRequest(), and clientReplyContext::processExpired().
◆ expireNow()
void StoreEntry::expireNow | ( | ) |
Definition at line 773 of file store.cc.
References debugs, expires, getMD5Text(), and squid_curtime.
◆ flush()
|
overridevirtual |
flush any buffered content.
This just clears DELAY_SENDING and Invokes the handlers to begin sending anything that may be buffered.
Reimplemented from Packable.
Reimplemented in CapturingStoreEntry.
Definition at line 1612 of file store.cc.
References DELAY_SENDING, EBIT_CLR, EBIT_TEST, flags, and invokeHandlers().
Referenced by TestRock::addEntry(), Mgr::Action::fillEntry(), MimeIcon::load(), netdbBinaryExchange(), WhoisState::readReply(), startWriting(), statObjects(), storeDigestRewriteResume(), storeErrorResponse(), HttpRequest::swapOut(), and TestUfs::testUfsSearch().
◆ forcePublicKey()
|
private |
Unconditionally sets public key for this store entry. Releases the old entry with the same public key (if any).
Definition at line 626 of file store.cc.
References assert, clearPrivate(), debugs, hasDisk(), hash_lookup(), hashDelete(), hashInsert(), MemObject::hasUris(), hash_link::key, mem_obj, store_table, storeDirSwapLog(), storeKeyText(), and SWAP_LOG_ADD.
Referenced by clearPublicKeyScope(), and setPublicKey().
◆ getMD5Text()
const char * StoreEntry::getMD5Text | ( | ) | const |
Definition at line 207 of file store.cc.
References hash_link::key, and storeKeyText().
Referenced by abort(), attachToDisk(), Store::CheckSwapMetaKey(), complete(), store_client::copy(), dump(), expireNow(), HeapKeyGen_StoreEntry_GDSF(), HeapKeyGen_StoreEntry_LFUDA(), HeapKeyGen_StoreEntry_LRU(), invokeHandlers(), lock(), neighborsUdpPing(), HttpStateData::processReplyHeader(), ClientHttpRequest::rangeBoundaryStr(), release(), setMemStatus(), statClientRequests(), statStoreEntry(), storeClientCopy2(), storeDigestAdd(), storeDigestAddable(), storeDigestDel(), storeDigestRewriteStart(), storeDirSwapLog(), storeLog(), storeSwapInStart(), storeUnregister(), swapOutFileClose(), unlock(), validLength(), and write().
◆ getSerialisedMetaData()
const char * StoreEntry::getSerialisedMetaData | ( | size_t & | length | ) | const |
- Returns
- a malloc()ed buffer containing a length-long packed swap header
Definition at line 1750 of file store.cc.
References Store::PackSwapMeta().
Referenced by storeSwapOutStart().
◆ hasDisk()
whether one of this StoreEntry owners has locked the corresponding disk entry (at the specified disk entry coordinates, if any)
Definition at line 1929 of file store.cc.
References checkDisk(), Must, swap_dirn, and swap_filen.
Referenced by MemStore::anchorEntry(), Store::Disks::anchorToCache(), Rock::SwapDir::anchorToCache(), Store::Controller::anchorToCache(), Store::Disk::canLog(), checkCachable(), Store::Controller::dereferenceIdle(), destroyStoreEntry(), Rock::SwapDir::disconnect(), disk(), Store::Disks::evictCached(), Rock::SwapDir::evictCached(), Fs::Ufs::UFSSwapDir::evictCached(), forcePublicKey(), mayStartSwapOut(), store_client::moreToRead(), neighborsUdpPing(), Rock::SwapDir::openStoreIO(), Store::Controller::referenceBusy(), release(), Fs::Ufs::UFSSwapDir::replacementRemove(), store_client::store_client(), storeCleanup(), storeDirSwapLog(), storeSwapInStart(), storeSwapOutStart(), swapOut(), Store::Controller::syncCollapsed(), Store::Disks::updateAnchored(), Rock::SwapDir::updateAnchored(), and validToSend().
◆ hasEtag()
bool StoreEntry::hasEtag | ( | ETag & | etag | ) | const |
Definition at line 1862 of file store.cc.
References Http::ETAG, hasFreshestReply(), and ETag::str.
Referenced by clientReplyContext::processExpired().
◆ hasFreshestReply()
|
inline |
- Return values
-
* the address of freshest reply (if mem_obj exists) nullptr when mem_obj does not exist
- See also
- MemObject::freshestReply()
Definition at line 53 of file Store.h.
References MemObject::freshestReply(), and mem_obj.
Referenced by hasEtag(), and refreshCheck().
◆ hashDelete()
void StoreEntry::hashDelete | ( | ) |
Definition at line 433 of file store.cc.
References hash_remove_link(), hash_link::key, store_table, and storeKeyFree().
Referenced by destroyStoreEntry(), forcePublicKey(), and setPrivateKey().
◆ hashInsert()
void StoreEntry::hashInsert | ( | const cache_key * | someKey | ) |
Definition at line 424 of file store.cc.
References assert, debugs, hash_join(), hash_link::key, store_table, storeKeyDup(), and storeKeyText().
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), Store::Controller::addReading(), forcePublicKey(), and setPrivateKey().
◆ hasIfMatchEtag()
bool StoreEntry::hasIfMatchEtag | ( | const HttpRequest & | request | ) | const |
Definition at line 1873 of file store.cc.
References HttpHeader::getList(), hasOneOfEtags(), Http::Message::header, and Http::IF_MATCH.
Referenced by clientReplyContext::processConditional().
◆ hasIfNoneMatchEtag()
bool StoreEntry::hasIfNoneMatchEtag | ( | const HttpRequest & | request | ) | const |
Definition at line 1880 of file store.cc.
References HttpRequest::flags, HttpHeader::getList(), hasOneOfEtags(), Http::Message::header, Http::IF_NONE_MATCH, RequestFlags::isRanged, HttpRequest::method, Http::METHOD_GET, and Http::METHOD_HEAD.
Referenced by clientReplyContext::processConditional().
◆ hasMemStore()
|
inline |
Definition at line 212 of file Store.h.
References MemObject::MemCache::index, mem_obj, and MemObject::memCache.
Referenced by MemStore::anchorToCache(), Store::Controller::anchorToCache(), destroyMemObject(), MemStore::disconnect(), MemStore::evictCached(), operator<<(), Store::Controller::syncCollapsed(), and MemStore::updateAnchored().
◆ hasOneOfEtags()
|
private |
Definition at line 1891 of file store.cc.
References String::append(), Http::ETAG, etagIsStrongEqual(), etagIsWeakEqual(), etagParseInit(), MemObject::freshestReply(), HttpHeader::getETag(), Http::Message::header, mem(), strListGetItem(), strListIsMember(), and String::termedBuf().
Referenced by hasIfMatchEtag(), and hasIfNoneMatchEtag().
◆ hasParsedReplyHeader()
bool StoreEntry::hasParsedReplyHeader | ( | ) | const |
Definition at line 231 of file store.cc.
References MemObject::baseReply(), debugs, mem_obj, and Http::Message::psParsed.
Referenced by MemStore::copyFromShm(), store_client::handleBodyFromDisk(), and clientReplyContext::storeNotOKTransferDone().
◆ hasTransients()
|
inline |
Definition at line 210 of file Store.h.
References MemObject::XitTable::index, mem_obj, and MemObject::xitTable.
Referenced by Transients::addEntry(), Store::Controller::allowCollapsing(), Store::Controller::allowSharing(), Store::Controller::anchorToCache(), CollapsedForwarding::Broadcast(), Store::Controller::checkFoundCandidate(), Store::Controller::checkTransients(), Transients::completeWriting(), destroyMemObject(), Transients::disconnect(), Transients::evictCached(), Transients::hasWriter(), Transients::monitorIo(), Store::Controller::noteStoppedSharedWriting(), operator<<(), Transients::readers(), Transients::status(), storeWritingCheckpoint(), Store::Controller::transientReaders(), Store::Controller::transientsReader(), and Store::Controller::transientsWriter().
◆ hittingRequiresCollapsing()
|
inline |
Definition at line 215 of file Store.h.
References EBIT_TEST, ENTRY_REQUIRES_COLLAPSING, and flags.
Referenced by Store::Controller::allowCollapsing(), Store::Controller::allowSharing(), Store::Controller::checkFoundCandidate(), ICPState::confirmAndPrepHit(), Store::Controller::dereferenceIdle(), Ipc::StoreMapAnchor::exportInto(), setCollapsingRequirement(), and StoreClient::startCollapsingOn().
◆ inUseCount()
|
static |
Definition at line 199 of file store.cc.
References Mem::Allocator::getInUseCount(), and pool.
Referenced by Store::Controller::getStats(), Store::Controller::stat(), and storeDigestCalcCap().
◆ invokeHandlers()
void StoreEntry::invokeHandlers | ( | ) |
Definition at line 838 of file store_client.cc.
References MemObject::clients, CodeContext::Current(), debugs, DELAY_SENDING, EBIT_TEST, ENTRY_FWD_HDR_WAIT, flags, getMD5Text(), dlink_list::head, mem_obj, MemObject::nclients, node::next, CodeContext::Reset(), sc, storeClientCopy2(), and swapOut().
Referenced by abort(), complete(), flush(), Store::Controller::syncCollapsed(), and write().
◆ isAccepting()
bool StoreEntry::isAccepting | ( | ) | const |
Definition at line 1988 of file store.cc.
References EBIT_TEST, ENTRY_ABORTED, flags, STORE_PENDING, and store_status.
Referenced by Client::abortOnBadEntry(), Store::Controller::checkFoundCandidate(), HttpStateData::processReplyBody(), WhoisState::readReply(), and urnHandleReply().
◆ isEmpty()
|
inline |
Check if the Store entry is empty
- Return values
-
true Store contains 0 bytes of data. false Store contains 1 or more bytes of data. false Store contains negative content !!!!!!
Definition at line 65 of file Store.h.
References MemObject::endOffset(), and mem().
Referenced by FwdState::checkRetry(), FwdState::completed(), Ssl::ServerBump::connectedOk(), errorAppendEntry(), Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), ClientHttpRequest::handleAdaptationFailure(), Client::handledEarlyAdaptationAbort(), HttpStateData::handleRequestBodyProducerAborted(), FwdState::retryOrBail(), ConnStateData::serveDelayedError(), startWriting(), and storeClientType().
◆ kickProducer()
void StoreEntry::kickProducer | ( | ) |
Definition at line 376 of file store.cc.
References deferredProducer, and ScheduleCallHere.
Referenced by store_client::copy(), and storeUnregister().
◆ lastModified() [1/2]
|
inline |
- Returns
- entry's 'effective' modification time
Definition at line 177 of file Store.h.
References lastModified_, and timestamp.
Referenced by modifiedSince(), and timestampsSet().
◆ lastModified() [2/2]
|
inline |
Definition at line 175 of file Store.h.
References lastModified_.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), clientReplyContext::cacheHit(), Ipc::StoreMapAnchor::exportInto(), htcpTstReply(), Fs::Ufs::UFSSwapDir::logEntry(), peerDigestFetchSetStats(), peerDigestRequest(), clientReplyContext::processExpired(), Fs::Ufs::RebuildState::rebuildFromDirectory(), refreshIsCachable(), refreshStaleness(), Ipc::StoreMapAnchor::set(), Store::UnpackIndexSwapMeta(), and UFSCleanLog::write().
◆ lengthWentBad()
|
private |
Definition at line 1009 of file store.cc.
References debugs, EBIT_SET, ENTRY_BAD_LENGTH, flags, and releaseRequest().
Referenced by complete(), and completeTruncated().
◆ lock()
void StoreEntry::lock | ( | const char * | context | ) |
claim shared ownership of this entry (for use in a given context) matching lock() and unlock() contexts eases leak triage but is optional
Definition at line 445 of file store.cc.
References debugs, getMD5Text(), and lock_count.
Referenced by abort(), Client::Client(), store_client::copy(), clientReplyContext::doGetMoreData(), clientReplyContext::forgetHit(), Mgr::Forwarder::Forwarder(), FwdState::FwdState(), heap_purgeNext(), Rock::IoState::IoState(), MimeIcon::load(), ClientHttpRequest::loggingEntry(), peerDigestRequest(), clientReplyContext::processExpired(), release(), Ssl::ServerBump::ServerBump(), clientReplyContext::setReplyToStoreEntry(), UrnState::start(), statObjectsStart(), store_client::store_client(), storeCreateEntry(), storeErrorResponse(), Ipc::StoreMapUpdate::StoreMapUpdate(), storeSwapOutStart(), storeUnregister(), TestPackableStream::testGetStream(), and whoisStart().
◆ locked()
|
inline |
Definition at line 145 of file Store.h.
References lock_count.
Referenced by abandon(), Store::Controller::checkFoundCandidate(), FwdState::dispatch(), doAbandon(), Store::EntryGuard::EntryGuard(), Rock::SwapDir::evictCached(), Fs::Ufs::UFSSwapDir::evictCached(), MemStore::evictCached(), heap_purgeNext(), lru_purgeNext(), lru_stats(), Store::Controller::memoryEvictCached(), neighborsHtcpReply(), neighborsUdpAck(), release(), storeDigestRewriteResume(), and Store::Controller::syncCollapsed().
◆ locks()
|
inline |
Definition at line 265 of file Store.h.
References lock_count.
Referenced by operator<<(), and statStoreEntry().
◆ makePrivate()
void StoreEntry::makePrivate | ( | const bool | shareable | ) |
Definition at line 174 of file store.cc.
References releaseRequest().
Referenced by HttpStateData::haveParsedReplyHeaders(), HttpStateData::processSurrogateControl(), and WhoisState::readReply().
◆ makePublic()
Definition at line 167 of file store.cc.
References EBIT_TEST, flags, RELEASE_REQUEST, and setPublicKey().
Referenced by adjustVary(), Store::Controller::allowCollapsing(), cacheNegatively(), HttpStateData::haveParsedReplyHeaders(), Ftp::Gateway::haveParsedReplyHeaders(), and WhoisState::readReply().
◆ mayStartHitting()
|
inline |
May the caller commit to treating this [previously locked] entry as a cache hit?
Definition at line 284 of file Store.h.
References EBIT_TEST, flags, KEY_PRIVATE, and shareableWhenPrivate.
Referenced by clientReplyContext::cacheHit(), and clientReplyContext::handleIMSReply().
◆ mayStartSwapOut()
bool StoreEntry::mayStartSwapOut | ( | ) |
Definition at line 328 of file store_swapout.cc.
References Store::Controller::accumulateMore(), assert, SquidConfig::cacheSwap, checkCachable(), Config, debugs, MemObject::SwapOut::decision, EBIT_TEST, MemObject::endOffset(), ENTRY_ABORTED, ENTRY_SPECIAL, MemObject::expectedReplySize(), flags, hasDisk(), MemObject::inmem_lo, MemObject::isContiguous(), mem_obj, Store::DiskConfig::n_configured, Store::Root(), shutting_down, store_maxobjsize, STORE_OK, store_status, MemObject::swapout, swapOutDecision(), swappingOut(), MemObject::SwapOut::swImpossible, MemObject::SwapOut::swPossible, MemObject::SwapOut::swStarted, and url().
Referenced by swapOut().
◆ mem() [1/2]
|
inline |
Definition at line 47 of file Store.h.
References assert, and mem_obj.
Referenced by TestRock::addEntry(), MemStore::anchorToCache(), Store::Controller::anchorToCache(), Client::blockCaching(), clientReplyContext::blockedHit(), store_client::canReadFromMemory(), Store::CheckSwapMetaUrl(), checkTooSmall(), clientReplyContext::cloneReply(), FwdState::complete(), contentLen(), MemStore::copyFromShm(), TestRock::createEntry(), Client::delayRead(), store_client::finishCallback(), clientReplyContext::handleIMSReply(), hasOneOfEtags(), isEmpty(), netdbExchangeHandleReply(), store_client::nextHttpReadOffset(), objectLen(), Store::PackFields(), peerDigestFetchReply(), HttpStateData::peerSupportsConnectionPinning(), clientReplyContext::processConditional(), store_client::readBody(), FwdState::reforward(), clientReplyContext::replyStatus(), reset(), store_client::sendingHttpHeaders(), clientReplyContext::sendNotModified(), clientReplyContext::storeOKTransferDone(), TestUfs::testUfsSearch(), timestampsSet(), store_client::tryParsingHttpHeaders(), Store::UnpackHitSwapMeta(), Store::UnpackNewSwapMetaVaryHeaders(), MemStore::updateHeadersOrThrow(), urnHandleReply(), and varyEvaluateMatch().
◆ mem() [2/2]
|
inline |
◆ memoryCachable()
bool StoreEntry::memoryCachable | ( | ) |
Definition at line 1276 of file store.cc.
References checkCachable(), Config, MemObject::data_hdr, MemObject::inmem_lo, mem_obj, SquidConfig::memory_cache_first, SquidConfig::onoff, refcount, shutting_down, mem_hdr::size(), and swappedOut().
Referenced by Store::Controller::keepForLocalMemoryCache(), and MemStore::shouldCache().
◆ memOutDecision()
void StoreEntry::memOutDecision | ( | const bool | willCacheInRam | ) |
Definition at line 1791 of file store.cc.
References assert, MemObject::MemCache::io, Store::ioDone, mem_obj, MemObject::memCache, and storeWritingCheckpoint().
Referenced by MemStore::startCaching(), and MemStore::write().
◆ modifiedSince()
bool StoreEntry::modifiedSince | ( | const time_t | ims, |
const int | imslen = -1 |
||
) | const |
Definition at line 1836 of file store.cc.
References assert, debugs, lastModified(), and url().
Referenced by clientIfRangeMatch(), clientReplyContext::handleIMSReply(), and clientReplyContext::processConditional().
◆ negativeCache()
void StoreEntry::negativeCache | ( | ) |
Set object for negative caching. Preserves any expiry information given by the server. In absence of proper expiry info it will set to expire immediately, or with HTTP-violations enabled the configured negative-TTL is observed
Definition at line 1321 of file store.cc.
References Config, debugs, EBIT_SET, ENTRY_NEGCACHED, expires, flags, SquidConfig::negativeTtl, and squid_curtime.
Referenced by abort(), cacheNegatively(), and storeErrorResponse().
◆ objectLen()
|
inline |
Definition at line 253 of file Store.h.
References mem(), and MemObject::object_sz.
Referenced by contentLen(), store_client::doCopy(), store_client::maybeWriteFromDiskToMemory(), store_client::moreToRead(), clientReplyContext::storeOKTransferDone(), storeSwapOutFileClosed(), validLength(), and Http::Stream::writeComplete().
◆ operator delete()
◆ operator new()
void * StoreEntry::operator new | ( | size_t | byteCount | ) |
Definition at line 149 of file store.cc.
References assert, and memPoolCreate.
◆ publicKey()
|
inline |
- Returns
- public key (if the entry has it) or nil (otherwise)
Definition at line 112 of file Store.h.
References EBIT_TEST, flags, hash_link::key, and KEY_PRIVATE.
Referenced by Store::Disks::evictCached(), Rock::SwapDir::evictCached(), MemStore::evictCached(), and Rock::SwapDir::openStoreIO().
◆ registerAbortCallback()
void StoreEntry::registerAbortCallback | ( | const AsyncCall::Pointer & | handler | ) |
Definition at line 1481 of file store.cc.
References MemObject::abortCallback, assert, and mem_obj.
Referenced by Ftp::Relay::Relay(), and FwdState::start().
◆ release()
void StoreEntry::release | ( | const bool | shareable = false | ) |
One of the three methods to get rid of an unlocked StoreEntry object. Removes all unlocked (and marks for eventual removal all locked) Store entries, including attached and unattached entries that have our key. Also destroys us if we are unlocked or makes us private otherwise.
Definition at line 1146 of file store.cc.
References debugs, destroyStoreEntry(), Store::Controller::evictCached(), getMD5Text(), hasDisk(), LateReleaseStack, lock(), locked(), releaseRequest(), Store::Root(), Store::Controller::store_dirs_rebuilding, STORE_LOG_RELEASE, and storeLog().
Referenced by doAbandon(), Store::Controller::handleIdleEntry(), clientReplyContext::handleIMSReply(), Ftp::Gateway::haveParsedReplyHeaders(), httpMaybeRemovePublic(), Fs::Ufs::UFSSwapDir::maintain(), clientReplyContext::purgeEntry(), Client::setFinalReply(), Store::Controller::syncCollapsed(), and TestRock::testRockSwapOut().
◆ releaseRequest()
void StoreEntry::releaseRequest | ( | const bool | shareable = false | ) |
Makes the StoreEntry private and marks the corresponding entry for eventual removal from the Store.
Definition at line 458 of file store.cc.
References debugs, EBIT_TEST, flags, RELEASE_REQUEST, setPrivateKey(), and shareableWhenPrivate.
Referenced by abort(), checkCachable(), htcpClrStoreEntry(), HttpStateData::HttpStateData(), lengthWentBad(), makePrivate(), Store::EntryGuard::onException(), peerDigestFetchReply(), clientReplyContext::processMiss(), Ftp::Relay::Relay(), release(), storeErrorResponse(), storeSwapOutFileClosed(), clientReplyContext::traceReply(), and DigestFetchState::~DigestFetchState().
◆ replaceHttpReply()
void StoreEntry::replaceHttpReply | ( | const HttpReplyPointer & | rep, |
const bool | andStartWriting = true |
||
) |
Definition at line 1705 of file store.cc.
References DBG_CRITICAL, debugs, mem_obj, MemObject::replaceBaseReply(), startWriting(), and url().
Referenced by adjustVary(), Mgr::Action::fillEntry(), ftpFail(), ftpSendReply(), ClientHttpRequest::handleAdaptedHeader(), internalStart(), MimeIcon::load(), netdbBinaryExchange(), clientReplyContext::processMiss(), clientReplyContext::purgeDoPurge(), clientReplyContext::sendNotModified(), Client::setFinalReply(), Ftp::Server::setReply(), WhoisState::setReplyToOK(), CacheManager::start(), storeDigestRewriteResume(), storeErrorResponse(), clientReplyContext::traceReply(), and urnHandleReply().
◆ reset()
void StoreEntry::reset | ( | ) |
Definition at line 1621 of file store.cc.
References debugs, expires, lastModified_, mem(), MemObject::reset(), timestamp, and url().
Referenced by FwdState::complete(), and HttpStateData::continueAfterParsingHeader().
◆ setCollapsingRequirement()
void StoreEntry::setCollapsingRequirement | ( | const bool | required | ) |
Definition at line 2012 of file store.cc.
References debugs, EBIT_CLR, EBIT_SET, ENTRY_REQUIRES_COLLAPSING, flags, and hittingRequiresCollapsing().
Referenced by Store::Controller::allowCollapsing(), Store::Controller::anchorToCache(), startWriting(), and Store::Controller::syncCollapsed().
◆ setMemStatus()
void StoreEntry::setMemStatus | ( | mem_status_t | new_status | ) |
Definition at line 1524 of file store.cc.
References RemovalPolicy::Add, assert, debugs, EBIT_TEST, MemStore::Enabled(), ENTRY_SPECIAL, flags, getMD5Text(), hot_obj_count, IN_MEMORY, MemObject::inmem_lo, mem_obj, mem_policy, mem_status, RemovalPolicy::Remove, and MemObject::repl.
Referenced by abort(), Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), MemStore::anchorEntry(), MemStore::copyFromShm(), destroyMemObject(), and Store::Controller::handleIdleEntry().
◆ setNoDelay()
void StoreEntry::setNoDelay | ( | bool const | newValue | ) |
Definition at line 250 of file store.cc.
References mem_obj, and MemObject::setNoDelay().
Referenced by HttpStateData::HttpStateData().
◆ setPrivateKey()
void StoreEntry::setPrivateKey | ( | const bool | shareable, |
const bool | permanent | ||
) |
Either fills this entry with private key or changes the existing key from public to private.
- Parameters
-
permanent whether this entry should be private forever.
Definition at line 548 of file store.cc.
References assert, debugs, EBIT_SET, EBIT_TEST, Store::Controller::evictCached(), flags, getKeyCounter(), hash_lookup(), hashDelete(), hashInsert(), MemObject::hasUris(), MemObject::id, hash_link::key, KEY_PRIVATE, mem_obj, RELEASE_REQUEST, Store::Root(), shareableWhenPrivate, store_table, and storeKeyPrivate().
Referenced by releaseRequest(), and storeCreateEntry().
◆ setPublicKey()
Definition at line 575 of file store.cc.
References Store::Controller::addWriting(), adjustVary(), assert, calcPublicKey(), debugs, EBIT_TEST, flags, forcePublicKey(), hash_link::key, KEY_PRIVATE, mem_obj, RELEASE_REQUEST, Store::Root(), and Store::EntryGuard::unlockAndReset().
Referenced by TestRock::createEntry(), MimeIcon::load(), makePublic(), storeCreateEntry(), storeDigestRewriteResume(), and TestUfs::testUfsSearch().
◆ startWriting()
void StoreEntry::startWriting | ( | ) |
pack and write reply headers and, maybe, body
Definition at line 1721 of file store.cc.
References assert, MemObject::baseReply(), buffer(), flush(), isEmpty(), MemObject::markEndOfReplyHeaders(), mem_obj, setCollapsingRequirement(), and MemObject::updatedReply().
Referenced by adjustVary(), replaceHttpReply(), and Client::setFinalReply().
◆ storeClientType()
store_client_t StoreEntry::storeClientType | ( | ) | const |
Definition at line 264 of file store.cc.
References assert, DBG_IMPORTANT, debugs, EBIT_TEST, MemObject::endOffset(), ENTRY_ABORTED, flags, MemObject::inmem_lo, isEmpty(), mem_obj, MemObject::nclients, MemObject::object_sz, STORE_DISK_CLIENT, STORE_MEM_CLIENT, STORE_OK, store_status, swap_status, SWAPOUT_NONE, swapoutFailed(), and swappedOut().
◆ storeErrorResponse()
void StoreEntry::storeErrorResponse | ( | HttpReply * | reply | ) |
Definition at line 1688 of file store.cc.
References buffer(), completeSuccessfully(), flush(), lock(), negativeCache(), releaseRequest(), replaceHttpReply(), and unlock().
Referenced by errorAppendEntry(), and clientReplyContext::setReplyToReply().
◆ storeWriterDone()
void StoreEntry::storeWriterDone | ( | ) |
Definition at line 1808 of file store.cc.
References storeWritingCheckpoint().
Referenced by MemStore::completeWriting(), MemStore::disconnect(), Rock::SwapDir::disconnect(), and storeSwapOutFileClosed().
◆ storeWritingCheckpoint()
|
protected |
If needed, signal transient entry readers that no more cache changes are expected and, hence, they should switch to Plan B instead of getting stuck waiting for us to start or finish storing the entry.
Definition at line 1761 of file store.cc.
References assert, debugs, MemObject::SwapOut::decision, hasTransients(), MemObject::MemCache::io, Store::ioDone, mem_obj, MemObject::memCache, Store::Controller::noteStoppedSharedWriting(), Store::Root(), MemObject::swapout, swappingOut(), MemObject::SwapOut::swImpossible, and MemObject::SwapOut::swStarted.
Referenced by memOutDecision(), storeWriterDone(), and swapOutDecision().
◆ swapOut()
void StoreEntry::swapOut | ( | ) |
Definition at line 153 of file store_swapout.cc.
References abort(), assert, MemObject::availableForSwapOut(), checkDeferRead(), DBG_CRITICAL, debugs, MemObject::SwapOut::decision, doPages(), EBIT_TEST, MemObject::endOffset(), ENTRY_ABORTED, flags, hasDisk(), MemObject::inmem_lo, MemObject::lowestMemReaderOffset(), mayStartSwapOut(), mem_obj, Store::Controller::memoryOut(), MemObject::object_sz, MemObject::objectBytesOnDisk(), StoreIOState::offset(), MemObject::SwapOut::queue_offset, RELEASE_REQUEST, Store::Root(), MemObject::SwapOut::sio, SM_PAGE_SIZE, STORE_OK, STORE_PENDING, store_status, storeSwapOutStart(), storeTooManyDiskFilesOpen(), MemObject::swapout, swapOutFileClose(), swappingOut(), MemObject::SwapOut::swPossible, url(), and StoreIOState::wroteAll.
Referenced by TestRock::addEntry(), invokeHandlers(), storeUnregister(), and TestUfs::testUfsSearch().
◆ swapOutDecision()
void StoreEntry::swapOutDecision | ( | const MemObject::SwapOut::Decision & | decision | ) |
Definition at line 1800 of file store.cc.
References assert, MemObject::SwapOut::decision, mem_obj, storeWritingCheckpoint(), and MemObject::swapout.
Referenced by mayStartSwapOut(), and storeSwapOutStart().
◆ swapoutFailed()
|
inline |
Definition at line 137 of file Store.h.
References swap_status, and SWAPOUT_FAILED.
Referenced by checkDisk(), store_client::store_client(), storeClientType(), storeSwapInStart(), and storeUnregister().
◆ swapOutFileClose()
void StoreEntry::swapOutFileClose | ( | int | how | ) |
Definition at line 257 of file store_swapout.cc.
References assert, debugs, getMD5Text(), RefCount< C >::getRaw(), mem_obj, MemObject::SwapOut::sio, storeClose(), and MemObject::swapout.
◆ swappedOut()
|
inline |
Definition at line 135 of file Store.h.
References swap_status, and SWAPOUT_DONE.
Referenced by Store::Disk::canLog(), checkDisk(), Fs::Ufs::UFSSwapDir::evictCached(), Store::Controller::handleIdleEntry(), memoryCachable(), storeClientType(), and storeUnregister().
◆ swappingOut()
|
inline |
Definition at line 133 of file Store.h.
References swap_status, and SWAPOUT_WRITING.
Referenced by checkDisk(), doPages(), store_client::fileRead(), mayStartSwapOut(), storeSwapOutFileClosed(), storeWritingCheckpoint(), swapOut(), and validToSend().
◆ timestampsSet()
bool StoreEntry::timestampsSet | ( | ) |
Definition at line 1387 of file store.cc.
References Http::AGE, HttpReply::date, debugs, describeTimestamps(), expires, MemObject::freshestReply(), HttpRequest::hier, lastModified(), lastModified_, mem(), mem_obj, HierarchyLogEntry::peerResponseTime(), MemObject::request, squid_curtime, and timestamp.
Referenced by TestRock::addEntry(), adjustVary(), ftpWriteTransferDone(), ClientHttpRequest::handleAdaptedHeader(), HttpStateData::haveParsedReplyHeaders(), Ftp::Gateway::haveParsedReplyHeaders(), MimeIcon::load(), HttpStateData::processSurrogateControl(), WhoisState::readReply(), clientReplyContext::sendNotModified(), storeDigestRewriteFinish(), TestUfs::testUfsSearch(), and updateOnNotModified().
◆ touch()
void StoreEntry::touch | ( | ) |
Definition at line 452 of file store.cc.
References lastref, and squid_curtime.
◆ trimMemory()
void StoreEntry::trimMemory | ( | const bool | preserveSwappable | ) |
Definition at line 1814 of file store.cc.
References debugs, EBIT_TEST, ENTRY_SPECIAL, flags, IN_MEMORY, MemObject::inmem_lo, mem_obj, mem_status, MemObject::trimSwappable(), and MemObject::trimUnSwappable().
Referenced by Store::Controller::memoryOut().
◆ unlock()
int StoreEntry::unlock | ( | const char * | context | ) |
disclaim shared ownership; may remove entry from store and delete it returns remaining lock level (zero for unlocked and possibly gone entry)
Definition at line 469 of file store.cc.
References abandon(), assert, debugs, getMD5Text(), and lock_count.
Referenced by abort(), adjustVary(), store_client::copy(), ClientHttpRequest::doCallouts(), clientReplyContext::forgetHit(), heap_purgeDone(), ClientHttpRequest::loggingEntry(), Store::EntryGuard::onException(), peerCountMcastPeersAbort(), peerDigestFetchReply(), clientReplyContext::removeStoreReference(), statObjects(), storeDigestRewriteResume(), storeErrorResponse(), storeLateRelease(), storeSwapOutFileClosed(), storeUnregister(), TestRock::testRockSwapOut(), TestUfs::testUfsSearch(), Store::EntryGuard::unlockAndReset(), whoisClose(), Client::~Client(), DigestFetchState::~DigestFetchState(), FwdState::~FwdState(), netdbExchangeState::~netdbExchangeState(), PeerSelector::~PeerSelector(), store_client::~store_client(), and UrnState::~UrnState().
◆ unregisterAbortCallback()
void StoreEntry::unregisterAbortCallback | ( | const char * | reason | ) |
Avoid notifying anybody about a 3rd-party initiated StoreEntry abort. Calling this method does not cancel the already queued notification. TODO: Refactor to represent the end of (shared) ownership by our writer.
Definition at line 1489 of file store.cc.
References MemObject::abortCallback, assert, AsyncCall::cancel(), and mem_obj.
Referenced by FwdState::~FwdState().
◆ updateOnNotModified()
bool StoreEntry::updateOnNotModified | ( | const StoreEntry & | e304 | ) |
Updates easily-accessible non-Store-specific parts of the entry. Use Controller::updateOnNotModified() instead of this helper.
- Returns
- whether anything was actually updated
Definition at line 1452 of file store.cc.
References MemObject::appliedUpdates, assert, MemObject::baseReply(), Config, debugs, MemObject::freshestReply(), Here, SquidConfig::maxReplyHeaderSize, mem_obj, HttpReply::recreateOnNotModified(), timestampsSet(), ToSBuf(), and MemObject::updateReply().
Referenced by Store::Controller::updateOnNotModified().
◆ url()
const char * StoreEntry::url | ( | ) | const |
Definition at line 1566 of file store.cc.
References mem_obj, and MemObject::storeId().
Referenced by FwdState::complete(), FwdState::connectStart(), HttpStateData::continueAfterParsingHeader(), FwdState::dispatch(), ftpSendReply(), FwdState::FwdState(), Ftp::Gateway::Gateway(), clientReplyContext::handleIMSReply(), HttpStateData::handleMoreRequestBodyAvailable(), FwdState::handleUnregisteredServerEnd(), htcpClrStoreEntry(), htcpQuery(), HttpStateData::httpBuildRequestHeader(), httpStart(), HttpStateData::httpTimeout(), HttpStateData::keepaliveAccounting(), mayStartSwapOut(), modifiedSince(), neighborsUdpPing(), Store::PackFields(), peerSelect(), peerSelectIcpPing(), clientReplyContext::purgeEntry(), WhoisState::readReply(), FwdState::reforward(), replaceHttpReply(), reset(), clientReplyContext::sendMoreData(), UrnState::start(), CacheManager::start(), FwdState::Start(), HttpStateData::statusIfComplete(), storeDigestDel(), storeSwapOutFileClosed(), storeSwapOutStart(), swapOut(), FwdState::unregister(), PeerSelector::url(), urnHandleReply(), FwdState::useDestinations(), whoisTimeout(), netdbExchangeState::~netdbExchangeState(), and PeerSelector::~PeerSelector().
◆ validLength()
|
private |
whether the base response has all the body bytes we expect
- Returns
- true for responses with unknown/unspecified body length
- true for responses with the right number of accumulated body bytes
Definition at line 1204 of file store.cc.
References assert, MemObject::baseReply(), debugs, getMD5Text(), mem_obj, MemObject::method, Http::METHOD_HEAD, objectLen(), Http::scNoContent, and Http::scNotModified.
Referenced by complete().
◆ validToSend()
int StoreEntry::validToSend | ( | ) | const |
Definition at line 1347 of file store.cc.
References EBIT_TEST, ENTRY_ABORTED, ENTRY_NEGCACHED, expires, flags, hasDisk(), MemObject::inmem_lo, mem_obj, RELEASE_REQUEST, squid_curtime, and swappingOut().
Referenced by ICPState::confirmAndPrepHit().
◆ vappendf()
|
overridevirtual |
Append operation, with vsprintf(3)-style arguments.
- Note
- arguments may be evaluated more than once, be careful of side-effects
XXX: This method either should not exist or should not be virtual. Kids should not be forced to re-implement vappendf() logic. That logic should be implemented once, using other [Packable] APIs. Packable is not about providing a printf(3) service. Packable is about writing opaque data to various custom destinations.
Implements Packable.
Definition at line 821 of file store.cc.
References append(), assert, fatal(), LOCAL_ARRAY, and xstrerr().
Referenced by storeAppendPrintf(), and storeAppendVPrintf().
◆ write()
void StoreEntry::write | ( | StoreIOBuffer | writeBuffer | ) |
Definition at line 780 of file store.cc.
References assert, MemObject::baseReply(), debugs, EBIT_CLR, EBIT_TEST, ENTRY_FWD_HDR_WAIT, flags, getMD5Text(), Http::Message::hdr_sz, invokeHandlers(), StoreIOBuffer::length, mem_obj, StoreIOBuffer::offset, MemObject::readAheadPolicyCanRead(), STORE_PENDING, store_status, storeGetMemSpace(), and MemObject::write().
Referenced by append(), Client::handleMoreAdaptedBodyAvailable(), ClientHttpRequest::noteMoreBodyDataAvailable(), storeDirWriteCleanLogs(), and Client::storeReplyBody().
Friends And Related Function Documentation
◆ operator<<
|
friend |
Member Data Documentation
◆ deferredProducer
|
private |
Definition at line 331 of file Store.h.
Referenced by deferProducer(), and kickProducer().
◆ expires
time_t StoreEntry::expires |
Definition at line 225 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), clientReplyContext::buildReplyHeader(), checkNegativeHit(), describeTimestamps(), dump(), expireNow(), Ipc::StoreMapAnchor::exportInto(), htcpTstReply(), Fs::Ufs::UFSSwapDir::logEntry(), negativeCache(), peerDigestFetchSetStats(), peerDigestNewDelay(), Fs::Ufs::RebuildState::rebuildFromDirectory(), refreshStaleness(), reset(), Ipc::StoreMapAnchor::set(), CacheManager::start(), storeDigestRewriteFinish(), timestampsSet(), Store::UnpackIndexSwapMeta(), validToSend(), and UFSCleanLog::write().
◆ flags
uint16_t StoreEntry::flags |
Definition at line 231 of file Store.h.
Referenced by abort(), Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), Store::Controller::addWriting(), MemStore::anchorEntry(), Rock::SwapDir::anchorEntry(), buffer(), clientReplyContext::buildReplyHeader(), clientReplyContext::cacheHit(), Store::Disk::canLog(), Store::Disk::canStore(), checkCachable(), checkDisk(), checkNegativeHit(), CheckQuickAbortIsReasonable(), Store::CheckSwapMetaKey(), checkTooSmall(), Store::Controller::checkTransients(), clearPrivate(), clearPublicKeyScope(), complete(), FwdState::completed(), store_client::copy(), MemStore::copyToShm(), Store::Controller::dereferenceIdle(), FwdState::dispatch(), doAbandon(), dummy_action(), errorAppendEntry(), clientReplyContext::errorInStream(), Store::Disks::evictCached(), Ipc::StoreMapAnchor::exportInto(), flush(), Store::Controller::handleIdleEntry(), clientReplyContext::handleIMSReply(), Http::One::Server::handleReply(), HttpStateData::haveParsedReplyHeaders(), heap_add(), hittingRequiresCollapsing(), httpMaybeRemovePublic(), invokeHandlers(), isAccepting(), lengthWentBad(), MimeIcon::load(), Fs::Ufs::UFSSwapDir::logEntry(), makePublic(), FwdState::markStoredReplyAsWhole(), mayStartHitting(), mayStartSwapOut(), negativeCache(), neighborsHtcpReply(), neighborsUdpAck(), netdbExchangeHandleReply(), operator<<(), peerDigestFetchedEnough(), peerDigestRequest(), peerSelectIcpPing(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), HttpStateData::processReply(), HttpStateData::processReplyBody(), publicKey(), HttpStateData::readReply(), Fs::Ufs::RebuildState::rebuildFromDirectory(), Store::Controller::referenceBusy(), FwdState::reforward(), refreshCheck(), releaseRequest(), clientReplyContext::replyStatus(), HttpStateData::reusableReply(), clientReplyContext::sendClientOldEntry(), clientReplyContext::sendClientUpstreamResponse(), Client::sentRequestBody(), Ipc::StoreMapAnchor::set(), setCollapsingRequirement(), Client::setFinalReply(), setMemStatus(), setPrivateKey(), setPublicKey(), MemStore::shouldCache(), statObjects(), storeCleanup(), storeClientType(), storeCreatePureEntry(), storeDigestAddable(), storeDigestDel(), storeDigestRewriteResume(), storeDirSwapLog(), storeEntryFlags(), storeRebuildParseEntry(), storeSwapInStart(), swapOut(), Store::Controller::syncCollapsed(), TestCacheManager::testRegister(), trimMemory(), Store::UnpackIndexSwapMeta(), Store::Controller::updateOnNotModified(), urnHandleReply(), validToSend(), UFSCleanLog::write(), and write().
◆ key
|
inherited |
Definition at line 19 of file hash.h.
Referenced by ACLExternal::aclMatchExternal(), MemStore::anchorToCache(), Rock::SwapDir::anchorToCache(), Store::CheckSwapMetaKey(), clearPublicKeyScope(), ClientInfo::ClientInfo(), Rock::SwapDir::createStoreIO(), CommQuotaQueue::dequeue(), destroyStoreEntry(), CommQuotaQueue::enqueue(), external_acl_cache_add(), forcePublicKey(), fqdncache_entry::fqdncache_entry(), fqdncacheAddEntry(), fqdncacheParse(), getMD5Text(), hash_join(), hash_remove_link(), hashDelete(), hashInsert(), hashKeyStr(), Rock::SwapDir::hasReadableEntry(), htcpQuery(), IdleConnList::IdleConnList(), idnsStartQuery(), ipcache_entry::ipcache_entry(), ipcacheAddEntry(), ipcacheRelease(), Fs::Ufs::UFSSwapDir::logEntry(), Store::Controller::markedForDeletionAndAbandoned(), my_free(), ipcache_entry::name(), neighborsUdpPing(), net_db_name::net_db_name(), netdbHashInsert(), Ipc::StoreMap::openForUpdating(), Store::PackFields(), peerCountMcastPeersCreateAndSend(), publicKey(), read_passwd_file(), Ipc::StoreMapAnchor::set(), setPrivateKey(), setPublicKey(), ClientInfo::setWriteLimiter(), MemStore::startCaching(), storeDigestAdd(), storeDigestDel(), storeKeyHashHash(), storeKeyText(), storeRebuildParseEntry(), Store::UnpackIndexSwapMeta(), WIN32_StoreKey(), wordlistAdd(), UFSCleanLog::write(), ClientInfo::~ClientInfo(), ExternalACLEntry::~ExternalACLEntry(), fqdncache_entry::~fqdncache_entry(), IdleConnList::~IdleConnList(), and net_db_name::~net_db_name().
◆ lastModified_
|
private |
Definition at line 227 of file Store.h.
Referenced by describeTimestamps(), dump(), lastModified(), reset(), and timestampsSet().
◆ lastref
time_t StoreEntry::lastref |
Definition at line 224 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), describeTimestamps(), dump(), Ipc::StoreMapAnchor::exportInto(), HeapKeyGen_StoreEntry_GDSF(), HeapKeyGen_StoreEntry_LFUDA(), HeapKeyGen_StoreEntry_LRU(), Fs::Ufs::UFSSwapDir::logEntry(), lru_stats(), Fs::Ufs::RebuildState::rebuildFromDirectory(), Ipc::StoreMapAnchor::set(), storeCreatePureEntry(), touch(), Store::UnpackIndexSwapMeta(), and UFSCleanLog::write().
◆ lock_count
|
private |
◆ mem_obj
MemObject* StoreEntry::mem_obj |
Definition at line 220 of file Store.h.
Referenced by abort(), Store::Disks::accumulateMore(), Transients::addEntry(), Transients::addReaderEntry(), Transients::addWriterEntry(), adjustVary(), MemStore::anchorEntry(), append(), CollapsedForwarding::Broadcast(), bytesWanted(), clientReplyContext::cacheHit(), calcPublicKey(), checkCachable(), CheckQuickAbortIsReasonable(), checkTooBig(), checkTooSmall(), complete(), FwdState::complete(), FwdState::completed(), MemStore::completeWriting(), Transients::completeWriting(), store_client::copy(), MemStore::copyFromShm(), MemStore::copyFromShmSlice(), MemStore::copyToShm(), MemStore::copyToShmSlice(), createMemObject(), Store::Controller::dereferenceIdle(), destroyMemObject(), MemStore::disconnect(), Rock::SwapDir::disconnect(), Transients::disconnect(), store_client::doCopy(), doPages(), dump(), ensureMemObject(), errorAppendEntry(), FwdState::establishTunnelThruProxy(), MemStore::evictCached(), Transients::evictCached(), store_client::fileRead(), Rock::SwapDir::finalizeSwapoutSuccess(), findPreviouslyCachedEntry(), forcePublicKey(), Transients::get(), store_client::handleBodyFromDisk(), Store::Controller::handleIdleEntry(), hasFreshestReply(), hasMemStore(), hasParsedReplyHeader(), hasTransients(), Transients::hasWriter(), HttpStateData::haveParsedReplyHeaders(), heap_guessType(), HeapKeyGen_StoreEntry_GDSF(), HeapKeyGen_StoreEntry_LFUDA(), HeapKeyGen_StoreEntry_LRU(), httpMaybeRemovePublic(), invokeHandlers(), Transients::isReader(), Transients::isWriter(), Store::Controller::keepForLocalMemoryCache(), MimeIcon::load(), store_client::maybeWriteFromDiskToMemory(), mayStartSwapOut(), mem(), memoryCachable(), memOutDecision(), Transients::monitorIo(), neighborsHtcpReply(), neighborsUdpAck(), neighborsUdpPing(), objectSizeForDirSelection(), operator<<(), peerCountHandleIcpReply(), peerCountMcastPeersAbort(), peerCountMcastPeersCreateAndSend(), peerDigestFetchReply(), peerDigestFetchSetStats(), peerDigestSwapInCBlock(), Transients::readers(), store_client::readFromMemory(), store_client::readHeader(), HttpStateData::readReply(), Store::Controller::referenceBusy(), FwdState::reforward(), refreshCheck(), refreshCheckDigest(), refreshIsCachable(), registerAbortCallback(), repl_guessType(), replaceHttpReply(), setMemStatus(), setNoDelay(), LruPolicyData::setPolicyNode(), HeapPolicyData::setPolicyNode(), setPrivateKey(), setPublicKey(), MemStore::shouldCache(), store_client::skipHttpHeadersFromDisk(), FwdState::Start(), MemStore::startCaching(), startWriting(), statObjectsOpenfdFilter(), statObjectsVmFilter(), statStoreEntry(), Transients::status(), storeClientListAdd(), storeClientType(), storeDigestRewriteFinish(), storeDigestRewriteStart(), storeLog(), clientReplyContext::storeNotOKTransferDone(), storePendingNClients(), storeSwapInStart(), storeSwapOutFileClosed(), storeSwapOutStart(), storeUnregister(), storeWritingCheckpoint(), swapOut(), swapOutDecision(), swapOutFileClose(), Store::Controller::syncCollapsed(), TestRock::testRockSwapOut(), timestampsSet(), trimMemory(), unregisterAbortCallback(), MemStore::updateAnchored(), MemStore::updateHeaders(), Store::Controller::updateOnNotModified(), updateOnNotModified(), url(), validLength(), validToSend(), varyEvaluateMatch(), MemStore::write(), write(), and HttpStateData::wroteLast().
◆ mem_status
mem_status_t StoreEntry::mem_status |
Definition at line 239 of file Store.h.
Referenced by clientReplyContext::cacheHit(), complete(), Store::Controller::dereferenceIdle(), describeStatuses(), dump(), operator<<(), Store::Controller::referenceBusy(), setMemStatus(), MemStore::shouldCache(), storeSwapInStart(), trimMemory(), and Store::Controller::updateOnNotModified().
◆ next
|
inherited |
Definition at line 20 of file hash.h.
Referenced by clientdbGC(), Store::LocalSearch::copyBucket(), dump(), hash_join(), hash_lookup(), hash_next(), hash_remove_link(), IdleConnList::IdleConnList(), and lru_stats().
◆ ping_status
ping_status_t StoreEntry::ping_status |
Definition at line 241 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), Rock::SwapDir::anchorEntry(), describeStatuses(), FwdState::dispatch(), dump(), PeerSelector::handlePingTimeout(), neighborsHtcpReply(), neighborsUdpAck(), operator<<(), peerCountMcastPeersCreateAndSend(), peerSelectIcpPing(), PeerSelector::selectMore(), PeerSelector::selectPinned(), PeerSelector::selectSomeNeighbor(), PeerSelector::selectSomeNeighborReplies(), PeerSelector::startPingWaiting(), storeCreatePureEntry(), and PeerSelector::~PeerSelector().
◆ pool
|
staticprivate |
Definition at line 318 of file Store.h.
Referenced by inUseCount().
◆ refcount
uint16_t StoreEntry::refcount |
Definition at line 230 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), dump(), Ipc::StoreMapAnchor::exportInto(), HeapKeyGen_StoreEntry_GDSF(), HeapKeyGen_StoreEntry_LFUDA(), Fs::Ufs::UFSSwapDir::logEntry(), memoryCachable(), Fs::Ufs::RebuildState::rebuildFromDirectory(), Ipc::StoreMapAnchor::set(), statStoreEntry(), store_client::store_client(), storeCreatePureEntry(), Store::UnpackIndexSwapMeta(), and UFSCleanLog::write().
◆ repl
RemovalPolicyNode StoreEntry::repl |
Definition at line 221 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::dereference(), Rock::SwapDir::dereference(), heap_guessType(), Rock::SwapDir::ignoreReferences(), Fs::Ufs::UFSSwapDir::reference(), Rock::SwapDir::reference(), repl_guessType(), Fs::Ufs::UFSSwapDir::replacementAdd(), Fs::Ufs::UFSSwapDir::replacementRemove(), LruPolicyData::setPolicyNode(), HeapPolicyData::setPolicyNode(), and Rock::SwapDir::trackReferences().
◆ shareableWhenPrivate
|
private |
Nobody can find/lock KEY_PRIVATE entries, but some transactions (e.g., collapsed requests) find/lock a public entry before it becomes private. May such transactions start using the now-private entry they previously locked? This member should not affect transactions that already started reading from the entry.
Definition at line 327 of file Store.h.
Referenced by clearPrivate(), mayStartHitting(), operator<<(), releaseRequest(), and setPrivateKey().
◆ store_status
store_status_t StoreEntry::store_status |
Definition at line 243 of file Store.h.
Referenced by abort(), Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), MemStore::anchorEntry(), Rock::SwapDir::anchorEntry(), append(), clientReplyContext::cacheHit(), checkNegativeHit(), CheckQuickAbortIsReasonable(), FwdState::checkRetry(), checkTooSmall(), clientReplyContext::checkTransferDone(), complete(), FwdState::completed(), MemStore::copyFromShm(), describeStatuses(), doAbandon(), store_client::doCopy(), doPages(), dump(), errorAppendEntry(), HttpStateData::httpTimeout(), isAccepting(), mayStartSwapOut(), store_client::moreToRead(), operator<<(), FwdState::reforward(), storeClientType(), storeCreatePureEntry(), storeUnregister(), swapOut(), TestStoreController::testMaxSize(), TestStoreHashIndex::testMaxSize(), TestStoreHashIndex::testStats(), TestStoreController::testStats(), MemStore::write(), and write().
◆ swap_dirn
sdirno StoreEntry::swap_dirn |
Definition at line 237 of file Store.h.
Referenced by addedEntry(), attachToDisk(), checkDisk(), Fs::Ufs::UFSSwapDir::dereference(), Rock::SwapDir::dereference(), detachFromDisk(), disk(), dump(), hasDisk(), operator<<(), Fs::Ufs::UFSSwapDir::reference(), Rock::SwapDir::reference(), Fs::Ufs::UFSSwapDir::replacementRemove(), statStoreEntry(), storeDirSwapLog(), storeLog(), storeSwapOutFileClosed(), storeSwapOutStart(), TestRock::testRockSwapOut(), TestUfs::testUfsSearch(), and Store::Controller::updateOnNotModified().
◆ swap_file_sz
uint64_t StoreEntry::swap_file_sz |
Definition at line 229 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), MemStore::anchorEntry(), Store::Disk::canLog(), Fs::Ufs::UFSSwapDir::doubleCheck(), dump(), Fs::Ufs::UFSSwapDir::evictCached(), Ipc::StoreMapAnchor::exportInto(), Fs::Ufs::UFSSwapDir::finalizeSwapoutSuccess(), HeapKeyGen_StoreEntry_GDSF(), Rock::Rebuild::importEntry(), Fs::Ufs::UFSSwapDir::logEntry(), Rock::SwapDir::openStoreIO(), Fs::Ufs::RebuildState::rebuildFromDirectory(), Ipc::StoreMapAnchor::set(), storeDigestAddable(), storeRebuildParseEntry(), storeSwapOutFileClosed(), Store::UnpackHitSwapMeta(), Store::UnpackIndexSwapMeta(), Rock::SwapDir::updateAnchored(), MemStore::updateAnchoredWith(), and UFSCleanLog::write().
◆ swap_filen
sfileno StoreEntry::swap_filen |
Definition at line 235 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), attachToDisk(), checkDisk(), Fs::Ufs::UFSSwapDir::dereference(), Rock::SwapDir::dereference(), detachFromDisk(), Rock::SwapDir::disconnect(), Fs::Ufs::UFSSwapDir::doubleCheck(), dump(), Fs::Ufs::UFSSwapDir::dumpEntry(), Rock::SwapDir::evictCached(), Fs::Ufs::UFSSwapDir::evictCached(), hasDisk(), Fs::Ufs::UFSSwapDir::logEntry(), Fs::Ufs::UFSStrategy::open(), Rock::SwapDir::openStoreIO(), operator<<(), Fs::Ufs::UFSSwapDir::reference(), Rock::SwapDir::reference(), statStoreEntry(), storeDirSwapLog(), storeLog(), storeSwapOutFileClosed(), storeSwapOutStart(), TestRock::testRockSwapOut(), TestUfs::testUfsSearch(), StoreIOState::touchingStoreEntry(), Fs::Ufs::UFSStoreState::UFSStoreState(), Rock::SwapDir::updateAnchored(), Rock::SwapDir::updateHeaders(), and UFSCleanLog::write().
◆ swap_status
swap_status_t StoreEntry::swap_status |
Definition at line 245 of file Store.h.
Referenced by addedEntry(), attachToDisk(), checkDisk(), describeStatuses(), detachFromDisk(), dump(), operator<<(), storeClientType(), storeSwapOutFileClosed(), storeSwapOutStart(), swapoutFailed(), swappedOut(), swappingOut(), and TestRock::testRockSwapOut().
◆ timestamp
time_t StoreEntry::timestamp |
Definition at line 223 of file Store.h.
Referenced by Fs::Ufs::UFSSwapDir::addDiskRestore(), addedEntry(), clientReplyContext::buildReplyHeader(), describeTimestamps(), dump(), Ipc::StoreMapAnchor::exportInto(), htcpTstReply(), lastModified(), Fs::Ufs::UFSSwapDir::logEntry(), Store::PackFields(), Fs::Ufs::RebuildState::rebuildFromDirectory(), refreshCheck(), refreshStaleness(), reset(), clientReplyContext::sendNotModified(), Ipc::StoreMapAnchor::set(), storeCreatePureEntry(), timestampsSet(), Store::UnpackIndexSwapMeta(), and UFSCleanLog::write().
The documentation for this class was generated from the following files:
- src/Store.h
- src/store.cc
- src/store_client.cc
- src/store_swapout.cc
- src/tests/stub_store.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products