#include <AsyncJob.h>
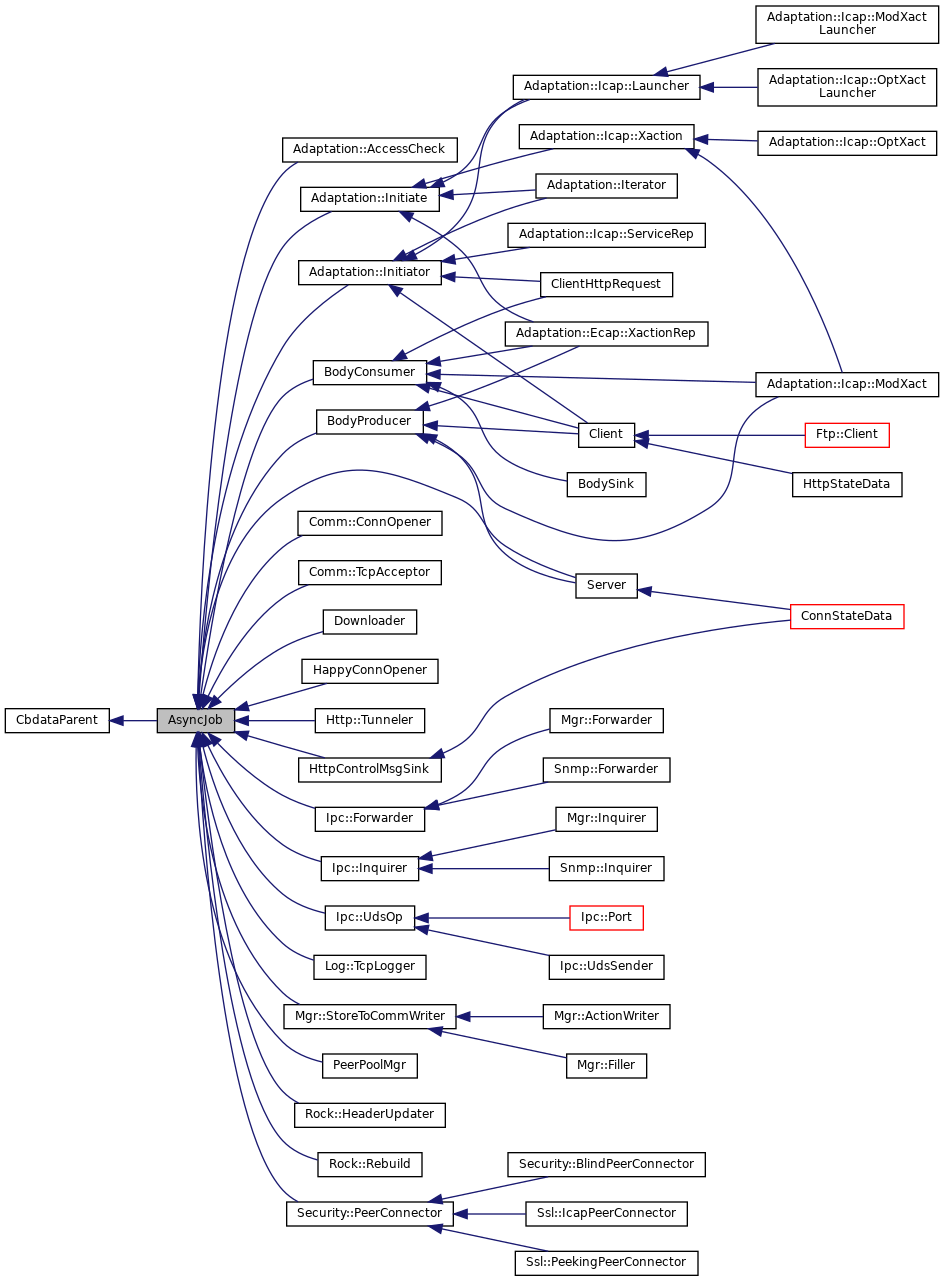
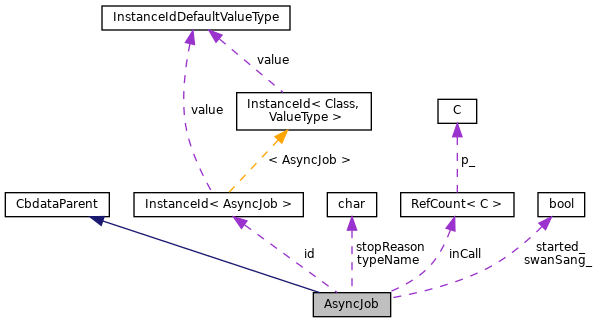
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
AsyncJob (const char *aTypeName) | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual void | start () |
called by AsyncStart; do not call directly More... | |
virtual bool | doneAll () const |
whether positive goal has been reached More... | |
virtual void | swanSong () |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
~AsyncJob () override | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Detailed Description
Base class for all asynchronous jobs
Definition at line 31 of file AsyncJob.h.
Member Typedef Documentation
◆ Pointer
typedef CbcPointer<AsyncJob> AsyncJob::Pointer |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ AsyncJob()
AsyncJob::AsyncJob | ( | const char * | aTypeName | ) |
Definition at line 43 of file AsyncJob.cc.
◆ ~AsyncJob()
|
overrideprotected |
Member Function Documentation
◆ callEnd()
|
virtual |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, done(), inCall, started_, status(), swanSang_, swanSong(), and typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and deleteThis().
◆ callException()
|
virtual |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
void AsyncJob::callStart | ( | AsyncCall & | call | ) |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, inCall, Must, status(), CbdataParent::toCbdata(), and typeName.
◆ canBeCalled()
bool AsyncJob::canBeCalled | ( | AsyncCall & | call | ) | const |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and inCall.
◆ deleteThis()
|
protected |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), callEnd(), debugs, inCall, JobMemFun(), Must, stopReason, and typeName.
Referenced by ConnStateData::connStateClosed().
◆ done()
|
protected |
Definition at line 106 of file AsyncJob.cc.
References doneAll(), and stopReason.
Referenced by callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact, ConnStateData, ClientHttpRequest, HappyConnOpener, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Ipc::UdsSender, Client, Rock::Rebuild, Adaptation::AccessCheck, Adaptation::Icap::Launcher, Security::PeerConnector, Downloader, Log::TcpLogger, Http::Tunneler, Adaptation::Iterator, Ipc::Forwarder, Comm::TcpAcceptor, Ipc::Inquirer, Rock::HeaderUpdater, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Mgr::Inquirer, Snmp::Inquirer, BodySink, Ipc::Port, and Comm::ConnOpener.
Definition at line 112 of file AsyncJob.cc.
Referenced by done(), Comm::ConnOpener::doneAll(), BodySink::doneAll(), Server::doneAll(), PeerPoolMgr::doneAll(), Rock::HeaderUpdater::doneAll(), Comm::TcpAcceptor::doneAll(), Adaptation::Iterator::doneAll(), Log::TcpLogger::doneAll(), Downloader::doneAll(), Security::PeerConnector::doneAll(), Adaptation::Icap::Launcher::doneAll(), Rock::Rebuild::doneAll(), Client::doneAll(), Ipc::UdsSender::doneAll(), Adaptation::Ecap::XactionRep::doneAll(), Adaptation::Icap::Xaction::doneAll(), Adaptation::Icap::ServiceRep::doneAll(), ClientHttpRequest::doneAll(), and ConnStateData::doneAll().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleStopRequest()
|
inline |
Definition at line 73 of file AsyncJob.h.
References mustStop().
◆ mustStop()
|
protected |
Definition at line 85 of file AsyncJob.cc.
References debugs, inCall, Must, stopReason, and typeName.
Referenced by HttpStateData::abortAll(), callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ RegisterWithCacheManager()
|
static |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotected |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by RegisterWithCacheManager().
◆ start()
|
protectedvirtual |
Reimplemented in Ipc::Port, ConnStateData, Adaptation::Icap::ModXact, Ftp::Client, HappyConnOpener, Ftp::Gateway, Ftp::Server, HttpStateData, Adaptation::Ecap::XactionRep, Ipc::UdsSender, Adaptation::Icap::Xaction, Rock::Rebuild, Downloader, Adaptation::AccessCheck, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Adaptation::Iterator, Http::One::Server, Ipc::Forwarder, Comm::TcpAcceptor, Ipc::Coordinator, Ipc::Inquirer, Rock::HeaderUpdater, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Mgr::Inquirer, Snmp::Inquirer, Adaptation::Icap::OptXact, Ipc::Strand, Mgr::Filler, and Mgr::ActionWriter.
Definition at line 59 of file AsyncJob.cc.
Referenced by Ipc::Port::start(), PeerPoolMgr::start(), Start(), Adaptation::Iterator::start(), Http::Tunneler::start(), Adaptation::AccessCheck::start(), Adaptation::Icap::Launcher::start(), Security::PeerConnector::start(), Adaptation::Icap::Xaction::start(), Ipc::UdsSender::start(), and ConnStateData::start().
◆ Start()
|
static |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, start(), and started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
protectedvirtual |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), stopReason, and MemBuf::terminate().
Referenced by callEnd(), callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ swanSong()
|
inlineprotectedvirtual |
Reimplemented in Adaptation::Icap::ModXactLauncher, Adaptation::Icap::ModXact, ConnStateData, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Client, Ipc::UdsSender, Rock::Rebuild, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Downloader, Adaptation::Iterator, Comm::TcpAcceptor, Ipc::Forwarder, Adaptation::Initiate, Ipc::Inquirer, Adaptation::Icap::OptXact, Rock::HeaderUpdater, Mgr::Forwarder, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Snmp::Forwarder, and Mgr::Filler.
Definition at line 61 of file AsyncJob.h.
Referenced by callEnd(), Server::swanSong(), Comm::ConnOpener::swanSong(), PeerPoolMgr::swanSong(), Rock::HeaderUpdater::swanSong(), Comm::TcpAcceptor::swanSong(), Http::Tunneler::swanSong(), Log::TcpLogger::swanSong(), Security::PeerConnector::swanSong(), Ipc::UdsSender::swanSong(), Client::swanSong(), and HappyConnOpener::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by callException(), and callStart().
Member Data Documentation
◆ id
const InstanceId<AsyncJob> AsyncJob::id |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protected |
Definition at line 86 of file AsyncJob.h.
Referenced by callEnd(), callStart(), canBeCalled(), deleteThis(), and mustStop().
◆ started_
|
protected |
Definition at line 88 of file AsyncJob.h.
Referenced by callEnd(), Start(), and ~AsyncJob().
◆ stopReason
|
protected |
Definition at line 84 of file AsyncJob.h.
Referenced by deleteThis(), done(), mustStop(), status(), and HappyConnOpener::status().
◆ swanSang_
|
protected |
Definition at line 89 of file AsyncJob.h.
Referenced by callEnd(), and ~AsyncJob().
◆ typeName
|
protected |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob(), callEnd(), callStart(), deleteThis(), mustStop(), Adaptation::Icap::Xaction::Xaction(), and ~AsyncJob().
The documentation for this class was generated from the following files:
- src/base/AsyncJob.h
- src/base/AsyncJob.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products