#include <Server.h>
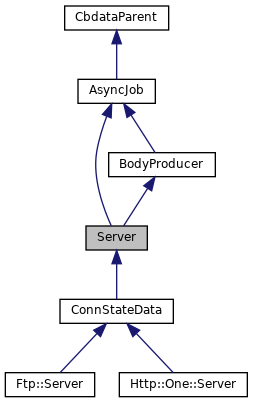
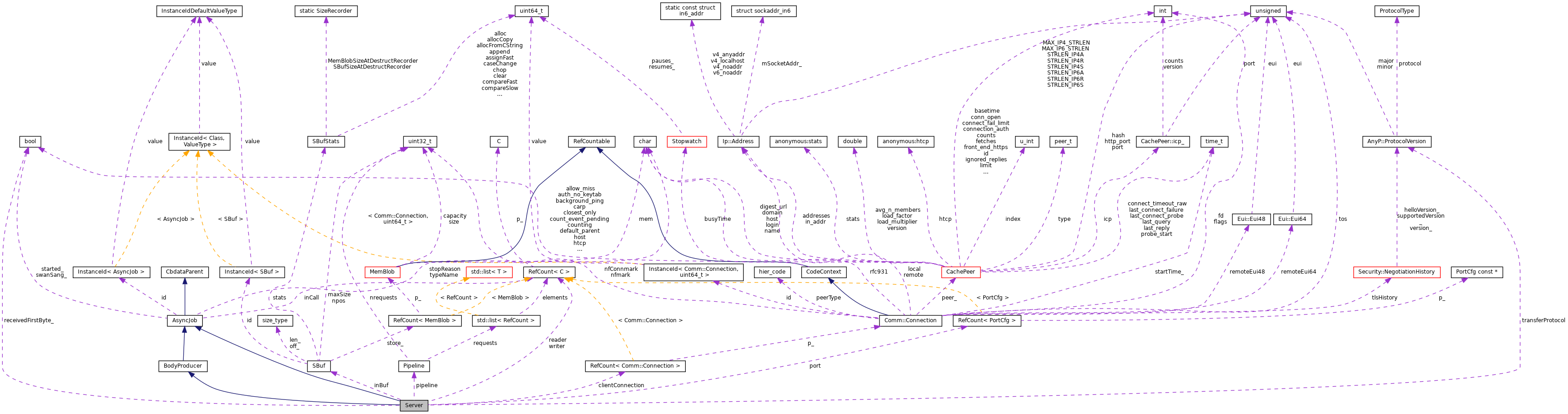
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
Public Member Functions | |
Server (const MasterXactionPointer &xact) | |
~Server () override | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
virtual bool | shouldCloseOnEof () const =0 |
whether to stop serving our client after reading EOF on its connection More... | |
void | readSomeData () |
maybe grow the inBuf and schedule Comm::Read() More... | |
virtual bool | handleReadData ()=0 |
virtual void | afterClientRead ()=0 |
processing to be done after a Comm::Read() More... | |
bool | reading () const |
whether Comm::Read() is scheduled More... | |
void | stopReading () |
cancels Comm::Read() if it is scheduled More... | |
virtual void | receivedFirstByte ()=0 |
Update flags and timeout after the first byte received. More... | |
virtual void | writeSomeData () |
maybe find some data to send and schedule a Comm::Write() More... | |
void | write (MemBuf *mb) |
schedule some data for a Comm::Write() More... | |
void | write (char *buf, int len) |
schedule some data for a Comm::Write() More... | |
virtual void | afterClientWrite (size_t) |
processing to sync state after a Comm::Write() More... | |
bool | writing () const |
whether Comm::Write() is scheduled More... | |
void | maybeMakeSpaceAvailable () |
grows the available read buffer space (if possible) More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
virtual void | noteMoreBodySpaceAvailable (RefCount< BodyPipe > bp)=0 |
virtual void | noteBodyConsumerAborted (RefCount< BodyPipe > bp)=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
Comm::ConnectionPointer | clientConnection |
AnyP::ProtocolVersion | transferProtocol |
AnyP::PortCfgPointer | port |
Squid listening port details where this connection arrived. More... | |
SBuf | inBuf |
read I/O buffer for the client connection More... | |
bool | receivedFirstByte_ |
true if at least one byte received on this connection More... | |
Pipeline | pipeline |
set of requests waiting to be serviced More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
virtual void | terminateAll (const Error &, const LogTagsErrors &)=0 |
abort any pending transactions and prevent new ones (by closing) More... | |
bool | mayBufferMoreRequestBytes () const |
whether client_request_buffer_max_size allows inBuf.length() increase More... | |
void | doClientRead (const CommIoCbParams &io) |
void | clientWriteDone (const CommIoCbParams &io) |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
AsyncCall::Pointer | reader |
set when we are reading More... | |
AsyncCall::Pointer | writer |
set when we are writing More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Detailed Description
Common base for all Server classes used to manage connections from clients.
Member Typedef Documentation
◆ Pointer [1/2]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [2/2]
|
inherited |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ Server()
Server::Server | ( | const MasterXactionPointer & | xact | ) |
Definition at line 26 of file Server.cc.
References clientConnection, and Comm::Connection::leaveOrphanage().
◆ ~Server()
Member Function Documentation
◆ afterClientRead()
|
pure virtual |
Implemented in ConnStateData.
Referenced by doClientRead().
◆ afterClientWrite()
|
inlinevirtual |
Reimplemented in ConnStateData.
Definition at line 86 of file Server.h.
Referenced by clientWriteDone().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ clientWriteDone()
|
protected |
callback handling the Comm::Write completion
Will call afterClientWrite(size_t) to sync the I/O state. Then writeSomeData() to initiate any followup writes that could be immediately done.
Definition at line 211 of file Server.cc.
References afterClientWrite(), clientConnection, CommCommonCbParams::conn, debugs, Comm::ERR_CLOSING, ERR_WRITE_ERROR, Helper::Error, Comm::Connection::fd, CommCommonCbParams::flag, LogTagsErrors::FromErrno(), Comm::IsConnOpen(), Must, SysErrorDetail::NewIfAny(), CommIoCbParams::size, terminateAll(), writer, writeSomeData(), CommCommonCbParams::xerrno, and xstrerr().
Referenced by write().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ doClientRead()
|
protected |
Definition at line 115 of file Server.cc.
References LogTagsErrors::aborted, afterClientRead(), assert, Assure, StatCounters::client_http, clientConnection, commMarkHalfClosed(), Config, CommCommonCbParams::conn, debugs, Comm::ENDFILE, ERR_CLIENT_GONE, Comm::ERR_CLOSING, ERR_READ_ERROR, Helper::Error, Comm::Connection::fd, fd_note(), fd_table, CommCommonCbParams::flag, LogTagsErrors::FromErrno(), handleReadData(), inBuf, Comm::INPROGRESS, Comm::IsConnOpen(), SBuf::isEmpty(), StatCounters::kbytes_in, SBuf::length(), SquidConfig::maxRequestBufferSize, maybeMakeSpaceAvailable(), mayBufferMoreRequestBytes(), Must, SysErrorDetail::NewIfAny(), Comm::OK, reader, reading(), Comm::ReadNow(), readSomeData(), receivedFirstByte(), receivedFirstByte_, shouldCloseOnEof(), CommIoCbParams::size, SBuf::spaceSize(), statCounter, terminateAll(), CommCommonCbParams::xerrno, and xstrerr().
Referenced by readSomeData().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 37 of file Server.cc.
References clientConnection, AsyncJob::doneAll(), and Comm::IsConnOpen().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleReadData()
|
pure virtual |
called when new request data has been read from the socket
- Return values
-
false called comm_close or setReplyToError (the caller should bail) true we did not call comm_close or setReplyToError
Implemented in ConnStateData.
Referenced by doClientRead().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ maybeMakeSpaceAvailable()
void Server::maybeMakeSpaceAvailable | ( | ) |
Prepare inBuf for I/O. This method balances several conflicting desires:
- Do not read too few bytes at a time.
- Do not waste too much buffer space.
- Do not [re]allocate or memmove the buffer too much.
- Obey Config.maxRequestBufferSize limit.
Definition at line 74 of file Server.cc.
References SBufReservationRequirements::allowShared, CLIENT_REQ_BUF_SZ, Config, debugs, SBufReservationRequirements::idealSpace, inBuf, SBufReservationRequirements::maxCapacity, SquidConfig::maxRequestBufferSize, SBufReservationRequirements::minSpace, SBuf::reserve(), and SBuf::spaceSize().
Referenced by doClientRead().
◆ mayBufferMoreRequestBytes()
|
protected |
Definition at line 89 of file Server.cc.
References Config, debugs, inBuf, SBuf::length(), and SquidConfig::maxRequestBufferSize.
Referenced by doClientRead(), and readSomeData().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteBodyConsumerAborted()
Implemented in Adaptation::Ecap::XactionRep, ConnStateData, Adaptation::Icap::ModXact, Client, Http::One::Server, and Ftp::Server.
Referenced by BodyPipe::expectNoConsumption().
◆ noteMoreBodySpaceAvailable()
|
pure virtualinherited |
Implemented in Adaptation::Ecap::XactionRep, ConnStateData, Adaptation::Icap::ModXact, Ftp::Server, Client, and Http::One::Server.
Referenced by BodyPipe::postConsume().
◆ reading()
|
inline |
Definition at line 60 of file Server.h.
References reader.
Referenced by doClientRead(), readSomeData(), and stopReading().
◆ readSomeData()
void Server::readSomeData | ( | ) |
Definition at line 101 of file Server.cc.
References clientConnection, doClientRead(), JobCallback, mayBufferMoreRequestBytes(), Comm::Read(), reader, and reading().
Referenced by ConnStateData::clientAfterReadingRequests(), clientNegotiateSSL(), doClientRead(), ConnStateData::parseTlsHandshake(), ConnStateData::readNextRequest(), and ConnStateData::switchToHttps().
◆ receivedFirstByte()
|
pure virtual |
Implemented in ConnStateData.
Referenced by doClientRead().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ shouldCloseOnEof()
|
pure virtual |
Implemented in ConnStateData.
Referenced by doClientRead().
◆ start()
|
overridevirtual |
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), Client::serverComplete2(), and ConnStateData::~ConnStateData().
◆ stopReading()
void Server::stopReading | ( | ) |
Definition at line 60 of file Server.cc.
References clientConnection, Comm::Connection::fd, Comm::ReadCancel(), reader, and reading().
Referenced by ClientHttpRequest::processRequest().
◆ swanSong()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 51 of file Server.cc.
References clientConnection, Comm::Connection::close(), Comm::IsConnOpen(), and AsyncJob::swanSong().
Referenced by ConnStateData::swanSong().
◆ terminateAll()
|
protectedpure virtual |
Implemented in ConnStateData.
Referenced by clientWriteDone(), and doClientRead().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ write() [1/2]
|
inline |
Definition at line 79 of file Server.h.
References clientConnection, clientWriteDone(), JobCallback, Comm::Write(), and writer.
◆ write() [2/2]
|
inline |
Definition at line 72 of file Server.h.
References clientConnection, clientWriteDone(), JobCallback, Comm::Write(), and writer.
◆ writeSomeData()
|
inlinevirtual |
Definition at line 69 of file Server.h.
Referenced by clientWriteDone().
◆ writing()
Member Data Documentation
◆ clientConnection
Comm::ConnectionPointer Server::clientConnection |
Definition at line 100 of file Server.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::abortRequestParsing(), ConnStateData::acceptTls(), ConnStateData::afterClientRead(), Format::Format::assemble(), Auth::UserRequest::authenticate(), ConnStateData::buildFakeRequest(), ConnStateData::clientAfterReadingRequests(), clientBuildError(), ClientHttpRequest::ClientHttpRequest(), clientNegotiateSSL(), ConnStateData::clientPinnedConnectionClosed(), ConnStateData::clientPinnedConnectionRead(), clientProcessRequestFinished(), ClientRequestContext::clientRedirectDone(), ClientSocketContextPushDeferredIfNeeded(), clientSocketRecipient(), clientWriteDone(), ConnStateData::concurrentRequestQueueFilled(), ConnStateData::connStateClosed(), doClientRead(), doneAll(), ConnStateData::doneWithControlMsg(), ConnStateData::doPeekAndSpliceStep(), ConnStateData::endingShutdown(), ConnStateData::extendLifetime(), ConnStateData::fakeAConnectRequest(), ConnStateData::fillConnectionLevelDetails(), ConnStateData::getSslContextDone(), ConnStateData::getSslContextStart(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), ConnStateData::handleSslBumpHandshakeError(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), httpsCreate(), httpsEstablish(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), ConnStateData::initiateTunneledRequest(), ConnStateData::isOpen(), ConnStateData::kick(), HttpRequest::manager(), ClientHttpRequest::noteBodyProducerAborted(), ConnStateData::parseHttpRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::pinConnection(), ConnStateData::postHttpsAccept(), prepareAcceleratedURL(), prepareTransparentURL(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), ConnStateData::proxyProtocolError(), ConnStateData::quitAfterError(), ConnStateData::readNextRequest(), readSomeData(), ConnStateData::resetReadTimeout(), PeerSelector::resolveSelected(), ConnStateData::sendControlMsg(), ConnStateData::serveDelayedError(), Server(), ConnStateData::setAuth(), ConnStateData::splice(), ClientRequestContext::sslBumpAccessCheckDone(), ClientHttpRequest::sslBumpEstablish(), ClientHttpRequest::sslBumpStart(), ConnStateData::sslCrtdHandleReply(), ConnStateData::start(), ConnStateData::startPeekAndSplice(), statClientRequests(), stopReading(), ConnStateData::stopReceiving(), ConnStateData::stopSending(), ConnStateData::storeTlsContextToCache(), swanSong(), ConnStateData::swanSong(), ConnStateData::switchToHttps(), ConnStateData::terminateAll(), ConnStateData::transparent(), ConnStateData::tunnelOnError(), tunnelStart(), TunnelStateData::TunnelStateData(), ConnStateData::whenClientIpKnown(), write(), and ConnStateData::~ConnStateData().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inBuf
SBuf Server::inBuf |
Definition at line 113 of file Server.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::abortRequestParsing(), ConnStateData::buildFakeRequest(), ConnStateData::checkLogging(), ConnStateData::consumeInput(), doClientRead(), ConnStateData::getSslContextDone(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), httpsSslBumpAccessCheckDone(), maybeMakeSpaceAvailable(), mayBufferMoreRequestBytes(), ConnStateData::mayNeedToReadMoreBody(), ConnStateData::parseHttpRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::shouldCloseOnEof(), ConnStateData::startPeekAndSplice(), statClientRequests(), ConnStateData::stopSending(), ConnStateData::terminateAll(), and tunnelStartShoveling().
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ pipeline
Pipeline Server::pipeline |
Definition at line 118 of file Server.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::add(), ConnStateData::afterClientRead(), ConnStateData::afterClientWrite(), ConnStateData::checkLogging(), clientNegotiateSSL(), ConnStateData::clientPinnedConnectionRead(), clientSocketRecipient(), ConnStateData::concurrentRequestQueueFilled(), ConnStateData::doneWithControlMsg(), ConnStateData::fillChecklist(), ConnStateData::finishDechunkingRequest(), Http::Stream::finished(), ConnStateData::httpsPeeked(), httpsSslBumpStep2AccessCheckDone(), ConnStateData::kick(), ConnStateData::notePinnedConnectionBecameIdle(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::postHttpsAccept(), ConnStateData::sendControlMsg(), ConnStateData::shouldCloseOnEof(), ConnStateData::shouldPreserveClientData(), ConnStateData::splice(), ConnStateData::startPeekAndSplice(), ConnStateData::startShutdown(), statClientRequests(), ConnStateData::terminateAll(), ConnStateData::tunnelOnError(), and ConnStateData::updateError().
◆ port
AnyP::PortCfgPointer Server::port |
◆ reader
|
protected |
Definition at line 130 of file Server.h.
Referenced by doClientRead(), reading(), readSomeData(), and stopReading().
◆ receivedFirstByte_
bool Server::receivedFirstByte_ |
Definition at line 115 of file Server.h.
Referenced by doClientRead(), ConnStateData::receivedFirstByte(), ConnStateData::requestTimeout(), and ConnStateData::switchToHttps().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ transferProtocol
AnyP::ProtocolVersion Server::transferProtocol |
The transfer protocol currently being spoken on this connection. HTTP/1.x CONNECT, HTTP/1.1 Upgrade and HTTP/2 SETTINGS offer the ability to change protocols on the fly.
Definition at line 107 of file Server.h.
Referenced by buildUrlFromHost(), ConnStateData::parseHttpRequest(), prepareAcceleratedURL(), ConnStateData::prepareTlsSwitchingURL(), prepareTransparentURL(), ConnStateData::splice(), and ConnStateData::switchToHttps().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ writer
|
protected |
Definition at line 131 of file Server.h.
Referenced by clientWriteDone(), write(), and writing().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products