#include <HappyConnOpener.h>
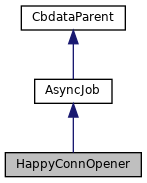
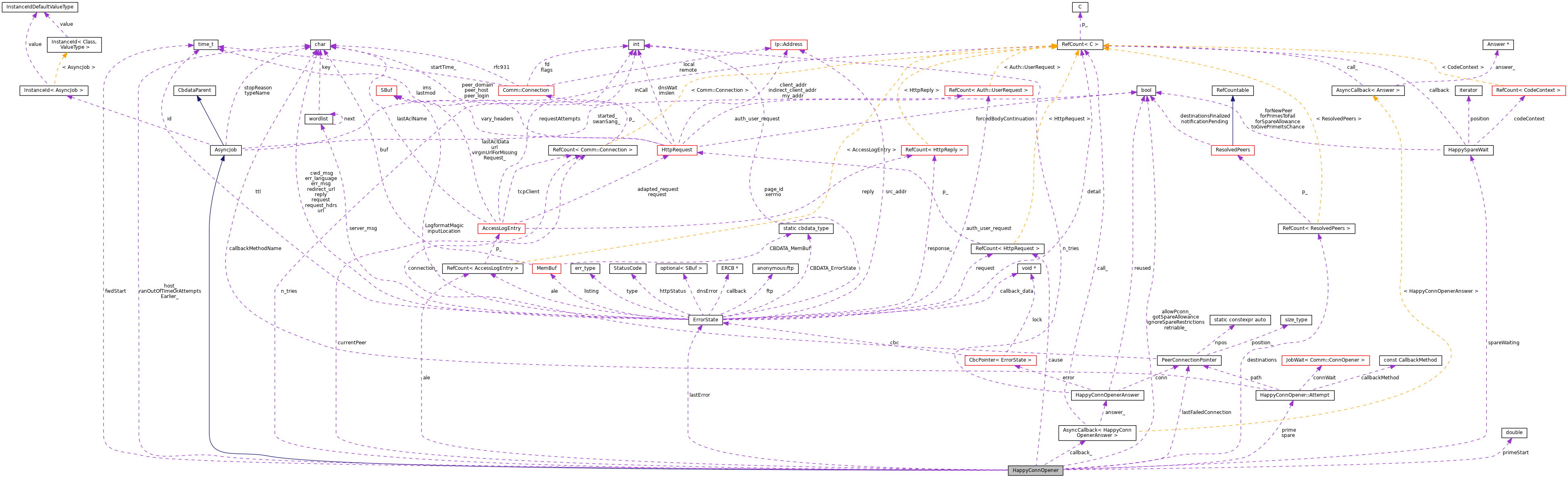
Classes | |
class | Attempt |
a connection opening attempt in progress (or falsy) More... | |
Public Types | |
typedef HappyConnOpenerAnswer | Answer |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
HappyConnOpener (const ResolvedPeersPointer &, const AsyncCallback< Answer > &, const HttpRequestPointer &, time_t aFwdStart, int tries, const AccessLogEntryPointer &) | |
~HappyConnOpener () override | |
void | allowPersistent (bool permitted) |
configures reuse of old connections More... | |
void | setRetriable (bool retriable) |
configures whether the request may be retried later if things go wrong More... | |
void | setHost (const char *) |
configures the origin server domain name More... | |
void | noteCandidatesChange () |
reacts to changes in the destinations list More... | |
void | noteGavePrimeItsChance () |
reacts to expired happy_eyeballs_connect_timeout More... | |
void | noteSpareAllowance () |
reacts to satisfying happy_eyeballs_connect_gap and happy_eyeballs_connect_limit More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
HappyAbsoluteTime | primeStart = 0 |
the start of the first connection attempt for the currentPeer More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (HappyConnOpener) | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
void | maybeOpenPrimeConnection () |
starts a prime connection attempt if possible or does nothing otherwise More... | |
void | maybeOpenSpareConnection () |
if possible, starts a spare connection attempt More... | |
void | maybeGivePrimeItsChance () |
void | stopGivingPrimeItsChance () |
called when the prime attempt has used up its chance for a solo victory More... | |
void | stopWaitingForSpareAllowance () |
called when the spare attempt should no longer obey spare connection limits More... | |
void | startConnecting (Attempt &, PeerConnectionPointer &) |
starts opening (or reusing) a connection to the given destination More... | |
void | openFreshConnection (Attempt &, PeerConnectionPointer &) |
bool | reuseOldConnection (PeerConnectionPointer &) |
void | notePrimeConnectDone (const CommConnectCbParams &) |
Comm::ConnOpener callback for the prime connection attempt. More... | |
void | noteSpareConnectDone (const CommConnectCbParams &) |
Comm::ConnOpener callback for the spare connection attempt. More... | |
void | handleConnOpenerAnswer (Attempt &, const CommConnectCbParams &, const char *connDescription) |
prime/spare-agnostic processing of a Comm::ConnOpener result More... | |
void | checkForNewConnection () |
void | updateSpareWaitAfterPrimeFailure () |
reacts to a prime attempt failure More... | |
void | cancelSpareWait (const char *reason) |
stops waiting for the right conditions to open a spare connection More... | |
bool | ranOutOfTimeOrAttempts () const |
Check for maximum connection tries and forwarding time restrictions. More... | |
ErrorState * | makeError (const err_type type) const |
Answer * | futureAnswer (const PeerConnectionPointer &) |
void | sendSuccess (const PeerConnectionPointer &conn, bool reused, const char *connKind) |
send a successful result to the initiator (if it still needs an answer) More... | |
void | sendFailure () |
inform the initiator about our failure to connect (if needed) More... | |
void | cancelAttempt (Attempt &, const char *reason) |
cancels the in-progress attempt, making its path a future candidate More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
const time_t | fwdStart |
requestor start time More... | |
AsyncCallback< Answer > | callback_ |
answer destination More... | |
ResolvedPeersPointer | destinations |
Candidate paths. Shared with the initiator. May not be finalized yet. More... | |
Attempt | prime |
current connection opening attempt on the prime track (if any) More... | |
Attempt | spare |
current connection opening attempt on the spare track (if any) More... | |
Comm::ConnectionPointer | currentPeer |
HappySpareWait | spareWaiting |
preconditions for an attempt to open a spare connection More... | |
AccessLogEntryPointer | ale |
transaction details More... | |
ErrorState * | lastError = nullptr |
last problem details (or nil) More... | |
PeerConnectionPointer | lastFailedConnection |
nil if none has failed More... | |
bool | ignoreSpareRestrictions = false |
whether spare connection attempts disregard happy_eyeballs_* settings More... | |
bool | gotSpareAllowance = false |
whether we have received a permission to open a spare while spares are limited More... | |
bool | allowPconn_ = true |
whether persistent connections are allowed More... | |
bool | retriable_ = true |
whether we are opening connections for a request that may be resent More... | |
const char * | host_ = nullptr |
origin server domain name (or equivalent) More... | |
HttpRequestPointer | cause |
the request that needs a to-server connection More... | |
int | n_tries |
const char * | ranOutOfTimeOrAttemptsEarlier_ = nullptr |
Reason to ran out of time or attempts. More... | |
Friends | |
class | HappyOrderEnforcer |
std::ostream & | operator<< (std::ostream &, const Attempt &) |
HappyConnOpener::Attempt printer for debugging. More... | |
Detailed Description
A TCP connection opening algorithm based on Happy Eyeballs (RFC 8305). Maintains two concurrent connection opening tracks: prime and spare. Shares ResolvedPeers list with the job initiator.
Definition at line 105 of file HappyConnOpener.h.
Member Typedef Documentation
◆ Answer
Definition at line 109 of file HappyConnOpener.h.
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ HappyConnOpener()
HappyConnOpener::HappyConnOpener | ( | const ResolvedPeersPointer & | dests, |
const AsyncCallback< Answer > & | callback, | ||
const HttpRequestPointer & | request, | ||
time_t | aFwdStart, | ||
int | tries, | ||
const AccessLogEntryPointer & | anAle | ||
) |
Definition at line 330 of file HappyConnOpener.cc.
References assert, and destinations.
◆ ~HappyConnOpener()
|
override |
Definition at line 344 of file HappyConnOpener.cc.
Member Function Documentation
◆ allowPersistent()
|
inline |
Definition at line 116 of file HappyConnOpener.h.
References allowPconn_.
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cancelAttempt()
|
private |
Definition at line 511 of file HappyConnOpener.cc.
References HappyConnOpener::Attempt::cancel(), destinations, Must, HappyConnOpener::Attempt::path, and ResolvedPeers::reinstatePath().
Referenced by swanSong().
◆ cancelSpareWait()
|
private |
Definition at line 691 of file HappyConnOpener.cc.
References HappySpareWait::clear(), debugs, HappySpareWait::forSpareAllowance, Must, spareWaiting, stopGivingPrimeItsChance(), stopWaitingForSpareAllowance(), and HappySpareWait::toGivePrimeItsChance.
Referenced by checkForNewConnection(), swanSong(), and updateSpareWaitAfterPrimeFailure().
◆ CBDATA_CHILD()
|
private |
◆ checkForNewConnection()
|
private |
Called when an external event changes initiator interest, destinations, prime, spare, or spareWaiting. Leaves HappyConnOpener in one of these mutually exclusive "stable" states:
- Processing a single peer: currentPeer && !done() 1.1. Connecting: prime || spare 1.2. Waiting for spare gap and/or paths: !prime && !spare
- Waiting for a new peer: destinations->empty() && !destinations->destinationsFinalized && !currentPeer && !done()
- Terminating: done()
Definition at line 715 of file HappyConnOpener.cc.
References cancelSpareWait(), currentPeer, debugs, destinations, AsyncJob::done(), ResolvedPeers::doneWithPeer(), ResolvedPeers::doneWithSpares(), HappySpareWait::forNewPeer, gotSpareAllowance, ignoreSpareRestrictions, maybeOpenPrimeConnection(), maybeOpenSpareConnection(), Must, prime, ranOutOfTimeOrAttempts(), spare, and spareWaiting.
Referenced by handleConnOpenerAnswer(), noteCandidatesChange(), noteGavePrimeItsChance(), and start().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), checkForNewConnection(), Downloader::downloadFinished(), and maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 366 of file HappyConnOpener.cc.
References callback_, destinations, ResolvedPeers::destinationsFinalized, ResolvedPeers::empty(), prime, ranOutOfTimeOrAttempts(), and spare.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ futureAnswer()
|
private |
- Returns
- pre-filled Answer if the initiator needs an answer (or nil)
Definition at line 485 of file HappyConnOpener.cc.
References callback_, and n_tries.
Referenced by sendFailure(), and sendSuccess().
◆ handleConnOpenerAnswer()
|
private |
Definition at line 616 of file HappyConnOpener.cc.
References checkForNewConnection(), CommCommonCbParams::conn, debugs, ERR_CONNECT_FAIL, PeerConnectionPointer::finalize(), HappyConnOpener::Attempt::finish(), CommCommonCbParams::flag, Comm::Connection::getPeer(), lastError, lastFailedConnection, makeError(), Must, n_tries, NoteOutgoingConnectionFailure(), Comm::OK, HappyConnOpener::Attempt::path, sendSuccess(), spareWaiting, updateSpareWaitAfterPrimeFailure(), CommCommonCbParams::xerrno, and ErrorState::xerrno.
Referenced by notePrimeConnectDone(), and noteSpareConnectDone().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ makeError()
|
private |
Create "503 Service Unavailable" or "504 Gateway Timeout" error depending on whether this is a validation request.
RFC 9111 section 5.2.2 Cache-Control response directives
section 5.2.2.2 must-revalidate " if a cache is disconnected, the cache MUST generate an error response rather than reuse the stale response. The generated status code SHOULD be 504 (Gateway Timeout) unless another error status code is more applicable."
section 5.2.2.8 proxy-revalidate " analogous to must-revalidate "
section 5.2.2.10 s-maxage " incorporates the semantics of the proxy-revalidate response directive "
Definition at line 476 of file HappyConnOpener.cc.
References ale, cause, HttpRequest::flags, RefCount< C >::getRaw(), RequestFlags::needValidation, Http::scGatewayTimeout, and Http::scServiceUnavailable.
Referenced by handleConnOpenerAnswer(), and sendFailure().
◆ maybeGivePrimeItsChance()
|
private |
starts waiting for a spare permission (if spare connections may be possible) or does nothing (otherwise)
Definition at line 814 of file HappyConnOpener.cc.
References Config, SquidConfig::connect_limit, currentPeer, debugs, destinations, ResolvedPeers::doneWithSpares(), HappyOrderEnforcer::enqueue(), HappySpareWait::forNewPeer, HappySpareWait::forPrimesToFail, SquidConfig::happyEyeballs, Must, prime, PrimeChanceGiver::readyNow(), spare, spareWaiting, ThePrimeChanceGiver, and HappySpareWait::toGivePrimeItsChance.
Referenced by maybeOpenPrimeConnection().
◆ maybeOpenPrimeConnection()
|
private |
Definition at line 782 of file HappyConnOpener.cc.
References current_dtime, currentPeer, debugs, destinations, AsyncJob::done(), ResolvedPeers::empty(), ResolvedPeers::extractFront(), ResolvedPeers::extractPrime(), maybeGivePrimeItsChance(), Must, prime, primeStart, and startConnecting().
Referenced by checkForNewConnection().
◆ maybeOpenSpareConnection()
|
private |
Definition at line 845 of file HappyConnOpener.cc.
References currentPeer, destinations, ResolvedPeers::empty(), HappyOrderEnforcer::enqueue(), ResolvedPeers::extractSpare(), HappySpareWait::forSpareAllowance, gotSpareAllowance, ResolvedPeers::haveSpare(), ignoreSpareRestrictions, SpareAllowanceGiver::jobGotInstantAllowance(), Must, ranOutOfTimeOrAttempts(), SpareAllowanceGiver::readyNow(), spare, spareWaiting, startConnecting(), and TheSpareAllowanceGiver.
Referenced by checkForNewConnection().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteCandidatesChange()
void HappyConnOpener::noteCandidatesChange | ( | ) |
Definition at line 534 of file HappyConnOpener.cc.
References checkForNewConnection(), destinations, and ResolvedPeers::notificationPending.
◆ noteGavePrimeItsChance()
void HappyConnOpener::noteGavePrimeItsChance | ( | ) |
Definition at line 755 of file HappyConnOpener.cc.
References checkForNewConnection(), HappySpareWait::clear(), Must, spareWaiting, and HappySpareWait::toGivePrimeItsChance.
◆ notePrimeConnectDone()
|
private |
Definition at line 598 of file HappyConnOpener.cc.
References handleConnOpenerAnswer(), and prime.
◆ noteSpareAllowance()
void HappyConnOpener::noteSpareAllowance | ( | ) |
Definition at line 763 of file HappyConnOpener.cc.
References HappySpareWait::clear(), currentPeer, destinations, ResolvedPeers::extractSpare(), HappySpareWait::forSpareAllowance, gotSpareAllowance, SpareAllowanceGiver::jobDroppedAllowance(), Must, ranOutOfTimeOrAttempts(), spare, spareWaiting, startConnecting(), and TheSpareAllowanceGiver.
◆ noteSpareConnectDone()
|
private |
Definition at line 605 of file HappyConnOpener.cc.
References gotSpareAllowance, handleConnOpenerAnswer(), SpareAllowanceGiver::jobUsedAllowance(), spare, and TheSpareAllowanceGiver.
◆ openFreshConnection()
|
private |
opens a fresh connection to the given destination must be called via startConnecting()
Definition at line 575 of file HappyConnOpener.cc.
References asyncCall(), HappyConnOpener::Attempt::callbackMethod, HappyConnOpener::Attempt::callbackMethodName, cause, Comm::Connection::cloneProfile(), Comm::Connection::connectTimeout(), HappyConnOpener::Attempt::connWait, fwdStart, GetMarkingsToServer(), RefCount< C >::getRaw(), host_, HappyConnOpener::Attempt::path, and JobWait< Job >::start().
Referenced by startConnecting().
◆ ranOutOfTimeOrAttempts()
|
private |
Definition at line 885 of file HappyConnOpener.cc.
References Config, debugs, SquidConfig::forward_max_tries, FwdState::ForwardTimeout(), fwdStart, n_tries, and ranOutOfTimeOrAttemptsEarlier_.
Referenced by checkForNewConnection(), doneAll(), maybeOpenSpareConnection(), and noteSpareAllowance().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ reuseOldConnection()
|
private |
reuses a persistent connection to the given destination (if possible)
- Returns
- true if and only if reuse was possible must be called via startConnecting()
Definition at line 558 of file HappyConnOpener.cc.
References allowPconn_, assert, PeerConnectionPointer::finalize(), fwdPconnPool, host_, n_tries, PconnPool::pop(), retriable_, and sendSuccess().
Referenced by startConnecting().
◆ sendFailure()
|
private |
Definition at line 520 of file HappyConnOpener.cc.
References assert, callback_, debugs, ERR_GATEWAY_FAILURE, futureAnswer(), lastError, lastFailedConnection, makeError(), and ScheduleCallHere.
Referenced by swanSong().
◆ sendSuccess()
|
private |
Definition at line 499 of file HappyConnOpener.cc.
References assert, callback_, debugs, futureAnswer(), and ScheduleCallHere.
Referenced by handleConnOpenerAnswer(), and reuseOldConnection().
◆ setHost()
void HappyConnOpener::setHost | ( | const char * | h | ) |
Definition at line 351 of file HappyConnOpener.cc.
◆ setRetriable()
|
inline |
Definition at line 119 of file HappyConnOpener.h.
References retriable_.
◆ start()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 359 of file HappyConnOpener.cc.
References checkForNewConnection(), destinations, and ResolvedPeers::notificationPending.
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startConnecting()
|
private |
Definition at line 542 of file HappyConnOpener.cc.
References allowPconn_, cause, HappyConnOpener::Attempt::connWait, HttpRequest::flags, Comm::Connection::getPeer(), Must, openFreshConnection(), HappyConnOpener::Attempt::path, reuseOldConnection(), and RequestFlags::sslBumped.
Referenced by maybeOpenPrimeConnection(), maybeOpenSpareConnection(), and noteSpareAllowance().
◆ status()
|
overrideprivatevirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 432 of file HappyConnOpener.cc.
References SBufStream::buf(), SBuf::c_str(), SBuf::clear(), destinations, n_tries, prime, spare, and AsyncJob::stopReason.
◆ stopGivingPrimeItsChance()
|
private |
Definition at line 672 of file HappyConnOpener.cc.
References HappyOrderEnforcer::dequeue(), Must, spareWaiting, ThePrimeChanceGiver, and HappySpareWait::toGivePrimeItsChance.
Referenced by cancelSpareWait(), and updateSpareWaitAfterPrimeFailure().
◆ stopWaitingForSpareAllowance()
|
private |
Definition at line 680 of file HappyConnOpener.cc.
References HappySpareWait::callback, HappyOrderEnforcer::dequeue(), HappySpareWait::forSpareAllowance, SpareAllowanceGiver::jobDroppedAllowance(), Must, spareWaiting, and TheSpareAllowanceGiver.
Referenced by cancelSpareWait().
◆ swanSong()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 389 of file HappyConnOpener.cc.
References callback_, cancelAttempt(), cancelSpareWait(), debugs, gotSpareAllowance, SpareAllowanceGiver::jobDroppedAllowance(), prime, sendFailure(), spare, spareWaiting, AsyncJob::swanSong(), and TheSpareAllowanceGiver.
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ updateSpareWaitAfterPrimeFailure()
|
private |
Definition at line 651 of file HappyConnOpener.cc.
References cancelSpareWait(), currentPeer, destinations, ResolvedPeers::doneWithPrimes(), ignoreSpareRestrictions, Must, prime, spareWaiting, stopGivingPrimeItsChance(), and HappySpareWait::toGivePrimeItsChance.
Referenced by handleConnOpenerAnswer().
Friends And Related Function Documentation
◆ HappyOrderEnforcer
|
friend |
Definition at line 218 of file HappyConnOpener.h.
◆ operator<<
|
friend |
Definition at line 418 of file HappyConnOpener.cc.
Member Data Documentation
◆ ale
|
private |
Definition at line 220 of file HappyConnOpener.h.
Referenced by makeError().
◆ allowPconn_
|
private |
Definition at line 232 of file HappyConnOpener.h.
Referenced by allowPersistent(), reuseOldConnection(), and startConnecting().
◆ callback_
|
private |
Definition at line 201 of file HappyConnOpener.h.
Referenced by doneAll(), futureAnswer(), sendFailure(), sendSuccess(), and swanSong().
◆ cause
|
private |
Definition at line 241 of file HappyConnOpener.h.
Referenced by makeError(), openFreshConnection(), and startConnecting().
◆ currentPeer
|
private |
CachePeer and IP address family of the peer we are trying to connect to now (or, if we are just waiting for paths to a new peer, nil)
Definition at line 214 of file HappyConnOpener.h.
Referenced by checkForNewConnection(), maybeGivePrimeItsChance(), maybeOpenPrimeConnection(), maybeOpenSpareConnection(), noteSpareAllowance(), and updateSpareWaitAfterPrimeFailure().
◆ destinations
|
private |
Definition at line 204 of file HappyConnOpener.h.
Referenced by cancelAttempt(), checkForNewConnection(), doneAll(), HappyConnOpener(), maybeGivePrimeItsChance(), maybeOpenPrimeConnection(), maybeOpenSpareConnection(), noteCandidatesChange(), noteSpareAllowance(), start(), status(), and updateSpareWaitAfterPrimeFailure().
◆ fwdStart
|
private |
Definition at line 198 of file HappyConnOpener.h.
Referenced by openFreshConnection(), and ranOutOfTimeOrAttempts().
◆ gotSpareAllowance
|
private |
Definition at line 229 of file HappyConnOpener.h.
Referenced by checkForNewConnection(), maybeOpenSpareConnection(), noteSpareAllowance(), noteSpareConnectDone(), and swanSong().
◆ host_
|
private |
Definition at line 238 of file HappyConnOpener.h.
Referenced by openFreshConnection(), reuseOldConnection(), setHost(), and ~HappyConnOpener().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ ignoreSpareRestrictions
|
private |
Definition at line 226 of file HappyConnOpener.h.
Referenced by checkForNewConnection(), maybeOpenSpareConnection(), and updateSpareWaitAfterPrimeFailure().
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ lastError
|
private |
Definition at line 222 of file HappyConnOpener.h.
Referenced by handleConnOpenerAnswer(), sendFailure(), and ~HappyConnOpener().
◆ lastFailedConnection
|
private |
Definition at line 223 of file HappyConnOpener.h.
Referenced by handleConnOpenerAnswer(), and sendFailure().
◆ n_tries
|
private |
number of our finished connection opening attempts (including pconn reuses) plus previously finished attempts supplied by the requestor
Definition at line 245 of file HappyConnOpener.h.
Referenced by futureAnswer(), handleConnOpenerAnswer(), ranOutOfTimeOrAttempts(), reuseOldConnection(), and status().
◆ prime
|
private |
Definition at line 207 of file HappyConnOpener.h.
Referenced by checkForNewConnection(), doneAll(), maybeGivePrimeItsChance(), maybeOpenPrimeConnection(), notePrimeConnectDone(), status(), swanSong(), and updateSpareWaitAfterPrimeFailure().
◆ primeStart
HappyAbsoluteTime HappyConnOpener::primeStart = 0 |
Definition at line 134 of file HappyConnOpener.h.
Referenced by maybeOpenPrimeConnection(), and PrimeChanceGiver::readyNow().
◆ ranOutOfTimeOrAttemptsEarlier_
|
mutableprivate |
Definition at line 248 of file HappyConnOpener.h.
Referenced by ranOutOfTimeOrAttempts().
◆ retriable_
|
private |
Definition at line 235 of file HappyConnOpener.h.
Referenced by reuseOldConnection(), and setRetriable().
◆ spare
|
private |
Definition at line 210 of file HappyConnOpener.h.
Referenced by checkForNewConnection(), doneAll(), maybeGivePrimeItsChance(), maybeOpenSpareConnection(), noteSpareAllowance(), noteSpareConnectDone(), status(), and swanSong().
◆ spareWaiting
|
private |
Definition at line 217 of file HappyConnOpener.h.
Referenced by cancelSpareWait(), checkForNewConnection(), HappyOrderEnforcer::dequeue(), HappyOrderEnforcer::enqueue(), handleConnOpenerAnswer(), maybeGivePrimeItsChance(), maybeOpenSpareConnection(), noteGavePrimeItsChance(), noteSpareAllowance(), stopGivingPrimeItsChance(), stopWaitingForSpareAllowance(), swanSong(), and updateSpareWaitAfterPrimeFailure().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
The documentation for this class was generated from the following files:
- src/HappyConnOpener.h
- src/HappyConnOpener.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products