#include <TcpLogger.h>
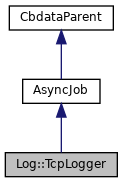
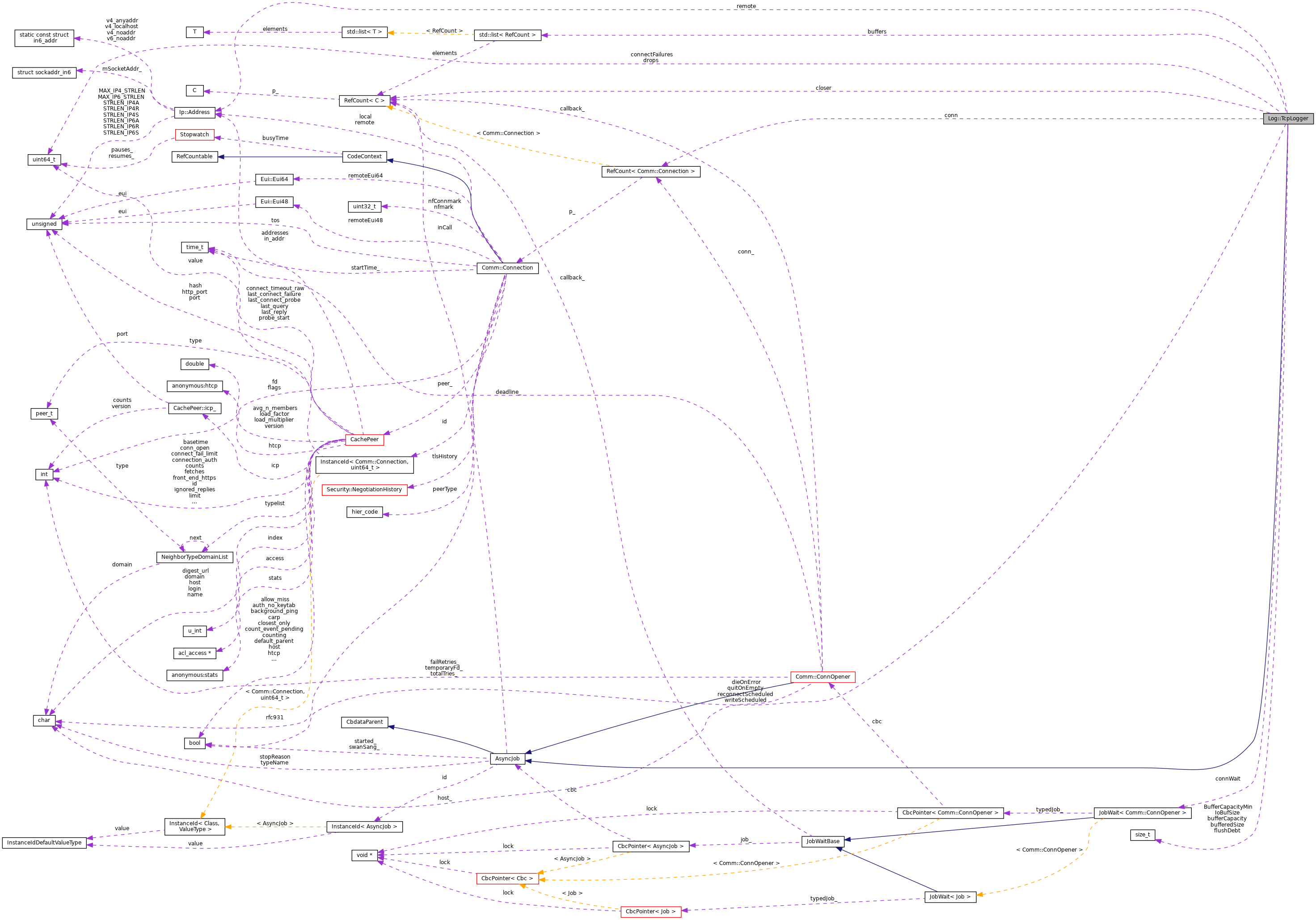
Public Types | |
typedef CbcPointer< TcpLogger > | Pointer |
Public Member Functions | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static int | Open (Logfile *lf, const char *path, size_t bufSz, int fatalFlag) |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
TcpLogger (size_t, bool, Ip::Address) | |
~TcpLogger () override | |
void | endGracefully () |
void | logRecord (const char *buf, size_t len) |
buffers record and possibly writes it to the remote logger More... | |
void | flush () |
write all currently buffered records ASAP More... | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (TcpLogger) | |
void | delayedReconnect () |
"sleep a little before trying to connect again" event callback More... | |
bool | canFit (const size_t len) const |
whether len more bytes can be buffered More... | |
void | appendRecord (const char *buf, size_t len) |
buffer a record that might exceed IoBufSize More... | |
void | appendChunk (const char *chunk, const size_t len) |
buffer a record chunk without splitting it across buffers More... | |
void | writeIfNeeded () |
starts writing if and only if it is time to write accumulated records More... | |
void | writeIfPossible () |
starts writing if possible More... | |
void | doConnect () |
starts [re]connecting to the remote logger More... | |
void | disconnect () |
close our connection now, without flushing More... | |
void | connectDone (const CommConnectCbParams &conn) |
Comm::ConnOpener callback. More... | |
void | writeDone (const CommIoCbParams &io) |
Comm::Write callback. More... | |
void | handleClosure (const CommCloseCbParams &io) |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | Flush (Logfile *lf) |
static void | WriteLine (Logfile *lf, const char *buf, size_t len) |
static void | StartLine (Logfile *lf) |
static void | EndLine (Logfile *lf) |
static void | Rotate (Logfile *lf, const int16_t) |
static void | Close (Logfile *lf) |
static TcpLogger * | StillLogging (Logfile *lf) |
static void | DelayedReconnect (void *data) |
Log::TcpLogger::delayedReconnect() wrapper. More... | |
Private Attributes | |
bool | dieOnError |
std::list< MemBlobPointer > | buffers |
I/O buffers. More... | |
size_t | bufferCapacity |
bufferedSize limit More... | |
size_t | bufferedSize |
number of log record bytes stored in RAM now More... | |
size_t | flushDebt |
how many record bytes we still need to write ASAP More... | |
bool | quitOnEmpty |
whether this job should quit when buffers are empty More... | |
bool | reconnectScheduled |
we are sleeping before the next connection attempt More... | |
bool | writeScheduled |
we are waiting for the latest write() results More... | |
Comm::ConnectionPointer | conn |
opened connection to the remote logger More... | |
Ip::Address | remote |
where the remote logger expects our records More... | |
AsyncCall::Pointer | closer |
handles unexpected/external conn closures More... | |
JobWait< Comm::ConnOpener > | connWait |
waits for a connection to the remote logger to be established/opened More... | |
uint64_t | connectFailures |
number of sequential connection failures More... | |
uint64_t | drops |
number of records dropped during the current outage More... | |
Static Private Attributes | |
static const size_t | IoBufSize = 2*MAX_URL |
fixed I/O buffer size More... | |
static const size_t | BufferCapacityMin = 2*Log::TcpLogger::IoBufSize |
minimum bufferCapacity value More... | |
Detailed Description
Sends log records to a remote TCP logger at the configured IP:port address. Handles loss of connectivity, record buffering, and buffer overflows.
Definition at line 33 of file TcpLogger.h.
Member Typedef Documentation
◆ Pointer
typedef CbcPointer<TcpLogger> Log::TcpLogger::Pointer |
Definition at line 38 of file TcpLogger.h.
Constructor & Destructor Documentation
◆ TcpLogger()
|
protected |
Definition at line 36 of file TcpLogger.cc.
References bufferCapacity, BufferCapacityMin, DBG_IMPORTANT, debugs, MY_DEBUG_SECTION, and remote.
◆ ~TcpLogger()
|
overrideprotected |
Definition at line 60 of file TcpLogger.cc.
References assert.
Member Function Documentation
◆ appendChunk()
|
private |
Definition at line 220 of file TcpLogger.cc.
References debugs, Must, and MY_DEBUG_SECTION.
◆ appendRecord()
|
private |
Definition at line 200 of file TcpLogger.cc.
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ canFit()
|
private |
Definition at line 158 of file TcpLogger.cc.
References DBG_CRITICAL, DBG_IMPORTANT, debugs, fatal(), and MY_DEBUG_SECTION.
◆ CBDATA_CHILD()
|
private |
◆ Close()
|
staticprivate |
Definition at line 435 of file TcpLogger.cc.
References asyncCall(), Logfile::data, debugs, endGracefully(), and ScheduleCallHere.
◆ connectDone()
|
private |
Definition at line 263 of file TcpLogger.cc.
References comm_add_close_handler(), CommCommonCbParams::conn, DBG_IMPORTANT, debugs, DelayedReconnect(), eventAdd(), CommCommonCbParams::flag, handleClosure(), JobCallback, Must, MY_DEBUG_SECTION, and Comm::OK.
Referenced by doConnect().
◆ delayedReconnect()
|
private |
◆ DelayedReconnect()
|
staticprivate |
Definition at line 304 of file TcpLogger.cc.
References assert, Logfile::data, delayedReconnect(), JobCallback, MY_DEBUG_SECTION, and ScheduleCallHere.
Referenced by connectDone().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ disconnect()
|
private |
Definition at line 381 of file TcpLogger.cc.
References comm_remove_close_handler().
◆ doConnect()
|
private |
Definition at line 241 of file TcpLogger.cc.
References connectDone(), debugs, Ip::Address::isIPv4(), JobCallback, Comm::Connection::local, Must, MY_DEBUG_SECTION, Comm::Connection::remote, Ip::Address::setAnyAddr(), Ip::Address::setIPv4(), and shutting_down.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 73 of file TcpLogger.cc.
References debugs, AsyncJob::doneAll(), and MY_DEBUG_SECTION.
◆ endGracefully()
|
protected |
Called when Squid is reconfiguring (or exiting) to give us a chance to flush remaining buffers and end this job w/o loss of data. No new log records are expected. Must be used as (or inside) an async job call and will result in [eventual] job termination.
Definition at line 102 of file TcpLogger.cc.
References assert.
Referenced by Close().
◆ EndLine()
|
staticprivate |
Definition at line 423 of file TcpLogger.cc.
References SquidConfig::buffered_logs, Config, and SquidConfig::onoff.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ flush()
|
protected |
Definition at line 111 of file TcpLogger.cc.
◆ Flush()
|
staticprivate |
Definition at line 404 of file TcpLogger.cc.
◆ handleClosure()
|
private |
This is our comm_close_handler. It is called when some external force (e.g., reconfigure or shutdown) is closing the connection (rather than us).
Definition at line 367 of file TcpLogger.cc.
References assert.
Referenced by connectDone().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ logRecord()
|
protected |
Definition at line 118 of file TcpLogger.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ Open()
Definition at line 452 of file TcpLogger.cc.
References assert, Logfile::data, DBG_IMPORTANT, debugs, Logfile::f_close, Logfile::f_flush, Logfile::f_lineend, Logfile::f_linestart, Logfile::f_linewrite, Logfile::f_rotate, FALSE, Logfile::fatal, fatalf(), Logfile::flags, GetHostWithPort(), Logfile::path, safe_free, AsyncJob::Start(), and xstrdup.
Referenced by logfileOpen(), and STUB_RETVAL().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ Rotate()
|
staticprivate |
Definition at line 430 of file TcpLogger.cc.
◆ start()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 67 of file TcpLogger.cc.
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ StartLine()
|
staticprivate |
Definition at line 418 of file TcpLogger.cc.
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ StillLogging()
|
staticprivate |
Converts Logfile into a pointer to a valid TcpLogger job or, if the logger job has quit, into a nill pointer
Definition at line 396 of file TcpLogger.cc.
References Logfile::data.
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 95 of file TcpLogger.cc.
References AsyncJob::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ writeDone()
|
private |
Definition at line 331 of file TcpLogger.cc.
References debugs, Comm::ERR_CLOSING, CommCommonCbParams::flag, Must, MY_DEBUG_SECTION, Comm::OK, MemBlob::size, CommIoCbParams::size, CommCommonCbParams::xerrno, and xstrerr().
Referenced by writeIfPossible().
◆ writeIfNeeded()
|
private |
Definition at line 126 of file TcpLogger.cc.
◆ writeIfPossible()
|
private |
Definition at line 135 of file TcpLogger.cc.
References debugs, fd_table, JobCallback, MemBlob::mem, MY_DEBUG_SECTION, MemBlob::size, Comm::Write(), and writeDone().
◆ WriteLine()
Definition at line 411 of file TcpLogger.cc.
Member Data Documentation
◆ bufferCapacity
|
private |
Definition at line 100 of file TcpLogger.h.
Referenced by TcpLogger().
◆ BufferCapacityMin
|
staticprivate |
Definition at line 92 of file TcpLogger.h.
Referenced by TcpLogger().
◆ bufferedSize
|
private |
Definition at line 101 of file TcpLogger.h.
◆ buffers
|
private |
Definition at line 99 of file TcpLogger.h.
◆ closer
|
private |
Definition at line 110 of file TcpLogger.h.
◆ conn
|
private |
Definition at line 108 of file TcpLogger.h.
◆ connectFailures
|
private |
Definition at line 115 of file TcpLogger.h.
◆ connWait
|
private |
Definition at line 113 of file TcpLogger.h.
◆ dieOnError
|
private |
Whether this job must kill Squid on the first unrecoverable error. Note that we may be able to recover from a failure to connect, but we cannot recover from forgetting (dropping) a record while connecting.
Definition at line 97 of file TcpLogger.h.
◆ drops
|
private |
Definition at line 116 of file TcpLogger.h.
◆ flushDebt
|
private |
Definition at line 102 of file TcpLogger.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ IoBufSize
Definition at line 91 of file TcpLogger.h.
◆ quitOnEmpty
|
private |
Definition at line 104 of file TcpLogger.h.
◆ reconnectScheduled
|
private |
Definition at line 105 of file TcpLogger.h.
◆ remote
|
private |
Definition at line 109 of file TcpLogger.h.
Referenced by TcpLogger().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ writeScheduled
|
private |
Definition at line 106 of file TcpLogger.h.
The documentation for this class was generated from the following files:
- src/log/TcpLogger.h
- src/log/TcpLogger.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products