#include <ConnOpener.h>
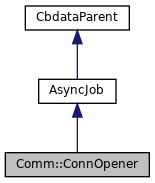
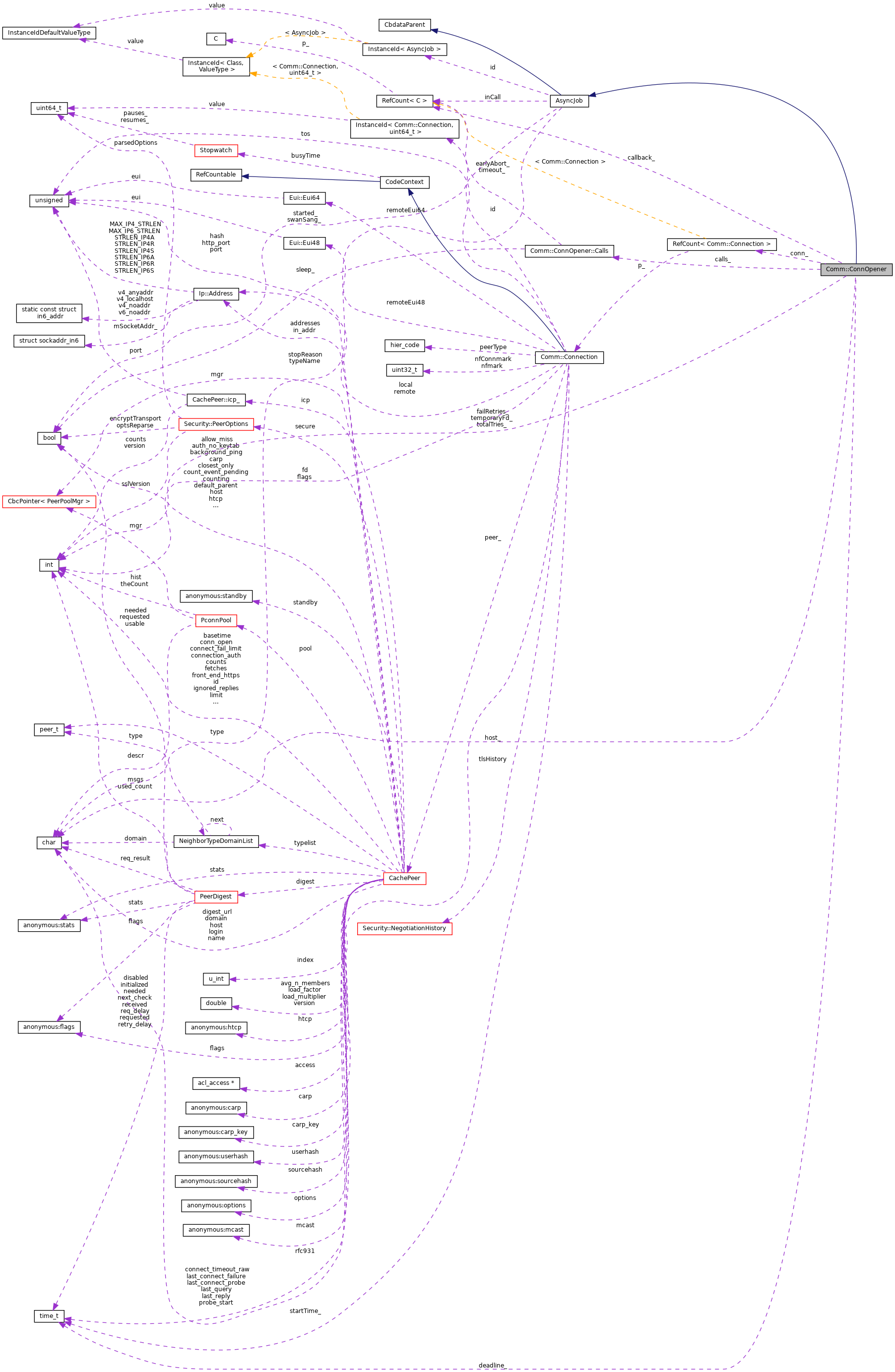
Classes | |
struct | Calls |
handles to calls which we may need to cancel. More... | |
Public Types | |
typedef CbcPointer< ConnOpener > | Pointer |
Public Member Functions | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
ConnOpener (const Comm::ConnectionPointer &, const AsyncCall::Pointer &handler, time_t connect_timeout) | |
~ConnOpener () override | |
void | setHost (const char *) |
set the hostname note for this connection More... | |
const char * | getHost () const |
get the hostname noted for this connection More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | start () override |
called by AsyncStart; do not call directly More... | |
void | swanSong () override |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (ConnOpener) | |
ConnOpener (const ConnOpener &) | |
ConnOpener & | operator= (const ConnOpener &c) |
void | earlyAbort (const CommCloseCbParams &) |
void | timeout (const CommTimeoutCbParams &) |
void | sendAnswer (Comm::Flag errFlag, int xerrno, const char *why) |
void | doConnect () |
Make an FD connection attempt. More... | |
void | connected () |
void | lookupLocalAddress () |
void | retrySleep () |
Close and wait a little before trying to open and connect again. More... | |
void | restart () |
called at the end of Comm::ConnOpener::DelayedConnectRetry event More... | |
bool | createFd () |
void | closeFd () |
cleans I/O state and ends I/O for temporaryFd_ More... | |
void | keepFd () |
cleans I/O state and moves temporaryFd_ to the conn_ for long-term use More... | |
void | cleanFd () |
void | cancelSleep () |
cleans up this job sleep state More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | InProgressConnectRetry (int fd, void *data) |
static void | DelayedConnectRetry (void *data) |
Private Attributes | |
char * | host_ |
domain name we are trying to connect to. More... | |
int | temporaryFd_ |
the FD being opened. Do NOT set conn_->fd until it is fully open. More... | |
Comm::ConnectionPointer | conn_ |
single connection currently to be opened. More... | |
AsyncCall::Pointer | callback_ |
handler to be called on connection completion. More... | |
int | totalTries_ |
total number of connection attempts over all destinations so far. More... | |
int | failRetries_ |
number of retries current destination has been tried. More... | |
time_t | deadline_ |
if we are not done by then, we will call back with Comm::TIMEOUT More... | |
struct Comm::ConnOpener::Calls | calls_ |
Detailed Description
Asynchronously opens a TCP connection. Returns CommConnectCbParams: either Comm::OK with an open connection or another Comm::Flag with a closed one.
Definition at line 24 of file ConnOpener.h.
Member Typedef Documentation
◆ Pointer
Definition at line 29 of file ConnOpener.h.
Constructor & Destructor Documentation
◆ ConnOpener() [1/2]
Comm::ConnOpener::ConnOpener | ( | const Comm::ConnectionPointer & | c, |
const AsyncCall::Pointer & | handler, | ||
time_t | connect_timeout | ||
) |
Definition at line 32 of file ConnOpener.cc.
References assert, conn_, debugs, and Comm::Connection::isOpen().
◆ ~ConnOpener()
|
override |
Definition at line 53 of file ConnOpener.cc.
References safe_free.
◆ ConnOpener() [2/2]
|
private |
Member Function Documentation
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cancelSleep()
|
private |
Definition at line 409 of file ConnOpener.cc.
References debugs.
◆ CBDATA_CHILD()
|
private |
◆ cleanFd()
|
private |
cleans up this job I/O state without closing temporaryFd required before closing temporaryFd or keeping it in conn_ leaves FD bare so must only be called via closeFd() or keepFd()
Definition at line 173 of file ConnOpener.cc.
References comm_remove_close_handler(), COMM_SELECT_WRITE, debugs, fd_table, Must, Comm::SetSelect(), fde::timeout, fde::timeoutHandler, fde::write_data, and fde::write_handler.
◆ closeFd()
|
private |
Definition at line 219 of file ConnOpener.cc.
References comm_close.
◆ connected()
|
private |
Definition at line 327 of file ConnOpener.cc.
◆ createFd()
|
private |
Create a socket for the future connection or return false. If false is returned, done() is guaranteed to return true and end the job.
Definition at line 278 of file ConnOpener.cc.
References assert, comm_add_close_handler(), comm_open(), debugs, earlyAbort(), Comm::ERR_CONNECT, fd_table, CachePeer::flags, JobCallback, Must, CachePeer::params, Ip::Qos::setSockNfmark(), Ip::Qos::setSockTos(), squid_curtime, Squid_MaxFD, and timeout().
◆ DelayedConnectRetry()
|
staticprivate |
Definition at line 490 of file ConnOpener.cc.
References assert, JobCallback, restart(), and ScheduleCallHere.
Referenced by retrySleep().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ doConnect()
|
private |
Definition at line 356 of file ConnOpener.cc.
References comm_connect_addr(), COMM_SELECT_WRITE, Config, SquidConfig::connect_retries, debugs, Comm::ERR_CONNECT, Comm::INPROGRESS, InProgressConnectRetry(), Must, Comm::OK, Comm::SetSelect(), and xstrerr().
Referenced by InProgressConnectRetry().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 59 of file ConnOpener.cc.
References AsyncJob::doneAll(), and Must.
◆ earlyAbort()
|
private |
Abort connection attempt. Handles the case(s) when a partially setup connection gets closed early.
Definition at line 448 of file ConnOpener.cc.
References CommCommonCbParams::conn, debugs, Comm::ERR_CLOSING, and CommCommonCbParams::xerrno.
Referenced by createFd().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ getHost()
const char * Comm::ConnOpener::getHost | ( | ) | const |
Definition at line 112 of file ConnOpener.cc.
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ InProgressConnectRetry()
|
staticprivate |
Definition at line 472 of file ConnOpener.cc.
References assert, doConnect(), JobCallback, and ScheduleCallHere.
Referenced by doConnect().
◆ keepFd()
|
private |
Definition at line 238 of file ConnOpener.cc.
References Must.
◆ lookupLocalAddress()
|
private |
Lookup local-end address and port of the TCP link just opened. This ensure the connection local details are set correctly
Definition at line 430 of file ConnOpener.cc.
References DBG_IMPORTANT, debugs, and xstrerr().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ operator=()
|
private |
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ restart()
|
private |
Definition at line 266 of file ConnOpener.cc.
References debugs.
Referenced by DelayedConnectRetry().
◆ retrySleep()
|
private |
Definition at line 397 of file ConnOpener.cc.
References DelayedConnectRetry(), eventAdd(), and Must.
◆ sendAnswer()
|
private |
Connection attempt are completed. One way or the other. Pass the results back to the external handler.
Definition at line 122 of file ConnOpener.cc.
References assert, Config, debugs, ipcacheMarkBadAddr(), ipcacheMarkGoodAddr(), netdbDeleteAddrNetwork(), Comm::OK, SquidConfig::onoff, CachePeer::params, ScheduleCallHere, and SquidConfig::test_reachability.
◆ setHost()
void Comm::ConnOpener::setHost | ( | const char * | new_host | ) |
Definition at line 100 of file ConnOpener.cc.
References safe_free, and xstrdup.
Referenced by idnsInitVC(), and peerProbeConnect().
◆ start()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 250 of file ConnOpener.cc.
References Ip::EnableIpv6, IPV6_SPECIAL_V4MAPPING, and Must.
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 77 of file ConnOpener.cc.
References Comm::ERR_CONNECT, and AsyncJob::swanSong().
◆ timeout()
|
private |
Handles the case(s) when a partially setup connection gets timed out. NP: When commSetConnTimeout accepts generic CommCommonCbParams this can die.
Definition at line 461 of file ConnOpener.cc.
References debugs, and Comm::TIMEOUT.
Referenced by createFd().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
Member Data Documentation
◆ callback_
|
private |
Definition at line 71 of file ConnOpener.h.
◆ calls_
|
private |
◆ conn_
|
private |
Definition at line 70 of file ConnOpener.h.
Referenced by ConnOpener().
◆ deadline_
|
private |
Definition at line 77 of file ConnOpener.h.
◆ failRetries_
|
private |
Definition at line 74 of file ConnOpener.h.
◆ host_
|
private |
Definition at line 68 of file ConnOpener.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ temporaryFd_
|
private |
Definition at line 69 of file ConnOpener.h.
◆ totalTries_
|
private |
Definition at line 73 of file ConnOpener.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
The documentation for this class was generated from the following files:
- src/comm/ConnOpener.h
- src/comm/ConnOpener.cc
- src/tests/stub_libcomm.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products