#include <CachePeer.h>
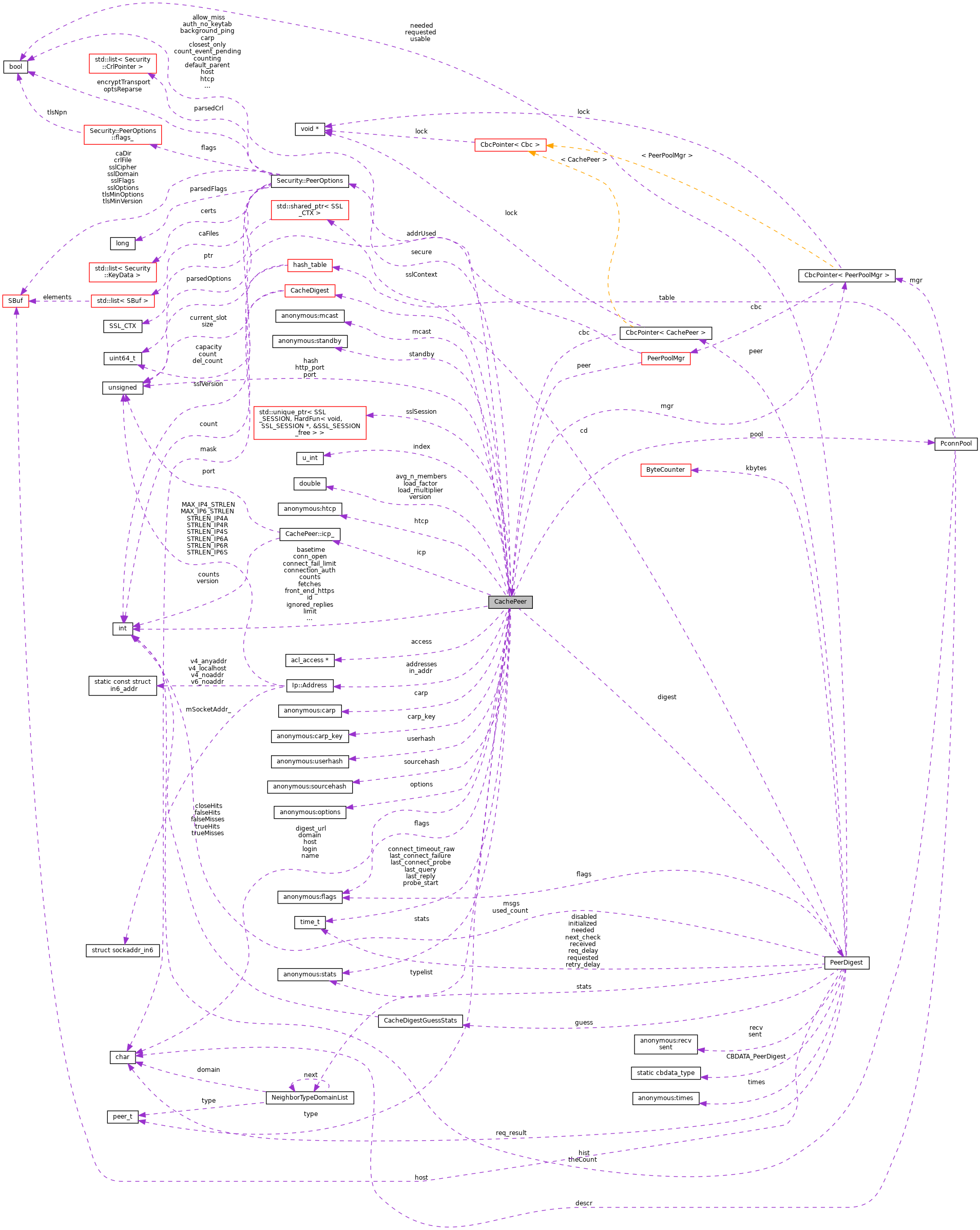
Classes | |
struct | icp_ |
Public Member Functions | |
CachePeer (const char *hostname) | |
~CachePeer () | |
void | noteSuccess () |
reacts to a successful establishment of a connection to this cache_peer More... | |
void | noteFailure () |
reacts to a failed attempt to establish a connection to this cache_peer More... | |
void | rename (const char *) |
(re)configure cache_peer name=value More... | |
time_t | connectTimeout () const |
Security::FuturePeerContext * | securityContext () |
Public Attributes | |
u_int | index = 0 |
n-th cache_peer directive, starting with 1 More... | |
char * | name = nullptr |
char * | host = nullptr |
peer_t | type = PEER_NONE |
Ip::Address | in_addr |
struct { | |
int pings_sent = 0 | |
int pings_acked = 0 | |
int fetches = 0 | |
int rtt = 0 | |
int ignored_replies = 0 | |
int n_keepalives_sent = 0 | |
int n_keepalives_recv = 0 | |
time_t probe_start = 0 | |
time_t last_query = 0 | |
time_t last_reply = 0 | |
time_t last_connect_failure = 0 | |
time_t last_connect_probe = 0 | |
int logged_state = PEER_ALIVE | |
so we can print dead/revived msgs More... | |
int conn_open = 0 | |
current opened connections More... | |
} | stats |
struct CachePeer::icp_ | icp |
struct { | |
double version = 0.0 | |
int counts [2] = {0, 0} | |
unsigned short port = 0 | |
} | htcp |
unsigned short | http_port = CACHE_HTTP_PORT |
NeighborTypeDomainList * | typelist = nullptr |
acl_access * | access = nullptr |
struct { | |
bool proxy_only = false | |
bool no_query = false | |
bool background_ping = false | |
bool no_digest = false | |
bool default_parent = false | |
bool roundrobin = false | |
bool weighted_roundrobin = false | |
bool mcast_responder = false | |
bool closest_only = false | |
bool htcp = false | |
bool htcp_oldsquid = false | |
bool htcp_no_clr = false | |
bool htcp_no_purge_clr = false | |
bool htcp_only_clr = false | |
bool htcp_forward_clr = false | |
bool no_netdb_exchange = false | |
bool no_delay = false | |
bool allow_miss = false | |
bool carp = false | |
struct { | |
bool set = false | |
bool scheme = false | |
bool host = false | |
bool port = false | |
bool path = false | |
bool params = false | |
} carp_key | |
bool userhash = false | |
bool sourcehash = false | |
bool originserver = false | |
bool no_tproxy = false | |
bool mcast_siblings = false | |
bool auth_no_keytab = false | |
} | options |
int | weight = 1 |
int | basetime = 0 |
struct { | |
double avg_n_members = 0.0 | |
int n_times_counted = 0 | |
int n_replies_expected = 0 | |
int ttl = 0 | |
int id = 0 | |
struct { | |
bool count_event_pending = false | |
bool counting = false | |
} flags | |
} | mcast |
PeerDigest * | digest = nullptr |
char * | digest_url = nullptr |
int | tcp_up = 0 |
bool | reprobe = false |
whether to do another TCP probe after current TCP probes More... | |
Ip::Address | addresses [10] |
int | n_addresses = 0 |
int | rr_count = 0 |
int | testing_now = 0 |
struct { | |
unsigned int hash = 0 | |
double load_multiplier = 0.0 | |
double load_factor = 0.0 | |
normalized weight value More... | |
} | carp |
struct { | |
unsigned int hash = 0 | |
double load_multiplier = 0.0 | |
double load_factor = 0.0 | |
normalized weight value More... | |
} | userhash |
struct { | |
unsigned int hash = 0 | |
double load_multiplier = 0.0 | |
double load_factor = 0.0 | |
normalized weight value More... | |
} | sourcehash |
char * | login = nullptr |
time_t | connect_timeout_raw = 0 |
connect_timeout; use connectTimeout() instead! More... | |
int | connect_fail_limit = 0 |
int | max_conn = 0 |
struct { | |
PconnPool * pool = nullptr | |
idle connection pool for this peer More... | |
CbcPointer< PeerPoolMgr > mgr | |
pool manager More... | |
int limit = 0 | |
the limit itself More... | |
bool waitingForClose = false | |
a conn must close before we open a standby conn More... | |
} | standby |
optional "cache_peer standby=limit" feature More... | |
char * | domain = nullptr |
Forced domain. More... | |
Security::PeerOptions | secure |
security settings for peer connection More... | |
Security::ContextPointer | sslContext |
Security::FuturePeerContext | tlsContext |
Security::SessionStatePointer | sslSession |
int | front_end_https = 0 |
0 - off, 1 - on, 2 - auto More... | |
int | connection_auth = 2 |
0 - off, 1 - on, 2 - auto More... | |
PrecomputedCodeContextPointer | probeCodeContext |
Private Member Functions | |
CBDATA_CLASS (CachePeer) | |
void | countFailure () |
Detailed Description
Definition at line 28 of file CachePeer.h.
Constructor & Destructor Documentation
◆ CachePeer()
|
explicit |
Definition at line 25 of file CachePeer.cc.
◆ ~CachePeer()
CachePeer::~CachePeer | ( | ) |
Definition at line 34 of file CachePeer.cc.
References access, aclDestroyAccessList(), PeerPoolMgr::Checkpoint(), digest, digest_url, domain, host, login, name, NeighborTypeDomainList::next, standby, typelist, and xfree.
Member Function Documentation
◆ CBDATA_CLASS()
|
private |
◆ connectTimeout()
time_t CachePeer::connectTimeout | ( | ) | const |
- Returns
- the effective connect timeout for the given peer
Definition at line 120 of file CachePeer.cc.
References Config, connect_timeout_raw, SquidConfig::peer_connect, and SquidConfig::Timeout.
Referenced by Comm::Connection::connectTimeout(), PeerPoolMgr::handleOpenedConnection(), PeerPoolMgr::openNewConnection(), and peerProbeConnect().
◆ countFailure()
|
private |
◆ noteFailure()
void CachePeer::noteFailure | ( | ) |
noteFailure() helper for handling failures attributed to this peer
Definition at line 86 of file CachePeer.cc.
References assert, DBG_IMPORTANT, debugs, name, neighborTypeStr(), PEER_ALIVE, PEER_DEAD, squid_curtime, stats, and tcp_up.
Referenced by NoteOutgoingConnectionFailure(), and peerProbeConnectDone().
◆ noteSuccess()
void CachePeer::noteSuccess | ( | ) |
Definition at line 71 of file CachePeer.cc.
References connect_fail_limit, debugs, peerAlive(), and tcp_up.
Referenced by NoteOutgoingConnectionSuccess(), and peerProbeConnectDone().
◆ rename()
void CachePeer::rename | ( | const char * | newName | ) |
◆ securityContext()
Security::FuturePeerContext * CachePeer::securityContext | ( | ) |
TLS settings for communicating with this TLS cache_peer (if encryption is required; see secure.encryptTransport) or nil (otherwise)
Definition at line 63 of file CachePeer.cc.
References Security::PeerOptions::encryptTransport, secure, and tlsContext.
Referenced by Security::BlindPeerConnector::peerContext().
Member Data Documentation
◆ access
acl_access* CachePeer::access = nullptr |
Definition at line 106 of file CachePeer.h.
Referenced by peerAllowedToUse(), and ~CachePeer().
◆ addresses
Ip::Address CachePeer::addresses[10] |
Definition at line 179 of file CachePeer.h.
Referenced by PeerPoolMgr::openNewConnection(), peerDNSConfigure(), and peerProbeConnect().
◆ allow_miss
bool CachePeer::allow_miss = false |
Definition at line 130 of file CachePeer.h.
Referenced by dump_peer_options(), and HttpStateData::sendRequest().
◆ auth_no_keytab
bool CachePeer::auth_no_keytab = false |
Definition at line 147 of file CachePeer.h.
Referenced by HttpRequest::prepForPeering().
◆ avg_n_members
double CachePeer::avg_n_members = 0.0 |
Definition at line 154 of file CachePeer.h.
Referenced by peerCountMcastPeersAbort().
◆ background_ping
bool CachePeer::background_ping = false |
Definition at line 111 of file CachePeer.h.
Referenced by dump_peer_options(), and peerWouldBePinged().
◆ basetime
int CachePeer::basetime = 0 |
Definition at line 151 of file CachePeer.h.
Referenced by getWeightedRoundRobinParent(), PeerSelector::handleHtcpParentMiss(), and PeerSelector::handleIcpParentMiss().
◆ carp [1/2]
bool CachePeer::carp = false |
Definition at line 131 of file CachePeer.h.
Referenced by dump_peer_options().
◆ carp [2/2]
struct { ... } CachePeer::carp |
◆ carp_key
struct { ... } CachePeer::carp_key |
◆ closest_only
bool CachePeer::closest_only = false |
Definition at line 117 of file CachePeer.h.
Referenced by dump_peer_options(), PeerSelector::handleHtcpParentMiss(), and PeerSelector::handleIcpParentMiss().
◆ conn_open
int CachePeer::conn_open = 0 |
Definition at line 86 of file CachePeer.h.
Referenced by peerCanOpenMore(), and peerConnClosed().
◆ connect_fail_limit
int CachePeer::connect_fail_limit = 0 |
Definition at line 204 of file CachePeer.h.
Referenced by dump_peer_options(), and noteSuccess().
◆ connect_timeout_raw
time_t CachePeer::connect_timeout_raw = 0 |
Definition at line 203 of file CachePeer.h.
Referenced by connectTimeout(), and dump_peer_options().
◆ connection_auth
int CachePeer::connection_auth = 2 |
Definition at line 226 of file CachePeer.h.
Referenced by dump_peer_options(), and HttpStateData::peerSupportsConnectionPinning().
◆ count_event_pending
bool CachePeer::count_event_pending = false |
Definition at line 161 of file CachePeer.h.
◆ counting
bool CachePeer::counting = false |
Definition at line 162 of file CachePeer.h.
◆ counts
int CachePeer::counts[2] = {0, 0} |
Definition at line 99 of file CachePeer.h.
Referenced by neighborAliveHtcp().
◆ default_parent
bool CachePeer::default_parent = false |
Definition at line 113 of file CachePeer.h.
Referenced by dump_peer_options().
◆ digest
PeerDigest* CachePeer::digest = nullptr |
Definition at line 167 of file CachePeer.h.
Referenced by peerDigestLookup(), and ~CachePeer().
◆ digest_url
char* CachePeer::digest_url = nullptr |
Definition at line 168 of file CachePeer.h.
Referenced by dump_peer_options(), and ~CachePeer().
◆ domain
char* CachePeer::domain = nullptr |
Definition at line 215 of file CachePeer.h.
Referenced by dump_peer_options(), HttpRequest::prepForPeering(), and ~CachePeer().
◆ fetches
int CachePeer::fetches = 0 |
Definition at line 75 of file CachePeer.h.
Referenced by snmp_meshPtblFn().
◆ flags
struct { ... } CachePeer::flags |
◆ front_end_https
int CachePeer::front_end_https = 0 |
Definition at line 225 of file CachePeer.h.
Referenced by HttpStateData::sendRequest().
◆ hash
unsigned int CachePeer::hash = 0 |
Definition at line 185 of file CachePeer.h.
◆ host [1/2]
char* CachePeer::host = nullptr |
The lowercase version of the configured cache_peer hostname. May not be unique among cache_peers. Never nil.
Definition at line 66 of file CachePeer.h.
Referenced by CachePeer(), TunnelStateData::getHost(), httpFixupAuthentication(), netdbExchangeStart(), netdbExchangeUpdatePeer(), netdbPeerAdd(), netdbUpdatePeer(), PeerSelector::noteIps(), peerAlive(), peerNoteDigestLookup(), PeerPoolMgr::PeerPoolMgr(), peerProbeConnect(), PeerSelector::resolveSelected(), snmp_meshPtblFn(), and ~CachePeer().
◆ host [2/2]
bool CachePeer::host = false |
Definition at line 135 of file CachePeer.h.
◆ htcp [1/2]
struct { ... } CachePeer::htcp |
Referenced by dump_peer_options(), and neighborAliveHtcp().
◆ htcp [2/2]
bool CachePeer::htcp = false |
Definition at line 119 of file CachePeer.h.
◆ htcp_forward_clr
bool CachePeer::htcp_forward_clr = false |
Definition at line 124 of file CachePeer.h.
Referenced by dump_peer_options().
◆ htcp_no_clr
bool CachePeer::htcp_no_clr = false |
Definition at line 121 of file CachePeer.h.
Referenced by dump_peer_options().
◆ htcp_no_purge_clr
bool CachePeer::htcp_no_purge_clr = false |
Definition at line 122 of file CachePeer.h.
Referenced by dump_peer_options().
◆ htcp_oldsquid
bool CachePeer::htcp_oldsquid = false |
Definition at line 120 of file CachePeer.h.
Referenced by dump_peer_options(), htcpClear(), and htcpQuery().
◆ htcp_only_clr
bool CachePeer::htcp_only_clr = false |
Definition at line 123 of file CachePeer.h.
Referenced by dump_peer_options().
◆ http_port
unsigned short CachePeer::http_port = CACHE_HTTP_PORT |
Definition at line 104 of file CachePeer.h.
Referenced by netdbExchangeStart(), PeerPoolMgr::openNewConnection(), peerAllowedToUse(), peerProbeConnect(), and snmp_meshPtblFn().
◆ icp
struct CachePeer::icp_ CachePeer::icp |
Referenced by neighborAlive(), neighborsUdpAck(), peerDNSConfigure(), peerWouldBePinged(), and snmp_meshPtblFn().
◆ id
int CachePeer::id = 0 |
Definition at line 158 of file CachePeer.h.
Referenced by peerCountMcastPeersCreateAndSend().
◆ ignored_replies
int CachePeer::ignored_replies = 0 |
Definition at line 77 of file CachePeer.h.
Referenced by neighborCountIgnored(), and snmp_meshPtblFn().
◆ in_addr
Ip::Address CachePeer::in_addr |
Definition at line 70 of file CachePeer.h.
Referenced by PeerSelector::handleHtcpParentMiss(), PeerSelector::handleIcpParentMiss(), htcpClear(), htcpQuery(), peerCountMcastPeersCreateAndSend(), and peerDNSConfigure().
◆ index
u_int CachePeer::index = 0 |
Definition at line 53 of file CachePeer.h.
Referenced by snmp_meshPtblFn().
◆ last_connect_failure
time_t CachePeer::last_connect_failure = 0 |
Definition at line 83 of file CachePeer.h.
◆ last_connect_probe
time_t CachePeer::last_connect_probe = 0 |
Definition at line 84 of file CachePeer.h.
Referenced by peerProbeConnect(), and peerProbeIsBusy().
◆ last_query
time_t CachePeer::last_query = 0 |
Definition at line 81 of file CachePeer.h.
Referenced by peerWouldBePinged().
◆ last_reply
time_t CachePeer::last_reply = 0 |
Definition at line 82 of file CachePeer.h.
Referenced by peerAlive().
◆ limit
int CachePeer::limit = 0 |
Definition at line 211 of file CachePeer.h.
Referenced by PeerPoolMgr::checkpoint(), PeerPoolMgr::doneAll(), and dump_peer_options().
◆ load_factor
double CachePeer::load_factor = 0.0 |
Definition at line 187 of file CachePeer.h.
◆ load_multiplier
double CachePeer::load_multiplier = 0.0 |
Definition at line 186 of file CachePeer.h.
◆ logged_state
int CachePeer::logged_state = PEER_ALIVE |
Definition at line 85 of file CachePeer.h.
Referenced by peerAlive().
◆ login
char* CachePeer::login = nullptr |
Definition at line 202 of file CachePeer.h.
Referenced by dump_peer_options(), netdbExchangeStart(), HttpRequest::prepForPeering(), and ~CachePeer().
◆ max_conn
int CachePeer::max_conn = 0 |
Definition at line 205 of file CachePeer.h.
Referenced by dump_peer_options(), and peerCanOpenMore().
◆ mcast
struct { ... } CachePeer::mcast |
◆ mcast_responder
bool CachePeer::mcast_responder = false |
Definition at line 116 of file CachePeer.h.
Referenced by dump_peer_options(), ignoreMulticastReply(), and peerWouldBePinged().
◆ mcast_siblings
bool CachePeer::mcast_siblings = false |
Definition at line 146 of file CachePeer.h.
Referenced by dump_peer_options(), neighborType(), and peerAllowedToUse().
◆ mgr
CbcPointer<PeerPoolMgr> CachePeer::mgr |
Definition at line 210 of file CachePeer.h.
Referenced by peerAlive(), peerConnClosed(), and peerDNSConfigure().
◆ n_addresses
int CachePeer::n_addresses = 0 |
Definition at line 180 of file CachePeer.h.
Referenced by neighborUp(), PeerPoolMgr::openNewConnection(), peerAlive(), peerDNSConfigure(), peerProbeConnect(), and peerWouldBePinged().
◆ n_keepalives_recv
int CachePeer::n_keepalives_recv = 0 |
Definition at line 79 of file CachePeer.h.
Referenced by HttpStateData::keepaliveAccounting(), HttpStateData::sendRequest(), and snmp_meshPtblFn().
◆ n_keepalives_sent
int CachePeer::n_keepalives_sent = 0 |
Definition at line 78 of file CachePeer.h.
Referenced by HttpStateData::keepaliveAccounting(), HttpStateData::sendRequest(), and snmp_meshPtblFn().
◆ n_replies_expected
int CachePeer::n_replies_expected = 0 |
Definition at line 156 of file CachePeer.h.
Referenced by peerCountMcastPeersAbort().
◆ n_times_counted
int CachePeer::n_times_counted = 0 |
Definition at line 155 of file CachePeer.h.
Referenced by peerCountMcastPeersAbort().
◆ name
char* CachePeer::name = nullptr |
cache_peer name (if explicitly configured) or hostname (otherwise). Unique across already configured cache_peers in the current configuration. The value may change during CachePeer configuration. The value affects various peer selection hashes (e.g., carp.hash). Preserves configured spelling (i.e. does not lower letters case). Never nil.
Definition at line 61 of file CachePeer.h.
Referenced by assembleVaryKey(), GetNfmarkToServer(), getOutgoingAddress(), GetTosToServer(), noteFailure(), operator<<(), parse_peer_access(), rename(), HierarchyLogEntry::resetPeerNotes(), and ~CachePeer().
◆ no_delay
bool CachePeer::no_delay = false |
Definition at line 128 of file CachePeer.h.
Referenced by TunnelStateData::connectDone(), dump_peer_options(), FwdState::establishTunnelThruProxy(), HttpStateData::HttpStateData(), and switchToTunnel().
◆ no_digest
bool CachePeer::no_digest = false |
Definition at line 112 of file CachePeer.h.
Referenced by dump_peer_options().
◆ no_netdb_exchange
bool CachePeer::no_netdb_exchange = false |
Definition at line 126 of file CachePeer.h.
Referenced by dump_peer_options(), and peerDNSConfigure().
◆ no_query
bool CachePeer::no_query = false |
Definition at line 110 of file CachePeer.h.
Referenced by dump_peer_options(), neighborUp(), and peerWouldBePinged().
◆ no_tproxy
bool CachePeer::no_tproxy = false |
Definition at line 145 of file CachePeer.h.
Referenced by dump_peer_options(), and getOutgoingAddress().
◆ options
struct { ... } CachePeer::options |
Referenced by TunnelStateData::connectDone(), dump_peer_options(), FwdState::establishTunnelThruProxy(), getOutgoingAddress(), PeerSelector::handleHtcpParentMiss(), PeerSelector::handleIcpParentMiss(), htcpClear(), htcpQuery(), HttpStateData::HttpStateData(), ignoreMulticastReply(), neighborType(), neighborUp(), neighborUpdateRtt(), peerAllowedToUse(), peerCountHandleIcpReply(), peerDNSConfigure(), HttpStateData::peerSupportsConnectionPinning(), peerWouldBePinged(), HttpRequest::prepForPeering(), HttpStateData::sendRequest(), and switchToTunnel().
◆ originserver
bool CachePeer::originserver = false |
Definition at line 144 of file CachePeer.h.
Referenced by dump_peer_options(), HttpStateData::HttpStateData(), peerAllowedToUse(), and HttpStateData::peerSupportsConnectionPinning().
◆ params
bool CachePeer::params = false |
Definition at line 138 of file CachePeer.h.
Referenced by Comm::ConnOpener::createFd(), HttpStateData::httpStateConnClosed(), and Comm::ConnOpener::sendAnswer().
◆ path
bool CachePeer::path = false |
Definition at line 137 of file CachePeer.h.
Referenced by unlinkdUnlink().
◆ pings_acked
int CachePeer::pings_acked = 0 |
Definition at line 74 of file CachePeer.h.
Referenced by neighborAlive(), neighborAliveHtcp(), neighborsUdpAck(), neighborUpdateRtt(), and snmp_meshPtblFn().
◆ pings_sent
int CachePeer::pings_sent = 0 |
Definition at line 73 of file CachePeer.h.
Referenced by snmp_meshPtblFn().
◆ pool
PconnPool* CachePeer::pool = nullptr |
Definition at line 209 of file CachePeer.h.
Referenced by PeerPoolMgr::checkpoint(), PeerPoolMgr::closeOldConnections(), peerHasConnAvailable(), PeerPoolMgr::pushNewConnection(), and PeerPoolMgr::validPeer().
◆ port [1/2]
unsigned short CachePeer::port = 0 |
Definition at line 100 of file CachePeer.h.
◆ port [2/2]
bool CachePeer::port = false |
Definition at line 136 of file CachePeer.h.
◆ probe_start
time_t CachePeer::probe_start = 0 |
Definition at line 80 of file CachePeer.h.
Referenced by neighborUp(), and peerAlive().
◆ probeCodeContext
PrecomputedCodeContextPointer CachePeer::probeCodeContext |
Definition at line 228 of file CachePeer.h.
Referenced by neighborUp().
◆ proxy_only
bool CachePeer::proxy_only = false |
Definition at line 109 of file CachePeer.h.
Referenced by dump_peer_options(), and HttpStateData::HttpStateData().
◆ reprobe
bool CachePeer::reprobe = false |
Definition at line 177 of file CachePeer.h.
Referenced by peerProbeConnect(), and peerProbeConnectDone().
◆ roundrobin
bool CachePeer::roundrobin = false |
Definition at line 114 of file CachePeer.h.
Referenced by dump_peer_options().
◆ rr_count
int CachePeer::rr_count = 0 |
Definition at line 181 of file CachePeer.h.
Referenced by getRoundRobinParent(), and getWeightedRoundRobinParent().
◆ rtt
int CachePeer::rtt = 0 |
Definition at line 76 of file CachePeer.h.
Referenced by getWeightedRoundRobinParent(), neighborUpdateRtt(), peerCountHandleIcpReply(), peerCountMcastPeersAbort(), and snmp_meshPtblFn().
◆ scheme
bool CachePeer::scheme = false |
Definition at line 134 of file CachePeer.h.
◆ secure
Security::PeerOptions CachePeer::secure |
Definition at line 219 of file CachePeer.h.
Referenced by dump_peer_options(), PeerPoolMgr::handleOpenedConnection(), Security::BlindPeerConnector::initialize(), netdbExchangeStart(), FwdState::secureConnectionToPeerIfNeeded(), and securityContext().
◆ set
bool CachePeer::set = false |
Definition at line 133 of file CachePeer.h.
◆ sourcehash [1/2]
bool CachePeer::sourcehash = false |
Definition at line 143 of file CachePeer.h.
Referenced by dump_peer_options().
◆ sourcehash [2/2]
struct { ... } CachePeer::sourcehash |
◆ sslContext
Security::ContextPointer CachePeer::sslContext |
Definition at line 220 of file CachePeer.h.
◆ sslSession
Security::SessionStatePointer CachePeer::sslSession |
Definition at line 223 of file CachePeer.h.
Referenced by Security::BlindPeerConnector::initialize().
◆ standby
struct { ... } CachePeer::standby |
Referenced by PeerPoolMgr::checkpoint(), PeerPoolMgr::closeOldConnections(), PeerPoolMgr::doneAll(), dump_peer_options(), PeerPoolMgr::openNewConnection(), peerAlive(), peerConnClosed(), peerDNSConfigure(), peerHasConnAvailable(), PeerPoolMgr::pushNewConnection(), PeerPoolMgr::validPeer(), and ~CachePeer().
◆ stats
struct { ... } CachePeer::stats |
Referenced by getWeightedRoundRobinParent(), HttpStateData::keepaliveAccounting(), neighborAlive(), neighborAliveHtcp(), neighborCountIgnored(), neighborsUdpAck(), neighborUp(), neighborUpdateRtt(), noteFailure(), peerAlive(), peerCanOpenMore(), peerConnClosed(), peerCountHandleIcpReply(), peerCountMcastPeersAbort(), peerProbeConnect(), peerProbeIsBusy(), peerWouldBePinged(), HttpStateData::sendRequest(), and snmp_meshPtblFn().
◆ tcp_up
int CachePeer::tcp_up = 0 |
The number of failures sufficient to stop selecting this cache_peer. All cache_peer selection algorithms skip cache_peers with 0 tcp_up values. The initial 0 value prevents unprobed cache_peers from being selected.
Definition at line 174 of file CachePeer.h.
Referenced by neighborUp(), noteFailure(), noteSuccess(), and peerAlive().
◆ testing_now
int CachePeer::testing_now = 0 |
Definition at line 182 of file CachePeer.h.
Referenced by peerProbeConnect(), peerProbeConnectDone(), and peerProbeIsBusy().
◆ tlsContext
Security::FuturePeerContext CachePeer::tlsContext |
Definition at line 221 of file CachePeer.h.
Referenced by securityContext().
◆ ttl
int CachePeer::ttl = 0 |
Definition at line 157 of file CachePeer.h.
Referenced by dump_peer_options(), peerCountMcastPeersCreateAndSend(), and peerDNSConfigure().
◆ type
Definition at line 68 of file CachePeer.h.
Referenced by neighborType(), neighborTypeStr(), peerAllowedToUse(), peerCountMcastPeersCreateAndSend(), peerDNSConfigure(), peerWouldBePinged(), and snmp_meshPtblFn().
◆ typelist
NeighborTypeDomainList* CachePeer::typelist = nullptr |
Definition at line 105 of file CachePeer.h.
Referenced by neighborType(), and ~CachePeer().
◆ userhash [1/2]
bool CachePeer::userhash = false |
Definition at line 141 of file CachePeer.h.
Referenced by dump_peer_options().
◆ userhash [2/2]
struct { ... } CachePeer::userhash |
◆ version
double CachePeer::version = 0.0 |
Definition at line 98 of file CachePeer.h.
Referenced by neighborAliveHtcp().
◆ waitingForClose
bool CachePeer::waitingForClose = false |
Definition at line 212 of file CachePeer.h.
Referenced by PeerPoolMgr::openNewConnection(), and peerConnClosed().
◆ weight
int CachePeer::weight = 1 |
Definition at line 150 of file CachePeer.h.
Referenced by dump_peer_options(), getRoundRobinParent(), getWeightedRoundRobinParent(), PeerSelector::handleHtcpParentMiss(), PeerSelector::handleIcpParentMiss(), and peerSortWeight().
◆ weighted_roundrobin
bool CachePeer::weighted_roundrobin = false |
Definition at line 115 of file CachePeer.h.
Referenced by dump_peer_options(), neighborUpdateRtt(), and peerCountHandleIcpReply().
The documentation for this class was generated from the following files:
- src/CachePeer.h
- src/CachePeer.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products