#include <http.h>
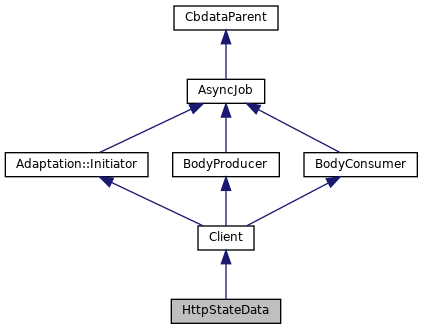
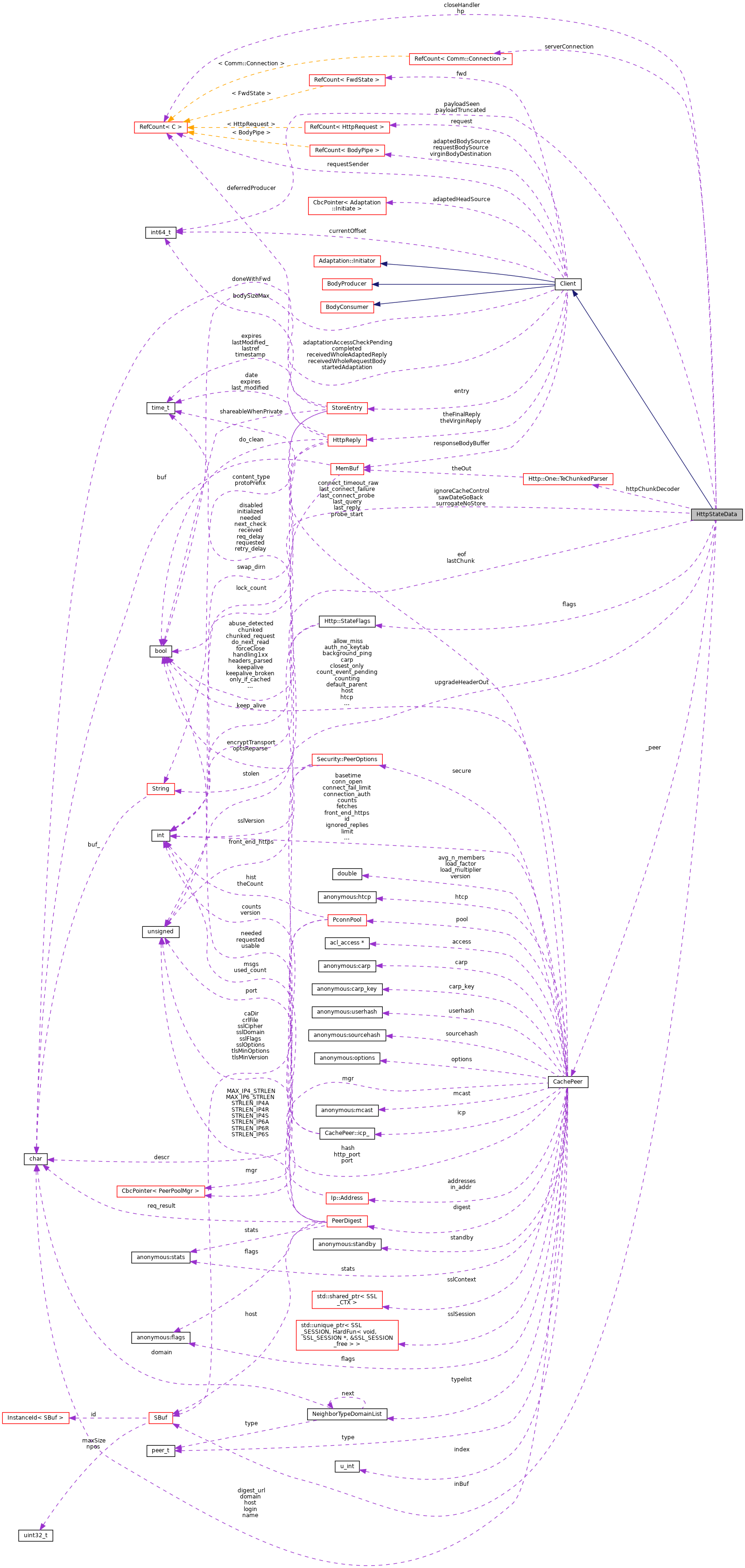
Classes | |
class | ReuseDecision |
assists in making and relaying entry caching/sharing decision More... | |
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
Public Member Functions | |
HttpStateData (FwdState *) | |
~HttpStateData () override | |
const Comm::ConnectionPointer & | dataConnection () const override |
bool | sendRequest () |
void | processReplyHeader () |
void | processReplyBody () override |
void | readReply (const CommIoCbParams &io) |
void | maybeReadVirginBody () override |
read response data from the network More... | |
ReuseDecision::Answers | reusableReply (ReuseDecision &decision) |
void | processSurrogateControl (HttpReply *) |
void | noteMoreBodyDataAvailable (BodyPipe::Pointer) override |
void | noteBodyProductionEnded (BodyPipe::Pointer) override |
void | noteBodyProducerAborted (BodyPipe::Pointer) override |
virtual bool | abortOnData (const char *reason) |
virtual HttpRequestPointer | originalRequest () |
a hack to reach HttpStateData::orignal_request More... | |
void | noteAdaptationAnswer (const Adaptation::Answer &answer) override |
void | noteAdaptationAclCheckDone (Adaptation::ServiceGroupPointer group) override |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override |
void | swanSong () override |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | serverComplete () |
void | markParsedVirginReplyAsWhole (const char *reasonWeAreSure) |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | httpBuildRequestHeader (HttpRequest *request, StoreEntry *entry, const AccessLogEntryPointer &al, HttpHeader *hdr_out, const Http::StateFlags &flags) |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
CachePeer * | _peer = nullptr |
int | eof = 0 |
int | lastChunk = 0 |
Http::StateFlags | flags |
SBuf | inBuf |
I/O buffer for receiving server responses. More... | |
bool | ignoreCacheControl = false |
bool | surrogateNoStore = false |
String * | upgradeHeaderOut = nullptr |
Upgrade header value sent to the origin server or cache peer. More... | |
StoreEntry * | entry = nullptr |
FwdState::Pointer | fwd |
HttpRequestPointer | request |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | noteDelayAwareReadChance () override |
void | processReply () |
void | proceedAfter1xx () |
restores state and resumes processing after 1xx is ignored or forwarded More... | |
void | handle1xx (const HttpReplyPointer &) |
ignore or start forwarding the 1xx response (a.k.a., control message) More... | |
void | drop1xx (const char *reason) |
virtual void | completeForwarding () |
bool | startRequestBodyFlow () |
void | handleRequestBodyProductionEnded () |
void | sendMoreRequestBody () |
bool | abortOnBadEntry (const char *abortReason) |
Entry-dependent callbacks use this check to quit if the entry went bad. More... | |
bool | blockCaching () |
whether to prevent caching of an otherwise cachable response More... | |
void | startAdaptation (const Adaptation::ServiceGroupPointer &group, HttpRequest *cause) |
Initiate an asynchronous adaptation transaction which will call us back. More... | |
void | adaptVirginReplyBody (const char *buf, ssize_t len) |
void | cleanAdaptation () |
virtual bool | doneWithAdaptation () const |
void | handleMoreAdaptedBodyAvailable () |
void | handleAdaptedBodyProductionEnded () |
void | handleAdaptedBodyProducerAborted () |
void | handleAdaptedHeader (Http::Message *msg) |
void | handleAdaptationCompleted () |
void | handleAdaptationBlocked (const Adaptation::Answer &answer) |
void | handleAdaptationAborted (bool bypassable=false) |
bool | handledEarlyAdaptationAbort () |
void | resumeBodyStorage () |
called by StoreEntry when it has more buffer space available More... | |
void | endAdaptedBodyConsumption () |
called when the entire adapted response body is consumed More... | |
const HttpReply * | virginReply () const |
HttpReply * | virginReply () |
HttpReply * | setVirginReply (HttpReply *r) |
HttpReply * | finalReply () |
HttpReply * | setFinalReply (HttpReply *r) |
void | adaptOrFinalizeReply () |
void | addVirginReplyBody (const char *buf, ssize_t len) |
void | storeReplyBody (const char *buf, ssize_t len) |
size_t | replyBodySpace (const MemBuf &readBuf, const size_t minSpace) const |
size_t | calcBufferSpaceToReserve (const size_t space, const size_t wantSpace) const |
determine how much space the buffer needs to reserve More... | |
void | adjustBodyBytesRead (const int64_t delta) |
initializes bodyBytesRead stats if needed and applies delta More... | |
void | delayRead () |
CbcPointer< Initiate > | initiateAdaptation (Initiate *x) |
< starts freshly created initiate and returns a safe pointer to it More... | |
void | clearAdaptation (CbcPointer< Initiate > &x) |
clears the pointer (does not call announceInitiatorAbort) More... | |
void | announceInitiatorAbort (CbcPointer< Initiate > &x) |
inform the transaction about abnormal termination and clear the pointer More... | |
bool | initiated (const CbcPointer< AsyncJob > &job) const |
Must(initiated(initiate)) instead of Must(initiate.set()), for clarity. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Private Types | |
enum | ConnectionStatus { INCOMPLETE_MSG, COMPLETE_PERSISTENT_MSG, COMPLETE_NONPERSISTENT_MSG } |
Private Member Functions | |
CBDATA_CHILD (HttpStateData) | |
ConnectionStatus | statusIfComplete () const |
ConnectionStatus | persistentConnStatus () const |
void | keepaliveAccounting (HttpReply *) |
void | checkDateSkew (HttpReply *) |
bool | continueAfterParsingHeader () |
void | truncateVirginBody () |
void | start () override |
called by AsyncStart; do not call directly More... | |
void | haveParsedReplyHeaders () override |
called when we have final (possibly adapted) reply headers; kids extend More... | |
bool | getMoreRequestBody (MemBuf &buf) override |
either fill buf with available [encoded] request body bytes or return false More... | |
void | closeServer () override |
bool | doneWithServer () const override |
void | abortAll (const char *reason) override |
abnormal transaction termination; reason is for debugging only More... | |
bool | mayReadVirginReplyBody () const override |
whether we may receive more virgin response body bytes More... | |
void | abortTransaction (const char *reason) |
size_t | calcReadBufferCapacityLimit () const |
std::optional< size_t > | canBufferMoreReplyBytes () const |
size_t | maybeMakeSpaceAvailable (size_t maxReadSize) |
virtual void | handleMoreRequestBodyAvailable () |
void | handleRequestBodyProducerAborted () override |
void | writeReplyBody () |
bool | decodeAndWriteReplyBody () |
bool | finishingBrokenPost () |
if broken posts are enabled for the request, try to fix and return true More... | |
bool | finishingChunkedRequest () |
if needed, write last-chunk to end the request body and return true More... | |
void | doneSendingRequestBody () override |
void | requestBodyHandler (MemBuf &) |
void | sentRequestBody (const CommIoCbParams &io) override |
void | wroteLast (const CommIoCbParams &io) |
called after writing the very last request byte (body, last-chunk, etc) More... | |
void | sendComplete () |
successfully wrote the entire request (including body, last-chunk, etc.) More... | |
void | httpStateConnClosed (const CommCloseCbParams ¶ms) |
void | httpTimeout (const CommTimeoutCbParams ¶ms) |
void | markPrematureReplyBodyEofFailure () |
called on a premature EOF discovered when reading response body More... | |
mb_size_t | buildRequestPrefix (MemBuf *mb) |
void | forwardUpgrade (HttpHeader &) |
bool | peerSupportsConnectionPinning () const |
const char * | blockSwitchingProtocols (const HttpReply &) const |
void | serverComplete2 () |
void | sendBodyIsTooLargeError () |
void | maybePurgeOthers () |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static bool | decideIfWeDoRanges (HttpRequest *orig_request) |
Private Attributes | |
Comm::ConnectionPointer | serverConnection |
AsyncCall::Pointer | closeHandler |
Http1::ResponseParserPointer | hp |
Parser being used at present to parse the HTTP/ICY server response. More... | |
Http1::TeChunkedParser * | httpChunkDecoder = nullptr |
int64_t | payloadSeen = 0 |
amount of message payload/body received so far. More... | |
int64_t | payloadTruncated = 0 |
positive when we read more than we wanted More... | |
bool | waitingForCommRead = false |
whether we are waiting for our Comm::Read() handler to be called More... | |
bool | sawDateGoBack = false |
bool | completed = false |
HttpReply * | theVirginReply = nullptr |
HttpReply * | theFinalReply = nullptr |
Detailed Description
Member Typedef Documentation
◆ Pointer [1/3]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [2/3]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [3/3]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Member Enumeration Documentation
◆ ConnectionStatus
|
private |
Constructor & Destructor Documentation
◆ HttpStateData()
HttpStateData::HttpStateData | ( | FwdState * | theFwdState | ) |
Definition at line 79 of file http.cc.
References _peer, cbdataReference, closeHandler, comm_add_close_handler(), debugs, Client::entry, Comm::Connection::fd, flags, HttpRequest::flags, Client::fwd, Comm::Connection::getPeer(), httpStateConnClosed(), JobCallback, CachePeer::no_delay, CachePeer::options, CachePeer::originserver, Http::StateFlags::peering, CachePeer::proxy_only, StoreEntry::releaseRequest(), Client::request, serverConnection, FwdState::serverConnection(), StoreEntry::setNoDelay(), RequestFlags::sslBumped, Http::StateFlags::toOrigin, and Http::StateFlags::tunneling.
◆ ~HttpStateData()
|
override |
Definition at line 115 of file http.cc.
References _peer, cbdataReferenceDone, debugs, httpChunkDecoder, serverConnection, and upgradeHeaderOut.
Member Function Documentation
◆ abortAll()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2680 of file http.cc.
References debugs, AsyncJob::mustStop(), and serverConnection.
Referenced by abortTransaction().
◆ abortOnBadEntry()
|
protectedinherited |
Definition at line 257 of file Client.cc.
References Client::abortOnData(), debugs, Client::entry, and StoreEntry::isAccepting().
Referenced by Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), Client::handleAdaptedHeader(), Client::handleMoreAdaptedBodyAvailable(), Client::noteAdaptationAclCheckDone(), and Client::resumeBodyStorage().
◆ abortOnData()
|
virtualinherited |
abnormal data transfer termination
- Return values
-
true the transaction will be terminated (abortAll called) false the transaction will survive
Reimplemented in Ftp::Relay.
Definition at line 310 of file Client.cc.
References Client::abortAll().
Referenced by Client::abortOnBadEntry(), Client::handleAdaptationBlocked(), Client::sendBodyIsTooLargeError(), and Client::sentRequestBody().
◆ abortTransaction()
|
inlineprivate |
Definition at line 117 of file http.h.
References abortAll().
Referenced by handleRequestBodyProducerAborted(), maybeReadVirginBody(), processReply(), processReplyBody(), and readReply().
◆ adaptOrFinalizeReply()
|
protectedinherited |
Definition at line 995 of file Client.cc.
References Client::adaptationAccessCheckPending, FwdState::al, debugs, Client::fwd, Adaptation::methodRespmod, Client::originalRequest(), Adaptation::pointPreCache, Client::setFinalReply(), Adaptation::AccessCheck::Start(), and Client::virginReply().
Referenced by processReply().
◆ adaptVirginReplyBody()
|
protectedinherited |
Definition at line 627 of file Client.cc.
References MemBuf::append(), assert, MemBuf::consume(), MemBuf::content(), MemBuf::contentSize(), debugs, MemBuf::init(), BodyPipe::putMoreData(), Client::responseBodyBuffer, Client::startedAdaptation, and Client::virginBodyDestination.
Referenced by Client::addVirginReplyBody().
◆ addVirginReplyBody()
|
protectedinherited |
Definition at line 1040 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptVirginReplyBody(), Client::adjustBodyBytesRead(), assert, Client::startedAdaptation, and Client::storeReplyBody().
Referenced by decodeAndWriteReplyBody(), Client::noteMoreBodySpaceAvailable(), and writeReplyBody().
◆ adjustBodyBytesRead()
|
protectedinherited |
Definition at line 1013 of file Client.cc.
References HierarchyLogEntry::bodyBytesRead, HttpRequest::hier, Must, and Client::originalRequest().
Referenced by Client::addVirginReplyBody().
◆ announceInitiatorAbort()
|
protectedinherited |
Definition at line 38 of file Initiator.cc.
References CallJobHere.
Referenced by Client::cleanAdaptation(), and ClientHttpRequest::~ClientHttpRequest().
◆ blockCaching()
|
protectedinherited |
Definition at line 551 of file Client.cc.
References SquidConfig::accessList, FwdState::al, Acl::Answer::allowed(), Config, debugs, Client::entry, ACLChecklist::fastCheck(), MemObject::freshestReply(), Client::fwd, StoreEntry::mem(), Client::originalRequest(), SquidConfig::storeMiss, ACLFilledChecklist::updateAle(), and ACLFilledChecklist::updateReply().
Referenced by Client::setFinalReply().
◆ blockSwitchingProtocols()
|
private |
- Return values
-
nil if the HTTP/101 (Switching Protocols) reply should be forwarded reason why an attempt to switch protocols should be stopped
Definition at line 822 of file http.cc.
References Http::CONNECTION, debugs, HttpHeader::getList(), HttpHeader::has(), HttpHeader::hasListMember(), Http::Message::header, strListGetItem(), Http::UPGRADE, and upgradeHeaderOut.
Referenced by handle1xx().
◆ buildRequestPrefix()
Definition at line 2360 of file http.cc.
References FwdState::al, MemBuf::append(), Packable::appendf(), assert, Http::AUTHORIZATION, RequestFlags::authSent, HttpHeader::clean(), RequestFlags::connectionAuth, crlf, HttpRequest::effectiveRequestUri(), Client::entry, flags, HttpRequest::flags, forwardUpgrade(), Client::fwd, HttpHeader::getList(), RefCount< C >::getRaw(), HttpHeader::has(), hoRequest, httpBuildRequestHeader(), HttpRequestMethod::image(), AnyP::ProtocolVersion::major, HttpRequest::method, AnyP::ProtocolVersion::minor, HttpHeader::packInto(), AnyP::Uri::path(), RequestFlags::pinned, AnyP::ProtocolVersion::protocol, AnyP::ProtocolType_str, Http::ProtocolVersion(), Client::request, MemBuf::size, SQUIDSBUFPH, SQUIDSBUFPRINT, Http::StateFlags::toOrigin, Http::UPGRADE, upgradeHeaderOut, and HttpRequest::url.
Referenced by sendRequest().
◆ calcBufferSpaceToReserve()
|
protectedinherited |
Definition at line 1065 of file Client.cc.
References BodyPipe::buf(), debugs, SBuf::maxSize, min(), MemBuf::potentialSpaceSize(), Client::responseBodyBuffer, and Client::virginBodyDestination.
Referenced by maybeMakeSpaceAvailable().
◆ calcReadBufferCapacityLimit()
|
private |
Desired inBuf capacity based on various capacity preferences/limits:
- a smaller buffer may not hold enough for look-ahead header/body parsers;
- a smaller buffer may result in inefficient tiny network reads;
- a bigger buffer may waste memory;
- a bigger buffer may exceed SBuf storage capabilities (SBuf::maxSize);
Definition at line 1645 of file http.cc.
References Config, flags, Http::StateFlags::headers_parsed, SquidConfig::maxReplyHeaderSize, SBuf::maxSize, and SquidConfig::readAheadGap.
Referenced by canBufferMoreReplyBytes().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ canBufferMoreReplyBytes()
|
private |
The maximum number of virgin reply bytes we may buffer before we violate the currently configured response buffering limits.
- Return values
-
std::nullopt means that no more virgin response bytes can be read 0 means that more virgin response bytes may be read later >0 is the number of bytes that can be read now (subject to other constraints)
Definition at line 1671 of file http.cc.
References calcReadBufferCapacityLimit(), debugs, inBuf, SBuf::length(), Client::responseBodyBuffer, and SBuf::spaceSize().
Referenced by maybeReadVirginBody(), and readReply().
◆ CBDATA_CHILD()
|
private |
◆ checkDateSkew()
|
private |
Definition at line 628 of file http.cc.
References HttpReply::date, debugs, flags, AnyP::Uri::host(), Client::request, squid_curtime, Http::StateFlags::toOrigin, and HttpRequest::url.
Referenced by processReplyHeader().
◆ cleanAdaptation()
|
protectedinherited |
Definition at line 602 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptedBodySource, Client::adaptedHeadSource, Adaptation::Initiator::announceInitiatorAbort(), assert, debugs, Client::doneWithAdaptation(), BodyConsumer::stopConsumingFrom(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
Referenced by Client::handleAdaptationCompleted(), and Client::swanSong().
◆ clearAdaptation()
|
protectedinherited |
Definition at line 32 of file Initiator.cc.
References CbcPointer< Cbc >::clear().
Referenced by ClientHttpRequest::handleAdaptedHeader(), Client::noteAdaptationAnswer(), and ClientHttpRequest::noteAdaptationAnswer().
◆ closeServer()
|
overrideprivatevirtual |
Use this to end communication with the server. The call cancels our closure handler and tells FwdState to forget about the connection.
Implements Client.
Definition at line 1771 of file http.cc.
References Comm::Connection::close(), closeHandler, comm_remove_close_handler(), debugs, Comm::Connection::fd, Client::fwd, Comm::IsConnOpen(), serverConnection, and FwdState::unregister().
Referenced by continueAfterParsingHeader(), drop1xx(), handleMoreRequestBodyAvailable(), httpTimeout(), readReply(), and wroteLast().
◆ completeForwarding()
|
protectedvirtualinherited |
default calls fwd->complete()
Reimplemented in Ftp::Gateway, and Ftp::Relay.
Definition at line 229 of file Client.cc.
References assert, FwdState::complete(), debugs, Client::doneWithFwd, and Client::fwd.
Referenced by Ftp::Relay::completeForwarding(), Ftp::Gateway::completeForwarding(), Client::handleAdaptationCompleted(), and Client::serverComplete2().
◆ continueAfterParsingHeader()
|
private |
- Return values
-
true if we can continue with processing the body or doing ICAP.
- Return values
-
false If we have not finished parsing the headers and may get more data. Schedules more reads to retrieve the missing data.
If we are done with parsing, check for errors
Definition at line 1318 of file http.cc.
References RequestFlags::accelerated, FwdState::al, assert, closeServer(), DBG_IMPORTANT, debugs, FwdState::dontRetry(), Client::entry, eof, ERR_INVALID_RESP, ERR_NONE, ERR_TOO_BIG, ERR_ZERO_SIZE_OBJECT, error(), FwdState::fail(), flags, HttpRequest::flags, Client::fwd, Http::StateFlags::handling1xx, Http::StateFlags::headers_parsed, inBuf, SBuf::length(), maybeReadVirginBody(), Must, AsyncJob::mustStop(), Http::ProtocolVersion(), Client::request, FwdState::request, StoreEntry::reset(), Http::scBadGateway, Http::scHeaderTooLarge, Http::scInvalidHeader, HttpRequest::url, StoreEntry::url(), and Client::virginReply().
Referenced by processReply().
◆ dataConnection()
|
overridevirtual |
- Returns
- primary or "request data connection"
Implements Client.
Definition at line 132 of file http.cc.
References serverConnection.
◆ decideIfWeDoRanges()
|
staticprivate |
Definition at line 2330 of file http.cc.
References RequestFlags::cachable, RequestFlags::connectionAuth, debugs, HttpRequest::flags, HttpRequest::getRangeOffsetLimit(), HttpHdrRange::offsetLimitExceeded(), HttpRequest::range, and Client::request.
Referenced by httpBuildRequestHeader().
◆ decodeAndWriteReplyBody()
|
private |
Definition at line 1450 of file http.cc.
References Client::addVirginReplyBody(), assert, Http::StateFlags::chunked, MemBuf::content(), MemBuf::contentSize(), CurrentException(), debugs, eof, flags, httpChunkDecoder, inBuf, MemBuf::init(), lastChunk, Client::markParsedVirginReplyAsWhole(), markPrematureReplyBodyEofFailure(), Http::One::TeChunkedParser::parse(), Http::One::Parser::remaining(), and Http::One::TeChunkedParser::setPayloadBuffer().
Referenced by processReplyBody().
◆ delayRead()
|
protectedinherited |
Defer reading until it is likely to become possible. Eventually, noteDelayAwareReadChance() will be called.
Definition at line 1028 of file Client.cc.
References Assure, asyncCall(), MemObject::delayRead(), Client::entry, StoreEntry::mem(), Client::noteDelayAwareReadChance(), and Client::waitingForDelayAwareReadChance.
Referenced by readReply().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 216 of file Client.cc.
References AsyncJob::doneAll(), Client::doneWithAdaptation(), and Client::doneWithServer().
◆ doneSendingRequestBody()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2603 of file http.cc.
References Http::StateFlags::chunked_request, debugs, Client::doneSendingRequestBody(), finishingBrokenPost(), finishingChunkedRequest(), flags, sendComplete(), and serverConnection.
◆ doneWithAdaptation()
|
protectedvirtualinherited |
did we end ICAP communication?
Definition at line 619 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptedBodySource, Client::adaptedHeadSource, and Client::virginBodyDestination.
Referenced by Client::cleanAdaptation(), Client::doneAll(), Client::handleAdaptedHeader(), Client::noteBodyConsumerAborted(), and Client::serverComplete2().
◆ doneWithServer()
|
overrideprivatevirtual |
did we end communication?
Implements Client.
Definition at line 1784 of file http.cc.
References Comm::IsConnOpen(), and serverConnection.
Referenced by mayReadVirginReplyBody().
◆ drop1xx()
|
protected |
if possible, safely ignores the received 1xx control message otherwise, terminates the server connection
Definition at line 804 of file http.cc.
References FwdState::al, closeServer(), debugs, ERR_INVALID_RESP, FwdState::fail(), flags, Client::fwd, RefCount< C >::getRaw(), AsyncJob::mustStop(), proceedAfter1xx(), Client::request, Http::scBadGateway, and Http::StateFlags::serverSwitchedProtocols.
Referenced by handle1xx().
◆ endAdaptedBodyConsumption()
|
protectedinherited |
Definition at line 828 of file Client.cc.
References Client::adaptedBodySource, Client::fwd, Client::handleAdaptationCompleted(), FwdState::markStoredReplyAsWhole(), Client::receivedWholeAdaptedReply, and BodyConsumer::stopConsumingFrom().
Referenced by Client::handleAdaptedBodyProductionEnded(), and Client::resumeBodyStorage().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finalReply()
|
protectedinherited |
Definition at line 129 of file Client.cc.
References assert, and Client::theFinalReply.
Referenced by haveParsedReplyHeaders(), and reusableReply().
◆ finishingBrokenPost()
|
private |
Definition at line 2551 of file http.cc.
References SquidConfig::accessList, ACLFilledChecklist::al, FwdState::al, Acl::Answer::allowed(), assert, SquidConfig::brokenPosts, closeHandler, Config, debugs, ACLChecklist::fastCheck(), Client::fwd, Comm::IsConnOpen(), JobCallback, Client::originalRequest(), Client::requestSender, serverConnection, ACLFilledChecklist::syncAle(), Comm::Write(), and wroteLast().
Referenced by doneSendingRequestBody().
◆ finishingChunkedRequest()
|
private |
Definition at line 2586 of file http.cc.
References debugs, flags, JobCallback, Must, Client::receivedWholeRequestBody, Client::requestSender, Http::StateFlags::sentLastChunk, serverConnection, Comm::Write(), and wroteLast().
Referenced by doneSendingRequestBody().
◆ forwardUpgrade()
|
private |
copies from-client Upgrade info into the given to-server header while honoring configuration filters and following HTTP requirements
Definition at line 2078 of file http.cc.
References ACLFilledChecklist::al, FwdState::al, ACLChecklist::changeAcl(), Config, Http::CONNECTION, debugs, ACLChecklist::fastCheck(), flags, HttpUpgradeProtocolAccess::forApplicable(), Http::StateFlags::forceClose, Client::fwd, HttpHeader::getList(), RefCount< C >::getRaw(), Http::Message::header, SquidConfig::http_upgrade_request_protocols, Http::Message::http_ver, Http::StateFlags::keepalive, Http::ProtocolVersion(), HttpHeader::putStr(), Client::request, String::size(), strListAdd(), strListGetItem(), String::termedBuf(), and Http::UPGRADE.
Referenced by buildRequestPrefix().
◆ getMoreRequestBody()
|
overrideprivatevirtual |
Reimplemented from Client.
Definition at line 2490 of file http.cc.
References MemBuf::append(), Packable::appendf(), Http::StateFlags::chunked_request, MemBuf::content(), MemBuf::contentSize(), flags, BodyPipe::getMoreData(), Client::getMoreRequestBody(), MemBuf::init(), MemBuf::max_capacity, Must, Client::receivedWholeRequestBody, Client::requestBodySource, and Http::StateFlags::sentLastChunk.
◆ handle1xx()
|
protected |
Definition at line 748 of file http.cc.
References SquidConfig::accessList, FwdState::al, Acl::Answer::allowed(), blockSwitchingProtocols(), CallJobHere1, HttpRequest::canHandle1xx(), HttpRequest::clientConnectionManager, Config, debugs, drop1xx(), ACLChecklist::fastCheck(), flags, HttpRequest::forcedBodyContinuation, Client::fwd, Http::StateFlags::handling1xx, JobCallback, Must, Client::originalRequest(), proceedAfter1xx(), AccessLogEntry::reply, SquidConfig::reply, Client::request, Http::scContinue, Http::scSwitchingProtocols, ConnStateData::sendControlMsg(), Http::StateFlags::serverSwitchedProtocols, HttpReply::sline, Http::StatusLine::status(), ACLFilledChecklist::syncAle(), ACLFilledChecklist::updateAle(), and ACLFilledChecklist::updateReply().
Referenced by processReplyHeader().
◆ handleAdaptationAborted()
|
protectedinherited |
Definition at line 883 of file Client.cc.
References Client::abortAll(), Client::abortOnBadEntry(), debugs, Client::entry, Client::handledEarlyAdaptationAbort(), and StoreEntry::isEmpty().
Referenced by Client::noteAdaptationAnswer().
◆ handleAdaptationBlocked()
|
protectedinherited |
Definition at line 922 of file Client.cc.
References Client::abortAll(), Client::abortOnBadEntry(), Client::abortOnData(), FwdState::al, Adaptation::Answer::blockedToChecklistAnswer(), debugs, HttpRequest::detailError(), FwdState::dontRetry(), Client::entry, ERR_ACCESS_DENIED, ERR_ICAP_FAILURE, ERR_NONE, FwdState::fail(), FindDenyInfoPage(), Client::fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), Client::request, and Http::scForbidden.
Referenced by Client::noteAdaptationAnswer().
◆ handleAdaptationCompleted()
|
protectedinherited |
Definition at line 864 of file Client.cc.
References Client::cleanAdaptation(), Client::closeServer(), Client::completeForwarding(), debugs, and Client::mayReadVirginReplyBody().
Referenced by Client::endAdaptedBodyConsumption(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedHeader(), and Client::noteBodyConsumerAborted().
◆ handleAdaptedBodyProducerAborted()
|
protectedinherited |
Definition at line 843 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, debugs, BodyPipe::exhausted(), Client::handleAdaptationCompleted(), Client::handledEarlyAdaptationAbort(), Must, and BodyConsumer::stopConsumingFrom().
Referenced by Client::noteBodyProducerAborted().
◆ handleAdaptedBodyProductionEnded()
|
protectedinherited |
Definition at line 813 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, Client::endAdaptedBodyConsumption(), BodyPipe::exhausted(), and Client::receivedWholeAdaptedReply.
Referenced by Client::noteBodyProductionEnded().
◆ handleAdaptedHeader()
|
protectedinherited |
Definition at line 715 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, assert, Http::Message::body_pipe, debugs, Client::doneWithAdaptation(), BodyPipe::expectNoConsumption(), Client::fwd, Client::handleAdaptationCompleted(), FwdState::markStoredReplyAsWhole(), BodyPipe::setConsumerIfNotLate(), and Client::setFinalReply().
Referenced by Client::noteAdaptationAnswer().
◆ handledEarlyAdaptationAbort()
|
protectedinherited |
If the store entry is still empty, fully handles adaptation abort, returning true. Otherwise just updates the request error detail and returns false.
Definition at line 899 of file Client.cc.
References Client::abortAll(), FwdState::al, debugs, HttpRequest::detailError(), FwdState::dontRetry(), Client::entry, ERR_ICAP_FAILURE, FwdState::fail(), Client::fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), Client::request, and Http::scInternalServerError.
Referenced by Client::handleAdaptationAborted(), and Client::handleAdaptedBodyProducerAborted().
◆ handleMoreAdaptedBodyAvailable()
|
protectedinherited |
Definition at line 766 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, assert, asyncCall(), BodyPipeCheckout::buf, BodyPipe::buf(), StoreEntry::bytesWanted(), BodyPipeCheckout::checkIn(), MemBuf::consume(), BodyPipe::consumedSize(), MemBuf::contentSize(), Client::currentOffset, debugs, StoreEntry::deferProducer(), Client::entry, StoreIOBuffer::length, Client::resumeBodyStorage(), and StoreEntry::write().
Referenced by Client::noteMoreBodyDataAvailable(), and Client::resumeBodyStorage().
◆ handleMoreRequestBodyAvailable()
|
privatevirtual |
Definition at line 2619 of file http.cc.
References Http::StateFlags::abuse_detected, assert, BodyPipe::buf(), HttpRequest::client_addr, closeServer(), DBG_IMPORTANT, debugs, Client::entry, eof, flags, MemBuf::hasContent(), Http::StateFlags::headers_parsed, Comm::IsConnOpen(), AsyncJob::mustStop(), Client::request, Client::requestBodySource, Http::scInvalidHeader, serverConnection, StoreEntry::url(), and Client::virginReply().
◆ handleRequestBodyProducerAborted()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2651 of file http.cc.
References abortTransaction(), FwdState::al, debugs, Client::entry, ERR_ICAP_FAILURE, FwdState::fail(), Client::fwd, Client::handleRequestBodyProducerAborted(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), FwdState::request, Http::scInternalServerError, and serverConnection.
◆ handleRequestBodyProductionEnded()
|
protectedinherited |
Definition at line 328 of file Client.cc.
References debugs, Client::doneSendingRequestBody(), Client::receivedWholeRequestBody, and Client::requestSender.
Referenced by Client::noteBodyProductionEnded().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ haveParsedReplyHeaders()
|
overrideprivatevirtual |
called when got final headers
Reimplemented from Client.
Definition at line 936 of file http.cc.
References assert, Http::Message::cache_control, HttpStateData::ReuseDecision::cacheNegatively, StoreEntry::cacheNegatively(), HttpStateData::ReuseDecision::cachePositively, debugs, HttpStateData::ReuseDecision::doNotCacheButShare, EBIT_SET, Client::entry, ENTRY_FWD_HDR_WAIT, ENTRY_REVALIDATE_ALWAYS, ENTRY_REVALIDATE_STALE, Client::finalReply(), findPreviouslyCachedEntry(), StoreEntry::flags, RefCount< C >::getRaw(), HttpHeader::has(), HttpHeader::hasListMember(), HttpHdrCc::hasMustRevalidate(), HttpHdrCc::hasNoCacheWithoutParameters(), HttpHdrCc::hasPrivate(), HttpHdrCc::hasProxyRevalidate(), HttpHdrCc::hasSMaxAge(), Client::haveParsedReplyHeaders(), Http::HDR_X_ACCELERATOR_VARY, Http::Message::header, httpMakeVaryMark(), httpMaybeRemovePublic(), ignoreCacheControl, SBuf::isEmpty(), Http::IsReforwardableStatus(), HttpStateData::ReuseDecision::make(), StoreEntry::makePrivate(), StoreEntry::makePublic(), StoreEntry::mem_obj, neighbors_do_private_keys, HttpReply::olderThan(), Http::PRAGMA, Client::request, reusableReply(), HttpStateData::ReuseDecision::reuseNot, sawDateGoBack, HttpReply::sline, Http::StatusLine::status(), StoreEntry::timestampsSet(), Http::VARY, and MemObject::vary_headers.
◆ httpBuildRequestHeader()
|
static |
- Precondition
- Handle X-Forwarded-For
If set to ON - append client IP or 'unknown'.
If set to OFF - append 'unknown'.
If set to TRANSPARENT - pass through unchanged.
If set to TRUNCATE - drop existing list and replace with client IP or 'unknown'.
If set to DELETE - do not copy through.
Definition at line 1904 of file http.cc.
References SquidConfig::Accel, RequestFlags::accelerated, HttpHeader::addEntry(), HttpHeader::addVia(), assert, AnyP::Uri::authority(), Http::AUTHORIZATION, base64_encode_final(), base64_encode_init(), base64_encode_len, base64_encode_update(), BBUF_SZ, SBuf::c_str(), RequestFlags::cachable, String::canGrowBy(), Http::StateFlags::chunked_request, String::clean(), HttpRequest::client_addr, Config, Http::CONNECTION, copyOneHeaderFromClientsideRequestToUpstreamRequest(), DBG_IMPORTANT, debugs, decideIfWeDoRanges(), HttpRequest::effectiveRequestUri(), Client::entry, HttpRequest::etag, flags, HttpRequest::flags, Http::StateFlags::front_end_https, Http::FRONT_END_HTTPS, HttpHeader::getCc(), HttpHeader::getEntry(), HttpHeader::getList(), getMaxAge(), AnyP::Uri::getScheme(), HttpHeader::has(), HttpHdrCc::hasMaxAge(), HttpHdrCc::hasNoCache(), Http::Message::header, hoRequest, Http::HOST, Http::Message::http_ver, httpFixupAuthentication(), httpHdrMangleList(), HttpHeaderInitPos, httpHeaderPutStrf(), Http::IF_MODIFIED_SINCE, Http::IF_NONE_MATCH, HttpRequest::ignoreRange(), Ip::Address::isNoAddr(), RequestFlags::isRanged, Http::StateFlags::keepalive, HttpRequest::lastmod, LOCAL_ARRAY, MAX_IPSTRLEN, MAX_URL, HttpHdrCc::maxAge(), HttpRequest::multipartRangeRequest(), Http::StateFlags::only_if_cached, HttpHdrCc::onlyIfCached(), opt_forwarded_for, HttpHeader::owner, HttpRequest::peer_domain, AnyP::PROTO_HTTPS, HttpHeader::putCc(), HttpHeader::putStr(), HttpHeader::putTime(), Client::request, ROR_REQUEST, String::size(), strListAdd(), Http::SURROGATE_CAPABILITY, SquidConfig::surrogate_id, String::termedBuf(), Http::StateFlags::toOrigin, Ip::Address::toStr(), Http::TRANSFER_ENCODING, HttpRequest::url, StoreEntry::url(), AnyP::Uri::userInfo(), SupportOrVeto::veto(), and Http::X_FORWARDED_FOR.
Referenced by buildRequestPrefix(), htcpClear(), htcpQuery(), and Http::Tunneler::writeRequest().
◆ httpStateConnClosed()
|
private |
Definition at line 138 of file http.cc.
References debugs, Client::doneWithFwd, AsyncJob::mustStop(), and CachePeer::params.
Referenced by HttpStateData().
◆ httpTimeout()
|
private |
Definition at line 146 of file http.cc.
References FwdState::al, closeServer(), debugs, Client::entry, ERR_READ_TIMEOUT, FwdState::fail(), Client::fwd, AsyncJob::mustStop(), FwdState::request, Http::scGatewayTimeout, serverConnection, STORE_PENDING, StoreEntry::store_status, and StoreEntry::url().
Referenced by sendComplete(), and sendRequest().
◆ initiateAdaptation()
|
protectedinherited |
Definition at line 23 of file Initiator.cc.
References Adaptation::Initiate::initiator().
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ initiated()
|
inlineprotectedinherited |
Definition at line 52 of file Initiator.h.
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ keepaliveAccounting()
|
private |
Definition at line 608 of file http.cc.
References _peer, HttpReply::bodySize(), Http::StateFlags::chunked, Config, DBG_IMPORTANT, debugs, SquidConfig::detect_broken_server_pconns, Client::entry, flags, HttpReply::keep_alive, Http::StateFlags::keepalive, Http::StateFlags::keepalive_broken, HttpRequest::method, CachePeer::n_keepalives_recv, CachePeer::n_keepalives_sent, SquidConfig::onoff, Http::StateFlags::peering, Client::request, CachePeer::stats, Http::StateFlags::tunneling, and StoreEntry::url().
Referenced by processReplyHeader().
◆ markParsedVirginReplyAsWhole()
|
inherited |
remember that the received virgin reply was parsed in its entirety, including its body (if any)
Definition at line 158 of file Client.cc.
References assert, debugs, Client::fwd, FwdState::markStoredReplyAsWhole(), and Client::startedAdaptation.
Referenced by decodeAndWriteReplyBody(), ftpReadTransferDone(), ftpWriteTransferDone(), and writeReplyBody().
◆ markPrematureReplyBodyEofFailure()
|
private |
Definition at line 1411 of file http.cc.
References FwdState::al, ERR_READ_ERROR, FwdState::fail(), Client::fwd, MakeNamedErrorDetail(), FwdState::request, and Http::scBadGateway.
Referenced by decodeAndWriteReplyBody(), and writeReplyBody().
◆ maybeMakeSpaceAvailable()
prepare read buffer for reading
- Returns
- the maximum number of bytes the caller should attempt to read
- Return values
-
0 means that the caller should delay reading
Definition at line 1697 of file http.cc.
References Client::calcBufferSpaceToReserve(), debugs, inBuf, SBuf::length(), SBuf::reserveSpace(), serverConnection, and SBuf::spaceSize().
Referenced by readReply().
◆ maybePurgeOthers()
|
privateinherited |
Definition at line 515 of file Client.cc.
References SBuf::c_str(), Http::CONTENT_LOCATION, debugs, HttpRequest::effectiveRequestUri(), RefCount< C >::getRaw(), Http::LOCATION, HttpRequest::method, purgeEntriesByHeader(), purgeEntriesByUrl(), HttpRequestMethod::purgesOthers(), Client::request, HttpReply::sline, Http::StatusLine::status(), and Client::theFinalReply.
Referenced by Client::haveParsedReplyHeaders().
◆ maybeReadVirginBody()
|
overridevirtual |
Implements Client.
Definition at line 1596 of file http.cc.
References abortTransaction(), assert, canBufferMoreReplyBytes(), debugs, eof, Comm::Connection::fd, fd_table, Comm::IsConnOpen(), JobCallback, lastChunk, Comm::MonitorsRead(), Comm::Read(), readReply(), serverConnection, waitingForCommRead, and Client::waitingForDelayAwareReadChance.
Referenced by continueAfterParsingHeader(), noteDelayAwareReadChance(), processReplyBody(), readReply(), and sendRequest().
◆ mayReadVirginReplyBody()
|
overrideprivatevirtual |
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by abortAll(), AsyncJob::callException(), continueAfterParsingHeader(), drop1xx(), handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), httpStateConnClosed(), httpTimeout(), proceedAfter1xx(), ConnStateData::proxyProtocolError(), readReply(), start(), and wroteLast().
◆ noteAdaptationAclCheckDone()
|
overridevirtualinherited |
AccessCheck calls this back with a possibly nil service group to signal whether adaptation is needed and where it should start.
Reimplemented from Adaptation::Initiator.
Definition at line 956 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptationAccessCheckPending, debugs, Client::originalRequest(), Client::processReplyBody(), Client::request, Client::sendBodyIsTooLargeError(), Client::setFinalReply(), Client::startAdaptation(), and Client::virginReply().
◆ noteAdaptationAnswer()
|
overridevirtualinherited |
called with the initial adaptation decision (adapt, block, error); virgin and/or adapted body transmission may continue after this
Implements Adaptation::Initiator.
Definition at line 695 of file Client.cc.
References Client::adaptedHeadSource, Adaptation::Answer::akBlock, Adaptation::Answer::akError, Adaptation::Answer::akForward, Adaptation::Initiator::clearAdaptation(), Adaptation::Answer::final, RefCount< C >::getRaw(), Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handleAdaptedHeader(), Adaptation::Answer::kind, and Adaptation::Answer::message.
◆ noteBodyConsumerAborted()
|
overridevirtualinherited |
Implements BodyProducer.
Definition at line 683 of file Client.cc.
References Client::doneWithAdaptation(), Client::handleAdaptationCompleted(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
◆ noteBodyProducerAborted()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 297 of file Client.cc.
References Client::adaptedBodySource, Client::handleAdaptedBodyProducerAborted(), Client::handleRequestBodyProducerAborted(), and Client::requestBodySource.
◆ noteBodyProductionEnded()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 283 of file Client.cc.
References Client::adaptedBodySource, Client::handleAdaptedBodyProductionEnded(), Client::handleRequestBodyProductionEnded(), and Client::requestBodySource.
◆ noteDelayAwareReadChance()
|
overrideprotectedvirtual |
Called when a previously delayed dataConnection() read may be possible.
- See also
- delayRead()
Implements Client.
Definition at line 1161 of file http.cc.
References maybeReadVirginBody(), and Client::waitingForDelayAwareReadChance.
◆ noteMoreBodyDataAvailable()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 269 of file Client.cc.
References Client::adaptedBodySource, Client::handleMoreAdaptedBodyAvailable(), Client::handleMoreRequestBodyAvailable(), and Client::requestBodySource.
◆ noteMoreBodySpaceAvailable()
|
overridevirtualinherited |
Implements BodyProducer.
Definition at line 669 of file Client.cc.
References Client::addVirginReplyBody(), Client::completed, Client::maybeReadVirginBody(), Client::responseBodyBuffer, and Client::serverComplete2().
◆ originalRequest()
|
virtualinherited |
Definition at line 568 of file Client.cc.
References Client::request.
Referenced by Client::adaptOrFinalizeReply(), Client::adjustBodyBytesRead(), Client::blockCaching(), finishingBrokenPost(), handle1xx(), Client::noteAdaptationAclCheckDone(), and Client::startRequestBodyFlow().
◆ peerSupportsConnectionPinning()
|
private |
returns true if the peer can support connection pinning
Definition at line 878 of file http.cc.
References _peer, CachePeer::connection_auth, Client::entry, flags, HttpRequest::flags, MemObject::freshestReply(), StoreEntry::mem(), CachePeer::options, CachePeer::originserver, RequestFlags::pinned, Http::PROXY_SUPPORT, Client::request, Http::scUnauthorized, and Http::StateFlags::tunneling.
Referenced by processReplyHeader().
◆ persistentConnStatus()
|
private |
- In chunked response we do not know the content length but we are absolutely sure about the end of response, so we are calling the statusIfComplete to decide if we can be persistent
- If the body size is known, we must wait until we've gotten all of it.
- If there is no message body or we got it all, we can be persistent
Definition at line 1111 of file http.cc.
References HttpReply::bodySize(), Http::StateFlags::chunked, COMPLETE_NONPERSISTENT_MSG, Http::Message::content_length, debugs, eof, flags, INCOMPLETE_MSG, Comm::IsConnOpen(), lastChunk, HttpRequest::method, payloadSeen, payloadTruncated, Client::request, serverConnection, statusIfComplete(), and Client::virginReply().
Referenced by processReplyBody().
◆ proceedAfter1xx()
|
protected |
Definition at line 849 of file http.cc.
References asyncCall(), CallJobHere, HttpRequest::clientConnectionManager, closeHandler, comm_remove_close_handler(), debugs, Client::doneWithFwd, Comm::Connection::fd, flags, Client::fwd, Http::StateFlags::handling1xx, inBuf, Must, AsyncJob::mustStop(), ConnStateData::noteTakeServerConnectionControl(), payloadSeen, processReply(), Client::request, ScheduleCallHere, serverConnection, Http::StateFlags::serverSwitchedProtocols, and FwdState::unregister().
Referenced by drop1xx(), and handle1xx().
◆ processReply()
|
protected |
processes the already read and buffered response data, possibly after waiting for asynchronous 1xx control message processing
Definition at line 1287 of file http.cc.
References abortTransaction(), Client::adaptOrFinalizeReply(), continueAfterParsingHeader(), debugs, EBIT_TEST, Client::entry, ENTRY_ABORTED, flags, StoreEntry::flags, Http::StateFlags::handling1xx, Http::StateFlags::headers_parsed, Must, processReplyBody(), and processReplyHeader().
Referenced by proceedAfter1xx(), and readReply().
◆ processReplyBody()
|
overridevirtual |
processReplyBody has two purposes: 1 - take the reply body data, if any, and put it into either the StoreEntry, or give it over to ICAP. 2 - see if we made it to the end of the response (persistent connections and such)
Implements Client.
Definition at line 1483 of file http.cc.
References abortTransaction(), Client::adaptationAccessCheckPending, RequestFlags::authSent, CallJobHere1, Http::StateFlags::chunked, HttpRequest::client_addr, HttpRequest::clientConnectionManager, closeHandler, comm_remove_close_handler(), commSetConnTimeout(), commUnsetConnTimeout(), COMPLETE_NONPERSISTENT_MSG, COMPLETE_PERSISTENT_MSG, Config, RequestFlags::connectionAuth, debugs, decodeAndWriteReplyBody(), EBIT_TEST, Client::entry, ENTRY_ABORTED, Comm::Connection::fd, flags, HttpRequest::flags, StoreEntry::flags, Client::fwd, fwdPconnPool, Http::StateFlags::headers_parsed, AnyP::Uri::host(), INCOMPLETE_MSG, StoreEntry::isAccepting(), Http::StateFlags::keepalive_broken, maybeReadVirginBody(), persistentConnStatus(), RequestFlags::pinned, PconnPool::push(), SquidConfig::read, Client::request, Client::serverComplete(), serverConnection, RequestFlags::spoofClientIp, SquidConfig::Timeout, FwdState::unregister(), HttpRequest::url, and writeReplyBody().
Referenced by processReply().
◆ processReplyHeader()
void HttpStateData::processReplyHeader | ( | ) |
This creates the error page itself.. its likely that the forward ported reply header max size patch generates non http conformant error pages - in which case the errors where should be 'BAD_GATEWAY' etc
Creates a blank header. If this routine is made incremental, this will not do
Definition at line 645 of file http.cc.
References assert, checkDateSkew(), Http::StateFlags::chunked, RequestFlags::connectionAuthDisabled, debugs, Client::entry, eof, flags, HttpRequest::flags, StoreEntry::getMD5Text(), AnyP::Uri::getScheme(), handle1xx(), Http::StateFlags::headers_parsed, HttpRequest::hier, hp, httpChunkDecoder, inBuf, Http::Is1xx(), keepaliveAccounting(), SBuf::length(), RefCount< HttpReply >::Make(), payloadSeen, HierarchyLogEntry::peer_reply_status, peerSupportsConnectionPinning(), processSurrogateControl(), AnyP::PROTO_HTTP, AnyP::PROTO_HTTPS, Http::ProtocolVersion(), Client::request, Http::scInvalidHeader, serverConnection, Http::StatusLine::set(), Client::setVirginReply(), HttpReply::sline, Http::Message::srcHttp, Http::Message::srcHttps, and HttpRequest::url.
Referenced by processReply().
◆ processSurrogateControl()
void HttpStateData::processSurrogateControl | ( | HttpReply * | reply | ) |
Definition at line 258 of file http.cc.
References SquidConfig::Accel, RequestFlags::accelerated, Config, HttpReply::date, Client::entry, HttpReply::expires, HttpRequest::flags, HttpHdrSc::getMergedTarget(), HttpHdrScTarget::hasMaxAge(), HttpHdrScTarget::hasNoStore(), ignoreCacheControl, StoreEntry::makePrivate(), HttpHdrScTarget::maxAge(), HttpHdrScTarget::maxStale(), HttpHdrScTarget::noStoreRemote(), SquidConfig::onoff, Client::request, HttpReply::surrogate_control, SquidConfig::surrogate_id, SquidConfig::surrogate_is_remote, surrogateNoStore, and StoreEntry::timestampsSet().
Referenced by processReplyHeader().
◆ readReply()
void HttpStateData::readReply | ( | const CommIoCbParams & | io | ) |
wait for Client::noteDelayAwareReadChance()
Definition at line 1168 of file http.cc.
References abortTransaction(), FwdState::al, StatCounters::all, BodyPipe::buf(), DelayId::bytesIn(), StoreEntry::bytesWanted(), canBufferMoreReplyBytes(), closeServer(), CommCommonCbParams::conn, MemBuf::contentSize(), debugs, Client::delayRead(), EBIT_TEST, Comm::ENDFILE, Client::entry, ENTRY_ABORTED, eof, Comm::ERR_CLOSING, ERR_READ_ERROR, FwdState::fail(), Comm::Connection::fd, CommCommonCbParams::flag, StoreEntry::flags, Client::fwd, MemBuf::hasContent(), HttpRequest::hier, IoStats::Http, StatCounters::http, inBuf, Comm::INPROGRESS, IOStats, Comm::IsConnOpen(), SBuf::isEmpty(), maybeMakeSpaceAvailable(), maybeReadVirginBody(), StoreEntry::mem_obj, MemObject::mostBytesAllowed(), Must, AsyncJob::mustStop(), HierarchyLogEntry::notePeerRead(), Comm::OK, payloadSeen, processReply(), IoStats::read_hist, Comm::ReadNow(), IoStats::reads, Client::request, FwdState::request, Client::responseBodyBuffer, Http::scBadGateway, StatCounters::server, serverConnection, CommIoCbParams::size, statCounter, Client::virginBodyDestination, waitingForCommRead, CommCommonCbParams::xerrno, and xstrerr().
Referenced by maybeReadVirginBody().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ replyBodySpace()
|
protectedinherited |
- Deprecated:
- use SBuf I/O API and calcBufferSpaceToReserve() instead
Definition at line 1100 of file Client.cc.
References BodyPipe::buf(), debugs, min(), MemBuf::potentialSpaceSize(), Client::responseBodyBuffer, MemBuf::spaceSize(), and Client::virginBodyDestination.
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestBodyHandler()
|
private |
◆ resumeBodyStorage()
|
protectedinherited |
Definition at line 750 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, Client::endAdaptedBodyConsumption(), BodyPipe::exhausted(), and Client::handleMoreAdaptedBodyAvailable().
Referenced by Client::handleMoreAdaptedBodyAvailable().
◆ reusableReply()
HttpStateData::ReuseDecision::Answers HttpStateData::reusableReply | ( | HttpStateData::ReuseDecision & | decision | ) |
Definition at line 296 of file http.cc.
References HttpStateData::ReuseDecision::answer, RequestFlags::auth, RequestFlags::authSent, Http::Message::cache_control, HttpStateData::ReuseDecision::cacheNegatively, HttpStateData::ReuseDecision::cachePositively, Config, Http::CONTENT_TYPE, HttpReply::date, debugs, HttpStateData::ReuseDecision::doNotCacheButShare, EBIT_TEST, Client::entry, HttpReply::expires, Client::finalReply(), HttpRequest::flags, StoreEntry::flags, HttpHeader::getStr(), HttpHdrCc::hasMustRevalidate(), HttpHdrCc::hasNoCacheWithoutParameters(), HttpHdrCc::hasNoCacheWithParameters(), HttpHdrCc::hasNoStore(), HttpHdrCc::hasPrivate(), HttpHdrCc::hasPublic(), HttpHdrCc::hasSMaxAge(), Http::Message::header, ignoreCacheControl, HttpStateData::ReuseDecision::make(), SquidConfig::negativeTtl, REFRESH_OVERRIDE, refreshIsCachable(), RELEASE_REQUEST, Client::request, HttpStateData::ReuseDecision::reuseNot, sawDateGoBack, Http::scBadGateway, Http::scBadRequest, Http::scConflict, Http::scContentTooLarge, Http::scExpectationFailed, Http::scFailedDependency, Http::scForbidden, Http::scFound, Http::scGatewayTimeout, Http::scGone, Http::scHeaderTooLarge, Http::scInsufficientStorage, Http::scInternalServerError, Http::scInvalidHeader, Http::scLengthRequired, Http::scLocked, Http::scMethodNotAllowed, Http::scMisdirectedRequest, Http::scMovedPermanently, Http::scMultipleChoices, Http::scNoContent, Http::scNonAuthoritativeInformation, Http::scNotAcceptable, Http::scNotFound, Http::scNotImplemented, Http::scNotModified, Http::scOkay, Http::scPartialContent, Http::scPaymentRequired, Http::scPermanentRedirect, Http::scPreconditionFailed, Http::scProxyAuthenticationRequired, Http::scRequestedRangeNotSatisfied, Http::scRequestTimeout, Http::scSeeOther, Http::scServiceUnavailable, Http::scTemporaryRedirect, Http::scUnauthorized, Http::scUnprocessableEntity, Http::scUnsupportedMediaType, Http::scUriTooLong, Http::scUseProxy, HttpReply::sline, Http::StatusLine::status(), and surrogateNoStore.
Referenced by haveParsedReplyHeaders().
◆ sendBodyIsTooLargeError()
|
privateinherited |
Definition at line 984 of file Client.cc.
References Client::abortOnData(), FwdState::al, FwdState::dontRetry(), ERR_TOO_BIG, FwdState::fail(), Client::fwd, RefCount< C >::getRaw(), Client::request, and Http::scForbidden.
Referenced by Client::noteAdaptationAclCheckDone().
◆ sendComplete()
|
private |
Definition at line 1752 of file http.cc.
References commSetConnTimeout(), Config, flags, httpTimeout(), JobCallback, SquidConfig::read, Http::StateFlags::request_sent, serverConnection, and SquidConfig::Timeout.
Referenced by doneSendingRequestBody(), and wroteLast().
◆ sendMoreRequestBody()
|
protectedinherited |
Definition at line 411 of file Client.cc.
References assert, MemBuf::contentSize(), Client::dataConnection(), debugs, Client::getMoreRequestBody(), Comm::IsConnOpen(), JobCallback, Client::requestBodySource, Client::requestSender, Client::sentRequestBody(), and Comm::Write().
Referenced by Client::handleMoreRequestBodyAvailable(), and Client::sentRequestBody().
◆ sendRequest()
bool HttpStateData::sendRequest | ( | ) |
Definition at line 2404 of file http.cc.
References _peer, CachePeer::allow_miss, assert, Http::Message::body_pipe, MemBuf::buf, buildRequestPrefix(), Http::StateFlags::chunked_request, closeHandler, commSetConnTimeout(), Config, Http::Message::content_length, debugs, flags, HttpRequest::flags, Http::StateFlags::front_end_https, CachePeer::front_end_https, CachePeer::host, httpTimeout(), MemBuf::init(), Comm::IsConnOpen(), JobCallback, Http::StateFlags::keepalive, SquidConfig::lifetime, maybeReadVirginBody(), Must, RequestFlags::mustKeepalive, CachePeer::n_keepalives_recv, CachePeer::n_keepalives_sent, neighborType(), Http::StateFlags::only_if_cached, SquidConfig::onoff, CachePeer::options, HttpRequest::peer_host, PEER_SIBLING, Http::Message::persistent(), RequestFlags::pinned, Client::request, Client::requestBodySource, Client::requestSender, sentRequestBody(), SquidConfig::server_pconns, serverConnection, RequestFlags::sslBumped, Client::startRequestBodyFlow(), CachePeer::stats, SquidConfig::Timeout, Http::StateFlags::tunneling, HttpRequest::url, Comm::Write(), and wroteLast().
Referenced by start().
◆ sentRequestBody()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2671 of file http.cc.
References StatCounters::http, Client::sentRequestBody(), StatCounters::server, CommIoCbParams::size, and statCounter.
Referenced by sendRequest().
◆ serverComplete()
|
inherited |
call when no server communication is expected
Definition at line 180 of file Client.cc.
References assert, Client::closeServer(), Client::completed, debugs, Client::doneWithServer(), Client::requestBodySource, Client::responseBodyBuffer, Client::serverComplete2(), and BodyConsumer::stopConsumingFrom().
Referenced by ftpReadQuit(), processReplyBody(), and Ftp::Relay::serverComplete().
◆ serverComplete2()
|
privateinherited |
Continuation of serverComplete
Definition at line 201 of file Client.cc.
References Client::completeForwarding(), debugs, Client::doneWithAdaptation(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
Referenced by Client::noteMoreBodySpaceAvailable(), and Client::serverComplete().
◆ setFinalReply()
Definition at line 136 of file Client.cc.
References FwdState::al, assert, Client::blockCaching(), debugs, EBIT_TEST, Client::entry, StoreEntry::flags, Client::fwd, Client::haveParsedReplyHeaders(), HTTPMSGLOCK(), StoreEntry::release(), RELEASE_REQUEST, StoreEntry::replaceHttpReply(), AccessLogEntry::reply, StoreEntry::startWriting(), and Client::theFinalReply.
Referenced by Client::adaptOrFinalizeReply(), Client::handleAdaptedHeader(), and Client::noteAdaptationAclCheckDone().
◆ setVirginReply()
Definition at line 116 of file Client.cc.
References FwdState::al, assert, debugs, Client::fwd, HTTPMSGLOCK(), AccessLogEntry::reply, and Client::theVirginReply.
Referenced by processReplyHeader().
◆ start()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 2531 of file http.cc.
References StatCounters::all, debugs, StatCounters::http, AsyncJob::mustStop(), sendRequest(), StatCounters::server, and statCounter.
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startAdaptation()
|
protectedinherited |
Definition at line 576 of file Client.cc.
References Client::adaptedHeadSource, FwdState::al, assert, Http::Message::body_pipe, debugs, HttpReply::expectingBody(), Client::fwd, Adaptation::Initiator::initiateAdaptation(), Adaptation::Initiator::initiated(), HttpRequest::method, Must, BodyPipe::setBodySize(), size, Client::startedAdaptation, Client::virginBodyDestination, and Client::virginReply().
Referenced by Client::noteAdaptationAclCheckDone().
◆ startRequestBodyFlow()
|
protectedinherited |
Definition at line 238 of file Client.cc.
References assert, Http::Message::body_pipe, debugs, Client::originalRequest(), Client::requestBodySource, BodyPipe::setConsumerIfNotLate(), and BodyPipe::status().
Referenced by sendRequest().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ statusIfComplete()
|
private |
- If the reply wants to close the connection, it takes precedence
- If we sent a Connection:close request header, then this can not be a persistent connection.
- If we banned reuse, then this cannot be a persistent connection.
- If we haven't sent the whole request then this can not be a persistent connection.
- What does the reply have to say about keep-alive?
Definition at line 1061 of file http.cc.
References COMPLETE_NONPERSISTENT_MSG, COMPLETE_PERSISTENT_MSG, debugs, Client::entry, flags, Http::StateFlags::forceClose, Http::Message::header, httpHeaderHasConnDir(), HttpReply::keep_alive, Http::StateFlags::keepalive, HttpRequest::method, Client::request, Http::StateFlags::request_sent, StoreEntry::url(), and Client::virginReply().
Referenced by persistentConnStatus().
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by Client::cleanAdaptation(), Client::doneSendingRequestBody(), Client::endAdaptedBodyConsumption(), ClientHttpRequest::endRequestSatisfaction(), Client::handleAdaptedBodyProducerAborted(), Client::handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), ClientHttpRequest::noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), Client::serverComplete(), Client::swanSong(), and ClientHttpRequest::~ClientHttpRequest().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), Client::serverComplete2(), and ConnStateData::~ConnStateData().
◆ storeReplyBody()
|
protectedinherited |
Definition at line 1056 of file Client.cc.
References Client::currentOffset, Client::entry, and StoreEntry::write().
Referenced by Client::addVirginReplyBody().
◆ swanSong()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Relay.
Definition at line 68 of file Client.cc.
References Client::adaptedBodySource, assert, Client::cleanAdaptation(), Client::closeServer(), Client::doneWithFwd, Client::doneWithServer(), Client::fwd, FwdState::handleUnregisteredServerEnd(), Client::requestBodySource, BodyConsumer::stopConsumingFrom(), AsyncJob::swanSong(), and Client::virginBodyDestination.
Referenced by Ftp::Relay::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ truncateVirginBody()
|
private |
truncate what we read if we read too much so that writeReplyBody() writes no more than what we should have read
Definition at line 1386 of file http.cc.
References assert, SBuf::chop(), Http::Message::content_length, debugs, HttpReply::expectingBody(), flags, Http::StateFlags::headers_parsed, inBuf, SBuf::length(), HttpRequest::method, payloadSeen, payloadTruncated, Client::request, and Client::virginReply().
Referenced by writeReplyBody().
◆ virginReply() [1/2]
|
protectedinherited |
◆ virginReply() [2/2]
|
protectedinherited |
Definition at line 102 of file Client.cc.
References assert, and Client::theVirginReply.
Referenced by Client::adaptOrFinalizeReply(), continueAfterParsingHeader(), handleMoreRequestBodyAvailable(), Client::noteAdaptationAclCheckDone(), persistentConnStatus(), Client::startAdaptation(), statusIfComplete(), truncateVirginBody(), and writeReplyBody().
◆ writeReplyBody()
|
private |
Call this when there is data from the origin server which should be sent to either StoreEntry, or to ICAP...
Definition at line 1424 of file http.cc.
References Client::addVirginReplyBody(), SBuf::consume(), eof, inBuf, SBuf::length(), Client::markParsedVirginReplyAsWhole(), markPrematureReplyBodyEofFailure(), HttpRequest::method, payloadSeen, payloadTruncated, SBuf::rawContent(), Client::request, truncateVirginBody(), and Client::virginReply().
Referenced by processReplyBody().
◆ wroteLast()
|
private |
Definition at line 1715 of file http.cc.
References FwdState::al, StatCounters::all, closeServer(), debugs, Client::entry, Comm::ERR_CLOSING, ERR_WRITE_ERROR, FwdState::fail(), CommCommonCbParams::fd, fd_bytes(), CommCommonCbParams::flag, Client::fwd, HttpRequest::hier, StatCounters::http, StoreEntry::mem_obj, AsyncJob::mustStop(), HierarchyLogEntry::notePeerWrite(), Client::request, FwdState::request, Http::scBadGateway, sendComplete(), StatCounters::server, serverConnection, CommIoCbParams::size, statCounter, Write, and CommCommonCbParams::xerrno.
Referenced by finishingBrokenPost(), finishingChunkedRequest(), and sendRequest().
Member Data Documentation
◆ _peer
CachePeer* HttpStateData::_peer = nullptr |
Definition at line 66 of file http.h.
Referenced by HttpStateData(), keepaliveAccounting(), peerSupportsConnectionPinning(), sendRequest(), and ~HttpStateData().
◆ adaptationAccessCheckPending
|
protectedinherited |
Definition at line 189 of file Client.h.
Referenced by Client::adaptOrFinalizeReply(), Client::addVirginReplyBody(), Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteAdaptationAclCheckDone(), and processReplyBody().
◆ adaptedBodySource
|
protectedinherited |
to consume adated response body
Definition at line 187 of file Client.h.
Referenced by Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::endAdaptedBodyConsumption(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), Client::handleAdaptedHeader(), Client::handleMoreAdaptedBodyAvailable(), Client::noteBodyProducerAborted(), Client::noteBodyProductionEnded(), Client::noteMoreBodyDataAvailable(), Client::resumeBodyStorage(), Client::swanSong(), and Client::~Client().
◆ adaptedHeadSource
|
protectedinherited |
to get adapted response headers
Definition at line 186 of file Client.h.
Referenced by Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteAdaptationAnswer(), and Client::startAdaptation().
◆ closeHandler
|
private |
Definition at line 95 of file http.h.
Referenced by closeServer(), finishingBrokenPost(), HttpStateData(), proceedAfter1xx(), processReplyBody(), and sendRequest().
◆ completed
|
privateinherited |
serverComplete() has been called
Definition at line 90 of file Client.h.
Referenced by Client::noteMoreBodySpaceAvailable(), and Client::serverComplete().
◆ currentOffset
|
protectedinherited |
Our current offset in the StoreEntry
Definition at line 172 of file Client.h.
Referenced by Ftp::Gateway::getCurrentOffset(), Client::handleMoreAdaptedBodyAvailable(), Client::haveParsedReplyHeaders(), Ftp::Gateway::setCurrentOffset(), and Client::storeReplyBody().
◆ doneWithFwd
|
protectedinherited |
whether we should not be talking to FwdState; XXX: clear fwd instead points to a string literal which is used only for debugging
Definition at line 202 of file Client.h.
Referenced by Client::completeForwarding(), httpStateConnClosed(), proceedAfter1xx(), and Client::swanSong().
◆ entry
|
inherited |
Definition at line 176 of file Client.h.
Referenced by Client::abortOnBadEntry(), Client::blockCaching(), buildRequestPrefix(), Client::Client(), continueAfterParsingHeader(), Client::delayRead(), ftpFail(), ftpSendReply(), ftpWriteTransferDone(), Ftp::Gateway::Gateway(), Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), Client::handleMoreAdaptedBodyAvailable(), handleMoreRequestBodyAvailable(), handleRequestBodyProducerAborted(), haveParsedReplyHeaders(), httpBuildRequestHeader(), HttpStateData(), httpTimeout(), keepaliveAccounting(), peerSupportsConnectionPinning(), processReply(), processReplyBody(), processReplyHeader(), processSurrogateControl(), readReply(), Ftp::Relay::Relay(), reusableReply(), Client::sentRequestBody(), Client::setFinalReply(), statusIfComplete(), Client::storeReplyBody(), wroteLast(), and Client::~Client().
◆ eof
int HttpStateData::eof = 0 |
Definition at line 67 of file http.h.
Referenced by continueAfterParsingHeader(), decodeAndWriteReplyBody(), handleMoreRequestBodyAvailable(), maybeReadVirginBody(), persistentConnStatus(), processReplyHeader(), readReply(), and writeReplyBody().
◆ flags
Http::StateFlags HttpStateData::flags |
Definition at line 69 of file http.h.
Referenced by buildRequestPrefix(), calcReadBufferCapacityLimit(), checkDateSkew(), continueAfterParsingHeader(), decodeAndWriteReplyBody(), doneSendingRequestBody(), drop1xx(), finishingChunkedRequest(), forwardUpgrade(), getMoreRequestBody(), handle1xx(), handleMoreRequestBodyAvailable(), httpBuildRequestHeader(), HttpStateData(), keepaliveAccounting(), peerSupportsConnectionPinning(), persistentConnStatus(), proceedAfter1xx(), processReply(), processReplyBody(), processReplyHeader(), sendComplete(), sendRequest(), statusIfComplete(), and truncateVirginBody().
◆ fwd
|
inherited |
Definition at line 177 of file Client.h.
Referenced by Client::adaptOrFinalizeReply(), Client::blockCaching(), buildRequestPrefix(), Client::Client(), closeServer(), Client::completeForwarding(), continueAfterParsingHeader(), drop1xx(), Client::endAdaptedBodyConsumption(), finishingBrokenPost(), forwardUpgrade(), ftpFail(), ftpSendReply(), handle1xx(), Client::handleAdaptationBlocked(), Client::handleAdaptedHeader(), Client::handledEarlyAdaptationAbort(), Client::handleRequestBodyProducerAborted(), handleRequestBodyProducerAborted(), HttpStateData(), httpTimeout(), Client::markParsedVirginReplyAsWhole(), markPrematureReplyBodyEofFailure(), proceedAfter1xx(), processReplyBody(), readReply(), Client::sendBodyIsTooLargeError(), Client::sentRequestBody(), Client::setFinalReply(), Client::setVirginReply(), Client::startAdaptation(), Client::swanSong(), and wroteLast().
◆ hp
|
private |
Definition at line 147 of file http.h.
Referenced by processReplyHeader().
◆ httpChunkDecoder
|
private |
Definition at line 148 of file http.h.
Referenced by decodeAndWriteReplyBody(), processReplyHeader(), and ~HttpStateData().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ ignoreCacheControl
bool HttpStateData::ignoreCacheControl = false |
Definition at line 71 of file http.h.
Referenced by haveParsedReplyHeaders(), processSurrogateControl(), and reusableReply().
◆ inBuf
SBuf HttpStateData::inBuf |
Definition at line 70 of file http.h.
Referenced by canBufferMoreReplyBytes(), continueAfterParsingHeader(), decodeAndWriteReplyBody(), maybeMakeSpaceAvailable(), proceedAfter1xx(), processReplyHeader(), readReply(), truncateVirginBody(), and writeReplyBody().
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ lastChunk
int HttpStateData::lastChunk = 0 |
Definition at line 68 of file http.h.
Referenced by decodeAndWriteReplyBody(), maybeReadVirginBody(), and persistentConnStatus().
◆ payloadSeen
|
private |
Definition at line 151 of file http.h.
Referenced by persistentConnStatus(), proceedAfter1xx(), processReplyHeader(), readReply(), truncateVirginBody(), and writeReplyBody().
◆ payloadTruncated
|
private |
Definition at line 153 of file http.h.
Referenced by persistentConnStatus(), truncateVirginBody(), and writeReplyBody().
◆ receivedWholeAdaptedReply
|
protectedinherited |
Definition at line 193 of file Client.h.
Referenced by Client::endAdaptedBodyConsumption(), and Client::handleAdaptedBodyProductionEnded().
◆ receivedWholeRequestBody
|
protectedinherited |
Definition at line 195 of file Client.h.
Referenced by finishingChunkedRequest(), getMoreRequestBody(), Client::handleRequestBodyProductionEnded(), and Client::sentRequestBody().
◆ request
|
inherited |
Definition at line 178 of file Client.h.
Referenced by buildRequestPrefix(), checkDateSkew(), continueAfterParsingHeader(), decideIfWeDoRanges(), drop1xx(), forwardUpgrade(), ftpFail(), ftpReadType(), ftpSendPassive(), ftpSendReply(), ftpSendStor(), ftpSendType(), ftpSendUser(), ftpTrySlashHack(), Ftp::Gateway::Gateway(), handle1xx(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), handleMoreRequestBodyAvailable(), haveParsedReplyHeaders(), httpBuildRequestHeader(), HttpStateData(), keepaliveAccounting(), Client::maybePurgeOthers(), Client::noteAdaptationAclCheckDone(), Client::originalRequest(), peerSupportsConnectionPinning(), persistentConnStatus(), proceedAfter1xx(), processReplyBody(), processReplyHeader(), processSurrogateControl(), readReply(), reusableReply(), Client::sendBodyIsTooLargeError(), sendRequest(), Client::sentRequestBody(), statusIfComplete(), truncateVirginBody(), writeReplyBody(), and wroteLast().
◆ requestBodySource
|
protectedinherited |
to consume request body
Definition at line 181 of file Client.h.
Referenced by Client::doneSendingRequestBody(), Client::getMoreRequestBody(), getMoreRequestBody(), handleMoreRequestBodyAvailable(), Client::handleRequestBodyProducerAborted(), Client::noteBodyProducerAborted(), Client::noteBodyProductionEnded(), Client::noteMoreBodyDataAvailable(), Client::sendMoreRequestBody(), sendRequest(), Client::sentRequestBody(), Client::serverComplete(), Client::startRequestBodyFlow(), Client::swanSong(), and Client::~Client().
◆ requestSender
|
protectedinherited |
set if we are expecting Comm::Write to call us back
Definition at line 182 of file Client.h.
Referenced by finishingBrokenPost(), finishingChunkedRequest(), Client::handleMoreRequestBodyAvailable(), Client::handleRequestBodyProducerAborted(), Client::handleRequestBodyProductionEnded(), Client::sendMoreRequestBody(), sendRequest(), and Client::sentRequestBody().
◆ responseBodyBuffer
|
protectedinherited |
Data temporarily buffered for ICAP
Definition at line 173 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::calcBufferSpaceToReserve(), canBufferMoreReplyBytes(), Client::noteMoreBodySpaceAvailable(), readReply(), Client::replyBodySpace(), Client::serverComplete(), and Client::~Client().
◆ sawDateGoBack
|
private |
Whether we received a Date header older than that of a matching cached response.
Definition at line 160 of file http.h.
Referenced by haveParsedReplyHeaders(), and reusableReply().
◆ serverConnection
|
private |
The current server connection. Maybe open, closed, or NULL. Use doneWithServer() to check if the server is available for use.
Definition at line 94 of file http.h.
Referenced by abortAll(), closeServer(), dataConnection(), doneSendingRequestBody(), doneWithServer(), finishingBrokenPost(), finishingChunkedRequest(), handleMoreRequestBodyAvailable(), handleRequestBodyProducerAborted(), HttpStateData(), httpTimeout(), maybeMakeSpaceAvailable(), maybeReadVirginBody(), persistentConnStatus(), proceedAfter1xx(), processReplyBody(), processReplyHeader(), readReply(), sendComplete(), sendRequest(), wroteLast(), and ~HttpStateData().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ startedAdaptation
|
protectedinherited |
Definition at line 190 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::addVirginReplyBody(), Client::markParsedVirginReplyAsWhole(), and Client::startAdaptation().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ surrogateNoStore
bool HttpStateData::surrogateNoStore = false |
Definition at line 72 of file http.h.
Referenced by processSurrogateControl(), and reusableReply().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ theFinalReply
|
privateinherited |
adapted reply from ICAP or virgin reply
Definition at line 209 of file Client.h.
Referenced by Client::finalReply(), Client::haveParsedReplyHeaders(), Client::maybePurgeOthers(), Client::setFinalReply(), and Client::~Client().
◆ theVirginReply
|
privateinherited |
reply received from the origin server
Definition at line 208 of file Client.h.
Referenced by Client::setVirginReply(), Client::virginReply(), and Client::~Client().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ upgradeHeaderOut
String* HttpStateData::upgradeHeaderOut = nullptr |
Definition at line 75 of file http.h.
Referenced by blockSwitchingProtocols(), buildRequestPrefix(), and ~HttpStateData().
◆ virginBodyDestination
|
protectedinherited |
to provide virgin response body
Definition at line 185 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::calcBufferSpaceToReserve(), Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteBodyConsumerAborted(), readReply(), Client::replyBodySpace(), Client::serverComplete2(), Client::startAdaptation(), Client::swanSong(), and Client::~Client().
◆ waitingForCommRead
|
private |
Definition at line 156 of file http.h.
Referenced by maybeReadVirginBody(), and readReply().
◆ waitingForDelayAwareReadChance
|
protectedinherited |
Definition at line 198 of file Client.h.
Referenced by Client::delayRead(), maybeReadVirginBody(), and noteDelayAwareReadChance().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products