#include <Checklist.h>
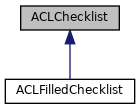
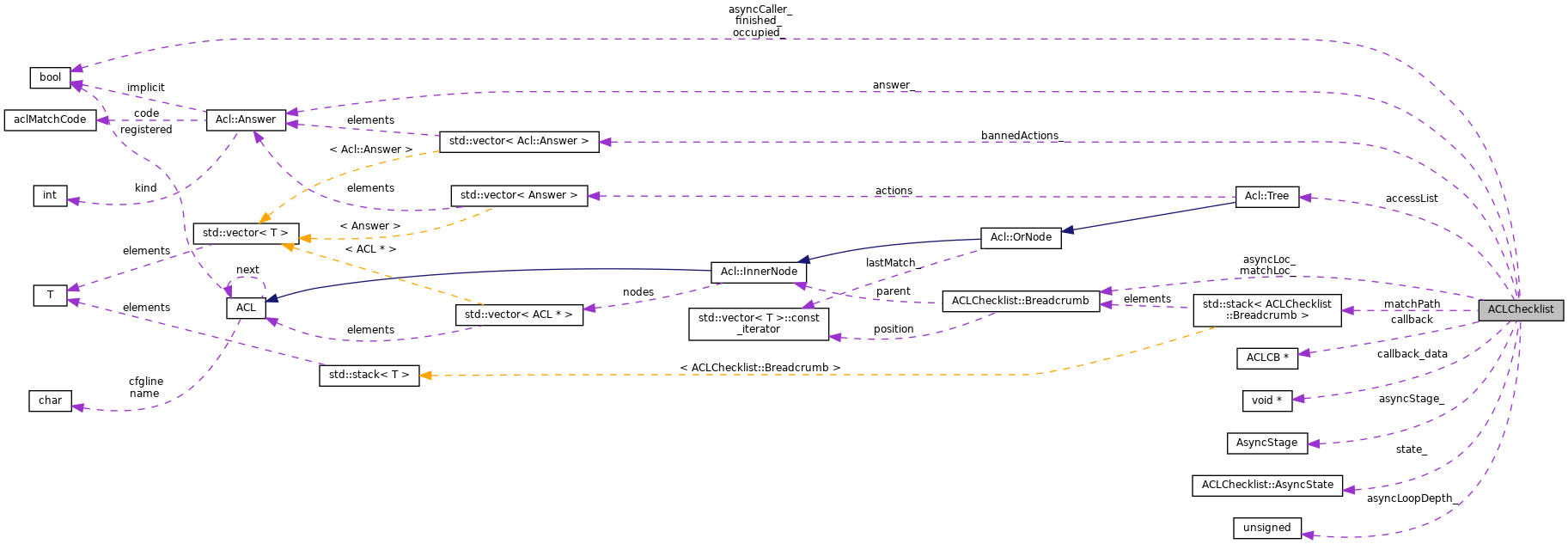
Classes | |
class | Breadcrumb |
Position of a child node within an Acl::Node tree. More... | |
Public Types | |
using | AsyncStarter = void(ACLFilledChecklist &, const Acl::Node &) |
a function that initiates asynchronous ACL checks; see goAsync() More... | |
Public Member Functions | |
ACLChecklist () | |
virtual | ~ACLChecklist () |
const Acl::Answer & | fastCheck () |
const Acl::Answer & | fastCheck (const ACLList *) |
bool | goAsync (AsyncStarter, const Acl::Node &) |
bool | matchChild (const Acl::InnerNode *parent, Acl::Nodes::const_iterator pos) |
bool | keepMatching () const |
Whether we should continue to match tree nodes or stop/pause. More... | |
bool | finished () const |
whether markFinished() was called More... | |
bool | asyncInProgress () const |
async call has been started and has not finished (or failed) yet More... | |
void | markFinished (const Acl::Answer &newAnswer, const char *reason) |
const Acl::Answer & | currentAnswer () const |
bool | bannedAction (const Acl::Answer &action) const |
whether the action is banned or not More... | |
void | banAction (const Acl::Answer &action) |
add action to the list of banned actions More... | |
virtual bool | hasRequest () const =0 |
virtual bool | hasReply () const =0 |
virtual bool | hasAle () const =0 |
virtual void | syncAle (HttpRequest *adaptedRequest, const char *logUri) const =0 |
assigns uninitialized adapted_request and url ALE components More... | |
virtual void | verifyAle () const =0 |
warns if there are uninitialized ALE components and fills them More... | |
void | changeAcl (const acl_access *) |
change the current ACL list More... | |
void | setLastCheckedName (const SBuf &name) |
remember the name of the last ACL being evaluated More... | |
void | resumeNonBlockingCheck () |
Public Attributes | |
ACLCB * | callback |
void * | callback_data |
Protected Member Functions | |
void | nonBlockingCheck (ACLCB *callback, void *callback_data) |
Private Types | |
enum | NodeMatchingResult { nmrMatch, nmrMismatch, nmrFinished, nmrNeedsAsync } |
possible outcomes when trying to match a single ACL node in a list More... | |
enum | AsyncStage { asyncNone, asyncStarting, asyncRunning, asyncFailed } |
Private Member Functions | |
void | checkCallback (const char *abortReason) |
void | matchAndFinish () |
performs (or resumes) an ACL tree match and, if successful, sets the action More... | |
Acl::TreePointer | swapAcl (const acl_access *) |
change the current ACL list More... | |
void | preCheck (const char *what) |
prepare for checking ACLs; called once per check More... | |
bool | prepNonBlocking () |
common parts of nonBlockingCheck() and resumeNonBlockingCheck() More... | |
void | completeNonBlocking () |
void | calcImplicitAnswer () |
bool | callerGone () |
Private Attributes | |
Acl::TreePointer | accessList |
bool | asyncCaller_ |
whether the caller supports async/slow ACLs More... | |
bool | occupied_ |
whether a check (fast or non-blocking) is in progress More... | |
bool | finished_ |
Acl::Answer | answer_ |
AsyncStage | asyncStage_ |
Breadcrumb | matchLoc_ |
location of the node running matches() now More... | |
Breadcrumb | asyncLoc_ |
currentNode_ that called goAsync() More... | |
unsigned | asyncLoopDepth_ |
how many times the current async state has resumed More... | |
std::stack< Breadcrumb > | matchPath |
suspended (due to an async lookup) matches() in the ACL tree More... | |
std::vector< Acl::Answer > | bannedActions_ |
the list of actions which must ignored during acl checks More... | |
std::optional< SBuf > | lastCheckedName_ |
the name of the last evaluated ACL (if any ACLs were evaluated) More... | |
Detailed Description
Base class for maintaining Squid and transaction state for access checks. Provides basic ACL checking methods. Its only child, ACLFilledChecklist, keeps the actual state data. The split is necessary to avoid exposing all ACL-related code to virtually Squid data types.
Definition at line 30 of file Checklist.h.
Member Typedef Documentation
◆ AsyncStarter
using ACLChecklist::AsyncStarter = void (ACLFilledChecklist &, const Acl::Node &) |
Definition at line 36 of file Checklist.h.
Member Enumeration Documentation
◆ AsyncStage
|
private |
Enumerator | |
---|---|
asyncNone | |
asyncStarting | |
asyncRunning | |
asyncFailed |
Definition at line 204 of file Checklist.h.
◆ NodeMatchingResult
|
private |
Enumerator | |
---|---|
nmrMatch | |
nmrMismatch | |
nmrFinished | |
nmrNeedsAsync |
Definition at line 190 of file Checklist.h.
Constructor & Destructor Documentation
◆ ACLChecklist()
ACLChecklist::ACLChecklist | ( | ) |
Definition at line 167 of file Checklist.cc.
◆ ~ACLChecklist()
|
virtual |
Definition at line 180 of file Checklist.cc.
References assert, asyncInProgress(), and debugs.
Member Function Documentation
◆ asyncInProgress()
|
inline |
Definition at line 101 of file Checklist.h.
References asyncNone, and asyncStage_.
Referenced by completeNonBlocking(), goAsync(), keepMatching(), markFinished(), matchChild(), Acl::Node::matches(), nonBlockingCheck(), Acl::InnerNode::resumeMatchingAt(), resumeNonBlockingCheck(), ~ACLChecklist(), and ACLFilledChecklist::~ACLFilledChecklist().
◆ banAction()
void ACLChecklist::banAction | ( | const Acl::Answer & | action | ) |
Definition at line 358 of file Checklist.cc.
References bannedActions_.
◆ bannedAction()
bool ACLChecklist::bannedAction | ( | const Acl::Answer & | action | ) | const |
Definition at line 350 of file Checklist.cc.
References bannedActions_, debugs, and Acl::Answer::kind.
Referenced by Acl::Tree::bannedAction().
◆ calcImplicitAnswer()
|
private |
When no rules matched, the answer is the inversion of the last rule action (or ACCESS_DUNNO if the reversal is not possible).
Definition at line 326 of file Checklist.cc.
References ACCESS_ALLOWED, ACCESS_DENIED, ACCESS_DUNNO, accessList, debugs, Acl::Tree::lastAction(), and markFinished().
Referenced by completeNonBlocking(), and fastCheck().
◆ callerGone()
|
private |
Definition at line 344 of file Checklist.cc.
References callback_data, and cbdataReferenceValid().
Referenced by prepNonBlocking().
◆ changeAcl()
void ACLChecklist::changeAcl | ( | const acl_access * | replacement | ) |
Definition at line 187 of file Checklist.cc.
References accessList.
Referenced by ACLFilledChecklist::ACLFilledChecklist(), fastCheck(), HttpStateData::forwardUpgrade(), swapAcl(), and ConnStateData::whenClientIpKnown().
◆ checkCallback()
|
private |
Calls non-blocking check callback with the answer and destroys self. If abortReason is provided, sets the final answer to ACCESS_DUNNO.
Definition at line 146 of file Checklist.cc.
References ACCESS_DUNNO, Assure, callback, callback_data, cbdataReferenceValidDone, currentAnswer(), finished(), markFinished(), and occupied_.
Referenced by completeNonBlocking(), nonBlockingCheck(), and prepNonBlocking().
◆ completeNonBlocking()
|
private |
Definition at line 34 of file Checklist.cc.
References assert, asyncInProgress(), calcImplicitAnswer(), checkCallback(), and finished().
Referenced by nonBlockingCheck(), and resumeNonBlockingCheck().
◆ currentAnswer()
|
inline |
Definition at line 106 of file Checklist.h.
References answer_.
Referenced by checkCallback(), fastCheck(), and FwdState::Start().
◆ fastCheck() [1/2]
const Acl::Answer & ACLChecklist::fastCheck | ( | ) |
Perform a blocking (immediate) check for a list of allow/deny rules. Each rule comes with a list of ACLs.
The first rule where all ACLs match wins. If there is such a rule, the result becomes that rule keyword (ACCESS_ALLOWED or ACCESS_DENIED).
If there are rules but all ACL lists mismatch, an implicit rule is used Its result is the negation of the keyword of the last seen rule.
Some ACLs may stop the check prematurely by setting an exceptional check result (e.g., ACCESS_AUTH_REQUIRED) instead of declaring a match or mismatch.
Some ACLs may require an async lookup which is prohibited by this method. In this case, the exceptional check result of ACCESS_DUNNO is immediately returned.
If there are no rules to check at all, the result becomes ACCESS_DUNNO.
Definition at line 298 of file Checklist.cc.
References accessList, asyncCaller_, calcImplicitAnswer(), currentAnswer(), debugs, finished(), matchAndFinish(), occupied_, and preCheck().
Referenced by accessLogLogTo(), aclFindNfMarkConfig(), aclMapTOS(), Client::blockCaching(), clientReplyContext::blockedHit(), ConnStateData::buildSslCertGenerationParams(), HttpReply::calcMaxBodySize(), Adaptation::Icap::Launcher::canRepeat(), FwdState::connectStart(), DelayId::DelayClient(), HttpStateData::finishingBrokenPost(), HttpStateData::forwardUpgrade(), getOutgoingAddress(), HttpRequest::getRangeOffsetLimit(), HttpStateData::handle1xx(), Ftp::Server::handleUploadRequest(), htcpAccessAllowed(), httpHdrAdd(), httpHdrMangle(), icpAccessAllowed(), ClientHttpRequest::logRequest(), HttpRequest::manager(), Note::match(), StoreClient::onCollapsingPath(), peerAllowedToUse(), Http::One::Server::processParsedRequest(), ConnStateData::proxyProtocolValidateClient(), schemesConfig(), Ftp::Client::sendPassive(), Http::Stream::sendStartOfMessage(), ConnStateData::serveDelayedError(), Security::KeyLogger::shouldLog(), snmpDecodePacket(), ssl_verify_cb(), FwdState::Start(), ConnStateData::tunnelOnError(), tunnelStart(), and ConnStateData::whenClientIpKnown().
◆ fastCheck() [2/2]
const Acl::Answer & ACLChecklist::fastCheck | ( | const ACLList * | list | ) |
Perform a blocking (immediate) check whether a list of ACLs matches. This method is meant to be used with squid.conf ACL-driven options that lack allow/deny keywords and are tested one ACL list at a time. Whether the checks for other occurrences of the same option continue after this call is up to the caller and option semantics.
If all ACLs match, the result becomes ACCESS_ALLOWED.
If all ACLs mismatch, the result becomes ACCESS_DENIED.
Some ACLs may stop the check prematurely by setting an exceptional check result (e.g., ACCESS_AUTH_REQUIRED) instead of declaring a match or mismatch.
Some ACLs may require an async lookup which is prohibited by this method. In this case, the exceptional check result of ACCESS_DUNNO is immediately returned.
If there are no ACLs to check at all, the result becomes ACCESS_ALLOWED.
Definition at line 273 of file Checklist.cc.
References ACCESS_DENIED, accessList, asyncCaller_, changeAcl(), currentAnswer(), finished(), markFinished(), matchAndFinish(), occupied_, preCheck(), and swapAcl().
◆ finished()
|
inline |
Definition at line 99 of file Checklist.h.
References finished_.
Referenced by checkCallback(), completeNonBlocking(), fastCheck(), keepMatching(), ACLFilledChecklist::markDestinationDomainChecked(), markFinished(), ACLFilledChecklist::markSourceDomainChecked(), and resumeNonBlockingCheck().
◆ goAsync()
bool ACLChecklist::goAsync | ( | AsyncStarter | starter, |
const Acl::Node & | acl | ||
) |
If slow lookups are allowed, switches into "async in progress" state. Otherwise, returns false; the caller is expected to handle the failure.
Definition at line 104 of file Checklist.cc.
References assert, asyncCaller_, asyncFailed, asyncInProgress(), asyncLoc_, asyncLoopDepth_, asyncNone, asyncRunning, asyncStage_, asyncStarting, debugs, Filled(), matchLoc_, and ACLChecklist::Breadcrumb::parent.
Referenced by ACLExternal::aclMatchExternal(), AuthenticateAcl(), and ACLDestinationIP::match().
◆ hasAle()
|
pure virtual |
Implemented in ACLFilledChecklist.
Referenced by Acl::Node::matches(), and ACLHasComponentData::parseComponent().
◆ hasReply()
|
pure virtual |
Implemented in ACLFilledChecklist.
Referenced by Acl::Node::matches(), and ACLHasComponentData::parseComponent().
◆ hasRequest()
|
pure virtual |
Implemented in ACLFilledChecklist.
Referenced by Acl::ConnectionsEncrypted::match(), Acl::Node::matches(), and ACLHasComponentData::parseComponent().
◆ keepMatching()
|
inline |
Definition at line 96 of file Checklist.h.
References asyncInProgress(), and finished().
Referenced by Acl::AllOf::doMatch(), Acl::NotNode::doMatch(), Acl::AndNode::doMatch(), Acl::OrNode::doMatch(), ACLMaxUserIP::match(), ACLExternal::match(), and ACLProxyAuth::match().
◆ markFinished()
void ACLChecklist::markFinished | ( | const Acl::Answer & | newAnswer, |
const char * | reason | ||
) |
called when no more ACLs should be checked; sets the final answer and prints a debugging message explaining the reason for that answer
Definition at line 45 of file Checklist.cc.
References answer_, assert, asyncInProgress(), debugs, finished(), finished_, Acl::Answer::lastCheckedName, and lastCheckedName_.
Referenced by calcImplicitAnswer(), checkCallback(), fastCheck(), ACLMaxUserIP::match(), ACLExternal::match(), ACLProxyAuth::match(), and matchAndFinish().
◆ matchAndFinish()
|
private |
Definition at line 257 of file Checklist.cc.
References accessList, markFinished(), Acl::Node::matches(), matchPath, ACLChecklist::Breadcrumb::parent, ACLChecklist::Breadcrumb::position, Acl::InnerNode::resumeMatchingAt(), and Acl::Tree::winningAction().
Referenced by fastCheck(), nonBlockingCheck(), and resumeNonBlockingCheck().
◆ matchChild()
bool ACLChecklist::matchChild | ( | const Acl::InnerNode * | parent, |
Acl::Nodes::const_iterator | pos | ||
) |
Matches (or resumes matching of) a child node at pos while maintaining resumption breadcrumbs if a [grand]child node goes async.
Definition at line 70 of file Checklist.cc.
References assert, asyncInProgress(), asyncLoc_, asyncLoopDepth_, ACLChecklist::Breadcrumb::clear(), matchLoc_, matchPath, ACLChecklist::Breadcrumb::parent, ACLChecklist::Breadcrumb::position, and Acl::InnerNode::resumeMatchingAt().
Referenced by Acl::AllOf::doMatch(), Acl::NotNode::doMatch(), Acl::AndNode::doMatch(), and Acl::OrNode::doMatch().
◆ nonBlockingCheck()
|
protected |
Start a non-blocking (async) check for a list of allow/deny rules. Each rule comes with a list of ACLs.
The callback specified will be called with the result of the check.
The first rule where all ACLs match wins. If there is such a rule, the result becomes that rule keyword (ACCESS_ALLOWED or ACCESS_DENIED).
If there are rules but all ACL lists mismatch, an implicit rule is used. Its result is the negation of the keyword of the last seen rule.
Some ACLs may stop the check prematurely by setting an exceptional check result (e.g., ACCESS_AUTH_REQUIRED) instead of declaring a match or mismatch.
If there are no rules to check at all, the result becomes ACCESS_DUNNO. Calling this method with no rules to check wastes a lot of CPU cycles and will result in a DBG_CRITICAL debugging message.
Kick off a non-blocking (slow) ACL access list test
NP: this should probably be made Async now.
The ACL list should NEVER be NULL when calling this method. Always caller should check for NULL and handle appropriate to its needs first. We cannot select a sensible default for all callers here.
Definition at line 206 of file Checklist.cc.
References accessList, asyncCaller_, asyncInProgress(), callback, callback_data, cbdataReference, checkCallback(), completeNonBlocking(), DBG_CRITICAL, debugs, matchAndFinish(), preCheck(), and prepNonBlocking().
◆ preCheck()
|
private |
Called first (and once) by all checks to initialize their state.
Definition at line 56 of file Checklist.cc.
References assert, asyncLoopDepth_, debugs, finished_, lastCheckedName_, and occupied_.
Referenced by fastCheck(), and nonBlockingCheck().
◆ prepNonBlocking()
|
private |
Definition at line 21 of file Checklist.cc.
References accessList, assert, callerGone(), and checkCallback().
Referenced by nonBlockingCheck(), and resumeNonBlockingCheck().
◆ resumeNonBlockingCheck()
void ACLChecklist::resumeNonBlockingCheck | ( | ) |
Resumes non-blocking check started by nonBlockingCheck() and suspended until some async operation updated Squid state.
Definition at line 230 of file Checklist.cc.
References assert, asyncFailed, asyncInProgress(), asyncNone, asyncRunning, asyncStage_, asyncStarting, completeNonBlocking(), finished(), matchAndFinish(), matchPath, and prepNonBlocking().
Referenced by LookupDone(), ACLDestinationIP::LookupDone(), ACLProxyAuth::LookupDone(), and ACLExternal::LookupDone().
◆ setLastCheckedName()
|
inline |
Definition at line 128 of file Checklist.h.
References lastCheckedName_.
Referenced by Acl::Node::matches().
◆ swapAcl()
|
private |
- Returns
- old accessList pointer (that may be nil)
Definition at line 193 of file Checklist.cc.
References accessList, and changeAcl().
Referenced by fastCheck().
◆ syncAle()
|
pure virtual |
Implemented in ACLFilledChecklist.
◆ verifyAle()
|
pure virtual |
Implemented in ACLFilledChecklist.
Referenced by Acl::Node::matches().
Member Data Documentation
◆ accessList
|
private |
Definition at line 164 of file Checklist.h.
Referenced by calcImplicitAnswer(), changeAcl(), fastCheck(), matchAndFinish(), nonBlockingCheck(), prepNonBlocking(), and swapAcl().
◆ answer_
|
private |
Definition at line 202 of file Checklist.h.
Referenced by currentAnswer(), and markFinished().
◆ asyncCaller_
|
private |
Definition at line 199 of file Checklist.h.
Referenced by fastCheck(), goAsync(), and nonBlockingCheck().
◆ asyncLoc_
|
private |
Definition at line 207 of file Checklist.h.
Referenced by goAsync(), and matchChild().
◆ asyncLoopDepth_
|
private |
Definition at line 208 of file Checklist.h.
Referenced by goAsync(), matchChild(), and preCheck().
◆ asyncStage_
|
private |
Definition at line 205 of file Checklist.h.
Referenced by asyncInProgress(), goAsync(), and resumeNonBlockingCheck().
◆ bannedActions_
|
private |
Definition at line 215 of file Checklist.h.
Referenced by banAction(), and bannedAction().
◆ callback
ACLCB* ACLChecklist::callback |
Definition at line 168 of file Checklist.h.
Referenced by checkCallback(), and nonBlockingCheck().
◆ callback_data
void* ACLChecklist::callback_data |
Definition at line 169 of file Checklist.h.
Referenced by callerGone(), checkCallback(), and nonBlockingCheck().
◆ finished_
|
private |
Definition at line 201 of file Checklist.h.
Referenced by finished(), markFinished(), and preCheck().
◆ lastCheckedName_
|
private |
Definition at line 218 of file Checklist.h.
Referenced by markFinished(), preCheck(), and setLastCheckedName().
◆ matchLoc_
|
private |
Definition at line 206 of file Checklist.h.
Referenced by goAsync(), and matchChild().
◆ matchPath
|
private |
Definition at line 213 of file Checklist.h.
Referenced by matchAndFinish(), matchChild(), and resumeNonBlockingCheck().
◆ occupied_
|
private |
Definition at line 200 of file Checklist.h.
Referenced by checkCallback(), fastCheck(), and preCheck().
The documentation for this class was generated from the following files:
- src/acl/Checklist.h
- src/acl/Checklist.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products