#include <Tree.h>
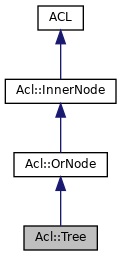
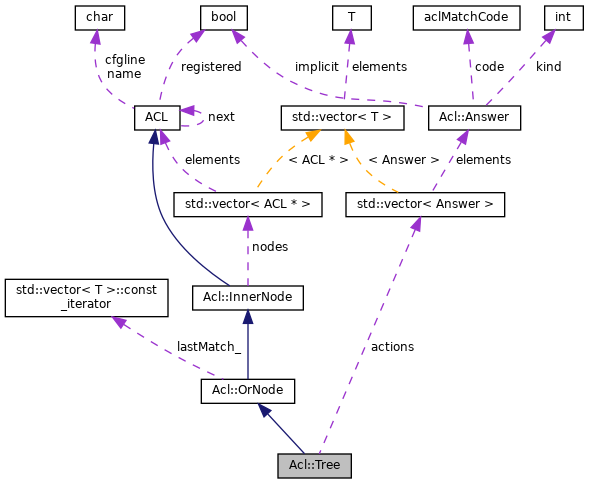
Public Types | |
using | Pointer = RefCount< Node > |
Public Member Functions | |
template<class ActionToStringConverter > | |
SBufList | treeDump (const char *name, ActionToStringConverter converter) const |
Answer | winningAction () const |
Returns the corresponding action after a successful tree match. More... | |
Answer | lastAction () const |
what action to use if no nodes matched More... | |
void | add (Acl::Node *rule, const Answer &action) |
appends and takes control over the rule with a given action More... | |
void | add (Acl::Node *rule) |
same as InnerNode::add() More... | |
virtual bool | bannedAction (ACLChecklist *, Nodes::const_iterator) const |
const char * | typeString () const override |
void | parse () override |
parses node representation in squid.conf; dies on failures More... | |
bool | resumeMatchingAt (ACLChecklist *checklist, Acl::Nodes::const_iterator pos) const |
Resumes matching (suspended by an async call) at the given position. More... | |
Nodes::size_type | childrenCount () const |
the number of children nodes More... | |
void | prepareForUse () override |
bool | empty () const override |
SBufList | dump () const override |
size_t | lineParse () |
void * | operator new (size_t) |
void | operator delete (void *) |
void | context (const SBuf &aName, const char *configuration) |
sets user-specified ACL name and squid.conf context More... | |
bool | matches (ACLChecklist *checklist) const |
void | parseFlags () |
configures Acl::Node options, throwing on configuration errors More... | |
virtual bool | isProxyAuth () const |
virtual bool | valid () const |
int | cacheMatchAcl (dlink_list *cache, ACLChecklist *) |
virtual int | matchForCache (ACLChecklist *checklist) |
void | dumpWhole (const char *directiveName, std::ostream &) |
Static Public Member Functions | |
static void | ParseNamedAcl (ConfigParser &, NamedAcls *&) |
parses acl directive parts that follow directive name (i.e. "acl") More... | |
static void | Initialize () |
static Acl::Node * | FindByName (const SBuf &) |
A configured ACL with a given name or nil. More... | |
Public Attributes | |
SBuf | name |
char * | cfgline = nullptr |
Protected Types | |
typedef std::vector< Answer > | Actions |
if not empty, contains actions corresponding to InnerNode::nodes More... | |
Protected Member Functions | |
bool | bannedAction (ACLChecklist *, Nodes::const_iterator) const override |
Acl::OrNode API. More... | |
Answer | actionAt (const Nodes::size_type pos) const |
computes action that corresponds to the position of the matched rule More... | |
virtual int | doMatch (ACLChecklist *checklist, Nodes::const_iterator start) const =0 |
int | match (ACLChecklist *checklist) override |
Matches the actual data in checklist against this Acl::Node. More... | |
Protected Attributes | |
Actions | actions |
Nodes::const_iterator | lastMatch_ |
Nodes | nodes |
children of this intermediate node More... | |
Private Member Functions | |
MEMPROXY_CLASS (Tree) | |
int | doMatch (ACLChecklist *checklist, Nodes::const_iterator start) const override |
virtual bool | requiresAle () const |
whether our (i.e. shallow) match() requires checklist to have a AccessLogEntry More... | |
virtual bool | requiresRequest () const |
whether our (i.e. shallow) match() requires checklist to have a request More... | |
virtual bool | requiresReply () const |
whether our (i.e. shallow) match() requires checklist to have a reply More... | |
virtual const Acl::Options & | options () |
virtual const Acl::Options & | lineOptions () |
Static Private Member Functions | |
static void | ParseNamed (ConfigParser &, NamedAcls &, const SBuf &name) |
parses acl directive parts that follow aclname More... | |
Detailed Description
An ORed set of rules at the top of the ACL expression tree with support for optional rule actions.
Member Typedef Documentation
◆ Actions
|
protected |
◆ Pointer
|
inherited |
Member Function Documentation
◆ actionAt()
|
protected |
Definition at line 30 of file Tree.cc.
References ACCESS_ALLOWED, and assert.
Referenced by winningAction().
◆ add() [1/2]
void Acl::Tree::add | ( | Acl::Node * | rule | ) |
Definition at line 51 of file Tree.cc.
References Acl::InnerNode::add(), and assert.
◆ add() [2/2]
Definition at line 42 of file Tree.cc.
References Acl::InnerNode::add(), and assert.
Referenced by aclParseAclList().
◆ bannedAction() [1/2]
|
virtualinherited |
whether the given rule should be excluded from matching tests based on its action
Definition at line 99 of file BoolOps.cc.
◆ bannedAction() [2/2]
|
overrideprotected |
Definition at line 59 of file Tree.cc.
References assert, and ACLChecklist::bannedAction().
◆ cacheMatchAcl()
|
inherited |
Definition at line 401 of file Acl.cc.
References acl_proxy_auth_match_cache::acl_data, dlink_node::data, debugs, dlinkAddTail(), dlink_list::head, acl_proxy_auth_match_cache::link, acl_proxy_auth_match_cache::matchrv, and dlink_node::next.
Referenced by ACLProxyAuth::matchProxyAuth().
◆ childrenCount()
|
inlineinherited |
Definition at line 29 of file InnerNode.h.
References Acl::InnerNode::nodes.
Referenced by aclParseAccessLine(), and Acl::AllOf::parse().
◆ context()
|
inherited |
Definition at line 220 of file Acl.cc.
References safe_free, and xstrdup.
Referenced by aclParseAccessLine(), aclParseAclList(), Acl::AllOf::parse(), and ParseAclWithAction().
◆ doMatch() [1/2]
|
protectedpure virtualinherited |
checks whether the nodes match, starting with the given one kids determine what a match means for their type of intermediate nodes
Implemented in Acl::AndNode, Acl::NotNode, and Acl::AllOf.
◆ doMatch() [2/2]
|
overrideprivateinherited |
Definition at line 105 of file BoolOps.cc.
References ACLChecklist::keepMatching(), and ACLChecklist::matchChild().
◆ dump()
|
overridevirtualinherited |
Implements Acl::Node.
Definition at line 81 of file InnerNode.cc.
◆ dumpWhole()
|
inherited |
◆ empty()
|
overridevirtualinherited |
Implements Acl::Node.
Definition at line 30 of file InnerNode.cc.
Referenced by aclParseAccessLine().
◆ FindByName()
Definition at line 159 of file Acl.cc.
References assert, Config, debugs, and SquidConfig::namedAcls.
Referenced by aclIsProxyAuth(), Acl::InnerNode::lineParse(), parse_ftp_epsv(), and TestACLMaxUserIP::testParseLine().
◆ Initialize()
|
staticinherited |
Definition at line 471 of file Acl.cc.
References Config, debugs, and SquidConfig::namedAcls.
Referenced by serverConnectionsOpen().
◆ isProxyAuth()
|
virtualinherited |
Reimplemented in ACLExternal, and ACLProxyAuth.
◆ lastAction()
Acl::Answer Acl::Tree::lastAction | ( | ) | const |
Definition at line 21 of file Tree.cc.
References ACCESS_DUNNO.
Referenced by ACLChecklist::calcImplicitAnswer().
◆ lineOptions()
|
inlineprivatevirtualinherited |
- Returns
- (linked) "line" Options supported by this Acl::Node
- See also
- Acl::Node::options()
Reimplemented in ACLProxyAuth, Acl::ParameterizedNode< P >, Acl::ParameterizedNode< ACLData< const Security::CertErrors * > >, Acl::ParameterizedNode< ACLData< ACLChecklist * > >, Acl::ParameterizedNode< ACLData< Ip::Address > >, Acl::ParameterizedNode< ACLData< const char * > >, Acl::ParameterizedNode< ACLData< XactionStep > >, Acl::ParameterizedNode< ACLData< AnyP::ProtocolType > >, Acl::ParameterizedNode< ACLData< NotePairs::Entry * > >, Acl::ParameterizedNode< ACLData< HttpRequestMethod > >, Acl::ParameterizedNode< ACLData< int > >, Acl::ParameterizedNode< ACLData< err_type > >, Acl::ParameterizedNode< ACLData< const HttpHeader & > >, Acl::ParameterizedNode< ACLData< X509 * > >, Acl::ParameterizedNode< ACLTimeData >, Acl::ParameterizedNode< ACLData< hier_code > >, and ACLExtUser.
Definition at line 102 of file Node.h.
References Acl::NoOptions().
◆ lineParse()
|
inherited |
parses a [ [!]acl1 [!]acl2... ] sequence, appending to nodes
- Returns
- the number of parsed ACL names
Definition at line 44 of file InnerNode.cc.
References config_input_line, DBG_CRITICAL, debugs, Acl::Node::FindByName(), self_destruct(), ConfigParser::strtokFile(), and xstrdup.
Referenced by aclParseAccessLine(), aclParseAclList(), Acl::AllOf::parse(), and ParseAclWithAction().
◆ match()
|
overrideprotectedvirtualinherited |
Implements Acl::Node.
Definition at line 90 of file InnerNode.cc.
◆ matches()
|
inherited |
Orchestrates matching checklist against the Acl::Node using match(), after checking preconditions and while providing debugging.
- Returns
- true if and only if there was a successful match. Updates the checklist state on match, async, and failure.
Definition at line 189 of file Acl.cc.
References ACLChecklist::asyncInProgress(), DBG_IMPORTANT, debugs, ACLChecklist::hasAle(), ACLChecklist::hasReply(), ACLChecklist::hasRequest(), ACLChecklist::setLastCheckedName(), and ACLChecklist::verifyAle().
Referenced by ACLChecklist::matchAndFinish().
◆ matchForCache()
|
virtualinherited |
◆ MEMPROXY_CLASS()
|
private |
◆ operator delete()
|
inherited |
◆ operator new()
|
inherited |
◆ options()
|
inlineprivatevirtualinherited |
- Returns
- (linked) 'global' Options supported by this Acl::Node
Reimplemented in Acl::ServerNameCheck, Acl::DestinationDomainCheck, Acl::AnnotationCheck, ACLMaxUserIP, and ACLDestinationIP.
Definition at line 98 of file Node.h.
References Acl::NoOptions().
◆ parse()
|
overridevirtualinherited |
◆ parseFlags()
|
inherited |
Definition at line 360 of file Acl.cc.
References Acl::ParseFlags().
◆ ParseNamed()
|
staticprivateinherited |
Definition at line 253 of file Acl.cc.
References A, Assure, SBuf::cmp(), config_input_line, DBG_CRITICAL, DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, ConfigParser::destruct(), fatalf(), HttpPortList, Acl::Make(), and ConfigParser::NextToken().
◆ ParseNamedAcl()
|
staticinherited |
Definition at line 229 of file Acl.cc.
References CallParser(), DBG_CRITICAL, debugs, ConfigParser::destruct(), RefCount< C >::Make(), and ConfigParser::NextToken().
Referenced by parse_acl(), and TestACLMaxUserIP::testParseLine().
◆ prepareForUse()
|
overridevirtualinherited |
Reimplemented from Acl::Node.
Definition at line 23 of file InnerNode.cc.
References Acl::InnerNode::nodes.
◆ requiresAle()
|
privatevirtualinherited |
Reimplemented in ACLExternal.
◆ requiresReply()
|
privatevirtualinherited |
Reimplemented in ACLHTTPStatus, Acl::ReplyHeaderCheck< header >, and Acl::HttpRepHeaderCheck.
◆ requiresRequest()
|
privatevirtualinherited |
Reimplemented in Acl::NoteCheck, ACLProxyAuth, ACLExternal, Acl::ServerNameCheck, ACLMaxUserIP, ACLExtUser, Acl::TransactionInitiator, Acl::RequestHeaderCheck< header >, Acl::DestinationDomainCheck, Acl::DestinationAsnCheck, Acl::HierCodeCheck, Acl::HttpReqHeaderCheck, Acl::MethodCheck, Acl::ProtocolCheck, Acl::UrlCheck, Acl::UrlLoginCheck, Acl::UrlPathCheck, and Acl::UrlPortCheck.
◆ resumeMatchingAt()
|
inherited |
Definition at line 96 of file InnerNode.cc.
References ACLChecklist::asyncInProgress(), and debugs.
Referenced by ACLChecklist::matchAndFinish(), and ACLChecklist::matchChild().
◆ treeDump()
|
inline |
dumps <name, action, rule, new line> tuples the supplied converter maps action.kind to a string
Definition at line 60 of file Tree.h.
References actions, SBuf::end(), Acl::InnerNode::nodes, SBuf::push_back(), and text.
Referenced by dump_http_upgrade_request_protocols(), dump_on_unsupported_protocol(), and Note::printAsNoteDirective().
◆ typeString()
|
overridevirtualinherited |
Implements Acl::Node.
Definition at line 93 of file BoolOps.cc.
◆ valid()
|
virtualinherited |
Reimplemented in ACLExternal, ACLProxyAuth, Acl::ServerNameCheck, ACLMaxUserIP, ACLMaxConnection, and ACLRandom.
◆ winningAction()
Acl::Answer Acl::Tree::winningAction | ( | ) | const |
Definition at line 15 of file Tree.cc.
References actionAt(), Acl::OrNode::lastMatch_, and Acl::InnerNode::nodes.
Referenced by ACLChecklist::matchAndFinish().
Member Data Documentation
◆ actions
|
protected |
Definition at line 49 of file Tree.h.
Referenced by treeDump().
◆ cfgline
◆ lastMatch_
|
mutableprotectedinherited |
Definition at line 72 of file BoolOps.h.
Referenced by winningAction().
◆ name
|
inherited |
Either aclname parameter from the explicitly configured acl directive or a label generated for an internal ACL tree node. All Node objects corresponding to one Squid configuration have unique names. See also: context() and FindByName().
Definition at line 81 of file Node.h.
Referenced by aclDestroyAccessList(), Acl::AnnotateClientCheck::match(), Acl::AnnotateTransactionCheck::match(), ACLRandom::match(), Acl::SourceDomainCheck::match(), ACLDestinationIP::match(), Acl::NotNode::NotNode(), ACLExternal::parse(), and ACLMaxConnection::prepareForUse().
◆ nodes
|
protectedinherited |
Definition at line 51 of file InnerNode.h.
Referenced by Acl::InnerNode::childrenCount(), Acl::InnerNode::prepareForUse(), treeDump(), and winningAction().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products