#include <Client.h>
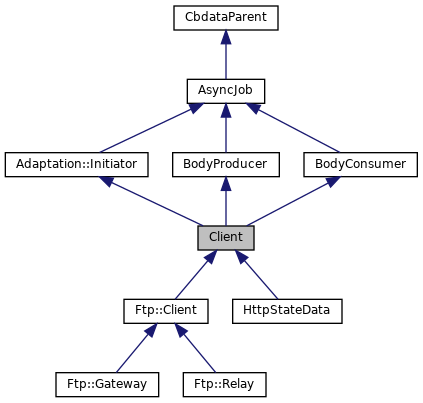
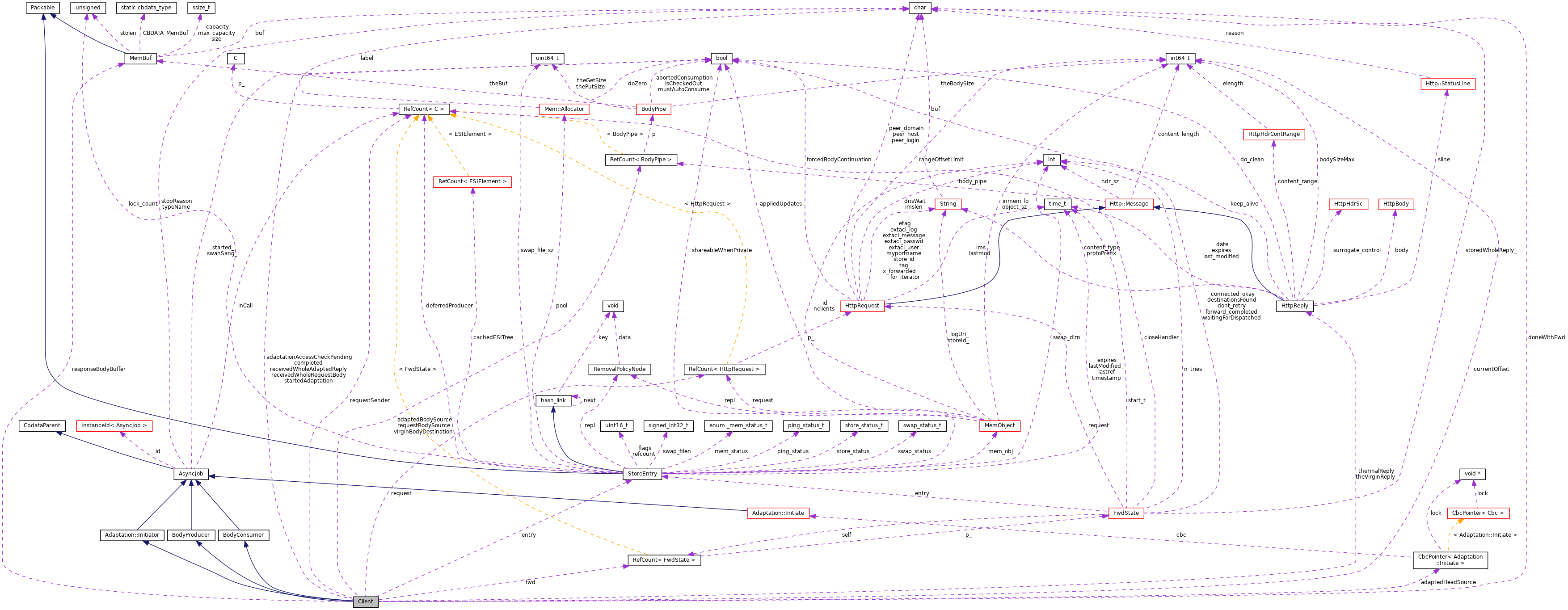
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
Public Member Functions | |
Client (FwdState *) | |
~Client () override | |
virtual const Comm::ConnectionPointer & | dataConnection () const =0 |
void | noteMoreBodyDataAvailable (BodyPipe::Pointer) override |
void | noteBodyProductionEnded (BodyPipe::Pointer) override |
void | noteBodyProducerAborted (BodyPipe::Pointer) override |
virtual void | maybeReadVirginBody ()=0 |
read response data from the network More... | |
virtual void | abortAll (const char *reason)=0 |
abnormal transaction termination; reason is for debugging only More... | |
virtual bool | abortOnData (const char *reason) |
virtual HttpRequestPointer | originalRequest () |
a hack to reach HttpStateData::orignal_request More... | |
void | noteAdaptationAnswer (const Adaptation::Answer &answer) override |
void | noteAdaptationAclCheckDone (Adaptation::ServiceGroupPointer group) override |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override |
virtual bool | getMoreRequestBody (MemBuf &buf) |
either fill buf with available [encoded] request body bytes or return false More... | |
virtual void | processReplyBody ()=0 |
void | swanSong () override |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | serverComplete () |
void | markParsedVirginReplyAsWhole (const char *reasonWeAreSure) |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
StoreEntry * | entry = nullptr |
FwdState::Pointer | fwd |
HttpRequestPointer | request |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
virtual void | haveParsedReplyHeaders () |
called when we have final (possibly adapted) reply headers; kids extend More... | |
virtual void | completeForwarding () |
bool | startRequestBodyFlow () |
void | handleMoreRequestBodyAvailable () |
void | handleRequestBodyProductionEnded () |
virtual void | handleRequestBodyProducerAborted ()=0 |
void | sendMoreRequestBody () |
virtual void | sentRequestBody (const CommIoCbParams &io)=0 |
virtual void | doneSendingRequestBody ()=0 |
virtual void | closeServer ()=0 |
virtual bool | doneWithServer () const =0 |
virtual bool | mayReadVirginReplyBody () const =0 |
whether we may receive more virgin response body bytes More... | |
virtual void | noteDelayAwareReadChance ()=0 |
bool | abortOnBadEntry (const char *abortReason) |
Entry-dependent callbacks use this check to quit if the entry went bad. More... | |
bool | blockCaching () |
whether to prevent caching of an otherwise cachable response More... | |
void | startAdaptation (const Adaptation::ServiceGroupPointer &group, HttpRequest *cause) |
Initiate an asynchronous adaptation transaction which will call us back. More... | |
void | adaptVirginReplyBody (const char *buf, ssize_t len) |
void | cleanAdaptation () |
virtual bool | doneWithAdaptation () const |
void | handleMoreAdaptedBodyAvailable () |
void | handleAdaptedBodyProductionEnded () |
void | handleAdaptedBodyProducerAborted () |
void | handleAdaptedHeader (Http::Message *msg) |
void | handleAdaptationCompleted () |
void | handleAdaptationBlocked (const Adaptation::Answer &answer) |
void | handleAdaptationAborted (bool bypassable=false) |
bool | handledEarlyAdaptationAbort () |
void | resumeBodyStorage () |
called by StoreEntry when it has more buffer space available More... | |
void | endAdaptedBodyConsumption () |
called when the entire adapted response body is consumed More... | |
const HttpReply * | virginReply () const |
HttpReply * | virginReply () |
HttpReply * | setVirginReply (HttpReply *r) |
HttpReply * | finalReply () |
HttpReply * | setFinalReply (HttpReply *r) |
void | adaptOrFinalizeReply () |
void | addVirginReplyBody (const char *buf, ssize_t len) |
void | storeReplyBody (const char *buf, ssize_t len) |
size_t | replyBodySpace (const MemBuf &readBuf, const size_t minSpace) const |
size_t | calcBufferSpaceToReserve (const size_t space, const size_t wantSpace) const |
determine how much space the buffer needs to reserve More... | |
void | adjustBodyBytesRead (const int64_t delta) |
initializes bodyBytesRead stats if needed and applies delta More... | |
void | delayRead () |
CbcPointer< Initiate > | initiateAdaptation (Initiate *x) |
< starts freshly created initiate and returns a safe pointer to it More... | |
void | clearAdaptation (CbcPointer< Initiate > &x) |
clears the pointer (does not call announceInitiatorAbort) More... | |
void | announceInitiatorAbort (CbcPointer< Initiate > &x) |
inform the transaction about abnormal termination and clear the pointer More... | |
bool | initiated (const CbcPointer< AsyncJob > &job) const |
Must(initiated(initiate)) instead of Must(initiate.set()), for clarity. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual void | start () |
called by AsyncStart; do not call directly More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
int64_t | currentOffset = 0 |
MemBuf * | responseBodyBuffer = nullptr |
BodyPipe::Pointer | requestBodySource |
AsyncCall::Pointer | requestSender |
BodyPipe::Pointer | virginBodyDestination |
CbcPointer< Adaptation::Initiate > | adaptedHeadSource |
BodyPipe::Pointer | adaptedBodySource |
bool | adaptationAccessCheckPending = false |
bool | startedAdaptation = false |
bool | receivedWholeAdaptedReply = false |
handleAdaptedBodyProductionEnded() was called More... | |
bool | receivedWholeRequestBody = false |
handleRequestBodyProductionEnded called More... | |
const char * | doneWithFwd = nullptr |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
void | serverComplete2 () |
void | sendBodyIsTooLargeError () |
void | maybePurgeOthers () |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
bool | completed = false |
HttpReply * | theVirginReply = nullptr |
HttpReply * | theFinalReply = nullptr |
Detailed Description
Client is a common base for classes such as HttpStateData and FtpStateData. All such classes must be able to consume request bodies from a BodyPipe or ICAP producer, adapt virgin responses using ICAP, and provide a consumer with responses.
Member Typedef Documentation
◆ Pointer [1/3]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [2/3]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [3/3]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ Client()
Client::Client | ( | FwdState * | theFwdState | ) |
Definition at line 38 of file Client.cc.
References entry, FwdState::entry, fwd, and StoreEntry::lock().
◆ ~Client()
|
override |
Definition at line 47 of file Client.cc.
References adaptedBodySource, assert, entry, HTTPMSGUNLOCK(), requestBodySource, responseBodyBuffer, theFinalReply, theVirginReply, StoreEntry::unlock(), and virginBodyDestination.
Member Function Documentation
◆ abortAll()
|
pure virtual |
Implemented in Ftp::Client, and HttpStateData.
Referenced by abortOnData(), handleAdaptationAborted(), handleAdaptationBlocked(), and handledEarlyAdaptationAbort().
◆ abortOnBadEntry()
|
protected |
Definition at line 258 of file Client.cc.
References abortOnData(), debugs, entry, and StoreEntry::isAccepting().
Referenced by handleAdaptationAborted(), handleAdaptationBlocked(), handleAdaptedBodyProducerAborted(), handleAdaptedBodyProductionEnded(), handleAdaptedHeader(), handleMoreAdaptedBodyAvailable(), noteAdaptationAclCheckDone(), and resumeBodyStorage().
◆ abortOnData()
|
virtual |
abnormal data transfer termination
- Return values
-
true the transaction will be terminated (abortAll called) false the transaction will survive
Reimplemented in Ftp::Relay.
Definition at line 311 of file Client.cc.
References abortAll().
Referenced by abortOnBadEntry(), handleAdaptationBlocked(), sendBodyIsTooLargeError(), and sentRequestBody().
◆ adaptOrFinalizeReply()
|
protected |
Definition at line 996 of file Client.cc.
References adaptationAccessCheckPending, FwdState::al, debugs, fwd, Adaptation::methodRespmod, originalRequest(), Adaptation::pointPreCache, setFinalReply(), Adaptation::AccessCheck::Start(), and virginReply().
Referenced by HttpStateData::processReply().
◆ adaptVirginReplyBody()
|
protected |
Definition at line 629 of file Client.cc.
References MemBuf::append(), assert, MemBuf::consume(), MemBuf::content(), MemBuf::contentSize(), debugs, MemBuf::init(), BodyPipe::putMoreData(), responseBodyBuffer, startedAdaptation, and virginBodyDestination.
Referenced by addVirginReplyBody().
◆ addVirginReplyBody()
|
protected |
Definition at line 1038 of file Client.cc.
References adaptationAccessCheckPending, adaptVirginReplyBody(), adjustBodyBytesRead(), assert, startedAdaptation, and storeReplyBody().
Referenced by HttpStateData::decodeAndWriteReplyBody(), noteMoreBodySpaceAvailable(), and HttpStateData::writeReplyBody().
◆ adjustBodyBytesRead()
|
protected |
Definition at line 1014 of file Client.cc.
References HierarchyLogEntry::bodyBytesRead, HttpRequest::hier, Must, and originalRequest().
Referenced by addVirginReplyBody().
◆ announceInitiatorAbort()
|
protectedinherited |
Definition at line 38 of file Initiator.cc.
References CallJobHere.
Referenced by ClientHttpRequest::~ClientHttpRequest(), and cleanAdaptation().
◆ blockCaching()
|
protected |
Definition at line 552 of file Client.cc.
References SquidConfig::accessList, ACLFilledChecklist::al, FwdState::al, Acl::Answer::allowed(), Config, debugs, entry, ACLChecklist::fastCheck(), MemObject::freshestReply(), fwd, HTTPMSGLOCK(), StoreEntry::mem(), originalRequest(), ACLFilledChecklist::reply, and SquidConfig::storeMiss.
Referenced by setFinalReply().
◆ calcBufferSpaceToReserve()
|
protected |
Definition at line 1063 of file Client.cc.
References BodyPipe::buf(), debugs, SBuf::maxSize, min(), MemBuf::potentialSpaceSize(), responseBodyBuffer, and virginBodyDestination.
Referenced by HttpStateData::maybeMakeSpaceAvailable().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in ConnStateData, ClientHttpRequest, Adaptation::Icap::ModXact, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Ipc::Forwarder, Ipc::Inquirer, and Ftp::Server.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by ConnStateData::callException(), Adaptation::Icap::Xaction::callException(), Ipc::Forwarder::callException(), Ipc::Inquirer::callException(), and Ftp::Server::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cleanAdaptation()
|
protected |
Definition at line 604 of file Client.cc.
References adaptationAccessCheckPending, adaptedBodySource, adaptedHeadSource, Adaptation::Initiator::announceInitiatorAbort(), assert, debugs, doneWithAdaptation(), BodyConsumer::stopConsumingFrom(), BodyProducer::stopProducingFor(), and virginBodyDestination.
Referenced by handleAdaptationCompleted(), and swanSong().
◆ clearAdaptation()
|
protectedinherited |
Definition at line 32 of file Initiator.cc.
References CbcPointer< Cbc >::clear().
Referenced by ClientHttpRequest::handleAdaptedHeader(), ClientHttpRequest::noteAdaptationAnswer(), and noteAdaptationAnswer().
◆ closeServer()
|
protectedpure virtual |
Use this to end communication with the server. The call cancels our closure handler and tells FwdState to forget about the connection.
Implemented in Ftp::Client, and HttpStateData.
Referenced by handleAdaptationCompleted(), serverComplete(), and swanSong().
◆ completeForwarding()
|
protectedvirtual |
default calls fwd->complete()
Reimplemented in Ftp::Gateway, and Ftp::Relay.
Definition at line 230 of file Client.cc.
References assert, FwdState::complete(), debugs, doneWithFwd, and fwd.
Referenced by Ftp::Gateway::completeForwarding(), Ftp::Relay::completeForwarding(), handleAdaptationCompleted(), and serverComplete2().
◆ dataConnection()
|
pure virtual |
- Returns
- primary or "request data connection"
Implemented in Ftp::Client, and HttpStateData.
Referenced by sendMoreRequestBody().
◆ delayRead()
|
protected |
Defer reading until it is likely to become possible. Eventually, noteDelayAwareReadChance() will be called.
Definition at line 1029 of file Client.cc.
References asyncCall(), MemObject::delayRead(), entry, StoreEntry::mem(), and noteDelayAwareReadChance().
Referenced by HttpStateData::readReply().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 217 of file Client.cc.
References AsyncJob::doneAll(), doneWithAdaptation(), and doneWithServer().
◆ doneSendingRequestBody()
|
protectedpure virtual |
Implemented in Ftp::Client, and HttpStateData.
Definition at line 340 of file Client.cc.
References assert, debugs, requestBodySource, and BodyConsumer::stopConsumingFrom().
Referenced by Ftp::Client::doneSendingRequestBody(), HttpStateData::doneSendingRequestBody(), handleRequestBodyProductionEnded(), and sentRequestBody().
◆ doneWithAdaptation()
|
protectedvirtual |
did we end ICAP communication?
Definition at line 621 of file Client.cc.
References adaptationAccessCheckPending, adaptedBodySource, adaptedHeadSource, and virginBodyDestination.
Referenced by cleanAdaptation(), doneAll(), handleAdaptedHeader(), noteBodyConsumerAborted(), and serverComplete2().
◆ doneWithServer()
|
protectedpure virtual |
did we end communication?
Implemented in Ftp::Client, and HttpStateData.
Referenced by doneAll(), serverComplete(), and swanSong().
◆ endAdaptedBodyConsumption()
|
protected |
Definition at line 830 of file Client.cc.
References adaptedBodySource, fwd, handleAdaptationCompleted(), FwdState::markStoredReplyAsWhole(), receivedWholeAdaptedReply, and BodyConsumer::stopConsumingFrom().
Referenced by handleAdaptedBodyProductionEnded(), and resumeBodyStorage().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finalReply()
|
protected |
Definition at line 129 of file Client.cc.
References assert, and theFinalReply.
Referenced by HttpStateData::haveParsedReplyHeaders(), and HttpStateData::reusableReply().
◆ getMoreRequestBody()
|
virtual |
Reimplemented in HttpStateData.
Definition at line 438 of file Client.cc.
References BodyPipe::getMoreData(), Must, and requestBodySource.
Referenced by HttpStateData::getMoreRequestBody(), and sendMoreRequestBody().
◆ handleAdaptationAborted()
|
protected |
Definition at line 885 of file Client.cc.
References abortAll(), abortOnBadEntry(), debugs, entry, handledEarlyAdaptationAbort(), and StoreEntry::isEmpty().
Referenced by noteAdaptationAnswer().
◆ handleAdaptationBlocked()
|
protected |
Definition at line 924 of file Client.cc.
References abortAll(), abortOnBadEntry(), abortOnData(), aclGetDenyInfoPage(), FwdState::al, Config, debugs, SquidConfig::denyInfoList, HttpRequest::detailError(), FwdState::dontRetry(), entry, ERR_ACCESS_DENIED, ERR_ICAP_FAILURE, ERR_NONE, FwdState::fail(), fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), request, Adaptation::Answer::ruleId, Http::scForbidden, and String::termedBuf().
Referenced by noteAdaptationAnswer().
◆ handleAdaptationCompleted()
|
protected |
Definition at line 866 of file Client.cc.
References cleanAdaptation(), closeServer(), completeForwarding(), debugs, and mayReadVirginReplyBody().
Referenced by endAdaptedBodyConsumption(), handleAdaptedBodyProducerAborted(), handleAdaptedHeader(), and noteBodyConsumerAborted().
◆ handleAdaptedBodyProducerAborted()
|
protected |
Definition at line 845 of file Client.cc.
References abortOnBadEntry(), adaptedBodySource, debugs, BodyPipe::exhausted(), handleAdaptationCompleted(), handledEarlyAdaptationAbort(), Must, and BodyConsumer::stopConsumingFrom().
Referenced by noteBodyProducerAborted().
◆ handleAdaptedBodyProductionEnded()
|
protected |
Definition at line 815 of file Client.cc.
References abortOnBadEntry(), adaptedBodySource, endAdaptedBodyConsumption(), BodyPipe::exhausted(), and receivedWholeAdaptedReply.
Referenced by noteBodyProductionEnded().
◆ handleAdaptedHeader()
|
protected |
Definition at line 717 of file Client.cc.
References abortOnBadEntry(), adaptedBodySource, assert, Http::Message::body_pipe, debugs, doneWithAdaptation(), BodyPipe::expectNoConsumption(), fwd, handleAdaptationCompleted(), FwdState::markStoredReplyAsWhole(), BodyPipe::setConsumerIfNotLate(), and setFinalReply().
Referenced by noteAdaptationAnswer().
◆ handledEarlyAdaptationAbort()
|
protected |
If the store entry is still empty, fully handles adaptation abort, returning true. Otherwise just updates the request error detail and returns false.
Definition at line 901 of file Client.cc.
References abortAll(), FwdState::al, debugs, HttpRequest::detailError(), FwdState::dontRetry(), entry, ERR_ICAP_FAILURE, FwdState::fail(), fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), request, and Http::scInternalServerError.
Referenced by handleAdaptationAborted(), and handleAdaptedBodyProducerAborted().
◆ handleMoreAdaptedBodyAvailable()
|
protected |
Definition at line 768 of file Client.cc.
References abortOnBadEntry(), adaptedBodySource, assert, asyncCall(), BodyPipeCheckout::buf, BodyPipe::buf(), StoreEntry::bytesWanted(), BodyPipeCheckout::checkIn(), MemBuf::consume(), BodyPipe::consumedSize(), MemBuf::contentSize(), currentOffset, debugs, StoreEntry::deferProducer(), entry, StoreIOBuffer::length, resumeBodyStorage(), and StoreEntry::write().
Referenced by noteMoreBodyDataAvailable(), and resumeBodyStorage().
◆ handleMoreRequestBodyAvailable()
|
protected |
Definition at line 319 of file Client.cc.
References debugs, requestSender, and sendMoreRequestBody().
Referenced by noteMoreBodyDataAvailable().
◆ handleRequestBodyProducerAborted()
|
protectedpure virtual |
Implemented in Ftp::Gateway, Ftp::Relay, and HttpStateData.
Definition at line 351 of file Client.cc.
References debugs, FwdState::dontRetry(), fwd, requestBodySource, requestSender, and BodyConsumer::stopConsumingFrom().
Referenced by Ftp::Gateway::handleRequestBodyProducerAborted(), Ftp::Relay::handleRequestBodyProducerAborted(), HttpStateData::handleRequestBodyProducerAborted(), and noteBodyProducerAborted().
◆ handleRequestBodyProductionEnded()
|
protected |
Definition at line 329 of file Client.cc.
References debugs, doneSendingRequestBody(), receivedWholeRequestBody, and requestSender.
Referenced by noteBodyProductionEnded().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ haveParsedReplyHeaders()
|
protectedvirtual |
called when got final headers
Reimplemented in Ftp::Gateway, and HttpStateData.
Definition at line 537 of file Client.cc.
References HttpReply::contentRange(), currentOffset, maybePurgeOthers(), Must, theFinalReply, and HttpHdrRangeSpec::UnknownPosition.
Referenced by Ftp::Gateway::haveParsedReplyHeaders(), HttpStateData::haveParsedReplyHeaders(), and setFinalReply().
◆ initiateAdaptation()
|
protectedinherited |
Definition at line 23 of file Initiator.cc.
References Adaptation::Initiate::initiator(), and Ident::Start().
Referenced by ClientHttpRequest::startAdaptation(), and startAdaptation().
◆ initiated()
|
inlineprotectedinherited |
Definition at line 52 of file Initiator.h.
References CbcPointer< Cbc >::set().
Referenced by ClientHttpRequest::startAdaptation(), and startAdaptation().
◆ markParsedVirginReplyAsWhole()
void Client::markParsedVirginReplyAsWhole | ( | const char * | reasonWeAreSure | ) |
remember that the received virgin reply was parsed in its entirety, including its body (if any)
Definition at line 158 of file Client.cc.
References assert, debugs, fwd, FwdState::markStoredReplyAsWhole(), and startedAdaptation.
Referenced by HttpStateData::decodeAndWriteReplyBody(), ftpReadTransferDone(), and HttpStateData::writeReplyBody().
◆ maybePurgeOthers()
|
private |
Definition at line 516 of file Client.cc.
References SBuf::c_str(), Http::CONTENT_LOCATION, debugs, HttpRequest::effectiveRequestUri(), RefCount< C >::getRaw(), Http::LOCATION, HttpRequest::method, purgeEntriesByHeader(), purgeEntriesByUrl(), HttpRequestMethod::purgesOthers(), request, HttpReply::sline, Http::StatusLine::status(), and theFinalReply.
Referenced by haveParsedReplyHeaders().
◆ maybeReadVirginBody()
|
pure virtual |
Implemented in Ftp::Client, and HttpStateData.
Referenced by noteMoreBodySpaceAvailable().
◆ mayReadVirginReplyBody()
|
protectedpure virtual |
Implemented in Ftp::Gateway, Ftp::Relay, and HttpStateData.
Referenced by handleAdaptationCompleted().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteAdaptationAclCheckDone()
|
overridevirtual |
AccessCheck calls this back with a possibly nil service group to signal whether adaptation is needed and where it should start.
Reimplemented from Adaptation::Initiator.
Definition at line 957 of file Client.cc.
References abortOnBadEntry(), adaptationAccessCheckPending, debugs, originalRequest(), processReplyBody(), request, sendBodyIsTooLargeError(), setFinalReply(), startAdaptation(), and virginReply().
◆ noteAdaptationAnswer()
|
overridevirtual |
called with the initial adaptation decision (adapt, block, error); virgin and/or adapted body transmission may continue after this
Implements Adaptation::Initiator.
Definition at line 697 of file Client.cc.
References adaptedHeadSource, Adaptation::Answer::akBlock, Adaptation::Answer::akError, Adaptation::Answer::akForward, Adaptation::Initiator::clearAdaptation(), Adaptation::Answer::final, RefCount< C >::getRaw(), handleAdaptationAborted(), handleAdaptationBlocked(), handleAdaptedHeader(), Adaptation::Answer::kind, and Adaptation::Answer::message.
◆ noteBodyConsumerAborted()
|
overridevirtual |
Implements BodyProducer.
Definition at line 685 of file Client.cc.
References doneWithAdaptation(), handleAdaptationCompleted(), BodyProducer::stopProducingFor(), and virginBodyDestination.
◆ noteBodyProducerAborted()
|
overridevirtual |
Implements BodyConsumer.
Definition at line 298 of file Client.cc.
References adaptedBodySource, handleAdaptedBodyProducerAborted(), handleRequestBodyProducerAborted(), and requestBodySource.
◆ noteBodyProductionEnded()
|
overridevirtual |
Implements BodyConsumer.
Definition at line 284 of file Client.cc.
References adaptedBodySource, handleAdaptedBodyProductionEnded(), handleRequestBodyProductionEnded(), and requestBodySource.
◆ noteDelayAwareReadChance()
|
protectedpure virtual |
Called when a previously delayed dataConnection() read may be possible.
- See also
- delayRead()
Implemented in Ftp::Client, and HttpStateData.
Referenced by delayRead().
◆ noteMoreBodyDataAvailable()
|
overridevirtual |
Implements BodyConsumer.
Definition at line 270 of file Client.cc.
References adaptedBodySource, handleMoreAdaptedBodyAvailable(), handleMoreRequestBodyAvailable(), and requestBodySource.
◆ noteMoreBodySpaceAvailable()
|
overridevirtual |
Implements BodyProducer.
Definition at line 671 of file Client.cc.
References addVirginReplyBody(), completed, maybeReadVirginBody(), responseBodyBuffer, and serverComplete2().
◆ originalRequest()
|
virtual |
Definition at line 570 of file Client.cc.
References request.
Referenced by adaptOrFinalizeReply(), adjustBodyBytesRead(), blockCaching(), HttpStateData::finishingBrokenPost(), HttpStateData::handle1xx(), noteAdaptationAclCheckDone(), serverComplete(), and startRequestBodyFlow().
◆ processReplyBody()
|
pure virtual |
Implemented in Ftp::Gateway, Ftp::Relay, and HttpStateData.
Referenced by noteAdaptationAclCheckDone().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ replyBodySpace()
- Deprecated:
- use SBuf I/O API and calcBufferSpaceToReserve() instead
Definition at line 1098 of file Client.cc.
References BodyPipe::buf(), debugs, min(), MemBuf::potentialSpaceSize(), responseBodyBuffer, MemBuf::spaceSize(), and virginBodyDestination.
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ resumeBodyStorage()
|
protected |
Definition at line 752 of file Client.cc.
References abortOnBadEntry(), adaptedBodySource, endAdaptedBodyConsumption(), BodyPipe::exhausted(), and handleMoreAdaptedBodyAvailable().
Referenced by handleMoreAdaptedBodyAvailable().
◆ sendBodyIsTooLargeError()
|
private |
Definition at line 985 of file Client.cc.
References abortOnData(), FwdState::al, FwdState::dontRetry(), ERR_TOO_BIG, FwdState::fail(), fwd, RefCount< C >::getRaw(), request, and Http::scForbidden.
Referenced by noteAdaptationAclCheckDone().
◆ sendMoreRequestBody()
|
protected |
Definition at line 412 of file Client.cc.
References assert, conn, MemBuf::contentSize(), dataConnection(), debugs, getMoreRequestBody(), Comm::IsConnOpen(), JobCallback, requestBodySource, requestSender, sentRequestBody(), and Comm::Write().
Referenced by handleMoreRequestBodyAvailable(), and sentRequestBody().
◆ sentRequestBody()
|
protectedpure virtual |
Implemented in Ftp::Client, and HttpStateData.
Definition at line 364 of file Client.cc.
References abortOnData(), FwdState::al, StatCounters::all, DBG_IMPORTANT, debugs, doneSendingRequestBody(), EBIT_TEST, entry, ENTRY_ABORTED, Comm::ERR_CLOSING, ERR_WRITE_ERROR, BodyPipe::exhausted(), FwdState::fail(), CommCommonCbParams::fd, fd_bytes(), FD_WRITE, CommCommonCbParams::flag, StoreEntry::flags, fwd, HttpRequest::hier, StatCounters::kbytes_out, HierarchyLogEntry::notePeerWrite(), receivedWholeRequestBody, request, FwdState::request, requestBodySource, requestSender, Http::scBadGateway, sendMoreRequestBody(), StatCounters::server, CommIoCbParams::size, statCounter, CommCommonCbParams::xerrno, ErrorState::xerrno, and xstrerr().
Referenced by sendMoreRequestBody(), Ftp::Client::sentRequestBody(), and HttpStateData::sentRequestBody().
◆ serverComplete()
void Client::serverComplete | ( | ) |
call when no server communication is expected
Definition at line 180 of file Client.cc.
References assert, closeServer(), completed, debugs, doneWithServer(), HttpRequest::hier, originalRequest(), requestBodySource, responseBodyBuffer, serverComplete2(), BodyConsumer::stopConsumingFrom(), and HierarchyLogEntry::stopPeerClock().
Referenced by ftpReadQuit(), HttpStateData::processReplyBody(), and Ftp::Relay::serverComplete().
◆ serverComplete2()
|
private |
Continuation of serverComplete
Definition at line 202 of file Client.cc.
References completeForwarding(), debugs, doneWithAdaptation(), BodyProducer::stopProducingFor(), and virginBodyDestination.
Referenced by noteMoreBodySpaceAvailable(), and serverComplete().
◆ setFinalReply()
Definition at line 136 of file Client.cc.
References FwdState::al, assert, blockCaching(), debugs, EBIT_TEST, entry, StoreEntry::flags, fwd, haveParsedReplyHeaders(), HTTPMSGLOCK(), StoreEntry::release(), RELEASE_REQUEST, StoreEntry::replaceHttpReply(), AccessLogEntry::reply, StoreEntry::startWriting(), and theFinalReply.
Referenced by adaptOrFinalizeReply(), handleAdaptedHeader(), and noteAdaptationAclCheckDone().
◆ setVirginReply()
Definition at line 116 of file Client.cc.
References FwdState::al, assert, debugs, fwd, HTTPMSGLOCK(), AccessLogEntry::reply, and theVirginReply.
Referenced by HttpStateData::processReplyHeader().
◆ start()
|
protectedvirtualinherited |
Reimplemented in Adaptation::AccessCheck, Adaptation::Ecap::XactionRep, Adaptation::Icap::Launcher, Adaptation::Icap::ModXact, Adaptation::Icap::OptXact, Adaptation::Icap::Xaction, Adaptation::Iterator, ConnStateData, Ftp::Client, Ftp::Gateway, Ftp::Relay, Http::Tunneler, Comm::ConnOpener, Comm::TcpAcceptor, Downloader, Rock::HeaderUpdater, Rock::Rebuild, HappyConnOpener, HttpStateData, Ipc::Coordinator, Ipc::Forwarder, Ipc::Inquirer, Ipc::Strand, Ipc::UdsSender, Log::TcpLogger, Mgr::ActionWriter, Mgr::Filler, Mgr::Inquirer, Mgr::StoreToCommWriter, PeerPoolMgr, Security::PeerConnector, Ftp::Server, Http::One::Server, Server, Snmp::Inquirer, and Ipc::Port.
Definition at line 59 of file AsyncJob.cc.
Referenced by Adaptation::AccessCheck::start(), Adaptation::Icap::Launcher::start(), Adaptation::Icap::Xaction::start(), Adaptation::Iterator::start(), ConnStateData::start(), Http::Tunneler::start(), Ipc::UdsSender::start(), PeerPoolMgr::start(), Security::PeerConnector::start(), Ipc::Port::start(), and AsyncJob::Start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ startAdaptation()
|
protected |
Definition at line 578 of file Client.cc.
References adaptedHeadSource, FwdState::al, assert, Http::Message::body_pipe, debugs, HttpReply::expectingBody(), fwd, Adaptation::Initiator::initiateAdaptation(), Adaptation::Initiator::initiated(), HttpRequest::method, Must, BodyPipe::setBodySize(), size, startedAdaptation, virginBodyDestination, and virginReply().
Referenced by noteAdaptationAclCheckDone().
◆ startRequestBodyFlow()
|
protected |
Definition at line 239 of file Client.cc.
References assert, Http::Message::body_pipe, debugs, originalRequest(), requestBodySource, BodyPipe::setConsumerIfNotLate(), and BodyPipe::status().
Referenced by HttpStateData::sendRequest().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Ecap::XactionRep, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Adaptation::Initiate, Http::Tunneler, Comm::TcpAcceptor, HappyConnOpener, Ipc::Inquirer, and Security::PeerConnector.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Adaptation::Initiate::status(), and Comm::TcpAcceptor::status().
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by ClientHttpRequest::~ClientHttpRequest(), cleanAdaptation(), doneSendingRequestBody(), endAdaptedBodyConsumption(), ClientHttpRequest::endRequestSatisfaction(), handleAdaptedBodyProducerAborted(), handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), ClientHttpRequest::noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), serverComplete(), and swanSong().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by ConnStateData::~ConnStateData(), cleanAdaptation(), ConnStateData::finishDechunkingRequest(), noteBodyConsumerAborted(), and serverComplete2().
◆ storeReplyBody()
|
protected |
Definition at line 1054 of file Client.cc.
References currentOffset, entry, and StoreEntry::write().
Referenced by addVirginReplyBody().
◆ swanSong()
|
overridevirtual |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Relay.
Definition at line 68 of file Client.cc.
References adaptedBodySource, assert, cleanAdaptation(), closeServer(), doneWithFwd, doneWithServer(), fwd, FwdState::handleUnregisteredServerEnd(), requestBodySource, BodyConsumer::stopConsumingFrom(), AsyncJob::swanSong(), and virginBodyDestination.
Referenced by Ftp::Relay::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ virginReply() [1/2]
|
protected |
Definition at line 102 of file Client.cc.
References assert, and theVirginReply.
◆ virginReply() [2/2]
|
protected |
Definition at line 109 of file Client.cc.
References assert, and theVirginReply.
Referenced by adaptOrFinalizeReply(), HttpStateData::continueAfterParsingHeader(), HttpStateData::handleMoreRequestBodyAvailable(), noteAdaptationAclCheckDone(), HttpStateData::persistentConnStatus(), startAdaptation(), HttpStateData::statusIfComplete(), HttpStateData::truncateVirginBody(), and HttpStateData::writeReplyBody().
Member Data Documentation
◆ adaptationAccessCheckPending
|
protected |
Definition at line 189 of file Client.h.
Referenced by adaptOrFinalizeReply(), addVirginReplyBody(), cleanAdaptation(), doneWithAdaptation(), noteAdaptationAclCheckDone(), and HttpStateData::processReplyBody().
◆ adaptedBodySource
|
protected |
to consume adated response body
Definition at line 187 of file Client.h.
Referenced by ~Client(), cleanAdaptation(), doneWithAdaptation(), endAdaptedBodyConsumption(), handleAdaptedBodyProducerAborted(), handleAdaptedBodyProductionEnded(), handleAdaptedHeader(), handleMoreAdaptedBodyAvailable(), noteBodyProducerAborted(), noteBodyProductionEnded(), noteMoreBodyDataAvailable(), resumeBodyStorage(), and swanSong().
◆ adaptedHeadSource
|
protected |
to get adapted response headers
Definition at line 186 of file Client.h.
Referenced by cleanAdaptation(), doneWithAdaptation(), noteAdaptationAnswer(), and startAdaptation().
◆ completed
|
private |
serverComplete() has been called
Definition at line 90 of file Client.h.
Referenced by noteMoreBodySpaceAvailable(), and serverComplete().
◆ currentOffset
|
protected |
Our current offset in the StoreEntry
Definition at line 172 of file Client.h.
Referenced by Ftp::Gateway::getCurrentOffset(), handleMoreAdaptedBodyAvailable(), haveParsedReplyHeaders(), Ftp::Gateway::setCurrentOffset(), and storeReplyBody().
◆ doneWithFwd
|
protected |
whether we should not be talking to FwdState; XXX: clear fwd instead points to a string literal which is used only for debugging
Definition at line 199 of file Client.h.
Referenced by completeForwarding(), HttpStateData::httpStateConnClosed(), HttpStateData::proceedAfter1xx(), and swanSong().
◆ entry
StoreEntry* Client::entry = nullptr |
Definition at line 176 of file Client.h.
Referenced by Client(), Ftp::Gateway::Gateway(), HttpStateData::HttpStateData(), Ftp::Relay::Relay(), ~Client(), abortOnBadEntry(), blockCaching(), HttpStateData::buildRequestPrefix(), HttpStateData::continueAfterParsingHeader(), delayRead(), ftpFail(), ftpSendReply(), ftpWriteTransferDone(), handleAdaptationAborted(), handleAdaptationBlocked(), handledEarlyAdaptationAbort(), handleMoreAdaptedBodyAvailable(), HttpStateData::handleMoreRequestBodyAvailable(), HttpStateData::handleRequestBodyProducerAborted(), HttpStateData::haveParsedReplyHeaders(), HttpStateData::httpBuildRequestHeader(), HttpStateData::httpTimeout(), HttpStateData::keepaliveAccounting(), HttpStateData::peerSupportsConnectionPinning(), HttpStateData::processReply(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), HttpStateData::processSurrogateControl(), HttpStateData::readReply(), HttpStateData::reusableReply(), sentRequestBody(), setFinalReply(), HttpStateData::statusIfComplete(), storeReplyBody(), and HttpStateData::wroteLast().
◆ fwd
FwdState::Pointer Client::fwd |
Definition at line 177 of file Client.h.
Referenced by Client(), HttpStateData::HttpStateData(), adaptOrFinalizeReply(), blockCaching(), HttpStateData::buildRequestPrefix(), HttpStateData::closeServer(), completeForwarding(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), endAdaptedBodyConsumption(), HttpStateData::finishingBrokenPost(), HttpStateData::forwardUpgrade(), ftpFail(), ftpSendReply(), HttpStateData::handle1xx(), handleAdaptationBlocked(), handleAdaptedHeader(), handledEarlyAdaptationAbort(), HttpStateData::handleRequestBodyProducerAborted(), handleRequestBodyProducerAborted(), HttpStateData::httpTimeout(), markParsedVirginReplyAsWhole(), HttpStateData::markPrematureReplyBodyEofFailure(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), HttpStateData::readReply(), sendBodyIsTooLargeError(), sentRequestBody(), setFinalReply(), setVirginReply(), startAdaptation(), swanSong(), and HttpStateData::wroteLast().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ receivedWholeAdaptedReply
|
protected |
Definition at line 193 of file Client.h.
Referenced by endAdaptedBodyConsumption(), and handleAdaptedBodyProductionEnded().
◆ receivedWholeRequestBody
|
protected |
Definition at line 195 of file Client.h.
Referenced by HttpStateData::finishingChunkedRequest(), HttpStateData::getMoreRequestBody(), handleRequestBodyProductionEnded(), and sentRequestBody().
◆ request
HttpRequestPointer Client::request |
Definition at line 178 of file Client.h.
Referenced by Ftp::Gateway::Gateway(), HttpStateData::HttpStateData(), HttpStateData::buildRequestPrefix(), HttpStateData::checkDateSkew(), HttpStateData::continueAfterParsingHeader(), HttpStateData::decideIfWeDoRanges(), HttpStateData::drop1xx(), HttpStateData::forwardUpgrade(), ftpFail(), ftpReadType(), ftpSendPassive(), ftpSendReply(), ftpSendStor(), ftpSendType(), ftpSendUser(), ftpTrySlashHack(), HttpStateData::handle1xx(), handleAdaptationBlocked(), handledEarlyAdaptationAbort(), HttpStateData::handleMoreRequestBodyAvailable(), HttpStateData::haveParsedReplyHeaders(), HttpStateData::httpBuildRequestHeader(), HttpStateData::keepaliveAccounting(), maybePurgeOthers(), noteAdaptationAclCheckDone(), originalRequest(), HttpStateData::peerSupportsConnectionPinning(), HttpStateData::persistentConnStatus(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), HttpStateData::processSurrogateControl(), HttpStateData::readReply(), HttpStateData::reusableReply(), sendBodyIsTooLargeError(), HttpStateData::sendRequest(), sentRequestBody(), HttpStateData::statusIfComplete(), HttpStateData::truncateVirginBody(), HttpStateData::writeReplyBody(), and HttpStateData::wroteLast().
◆ requestBodySource
|
protected |
to consume request body
Definition at line 181 of file Client.h.
Referenced by ~Client(), doneSendingRequestBody(), getMoreRequestBody(), HttpStateData::getMoreRequestBody(), HttpStateData::handleMoreRequestBodyAvailable(), handleRequestBodyProducerAborted(), noteBodyProducerAborted(), noteBodyProductionEnded(), noteMoreBodyDataAvailable(), sendMoreRequestBody(), HttpStateData::sendRequest(), sentRequestBody(), serverComplete(), startRequestBodyFlow(), and swanSong().
◆ requestSender
|
protected |
set if we are expecting Comm::Write to call us back
Definition at line 182 of file Client.h.
Referenced by HttpStateData::finishingBrokenPost(), HttpStateData::finishingChunkedRequest(), handleMoreRequestBodyAvailable(), handleRequestBodyProducerAborted(), handleRequestBodyProductionEnded(), sendMoreRequestBody(), HttpStateData::sendRequest(), and sentRequestBody().
◆ responseBodyBuffer
|
protected |
Data temporarily buffered for ICAP
Definition at line 173 of file Client.h.
Referenced by ~Client(), adaptVirginReplyBody(), calcBufferSpaceToReserve(), noteMoreBodySpaceAvailable(), replyBodySpace(), and serverComplete().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ startedAdaptation
|
protected |
Definition at line 190 of file Client.h.
Referenced by adaptVirginReplyBody(), addVirginReplyBody(), markParsedVirginReplyAsWhole(), and startAdaptation().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ theFinalReply
|
private |
adapted reply from ICAP or virgin reply
Definition at line 206 of file Client.h.
Referenced by ~Client(), finalReply(), haveParsedReplyHeaders(), maybePurgeOthers(), and setFinalReply().
◆ theVirginReply
|
private |
reply received from the origin server
Definition at line 205 of file Client.h.
Referenced by ~Client(), setVirginReply(), and virginReply().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ virginBodyDestination
|
protected |
to provide virgin response body
Definition at line 185 of file Client.h.
Referenced by ~Client(), adaptVirginReplyBody(), calcBufferSpaceToReserve(), cleanAdaptation(), doneWithAdaptation(), noteBodyConsumerAborted(), replyBodySpace(), serverComplete2(), startAdaptation(), and swanSong().
The documentation for this class was generated from the following files: