#include <TcpAcceptor.h>
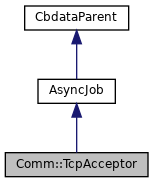

Public Types | |
typedef CbcPointer< Comm::TcpAcceptor > | Pointer |
Public Member Functions | |
TcpAcceptor (const Comm::ConnectionPointer &conn, const char *note, const Subscription::Pointer &aSub) | |
TcpAcceptor (const AnyP::PortCfgPointer &listenPort, const char *note, const Subscription::Pointer &aSub) | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | subscribe (const Subscription::Pointer &aSub) |
void | unsubscribe (const char *reason) |
void | acceptNext () |
void | notify (const Comm::Flag flag, const Comm::ConnectionPointer &details) const |
Call the subscribed callback handler with details about a new connection. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
Static Protected Member Functions | |
static bool | okToAccept () |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
int | errcode |
errno code of the last accept() or listen() action if one occurred. More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (TcpAcceptor) | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
TcpAcceptor (const TcpAcceptor &) | |
void | acceptOne () |
bool | acceptInto (Comm::ConnectionPointer &) |
void | setListen () |
void | handleClosure (const CommCloseCbParams &io) |
bool | intendedForUserConnections () const |
whether we are listening on one of the squid.conf *ports More... | |
void | logAcceptError (const ConnectionPointer &tcpClient) const |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | doAccept (int fd, void *data) |
Method callback for whenever an FD is ready to accept a client connection. More... | |
Private Attributes | |
Subscription::Pointer | theCallSub |
used to generate AsyncCalls handling our events. More... | |
ConnectionPointer | conn |
AnyP::PortCfgPointer | listenPort_ |
configuration details of the listening port (if provided) More... | |
AsyncCall::Pointer | closer_ |
listen socket closure handler More... | |
Friends | |
class | AcceptLimiter |
Detailed Description
Listens on a Comm::Connection for new incoming connections and emits an active Comm::Connection descriptor for the new client.
Handles all event limiting required to quash inbound connection floods within the global FD limits of available Squid_MaxFD and client_ip_max_connections.
Fills the emitted connection with all connection details able to be looked up. Currently these are the local/remote IP:port details and the listening socket transparent-mode flag.
Definition at line 38 of file TcpAcceptor.h.
Member Typedef Documentation
◆ Pointer
Definition at line 43 of file TcpAcceptor.h.
Constructor & Destructor Documentation
◆ TcpAcceptor() [1/3]
|
private |
◆ TcpAcceptor() [2/3]
Comm::TcpAcceptor::TcpAcceptor | ( | const Comm::ConnectionPointer & | conn, |
const char * | note, | ||
const Subscription::Pointer & | aSub | ||
) |
Definition at line 43 of file TcpAcceptor.cc.
◆ TcpAcceptor() [3/3]
Comm::TcpAcceptor::TcpAcceptor | ( | const AnyP::PortCfgPointer & | listenPort, |
const char * | note, | ||
const Subscription::Pointer & | aSub | ||
) |
Definition at line 51 of file TcpAcceptor.cc.
Member Function Documentation
◆ acceptInto()
|
private |
acceptOne() helper: accept(2) a new TCP connection and fill in its details
- Return values
-
false indicates that details do not contain a usable TCP connection, but future attempts to accept may still be successful
Definition at line 345 of file TcpAcceptor.cc.
References StatCounters::accepts, Comm::ApplyTcpKeepAlive(), SquidConfig::client_ip_max_connections, clientdbEstablished(), COMM_INTERCEPTION, COMM_TRANSPARENT, commSetCloseOnExec(), commSetNonBlocking(), Config, conn, DBG_IMPORTANT, debugs, Ip::Qos::dirAccepted, Comm::Connection::enterOrphanage(), F(), Comm::Connection::fd, fd_open(), FD_SOCKET, fd_table, Comm::Connection::flags, Ip::Address::FreeAddr(), Ip::Qos::getNfConnmark(), Here, ignoreErrno(), incoming_sockets_accepted, Ip::Address::InitAddr(), Ip::Interceptor, Ip::Address::isIPv4(), Ip::Address::isIPv6(), Comm::Connection::local, Eui::Eui48::lookup(), Eui::Eui64::lookup(), MAX_IPSTRLEN, Comm::Connection::nfConnmark, Ip::Address::port(), Comm::Connection::remote, Comm::Connection::remoteEui48, Comm::Connection::remoteEui64, Ip::Address::setEmpty(), StatCounters::sock, statCounter, StatCounters::syscalls, Eui::TheConfig, ToSBuf(), Ip::Address::toStr(), and xstrerr().
◆ acceptNext()
|
protected |
Try and accept another connection (synchronous). If one is pending already the subscribed callback handler will be scheduled to handle it before this method returns.
Definition at line 313 of file TcpAcceptor.cc.
References conn, debugs, Comm::IsConnOpen(), and Must.
Referenced by doAccept().
◆ acceptOne()
|
private |
Definition at line 265 of file TcpAcceptor.cc.
References Assure, CallBack(), Comm::Connection::close(), Comm::COMM_ERROR, COMM_SELECT_READ, conn, CurrentException(), DBG_CRITICAL, debugs, Debug::Extra(), Comm::Connection::isOpen(), Comm::OK, and Comm::SetSelect().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in ConnStateData, ClientHttpRequest, Adaptation::Icap::ModXact, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Ipc::Forwarder, Ipc::Inquirer, and Ftp::Server.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by ConnStateData::callException(), Adaptation::Icap::Xaction::callException(), Ipc::Forwarder::callException(), Ipc::Inquirer::callException(), and Ftp::Server::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ doAccept()
|
staticprivate |
This private callback is called whenever a filedescriptor is ready to dupe itself and fob off an accept()ed connection
It will either do that accept operation. Or if there are not enough FD available to do the clone safely will push the listening FD into a list of deferred operations. The list gets kicked and the dupe/accept() actually done later when enough sockets become available.
Definition at line 211 of file TcpAcceptor.cc.
References acceptNext(), debugs, Comm::AcceptLimiter::defer(), fatal(), fatalf(), Comm::AcceptLimiter::Instance(), isOpen(), and Must.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 91 of file TcpAcceptor.cc.
References conn, AsyncJob::doneAll(), and Comm::IsConnOpen().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleClosure()
|
private |
called when listening descriptor is closed by an external force such as clientHttpConnectionsClose()
Definition at line 191 of file TcpAcceptor.cc.
Referenced by setListen().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ intendedForUserConnections()
|
inlineprivate |
Definition at line 107 of file TcpAcceptor.h.
References listenPort_.
◆ logAcceptError()
|
private |
Definition at line 249 of file TcpAcceptor.cc.
References accessLogLog(), CallBack(), Comm::Connection::local, Comm::Connection::remote, AccessLogEntry::setVirginUrlForMissingRequest(), ACLFilledChecklist::src_addr, AccessLogEntry::tcpClient, and AccessLogEntry::url.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ notify()
|
protected |
Definition at line 321 of file TcpAcceptor.cc.
References CommCommonCbParams::conn, conn, Comm::ERR_CLOSING, CommCommonCbParams::fd, CommCommonCbParams::flag, CommAcceptCbParams::port, ScheduleCallHere, and CommCommonCbParams::xerrno.
◆ okToAccept()
|
staticprotected |
Method to test if there are enough file descriptors to open a new client connection if not the accept() will be postponed
Definition at line 233 of file TcpAcceptor.cc.
References DBG_CRITICAL, debugs, fdNFree(), RESERVED_FD, and squid_curtime.
Referenced by Comm::AcceptLimiter::kick().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ setListen()
|
private |
New-style listen and accept routines
setListen simply registers our interest in an FD for listening. The constructor takes a callback to call when an FD has been accept()ed some time later.
Definition at line 151 of file TcpAcceptor.cc.
References SquidConfig::accept_filter, comm_add_close_handler(), Config, conn, DBG_CRITICAL, DBG_IMPORTANT, debugs, handleClosure(), JobCallback, Squid_MaxFD, xstrerr(), and xstrncpy().
◆ start()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 75 of file TcpAcceptor.cc.
References COMM_SELECT_READ, conn, debugs, Comm::IsConnOpen(), Must, and Comm::SetSelect().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ status()
|
overrideprivatevirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 124 of file TcpAcceptor.cc.
References MemBuf::append(), Packable::appendf(), conn, MemBuf::content(), MAX_IPSTRLEN, MemBuf::reset(), and AsyncJob::status().
◆ subscribe()
|
protected |
Subscribe a handler to receive calls back about new connections. Unsubscribes any existing subscribed handler.
Definition at line 60 of file TcpAcceptor.cc.
References debugs.
◆ swanSong()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 108 of file TcpAcceptor.cc.
References comm_remove_close_handler(), conn, debugs, Comm::AcceptLimiter::Instance(), Comm::IsConnOpen(), MYNAME, Comm::AcceptLimiter::removeDead(), and AsyncJob::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ unsubscribe()
|
protected |
Remove the currently waiting callback subscription. Already scheduled callbacks remain scheduled.
Definition at line 68 of file TcpAcceptor.cc.
References debugs.
Friends And Related Function Documentation
◆ AcceptLimiter
|
friend |
Definition at line 84 of file TcpAcceptor.h.
Member Data Documentation
◆ closer_
|
private |
Definition at line 97 of file TcpAcceptor.h.
◆ conn
|
private |
conn being listened on for new connections Reserved for read-only use.
Definition at line 91 of file TcpAcceptor.h.
◆ errcode
|
protected |
Definition at line 78 of file TcpAcceptor.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ listenPort_
|
private |
Definition at line 94 of file TcpAcceptor.h.
Referenced by intendedForUserConnections().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ theCallSub
|
private |
Definition at line 87 of file TcpAcceptor.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
The documentation for this class was generated from the following files:
- src/comm/TcpAcceptor.h
- src/comm/TcpAcceptor.cc