#include <ModXact.h>
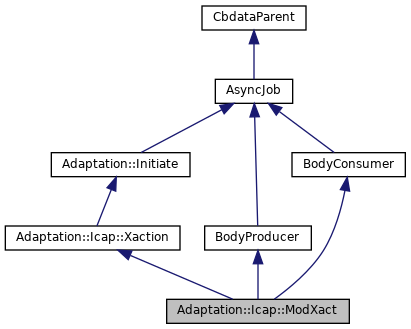
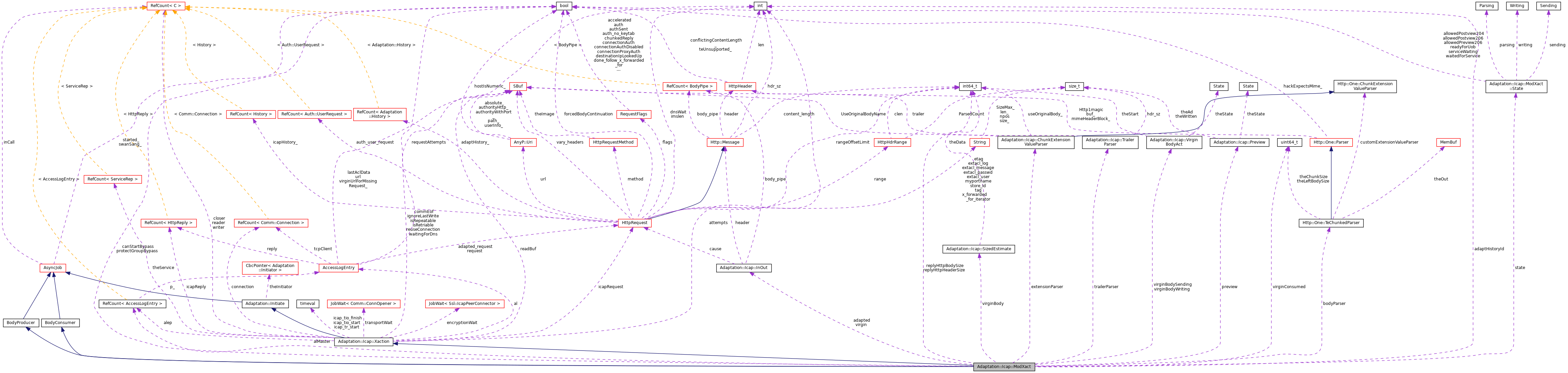
Classes | |
class | State |
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
Public Member Functions | |
ModXact (Http::Message *virginHeader, HttpRequest *virginCause, AccessLogEntry::Pointer &alp, ServiceRep::Pointer &s) | |
~ModXact () override | |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override |
void | noteMoreBodyDataAvailable (BodyPipe::Pointer) override |
void | noteBodyProductionEnded (BodyPipe::Pointer) override |
void | noteBodyProducerAborted (BodyPipe::Pointer) override |
void | startShoveling () override |
starts sending/receiving ICAP messages More... | |
void | handleCommWrote (size_t size) override |
void | handleCommRead (size_t size) override |
void | handleCommWroteHeaders () |
void | handleCommWroteBody () |
void | noteServiceReady () |
void | noteServiceAvailable () |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
void | detailError (const ErrorDetail::Pointer &errDetail) override |
record error detail in the virgin request if possible More... | |
void | clearError () override |
clear stored error details, if any; used for retries/repeats More... | |
AccessLogEntry::Pointer | masterLogEntry () override |
The master transaction log entry. More... | |
void | disableRetries () |
void | disableRepeats (const char *reason) |
bool | retriable () const |
bool | repeatable () const |
void | noteCommConnected (const CommConnectCbParams &io) |
called when the connection attempt to an ICAP service completes (successfully or not) More... | |
void | noteCommWrote (const CommIoCbParams &io) |
void | noteCommRead (const CommIoCbParams &io) |
void | noteCommTimedout (const CommTimeoutCbParams &io) |
void | noteCommClosed (const CommCloseCbParams &io) |
void | callEnd () override |
called right after the called job method More... | |
void | dnsLookupDone (std::optional< Ip::Address >) |
ServiceRep & | service () |
void | initiator (const CbcPointer< Initiator > &i) |
sets initiator More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
InOut | virgin |
InOut | adapted |
HttpRequest * | icapRequest |
sent (or at least created) ICAP request More... | |
HttpReply::Pointer | icapReply |
received ICAP reply, if any More... | |
int | attempts |
the number of times we tried to get to the service, including this time More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | noteInitiatorAborted () override |
void | handleSecuredPeer (Security::EncryptorAnswer &answer) |
void | openConnection () |
void | closeConnection () |
bool | haveConnection () const |
void | scheduleRead () |
void | scheduleWrite (MemBuf &buf) |
void | updateTimeout () |
void | cancelRead () |
bool | parseHttpMsg (Http::Message *msg) |
bool | mayReadMore () const |
bool | doneWithIo () const |
const char * | status () const override |
internal cleanup; do not call directly More... | |
void | setOutcome (const XactOutcome &xo) |
void | tellQueryAborted (bool final) |
void | sendAnswer (const Answer &answer) |
void | clearInitiator () |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
Adaptation::Icap::ServiceRep::Pointer | theService |
SBuf | readBuf |
bool | commEof |
bool | reuseConnection |
bool | isRetriable |
can retry on persistent connection failures More... | |
bool | isRepeatable |
can repeat if no or unsatisfactory response More... | |
bool | ignoreLastWrite |
bool | waitingForDns |
expecting a ipcache_nbgethostbyname() callback More... | |
AsyncCall::Pointer | reader |
AsyncCall::Pointer | writer |
AccessLogEntry::Pointer | alep |
icap.log entry More... | |
AccessLogEntry & | al |
short for *alep More... | |
timeval | icap_tr_start |
timeval | icap_tio_start |
timeval | icap_tio_finish |
CbcPointer< Initiator > | theInitiator |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (ModXact) | |
void | start () override |
called by AsyncStart; do not call directly More... | |
const HttpRequest & | virginRequest () const |
locates the request, either as a cause or as a virgin message itself More... | |
void | estimateVirginBody () |
void | makeAdaptedBodyPipe (const char *what) |
void | waitForService () |
bool | doneSending () const |
void | startWriting () |
void | writeMore () |
void | writePreviewBody () |
void | writePrimeBody () |
void | writeSomeBody (const char *label, size_t size) |
void | decideWritingAfterPreview (const char *previewKind) |
determine state.writing after we wrote the entire preview More... | |
void | startReading () |
void | readMore () |
bool | doneReading () const override |
bool | doneWriting () const override |
size_t | virginContentSize (const VirginBodyAct &act) const |
const char * | virginContentData (const VirginBodyAct &act) const |
bool | virginBodyEndReached (const VirginBodyAct &act) const |
void | makeRequestHeaders (MemBuf &buf) |
void | makeAllowHeader (MemBuf &buf) |
void | makeUsernameHeader (const HttpRequest *request, MemBuf &buf) |
void | addLastRequestChunk (MemBuf &buf) |
void | openChunk (MemBuf &buf, size_t chunkSize, bool ieof) |
void | closeChunk (MemBuf &buf) |
void | virginConsume () |
void | finishNullOrEmptyBodyPreview (MemBuf &buf) |
void | decideOnPreview () |
void | decideOnRetries () |
bool | shouldAllow204 () |
bool | shouldAllow206any () |
bool | shouldAllow206in () |
bool | shouldAllow206out () |
bool | canBackupEverything () const |
void | prepBackup (size_t expectedSize) |
void | backup (const MemBuf &buf) |
void | parseMore () |
void | parseHeaders () |
void | parseIcapHead () |
void | parseHttpHead () |
bool | parseHead (Http::Message *head) |
void | decideOnParsingBody () |
void | parseBody () |
void | parseIcapTrailer () |
void | maybeAllocateHttpMsg () |
void | handle100Continue () |
bool | validate200Ok () |
void | handle200Ok () |
void | handle204NoContent () |
void | handle206PartialContent () |
void | handleUnknownScode () |
void | bypassFailure () |
void | startSending () |
void | disableBypass (const char *reason, bool includeGroupBypass) |
void | prepEchoing () |
void | prepPartialBodyEchoing (uint64_t pos) |
void | echoMore () |
void | updateSources () |
Update the Http::Message sources. More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
void | stopReceiving () |
void | stopSending (bool nicely) |
void | stopWriting (bool nicely) |
void | stopParsing (const bool checkUnparsedData=true) |
void | stopBackup () |
void | fillPendingStatus (MemBuf &buf) const override |
void | fillDoneStatus (MemBuf &buf) const override |
bool | fillVirginHttpHeader (MemBuf &) const override |
template<class Part > | |
bool | parsePart (Part *part, const char *description) |
void | packHead (MemBuf &httpBuf, const Http::Message *head) |
void | encapsulateHead (MemBuf &icapBuf, const char *section, MemBuf &httpBuf, const Http::Message *head) |
bool | gotEncapsulated (const char *section) const |
bool | expectHttpHeader () const |
whether ICAP response header indicates HTTP header presence More... | |
bool | expectHttpBody () const |
whether ICAP response header indicates HTTP body presence More... | |
bool | expectIcapTrailers () const |
whether ICAP response header indicates ICAP trailers presence More... | |
void | checkConsuming () |
void | finalizeLogInfo () override |
void | useTransportConnection (const Comm::ConnectionPointer &) |
void | useIcapConnection (const Comm::ConnectionPointer &) |
react to the availability of a fully-ready ICAP connection More... | |
void | dieOnConnectionFailure () |
void | tellQueryAborted () |
void | maybeLog () |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
SizedEstimate | virginBody |
VirginBodyAct | virginBodyWriting |
VirginBodyAct | virginBodySending |
uint64_t | virginConsumed |
Preview | preview |
Http1::TeChunkedParser * | bodyParser |
bool | canStartBypass |
bool | protectGroupBypass |
int64_t | replyHttpHeaderSize |
int64_t | replyHttpBodySize |
int | adaptHistoryId |
adaptation history slot reservation More... | |
TrailerParser * | trailerParser |
ChunkExtensionValueParser | extensionParser |
class Adaptation::Icap::ModXact::State | state |
AccessLogEntry::Pointer | alMaster |
Master transaction AccessLogEntry. More... | |
JobWait< Comm::ConnOpener > | transportWait |
waits for a transport connection to the ICAP server to be established/opened More... | |
JobWait< Ssl::IcapPeerConnector > | encryptionWait |
waits for the established transport connection to be secured/encrypted More... | |
Comm::ConnectionPointer | connection |
open and, if necessary, secured connection to the ICAP server (or nil) More... | |
AsyncCall::Pointer | closer |
Detailed Description
Member Typedef Documentation
◆ Pointer [1/3]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [2/3]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [3/3]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ ModXact()
Adaptation::Icap::ModXact::ModXact | ( | Http::Message * | virginHeader, |
HttpRequest * | virginCause, | ||
AccessLogEntry::Pointer & | alp, | ||
ServiceRep::Pointer & | s | ||
) |
Definition at line 54 of file ModXact.cc.
References assert, debugs, Adaptation::Icap::Xaction::icapReply, HttpReply::protoPrefix, Adaptation::Icap::InOut::setCause(), Adaptation::Icap::InOut::setHeader(), Adaptation::Icap::Xaction::status(), and virgin.
◆ ~ModXact()
|
override |
Definition at line 1286 of file ModXact.cc.
Member Function Documentation
◆ addLastRequestChunk()
|
private |
Definition at line 369 of file ModXact.cc.
◆ backup()
|
private |
◆ bypassFailure()
|
private |
Definition at line 691 of file ModXact.cc.
◆ callEnd()
|
overridevirtualinherited |
called right after the called job method
Reimplemented from AsyncJob.
Definition at line 379 of file Xaction.cc.
References AsyncJob::callEnd(), and debugs.
◆ callException()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 665 of file ModXact.cc.
References Adaptation::Icap::Xaction::callException(), debugs, Here, and TextException::id().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBackupEverything()
|
private |
Definition at line 1694 of file ModXact.cc.
References TheBackupLimit.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cancelRead()
|
protectedinherited |
Definition at line 487 of file Xaction.cc.
References Must, and Comm::ReadCancel().
◆ CBDATA_CHILD()
|
private |
◆ checkConsuming()
|
private |
Definition at line 640 of file ModXact.cc.
References debugs.
◆ clearError()
|
overridevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 2004 of file ModXact.cc.
References HttpRequest::clearError().
◆ clearInitiator()
|
protectedinherited |
Definition at line 74 of file Initiate.cc.
◆ closeChunk()
|
private |
Definition at line 381 of file ModXact.cc.
References MemBuf::append(), and crlf.
◆ closeConnection()
|
protectedinherited |
Definition at line 218 of file Xaction.cc.
References comm_remove_close_handler(), commUnsetConnTimeout(), debugs, Adaptation::Icap::xoError, and Adaptation::Icap::xoGone.
◆ decideOnParsingBody()
|
private |
Definition at line 1141 of file ModXact.cc.
References debugs, Must, and Http::One::TeChunkedParser::parseExtensionValuesWith().
◆ decideOnPreview()
|
private |
Definition at line 1634 of file ModXact.cc.
References debugs, min(), Must, Adaptation::Icap::Config::preview_enable, TheBackupLimit, and Adaptation::Icap::TheConfig.
◆ decideOnRetries()
|
private |
Definition at line 1711 of file ModXact.cc.
◆ decideWritingAfterPreview()
|
private |
Definition at line 292 of file ModXact.cc.
References debugs.
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ detailError()
|
overridevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 1992 of file ModXact.cc.
References HttpRequest::detailError(), and ERR_ICAP_FAILURE.
◆ dieOnConnectionFailure()
|
privateinherited |
Definition at line 306 of file Xaction.cc.
References debugs, MakeNamedErrorDetail(), and TexcHere.
Referenced by Adaptation::Icap::Xaction::dnsLookupDone().
◆ disableBypass()
|
private |
Definition at line 717 of file ModXact.cc.
References debugs.
◆ disableRepeats()
|
inherited |
Definition at line 123 of file Xaction.cc.
References debugs.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ disableRetries()
|
inherited |
Definition at line 116 of file Xaction.cc.
References debugs.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ dnsLookupDone()
|
inherited |
Definition at line 187 of file Xaction.cc.
References assert, CallJobHere, Adaptation::Service::cfg(), Adaptation::Icap::Config::connect_timeout(), DBG_IMPORTANT, debugs, Adaptation::Icap::Xaction::dieOnConnectionFailure(), getOutgoingAddress(), Adaptation::ServiceConfig::host, JobCallback, Adaptation::Icap::Xaction::noteCommConnected(), Adaptation::ServiceConfig::port, Ip::Address::port(), Comm::Connection::remote, String::termedBuf(), and Adaptation::Icap::TheConfig.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 528 of file ModXact.cc.
References Adaptation::Icap::Xaction::doneAll().
◆ doneReading()
|
inlineoverrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 207 of file ModXact.h.
References Adaptation::Icap::Xaction::commEof, Adaptation::Icap::ModXact::State::doneParsing(), and state.
◆ doneSending()
|
private |
Definition at line 608 of file ModXact.cc.
◆ doneWithIo()
|
protectedinherited |
Definition at line 533 of file Xaction.cc.
◆ doneWriting()
|
inlineoverrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 208 of file ModXact.h.
References Adaptation::Icap::ModXact::State::doneWriting(), and state.
◆ echoMore()
|
private |
Definition at line 575 of file ModXact.cc.
◆ encapsulateHead()
|
private |
Definition at line 1581 of file ModXact.cc.
References HttpHeader::addEntry(), Packable::appendf(), MemBuf::contentSize(), HttpHeader::delById(), RefCount< C >::getRaw(), head, Http::Message::header, Http::Message::http_ver, HttpHeaderInitPos, Http::Message::inheritProperties(), HttpRequest::method, Must, Http::PROXY_AUTHENTICATE, HttpHeader::putStr(), HttpHeader::removeHopByHopEntries(), HttpReply::sline, Http::UPGRADE, and HttpRequest::url.
◆ estimateVirginBody()
|
private |
Definition at line 1819 of file ModXact.cc.
References Http::Message::body_pipe, BodyPipe::buf(), debugs, Http::Message::expectingBody(), Http::Message::header, MemBuf::max_capacity, Http::METHOD_NONE, Must, size, and TheBackupLimit.
◆ expectHttpBody()
|
private |
Definition at line 1123 of file ModXact.cc.
◆ expectHttpHeader()
|
private |
Definition at line 1118 of file ModXact.cc.
◆ expectIcapTrailers()
|
private |
Definition at line 1128 of file ModXact.cc.
References Http::ALLOW, DBG_IMPORTANT, debugs, Must, String::size(), and Http::TRAILER.
◆ fillDoneStatus()
|
overrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 1785 of file ModXact.cc.
References MemBuf::append(), Packable::appendf(), and Adaptation::Icap::Xaction::fillDoneStatus().
◆ fillPendingStatus()
|
overrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 1745 of file ModXact.cc.
References MemBuf::append(), Packable::appendf(), and Adaptation::Icap::Xaction::fillPendingStatus().
◆ fillVirginHttpHeader()
|
overrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 1982 of file ModXact.cc.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finalizeLogInfo()
|
overrideprivatevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 1315 of file ModXact.cc.
References assert, Http::Message::body_pipe, Adaptation::Service::cfg(), HttpRequest::client_addr, HttpReply::content_type, Adaptation::Icap::Xaction::finalizeLogInfo(), Http::Message::hdr_sz, HTTPMSGLOCK(), HttpRequest::icapHistory(), ICP_INVALID, Adaptation::Icap::History::log_uri, Adaptation::Icap::History::logType, max(), Adaptation::ServiceConfig::method, Must, prepareLogWithRequestDetails(), BodyPipe::producedSize(), String::size(), HttpReply::sline, Adaptation::Icap::History::ssluser, Http::StatusLine::status(), String::termedBuf(), and zero.
◆ finishNullOrEmptyBodyPreview()
|
private |
Definition at line 1731 of file ModXact.cc.
References Must.
◆ gotEncapsulated()
|
private |
Definition at line 1810 of file ModXact.cc.
◆ handle100Continue()
|
private |
Definition at line 896 of file ModXact.cc.
References Must.
◆ handle200Ok()
|
private |
Definition at line 915 of file ModXact.cc.
◆ handle204NoContent()
|
private |
Definition at line 923 of file ModXact.cc.
◆ handle206PartialContent()
|
private |
Definition at line 929 of file ModXact.cc.
◆ handleCommRead()
|
overridevirtual |
Implements Adaptation::Icap::Xaction.
Definition at line 567 of file ModXact.cc.
References current_time, and Must.
◆ handleCommWrote()
|
overridevirtual |
◆ handleCommWroteBody()
void Adaptation::Icap::ModXact::handleCommWroteBody | ( | ) |
Definition at line 478 of file ModXact.cc.
◆ handleCommWroteHeaders()
void Adaptation::Icap::ModXact::handleCommWroteHeaders | ( | ) |
Definition at line 218 of file ModXact.cc.
References Must.
◆ handleSecuredPeer()
|
protectedinherited |
Definition at line 717 of file Xaction.cc.
References assert, Security::EncryptorAnswer::conn, debugs, Security::EncryptorAnswer::error, Comm::Connection::fd, fd_table, CbcPointer< Cbc >::get(), Comm::Connection::isOpen(), MakeNamedErrorDetail(), Comm::Connection::noteClosure(), TexcHere, and Security::EncryptorAnswer::tunneled.
Referenced by Adaptation::Icap::Xaction::useTransportConnection().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ handleUnknownScode()
|
private |
Definition at line 1051 of file ModXact.cc.
References TexcHere.
◆ haveConnection()
|
protectedinherited |
Definition at line 540 of file Xaction.cc.
◆ initiator()
|
inherited |
Definition at line 54 of file Initiate.cc.
References Must.
Referenced by Adaptation::Initiator::initiateAdaptation().
◆ makeAdaptedBodyPipe()
|
private |
Definition at line 1859 of file ModXact.cc.
◆ makeAllowHeader()
|
private |
Definition at line 1519 of file ModXact.cc.
References Packable::appendf(), and debugs.
◆ makeRequestHeaders()
|
private |
Definition at line 1379 of file ModXact.cc.
References HttpRequest::adaptHistory(), NotePairs::add(), MemBuf::append(), Packable::appendf(), base64_encode_final(), base64_encode_init(), base64_encode_len, base64_encode_update(), MemBuf::clean(), HttpRequest::client_addr, MemBuf::content(), MemBuf::contentSize(), crlf, HttpRequest::extacl_passwd, HttpRequest::extacl_user, Time::FormatRfc1123(), Adaptation::History::getXxRecord(), NotePairs::hasPair(), Http::Message::header, Adaptation::ServiceConfig::host, HttpRequest::indirect_client_addr, MemBuf::init(), Ip::Address::isAnyAddr(), Ip::Address::isNoAddr(), SBuf::length(), Adaptation::Config::masterx_shared_name, MAX_IPSTRLEN, MAX_LOGIN_SZ, Adaptation::Config::metaHeaders(), Adaptation::History::metaHeaders, Adaptation::ServiceConfig::method, Adaptation::methodReqmod, Adaptation::methodRespmod, Adaptation::ServiceConfig::methodStr(), Must, Adaptation::ServiceConfig::port, Http::PROXY_AUTHENTICATE, Http::PROXY_AUTHORIZATION, String::rawBuf(), SBuf::rawContent(), Adaptation::Icap::Config::reuse_connections, Adaptation::Config::send_client_ip, Adaptation::Config::send_username, String::size(), squid_curtime, SQUIDSTRINGPH, SQUIDSTRINGPRINT, Adaptation::Icap::TheConfig, Ip::Address::toStr(), Adaptation::ServiceConfig::uri, and Adaptation::Config::use_indirect_client.
◆ makeUsernameHeader()
|
private |
Definition at line 1552 of file ModXact.cc.
References Packable::appendf(), HttpRequest::auth_user_request, base64_encode_final(), base64_encode_init(), base64_encode_len, base64_encode_update(), Adaptation::Icap::Config::client_username_encode, Adaptation::Icap::Config::client_username_header, HttpRequest::extacl_user, MAX_LOGIN_SZ, String::size(), String::termedBuf(), Adaptation::Icap::TheConfig, and Auth::UserRequest::username().
◆ masterLogEntry()
|
inlineoverridevirtual |
Reimplemented from Adaptation::Icap::Xaction.
Definition at line 182 of file ModXact.h.
References alMaster.
◆ maybeAllocateHttpMsg()
|
private |
Definition at line 730 of file ModXact.cc.
References Adaptation::methodReqmod, TexcHere, Adaptation::Icap::xoModified, and Adaptation::Icap::xoSatisfied.
◆ maybeLog()
|
privateinherited |
Definition at line 604 of file Xaction.cc.
References IcapLogfileStatus, icapLogLog(), and LOG_ENABLE.
◆ mayReadMore()
|
protectedinherited |
Definition at line 517 of file Xaction.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteBodyConsumerAborted()
|
overridevirtual |
Implements BodyProducer.
Definition at line 1279 of file ModXact.cc.
References MakeNamedErrorDetail().
◆ noteBodyProducerAborted()
|
overridevirtual |
◆ noteBodyProductionEnded()
|
overridevirtual |
◆ noteCommClosed()
|
inherited |
Definition at line 359 of file Xaction.cc.
References MakeNamedErrorDetail().
Referenced by Adaptation::Icap::Xaction::useIcapConnection().
◆ noteCommConnected()
|
inherited |
Definition at line 253 of file Xaction.cc.
References CommCommonCbParams::conn, CommCommonCbParams::flag, and Comm::OK.
Referenced by Adaptation::Icap::Xaction::dnsLookupDone().
◆ noteCommRead()
|
inherited |
Definition at line 426 of file Xaction.cc.
References CommCommonCbParams::conn, debugs, Comm::ENDFILE, CommCommonCbParams::flag, Comm::INPROGRESS, Must, Comm::OK, Comm::ReadNow(), CommIoCbParams::size, CommCommonCbParams::xerrno, Adaptation::Icap::xoRace, and xstrerr().
Referenced by Adaptation::Icap::Xaction::scheduleRead().
◆ noteCommTimedout()
|
inherited |
Definition at line 347 of file Xaction.cc.
References assert, debugs, and Here.
Referenced by Adaptation::Icap::Xaction::updateTimeout().
◆ noteCommWrote()
|
inherited |
Definition at line 329 of file Xaction.cc.
References debugs, CommCommonCbParams::flag, Must, Comm::OK, and CommIoCbParams::size.
Referenced by Adaptation::Icap::Xaction::scheduleWrite().
◆ noteInitiatorAborted()
|
overrideprotectedvirtualinherited |
Implements Adaptation::Initiate.
Definition at line 546 of file Xaction.cc.
References debugs, MakeNamedErrorDetail(), and Adaptation::Icap::xoGone.
◆ noteMoreBodyDataAvailable()
|
overridevirtual |
Implements BodyConsumer.
Definition at line 1233 of file ModXact.cc.
◆ noteMoreBodySpaceAvailable()
|
overridevirtual |
◆ noteServiceAvailable()
void Adaptation::Icap::ModXact::noteServiceAvailable | ( | ) |
◆ noteServiceReady()
void Adaptation::Icap::ModXact::noteServiceReady | ( | ) |
Definition at line 151 of file ModXact.cc.
References Must, and TexcHere.
Referenced by waitForService().
◆ openChunk()
Definition at line 376 of file ModXact.cc.
References Packable::appendf().
◆ openConnection()
|
protectedinherited |
Definition at line 161 of file Xaction.cc.
References assert, Adaptation::Service::cfg(), debugs, Adaptation::Icap::ServiceRep::getIdleConnection(), Adaptation::ServiceConfig::host, icapLookupDnsResults(), ipcache_nbgethostbyname(), Must, Adaptation::ServiceConfig::port, Adaptation::Icap::Config::reuse_connections, String::termedBuf(), and Adaptation::Icap::TheConfig.
◆ packHead()
|
private |
Definition at line 1628 of file ModXact.cc.
References head.
◆ parseBody()
|
private |
Definition at line 1161 of file ModXact.cc.
References BodyPipeCheckout::buf, BodyPipeCheckout::checkIn(), debugs, and Must.
◆ parseHead()
|
private |
Definition at line 1109 of file ModXact.cc.
References head.
◆ parseHeaders()
|
private |
Definition at line 746 of file ModXact.cc.
◆ parseHttpHead()
|
private |
Definition at line 1061 of file ModXact.cc.
References Must.
◆ parseHttpMsg()
|
protectedinherited |
Definition at line 497 of file Xaction.cc.
References debugs, error(), Http::Message::hdr_sz, Must, Http::Message::parse(), Http::Message::reset(), and Http::scNone.
◆ parseIcapHead()
|
private |
Definition at line 789 of file ModXact.cc.
References HttpRequest::adaptHistory(), HttpRequest::adaptLogHistory(), debugs, httpHeaderHasConnDir(), Adaptation::Config::masterx_shared_name, Must, Adaptation::History::recordMeta(), Http::scContinue, Http::scCreated, Http::scNoContent, Http::scOkay, Http::scPartialContent, String::size(), TexcHere, Adaptation::History::updateNextServices(), Adaptation::History::updateXxRecord(), and Http::X_NEXT_SERVICES.
◆ parseIcapTrailer()
|
private |
Parses ICAP trailers and stops parsing, if all trailer data have been received.
Definition at line 878 of file ModXact.cc.
References debugs.
◆ parseMore()
|
private |
Definition at line 650 of file ModXact.cc.
References debugs.
◆ parsePart()
|
private |
parses a message header or trailer
- Returns
- true on success
- false if more data is needed
- Exceptions
-
TextException on unrecoverable error
Definition at line 1091 of file ModXact.cc.
References debugs, error(), Must, and Http::scNone.
◆ prepBackup()
|
private |
◆ prepEchoing()
|
private |
Definition at line 949 of file ModXact.cc.
References Http::Message::body_pipe, BodyPipe::bodySize(), BodyPipe::bodySizeKnown(), MemBuf::clean(), MemBuf::content(), MemBuf::contentSize(), debugs, error(), RefCount< C >::getRaw(), Http::Message::header, Http::Message::inheritProperties(), MemBuf::init(), Must, Http::scNone, MemBuf::terminate(), and Adaptation::Icap::xoEcho.
◆ prepPartialBodyEchoing()
|
private |
Called when we received use-original-body chunk extension in 206 response. We actually start sending (echoing or not) in startSending().
Definition at line 1022 of file ModXact.cc.
References debugs, Must, and Adaptation::Icap::xoPartEcho.
◆ readMore()
|
private |
Definition at line 546 of file ModXact.cc.
References debugs.
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ repeatable()
|
inlineinherited |
Definition at line 53 of file Xaction.h.
References Adaptation::Icap::Xaction::isRepeatable.
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ retriable()
|
inlineinherited |
Definition at line 52 of file Xaction.h.
References Adaptation::Icap::Xaction::isRetriable.
◆ scheduleRead()
|
protectedinherited |
Definition at line 413 of file Xaction.cc.
References JobCallback, Must, Adaptation::Icap::Xaction::noteCommRead(), and Comm::Read().
◆ scheduleWrite()
|
protectedinherited |
Definition at line 316 of file Xaction.cc.
References JobCallback, Must, Adaptation::Icap::Xaction::noteCommWrote(), and Comm::Write().
◆ sendAnswer()
|
protectedinherited |
Definition at line 79 of file Initiate.cc.
References Adaptation::Initiator::noteAdaptationAnswer(), and ScheduleCallHere.
◆ service()
|
inherited |
Definition at line 110 of file Xaction.cc.
References Must.
Referenced by Adaptation::Icap::ConnWaiterDialer::ConnWaiterDialer().
◆ setOutcome()
|
protectedinherited |
Definition at line 560 of file Xaction.cc.
References debugs, and Adaptation::Icap::xoUnknown.
◆ shouldAllow204()
|
private |
Definition at line 1666 of file ModXact.cc.
◆ shouldAllow206any()
|
private |
Definition at line 1675 of file ModXact.cc.
References Adaptation::Icap::Config::allow206_enable, and Adaptation::Icap::TheConfig.
◆ shouldAllow206in()
|
private |
Definition at line 1682 of file ModXact.cc.
◆ shouldAllow206out()
|
private |
Definition at line 1688 of file ModXact.cc.
◆ start()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 88 of file ModXact.cc.
References Adaptation::History::recordXactStart(), and Adaptation::Icap::Xaction::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startReading()
|
private |
Definition at line 535 of file ModXact.cc.
References Must.
◆ startSending()
|
private |
Definition at line 769 of file ModXact.cc.
References Adaptation::Answer::Forward().
◆ startShoveling()
|
overridevirtual |
Implements Adaptation::Icap::Xaction.
Definition at line 189 of file ModXact.cc.
References MemBuf::content(), current_time, debugs, MemBuf::init(), Must, and MemBuf::terminate().
◆ startWriting()
|
private |
Definition at line 179 of file ModXact.cc.
◆ status()
|
overrideprotectedvirtualinherited |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 635 of file Xaction.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), and MemBuf::terminate().
Referenced by ModXact().
◆ stopBackup()
|
private |
Definition at line 518 of file ModXact.cc.
References debugs.
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by Client::cleanAdaptation(), Client::doneSendingRequestBody(), Client::endAdaptedBodyConsumption(), ClientHttpRequest::endRequestSatisfaction(), Client::handleAdaptedBodyProducerAborted(), Client::handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), ClientHttpRequest::noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), Client::serverComplete(), Client::swanSong(), and ClientHttpRequest::~ClientHttpRequest().
◆ stopParsing()
|
private |
Definition at line 1213 of file ModXact.cc.
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), Client::serverComplete2(), and ConnStateData::~ConnStateData().
◆ stopReceiving()
|
private |
◆ stopSending()
|
private |
Definition at line 614 of file ModXact.cc.
◆ stopWriting()
|
private |
Definition at line 486 of file ModXact.cc.
References debugs.
◆ swanSong()
|
overrideprivatevirtual |
Reimplemented from AsyncJob.
Definition at line 1293 of file ModXact.cc.
References debugs, MakeNamedErrorDetail(), Adaptation::History::recordXactFinish(), and Adaptation::Icap::Xaction::swanSong().
◆ tellQueryAborted() [1/2]
|
privateinherited |
Definition at line 591 of file Xaction.cc.
References CallJobHere1.
◆ tellQueryAborted() [2/2]
|
protectedinherited |
Definition at line 87 of file Initiate.cc.
References Adaptation::Answer::Error().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ updateSources()
|
private |
Definition at line 2015 of file ModXact.cc.
References Must, Http::Message::srcIcap, and Http::Message::srcIcaps.
◆ updateTimeout()
|
protectedinherited |
Definition at line 395 of file Xaction.cc.
References commSetConnTimeout(), commUnsetConnTimeout(), Adaptation::Icap::Config::io_timeout(), JobCallback, Must, Adaptation::Icap::Xaction::noteCommTimedout(), and Adaptation::Icap::TheConfig.
◆ useIcapConnection()
|
privateinherited |
Definition at line 290 of file Xaction.cc.
References assert, asyncCall(), comm_add_close_handler(), Comm::IsConnOpen(), and Adaptation::Icap::Xaction::noteCommClosed().
◆ useTransportConnection()
|
privateinherited |
React to the availability of a transport connection to the ICAP service. The given connection may (or may not) be secured already.
Definition at line 268 of file Xaction.cc.
References assert, asyncCallback, Adaptation::Icap::Config::connect_timeout(), Comm::Connection::fd, fd_table, Adaptation::Icap::Xaction::handleSecuredPeer(), Comm::IsConnOpen(), and Adaptation::Icap::TheConfig.
◆ validate200Ok()
|
private |
Definition at line 887 of file ModXact.cc.
References Adaptation::methodReqmod, and Adaptation::methodRespmod.
◆ virginBodyEndReached()
|
private |
Definition at line 395 of file ModXact.cc.
References Adaptation::Icap::VirginBodyAct::active(), and Adaptation::Icap::VirginBodyAct::offset().
◆ virginConsume()
|
private |
Definition at line 424 of file ModXact.cc.
References BodyPipe::buf(), BodyPipe::consume(), MemBuf::contentSize(), debugs, min(), Must, size, MemBuf::spaceSize(), and BodyPipe::status().
◆ virginContentData()
|
private |
Definition at line 416 of file ModXact.cc.
References Adaptation::Icap::VirginBodyAct::active(), Must, and Adaptation::Icap::VirginBodyAct::offset().
◆ virginContentSize()
|
private |
Definition at line 404 of file ModXact.cc.
References Adaptation::Icap::VirginBodyAct::active(), Must, and Adaptation::Icap::VirginBodyAct::offset().
◆ virginRequest()
|
private |
Definition at line 386 of file ModXact.cc.
References Must.
◆ waitForService()
|
private |
Definition at line 109 of file ModXact.cc.
References debugs, JobCallback, Must, noteServiceAvailable(), noteServiceReady(), Adaptation::srvBlock, Adaptation::srvWait, and TexcHere.
◆ writeMore()
|
private |
Definition at line 238 of file ModXact.cc.
◆ writePreviewBody()
|
private |
◆ writePrimeBody()
|
private |
◆ writeSomeBody()
|
private |
Definition at line 319 of file ModXact.cc.
References MemBuf::append(), MemBuf::clean(), MemBuf::contentSize(), debugs, MemBuf::hasContent(), MemBuf::init(), min(), Must, and size.
Member Data Documentation
◆ adapted
◆ adaptHistoryId
◆ al
|
protectedinherited |
◆ alep
|
protectedinherited |
◆ alMaster
|
private |
Definition at line 373 of file ModXact.h.
Referenced by masterLogEntry().
◆ attempts
|
inherited |
Definition at line 67 of file Xaction.h.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ bodyParser
|
private |
◆ canStartBypass
◆ closer
|
privateinherited |
◆ commEof
|
protectedinherited |
Definition at line 141 of file Xaction.h.
Referenced by Adaptation::Icap::OptXact::doneReading(), and doneReading().
◆ connection
|
privateinherited |
◆ encryptionWait
|
privateinherited |
◆ extensionParser
|
private |
◆ icap_tio_finish
|
protectedinherited |
Definition at line 156 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::Xaction().
◆ icap_tio_start
|
protectedinherited |
Definition at line 155 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::Xaction().
◆ icap_tr_start
|
protectedinherited |
Definition at line 154 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::Xaction().
◆ icapReply
|
inherited |
◆ icapRequest
|
inherited |
Definition at line 63 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::Xaction().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ ignoreLastWrite
|
protectedinherited |
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ isRepeatable
|
protectedinherited |
Definition at line 144 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::repeatable().
◆ isRetriable
|
protectedinherited |
Definition at line 143 of file Xaction.h.
Referenced by Adaptation::Icap::Xaction::retriable().
◆ preview
◆ protectGroupBypass
|
private |
◆ readBuf
|
protectedinherited |
◆ reader
|
protectedinherited |
◆ replyHttpBodySize
|
private |
◆ replyHttpHeaderSize
|
private |
◆ reuseConnection
|
protectedinherited |
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ state
|
private |
Referenced by doneReading(), and doneWriting().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ theInitiator
|
protectedinherited |
Definition at line 51 of file Initiate.h.
◆ theService
|
protectedinherited |
◆ trailerParser
|
private |
◆ transportWait
|
privateinherited |
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ virgin
InOut Adaptation::Icap::ModXact::virgin |
◆ virginBody
|
private |
◆ virginBodySending
|
private |
◆ virginBodyWriting
|
private |
◆ virginConsumed
|
private |
◆ waitingForDns
|
protectedinherited |
◆ writer
|
protectedinherited |
The documentation for this class was generated from the following files:
- src/adaptation/icap/ModXact.h
- src/adaptation/icap/ModXact.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products