#include <Notes.h>
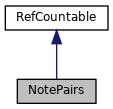
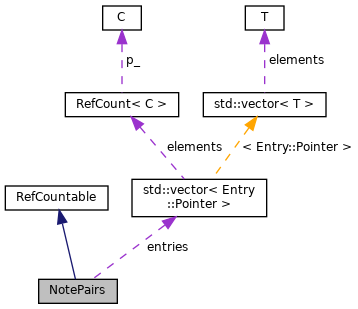
Classes | |
class | Entry |
Used to store a note key/value pair. More... | |
Public Types | |
typedef RefCount< NotePairs > | Pointer |
typedef std::vector< Entry::Pointer > | Entries |
The key/value pair entries. More... | |
typedef std::vector< SBuf > | Names |
Public Member Functions | |
NotePairs () | |
NotePairs & | operator= (NotePairs const &)=delete |
NotePairs (NotePairs const &)=delete | |
void | append (const NotePairs *src) |
Append the entries of the src NotePairs list to our list. More... | |
void | replaceOrAddOrAppend (const NotePairs *src, const Names &appendables) |
void | replaceOrAdd (const NotePairs *src) |
void | appendNewOnly (const NotePairs *src) |
bool | find (SBuf &resultNote, const char *noteKey, const char *sep=",") const |
const char * | findFirst (const char *noteKey) const |
void | add (const SBuf &key, const SBuf &value) |
void | add (const char *key, const char *value) |
void | remove (const char *key) |
void | remove (const SBuf &key) |
void | addStrList (const SBuf &key, const SBuf &values, const CharacterSet &delimiters) |
bool | hasPair (const SBuf &key, const SBuf &value) const |
void | print (std::ostream &os, const char *nameValueSeparator, const char *entryTerminator) const |
bool | empty () const |
void | clear () |
const Entries & | expandListEntries (const CharacterSet *delimiters) const |
Private Attributes | |
Entries | entries |
The key/value pair entries. More... | |
Detailed Description
Member Typedef Documentation
◆ Entries
typedef std::vector<Entry::Pointer> NotePairs::Entries |
◆ Names
typedef std::vector<SBuf> NotePairs::Names |
◆ Pointer
typedef RefCount<NotePairs> NotePairs::Pointer |
Constructor & Destructor Documentation
◆ NotePairs() [1/2]
◆ NotePairs() [2/2]
|
delete |
Member Function Documentation
◆ add() [1/2]
void NotePairs::add | ( | const char * | key, |
const char * | value | ||
) |
◆ add() [2/2]
Adds a note key and value to the notes list. If the key name already exists in the list, add the given value to its set of values.
Definition at line 317 of file Notes.cc.
References entries.
Referenced by ClientHttpRequest::logRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), redirectHandleReply(), redirectStart(), Adaptation::Ecap::XactionRep::start(), storeIdStart(), Ssl::Helper::Submit(), Note::updateNotePairs(), and UpdateRequestNotes().
◆ addStrList()
void NotePairs::addStrList | ( | const SBuf & | key, |
const SBuf & | values, | ||
const CharacterSet & | delimiters | ||
) |
Adds a note key and values strList to the notes list. If the key name already exists in the list, add the new values to its set of values.
Definition at line 364 of file Notes.cc.
References AppendTokens(), and entries.
Referenced by Note::updateNotePairs().
◆ append()
void NotePairs::append | ( | const NotePairs * | src | ) |
Definition at line 379 of file Notes.cc.
References entries.
Referenced by Http::One::Server::buildHttpRequest(), externalAclHandleReply(), redirectHandleReply(), replaceOrAdd(), replaceOrAddOrAppend(), and ExternalACLEntry::update().
◆ appendNewOnly()
void NotePairs::appendNewOnly | ( | const NotePairs * | src | ) |
◆ clear()
|
inline |
Definition at line 262 of file Notes.h.
References entries.
Referenced by ExternalACLEntry::update().
◆ empty()
|
inline |
- Returns
- true if there are not entries in the list
Definition at line 260 of file Notes.h.
References entries.
Referenced by HttpRequest::hasNotes(), ConnStateData::hasNotes(), Helper::operator<<(), and Note::updateNotePairs().
◆ expandListEntries()
const NotePairs::Entries & NotePairs::expandListEntries | ( | const CharacterSet * | delimiters | ) | const |
If delimiters are provided, returns another Entries, converting each single multi-token pair to multiple single-token pairs; returns existing entries otherwise.
Definition at line 351 of file Notes.cc.
References AppendTokens(), and entries.
Referenced by Acl::NoteCheck::matchNotes().
◆ find()
bool NotePairs::find | ( | SBuf & | resultNote, |
const char * | noteKey, | ||
const char * | sep = "," |
||
) | const |
- Parameters
-
resultNote a comma separated list of notes with key 'noteKey'.
- Returns
- true if there are entries with the given 'noteKey'. Use findFirst() instead when a unique kv-pair is needed.
Definition at line 281 of file Notes.cc.
References SBuf::append(), SBuf::clear(), entries, SBuf::isEmpty(), and SBuf::length().
Referenced by Format::Format::assemble(), and Auth::UserRequest::denyMessageFromHelper().
◆ findFirst()
const char * NotePairs::findFirst | ( | const char * | noteKey | ) | const |
- Returns
- the first note value for this key or an empty string.
Definition at line 302 of file Notes.cc.
References entries.
Referenced by ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientStoreIdDone(), externalAclHandleReply(), and UpdateRequestNotes().
◆ hasPair()
- Returns
- true if the key/value pair is already stored
Definition at line 370 of file Notes.cc.
References entries.
Referenced by appendNewOnly(), Adaptation::Icap::ModXact::makeRequestHeaders(), and Adaptation::Ecap::XactionRep::start().
◆ operator=()
◆ print()
void NotePairs::print | ( | std::ostream & | os, |
const char * | nameValueSeparator, | ||
const char * | entryTerminator | ||
) | const |
Reports all entries (if any), printing exactly four items for each: entry name, nameValueSeparator, entry value, and entry terminator.
Definition at line 295 of file Notes.cc.
References entries.
Referenced by Format::Format::assemble(), and Helper::operator<<().
◆ remove() [1/2]
void NotePairs::remove | ( | const char * | key | ) |
Remove all notes with a given key. If keyLen is not provided, the key is assumed null-terminated.
Definition at line 323 of file Notes.cc.
References entries.
Referenced by replaceOrAdd(), replaceOrAddOrAppend(), Note::updateNotePairs(), and UpdateRequestNotes().
◆ remove() [2/2]
void NotePairs::remove | ( | const SBuf & | key | ) |
◆ replaceOrAdd()
void NotePairs::replaceOrAdd | ( | const NotePairs * | src | ) |
◆ replaceOrAddOrAppend()
Member Data Documentation
◆ entries
|
private |
Definition at line 269 of file Notes.h.
Referenced by add(), addStrList(), append(), appendNewOnly(), clear(), empty(), expandListEntries(), find(), findFirst(), hasPair(), print(), remove(), replaceOrAdd(), and replaceOrAddOrAppend().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products