#include <HttpRequest.h>
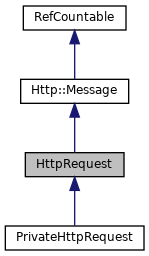

Public Types | |
typedef RefCount< HttpRequest > | Pointer |
enum | Sources { srcUnknown = 0, srcHttps = 1 << 0, srcFtps = 1 << 1, srcIcaps = 1 << 2, srcEcaps = 1 << 3, srcHttp = 1 << (16 + 0), srcFtp = 1 << (16 + 1), srcIcap = 1 << (16 + 2), srcEcap = 1 << (16 + 3), srcWhois = 1 << (16 + 15), srcUnsafe = 0xFFFF0000, srcSafe = 0x0000FFFF } |
Who may have created or modified this message? More... | |
enum | ParseState { psReadyToParseStartLine = 0, psReadyToParseHeaders, psParsed, psError } |
parse state of HttpReply or HttpRequest More... | |
Public Member Functions | |
HttpRequest (const MasterXaction::Pointer &) | |
HttpRequest (const HttpRequestMethod &aMethod, AnyP::ProtocolType aProtocol, const char *schemeImage, const char *aUrlpath, const MasterXaction::Pointer &) | |
~HttpRequest () override | |
void | reset () override |
void | initHTTP (const HttpRequestMethod &aMethod, AnyP::ProtocolType aProtocol, const char *schemeImage, const char *aUrlpath) |
HttpRequest * | clone () const override |
bool | maybeCacheable () |
bool | conditional () const |
has at least one recognized If-* header More... | |
bool | canHandle1xx () const |
whether the client is likely to be able to handle a 1xx reply More... | |
char * | canonicalCleanUrl () const |
Adaptation::History::Pointer | adaptLogHistory () const |
Returns possibly nil history, creating it if adapt. logging is enabled. More... | |
Adaptation::History::Pointer | adaptHistory (bool createIfNone=false) const |
Returns possibly nil history, creating it if requested. More... | |
void | adaptHistoryImport (const HttpRequest &them) |
Makes their history ours, throwing on conflicts. More... | |
Adaptation::Icap::History::Pointer | icapHistory () const |
Returns possibly nil history, creating it if icap logging is enabled. More... | |
void | prepForPeering (const CachePeer &peer) |
get ready to be sent to the given cache_peer, including originserver More... | |
void | prepForDirect () |
get ready to be sent directly to an origin server, excluding originserver More... | |
void | recordLookup (const Dns::LookupDetails &detail) |
void | detailError (const err_type c, const ErrorDetail::Pointer &d) |
sets error detail if no earlier detail was available More... | |
void | clearError () |
clear error details, useful for retries/repeats More... | |
void | manager (const CbcPointer< ConnStateData > &aMgr, const AccessLogEntryPointer &al) |
associates the request with a from-client connection manager More... | |
const SBuf & | effectiveRequestUri () const |
RFC 7230 section 5.5 - Effective Request URI. More... | |
bool | multipartRangeRequest () const |
bool | parseFirstLine (const char *start, const char *end) override |
bool | expectingBody (const HttpRequestMethod &unused, int64_t &) const override |
bool | bodyNibbled () const |
int | prefixLen () const |
void | swapOut (StoreEntry *e) |
void | pack (Packable *p) const |
ConnStateData * | pinnedConnection () |
const SBuf | storeId () |
void | ignoreRange (const char *reason) |
forgets about the cached Range header (for a reason) More... | |
int64_t | getRangeOffsetLimit () |
NotePairs::Pointer | notes () |
bool | hasNotes () const |
void | configureContentLengthInterpreter (Http::ContentLengthInterpreter &) override |
configures the interpreter as needed More... | |
Http::StatusCode | checkEntityFraming () const |
bool | parseHeader (Http1::Parser &hp) |
bool | parseHeader (const char *buffer, const size_t size) |
void | packInto (Packable *, bool full_uri) const |
produce a message copy, except for a few connection-specific settings More... | |
void | setContentLength (int64_t) |
[re]sets Content-Length header and cached value More... | |
bool | persistent () const |
void | putCc (const HttpHdrCc &) |
bool | parse (const char *buf, const size_t sz, bool eol, Http::StatusCode *error) |
bool | parseCharBuf (const char *buf, ssize_t end) |
int | httpMsgParseStep (const char *buf, int len, int atEnd) |
virtual int | httpMsgParseError () |
void | firstLineBuf (MemBuf &) |
useful for debugging More... | |
Static Public Member Functions | |
static void | httpRequestPack (void *obj, Packable *p) |
static HttpRequest * | FromUrl (const SBuf &url, const MasterXaction::Pointer &, const HttpRequestMethod &method=Http::METHOD_GET) |
static HttpRequest * | FromUrlXXX (const char *url, const MasterXaction::Pointer &, const HttpRequestMethod &method=Http::METHOD_GET) |
Protected Member Functions | |
void | clean () |
void | init () |
void | packFirstLineInto (Packable *p, bool full_uri) const override |
bool | sanityCheckStartLine (const char *buf, const size_t hdr_len, Http::StatusCode *error) override |
void | hdrCacheInit () override |
bool | inheritProperties (const Http::Message *) override |
bool | parseHeader (Http1::Parser &, Http::ContentLengthInterpreter &) |
Private Member Functions | |
MEMPROXY_CLASS (HttpRequest) | |
Private Attributes | |
Adaptation::History::Pointer | adaptHistory_ |
per-HTTP transaction info More... | |
Adaptation::Icap::History::Pointer | icapHistory_ |
per-HTTP transaction info More... | |
int64_t | rangeOffsetLimit |
NotePairs::Pointer | theNotes |
Detailed Description
Definition at line 48 of file HttpRequest.h.
Member Typedef Documentation
◆ Pointer
typedef RefCount<HttpRequest> HttpRequest::Pointer |
Definition at line 53 of file HttpRequest.h.
Member Enumeration Documentation
◆ ParseState
|
inherited |
◆ Sources
|
inherited |
Constructor & Destructor Documentation
◆ HttpRequest() [1/2]
HttpRequest::HttpRequest | ( | const MasterXaction::Pointer & | mx | ) |
◆ HttpRequest() [2/2]
HttpRequest::HttpRequest | ( | const HttpRequestMethod & | aMethod, |
AnyP::ProtocolType | aProtocol, | ||
const char * | schemeImage, | ||
const char * | aUrlpath, | ||
const MasterXaction::Pointer & | mx | ||
) |
Definition at line 50 of file HttpRequest.cc.
References assert, debugs, init(), and initHTTP().
◆ ~HttpRequest()
|
override |
Definition at line 61 of file HttpRequest.cc.
Member Function Documentation
◆ adaptHistory()
Adaptation::History::Pointer HttpRequest::adaptHistory | ( | bool | createIfNone = false | ) | const |
Definition at line 403 of file HttpRequest.cc.
References adaptHistory_, and debugs.
Referenced by adaptLogHistory(), Format::Format::assemble(), inheritProperties(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Ecap::XactionRep::masterxSharedValue(), Acl::AdaptationServiceCheck::match(), Adaptation::Icap::ModXact::parseIcapHead(), Adaptation::Iterator::start(), Adaptation::Iterator::step(), Adaptation::Ecap::XactionRep::updateHistory(), Adaptation::Iterator::updatePlan(), and Ftp::Server::writeErrorReply().
◆ adaptHistoryImport()
void HttpRequest::adaptHistoryImport | ( | const HttpRequest & | them | ) |
Definition at line 420 of file HttpRequest.cc.
References adaptHistory_, and Must.
◆ adaptLogHistory()
Adaptation::History::Pointer HttpRequest::adaptLogHistory | ( | ) | const |
Definition at line 414 of file HttpRequest.cc.
References adaptHistory(), and Log::TheConfig.
Referenced by Adaptation::Icap::ModXact::parseIcapHead(), prepareLogWithRequestDetails(), Adaptation::Ecap::XactionRep::start(), Adaptation::Ecap::XactionRep::swanSong(), and Adaptation::Ecap::XactionRep::updateHistory().
◆ bodyNibbled()
bool HttpRequest::bodyNibbled | ( | ) | const |
Definition at line 439 of file HttpRequest.cc.
References Http::Message::body_pipe, and BodyPipe::consumedSize().
Referenced by FwdState::checkRetry(), and FwdState::reforward().
◆ canHandle1xx()
bool HttpRequest::canHandle1xx | ( | ) | const |
Definition at line 634 of file HttpRequest.cc.
References Http::EXPECT, HttpHeader::has(), Http::Message::header, Http::Message::http_ver, and Http::ProtocolVersion().
Referenced by HttpStateData::handle1xx().
◆ canonicalCleanUrl()
char * HttpRequest::canonicalCleanUrl | ( | ) | const |
- Returns
- a pointer to a local static buffer containing request URI that honors strip_query_terms and %-encodes unsafe URI characters
Definition at line 812 of file HttpRequest.cc.
References effectiveRequestUri(), AnyP::Uri::getScheme(), method, url, and urlCanonicalCleanWithoutRequest().
Referenced by ClientHttpRequest::setLogUriToRequestUri(), and urlCanonicalFakeHttps().
◆ checkEntityFraming()
Http::StatusCode HttpRequest::checkEntityFraming | ( | ) | const |
Check whether the message framing headers are valid.
- Returns
- Http::scNone or an HTTP error status
Definition at line 646 of file HttpRequest.cc.
References HttpHeader::chunked(), HttpHeader::conflictingContentLength(), Http::Message::content_length, Http::Message::header, Http::Message::http_ver, HttpRequestMethod::id(), method, Http::METHOD_DELETE, Http::METHOD_GET, Http::METHOD_HEAD, Http::METHOD_LINK, Http::METHOD_POST, Http::METHOD_PUT, Http::METHOD_UNLINK, Http::ProtocolVersion(), Http::scBadRequest, Http::scLengthRequired, Http::scNone, Http::scNotImplemented, and HttpHeader::unsupportedTe().
Referenced by clientProcessRequest().
◆ clean()
|
protected |
Definition at line 120 of file HttpRequest.cc.
References adaptHistory_, auth_user_request, Http::Message::body_pipe, Http::Message::cache_control, HttpHeader::clean(), String::clean(), AnyP::Uri::clear(), SBuf::clear(), etag, extacl_log, extacl_message, extacl_passwd, extacl_user, Http::Message::header, icapHistory_, myportname, range, tag, theNotes, url, and vary_headers.
Referenced by reset(), and ~HttpRequest().
◆ clearError()
void HttpRequest::clearError | ( | ) |
Definition at line 464 of file HttpRequest.cc.
References Error::clear(), debugs, and error.
Referenced by Adaptation::Icap::ModXact::clearError(), FwdState::connectStart(), and Adaptation::Iterator::step().
◆ clone()
|
overridevirtual |
Implements Http::Message.
Definition at line 174 of file HttpRequest.cc.
References HttpHeader::append(), assert, Http::Message::body_pipe, error, etag, extacl_log, extacl_message, Http::Message::hdr_sz, hdrCacheInit(), Http::Message::header, hier, Http::Message::http_ver, HttpRequest(), ims, imslen, inheritProperties(), lastmod, masterXaction, method, Http::Message::pstate, tag, url, and vary_headers.
Referenced by ClientRequestContext::clientRedirectDone().
◆ conditional()
bool HttpRequest::conditional | ( | ) | const |
Definition at line 571 of file HttpRequest.cc.
References flags, HttpHeader::has(), Http::Message::header, Http::IF_MATCH, Http::IF_NONE_MATCH, and RequestFlags::ims.
Referenced by clientReplyContext::cacheHit().
◆ configureContentLengthInterpreter()
|
inlineoverridevirtual |
Implements Http::Message.
Definition at line 247 of file HttpRequest.h.
◆ detailError()
|
inline |
Definition at line 101 of file HttpRequest.h.
References error, and Error::update().
Referenced by Adaptation::Icap::ModXact::detailError(), ClientHttpRequest::handleAdaptationBlock(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), and ClientHttpRequest::noteBodyProducerAborted().
◆ effectiveRequestUri()
const SBuf & HttpRequest::effectiveRequestUri | ( | ) | const |
Definition at line 743 of file HttpRequest.cc.
References AnyP::Uri::absolute(), AnyP::Uri::authority(), AnyP::Uri::getScheme(), HttpRequestMethod::id(), method, Http::METHOD_CONNECT, AnyP::PROTO_AUTHORITY_FORM, and url.
Referenced by Format::Format::assemble(), ConnStateData::buildFakeRequest(), HttpStateData::buildRequestPrefix(), canonicalCleanUrl(), carpSelectParent(), ClientRequestContext::clientAccessCheckDone(), ClientRequestContext::clientRedirectDone(), ErrorState::compileLegacyCode(), AccessLogEntry::effectiveVirginUrl(), ClientRequestContext::hostHeaderVerifyFailed(), htcpClear(), HttpStateData::httpBuildRequestHeader(), Client::maybePurgeOthers(), packFirstLineInto(), clientReplyContext::purgeAllCached(), clientReplyContext::purgeDoPurge(), refreshCheck(), ClientHttpRequest::resetRequest(), ClientHttpRequest::resetRequestXXX(), Ssl::ServerBump::ServerBump(), storeId(), PeerSelector::url(), Ftp::UrlWith2f(), and ACLFilledChecklist::verifyAle().
◆ expectingBody()
|
overridevirtual |
Implements Http::Message.
Definition at line 487 of file HttpRequest.cc.
References HttpHeader::chunked(), Http::Message::content_length, and Http::Message::header.
◆ firstLineBuf()
|
inherited |
Definition at line 270 of file Message.cc.
◆ FromUrl()
|
static |
Definition at line 516 of file HttpRequest.cc.
References HttpRequest(), method, AnyP::Uri::parse(), and url.
Referenced by Downloader::buildRequest(), FromUrlXXX(), Ftp::Server::parseOneRequest(), TestHttpRequest::testCreateFromUrl(), and TestHttpRequest::testIPv6HostColonBug().
◆ FromUrlXXX()
|
static |
- Deprecated:
- use SBuf variant instead
Definition at line 527 of file HttpRequest.cc.
References FromUrl(), method, and url.
Referenced by Http::One::Server::buildHttpRequest(), htcpUnpackSpecifier(), icpGetRequest(), MimeIcon::load(), netdbExchangeStart(), peerCountMcastPeersCreateAndSend(), peerDigestRequest(), UrnState::setUriResFromRequest(), Mgr::Inquirer::start(), and storeDigestRewriteStart().
◆ getRangeOffsetLimit()
int64_t HttpRequest::getRangeOffsetLimit | ( | ) |
Definition at line 593 of file HttpRequest.cc.
References Acl::Answer::allowed(), client_addr, Config, debugs, ACLChecklist::fastCheck(), ACLFilledChecklist::my_addr, my_addr, rangeOffsetLimit, SquidConfig::rangeOffsetLimit, and ACLFilledChecklist::src_addr.
Referenced by Http::Stream::buildRangeHeader(), CheckQuickAbortIsReasonable(), and HttpStateData::decideIfWeDoRanges().
◆ hasNotes()
|
inline |
Definition at line 245 of file HttpRequest.h.
References NotePairs::empty(), and theNotes.
Referenced by Http::One::Server::buildHttpRequest().
◆ hdrCacheInit()
|
overrideprotectedvirtual |
Reimplemented from Http::Message.
Definition at line 378 of file HttpRequest.cc.
References assert, HttpHeader::getRange(), Http::Message::hdrCacheInit(), Http::Message::header, and range.
Referenced by clone().
◆ httpMsgParseError()
|
virtualinherited |
Reimplemented in HttpReply.
Definition at line 221 of file Message.cc.
Referenced by HttpReply::httpMsgParseError().
◆ httpMsgParseStep()
parses a 0-terminated buffer into Http::Message.
- Return values
-
1 success 0 need more data (partial parse) -1 parse error
Definition at line 151 of file Message.cc.
References assert, httpMsgIsolateStart(), Http::Message::psParsed, Http::Message::psReadyToParseHeaders, and Http::Message::psReadyToParseStartLine.
◆ httpRequestPack()
|
static |
◆ icapHistory()
Adaptation::Icap::History::Pointer HttpRequest::icapHistory | ( | ) | const |
Definition at line 388 of file HttpRequest.cc.
References debugs, icapHistory_, IcapLogfileStatus, LOG_ENABLE, and Log::TheConfig.
Referenced by Adaptation::AccessCheck::AccessCheck(), ClientHttpRequest::doCallouts(), Adaptation::Icap::ModXact::finalizeLogInfo(), inheritProperties(), ClientHttpRequest::noteAdaptationAclCheckDone(), prepareLogWithRequestDetails(), and Adaptation::Icap::ModXactLauncher::updateHistory().
◆ ignoreRange()
void HttpRequest::ignoreRange | ( | const char * | reason | ) |
Definition at line 620 of file HttpRequest.cc.
Referenced by clientInterpretRequestHeaders(), HttpStateData::httpBuildRequestHeader(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), and clientReplyContext::setReplyToStoreEntry().
◆ inheritProperties()
|
overrideprotectedvirtual |
Implements Http::Message.
Definition at line 213 of file HttpRequest.cc.
References adaptHistory(), adaptHistory_, auth_user_request, client_addr, clientConnectionManager, RequestFlags::cloneAdaptationImmune(), dnsWait, downloader, error, extacl_passwd, extacl_user, flags, forcedBodyContinuation, icapHistory(), icapHistory_, indirect_client_addr, my_addr, myportname, Http::Message::sources, and theNotes.
Referenced by clone().
◆ init()
|
protected |
Definition at line 76 of file HttpRequest.cc.
References adaptHistory_, auth_user_request, Http::Message::body_pipe, AnyP::Uri::clear(), Error::clear(), client_addr, dnsWait, error, extacl_log, extacl_message, extacl_passwd, extacl_user, flags, forcedBodyContinuation, icapHistory_, ims, imslen, indirect_client_addr, lastmod, method, Http::METHOD_NONE, my_addr, myportname, null_string, peer_domain, peer_login, Http::Message::psReadyToParseStartLine, Http::Message::pstate, range, rangeOffsetLimit, Ip::Address::setEmpty(), tag, url, and vary_headers.
Referenced by HttpRequest(), and reset().
◆ initHTTP()
void HttpRequest::initHTTP | ( | const HttpRequestMethod & | aMethod, |
AnyP::ProtocolType | aProtocol, | ||
const char * | schemeImage, | ||
const char * | aUrlpath | ||
) |
Definition at line 68 of file HttpRequest.cc.
References method, AnyP::Uri::path(), AnyP::Uri::setScheme(), and url.
Referenced by HttpRequest().
◆ manager()
void HttpRequest::manager | ( | const CbcPointer< ConnStateData > & | aMgr, |
const AccessLogEntryPointer & | al | ||
) |
Definition at line 773 of file HttpRequest.cc.
References SquidConfig::accessList, ACLFilledChecklist::al, Acl::Answer::allowed(), client_addr, Server::clientConnection, clientConnectionManager, COMM_INTERCEPTION, COMM_TRANSPARENT, Config, ACLChecklist::fastCheck(), flags, RequestFlags::ignoreCc, indirect_client_addr, RequestFlags::intercepted, RequestFlags::interceptTproxy, my_addr, myportname, port, ConnStateData::port, SquidConfig::spoof_client_ip, RequestFlags::spoofClientIp, and ACLFilledChecklist::syncAle().
Referenced by ConnStateData::buildFakeRequest(), and clientProcessRequest().
◆ maybeCacheable()
bool HttpRequest::maybeCacheable | ( | ) |
Whether response to this request is potentially cachable
- Return values
-
false Not cacheable. true Possibly cacheable. Response factors will determine.
Are responses to this request possible cacheable ? If false then no matter what the response must not be cached.
Definition at line 537 of file HttpRequest.cc.
References Http::Message::cache_control, flags, AnyP::Uri::getScheme(), HttpHdrCc::hasNoStore(), RequestFlags::hostVerified, RequestFlags::ignoreCc, RequestFlags::intercepted, RequestFlags::interceptTproxy, method, AnyP::PROTO_HTTP, AnyP::PROTO_HTTPS, HttpRequestMethod::respMaybeCacheable(), and url.
Referenced by clientInterpretRequestHeaders().
◆ MEMPROXY_CLASS()
|
private |
◆ multipartRangeRequest()
bool HttpRequest::multipartRangeRequest | ( | ) | const |
Definition at line 433 of file HttpRequest.cc.
References range, and HttpHdrRange::specs.
Referenced by clientReplyContext::buildReplyHeader(), HttpStateData::httpBuildRequestHeader(), and ClientHttpRequest::multipartRangeRequest().
◆ notes()
NotePairs::Pointer HttpRequest::notes | ( | ) |
- Returns
- existing non-empty transaction annotations, creates and returns empty annotations otherwise
Definition at line 751 of file HttpRequest.cc.
References theNotes.
Referenced by Http::One::Server::buildHttpRequest(), ClientHttpRequest::initRequest(), ClientHttpRequest::logRequest(), AccessLogEntry::syncNotes(), and UpdateRequestNotes().
◆ pack()
void HttpRequest::pack | ( | Packable * | p | ) | const |
Definition at line 343 of file HttpRequest.cc.
References Packable::append(), Packable::appendf(), assert, Http::Message::header, Http::Message::http_ver, HttpRequestMethod::image(), AnyP::ProtocolVersion::major, method, AnyP::ProtocolVersion::minor, HttpHeader::packInto(), AnyP::Uri::path(), SQUIDSBUFPH, SQUIDSBUFPRINT, and url.
Referenced by ErrorState::Dump(), Ftp::Server::handleRequest(), httpRequestPack(), and swapOut().
◆ packFirstLineInto()
|
overrideprotectedvirtual |
Implements Http::Message.
Definition at line 471 of file HttpRequest.cc.
References Packable::appendf(), effectiveRequestUri(), Http::Message::http_ver, HttpRequestMethod::image(), AnyP::ProtocolVersion::major, method, AnyP::ProtocolVersion::minor, AnyP::Uri::path(), SQUIDSBUFPH, SQUIDSBUFPRINT, and url.
◆ packInto()
|
inherited |
Definition at line 253 of file Message.cc.
References Packable::append().
◆ parse()
|
inherited |
Definition at line 68 of file Message.cc.
References assert, Config, DBG_IMPORTANT, debugs, error(), headersEnd(), int, SquidConfig::maxReplyHeaderSize, Http::scHeaderTooLarge, Http::scInvalidHeader, and Http::scNone.
Referenced by Adaptation::Icap::Xaction::parseHttpMsg(), and HttpReply::parseTerminatedPrefix().
◆ parseCharBuf()
|
inherited |
parseCharBuf() takes character buffer of HTTP headers (buf), which may not be NULL-terminated, and fills in an Http::Message structure. The parameter 'end' specifies the offset to the end of the reply headers. The caller may know where the end is, but is unable to NULL-terminate the buffer. This function returns true on success.
Definition at line 129 of file Message.cc.
References MemBuf::append(), MemBuf::buf, MemBuf::clean(), MemBuf::init(), MemBuf::size, and MemBuf::terminate().
◆ parseFirstLine()
|
overridevirtual |
Implements Http::Message.
Definition at line 293 of file HttpRequest.cc.
References DBG_IMPORTANT, debugs, findTrailingHTTPVersion(), Http::Message::http_ver, HttpRequestMethod::HttpRequestMethodXXX(), AnyP::ProtocolVersion::major, method, Http::METHOD_NONE, AnyP::ProtocolVersion::minor, AnyP::Uri::parse(), url, w_space, and xisspace.
◆ parseHeader() [1/3]
bool HttpRequest::parseHeader | ( | const char * | buffer, |
const size_t | size | ||
) |
Parses request header from the buffer. Use it in contexts where the Parser object not available.
Definition at line 717 of file HttpRequest.cc.
References Http::Message::header, HttpHeader::parse(), and size.
◆ parseHeader() [2/3]
|
protectedinherited |
Definition at line 201 of file Message.cc.
References SBuf::c_str(), Http::One::Parser::headerBlockSize(), Http::One::Parser::messageHeaderSize(), Http::One::Parser::mimeHeader(), Http::Message::psError, and Http::Message::psParsed.
◆ parseHeader() [3/3]
bool HttpRequest::parseHeader | ( | Http1::Parser & | hp | ) |
Parses request header using Parser. Use it in contexts where the Parser object is available.
Definition at line 710 of file HttpRequest.cc.
Referenced by Http::One::Server::buildHttpRequest(), and htcpSpecifier::checkHit().
◆ persistent()
|
inherited |
- Return values
-
true the message sender asks to keep the connection open. false the message sender will close the connection.
Factors other than the headers may result in connection closure.
Definition at line 236 of file Message.cc.
References httpHeaderHasConnDir(), and Http::ProtocolVersion().
Referenced by clientReplyContext::buildReplyHeader(), clientSetKeepaliveFlag(), HttpReply::hdrCacheInit(), and HttpStateData::sendRequest().
◆ pinnedConnection()
ConnStateData * HttpRequest::pinnedConnection | ( | ) |
Definition at line 724 of file HttpRequest.cc.
References clientConnectionManager, CbcPointer< Cbc >::get(), ConnStateData::pinned, and ConnStateData::pinning.
Referenced by ConnStateData::BorrowPinnedConnection(), clientReplyContext::buildReplyHeader(), clientCheckPinning(), FwdState::connectStart(), FwdState::reactToZeroSizeObject(), PeerSelector::selectPinned(), FwdState::usePinned(), and TunnelStateData::usePinned().
◆ prefixLen()
int HttpRequest::prefixLen | ( | ) | const |
Definition at line 368 of file HttpRequest.cc.
References Http::Message::header, HttpRequestMethod::image(), HttpHeader::len, SBuf::length(), method, AnyP::Uri::path(), and url.
Referenced by peerDigestFetchSetStats(), and clientReplyContext::traceReply().
◆ prepForDirect()
void HttpRequest::prepForDirect | ( | ) |
Definition at line 455 of file HttpRequest.cc.
References RequestFlags::auth_no_keytab, debugs, flags, peer_domain, and peer_login.
Referenced by TunnelStateData::connectDone(), FwdState::dispatch(), and switchToTunnel().
◆ prepForPeering()
void HttpRequest::prepForPeering | ( | const CachePeer & | peer | ) |
Definition at line 445 of file HttpRequest.cc.
References RequestFlags::auth_no_keytab, CachePeer::auth_no_keytab, debugs, CachePeer::domain, flags, CachePeer::login, CachePeer::options, peer_domain, and peer_login.
Referenced by TunnelStateData::connectDone(), FwdState::dispatch(), and switchToTunnel().
◆ putCc()
|
inherited |
copies Cache-Control header to this message, overwriting existing Cache-Control header(s), if any
Definition at line 33 of file Message.cc.
Referenced by HttpReply::make304(), and CacheManager::PutCommonResponseHeaders().
◆ recordLookup()
void HttpRequest::recordLookup | ( | const Dns::LookupDetails & | detail | ) |
Definition at line 579 of file HttpRequest.cc.
References debugs, dnsWait, and Dns::LookupDetails::wait.
Referenced by ClientRequestContext::hostHeaderIpVerify(), ACLDestinationIP::LookupDone(), LookupDone(), and PeerSelector::noteLookup().
◆ reset()
|
overridevirtual |
Implements Http::Message.
Definition at line 167 of file HttpRequest.cc.
◆ sanityCheckStartLine()
|
overrideprotectedvirtual |
Checks the first line of an HTTP request is valid currently just checks the request method is present.
NP: Other errors are left for detection later in the parse.
Implements Http::Message.
Definition at line 267 of file HttpRequest.cc.
References debugs, HttpRequestMethod::HttpRequestMethodXXX(), Http::METHOD_NONE, and Http::scInvalidHeader.
Referenced by PrivateHttpRequest::doSanityCheckStartLine().
◆ setContentLength()
|
inherited |
Definition at line 228 of file Message.cc.
References Http::CONTENT_LENGTH.
◆ storeId()
const SBuf HttpRequest::storeId | ( | ) |
Returns the current StoreID for the request as a nul-terminated char*. Always returns the current id for the request (either the effective request URI or modified ID by the helper).
Definition at line 732 of file HttpRequest.cc.
References debugs, effectiveRequestUri(), String::size(), store_id, and StringToSBuf().
Referenced by clientReplyContext::cacheHit(), ClientHttpRequest::doCallouts(), and storeKeyPublicByRequestMethod().
◆ swapOut()
void HttpRequest::swapOut | ( | StoreEntry * | e | ) |
Definition at line 333 of file HttpRequest.cc.
References assert, StoreEntry::buffer(), StoreEntry::flush(), and pack().
Referenced by clientReplyContext::traceReply().
Member Data Documentation
◆ adaptHistory_
|
mutableprivate |
Definition at line 119 of file HttpRequest.h.
Referenced by adaptHistory(), adaptHistoryImport(), clean(), inheritProperties(), and init().
◆ auth_user_request
Auth::UserRequest::Pointer HttpRequest::auth_user_request |
Definition at line 127 of file HttpRequest.h.
Referenced by Format::Format::assemble(), Auth::UserRequest::authenticate(), authTryGetUser(), ConnStateData::buildFakeRequest(), clientReplyContext::buildReplyHeader(), ClientHttpRequest::calloutsError(), clean(), ClientRequestContext::clientAccessCheckDone(), clientProcessRequest(), ErrorState::compileLegacyCode(), constructHelperQuery(), DelayId::DelayClient(), Auth::UserRequest::helperRequestKeyExtras(), ClientRequestContext::hostHeaderVerifyFailed(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), httpFixupAuthentication(), inheritProperties(), init(), Adaptation::Icap::ModXact::makeUsernameHeader(), peerUserHashSelectParent(), Log::Format::SquidIcap(), Log::Format::SquidNative(), ClientHttpRequest::sslBumpEstablish(), statClientRequests(), Auth::UserRequest::tryToAuthenticateAndSetAuthUser(), and Adaptation::Ecap::XactionRep::usernameValue().
◆ body_pipe
|
inherited |
Definition at line 97 of file Message.h.
Referenced by bodyNibbled(), FwdState::checkRetriable(), clean(), HttpReply::clean(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), clone(), HttpReply::clone(), Adaptation::Ecap::MessageRep::clone(), FwdState::doneWithRetries(), Adaptation::Icap::ModXact::estimateVirginBody(), Adaptation::Icap::ModXact::finalizeLogInfo(), ClientHttpRequest::handleAdaptationFailure(), Client::handleAdaptedHeader(), init(), Adaptation::Icap::ModXact::prepEchoing(), HttpStateData::sendRequest(), Adaptation::Icap::InOut::setHeader(), Adaptation::Message::ShortCircuit(), Client::startAdaptation(), Client::startRequestBodyFlow(), Adaptation::Ecap::XactionRep::useAdapted(), and Adaptation::Ecap::XactionRep::useVirgin().
◆ cache_control
|
inherited |
Definition at line 76 of file Message.h.
Referenced by clean(), clientInterpretRequestHeaders(), HttpStateData::haveParsedReplyHeaders(), HttpReply::hdrCacheClean(), HttpReply::hdrExpirationTime(), MimeIcon::load(), HttpReply::make304(), maybeCacheable(), ClientHttpRequest::onlyIfCached(), refreshCheck(), and HttpStateData::reusableReply().
◆ client_addr
Ip::Address HttpRequest::client_addr |
Definition at line 149 of file HttpRequest.h.
Referenced by Format::Format::assemble(), ClientRequestContext::clientAccessCheck(), Adaptation::Ecap::XactionRep::clientIpValue(), DelayId::DelayClient(), ClientHttpRequest::doCallouts(), ErrorState::ErrorState(), Adaptation::Icap::ModXact::finalizeLogInfo(), getOutgoingAddress(), getRangeOffsetLimit(), HttpStateData::handleMoreRequestBodyAvailable(), HttpStateData::httpBuildRequestHeader(), inheritProperties(), init(), Adaptation::Icap::ModXact::makeRequestHeaders(), manager(), PeerSelector::noteIp(), peerSourceHashSelectParent(), HttpStateData::processReplyBody(), ACLFilledChecklist::setRequest(), FwdState::Start(), and tunnelStart().
◆ clientConnectionManager
CbcPointer<ConnStateData> HttpRequest::clientConnectionManager |
The client connection manager, if known; Used for any response actions needed directly to the client. ie 1xx forwarding or connection pinning state changes
Definition at line 230 of file HttpRequest.h.
Referenced by Format::Format::assemble(), clientCheckPinning(), FwdState::completed(), copyResultsFromEntry(), FwdState::dispatch(), HttpStateData::handle1xx(), inheritProperties(), manager(), Ssl::PeekingPeerConnector::PeekingPeerConnector(), pinnedConnection(), HttpStateData::proceedAfter1xx(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), HttpStateData::processReplyBody(), PeerSelector::resolveSelected(), ACLFilledChecklist::setRequest(), FwdState::successfullyConnectedToPeer(), and switchToTunnel().
◆ content_length
|
inherited |
Definition at line 83 of file Message.h.
Referenced by HttpReply::bodySize(), Http::Stream::buildRangeHeader(), HttpHdrRange::canonize(), checkEntityFraming(), StoreEntry::checkTooBig(), StoreEntry::checkTooSmall(), clientPackRangeHdr(), clientProcessRequest(), HttpReply::expectingBody(), expectingBody(), Downloader::handleReply(), HttpReply::hdrCacheInit(), HttpStateData::persistentConnStatus(), HttpReply::redirect(), refreshIsCachable(), HttpStateData::sendRequest(), HttpReply::setHeaders(), storeLog(), clientReplyContext::storeNotOKTransferDone(), HttpStateData::truncateVirginBody(), and HttpReply::validatorsMatch().
◆ dnsWait
int HttpRequest::dnsWait |
Definition at line 159 of file HttpRequest.h.
Referenced by Format::Format::assemble(), inheritProperties(), init(), prepareLogWithRequestDetails(), and recordLookup().
◆ downloader
CbcPointer<Downloader> HttpRequest::downloader |
Definition at line 233 of file HttpRequest.h.
Referenced by inheritProperties().
◆ error
Error HttpRequest::error |
Definition at line 161 of file HttpRequest.h.
Referenced by ErrorState::BuildHttpReply(), clearError(), clone(), detailError(), AccessLogEntry::error(), inheritProperties(), init(), prepareLogWithRequestDetails(), ConnStateData::serveDelayedError(), ClientHttpRequest::updateCounters(), ClientHttpRequest::updateError(), AccessLogEntry::updateError(), Ftp::Server::writeErrorReply(), and ClientHttpRequest::~ClientHttpRequest().
◆ etag
String HttpRequest::etag |
Definition at line 189 of file HttpRequest.h.
Referenced by clean(), clone(), HttpStateData::httpBuildRequestHeader(), clientReplyContext::processExpired(), clientReplyContext::restoreState(), and clientReplyContext::saveState().
◆ extacl_log
String HttpRequest::extacl_log |
Definition at line 180 of file HttpRequest.h.
Referenced by Format::Format::assemble(), clean(), clone(), copyResultsFromEntry(), and init().
◆ extacl_message
String HttpRequest::extacl_message |
Definition at line 182 of file HttpRequest.h.
Referenced by clean(), clone(), ErrorState::compileLegacyCode(), copyResultsFromEntry(), and init().
◆ extacl_passwd
String HttpRequest::extacl_passwd |
Definition at line 178 of file HttpRequest.h.
Referenced by clean(), copyResultsFromEntry(), httpFixupAuthentication(), inheritProperties(), init(), and Adaptation::Icap::ModXact::makeRequestHeaders().
◆ extacl_user
String HttpRequest::extacl_user |
Definition at line 176 of file HttpRequest.h.
Referenced by Format::Format::assemble(), clean(), copyResultsFromEntry(), AccessLogEntry::getExtUser(), httpFixupAuthentication(), inheritProperties(), init(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Icap::ModXact::makeUsernameHeader(), ACLExtUser::match(), prepareLogWithRequestDetails(), statClientRequests(), and Adaptation::Ecap::XactionRep::usernameValue().
◆ flags
RequestFlags HttpRequest::flags |
Definition at line 141 of file HttpRequest.h.
Referenced by StoreEntry::adjustVary(), clientReplyContext::blockedHit(), clientReplyContext::buildReplyHeader(), HttpStateData::buildRequestPrefix(), clientReplyContext::cacheHit(), ClientHttpRequest::checkForInternalAccess(), ClientRequestContext::checkNoCacheDone(), CheckQuickAbortIsReasonable(), FwdState::checkRetry(), clientReplyContext::checkTransferDone(), ClientRequestContext::clientAccessCheck(), ClientRequestContext::clientAccessCheckDone(), clientCheckPinning(), TunnelStateData::clientExpectsConnectResponse(), clientFollowXForwardedForCheck(), clientHierarchical(), clientInterpretRequestHeaders(), clientProcessRequest(), clientProcessRequestFinished(), clientSetKeepaliveFlag(), FwdState::completed(), conditional(), HttpStateData::continueAfterParsingHeader(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), HttpStateData::decideIfWeDoRanges(), FwdState::dispatch(), ClientHttpRequest::doCallouts(), errorAppendEntry(), FindGoodListeningPortAddress(), getOutgoingAddress(), ClientHttpRequest::handleAdaptedHeader(), clientReplyContext::handleIMSReply(), Http::One::Server::handleReply(), StoreEntry::hasIfNoneMatchEtag(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), HttpStateData::httpBuildRequestHeader(), httpFixupAuthentication(), httpMaybeRemovePublic(), HttpStateData::HttpStateData(), clientReplyContext::identifyFoundObject(), clientReplyContext::identifyStoreObject(), inheritProperties(), init(), ConnStateData::initiateTunneledRequest(), internalStart(), ACLDestinationIP::LookupDone(), HappyConnOpener::makeError(), manager(), ACLDestinationIP::match(), ACLProxyAuth::matchProxyAuth(), maybeCacheable(), neighborsDigestSelect(), netdbExchangeStart(), ErrorState::NewForwarding(), FwdState::noteConnection(), PeerSelector::noteIp(), CacheManager::ParseHeaders(), peerAllowedToUse(), peerDigestRequest(), peerSelectIcpPing(), HttpStateData::peerSupportsConnectionPinning(), peerWouldBePinged(), ConnStateData::pinConnection(), FwdState::pinnedCanRetry(), prepForDirect(), prepForPeering(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), ClientHttpRequest::processRequest(), HttpStateData::processSurrogateControl(), ConnStateData::quitAfterError(), FwdState::reforward(), refreshCheck(), refreshCheckHTTP(), clientReplyContext::replyStatus(), ClientHttpRequest::resetRequestXXX(), PeerSelector::resolveSelected(), HttpStateData::reusableReply(), FwdState::secureConnectionToPeer(), FwdState::secureConnectionToPeerIfNeeded(), PeerSelector::selectMore(), clientReplyContext::sendNotModified(), HttpStateData::sendRequest(), clientReplyContext::sendStreamError(), Ssl::ServerBump::ServerBump(), clientReplyContext::setReplyToError(), ClientRequestContext::sslBumpAccessCheck(), ClientHttpRequest::sslBumpStart(), FwdState::Start(), FwdState::start(), HappyConnOpener::startConnecting(), FwdState::usePinned(), and TunnelStateData::usePinned().
◆ forcedBodyContinuation
bool HttpRequest::forcedBodyContinuation |
Definition at line 192 of file HttpRequest.h.
Referenced by HttpStateData::handle1xx(), Ftp::Server::handleUploadRequest(), inheritProperties(), init(), and Http::One::Server::processParsedRequest().
◆ hdr_sz
|
inherited |
Definition at line 81 of file Message.h.
Referenced by StoreEntry::append(), clone(), HttpReply::clone(), StoreEntry::contentLen(), store_client::doCopy(), MemObject::expectedReplySize(), Adaptation::Icap::ModXact::finalizeLogInfo(), store_client::finishCallback(), MemObject::markEndOfReplyHeaders(), store_client::nextHttpReadOffset(), Adaptation::Icap::Xaction::parseHttpMsg(), HttpReply::parseTerminatedPrefix(), clientReplyContext::processReplyAccessResult(), MemObject::readAheadPolicyCanRead(), store_client::sendingHttpHeaders(), store_client::skipHttpHeadersFromDisk(), clientReplyContext::storeOKTransferDone(), MemStore::updateHeadersOrThrow(), and StoreEntry::write().
◆ header
|
inherited |
Definition at line 74 of file Message.h.
Referenced by StoreEntry::adjustVary(), Ftp::Gateway::appendSuccessHeader(), Format::Format::assemble(), assembleVaryKey(), Auth::UserRequest::authenticate(), HttpStateData::blockSwitchingProtocols(), ConnStateData::buildFakeRequest(), ErrorState::BuildHttpReply(), Http::One::Server::buildHttpRequest(), Http::Stream::buildRangeHeader(), clientReplyContext::buildReplyHeader(), canHandle1xx(), checkEntityFraming(), clean(), HttpReply::clean(), ClientRequestContext::clientAccessCheck(), clientCheckPinning(), clientGetMoreData(), clientIfRangeMatch(), clientInterpretRequestHeaders(), clientPackRangeHdr(), clientProcessRequest(), clone(), HttpReply::clone(), Adaptation::Ecap::MessageRep::clone(), ErrorState::compileLegacyCode(), conditional(), Adaptation::Icap::Options::configure(), Ftp::Relay::createHttpReply(), Adaptation::Icap::ModXact::encapsulateHead(), Adaptation::Icap::ModXact::estimateVirginBody(), HttpReply::expectingBody(), expectingBody(), Ssl::ErrorDetailsManager::findDetail(), HttpStateData::forwardUpgrade(), Ftp::Gateway::ftpAuthRequired(), ftpSendStor(), Ftp::Server::handleFeatReply(), Ftp::Server::handleRequest(), StoreEntry::hasIfMatchEtag(), StoreEntry::hasIfNoneMatchEtag(), StoreEntry::hasOneOfEtags(), HttpStateData::haveParsedReplyHeaders(), HttpReply::hdrCacheInit(), hdrCacheInit(), HttpReply::hdrExpirationTime(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), HttpStateData::httpBuildRequestHeader(), Log::Format::HttpdCombined(), httpMakeVaryMark(), Ftp::HttpReplyWrapper(), Auth::SchemeConfig::isCP1251EncodingAllowed(), MimeIcon::load(), TemplateFile::loadFor(), Ftp::Gateway::loginFailed(), HttpReply::make304(), Adaptation::Icap::ModXact::makeRequestHeaders(), pack(), HttpReply::packHeadersUsingFastPacker(), parseHeader(), CacheManager::ParseHeaders(), Adaptation::Icap::OptXact::parseResponse(), peerDigestRequest(), HttpReply::prefixLen(), prefixLen(), prepareLogWithRequestDetails(), Adaptation::Icap::ModXact::prepEchoing(), Ftp::PrintReply(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), Http::One::Server::processParsedRequest(), purgeEntriesByHeader(), CacheManager::PutCommonResponseHeaders(), HttpReply::recreateOnNotModified(), HttpReply::redirect(), HttpReply::removeIrrelevantContentLength(), HttpStateData::reusableReply(), Ftp::Server::setDataCommand(), HttpReply::setHeaders(), UrnState::setUriResFromRequest(), Log::Format::SquidReferer(), Log::Format::SquidUserAgent(), Mgr::Inquirer::start(), CacheManager::start(), HttpStateData::statusIfComplete(), urlCheckRequest(), Adaptation::Ecap::XactionRep::useAdapted(), Adaptation::Ecap::XactionRep::useVirgin(), HttpReply::validatorsMatch(), varyEvaluateMatch(), Http::One::Server::writeControlMsgAndCall(), Ftp::Server::writeCustomReply(), Ftp::Server::writeErrorReply(), Ftp::Server::writeForwardedReply(), and Ftp::Server::writeForwardedReplyAndCall().
◆ hier
HierarchyLogEntry HttpRequest::hier |
Definition at line 157 of file HttpRequest.h.
Referenced by Client::adjustBodyBytesRead(), Format::Format::assemble(), TunnelStateData::checkRetry(), clone(), ErrorState::compileLegacyCode(), StoreEntry::complete(), ErrorState::Dump(), PeerSelector::handlePath(), neighborsDigestSelect(), peerNoteDigestLookup(), peerSelect(), prepareLogWithRequestDetails(), HttpStateData::processReplyHeader(), HttpStateData::readReply(), TunnelStateData::readServer(), PeerSelector::resolveSelected(), clientReplyContext::sendMoreData(), Client::sentRequestBody(), ConnStateData::serveDelayedError(), TunnelStateData::startConnecting(), switchToTunnel(), FwdState::syncHierNote(), TunnelStateData::syncHierNote(), PeeringActivityTimer::timer(), StoreEntry::timestampsSet(), ClientHttpRequest::updateCounters(), TunnelStateData::writeServerDone(), and HttpStateData::wroteLast().
◆ http_ver
|
inherited |
HTTP-Version field in the first line of the message. see RFC 7230 section 3.1
Definition at line 72 of file Message.h.
Referenced by Format::Format::assemble(), ErrorState::BuildHttpReply(), clientReplyContext::buildReplyHeader(), canHandle1xx(), checkEntityFraming(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), clientSetKeepaliveFlag(), clone(), HttpReply::clone(), ErrorState::compileLegacyCode(), Adaptation::Icap::ModXact::encapsulateHead(), HttpStateData::forwardUpgrade(), HttpReply::hdrCacheInit(), htcpClear(), htcpQuery(), HttpStateData::httpBuildRequestHeader(), netdbExchangeState::netdbExchangeState(), pack(), packFirstLineInto(), parseFirstLine(), prepareLogWithRequestDetails(), switchToTunnel(), and tunnelStart().
◆ icapHistory_
|
mutableprivate |
Definition at line 122 of file HttpRequest.h.
Referenced by clean(), icapHistory(), inheritProperties(), and init().
◆ ims
time_t HttpRequest::ims |
Definition at line 145 of file HttpRequest.h.
Referenced by clientInterpretRequestHeaders(), clone(), clientReplyContext::handleIMSReply(), init(), and clientReplyContext::processConditional().
◆ imslen
int HttpRequest::imslen |
Definition at line 147 of file HttpRequest.h.
Referenced by clientInterpretRequestHeaders(), clone(), clientReplyContext::handleIMSReply(), init(), and clientReplyContext::processConditional().
◆ indirect_client_addr
Ip::Address HttpRequest::indirect_client_addr |
Definition at line 152 of file HttpRequest.h.
Referenced by ClientRequestContext::clientAccessCheck(), clientFollowXForwardedForCheck(), Adaptation::Ecap::XactionRep::clientIpValue(), DelayId::DelayClient(), AccessLogEntry::getLogClientIp(), getOutgoingAddress(), inheritProperties(), init(), Adaptation::Icap::ModXact::makeRequestHeaders(), manager(), and ACLFilledChecklist::setRequest().
◆ lastmod
time_t HttpRequest::lastmod |
Definition at line 165 of file HttpRequest.h.
Referenced by clone(), HttpStateData::httpBuildRequestHeader(), init(), clientReplyContext::processExpired(), clientReplyContext::restoreState(), and clientReplyContext::saveState().
◆ masterXaction
MasterXaction::Pointer HttpRequest::masterXaction |
Definition at line 236 of file HttpRequest.h.
Referenced by Format::Format::assemble(), clone(), AccessLogEntry::codeContextGist(), htcpSpecifier::codeContextGist(), AccessLogEntry::detailCodeContext(), htcpSpecifier::detailCodeContext(), FindGoodListeningPortAddress(), Acl::TransactionInitiator::match(), UrnState::setUriResFromRequest(), and Adaptation::Ecap::XactionRep::updateSources().
◆ method
HttpRequestMethod HttpRequest::method |
Definition at line 114 of file HttpRequest.h.
Referenced by StoreEntry::adjustVary(), asnCacheStart(), Format::Format::assemble(), ConnStateData::buildFakeRequest(), ErrorState::BuildHttpReply(), clientReplyContext::buildReplyHeader(), HttpStateData::buildRequestPrefix(), clientReplyContext::cacheHit(), canonicalCleanUrl(), checkEntityFraming(), FwdState::checkRetriable(), ClientRequestContext::clientAccessCheckDone(), clientGetMoreData(), clientHierarchical(), clientInterpretRequestHeaders(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), clientSetKeepaliveFlag(), clone(), ErrorState::compileLegacyCode(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), FwdState::dispatch(), ClientHttpRequest::doCallouts(), clientReplyContext::doGetMoreData(), effectiveRequestUri(), Adaptation::Icap::ModXact::encapsulateHead(), HttpReply::expectedBodyTooLarge(), FromUrl(), FromUrlXXX(), ftpSendPassive(), Ftp::Gateway::Gateway(), ClientHttpRequest::gotEnough(), ClientHttpRequest::handleAdaptedHeader(), Ftp::Server::handleRequest(), StoreEntry::hasIfNoneMatchEtag(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), htcpClear(), htcpQuery(), httpStart(), init(), initHTTP(), HttpStateData::keepaliveAccounting(), maybeCacheable(), Client::maybePurgeOthers(), FwdState::noteConnection(), pack(), packFirstLineInto(), parseFirstLine(), CacheManager::ParseHeaders(), peerAllowedToUse(), peerDigestRequest(), peerSelect(), HttpStateData::persistentConnStatus(), prefixLen(), prepareLogWithRequestDetails(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), clientReplyContext::processReplyAccessResult(), ClientHttpRequest::processRequest(), clientReplyContext::purgeDoPurge(), purgeEntriesByHeader(), clientReplyContext::replyStatus(), FwdState::secureConnectionToPeerIfNeeded(), PeerSelector::selectMore(), PeerSelector::selectSomeParent(), clientReplyContext::sendNotModified(), clientReplyContext::sendNotModifiedOrPreconditionFailedError(), ConnStateData::serveDelayedError(), Ssl::ServerBump::ServerBump(), Ftp::Server::setReply(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), ClientRequestContext::sslBumpAccessCheck(), Client::startAdaptation(), clientReplyContext::startError(), HttpStateData::statusIfComplete(), storeGetPublicByRequest(), storeKeyPublicByRequest(), switchToTunnel(), TestHttpRequest::testCreateFromUrl(), TestHttpRequest::testIPv6HostColonBug(), clientReplyContext::traceReply(), HttpStateData::truncateVirginBody(), tunnelStart(), urlCanonicalFakeHttps(), urlCheckRequest(), urnHandleReply(), and HttpStateData::writeReplyBody().
◆ my_addr
Ip::Address HttpRequest::my_addr |
Definition at line 155 of file HttpRequest.h.
Referenced by ClientHttpRequest::doCallouts(), getRangeOffsetLimit(), inheritProperties(), init(), manager(), ACLFilledChecklist::setRequest(), and tunnelStart().
◆ myportname
String HttpRequest::myportname |
Definition at line 172 of file HttpRequest.h.
Referenced by clean(), inheritProperties(), init(), manager(), and ConnStateData::postHttpsAccept().
◆ peer_domain
char* HttpRequest::peer_domain |
Definition at line 170 of file HttpRequest.h.
Referenced by copyOneHeaderFromClientsideRequestToUpstreamRequest(), HttpStateData::httpBuildRequestHeader(), init(), prepForDirect(), and prepForPeering().
◆ peer_login
char* HttpRequest::peer_login |
Definition at line 163 of file HttpRequest.h.
Referenced by clientReplyContext::buildReplyHeader(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), httpFixupAuthentication(), init(), prepForDirect(), and prepForPeering().
◆ pstate
|
inherited |
Definition at line 94 of file Message.h.
Referenced by clone(), HttpReply::clone(), init(), HttpReply::init(), and HttpReply::parseTerminatedPrefix().
◆ range
HttpHdrRange* HttpRequest::range |
Definition at line 143 of file HttpRequest.h.
Referenced by Http::Stream::buildRangeHeader(), CheckQuickAbortIsReasonable(), clean(), clientInterpretRequestHeaders(), HttpStateData::decideIfWeDoRanges(), hdrCacheInit(), ignoreRange(), init(), ClientHttpRequest::mRangeCLen(), multipartRangeRequest(), ClientHttpRequest::prepPartialResponseGeneration(), and clientReplyContext::processReplyAccessResult().
◆ rangeOffsetLimit
|
mutableprivate |
Definition at line 261 of file HttpRequest.h.
Referenced by getRangeOffsetLimit(), and init().
◆ sources
|
inherited |
Definition at line 99 of file Message.h.
Referenced by Ftp::Gateway::appendSuccessHeader(), ConnStateData::buildFakeRequest(), clientProcessRequest(), Ftp::Relay::forwardReply(), Http::Tunneler::handleResponse(), HttpReply::inheritProperties(), inheritProperties(), Acl::ConnectionsEncrypted::match(), WhoisState::setReplyToOK(), Ftp::Relay::startDataDownload(), and Adaptation::Ecap::XactionRep::updateSources().
◆ store_id
String HttpRequest::store_id |
If defined, store_id_program mapped the request URL to this ID. Store uses this ID (and not the URL) to find and store entries, avoiding caching duplicate entries when different URLs point to "essentially the same" cachable resource.
Definition at line 139 of file HttpRequest.h.
Referenced by ClientRequestContext::clientStoreIdDone(), and storeId().
◆ tag
String HttpRequest::tag |
Definition at line 174 of file HttpRequest.h.
Referenced by Format::Format::assemble(), clean(), clone(), copyResultsFromEntry(), DelayId::DelayClient(), and init().
◆ theNotes
|
private |
annotations added by the note directive and helpers and(or) by annotate_transaction/annotate_client ACLs.
Definition at line 265 of file HttpRequest.h.
Referenced by clean(), hasNotes(), inheritProperties(), and notes().
◆ url
AnyP::Uri HttpRequest::url |
Definition at line 115 of file HttpRequest.h.
Referenced by asnCacheStart(), Format::Format::assemble(), ConnStateData::borrowPinnedConnection(), ConnStateData::buildFakeRequest(), Http::One::Server::buildHttpRequest(), HttpStateData::buildRequestPrefix(), clientReplyContext::cacheHit(), canonicalCleanUrl(), carpSelectParent(), HttpStateData::checkDateSkew(), ClientHttpRequest::checkForInternalAccess(), PeerSelector::checkNetdbDirect(), clean(), clientHierarchical(), clientInterpretRequestHeaders(), ClientRequestContext::clientRedirectDone(), clone(), ErrorState::compileLegacyCode(), TunnelStateData::connectDone(), FwdState::connectStart(), HttpStateData::continueAfterParsingHeader(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), FwdState::dispatch(), doV2Query(), effectiveRequestUri(), Adaptation::Icap::ModXact::encapsulateHead(), findUsableParentAtHostname(), FromUrl(), FromUrlXXX(), ftpFail(), ftpReadType(), ftpSendType(), ftpSendUser(), ftpTrySlashHack(), getDefaultParent(), getFirstUpParent(), TunnelStateData::getHost(), getRoundRobinParent(), getWeightedRoundRobinParent(), PeerSelector::handleHtcpParentMiss(), PeerSelector::handleIcpParentMiss(), ClientRequestContext::hostHeaderVerify(), htcpTstReply(), HttpStateData::httpBuildRequestHeader(), clientReplyContext::identifyFoundObject(), init(), initHTTP(), ConnStateData::initiateTunneledRequest(), internalStart(), ACLDestinationIP::match(), maybeCacheable(), neighborsHtcpReply(), neighborsUdpAck(), neighborsUdpPing(), netdbClosestParent(), netdbExchangeStart(), FwdState::noteConnection(), TunnelStateData::noteConnection(), PeerSelector::noteIp(), PeerSelector::noteIps(), operator<<(), pack(), packFirstLineInto(), parseFirstLine(), peerAllowedToUse(), peerDigestRequest(), PeerPoolMgr::PeerPoolMgr(), ConnStateData::pinConnection(), ConnStateData::postHttpsAccept(), prefixLen(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), purgeEntriesByHeader(), clientReplyContext::purgeRequest(), PeerSelector::resolveSelected(), FwdState::secureConnectionToPeerIfNeeded(), PeerSelector::selectAllParents(), PeerSelector::selectMore(), PeerSelector::selectSomeDirect(), PeerSelector::selectSomeNeighbor(), PeerSelector::selectSomeParent(), HttpStateData::sendRequest(), ConnStateData::serveDelayedError(), Ssl::ServerBump::ServerBump(), UrnState::setUriResFromRequest(), CacheManager::start(), FwdState::Start(), TunnelStateData::startConnecting(), ACLDestinationIP::StartLookup(), FwdState::successfullyConnectedToPeer(), TestHttpRequest::testCreateFromUrl(), TestHttpRequest::testIPv6HostColonBug(), Http::Tunneler::Tunneler(), urlCanonicalFakeHttps(), urlCheckRequest(), Ftp::UrlWith2f(), FwdState::usePinned(), TunnelStateData::usePinned(), Adaptation::Service::wants(), and whoisStart().
◆ vary_headers
SBuf HttpRequest::vary_headers |
Definition at line 168 of file HttpRequest.h.
Referenced by StoreEntry::adjustVary(), clean(), clone(), init(), clientReplyContext::processExpired(), clientReplyContext::purgeDoPurge(), storeKeyPublicByRequestMethod(), and varyEvaluateMatch().
◆ x_forwarded_for_iterator
String HttpRequest::x_forwarded_for_iterator |
Definition at line 185 of file HttpRequest.h.
Referenced by ClientRequestContext::clientAccessCheck(), and clientFollowXForwardedForCheck().
The documentation for this class was generated from the following files:
- src/HttpRequest.h
- src/HttpRequest.cc
- src/tests/stub_HttpRequest.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products