#include <HttpHdrCc.h>
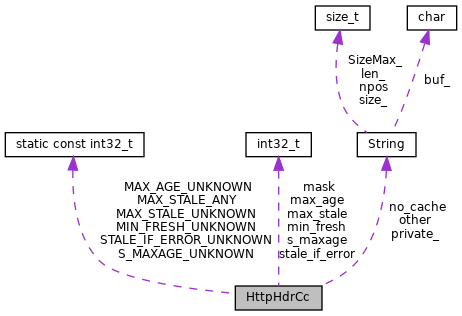
Public Member Functions | |
HttpHdrCc () | |
void | clear () |
reset data-members to default state More... | |
bool | parse (const String &s) |
parse a header-string and fill in appropriate values. More... | |
bool | hasPublic () const |
void | Public (bool v) |
void | clearPublic () |
bool | hasPrivate (const String **val=nullptr) const |
void | Private (const String &v) |
void | clearPrivate () |
bool | hasNoCacheWithParameters () const |
bool | hasNoCacheWithoutParameters () const |
bool | hasNoCache (const String **val=nullptr) const |
void | noCache (const String &v) |
void | clearNoCache () |
bool | hasNoStore () const |
void | noStore (bool v) |
void | clearNoStore () |
bool | hasNoTransform () const |
void | noTransform (bool v) |
void | clearNoTransform () |
bool | hasMustRevalidate () const |
void | mustRevalidate (bool v) |
void | clearMustRevalidate () |
bool | hasProxyRevalidate () const |
void | proxyRevalidate (bool v) |
void | clearProxyRevalidate () |
bool | hasMaxAge (int32_t *val=nullptr) const |
void | maxAge (int32_t v) |
void | clearMaxAge () |
bool | hasSMaxAge (int32_t *val=nullptr) const |
void | sMaxAge (int32_t v) |
void | clearSMaxAge () |
bool | hasMaxStale (int32_t *val=nullptr) const |
void | maxStale (int32_t v) |
void | clearMaxStale () |
bool | hasMinFresh (int32_t *val=nullptr) const |
void | minFresh (int32_t v) |
void | clearMinFresh () |
bool | hasOnlyIfCached () const |
void | onlyIfCached (bool v) |
void | clearOnlyIfCached () |
bool | hasStaleIfError (int32_t *val=nullptr) const |
void | staleIfError (int32_t v) |
void | clearStaleIfError () |
bool | hasImmutable () const |
void | Immutable (bool v) |
void | clearImmutable () |
bool | isSet (HttpHdrCcType id) const |
check whether the attribute value supplied by id is set More... | |
void | packInto (Packable *p) const |
Public Attributes | |
String | other |
Static Public Attributes | |
static const int32_t | MAX_AGE_UNKNOWN =-1 |
static const int32_t | S_MAXAGE_UNKNOWN =-1 |
static const int32_t | MAX_STALE_UNKNOWN =-1 |
static const int32_t | MAX_STALE_ANY =0x7fffffff |
static const int32_t | STALE_IF_ERROR_UNKNOWN =-1 |
static const int32_t | MIN_FRESH_UNKNOWN =-1 |
Private Member Functions | |
MEMPROXY_CLASS (HttpHdrCc) | |
template<class Value > | |
bool | hasDirective (const HttpHdrCcType hdrType, const Value &parsedVal, Value *outVal=nullptr) const |
implements typical has*() method logic More... | |
void | setMask (HttpHdrCcType id, bool newval=true) |
low-level part of the public set method, performs no checks More... | |
void | setValue (int32_t &value, int32_t new_value, HttpHdrCcType hdr, bool setting=true) |
Private Attributes | |
int32_t | mask |
int32_t | max_age |
int32_t | s_maxage |
int32_t | max_stale |
int32_t | stale_if_error |
int32_t | min_fresh |
String | private_ |
List of headers sent as value for CC:private="...". May be empty/undefined if the value is missing. More... | |
String | no_cache |
List of headers sent as value for CC:no-cache="...". May be empty/undefined if the value is missing. More... | |
Detailed Description
Http Cache-Control header representation
Store and parse the Cache-Control HTTP header.
Definition at line 43 of file HttpHdrCc.h.
Constructor & Destructor Documentation
◆ HttpHdrCc()
|
inline |
Definition at line 57 of file HttpHdrCc.h.
Referenced by clear().
Member Function Documentation
◆ clear()
void HttpHdrCc::clear | ( | ) |
Definition at line 92 of file HttpHdrCc.cc.
References HttpHdrCc().
◆ clearImmutable()
|
inline |
Definition at line 157 of file HttpHdrCc.h.
References CC_IMMUTABLE, and setMask().
◆ clearMaxAge()
|
inline |
Definition at line 124 of file HttpHdrCc.h.
References CC_MAX_AGE, max_age, MAX_AGE_UNKNOWN, and setValue().
Referenced by parse().
◆ clearMaxStale()
|
inline |
Definition at line 137 of file HttpHdrCc.h.
References CC_MAX_STALE, max_stale, MAX_STALE_UNKNOWN, and setValue().
◆ clearMinFresh()
|
inline |
Definition at line 142 of file HttpHdrCc.h.
References CC_MIN_FRESH, min_fresh, MIN_FRESH_UNKNOWN, and setValue().
Referenced by parse().
◆ clearMustRevalidate()
|
inline |
Definition at line 114 of file HttpHdrCc.h.
References CC_MUST_REVALIDATE, and setMask().
◆ clearNoCache()
|
inline |
Definition at line 99 of file HttpHdrCc.h.
References CC_NO_CACHE, String::clean(), no_cache, and setMask().
◆ clearNoStore()
|
inline |
Definition at line 104 of file HttpHdrCc.h.
References CC_NO_STORE, and setMask().
◆ clearNoTransform()
|
inline |
Definition at line 109 of file HttpHdrCc.h.
References CC_NO_TRANSFORM, and setMask().
◆ clearOnlyIfCached()
|
inline |
Definition at line 147 of file HttpHdrCc.h.
References CC_ONLY_IF_CACHED, and setMask().
◆ clearPrivate()
|
inline |
Definition at line 84 of file HttpHdrCc.h.
References CC_PRIVATE, String::clean(), private_, and setMask().
◆ clearProxyRevalidate()
|
inline |
Definition at line 119 of file HttpHdrCc.h.
References CC_PROXY_REVALIDATE, and setMask().
◆ clearPublic()
|
inline |
Definition at line 71 of file HttpHdrCc.h.
◆ clearSMaxAge()
|
inline |
Definition at line 129 of file HttpHdrCc.h.
References CC_S_MAXAGE, MAX_AGE_UNKNOWN, s_maxage, and setValue().
Referenced by parse().
◆ clearStaleIfError()
|
inline |
Definition at line 152 of file HttpHdrCc.h.
References CC_STALE_IF_ERROR, setValue(), stale_if_error, and STALE_IF_ERROR_UNKNOWN.
Referenced by parse().
◆ hasDirective()
|
inlineprivate |
Definition at line 184 of file HttpHdrCc.h.
References isSet().
Referenced by hasMaxAge(), hasMaxStale(), hasMinFresh(), hasNoCache(), hasPrivate(), hasSMaxAge(), and hasStaleIfError().
◆ hasImmutable()
|
inline |
Definition at line 155 of file HttpHdrCc.h.
References CC_IMMUTABLE, and isSet().
◆ hasMaxAge()
|
inline |
Definition at line 122 of file HttpHdrCc.h.
References CC_MAX_AGE, hasDirective(), and max_age.
Referenced by HttpReply::hdrExpirationTime(), HttpStateData::httpBuildRequestHeader(), and refreshCheck().
◆ hasMaxStale()
|
inline |
Definition at line 132 of file HttpHdrCc.h.
References CC_MAX_STALE, hasDirective(), and max_stale.
Referenced by refreshCheck().
◆ hasMinFresh()
|
inline |
Definition at line 140 of file HttpHdrCc.h.
References CC_MIN_FRESH, hasDirective(), and min_fresh.
Referenced by refreshCheck().
◆ hasMustRevalidate()
|
inline |
Definition at line 112 of file HttpHdrCc.h.
References CC_MUST_REVALIDATE, and isSet().
Referenced by HttpStateData::haveParsedReplyHeaders(), and HttpStateData::reusableReply().
◆ hasNoCache()
|
inline |
Definition at line 89 of file HttpHdrCc.h.
References CC_NO_CACHE, hasDirective(), and no_cache.
Referenced by clientInterpretRequestHeaders(), hasNoCacheWithoutParameters(), hasNoCacheWithParameters(), and HttpStateData::httpBuildRequestHeader().
◆ hasNoCacheWithoutParameters()
|
inline |
Definition at line 88 of file HttpHdrCc.h.
References hasNoCache(), no_cache, and String::size().
Referenced by HttpStateData::haveParsedReplyHeaders(), and HttpStateData::reusableReply().
◆ hasNoCacheWithParameters()
|
inline |
Definition at line 87 of file HttpHdrCc.h.
References hasNoCache(), no_cache, and String::size().
Referenced by HttpStateData::reusableReply().
◆ hasNoStore()
|
inline |
Definition at line 102 of file HttpHdrCc.h.
References CC_NO_STORE, and isSet().
Referenced by HttpRequest::maybeCacheable(), and HttpStateData::reusableReply().
◆ hasNoTransform()
|
inline |
Definition at line 107 of file HttpHdrCc.h.
References CC_NO_TRANSFORM, and isSet().
◆ hasOnlyIfCached()
|
inline |
Definition at line 145 of file HttpHdrCc.h.
References CC_ONLY_IF_CACHED, and isSet().
Referenced by ClientHttpRequest::onlyIfCached().
◆ hasPrivate()
|
inline |
Definition at line 74 of file HttpHdrCc.h.
References CC_PRIVATE, hasDirective(), and private_.
Referenced by HttpStateData::haveParsedReplyHeaders(), and HttpStateData::reusableReply().
◆ hasProxyRevalidate()
|
inline |
Definition at line 117 of file HttpHdrCc.h.
References CC_PROXY_REVALIDATE, and isSet().
Referenced by HttpStateData::haveParsedReplyHeaders().
◆ hasPublic()
|
inline |
Definition at line 69 of file HttpHdrCc.h.
References CC_PUBLIC, and isSet().
Referenced by HttpStateData::reusableReply().
◆ hasSMaxAge()
|
inline |
Definition at line 127 of file HttpHdrCc.h.
References CC_S_MAXAGE, hasDirective(), and s_maxage.
Referenced by HttpStateData::haveParsedReplyHeaders(), HttpReply::hdrExpirationTime(), and HttpStateData::reusableReply().
◆ hasStaleIfError()
|
inline |
Definition at line 150 of file HttpHdrCc.h.
References CC_STALE_IF_ERROR, hasDirective(), and stale_if_error.
Referenced by refreshCheck().
◆ Immutable()
|
inline |
Definition at line 156 of file HttpHdrCc.h.
References CC_IMMUTABLE, and setMask().
Referenced by parse().
◆ isSet()
|
inline |
Definition at line 160 of file HttpHdrCc.h.
References assert, CC_ENUM_END, EBIT_TEST, and mask.
Referenced by hasDirective(), hasImmutable(), hasMustRevalidate(), hasNoStore(), hasNoTransform(), hasOnlyIfCached(), hasProxyRevalidate(), hasPublic(), httpHdrCcUpdateStats(), packInto(), and parse().
◆ maxAge()
|
inline |
Definition at line 123 of file HttpHdrCc.h.
References CC_MAX_AGE, max_age, and setValue().
Referenced by HttpStateData::httpBuildRequestHeader(), and MimeIcon::load().
◆ maxStale()
|
inline |
Definition at line 136 of file HttpHdrCc.h.
References CC_MAX_STALE, max_stale, and setValue().
Referenced by parse().
◆ MEMPROXY_CLASS()
|
private |
◆ minFresh()
|
inline |
Definition at line 141 of file HttpHdrCc.h.
References CC_MIN_FRESH, min_fresh, and setValue().
◆ mustRevalidate()
|
inline |
Definition at line 113 of file HttpHdrCc.h.
References CC_MUST_REVALIDATE, and setMask().
Referenced by parse().
◆ noCache()
|
inline |
Definition at line 90 of file HttpHdrCc.h.
References String::append(), CC_NO_CACHE, no_cache, setMask(), and String::size().
Referenced by CacheManager::PutCommonResponseHeaders().
◆ noStore()
|
inline |
Definition at line 103 of file HttpHdrCc.h.
References CC_NO_STORE, and setMask().
Referenced by parse(), and CacheManager::PutCommonResponseHeaders().
◆ noTransform()
|
inline |
Definition at line 108 of file HttpHdrCc.h.
References CC_NO_TRANSFORM, and setMask().
Referenced by parse().
◆ onlyIfCached()
|
inline |
Definition at line 146 of file HttpHdrCc.h.
References CC_ONLY_IF_CACHED, and setMask().
Referenced by HttpStateData::httpBuildRequestHeader(), and parse().
◆ packInto()
void HttpHdrCc::packInto | ( | Packable * | p | ) | const |
Definition at line 268 of file HttpHdrCc.cc.
References Packable::appendf(), assert, CC_ENUM_END, CC_IMMUTABLE, CC_MAX_AGE, CC_MAX_STALE, CC_MIN_FRESH, CC_MUST_REVALIDATE, CC_NO_CACHE, CC_NO_STORE, CC_NO_TRANSFORM, CC_ONLY_IF_CACHED, CC_OTHER, CC_PRIVATE, CC_PROXY_REVALIDATE, CC_PUBLIC, CC_S_MAXAGE, CC_STALE_IF_ERROR, ccNameByType(), isSet(), mask, max_age, max_stale, MAX_STALE_ANY, min_fresh, no_cache, other, private_, s_maxage, String::size(), SQUIDSTRINGPH, SQUIDSTRINGPRINT, and stale_if_error.
Referenced by HttpHeader::putCc().
◆ parse()
bool HttpHdrCc::parse | ( | const String & | s | ) |
Definition at line 117 of file HttpHdrCc.cc.
References String::append(), CC_IMMUTABLE, CC_MAX_AGE, CC_MAX_STALE, CC_MIN_FRESH, CC_MUST_REVALIDATE, CC_NO_CACHE, CC_NO_STORE, CC_NO_TRANSFORM, CC_ONLY_IF_CACHED, CC_OTHER, CC_PRIVATE, CC_PROXY_REVALIDATE, CC_PUBLIC, CC_S_MAXAGE, CC_STALE_IF_ERROR, ccTypeByName(), String::clean(), clearMaxAge(), clearMinFresh(), clearSMaxAge(), clearStaleIfError(), debugs, httpHeaderParseInt(), httpHeaderParseQuotedString(), Immutable(), isSet(), mask, max_age, max_stale, MAX_STALE_ANY, maxStale(), min_fresh, mustRevalidate(), no_cache, noStore(), noTransform(), onlyIfCached(), other, private_, proxyRevalidate(), Public(), s_maxage, setMask(), String::size(), stale_if_error, and strListGetItem().
Referenced by HttpHeader::getCc().
◆ Private()
|
inline |
Definition at line 75 of file HttpHdrCc.h.
References String::append(), CC_PRIVATE, private_, setMask(), and String::size().
Referenced by Ftp::HttpReplyWrapper().
◆ proxyRevalidate()
|
inline |
Definition at line 118 of file HttpHdrCc.h.
References CC_PROXY_REVALIDATE, and setMask().
Referenced by parse().
◆ Public()
|
inline |
Definition at line 70 of file HttpHdrCc.h.
References CC_PUBLIC, and setMask().
Referenced by parse().
◆ setMask()
|
inlineprivate |
Definition at line 194 of file HttpHdrCc.h.
References EBIT_CLR, EBIT_SET, and mask.
Referenced by clearImmutable(), clearMustRevalidate(), clearNoCache(), clearNoStore(), clearNoTransform(), clearOnlyIfCached(), clearPrivate(), clearProxyRevalidate(), clearPublic(), Immutable(), mustRevalidate(), noCache(), noStore(), noTransform(), onlyIfCached(), parse(), Private(), proxyRevalidate(), Public(), and setValue().
◆ setValue()
|
private |
set a data member to a new value, and set the corresponding mask-bit. if setting is false, then the mask-bit is cleared.
Definition at line 100 of file HttpHdrCc.cc.
References debugs, and setMask().
Referenced by clearMaxAge(), clearMaxStale(), clearMinFresh(), clearSMaxAge(), clearStaleIfError(), maxAge(), maxStale(), minFresh(), sMaxAge(), and staleIfError().
◆ sMaxAge()
|
inline |
Definition at line 128 of file HttpHdrCc.h.
References CC_S_MAXAGE, s_maxage, and setValue().
◆ staleIfError()
|
inline |
Definition at line 151 of file HttpHdrCc.h.
References CC_STALE_IF_ERROR, setValue(), and stale_if_error.
Member Data Documentation
◆ mask
|
private |
bit-mask representing what header values are set among those recognized by squid.
managed via EBIT_SET/TEST/CLR
Definition at line 173 of file HttpHdrCc.h.
Referenced by isSet(), packInto(), parse(), and setMask().
◆ max_age
|
private |
Definition at line 174 of file HttpHdrCc.h.
Referenced by clearMaxAge(), hasMaxAge(), maxAge(), packInto(), and parse().
◆ MAX_AGE_UNKNOWN
|
static |
Definition at line 48 of file HttpHdrCc.h.
Referenced by clearMaxAge(), and clearSMaxAge().
◆ max_stale
|
private |
Definition at line 176 of file HttpHdrCc.h.
Referenced by clearMaxStale(), hasMaxStale(), maxStale(), packInto(), and parse().
◆ MAX_STALE_ANY
|
static |
used to mark a valueless Cache-Control: max-stale directive, which instructs us to treat responses of any age as fresh
Definition at line 53 of file HttpHdrCc.h.
Referenced by packInto(), parse(), and refreshCheck().
◆ MAX_STALE_UNKNOWN
|
static |
Definition at line 50 of file HttpHdrCc.h.
Referenced by clearMaxStale().
◆ min_fresh
|
private |
Definition at line 178 of file HttpHdrCc.h.
Referenced by clearMinFresh(), hasMinFresh(), minFresh(), packInto(), and parse().
◆ MIN_FRESH_UNKNOWN
|
static |
Definition at line 55 of file HttpHdrCc.h.
Referenced by clearMinFresh().
◆ no_cache
|
private |
Definition at line 180 of file HttpHdrCc.h.
Referenced by clearNoCache(), hasNoCache(), hasNoCacheWithoutParameters(), hasNoCacheWithParameters(), noCache(), packInto(), and parse().
◆ other
String HttpHdrCc::other |
comma-separated representation of the header values which were received but are not recognized.
Definition at line 207 of file HttpHdrCc.h.
Referenced by packInto(), and parse().
◆ private_
|
private |
Definition at line 179 of file HttpHdrCc.h.
Referenced by clearPrivate(), hasPrivate(), packInto(), parse(), and Private().
◆ s_maxage
|
private |
Definition at line 175 of file HttpHdrCc.h.
Referenced by clearSMaxAge(), hasSMaxAge(), packInto(), parse(), and sMaxAge().
◆ S_MAXAGE_UNKNOWN
|
static |
Definition at line 49 of file HttpHdrCc.h.
◆ stale_if_error
|
private |
Definition at line 177 of file HttpHdrCc.h.
Referenced by clearStaleIfError(), hasStaleIfError(), packInto(), parse(), and staleIfError().
◆ STALE_IF_ERROR_UNKNOWN
|
static |
Definition at line 54 of file HttpHdrCc.h.
Referenced by clearStaleIfError().
The documentation for this class was generated from the following files:
- src/HttpHdrCc.h
- src/HttpHdrCc.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products