#include <SquidString.h>
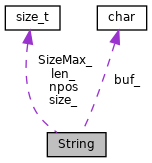
Public Types | |
typedef size_t | size_type |
Public Member Functions | |
String ()=default | |
String (char const *) | |
String (String const &) | |
String (String &&S) | |
~String () | |
String & | operator= (char const *) |
String & | operator= (String const &) |
String & | operator= (String &&S) |
bool | operator== (String const &) const |
bool | operator!= (String const &) const |
char | operator[] (unsigned int aPos) const |
size_type | size () const |
int | psize () const |
char const * | rawBuf () const |
char const * | termedBuf () const |
void | assign (const char *str, int len) |
void | clean () |
void | reset (char const *str) |
void | append (char const *buf, int len) |
void | append (char const *buf) |
void | append (char const) |
void | append (String const &) |
void | absorb (String &old) |
const char * | pos (char const *aString) const |
const char * | pos (char const ch) const |
size_type | find (char const ch) const |
size_type | find (char const *aString) const |
const char * | rpos (char const ch) const |
size_type | rfind (char const ch) const |
int | cmp (char const *) const |
int | cmp (char const *, size_type count) const |
int | cmp (String const &) const |
int | caseCmp (char const *) const |
int | caseCmp (char const *, size_type count) const |
int | caseCmp (String const &str) const |
bool | canGrowBy (const size_type growthLen) const |
whether appending growthLen characters is safe (i.e., unlikely to assert) More... | |
String | substr (size_type from, size_type to) const |
void | cut (size_type newLength) |
Static Public Member Functions | |
static size_type | SizeMaxXXX () |
static bool | CanGrowTo (size_type totalLen, const size_type extras=0) |
Static Public Attributes | |
static const size_type | npos = static_cast<size_type>(-1) |
Private Member Functions | |
void | allocAndFill (const char *str, int len) |
void | allocBuffer (size_type sz) |
void | setBuffer (char *buf, size_type sz) |
bool | defined () const |
bool | undefined () const |
void | set (char const *loc, char const ch) |
void | cutPointer (char const *loc) |
Static Private Member Functions | |
static bool | SafeAdd (size_type &base, size_type extra) |
returns true after increasing the first argument by extra if the sum does not exceed SizeMax_ More... | |
Private Attributes | |
size_type | size_ = 0 |
size_type | len_ = 0 |
char * | buf_ = nullptr |
Static Private Attributes | |
static const size_type | SizeMax_ = 65535 |
Detailed Description
Definition at line 25 of file SquidString.h.
Member Typedef Documentation
◆ size_type
typedef size_t String::size_type |
Definition at line 38 of file SquidString.h.
Constructor & Destructor Documentation
◆ String() [1/4]
|
default |
◆ String() [2/4]
String::String | ( | char const * | aString | ) |
Definition at line 36 of file String.cc.
References allocAndFill().
◆ String() [3/4]
String::String | ( | String const & | old | ) |
Definition at line 96 of file String.cc.
References allocAndFill(), rawBuf(), and size().
◆ String() [4/4]
|
inline |
Definition at line 32 of file SquidString.h.
◆ ~String()
Member Function Documentation
◆ absorb()
void String::absorb | ( | String & | old | ) |
◆ allocAndFill()
|
private |
Definition at line 87 of file String.cc.
References allocBuffer(), assert, buf_, and len_.
Referenced by String(), assign(), operator=(), and reset().
◆ allocBuffer()
|
private |
Definition at line 18 of file String.cc.
References assert, memAllocString(), setBuffer(), and undefined().
Referenced by allocAndFill(), and append().
◆ append() [1/4]
void String::append | ( | char const * | buf | ) |
◆ append() [2/4]
void String::append | ( | char const * | buf, |
int | len | ||
) |
Definition at line 130 of file String.cc.
References absorb(), allocBuffer(), assert, buf_, canGrowBy(), len_, rawBuf(), size_, and xstrncpy().
Referenced by Adaptation::DynamicGroupCfg::add(), append(), CapturingStoreEntry::append(), Adaptation::Icap::ServiceRep::checkOptions(), Ipc::Port::CoordinatorAddr(), Adaptation::ServiceGroup::finalize(), Rock::SwapDir::freeSlotsPath(), ftpListDir(), ftpReadCwd(), StoreEntry::hasOneOfEtags(), httpHeaderParseQuotedString(), Ipc::Port::MakeAddr(), MetadataId(), HttpHdrCc::noCache(), HttpHdrCc::parse(), Rock::SwapDir::parse(), HttpHdrCc::Private(), QueuesId(), ConfigParser::QuoteString(), ClientHttpRequest::rangeBoundaryStr(), ReadersId(), and strListAdd().
◆ append() [3/4]
void String::append | ( | char const | chr | ) |
◆ append() [4/4]
void String::append | ( | String const & | old | ) |
◆ assign()
void String::assign | ( | const char * | str, |
int | len | ||
) |
Definition at line 78 of file String.cc.
References allocAndFill(), and clean().
Referenced by ESIVarState::addVariable(), ESISegment::dumpOne(), Ipc::TypedMsgHdr::getString(), HttpReply::hdrCacheInit(), httpHeaderParseQuotedString(), HttpHeaderEntry::parse(), SBufToString(), Adaptation::DynamicServiceChain::Split(), and substr().
◆ canGrowBy()
|
inline |
Definition at line 124 of file SquidString.h.
References CanGrowTo(), and size().
Referenced by append(), HttpStateData::httpBuildRequestHeader(), and strListAdd().
◆ CanGrowTo()
Whether creating a totalLen-character string is safe (i.e., unlikely to assert). Optional extras can be used for overflow-safe length addition. Implementation has to add 1 because many String allocation methods do.
Definition at line 122 of file SquidString.h.
References SafeAdd().
Referenced by canGrowBy().
◆ caseCmp() [1/3]
int String::caseCmp | ( | char const * | aString | ) | const |
Definition at line 266 of file String.cc.
References nilCmp(), size(), and termedBuf().
Referenced by caseCmp(), Adaptation::Config::dumpService(), Mgr::QueryParams::find(), Ftp::Server::handleEpsvRequest(), HttpHeader::parse(), Http::StatusLine::parse(), HttpHdrRange::parseInit(), and Http::One::Server::processParsedRequest().
◆ caseCmp() [2/3]
int String::caseCmp | ( | char const * | aString, |
String::size_type | count | ||
) | const |
Definition at line 276 of file String.cc.
References nilCmp(), size(), and termedBuf().
◆ caseCmp() [3/3]
Definition at line 115 of file SquidString.h.
◆ clean()
void String::clean | ( | ) |
Definition at line 103 of file String.cc.
References buf_, defined(), len_, memFreeString(), and size_.
Referenced by ~String(), absorb(), assembleVaryKey(), assign(), HttpRequest::clean(), HttpHdrScTarget::clearContent(), HttpHdrCc::clearNoCache(), HttpHdrCc::clearPrivate(), clientFollowXForwardedForCheck(), clientInterpretRequestHeaders(), ClientHttpRequest::freeResources(), Ipc::TypedMsgHdr::getString(), HttpReply::hdrCacheClean(), HttpStateData::httpBuildRequestHeader(), httpHeaderParseQuotedString(), httpMakeVaryMark(), operator=(), HttpHdrCc::parse(), ConfigParser::QuoteString(), reset(), clientReplyContext::restoreState(), and MemObject::setUris().
◆ cmp() [1/3]
int String::cmp | ( | char const * | aString | ) | const |
Definition at line 236 of file String.cc.
References nilCmp(), size(), and termedBuf().
Referenced by TestConfigParser::doParseQuotedTest(), Ftp::Server::handleEpsvRequest(), operator!=(), operator<(), operator==(), Http::StatusLine::parse(), HttpReply::sanityCheckStartLine(), TestString::testCmpDefault(), TestString::testCmpEmptyString(), and TestString::testCmpNotEmptyDefault().
◆ cmp() [2/3]
int String::cmp | ( | char const * | aString, |
String::size_type | count | ||
) | const |
Definition at line 246 of file String.cc.
References nilCmp(), size(), and termedBuf().
◆ cmp() [3/3]
Definition at line 256 of file String.cc.
References nilCmp(), size(), and termedBuf().
◆ cut()
void String::cut | ( | String::size_type | newLength | ) |
Definition at line 203 of file String.cc.
Referenced by clientFollowXForwardedForCheck(), and Adaptation::ServiceGroup::finalize().
◆ cutPointer()
|
inlineprivate |
Definition at line 155 of file SquidString.h.
◆ defined()
|
inlineprivate |
Definition at line 135 of file SquidString.h.
References buf_.
Referenced by clean(), and undefined().
◆ find() [1/2]
String::size_type String::find | ( | char const * | aString | ) | const |
◆ find() [2/2]
String::size_type String::find | ( | char const | ch | ) | const |
offset from string start of the first occurrence of ch returns String::npos if ch is not found
Definition at line 429 of file String.cc.
References npos, pos(), and rawBuf().
Referenced by strListIsSubstr().
◆ operator!=()
bool String::operator!= | ( | String const & | that | ) | const |
◆ operator=() [1/3]
String & String::operator= | ( | char const * | aString | ) |
◆ operator=() [2/3]
◆ operator=() [3/3]
◆ operator==()
bool String::operator== | ( | String const & | that | ) | const |
◆ operator[]()
|
inline |
Retrieve a single character in the string.
- Parameters
-
aPos Position of character to retrieve.
Definition at line 63 of file SquidString.h.
◆ pos() [1/2]
const char * String::pos | ( | char const * | aString | ) | const |
Definition at line 405 of file String.cc.
References termedBuf(), and undefined().
Referenced by Http::ContentLengthInterpreter::checkField(), esiEnableProcessing(), and find().
◆ pos() [2/2]
const char * String::pos | ( | char const | ch | ) | const |
Definition at line 413 of file String.cc.
References termedBuf(), and undefined().
◆ psize()
|
inline |
variant of size() suited to be used for printf-alikes. throws when size() >= INT_MAX
Definition at line 77 of file SquidString.h.
References INT_MAX, Must, and size().
Referenced by Ipc::TypedMsgHdr::putString(), and HttpReply::sanityCheckStartLine().
◆ rawBuf()
|
inline |
Returns a raw pointer to the underlying backing store. The caller has been verified not to make any assumptions about null-termination
Definition at line 86 of file SquidString.h.
References buf_.
Referenced by String(), append(), clientReplyContext::buildReplyHeader(), caseCmp(), Http::ContentLengthInterpreter::checkField(), Http::ContentLengthInterpreter::checkList(), clientCheckPinning(), ESIVariableExpression::eval(), ESIVariableLanguage::eval(), ESIAssign::evaluateVariable(), find(), httpFixupAuthentication(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Ecap::XactionRep::masterxSharedValue(), operator<<(), operator=(), HttpHeaderEntry::packInto(), ESIAssign::process(), Ipc::TypedMsgHdr::putString(), ESIVarState::removeVariable(), rfind(), StringToSBuf(), substr(), and Adaptation::Ecap::XactionRep::usernameValue().
◆ reset()
void String::reset | ( | char const * | str | ) |
Definition at line 122 of file String.cc.
References allocAndFill(), and clean().
Referenced by ftpReadCwd(), and operator=().
◆ rfind()
String::size_type String::rfind | ( | char const | ch | ) | const |
◆ rpos()
const char * String::rpos | ( | char const | ch | ) | const |
Definition at line 421 of file String.cc.
References termedBuf(), and undefined().
Referenced by rfind().
◆ SafeAdd()
◆ set()
|
inlineprivate |
Definition at line 149 of file SquidString.h.
◆ setBuffer()
|
private |
Definition at line 28 of file String.cc.
References assert, buf_, size_, SizeMax_, and undefined().
Referenced by absorb(), and allocBuffer().
◆ size()
|
inline |
Definition at line 73 of file SquidString.h.
References len_.
Referenced by String(), ESIAssign::addElement(), Format::Format::assemble(), ESIVarState::buildVary(), canGrowBy(), caseCmp(), Adaptation::Icap::Options::cfgIntHeader(), Http::ContentLengthInterpreter::checkField(), Http::ContentLengthInterpreter::checkList(), CacheManager::CheckPassword(), clientFollowXForwardedForCheck(), cmp(), Adaptation::Icap::Options::configure(), Ipc::Port::CoordinatorAddr(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), copyResultsFromEntry(), HttpHeader::delAt(), ESIVariableExpression::eval(), ESIVariableLanguage::eval(), ESIAssign::evaluateVariable(), Adaptation::Icap::ModXact::expectIcapTrailers(), Adaptation::ServiceGroup::finalize(), Adaptation::Icap::ModXact::finalizeLogInfo(), Mgr::QueryParams::find(), HttpStateData::forwardUpgrade(), Mgr::QueryParams::get(), AccessLogEntry::getExtUser(), HttpHeader::getList(), Ftp::Server::handleEprtRequest(), Ftp::Server::handleEpsvRequest(), Ftp::Server::handlePasvRequest(), Ftp::Server::handlePortRequest(), HttpHdrCc::hasNoCacheWithoutParameters(), HttpHdrCc::hasNoCacheWithParameters(), HttpHdrScTarget::hasTarget(), MemObject::hasUris(), HttpStateData::httpBuildRequestHeader(), httpFixupAuthentication(), HttpHeaderEntry::length(), MemObject::logUri(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Icap::ModXact::makeUsernameHeader(), Adaptation::Ecap::XactionRep::masterxSharedValue(), ACLExtUser::match(), HttpHdrCc::noCache(), operator<<(), operator=(), HttpHdrCc::packInto(), HttpHeaderEntry::packInto(), Adaptation::ServiceConfig::parse(), Ssl::ErrorDetailFile::parse(), Http::StatusLine::parse(), HttpHdrCc::parse(), Adaptation::Icap::ModXact::parseIcapHead(), HttpHdrCc::Private(), ESIAssign::process(), psize(), Ipc::Coordinator::registerStrand(), ESIVarState::removeVariable(), HttpReply::sanityCheckStartLine(), Ftp::Relay::sendCommand(), SlowlyParseQuotedField(), statClientRequests(), HttpRequest::storeId(), clientReplyContext::storeId(), MemObject::storeId(), storeLog(), strHdrAcptLangGetItem(), StringToSBuf(), strListAdd(), substr(), Mgr::QueryParams::unpack(), Adaptation::Ecap::XactionRep::usernameValue(), Adaptation::Ecap::HeaderRep::value(), Http::One::Server::writeControlMsgAndCall(), and Ftp::Server::writeErrorReply().
◆ SizeMaxXXX()
|
inlinestatic |
The absolute size limit on data held in a String. Since Strings can be nil-terminated implicitly it is best to ensure the useful content length is strictly less than this limit.
Definition at line 71 of file SquidString.h.
References SizeMax_.
Referenced by HttpHeader::updateOrAddStr().
◆ substr()
String String::substr | ( | String::size_type | from, |
String::size_type | to | ||
) | const |
Definition at line 190 of file String.cc.
References assign(), Must, rawBuf(), and size().
Referenced by strHdrAcptLangGetItem(), and TestString::testSubstr().
◆ termedBuf()
|
inline |
Returns a raw pointer to the underlying backing store. The caller requires it to be null-terminated.
Definition at line 92 of file SquidString.h.
References buf_.
Referenced by Mgr::ActionParams::ActionParams(), Mgr::Response::Response(), aclMatchExternal(), addedEntry(), Format::Format::assemble(), assembleVaryKey(), clientReplyContext::buildReplyHeader(), Ssl::ErrorDetailsManager::cacheDetails(), caseCmp(), Adaptation::Icap::Options::cfgIntHeader(), clientFollowXForwardedForCheck(), HttpHeaderEntry::clone(), IpcIoFile::close(), cmp(), ErrorState::compileLegacyCode(), CacheManager::createRequestedAction(), Mgr::Action::createStoreEntry(), Adaptation::Icap::Xaction::dnsLookupDone(), TestConfigParser::doParseQuotedTest(), Adaptation::Icap::Xaction::finalizeLogInfo(), Adaptation::Icap::ModXact::finalizeLogInfo(), Adaptation::Ecap::FindAdapterService(), HttpStateData::forwardUpgrade(), Rock::SwapDir::freeSlotsPath(), HttpHeader::getContRange(), HttpHeader::getETag(), AccessLogEntry::getExtUser(), HttpHeaderEntry::getInt(), HttpHeaderEntry::getInt64(), HttpHeader::getLastStr(), HttpHeader::getList(), HttpHeader::getStr(), HttpHeader::getTime(), HttpHeader::getTimeOrTag(), ClientHttpRequest::handleAdaptationBlock(), Client::handleAdaptationBlocked(), Ftp::Server::handleEprtRequest(), Ftp::Server::handlePortRequest(), Ftp::Server::handleRequest(), HttpHeader::hasNamed(), StoreEntry::hasOneOfEtags(), HttpStateData::httpBuildRequestHeader(), httpFixupAuthentication(), httpHeaderParseQuotedString(), TemplateFile::language(), TemplateFile::loadDefault(), MemObject::logUri(), Adaptation::Icap::ModXact::makeUsernameHeader(), ACLExtUser::match(), IpcIoFile::open(), Adaptation::Icap::Xaction::openConnection(), LoadableModule::openModule(), Adaptation::ServiceConfig::parse(), Ssl::ErrorDetailFile::parse(), HttpHeaderEntry::parse(), Rock::SwapDir::parse(), parse_HeaderWithAclList(), HttpHdrRange::parseInit(), pos(), prepareLogWithRequestDetails(), Ftp::PrintReply(), ConfigParser::QuoteString(), rpos(), Ftp::Relay::sendCommand(), ErrorPageFile::setDefault(), SlowlyParseQuotedField(), Log::Format::SquidIcap(), statClientRequests(), clientReplyContext::storeId(), MemObject::storeId(), storeLog(), strListGetItem(), TemplateFile::tryLoadTemplate(), Adaptation::Ecap::UnregisterAdapterService(), Adaptation::Ecap::HeaderRep::value(), Http::One::Server::writeControlMsgAndCall(), and Ftp::Server::writeErrorReply().
◆ undefined()
|
inlineprivate |
Definition at line 136 of file SquidString.h.
References defined().
Referenced by allocBuffer(), pos(), rpos(), and setBuffer().
Member Data Documentation
◆ buf_
|
private |
Definition at line 147 of file SquidString.h.
Referenced by absorb(), allocAndFill(), append(), clean(), cut(), cutPointer(), defined(), operator=(), operator[](), rawBuf(), set(), setBuffer(), and termedBuf().
◆ len_
|
private |
Definition at line 141 of file SquidString.h.
Referenced by absorb(), allocAndFill(), append(), clean(), cut(), cutPointer(), operator=(), and size().
◆ npos
Definition at line 39 of file SquidString.h.
Referenced by find(), Adaptation::ServiceConfig::grokUri(), rfind(), and strListIsSubstr().
◆ size_
|
private |
Definition at line 139 of file SquidString.h.
Referenced by absorb(), append(), clean(), cutPointer(), operator=(), operator[](), set(), and setBuffer().
◆ SizeMax_
|
staticprivate |
64K limit protects some fixed-size buffers
Definition at line 143 of file SquidString.h.
Referenced by SafeAdd(), setBuffer(), and SizeMaxXXX().
The documentation for this class was generated from the following files:
- src/SquidString.h
- src/String.cc