#include <HttpReply.h>
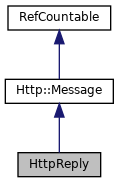
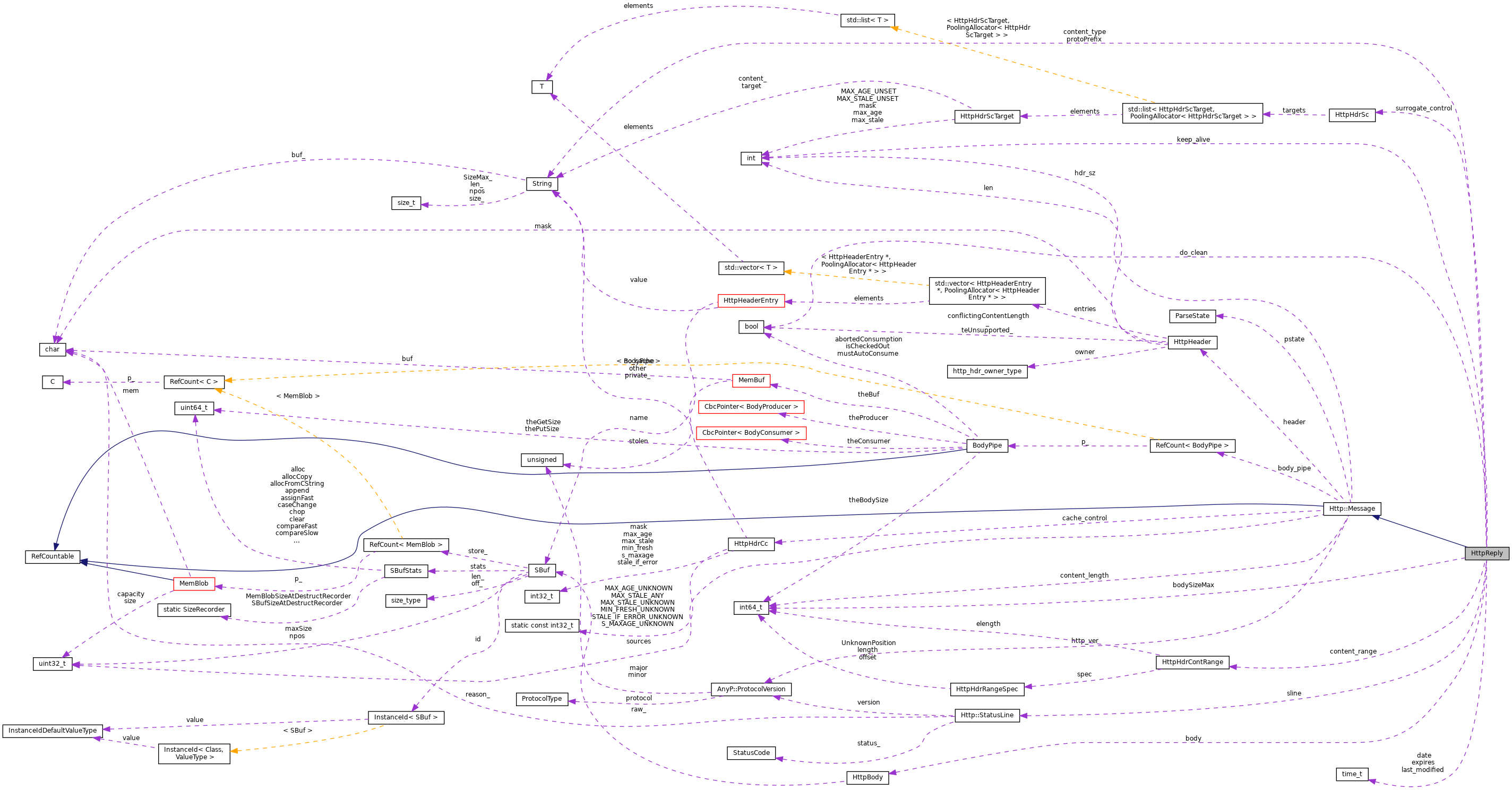
Public Types | |
typedef RefCount< HttpReply > | Pointer |
enum | Sources { srcUnknown = 0, srcHttps = 1 << 0, srcFtps = 1 << 1, srcIcaps = 1 << 2, srcEcaps = 1 << 3, srcHttp = 1 << (16 + 0), srcFtp = 1 << (16 + 1), srcIcap = 1 << (16 + 2), srcEcap = 1 << (16 + 3), srcWhois = 1 << (16 + 15), srcUnsafe = 0xFFFF0000, srcSafe = 0x0000FFFF } |
Who may have created or modified this message? More... | |
enum | ParseState { psReadyToParseStartLine = 0, psReadyToParseHeaders, psParsed, psError } |
parse state of HttpReply or HttpRequest More... | |
Public Member Functions | |
HttpReply () | |
~HttpReply () override | |
void | reset () override |
bool | sanityCheckStartLine (const char *buf, const size_t hdr_len, Http::StatusCode *error) override |
const HttpHdrContRange * | contentRange () const |
int | httpMsgParseError () override |
bool | expectingBody (const HttpRequestMethod &, int64_t &) const override |
bool | inheritProperties (const Http::Message *) override |
Pointer | recreateOnNotModified (const HttpReply &reply304) const |
void | setHeaders (Http::StatusCode status, const char *reason, const char *ctype, int64_t clen, time_t lmt, time_t expires) |
MemBuf * | pack () const |
HttpReplyPointer | make304 () const |
void | redirect (Http::StatusCode, const char *) |
int64_t | bodySize (const HttpRequestMethod &) const |
bool | receivedBodyTooLarge (HttpRequest &, int64_t receivedBodySize) |
bool | expectedBodyTooLarge (HttpRequest &request) |
int | validatorsMatch (HttpReply const *other) const |
void | packHeadersUsingFastPacker (Packable &p) const |
void | packHeadersUsingSlowPacker (Packable &p) const |
same as packHeadersUsingFastPacker() but assumes that p cannot quickly process small additions More... | |
HttpReply * | clone () const override |
void | hdrCacheInit () override |
bool | olderThan (const HttpReply *them) const |
void | removeIrrelevantContentLength () |
Some response status codes prohibit sending Content-Length (RFC 7230 section 3.3.2). More... | |
void | configureContentLengthInterpreter (Http::ContentLengthInterpreter &) override |
configures the interpreter as needed More... | |
bool | parseHeader (Http1::Parser &hp) |
parses reply header using Parser More... | |
size_t | parseTerminatedPrefix (const char *, size_t) |
size_t | prefixLen () const |
void | packInto (Packable *, bool full_uri) const |
produce a message copy, except for a few connection-specific settings More... | |
void | setContentLength (int64_t) |
[re]sets Content-Length header and cached value More... | |
bool | persistent () const |
void | putCc (const HttpHdrCc &) |
bool | parse (const char *buf, const size_t sz, bool eol, Http::StatusCode *error) |
bool | parseCharBuf (const char *buf, ssize_t end) |
int | httpMsgParseStep (const char *buf, int len, int atEnd) |
void | firstLineBuf (MemBuf &) |
useful for debugging More... | |
Static Public Member Functions | |
static HttpReplyPointer | MakeConnectionEstablished () |
construct and return an HTTP/200 (Connection Established) response More... | |
Public Attributes | |
time_t | date |
time_t | last_modified |
time_t | expires |
String | content_type |
HttpHdrSc * | surrogate_control |
short int | keep_alive |
Http::StatusLine | sline |
HttpBody | body |
String | protoPrefix |
bool | do_clean |
AnyP::ProtocolVersion | http_ver |
HttpHeader | header |
HttpHdrCc * | cache_control = nullptr |
int | hdr_sz = 0 |
int64_t | content_length = 0 |
ParseState | pstate = Http::Message::psReadyToParseStartLine |
the current parsing state More... | |
BodyPipe::Pointer | body_pipe |
optional pipeline to receive message body More... | |
uint32_t | sources = 0 |
The message sources. More... | |
Protected Member Functions | |
void | packFirstLineInto (Packable *p, bool) const override |
bool | parseFirstLine (const char *start, const char *end) override |
bool | parseHeader (Http1::Parser &, Http::ContentLengthInterpreter &) |
Private Member Functions | |
MEMPROXY_CLASS (HttpReply) | |
void | init () |
void | clean () |
void | hdrCacheClean () |
void | packInto (MemBuf &) const |
MemBuf * | packed304Reply () const |
time_t | hdrExpirationTime () |
void | calcMaxBodySize (HttpRequest &request) const |
Private Attributes | |
int64_t | bodySizeMax |
HttpHdrContRange * | content_range |
parsed Content-Range; nil for non-206 responses! More... | |
Detailed Description
Definition at line 24 of file HttpReply.h.
Member Typedef Documentation
◆ Pointer
typedef RefCount<HttpReply> HttpReply::Pointer |
Definition at line 29 of file HttpReply.h.
Member Enumeration Documentation
◆ ParseState
|
inherited |
◆ Sources
|
inherited |
Constructor & Destructor Documentation
◆ HttpReply()
HttpReply::HttpReply | ( | ) |
◆ ~HttpReply()
|
override |
Definition at line 44 of file HttpReply.cc.
Member Function Documentation
◆ bodySize()
int64_t HttpReply::bodySize | ( | const HttpRequestMethod & | method | ) | const |
Definition at line 377 of file HttpReply.cc.
References Http::Message::content_length, HttpRequestMethod::id(), AnyP::ProtocolVersion::major, Http::METHOD_HEAD, Http::scNoContent, Http::scNotModified, Http::scOkay, sline, Http::StatusLine::status(), and Http::StatusLine::version.
Referenced by clientReplyContext::buildReplyHeader(), MemObject::expectedReplySize(), ClientHttpRequest::gotEnough(), HttpStateData::keepaliveAccounting(), HttpStateData::persistentConnStatus(), and clientReplyContext::replyStatus().
◆ calcMaxBodySize()
|
private |
Calculates and stores maximum body size if needed. Used by receivedBodyTooLarge() and expectedBodyTooLarge().
Definition at line 586 of file HttpReply.cc.
References Acl::Answer::allowed(), bodySizeMax, Config, debugs, ACLChecklist::fastCheck(), SquidConfig::ReplyBodySize, and ACLFilledChecklist::updateReply().
Referenced by expectedBodyTooLarge(), and receivedBodyTooLarge().
◆ clean()
|
private |
Definition at line 73 of file HttpReply.cc.
References body, Http::Message::body_pipe, bodySizeMax, Http::StatusLine::clean(), HttpHeader::clean(), HttpBody::clear(), hdrCacheClean(), Http::Message::header, and sline.
Referenced by reset(), and ~HttpReply().
◆ clone()
|
overridevirtual |
Clone this reply. Could be done as a copy-contructor but we do not want to accidentally copy a HttpReply..
Implements Http::Message.
Definition at line 611 of file HttpReply.cc.
References HttpHeader::append(), Http::Message::body_pipe, Http::Message::hdr_sz, hdrCacheInit(), Http::Message::header, Http::Message::http_ver, HttpReply(), Http::Message::pstate, and sline.
Referenced by clientReplyContext::cloneReply(), and recreateOnNotModified().
◆ configureContentLengthInterpreter()
|
overridevirtual |
Implements Http::Message.
Definition at line 501 of file HttpReply.cc.
References Http::ContentLengthInterpreter::applyStatusCodeRules(), sline, and Http::StatusLine::status().
◆ contentRange()
const HttpHdrContRange * HttpReply::contentRange | ( | ) | const |
- Returns
- parsed Content-Range for a 206 response and nil for others
Definition at line 345 of file HttpReply.cc.
References assert, content_range, Http::scPartialContent, sline, and Http::StatusLine::status().
Referenced by Http::Stream::buildRangeHeader(), HttpHdrRange::canonize(), and Client::haveParsedReplyHeaders().
◆ expectedBodyTooLarge()
bool HttpReply::expectedBodyTooLarge | ( | HttpRequest & | request | ) |
Checks whether expected body exceeds known maximum size. Requires a prior call to calcMaxBodySize().
Definition at line 565 of file HttpReply.cc.
References bodySizeMax, calcMaxBodySize(), debugs, expectingBody(), and HttpRequest::method.
Referenced by clientReplyContext::processReplyAccess().
◆ expectingBody()
|
overridevirtual |
Implements Http::Message.
Definition at line 528 of file HttpReply.cc.
References HttpHeader::chunked(), Http::Message::content_length, Http::Message::header, Http::METHOD_HEAD, Http::scNoContent, Http::scNotModified, Http::scOkay, sline, and Http::StatusLine::status().
Referenced by expectedBodyTooLarge(), Client::startAdaptation(), and HttpStateData::truncateVirginBody().
◆ firstLineBuf()
|
inherited |
Definition at line 270 of file Message.cc.
◆ hdrCacheClean()
|
private |
Definition at line 353 of file HttpReply.cc.
References Http::Message::cache_control, String::clean(), content_range, content_type, and surrogate_control.
Referenced by clean(), and recreateOnNotModified().
◆ hdrCacheInit()
|
overridevirtual |
Reimplemented from Http::Message.
Definition at line 321 of file HttpReply.cc.
References String::assign(), Http::CONTENT_LENGTH, Http::Message::content_length, content_range, Http::CONTENT_TYPE, content_type, date, Http::DATE, expires, HttpHeader::getContRange(), HttpHeader::getInt64(), HttpHeader::getSc(), HttpHeader::getStr(), HttpHeader::getTime(), Http::Message::hdrCacheInit(), hdrExpirationTime(), Http::Message::header, Http::Message::http_ver, keep_alive, last_modified, Http::LAST_MODIFIED, Http::Message::persistent(), Http::scPartialContent, sline, Http::StatusLine::status(), surrogate_control, and Http::StatusLine::version.
Referenced by clone(), Ftp::HttpReplyWrapper(), init(), and recreateOnNotModified().
◆ hdrExpirationTime()
|
private |
Definition at line 283 of file HttpReply.cc.
References Http::Message::cache_control, Config, date, Http::DATE, Http::EXPIRES, HttpHeader::getTime(), HttpHeader::has(), HttpHdrCc::hasMaxAge(), HttpHdrCc::hasSMaxAge(), Http::Message::header, SquidConfig::onoff, squid_curtime, Http::VARY, and SquidConfig::vary_ignore_expire.
Referenced by hdrCacheInit().
◆ httpMsgParseError()
|
overridevirtual |
Reimplemented from Http::Message.
Definition at line 515 of file HttpReply.cc.
References Http::Message::httpMsgParseError(), Http::ProtocolVersion(), Http::scInvalidHeader, Http::StatusLine::set(), and sline.
◆ httpMsgParseStep()
parses a 0-terminated buffer into Http::Message.
- Return values
-
1 success 0 need more data (partial parse) -1 parse error
Definition at line 151 of file Message.cc.
References assert, httpMsgIsolateStart(), Http::Message::psParsed, Http::Message::psReadyToParseHeaders, and Http::Message::psReadyToParseStartLine.
◆ inheritProperties()
|
overridevirtual |
Implements Http::Message.
Definition at line 627 of file HttpReply.cc.
References keep_alive, and Http::Message::sources.
◆ init()
|
private |
initialize
Definition at line 51 of file HttpReply.cc.
References do_clean, hdrCacheInit(), Http::StatusLine::init(), Http::Message::psReadyToParseStartLine, Http::Message::pstate, and sline.
Referenced by HttpReply(), and reset().
◆ make304()
HttpReplyPointer HttpReply::make304 | ( | ) | const |
construct a 304 reply and return it
Definition at line 129 of file HttpReply.cc.
References HttpHeader::addEntry(), Http::Message::cache_control, HttpHeaderEntry::clone(), content_type, Http::CONTENT_TYPE, date, Http::DATE, expires, Http::EXPIRES, HttpHeader::findEntry(), Http::Message::header, last_modified, Http::LAST_MODIFIED, Http::OTHER, Http::ProtocolVersion(), Http::Message::putCc(), Http::scNotModified, Http::StatusLine::set(), and sline.
Referenced by packed304Reply(), and clientReplyContext::sendNotModified().
◆ MakeConnectionEstablished()
|
static |
Definition at line 121 of file HttpReply.cc.
References Http::ProtocolVersion(), Http::scOkay, Http::StatusLine::set(), and sline.
Referenced by TunnelStateData::notePeerReadyToShovel(), and ClientHttpRequest::sslBumpStart().
◆ MEMPROXY_CLASS()
|
private |
◆ olderThan()
bool HttpReply::olderThan | ( | const HttpReply * | them | ) | const |
whether our Date header value is smaller than theirs
- Returns
- false if any information is missing
Definition at line 638 of file HttpReply.cc.
References date.
Referenced by HttpStateData::haveParsedReplyHeaders().
◆ pack()
MemBuf * HttpReply::pack | ( | ) | const |
- Returns
- a ready to use mem buffer with a packed reply
Definition at line 112 of file HttpReply.cc.
References MemBuf::init(), and packInto().
Referenced by errorSend(), TunnelStateData::notePeerReadyToShovel(), Http::Stream::sendStartOfMessage(), Mgr::Inquirer::start(), and Http::One::Server::writeControlMsgAndCall().
◆ packed304Reply()
|
private |
- Returns
- construct 304 reply and pack it into a MemBuf
Definition at line 159 of file HttpReply.cc.
References make304().
◆ packFirstLineInto()
|
inlineoverrideprotectedvirtual |
Implements Http::Message.
Definition at line 166 of file HttpReply.h.
References Http::StatusLine::packInto(), and sline.
◆ packHeadersUsingFastPacker()
void HttpReply::packHeadersUsingFastPacker | ( | Packable & | p | ) | const |
adds status line and header to the given Packable assumes that p
can quickly process small additions
Definition at line 87 of file HttpReply.cc.
References Packable::append(), Http::Message::header, Http::StatusLine::packInto(), HttpHeader::packInto(), and sline.
Referenced by packHeadersUsingSlowPacker(), packInto(), and AccessLogEntry::packReplyHeaders().
◆ packHeadersUsingSlowPacker()
void HttpReply::packHeadersUsingSlowPacker | ( | Packable & | p | ) | const |
Definition at line 95 of file HttpReply.cc.
References Packable::append(), MemBuf::content(), MemBuf::contentSize(), MemBuf::init(), and packHeadersUsingFastPacker().
Referenced by TestRock::addEntry(), TestUfs::testUfsSearch(), and MemStore::updateHeadersOrThrow().
◆ packInto() [1/2]
|
private |
Definition at line 104 of file HttpReply.cc.
References body, packHeadersUsingFastPacker(), and HttpBody::packInto().
Referenced by pack().
◆ packInto() [2/2]
|
inherited |
Definition at line 253 of file Message.cc.
References Packable::append().
◆ parse()
|
inherited |
Definition at line 68 of file Message.cc.
References assert, Config, DBG_IMPORTANT, debugs, error(), headersEnd(), int, SquidConfig::maxReplyHeaderSize, Http::scHeaderTooLarge, Http::scInvalidHeader, and Http::scNone.
Referenced by Adaptation::Icap::Xaction::parseHttpMsg(), and parseTerminatedPrefix().
◆ parseCharBuf()
|
inherited |
parseCharBuf() takes character buffer of HTTP headers (buf), which may not be NULL-terminated, and fills in an Http::Message structure. The parameter 'end' specifies the offset to the end of the reply headers. The caller may know where the end is, but is unable to NULL-terminate the buffer. This function returns true on success.
Definition at line 129 of file Message.cc.
References MemBuf::append(), MemBuf::buf, MemBuf::clean(), MemBuf::init(), MemBuf::size, and MemBuf::terminate().
◆ parseFirstLine()
|
overrideprotectedvirtual |
Implements Http::Message.
Definition at line 457 of file HttpReply.cc.
References Http::StatusLine::parse(), protoPrefix, and sline.
◆ parseHeader() [1/2]
|
protectedinherited |
Definition at line 201 of file Message.cc.
References SBuf::c_str(), Http::One::Parser::headerBlockSize(), Http::One::Parser::messageHeaderSize(), Http::One::Parser::mimeHeader(), Http::Message::psError, and Http::Message::psParsed.
◆ parseHeader() [2/2]
bool HttpReply::parseHeader | ( | Http1::Parser & | hp | ) |
Definition at line 507 of file HttpReply.cc.
Referenced by Http::Tunneler::handleResponse().
◆ parseTerminatedPrefix()
Parses response status line and headers at the start of the given NUL-terminated buffer of the given size. Respects reply_header_max_size. Assures pstate becomes Http::Message::psParsed on (and only on) success.
- Returns
- the number of bytes in a successfully parsed prefix (or zero)
- Return values
-
0 implies that more data is needed to parse the response prefix
Definition at line 463 of file HttpReply.cc.
References Assure, Config, debugs, error(), Debug::Extra(), Http::Message::hdr_sz, Here, Less(), SquidConfig::maxReplyHeaderSize, Http::Message::parse(), Http::Message::psParsed, Http::Message::pstate, Http::scNone, and ToSBuf().
◆ persistent()
|
inherited |
- Return values
-
true the message sender asks to keep the connection open. false the message sender will close the connection.
Factors other than the headers may result in connection closure.
Definition at line 236 of file Message.cc.
References httpHeaderHasConnDir(), and Http::ProtocolVersion().
Referenced by clientReplyContext::buildReplyHeader(), clientSetKeepaliveFlag(), hdrCacheInit(), and HttpStateData::sendRequest().
◆ prefixLen()
size_t HttpReply::prefixLen | ( | ) | const |
approximate size of a "status-line CRLF headers CRLF" sequence
- See also
- HttpRequest::prefixLen()
Definition at line 495 of file HttpReply.cc.
References Http::Message::header, HttpHeader::len, Http::StatusLine::packedLength(), and sline.
◆ putCc()
|
inherited |
copies Cache-Control header to this message, overwriting existing Cache-Control header(s), if any
Definition at line 33 of file Message.cc.
Referenced by make304(), and CacheManager::PutCommonResponseHeaders().
◆ receivedBodyTooLarge()
bool HttpReply::receivedBodyTooLarge | ( | HttpRequest & | request, |
int64_t | receivedBodySize | ||
) |
Checks whether received body exceeds known maximum size. Requires a prior call to calcMaxBodySize().
Definition at line 557 of file HttpReply.cc.
References bodySizeMax, calcMaxBodySize(), and debugs.
Referenced by clientReplyContext::replyStatus().
◆ recreateOnNotModified()
HttpReply::Pointer HttpReply::recreateOnNotModified | ( | const HttpReply & | reply304 | ) | const |
- Returns
- nil (if no updates are necessary)
- a new reply combining this reply with 304 updates (otherwise)
Definition at line 265 of file HttpReply.cc.
References clone(), HttpHeader::compact(), hdrCacheClean(), hdrCacheInit(), Http::Message::header, HttpHeader::needUpdate(), and HttpHeader::update().
Referenced by StoreEntry::updateOnNotModified().
◆ redirect()
void HttpReply::redirect | ( | Http::StatusCode | status, |
const char * | loc | ||
) |
Definition at line 205 of file HttpReply.cc.
References Http::CONTENT_LENGTH, Http::Message::content_length, date, Http::DATE, Http::Message::header, Http::LOCATION, Http::ProtocolVersion(), HttpHeader::putInt64(), HttpHeader::putStr(), HttpHeader::putTime(), Http::SERVER, Http::StatusLine::set(), sline, squid_curtime, and visible_appname_string.
Referenced by clientReplyContext::processMiss().
◆ removeIrrelevantContentLength()
void HttpReply::removeIrrelevantContentLength | ( | ) |
Definition at line 646 of file HttpReply.cc.
References Http::CONTENT_LENGTH, debugs, HttpHeader::delById(), Http::Message::header, Http::ProhibitsContentLength(), sline, and Http::StatusLine::status().
Referenced by clientReplyContext::buildReplyHeader(), and Http::One::Server::writeControlMsgAndCall().
◆ reset()
|
overridevirtual |
Implements Http::Message.
Definition at line 59 of file HttpReply.cc.
References clean(), init(), and protoPrefix.
Referenced by MemObject::reset(), and TestHttpReply::testSanityCheckFirstLine().
◆ sanityCheckStartLine()
|
overridevirtual |
Checks the first line of an HTTP Reply is valid. currently only checks "HTTP/" exists.
NP: not all error cases are detected yet. Some are left for detection later in parse.
Implements Http::Message.
Definition at line 402 of file HttpReply.cc.
References String::cmp(), debugs, error(), protoPrefix, String::psize(), Http::scInvalidHeader, String::size(), and xisdigit.
Referenced by TestHttpReply::testSanityCheckFirstLine().
◆ setContentLength()
|
inherited |
Definition at line 228 of file Message.cc.
References Http::CONTENT_LENGTH.
◆ setHeaders()
void HttpReply::setHeaders | ( | Http::StatusCode | status, |
const char * | reason, | ||
const char * | ctype, | ||
int64_t | clen, | ||
time_t | lmt, | ||
time_t | expires | ||
) |
set commonly used info with one call
Definition at line 170 of file HttpReply.cc.
References Http::CONTENT_LENGTH, Http::Message::content_length, Http::CONTENT_TYPE, content_type, date, Http::DATE, expires, Http::EXPIRES, Http::Message::header, last_modified, Http::LAST_MODIFIED, Http::MIME_VERSION, Http::ProtocolVersion(), HttpHeader::putInt64(), HttpHeader::putStr(), HttpHeader::putTime(), Http::SERVER, Http::StatusLine::set(), sline, squid_curtime, and visible_appname_string.
Referenced by StoreEntry::adjustVary(), Ftp::Gateway::appendSuccessHeader(), ErrorState::BuildHttpReply(), TestRock::createEntry(), Mgr::Action::fillEntry(), internalStart(), MimeIcon::load(), netdbBinaryExchange(), clientReplyContext::purgeDoPurge(), WhoisState::setReplyToOK(), Mgr::Inquirer::start(), storeDigestRewriteResume(), TestUfs::testUfsSearch(), clientReplyContext::traceReply(), and urnHandleReply().
◆ validatorsMatch()
Definition at line 223 of file HttpReply.cc.
References assert, Http::Message::content_length, Http::CONTENT_MD5, Http::ETAG, HttpHeader::getStrOrList(), Http::Message::header, last_modified, one, and two.
Member Data Documentation
◆ body
HttpBody HttpReply::body |
for small constant memory-resident text bodies only
Definition at line 58 of file HttpReply.h.
Referenced by ErrorState::BuildHttpReply(), clean(), packInto(), and CacheManager::start().
◆ body_pipe
|
inherited |
Definition at line 97 of file Message.h.
Referenced by HttpRequest::bodyNibbled(), FwdState::checkRetriable(), HttpRequest::clean(), clean(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), HttpRequest::clone(), clone(), Adaptation::Ecap::MessageRep::clone(), FwdState::doneWithRetries(), Adaptation::Icap::ModXact::estimateVirginBody(), Adaptation::Icap::ModXact::finalizeLogInfo(), ClientHttpRequest::handleAdaptationFailure(), Client::handleAdaptedHeader(), HttpRequest::init(), Adaptation::Icap::ModXact::prepEchoing(), HttpStateData::sendRequest(), Adaptation::Icap::InOut::setHeader(), Adaptation::Message::ShortCircuit(), Client::startAdaptation(), Client::startRequestBodyFlow(), Adaptation::Ecap::XactionRep::useAdapted(), and Adaptation::Ecap::XactionRep::useVirgin().
◆ bodySizeMax
|
mutableprivate |
cached result of calcMaxBodySize
Definition at line 161 of file HttpReply.h.
Referenced by calcMaxBodySize(), clean(), expectedBodyTooLarge(), and receivedBodyTooLarge().
◆ cache_control
|
inherited |
Definition at line 76 of file Message.h.
Referenced by HttpRequest::clean(), clientInterpretRequestHeaders(), HttpStateData::haveParsedReplyHeaders(), hdrCacheClean(), hdrExpirationTime(), MimeIcon::load(), make304(), HttpRequest::maybeCacheable(), ClientHttpRequest::onlyIfCached(), refreshCheck(), and HttpStateData::reusableReply().
◆ content_length
|
inherited |
Definition at line 83 of file Message.h.
Referenced by bodySize(), Http::Stream::buildRangeHeader(), HttpHdrRange::canonize(), HttpRequest::checkEntityFraming(), StoreEntry::checkTooBig(), StoreEntry::checkTooSmall(), clientPackRangeHdr(), clientProcessRequest(), expectingBody(), HttpRequest::expectingBody(), Downloader::handleReply(), hdrCacheInit(), HttpStateData::persistentConnStatus(), redirect(), refreshIsCachable(), HttpStateData::sendRequest(), setHeaders(), storeLog(), clientReplyContext::storeNotOKTransferDone(), HttpStateData::truncateVirginBody(), and validatorsMatch().
◆ content_range
|
private |
Definition at line 163 of file HttpReply.h.
Referenced by contentRange(), hdrCacheClean(), and hdrCacheInit().
◆ content_type
String HttpReply::content_type |
Definition at line 46 of file HttpReply.h.
Referenced by Adaptation::Icap::ModXact::finalizeLogInfo(), hdrCacheClean(), hdrCacheInit(), make304(), setHeaders(), and storeLog().
◆ date
time_t HttpReply::date |
- public, readable; never update these or their .hdr equivalents directly
Definition at line 40 of file HttpReply.h.
Referenced by HttpStateData::checkDateSkew(), hdrCacheInit(), hdrExpirationTime(), make304(), olderThan(), HttpStateData::processSurrogateControl(), redirect(), HttpStateData::reusableReply(), setHeaders(), storeLog(), and StoreEntry::timestampsSet().
◆ do_clean
bool HttpReply::do_clean |
Definition at line 62 of file HttpReply.h.
Referenced by init(), and ~HttpReply().
◆ expires
time_t HttpReply::expires |
Definition at line 44 of file HttpReply.h.
Referenced by hdrCacheInit(), make304(), HttpStateData::processSurrogateControl(), HttpStateData::reusableReply(), setHeaders(), storeDigestRewriteResume(), and storeLog().
◆ hdr_sz
|
inherited |
Definition at line 81 of file Message.h.
Referenced by StoreEntry::append(), HttpRequest::clone(), clone(), StoreEntry::contentLen(), store_client::doCopy(), MemObject::expectedReplySize(), Adaptation::Icap::ModXact::finalizeLogInfo(), store_client::finishCallback(), MemObject::markEndOfReplyHeaders(), store_client::nextHttpReadOffset(), Adaptation::Icap::Xaction::parseHttpMsg(), parseTerminatedPrefix(), clientReplyContext::processReplyAccessResult(), MemObject::readAheadPolicyCanRead(), store_client::sendingHttpHeaders(), store_client::skipHttpHeadersFromDisk(), clientReplyContext::storeOKTransferDone(), MemStore::updateHeadersOrThrow(), and StoreEntry::write().
◆ header
|
inherited |
Definition at line 74 of file Message.h.
Referenced by StoreEntry::adjustVary(), Ftp::Gateway::appendSuccessHeader(), Format::Format::assemble(), assembleVaryKey(), Auth::UserRequest::authenticate(), HttpStateData::blockSwitchingProtocols(), ConnStateData::buildFakeRequest(), ErrorState::BuildHttpReply(), Http::One::Server::buildHttpRequest(), Http::Stream::buildRangeHeader(), clientReplyContext::buildReplyHeader(), HttpRequest::canHandle1xx(), HttpRequest::checkEntityFraming(), HttpRequest::clean(), clean(), ClientRequestContext::clientAccessCheck(), clientCheckPinning(), clientGetMoreData(), clientIfRangeMatch(), clientInterpretRequestHeaders(), clientPackRangeHdr(), clientProcessRequest(), HttpRequest::clone(), clone(), Adaptation::Ecap::MessageRep::clone(), ErrorState::compileLegacyCode(), HttpRequest::conditional(), Adaptation::Icap::Options::configure(), Ftp::Relay::createHttpReply(), Adaptation::Icap::ModXact::encapsulateHead(), Adaptation::Icap::ModXact::estimateVirginBody(), expectingBody(), HttpRequest::expectingBody(), Ssl::ErrorDetailsManager::findDetail(), HttpStateData::forwardUpgrade(), Ftp::Gateway::ftpAuthRequired(), ftpSendStor(), Ftp::Server::handleFeatReply(), Ftp::Server::handleRequest(), StoreEntry::hasIfMatchEtag(), StoreEntry::hasIfNoneMatchEtag(), StoreEntry::hasOneOfEtags(), HttpStateData::haveParsedReplyHeaders(), hdrCacheInit(), HttpRequest::hdrCacheInit(), hdrExpirationTime(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), HttpStateData::httpBuildRequestHeader(), Log::Format::HttpdCombined(), httpMakeVaryMark(), Ftp::HttpReplyWrapper(), Auth::SchemeConfig::isCP1251EncodingAllowed(), MimeIcon::load(), TemplateFile::loadFor(), Ftp::Gateway::loginFailed(), make304(), Adaptation::Icap::ModXact::makeRequestHeaders(), HttpRequest::pack(), packHeadersUsingFastPacker(), HttpRequest::parseHeader(), CacheManager::ParseHeaders(), Adaptation::Icap::OptXact::parseResponse(), peerDigestRequest(), prefixLen(), HttpRequest::prefixLen(), prepareLogWithRequestDetails(), Adaptation::Icap::ModXact::prepEchoing(), Ftp::PrintReply(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), Http::One::Server::processParsedRequest(), purgeEntriesByHeader(), CacheManager::PutCommonResponseHeaders(), recreateOnNotModified(), redirect(), removeIrrelevantContentLength(), HttpStateData::reusableReply(), Ftp::Server::setDataCommand(), setHeaders(), UrnState::setUriResFromRequest(), Log::Format::SquidReferer(), Log::Format::SquidUserAgent(), Mgr::Inquirer::start(), CacheManager::start(), HttpStateData::statusIfComplete(), urlCheckRequest(), Adaptation::Ecap::XactionRep::useAdapted(), Adaptation::Ecap::XactionRep::useVirgin(), validatorsMatch(), varyEvaluateMatch(), Http::One::Server::writeControlMsgAndCall(), Ftp::Server::writeCustomReply(), Ftp::Server::writeErrorReply(), Ftp::Server::writeForwardedReply(), and Ftp::Server::writeForwardedReplyAndCall().
◆ http_ver
|
inherited |
HTTP-Version field in the first line of the message. see RFC 7230 section 3.1
Definition at line 72 of file Message.h.
Referenced by Format::Format::assemble(), ErrorState::BuildHttpReply(), clientReplyContext::buildReplyHeader(), HttpRequest::canHandle1xx(), HttpRequest::checkEntityFraming(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), clientSetKeepaliveFlag(), HttpRequest::clone(), clone(), ErrorState::compileLegacyCode(), Adaptation::Icap::ModXact::encapsulateHead(), HttpStateData::forwardUpgrade(), hdrCacheInit(), htcpClear(), htcpQuery(), HttpStateData::httpBuildRequestHeader(), netdbExchangeState::netdbExchangeState(), HttpRequest::pack(), HttpRequest::packFirstLineInto(), HttpRequest::parseFirstLine(), prepareLogWithRequestDetails(), switchToTunnel(), and tunnelStart().
◆ keep_alive
short int HttpReply::keep_alive |
Definition at line 53 of file HttpReply.h.
Referenced by clientReplyContext::buildReplyHeader(), hdrCacheInit(), inheritProperties(), HttpStateData::keepaliveAccounting(), and HttpStateData::statusIfComplete().
◆ last_modified
time_t HttpReply::last_modified |
Definition at line 42 of file HttpReply.h.
Referenced by hdrCacheInit(), make304(), setHeaders(), storeLog(), and validatorsMatch().
◆ protoPrefix
String HttpReply::protoPrefix |
e.g., "HTTP/"
Definition at line 60 of file HttpReply.h.
Referenced by Adaptation::Icap::ModXact::ModXact(), parseFirstLine(), Adaptation::Icap::OptXact::parseResponse(), reset(), sanityCheckStartLine(), and TestHttpReply::testSanityCheckFirstLine().
◆ pstate
|
inherited |
Definition at line 94 of file Message.h.
Referenced by HttpRequest::clone(), clone(), HttpRequest::init(), init(), and parseTerminatedPrefix().
◆ sline
Http::StatusLine HttpReply::sline |
- public, writable, but use httpReply* interfaces when possible
Definition at line 56 of file HttpReply.h.
Referenced by Auth::UserRequest::AddReplyAuthHeader(), bodySize(), Http::Stream::buildRangeHeader(), clientReplyContext::buildReplyHeader(), Adaptation::Icap::Launcher::canRepeat(), clean(), clone(), clientReplyContext::cloneReply(), FwdState::complete(), Adaptation::Icap::Options::configure(), configureContentLengthInterpreter(), contentRange(), Adaptation::Icap::ModXact::encapsulateHead(), ErrorState::ErrorState(), expectingBody(), Adaptation::Icap::ModXact::finalizeLogInfo(), HttpStateData::handle1xx(), Ftp::Server::handleDataReply(), clientReplyContext::handleIMSReply(), Http::Tunneler::handleResponse(), HttpStateData::haveParsedReplyHeaders(), hdrCacheInit(), httpMsgParseError(), Ftp::HttpReplyWrapper(), init(), make304(), MakeConnectionEstablished(), Client::maybePurgeOthers(), netdbExchangeHandleReply(), packFirstLineInto(), packHeadersUsingFastPacker(), parseFirstLine(), peerDigestFetchReply(), prefixLen(), clientReplyContext::processConditional(), Http::One::Server::processParsedRequest(), clientReplyContext::processReplyAccess(), HttpStateData::processReplyHeader(), redirect(), FwdState::reforward(), removeIrrelevantContentLength(), HttpStateData::reusableReply(), setHeaders(), clientReplyContext::setReplyToReply(), CacheManager::start(), storeLog(), Http::One::Server::writeControlMsgAndCall(), and Ftp::Server::writeErrorReply().
◆ sources
|
inherited |
Definition at line 99 of file Message.h.
Referenced by Ftp::Gateway::appendSuccessHeader(), ConnStateData::buildFakeRequest(), clientProcessRequest(), Ftp::Relay::forwardReply(), Http::Tunneler::handleResponse(), inheritProperties(), HttpRequest::inheritProperties(), Acl::ConnectionsEncrypted::match(), WhoisState::setReplyToOK(), Ftp::Relay::startDataDownload(), and Adaptation::Ecap::XactionRep::updateSources().
◆ surrogate_control
HttpHdrSc* HttpReply::surrogate_control |
Definition at line 48 of file HttpReply.h.
Referenced by hdrCacheClean(), hdrCacheInit(), and HttpStateData::processSurrogateControl().
The documentation for this class was generated from the following files:
- src/HttpReply.h
- src/HttpReply.cc
- src/tests/stub_HttpReply.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products