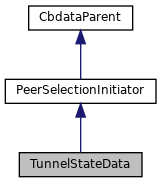
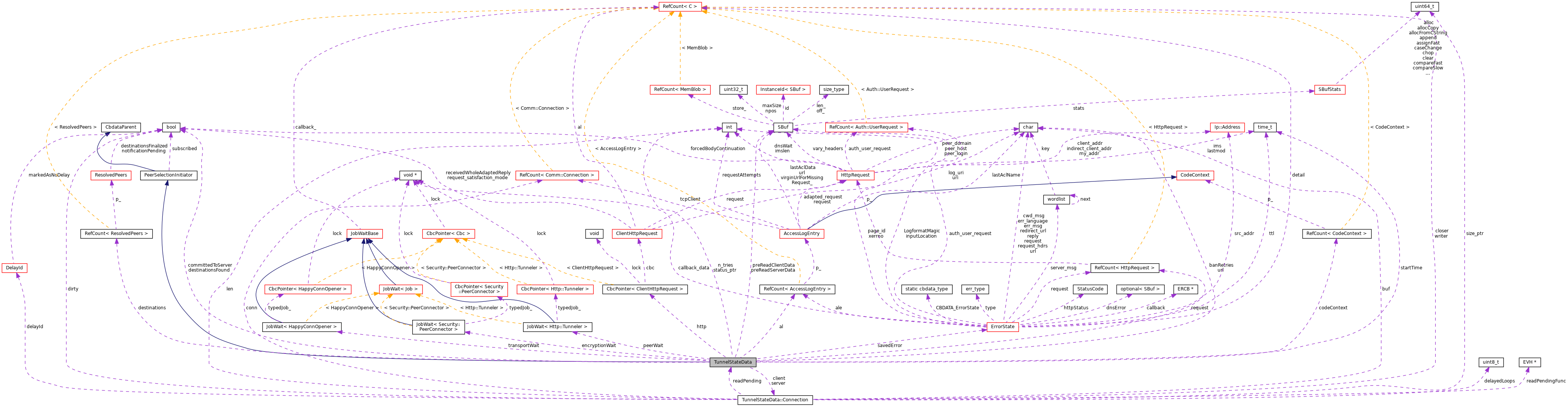
Classes | |
class | Connection |
Public Member Functions | |
TunnelStateData (ClientHttpRequest *) | |
~TunnelStateData () override | |
TunnelStateData (const TunnelStateData &) | |
TunnelStateData & | operator= (const TunnelStateData &) |
bool | noConnections () const |
void | closeConnections () |
closes both client and server connections More... | |
const char * | getHost () const |
void | commitToServer (const Comm::ConnectionPointer &) |
bool | clientExpectsConnectResponse () const |
Whether the client sent a CONNECT request to us. More... | |
void | startConnecting () |
void | closePendingConnection (const Comm::ConnectionPointer &conn, const char *reason) |
void | notePeerReadyToShovel (const Comm::ConnectionPointer &) |
called when negotiations with the peer have been successfully completed More... | |
void | copyRead (Connection &from, IOCB *completion) |
void | connectToPeer (const Comm::ConnectionPointer &) |
continue to set up connection to a peer, going async for SSL peers More... | |
void | secureConnectionToPeer (const Comm::ConnectionPointer &) |
encrypts an established TCP connection to peer More... | |
void | noteDestination (Comm::ConnectionPointer conn) override |
called when a new unique destination has been found More... | |
void | noteDestinationsEnd (ErrorState *selectionError) override |
void | syncHierNote (const Comm::ConnectionPointer &server, const char *origin) |
void | noteConnection (HappyConnOpenerAnswer &) |
void | connectDone (const Comm::ConnectionPointer &conn, const char *origin, const bool reused) |
Start using an established connection. More... | |
void | notifyConnOpener () |
makes sure connection opener knows that the destinations have changed More... | |
void | saveError (ErrorState *finalError) |
remembers an error to be used if there will be no more connection attempts More... | |
void | sendError (ErrorState *finalError, const char *reason) |
bool | keepGoingAfterRead (size_t len, Comm::Flag errcode, int xerrno, Connection &from, Connection &to) |
void | copy (size_t len, Connection &from, Connection &to, IOCB *) |
void | readServer (char *buf, size_t len, Comm::Flag errcode, int xerrno) |
void | readClient (char *buf, size_t len, Comm::Flag errcode, int xerrno) |
void | writeClientDone (char *buf, size_t len, Comm::Flag flag, int xerrno) |
void | writeServerDone (char *buf, size_t len, Comm::Flag flag, int xerrno) |
void | copyClientBytes () |
void | copyServerBytes () |
void | clientClosed () |
handles client-to-Squid connection closure; may destroy us More... | |
void | serverClosed () |
handles Squid-to-server connection closure; may destroy us More... | |
void | retryOrBail (const char *context) |
void | startSelectingDestinations (HttpRequest *request, const AccessLogEntry::Pointer &ale, StoreEntry *entry) |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | ReadClient (const Comm::ConnectionPointer &, char *buf, size_t len, Comm::Flag errcode, int xerrno, void *data) |
static void | ReadServer (const Comm::ConnectionPointer &, char *buf, size_t len, Comm::Flag errcode, int xerrno, void *data) |
static void | WriteClientDone (const Comm::ConnectionPointer &, char *buf, size_t len, Comm::Flag flag, int xerrno, void *data) |
static void | WriteServerDone (const Comm::ConnectionPointer &, char *buf, size_t len, Comm::Flag flag, int xerrno, void *data) |
Public Attributes | |
char * | url |
CbcPointer< ClientHttpRequest > | http |
HttpRequest::Pointer | request |
AccessLogEntryPointer | al |
Connection | client |
Connection | server |
int * | status_ptr |
pointer for logging HTTP status More... | |
SBuf | preReadClientData |
SBuf | preReadServerData |
time_t | startTime |
object creation time, before any peer selection/connection attempts More... | |
ResolvedPeersPointer | destinations |
paths for forwarding the request More... | |
bool | destinationsFound |
At least one candidate path found. More... | |
bool | committedToServer |
whether the decision to tunnel to a particular destination was final More... | |
int | n_tries |
the number of forwarding attempts so far More... | |
const char * | banRetries |
a reason to ban reforwarding attempts (or nil) More... | |
CodeContext::Pointer | codeContext |
our creator context More... | |
JobWait< HappyConnOpener > | transportWait |
waits for a transport connection to the peer to be established/opened More... | |
JobWait< Security::PeerConnector > | encryptionWait |
waits for the established transport connection to be secured/encrypted More... | |
JobWait< Http::Tunneler > | peerWait |
PeeringActivityTimer | peeringTimer |
Measures time spent on selecting and communicating with peers. More... | |
bool | subscribed = false |
whether noteDestination() and noteDestinationsEnd() calls are allowed More... | |
Private Member Functions | |
CBDATA_CHILD (TunnelStateData) | |
void | usePinned () |
send request on an existing connection dedicated to the requesting client More... | |
void | noteSecurityPeerConnectorAnswer (Security::EncryptorAnswer &) |
callback handler for the Security::PeerConnector encryptor More... | |
void | connectedToPeer (const Comm::ConnectionPointer &) |
called after connection setup (including any encryption) More... | |
void | establishTunnelThruProxy (const Comm::ConnectionPointer &) |
template<typename StepStart > | |
void | advanceDestination (const char *stepDescription, const Comm::ConnectionPointer &conn, const StepStart &startStep) |
starts a preparation step for an established connection; retries on failures More... | |
const char * | checkRetry () |
bool | transporting () const |
void | tunnelEstablishmentDone (Http::TunnelerAnswer &answer) |
resumes operations after the (possibly failed) HTTP CONNECT exchange More... | |
void | finishWritingAndDelete (Connection &) |
void | deleteThis () |
destroys the tunnel (after performing potentially-throwing cleanup) More... | |
void | cancelStep (const char *reason) |
bool | exhaustedTries () const |
whether we have used up all permitted forwarding attempts More... | |
void | updateAttempts (int) |
sets n_tries to the given value (while keeping ALE in sync) More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
ErrorState * | savedError = nullptr |
details of the "last tunneling attempt" failure (if it failed) More... | |
Detailed Description
TunnelStateData is the state engine performing the tasks for setup of a TCP tunnel from an existing open client FD to a server then shuffling binary data between the resulting FD pair.
Constructor & Destructor Documentation
◆ TunnelStateData() [1/2]
TunnelStateData::TunnelStateData | ( | ClientHttpRequest * | clientRequest | ) |
Definition at line 402 of file tunnel.cc.
References al, ClientHttpRequest::al, assert, AccessLogEntry::cache, client, Server::clientConnection, AccessLogEntry::CacheDetails::code, commCbCall(), commSetConnTimeout(), Config, TunnelStateData::Connection::conn, debugs, ClientHttpRequest::getConn(), http, TunnelStateData::Connection::initConnection(), SquidConfig::lifetime, LOG_TCP_TUNNEL, Must, ClientHttpRequest::out, TunnelStateData::Connection::readPendingFunc, request, ClientHttpRequest::request, server, ClientHttpRequest::Out::size, TunnelStateData::Connection::size_ptr, status_ptr, SquidConfig::Timeout, tunnelClientClosed, tunnelDelayedClientRead, tunnelDelayedServerRead, tunnelTimeout, LogTags::update(), ClientHttpRequest::uri, url, and xstrdup.
Referenced by switchToTunnel(), and tunnelStart().
◆ ~TunnelStateData()
|
override |
Definition at line 435 of file tunnel.cc.
References assert, cancelStep(), debugs, noConnections(), savedError, url, and xfree.
◆ TunnelStateData() [2/2]
TunnelStateData::TunnelStateData | ( | const TunnelStateData & | ) |
Member Function Documentation
◆ advanceDestination()
|
private |
Definition at line 1253 of file tunnel.cc.
References al, closePendingConnection(), CurrentException(), debugs, ERR_CANNOT_FORWARD, RefCount< C >::getRaw(), request, retryOrBail(), savedError, saveError(), and Http::scInternalServerError.
Referenced by connectedToPeer(), and connectToPeer().
◆ cancelStep()
|
private |
Notify a pending subtask, if any, that we no longer need its help. We do not have to do this – the subtask job will eventually end – but ending it earlier reduces waste and may reduce DoS attack surface.
Definition at line 1441 of file tunnel.cc.
References encryptionWait, peerWait, and transportWait.
Referenced by sendError(), and ~TunnelStateData().
◆ CBDATA_CHILD()
|
private |
◆ checkRetry()
|
private |
- Returns
- whether the request should be retried (nil) or the description why it should not
Definition at line 453 of file tunnel.cc.
References banRetries, FwdState::EnoughTimeToReForward(), exhaustedTries(), HttpRequest::hier, Http::IsReforwardableStatus(), noConnections(), HierarchyLogEntry::peer_reply_status, request, Http::scNone, shutting_down, and startTime.
Referenced by retryOrBail().
◆ clientClosed()
void TunnelStateData::clientClosed | ( | ) |
Definition at line 330 of file tunnel.cc.
References client, finishWritingAndDelete(), TunnelStateData::Connection::noteClosure(), and server.
Referenced by tunnelClientClosed().
◆ clientExpectsConnectResponse()
|
inline |
Definition at line 108 of file tunnel.cc.
References Ssl::ServerBump::at(), HttpRequest::flags, RequestFlags::forceTunnel, ClientHttpRequest::getConn(), http, RequestFlags::intercepted, RequestFlags::interceptTproxy, request, ConnStateData::serverBump(), tlsBump2, and tlsBump3.
Referenced by notePeerReadyToShovel(), and retryOrBail().
◆ closeConnections()
void TunnelStateData::closeConnections | ( | ) |
Definition at line 870 of file tunnel.cc.
References client, Comm::Connection::close(), TunnelStateData::Connection::conn, Comm::IsConnOpen(), and server.
Referenced by tunnelTimeout().
◆ closePendingConnection()
void TunnelStateData::closePendingConnection | ( | const Comm::ConnectionPointer & | conn, |
const char * | reason | ||
) |
Definition at line 861 of file tunnel.cc.
References assert, Comm::Connection::close(), TunnelStateData::Connection::conn, debugs, Comm::IsConnOpen(), and server.
Referenced by advanceDestination(), noteConnection(), noteSecurityPeerConnectorAnswer(), and tunnelEstablishmentDone().
◆ commitToServer()
void TunnelStateData::commitToServer | ( | const Comm::ConnectionPointer & | conn | ) |
store the given to-server connection; prohibit retries and do not look for any other destinations
Definition at line 1086 of file tunnel.cc.
References banRetries, committedToServer, TunnelStateData::Connection::initConnection(), server, PeerSelectionInitiator::subscribed, and tunnelServerClosed.
Referenced by notePeerReadyToShovel(), and switchToTunnel().
◆ connectDone()
void TunnelStateData::connectDone | ( | const Comm::ConnectionPointer & | conn, |
const char * | origin, | ||
const bool | reused | ||
) |
Definition at line 1138 of file tunnel.cc.
References connectToPeer(), Comm::Connection::getPeer(), RefCount< C >::getRaw(), AnyP::Uri::host(), Comm::IsConnOpen(), Must, netdbPingSite(), CachePeer::no_delay, notePeerReadyToShovel(), CachePeer::options, HttpRequest::prepForDirect(), HttpRequest::prepForPeering(), request, ResetMarkingsToServer(), server, TunnelStateData::Connection::setDelayId(), syncHierNote(), and HttpRequest::url.
Referenced by noteConnection(), and usePinned().
◆ connectedToPeer()
|
private |
Definition at line 1296 of file tunnel.cc.
References advanceDestination(), and establishTunnelThruProxy().
Referenced by connectToPeer(), and noteSecurityPeerConnectorAnswer().
◆ connectToPeer()
void TunnelStateData::connectToPeer | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 1229 of file tunnel.cc.
References advanceDestination(), connectedToPeer(), Comm::Connection::getPeer(), and secureConnectionToPeer().
Referenced by connectDone().
◆ copy()
void TunnelStateData::copy | ( | size_t | len, |
Connection & | from, | ||
Connection & | to, | ||
IOCB * | completion | ||
) |
Definition at line 696 of file tunnel.cc.
References TunnelStateData::Connection::buf, commCbCall(), debugs, and TunnelStateData::Connection::write().
Referenced by copyClientBytes(), copyServerBytes(), readClient(), readServer(), and tunnelStartShoveling().
◆ copyClientBytes()
void TunnelStateData::copyClientBytes | ( | ) |
Definition at line 933 of file tunnel.cc.
References TunnelStateData::Connection::buf, TunnelStateData::Connection::bytesIn(), client, SBuf::consume(), copy(), copyRead(), keepGoingAfterRead(), SBuf::length(), Comm::OK, preReadClientData, SBuf::rawContent(), ReadClient(), server, and WriteServerDone().
Referenced by tunnelStartShoveling(), and writeServerDone().
◆ copyRead()
void TunnelStateData::copyRead | ( | Connection & | from, |
IOCB * | completion | ||
) |
Definition at line 911 of file tunnel.cc.
References assert, TunnelStateData::Connection::buf, TunnelStateData::Connection::bytesWanted(), comm_read(), commCbCall(), TunnelStateData::Connection::conn, TunnelStateData::Connection::delayedLoops, eventAdd(), TunnelStateData::Connection::len, TunnelStateData::Connection::readPending, and TunnelStateData::Connection::readPendingFunc.
Referenced by copyClientBytes(), copyServerBytes(), tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ copyServerBytes()
void TunnelStateData::copyServerBytes | ( | ) |
Definition at line 947 of file tunnel.cc.
References TunnelStateData::Connection::buf, TunnelStateData::Connection::bytesIn(), client, SBuf::consume(), copy(), copyRead(), keepGoingAfterRead(), SBuf::length(), Comm::OK, preReadServerData, SBuf::rawContent(), ReadServer(), server, and WriteClientDone().
Referenced by tunnelStartShoveling(), and writeClientDone().
◆ deleteThis()
|
private |
Definition at line 379 of file tunnel.cc.
References assert, http, and noConnections().
Referenced by finishWritingAndDelete().
◆ establishTunnelThruProxy()
|
private |
Definition at line 1304 of file tunnel.cc.
References al, asyncCallback, Config, TunnelStateData::Connection::delayId, SquidConfig::lifetime, peerWait, request, server, JobWait< Job >::start(), SquidConfig::Timeout, and tunnelEstablishmentDone().
Referenced by connectedToPeer().
◆ exhaustedTries()
|
private |
Definition at line 1176 of file tunnel.cc.
References Config, SquidConfig::forward_max_tries, and n_tries.
Referenced by checkRetry().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finishWritingAndDelete()
|
private |
Gracefully handles non-retriable connection closure. If necessary, either starts closing the given connection or waits for its pending write to finish. Otherwise, immediately destroys the tunnel object. \prec The other Connection is not open.
Definition at line 341 of file tunnel.cc.
References Comm::Connection::close(), TunnelStateData::Connection::conn, debugs, deleteThis(), noConnections(), and TunnelStateData::Connection::writer.
Referenced by clientClosed(), retryOrBail(), and serverClosed().
◆ getHost()
|
inline |
Definition at line 99 of file tunnel.cc.
References TunnelStateData::Connection::conn, Comm::Connection::getPeer(), CachePeer::host, AnyP::Uri::host(), request, server, and HttpRequest::url.
Referenced by switchToTunnel().
◆ keepGoingAfterRead()
bool TunnelStateData::keepGoingAfterRead | ( | size_t | len, |
Comm::Flag | errcode, | ||
int | xerrno, | ||
Connection & | from, | ||
Connection & | to | ||
) |
Updates state after reading from client or server. Returns whether the caller should use the data just read.
Definition at line 653 of file tunnel.cc.
References cbdataReferenceValid(), Comm::Connection::close(), commCbCall(), commSetConnTimeout(), Config, TunnelStateData::Connection::conn, debugs, TunnelStateData::Connection::error(), Comm::IsConnOpen(), TunnelStateData::Connection::len, SquidConfig::read, SquidConfig::Timeout, and tunnelTimeout.
Referenced by copyClientBytes(), copyServerBytes(), readClient(), and readServer().
◆ noConnections()
bool TunnelStateData::noConnections | ( | ) | const |
Definition at line 1504 of file tunnel.cc.
References client, TunnelStateData::Connection::conn, Comm::IsConnOpen(), and server.
Referenced by checkRetry(), deleteThis(), finishWritingAndDelete(), and ~TunnelStateData().
◆ noteConnection()
void TunnelStateData::noteConnection | ( | HappyConnOpenerAnswer & | answer | ) |
called when a connection has been successfully established or when all candidate destinations have been tried and all have failed
Definition at line 1111 of file tunnel.cc.
References al, banRetries, CbcPointer< Cbc >::clear(), closePendingConnection(), HappyConnOpenerAnswer::conn, connectDone(), ERR_CANNOT_FORWARD, HappyConnOpenerAnswer::error, error(), Comm::Connection::fd, fd_table, CbcPointer< Cbc >::get(), RefCount< C >::getRaw(), AnyP::Uri::host(), Comm::IsConnOpen(), Must, HappyConnOpenerAnswer::n_tries, request, retryOrBail(), HappyConnOpenerAnswer::reused, saveError(), Http::scServiceUnavailable, syncHierNote(), transportWait, updateAttempts(), and HttpRequest::url.
Referenced by startConnecting().
◆ noteDestination()
|
overridevirtual |
Implements PeerSelectionInitiator.
Definition at line 1315 of file tunnel.cc.
References ResolvedPeers::addPath(), assert, destinations, destinationsFound, ResolvedPeers::empty(), notifyConnOpener(), startConnecting(), transporting(), transportWait, and usePinned().
◆ noteDestinationsEnd()
|
overridevirtual |
called when there will be no more noteDestination() calls
- Parameters
-
error is a possible reason why no destinations were found; it is guaranteed to be nil if there was at least one noteDestination() call
Implements PeerSelectionInitiator.
Definition at line 1342 of file tunnel.cc.
References al, assert, debugs, destinations, ResolvedPeers::destinationsFinalized, destinationsFound, ERR_CANNOT_FORWARD, RefCount< C >::getRaw(), MakeNamedErrorDetail(), Must, n_tries, notifyConnOpener(), request, savedError, saveError(), Http::scBadGateway, Http::scInternalServerError, sendError(), PeerSelectionInitiator::subscribed, transporting(), and transportWait.
◆ notePeerReadyToShovel()
void TunnelStateData::notePeerReadyToShovel | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 1067 of file tunnel.cc.
References al, assert, client, clientExpectsConnectResponse(), commCbCall(), commitToServer(), TunnelStateData::Connection::dirty, HttpReply::MakeConnectionEstablished(), HttpReply::pack(), AccessLogEntry::reply, Http::scOkay, status_ptr, tunnelConnectedWriteDone(), tunnelStartShoveling(), and TunnelStateData::Connection::write().
Referenced by connectDone(), and tunnelEstablishmentDone().
◆ noteSecurityPeerConnectorAnswer()
|
private |
callback handler for the connection encryptor
Definition at line 1272 of file tunnel.cc.
References al, assert, CbcPointer< Cbc >::clear(), closePendingConnection(), Security::EncryptorAnswer::conn, connectedToPeer(), encryptionWait, ERR_CANNOT_FORWARD, Security::EncryptorAnswer::error, error(), Comm::Connection::fd, fd_table, CbcPointer< Cbc >::get(), RefCount< C >::getRaw(), Comm::IsConnOpen(), request, retryOrBail(), saveError(), Http::scServiceUnavailable, and Security::EncryptorAnswer::tunneled.
Referenced by secureConnectionToPeer().
◆ notifyConnOpener()
void TunnelStateData::notifyConnOpener | ( | ) |
Definition at line 1520 of file tunnel.cc.
References CallJobHere, debugs, destinations, JobWait< Job >::job(), ResolvedPeers::notificationPending, and transportWait.
Referenced by noteDestination(), and noteDestinationsEnd().
◆ operator=()
TunnelStateData& TunnelStateData::operator= | ( | const TunnelStateData & | ) |
◆ readClient()
void TunnelStateData::readClient | ( | char * | buf, |
size_t | len, | ||
Comm::Flag | errcode, | ||
int | xerrno | ||
) |
Definition at line 628 of file tunnel.cc.
References TunnelStateData::Connection::bytesIn(), client, StatCounters::client_http, TunnelStateData::Connection::conn, copy(), debugs, TunnelStateData::Connection::delayedLoops, Comm::ERR_CLOSING, StatCounters::kbytes_in, keepGoingAfterRead(), server, statCounter, and WriteServerDone().
Referenced by ReadClient().
◆ ReadClient()
|
static |
Definition at line 619 of file tunnel.cc.
References assert, cbdataReferenceValid(), and readClient().
Referenced by copyClientBytes(), and tunnelDelayedClientRead().
◆ readServer()
void TunnelStateData::readServer | ( | char * | buf, |
size_t | len, | ||
Comm::Flag | errcode, | ||
int | xerrno | ||
) |
Definition at line 584 of file tunnel.cc.
References StatCounters::all, TunnelStateData::Connection::bytesIn(), client, TunnelStateData::Connection::conn, copy(), debugs, TunnelStateData::Connection::delayedLoops, Comm::ERR_CLOSING, HttpRequest::hier, keepGoingAfterRead(), HierarchyLogEntry::notePeerRead(), StatCounters::other, request, StatCounters::server, server, statCounter, and WriteClientDone().
Referenced by ReadServer().
◆ ReadServer()
|
static |
Definition at line 574 of file tunnel.cc.
References assert, cbdataReferenceValid(), debugs, and readServer().
Referenced by copyServerBytes(), and tunnelDelayedServerRead().
◆ retryOrBail()
void TunnelStateData::retryOrBail | ( | const char * | context | ) |
tries connecting to another destination, if available, otherwise, initiates the transaction termination
Definition at line 475 of file tunnel.cc.
References al, assert, checkRetry(), client, clientExpectsConnectResponse(), TunnelStateData::Connection::conn, debugs, destinations, TunnelStateData::Connection::dirty, ResolvedPeers::empty(), ERR_CANNOT_FORWARD, finishWritingAndDelete(), RefCount< C >::getRaw(), ErrorState::httpStatus, Comm::IsConnOpen(), peeringTimer, request, savedError, saveError(), Http::scInternalServerError, sendError(), server, startConnecting(), status_ptr, PeeringActivityTimer::stop(), and PeerSelectionInitiator::subscribed.
Referenced by advanceDestination(), noteConnection(), noteSecurityPeerConnectorAnswer(), and tunnelEstablishmentDone().
◆ saveError()
void TunnelStateData::saveError | ( | ErrorState * | finalError | ) |
Definition at line 1402 of file tunnel.cc.
References assert, debugs, error(), and savedError.
Referenced by advanceDestination(), noteConnection(), noteDestinationsEnd(), noteSecurityPeerConnectorAnswer(), retryOrBail(), and tunnelEstablishmentDone().
◆ secureConnectionToPeer()
void TunnelStateData::secureConnectionToPeer | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 1243 of file tunnel.cc.
References al, asyncCallback, encryptionWait, noteSecurityPeerConnectorAnswer(), request, and JobWait< Job >::start().
Referenced by connectToPeer().
◆ sendError()
void TunnelStateData::sendError | ( | ErrorState * | finalError, |
const char * | reason | ||
) |
Starts sending the given error message to the client, leading to the eventual transaction termination. Call with savedError to send savedError.
Definition at line 1413 of file tunnel.cc.
References assert, ErrorState::callback, ErrorState::callback_data, cancelStep(), client, TunnelStateData::Connection::conn, debugs, errorSend(), ErrorState::httpStatus, peeringTimer, savedError, status_ptr, PeeringActivityTimer::stop(), PeerSelectionInitiator::subscribed, and tunnelErrorComplete.
Referenced by noteDestinationsEnd(), retryOrBail(), and usePinned().
◆ serverClosed()
void TunnelStateData::serverClosed | ( | ) |
Definition at line 312 of file tunnel.cc.
References client, finishWritingAndDelete(), TunnelStateData::Connection::noteClosure(), peeringTimer, server, and PeeringActivityTimer::stop().
Referenced by tunnelServerClosed().
◆ startConnecting()
void TunnelStateData::startConnecting | ( | ) |
starts connecting to the next hop, either for the first time or while recovering from the previous connect failure
Definition at line 1449 of file tunnel.cc.
References al, assert, asyncCallback, destinations, ResolvedPeers::empty(), HttpRequest::hier, AnyP::Uri::host(), n_tries, noteConnection(), ResolvedPeers::notificationPending, HierarchyLogEntry::peer_reply_status, request, savedError, Http::scNone, JobWait< Job >::start(), startTime, transporting(), transportWait, and HttpRequest::url.
Referenced by noteDestination(), and retryOrBail().
◆ startSelectingDestinations()
|
inherited |
Initiates asynchronous peer selection that eventually results in zero or more noteDestination() calls and exactly one noteDestinationsEnd() call.
Definition at line 330 of file peer_select.cc.
References peerSelect(), and PeerSelectionInitiator::subscribed.
Referenced by FwdState::start(), and tunnelStart().
◆ syncHierNote()
void TunnelStateData::syncHierNote | ( | const Comm::ConnectionPointer & | conn, |
const char * | origin | ||
) |
update "hierarchy" annotations with a new (possibly failed) destination
- Parameters
-
origin the name of the origin server we were trying to reach
Definition at line 535 of file tunnel.cc.
References al, HttpRequest::hier, AccessLogEntry::hier, request, and HierarchyLogEntry::resetPeerNotes().
Referenced by connectDone(), noteConnection(), and usePinned().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ transporting()
|
private |
Whether a tunneling attempt to some selected destination X is in progress (after successfully opening/reusing a transport connection to X).
- See also
- transportWait
Definition at line 1395 of file tunnel.cc.
References committedToServer, encryptionWait, and peerWait.
Referenced by noteDestination(), noteDestinationsEnd(), and startConnecting().
◆ tunnelEstablishmentDone()
|
private |
Definition at line 1024 of file tunnel.cc.
References al, assert, CbcPointer< Cbc >::clear(), closePendingConnection(), Http::TunnelerAnswer::conn, ERR_CANNOT_FORWARD, error(), Comm::Connection::fd, fd_table, CbcPointer< Cbc >::get(), RefCount< C >::getRaw(), Comm::IsConnOpen(), Http::TunnelerAnswer::leftovers, TunnelStateData::Connection::len, Must, notePeerReadyToShovel(), Http::TunnelerAnswer::peerResponseStatus, peerWait, Http::TunnelerAnswer::positive(), preReadServerData, request, retryOrBail(), saveError(), Http::scNone, Http::scServiceUnavailable, server, Http::TunnelerAnswer::squidError, and status_ptr.
Referenced by establishTunnelThruProxy().
◆ updateAttempts()
|
private |
Definition at line 543 of file tunnel.cc.
References al, Assure, debugs, n_tries, and AccessLogEntry::requestAttempts.
Referenced by noteConnection(), and usePinned().
◆ usePinned()
|
private |
Definition at line 1469 of file tunnel.cc.
References al, Assure, RequestFlags::auth, ConnStateData::BorrowPinnedConnection(), connectDone(), debugs, error(), HttpRequest::flags, RefCount< C >::getRaw(), AnyP::Uri::host(), Must, n_tries, RequestFlags::pinned, HttpRequest::pinnedConnection(), request, sendError(), syncHierNote(), updateAttempts(), and HttpRequest::url.
Referenced by noteDestination().
◆ writeClientDone()
void TunnelStateData::writeClientDone | ( | char * | buf, |
size_t | len, | ||
Comm::Flag | flag, | ||
int | xerrno | ||
) |
Definition at line 811 of file tunnel.cc.
References cbdataReferenceValid(), client, StatCounters::client_http, Comm::Connection::close(), TunnelStateData::Connection::conn, copyServerBytes(), TunnelStateData::Connection::dataSent(), debugs, Comm::ERR_CLOSING, TunnelStateData::Connection::error(), Comm::IsConnOpen(), StatCounters::kbytes_out, Comm::OK, server, and statCounter.
Referenced by WriteClientDone().
◆ WriteClientDone()
|
static |
Definition at line 759 of file tunnel.cc.
References assert, cbdataReferenceValid(), client, writeClientDone(), and TunnelStateData::Connection::writer.
Referenced by copyServerBytes(), readServer(), and tunnelStartShoveling().
◆ writeServerDone()
void TunnelStateData::writeServerDone | ( | char * | buf, |
size_t | len, | ||
Comm::Flag | flag, | ||
int | xerrno | ||
) |
Definition at line 716 of file tunnel.cc.
References StatCounters::all, cbdataReferenceValid(), client, Comm::Connection::close(), TunnelStateData::Connection::conn, copyClientBytes(), TunnelStateData::Connection::dataSent(), debugs, Comm::ERR_CLOSING, TunnelStateData::Connection::error(), HttpRequest::hier, Comm::IsConnOpen(), HierarchyLogEntry::notePeerWrite(), Comm::OK, StatCounters::other, request, StatCounters::server, server, and statCounter.
Referenced by WriteServerDone().
◆ WriteServerDone()
|
static |
Definition at line 706 of file tunnel.cc.
References assert, cbdataReferenceValid(), server, TunnelStateData::Connection::writer, and writeServerDone().
Referenced by copyClientBytes(), and readClient().
Member Data Documentation
◆ al
AccessLogEntryPointer TunnelStateData::al |
Definition at line 97 of file tunnel.cc.
Referenced by advanceDestination(), establishTunnelThruProxy(), noteConnection(), noteDestinationsEnd(), notePeerReadyToShovel(), noteSecurityPeerConnectorAnswer(), retryOrBail(), secureConnectionToPeer(), startConnecting(), syncHierNote(), tunnelEstablishmentDone(), TunnelStateData(), updateAttempts(), and usePinned().
◆ banRetries
const char* TunnelStateData::banRetries |
Definition at line 199 of file tunnel.cc.
Referenced by checkRetry(), commitToServer(), and noteConnection().
◆ client
Connection TunnelStateData::client |
Definition at line 184 of file tunnel.cc.
Referenced by clientClosed(), closeConnections(), copyClientBytes(), copyServerBytes(), noConnections(), notePeerReadyToShovel(), readClient(), readServer(), retryOrBail(), sendError(), serverClosed(), tunnelConnectedWriteDone(), tunnelDelayedClientRead(), tunnelErrorComplete(), tunnelStartShoveling(), TunnelStateData(), WriteClientDone(), writeClientDone(), and writeServerDone().
◆ codeContext
CodeContext::Pointer TunnelStateData::codeContext |
Definition at line 202 of file tunnel.cc.
Referenced by tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ committedToServer
bool TunnelStateData::committedToServer |
Definition at line 194 of file tunnel.cc.
Referenced by commitToServer(), and transporting().
◆ destinations
ResolvedPeersPointer TunnelStateData::destinations |
Definition at line 190 of file tunnel.cc.
Referenced by noteDestination(), noteDestinationsEnd(), notifyConnOpener(), retryOrBail(), and startConnecting().
◆ destinationsFound
bool TunnelStateData::destinationsFound |
Definition at line 191 of file tunnel.cc.
Referenced by noteDestination(), and noteDestinationsEnd().
◆ encryptionWait
JobWait<Security::PeerConnector> TunnelStateData::encryptionWait |
Definition at line 208 of file tunnel.cc.
Referenced by cancelStep(), noteSecurityPeerConnectorAnswer(), secureConnectionToPeer(), transporting(), and tunnelStartShoveling().
◆ http
CbcPointer<ClientHttpRequest> TunnelStateData::http |
Definition at line 95 of file tunnel.cc.
Referenced by clientExpectsConnectResponse(), deleteThis(), tunnelConnectedWriteDone(), tunnelStart(), tunnelStartShoveling(), and TunnelStateData().
◆ n_tries
int TunnelStateData::n_tries |
Definition at line 196 of file tunnel.cc.
Referenced by exhaustedTries(), noteDestinationsEnd(), startConnecting(), updateAttempts(), and usePinned().
◆ peeringTimer
PeeringActivityTimer TunnelStateData::peeringTimer |
Definition at line 215 of file tunnel.cc.
Referenced by retryOrBail(), sendError(), and serverClosed().
◆ peerWait
JobWait<Http::Tunneler> TunnelStateData::peerWait |
waits for an HTTP CONNECT tunnel through a cache_peer to be negotiated over the (encrypted, if needed) transport connection to that cache_peer
Definition at line 212 of file tunnel.cc.
Referenced by cancelStep(), establishTunnelThruProxy(), transporting(), tunnelEstablishmentDone(), and tunnelStartShoveling().
◆ preReadClientData
SBuf TunnelStateData::preReadClientData |
Definition at line 187 of file tunnel.cc.
Referenced by copyClientBytes(), and tunnelStartShoveling().
◆ preReadServerData
SBuf TunnelStateData::preReadServerData |
Definition at line 188 of file tunnel.cc.
Referenced by copyServerBytes(), switchToTunnel(), and tunnelEstablishmentDone().
◆ request
HttpRequest::Pointer TunnelStateData::request |
Definition at line 96 of file tunnel.cc.
Referenced by advanceDestination(), checkRetry(), clientExpectsConnectResponse(), connectDone(), establishTunnelThruProxy(), getHost(), noteConnection(), noteDestinationsEnd(), noteSecurityPeerConnectorAnswer(), readServer(), retryOrBail(), secureConnectionToPeer(), startConnecting(), switchToTunnel(), syncHierNote(), tunnelEstablishmentDone(), tunnelStart(), TunnelStateData(), usePinned(), and writeServerDone().
◆ savedError
|
private |
Definition at line 261 of file tunnel.cc.
Referenced by advanceDestination(), noteDestinationsEnd(), retryOrBail(), saveError(), sendError(), startConnecting(), and ~TunnelStateData().
◆ server
Connection TunnelStateData::server |
Definition at line 184 of file tunnel.cc.
Referenced by clientClosed(), closeConnections(), closePendingConnection(), commitToServer(), connectDone(), copyClientBytes(), copyServerBytes(), establishTunnelThruProxy(), getHost(), noConnections(), readClient(), readServer(), retryOrBail(), serverClosed(), switchToTunnel(), tunnelDelayedServerRead(), tunnelErrorComplete(), tunnelEstablishmentDone(), tunnelStart(), tunnelStartShoveling(), TunnelStateData(), writeClientDone(), WriteServerDone(), and writeServerDone().
◆ startTime
time_t TunnelStateData::startTime |
Definition at line 189 of file tunnel.cc.
Referenced by checkRetry(), and startConnecting().
◆ status_ptr
int* TunnelStateData::status_ptr |
Definition at line 185 of file tunnel.cc.
Referenced by notePeerReadyToShovel(), retryOrBail(), sendError(), tunnelConnectedWriteDone(), tunnelEstablishmentDone(), tunnelStartShoveling(), and TunnelStateData().
◆ subscribed
|
inherited |
Definition at line 46 of file PeerSelectState.h.
Referenced by commitToServer(), FwdState::noteDestinationsEnd(), noteDestinationsEnd(), FwdState::reforward(), retryOrBail(), sendError(), PeerSelectionInitiator::startSelectingDestinations(), FwdState::stopAndDestroy(), and FwdState::useDestinations().
◆ transportWait
JobWait<HappyConnOpener> TunnelStateData::transportWait |
Definition at line 205 of file tunnel.cc.
Referenced by cancelStep(), noteConnection(), noteDestination(), noteDestinationsEnd(), notifyConnOpener(), startConnecting(), and tunnelStartShoveling().
◆ url
char* TunnelStateData::url |
Definition at line 94 of file tunnel.cc.
Referenced by tunnelStart(), TunnelStateData(), and ~TunnelStateData().
The documentation for this class was generated from the following file:
- src/tunnel.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products