#include <client_side.h>
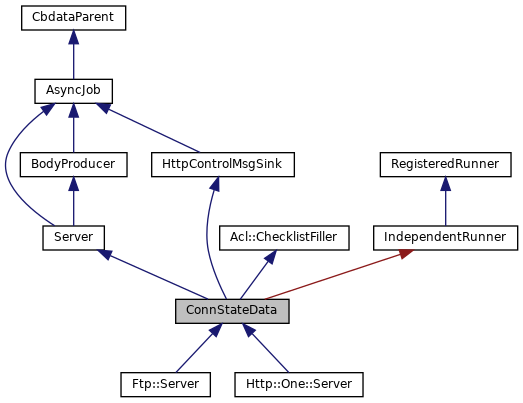
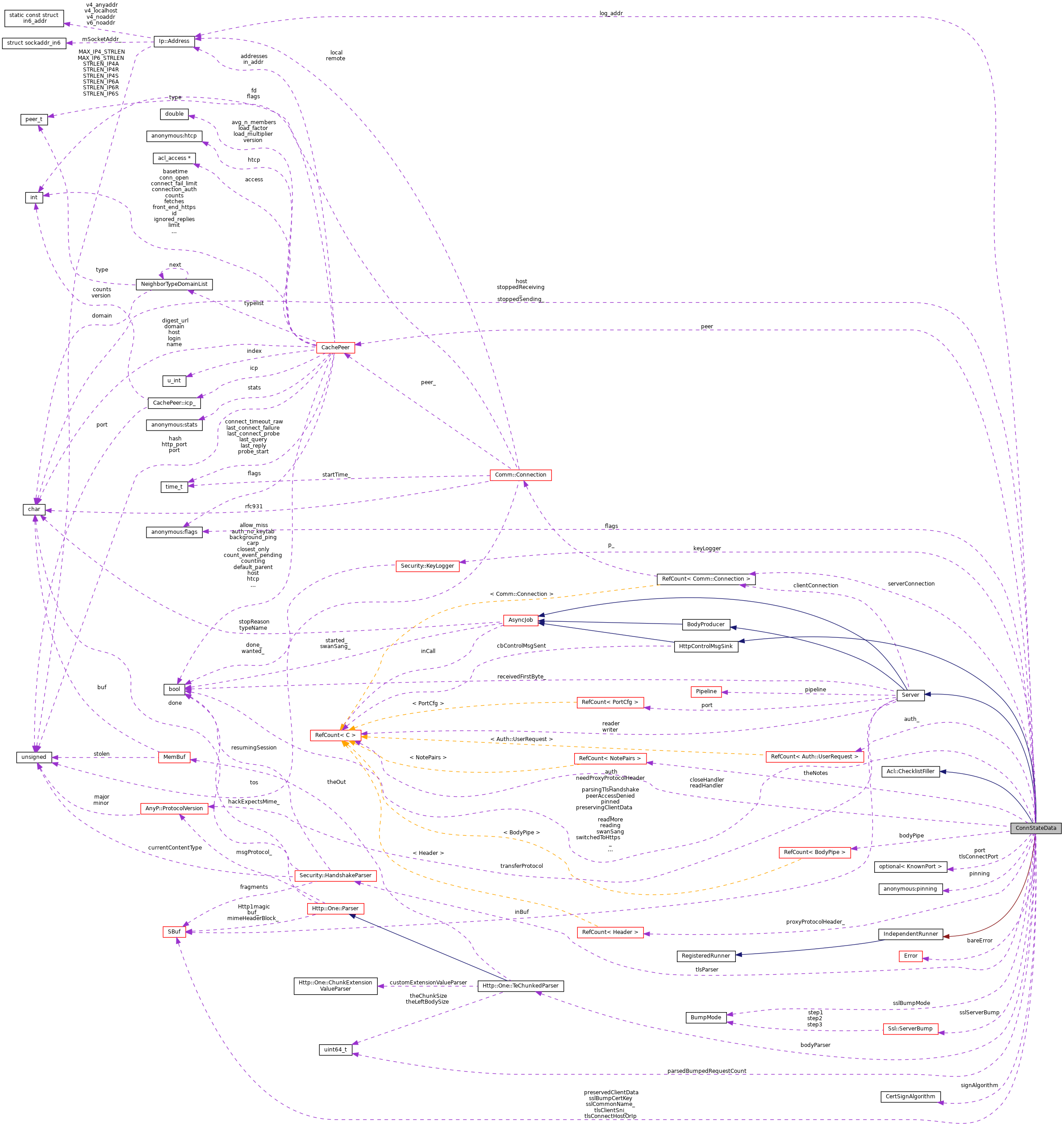
Classes | |
class | PinnedIdleContext |
parameters for the async notePinnedConnectionBecameIdle() call More... | |
class | ServerConnectionContext |
noteTakeServerConnectionControl() callback parameter More... | |
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
Public Member Functions | |
ConnStateData (const MasterXactionPointer &xact) | |
~ConnStateData () override | |
void | receivedFirstByte () override |
Update flags and timeout after the first byte received. More... | |
bool | handleReadData () override |
void | afterClientRead () override |
processing to be done after a Comm::Read() More... | |
void | afterClientWrite (size_t) override |
processing to sync state after a Comm::Write() More... | |
void | sendControlMsg (HttpControlMsg) override |
called to send the 1xx message and notify the Source More... | |
void | doneWithControlMsg () override |
void | readNextRequest () |
Traffic parsing. More... | |
void | kick () |
try to make progress on a transaction or read more I/O More... | |
bool | isOpen () const |
int64_t | mayNeedToReadMoreBody () const |
const Auth::UserRequest::Pointer & | getAuth () const |
void | setAuth (const Auth::UserRequest::Pointer &aur, const char *cause) |
bool | transparent () const |
const char * | stoppedReceiving () const |
true if we stopped receiving the request More... | |
const char * | stoppedSending () const |
true if we stopped sending the response More... | |
void | stopReceiving (const char *error) |
note request receiving error and close as soon as we write the response More... | |
void | stopSending (const char *error) |
note response sending error and close as soon as we read the request More... | |
void | resetReadTimeout (time_t timeout) |
(re)sets timeout for receiving more bytes from the client More... | |
void | extendLifetime () |
(re)sets client_lifetime timeout More... | |
void | expectNoForwarding () |
cleans up virgin request [body] forwarding state More... | |
BodyPipe::Pointer | expectRequestBody (int64_t size) |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override=0 |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override=0 |
bool | handleRequestBodyData () |
void | notePinnedConnectionBecameIdle (PinnedIdleContext pic) |
Called when a pinned connection becomes available for forwarding the next request. More... | |
void | pinBusyConnection (const Comm::ConnectionPointer &pinServerConn, const HttpRequest::Pointer &request) |
void | unpinConnection (const bool andClose) |
Undo pinConnection() and, optionally, close the pinned connection. More... | |
CachePeer * | pinnedPeer () const |
bool | pinnedAuth () const |
virtual void | notePeerConnection (Comm::ConnectionPointer) |
called just before a FwdState-dispatched job starts using connection More... | |
virtual void | clientPinnedConnectionClosed (const CommCloseCbParams &io) |
Our close handler called by Comm when the pinned connection is closed. More... | |
virtual void | noteTakeServerConnectionControl (ServerConnectionContext) |
void | clientReadFtpData (const CommIoCbParams &io) |
void | connStateClosed (const CommCloseCbParams &io) |
void | requestTimeout (const CommTimeoutCbParams ¶ms) |
void | lifetimeTimeout (const CommTimeoutCbParams ¶ms) |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
void | callException (const std::exception &) override |
called when the job throws during an async call More... | |
void | quitAfterError (HttpRequest *request) |
void | stopPinnedConnectionMonitoring () |
The caller assumes responsibility for connection closure detection. More... | |
Security::IoResult | acceptTls () |
void | postHttpsAccept () |
the second part of old httpsAccept, waiting for future HttpsServer home More... | |
void | startPeekAndSplice () |
Initializes and starts a peek-and-splice negotiation with the SSL client. More... | |
void | doPeekAndSpliceStep () |
void | httpsPeeked (PinnedIdleContext pic) |
called by FwdState when it is done bumping the server More... | |
bool | splice () |
Splice a bumped client connection on peek-and-splice mode. More... | |
void | getSslContextStart () |
Start to create dynamic Security::ContextPointer for host or uses static port SSL context. More... | |
void | getSslContextDone (Security::ContextPointer &) |
finish configuring the newly created SSL context" More... | |
void | sslCrtdHandleReply (const Helper::Reply &reply) |
Process response from ssl_crtd. More... | |
void | switchToHttps (ClientHttpRequest *, Ssl::BumpMode bumpServerMode) |
void | parseTlsHandshake () |
bool | switchedToHttps () const |
Ssl::ServerBump * | serverBump () |
void | setServerBump (Ssl::ServerBump *srvBump) |
const SBuf & | sslCommonName () const |
void | resetSslCommonName (const char *name) |
const SBuf & | tlsClientSni () const |
void | buildSslCertGenerationParams (Ssl::CertificateProperties &certProperties) |
bool | serveDelayedError (Http::Stream *) |
char * | prepareTlsSwitchingURL (const Http1::RequestParserPointer &hp) |
void | add (const Http::StreamPointer &context) |
registers a newly created stream More... | |
virtual bool | writeControlMsgAndCall (HttpReply *rep, AsyncCall::Pointer &call)=0 |
handle a control message received by context from a peer and call back More... | |
virtual void | handleReply (HttpReply *header, StoreIOBuffer receivedData)=0 |
void | consumeInput (const size_t byteCount) |
remove no longer needed leading bytes from the input buffer More... | |
Http::Stream * | abortRequestParsing (const char *const errUri) |
stop parsing the request and create context for relaying error info More... | |
bool | fakeAConnectRequest (const char *reason, const SBuf &payload) |
bool | initiateTunneledRequest (HttpRequest::Pointer const &cause, const char *reason, const SBuf &payload) |
generates and sends to tunnel.cc a fake request with a given payload More... | |
bool | shouldPreserveClientData () const |
ClientHttpRequest * | buildFakeRequest (SBuf &useHost, AnyP::KnownPort usePort, const SBuf &payload) |
build a fake http request More... | |
void | startShutdown () override |
void | endingShutdown () override |
NotePairs::Pointer | notes () |
bool | hasNotes () const |
const ProxyProtocol::HeaderPointer & | proxyProtocolHeader () const |
void | updateError (const Error &) |
if necessary, stores new error information (if any) More... | |
void | updateError (const err_type c, const ErrorDetailPointer &d) |
emplacement/convenience wrapper for updateError(const Error &) More... | |
void | fillChecklist (ACLFilledChecklist &) const override |
configure the given checklist (to reflect the current transaction state) More... | |
void | fillConnectionLevelDetails (ACLFilledChecklist &) const |
void | readSomeData () |
maybe grow the inBuf and schedule Comm::Read() More... | |
bool | reading () const |
whether Comm::Read() is scheduled More... | |
void | stopReading () |
cancels Comm::Read() if it is scheduled More... | |
virtual void | writeSomeData () |
maybe find some data to send and schedule a Comm::Write() More... | |
void | write (MemBuf *mb) |
schedule some data for a Comm::Write() More... | |
void | write (char *buf, int len) |
schedule some data for a Comm::Write() More... | |
bool | writing () const |
whether Comm::Write() is scheduled More... | |
void | maybeMakeSpaceAvailable () |
grows the available read buffer space (if possible) More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
void | wroteControlMsg (const CommIoCbParams &) |
callback to handle Comm::Write completion More... | |
Static Public Member Functions | |
static Comm::ConnectionPointer | BorrowPinnedConnection (HttpRequest *, const AccessLogEntryPointer &) |
static void | sslCrtdHandleReplyWrapper (void *data, const Helper::Reply &reply) |
Callback function. It is called when squid receive message from ssl_crtd. More... | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
Http1::TeChunkedParser * | bodyParser = nullptr |
parses HTTP/1.1 chunked request body More... | |
Ip::Address | log_addr |
struct { | |
bool readMore = true | |
needs comm_read (for this request or new requests) More... | |
bool swanSang = false | |
} | flags |
struct { | |
Comm::ConnectionPointer serverConnection | |
char * host = nullptr | |
host name of pinned connection More... | |
AnyP::Port port | |
destination port of the request that caused serverConnection More... | |
bool pinned = false | |
this connection was pinned More... | |
bool auth = false | |
pinned for www authentication More... | |
bool reading = false | |
we are monitoring for peer connection closure More... | |
bool zeroReply = false | |
server closed w/o response (ERR_ZERO_SIZE_OBJECT) More... | |
bool peerAccessDenied = false | |
cache_peer_access denied pinned connection reuse More... | |
CachePeer * peer = nullptr | |
CachePeer the connection goes via. More... | |
AsyncCall::Pointer readHandler | |
detects serverConnection closure More... | |
AsyncCall::Pointer closeHandler | |
The close handler for pinned server side connection. More... | |
} | pinning |
Ssl::BumpMode | sslBumpMode = Ssl::bumpEnd |
ssl_bump decision (Ssl::bumpEnd if n/a). More... | |
Security::HandshakeParser | tlsParser |
SBuf | preservedClientData |
Error | bareError |
a problem that occurred without a request (e.g., while parsing headers) More... | |
Security::KeyLogger | keyLogger |
managers logging of the being-accepted TLS connection secrets More... | |
Comm::ConnectionPointer | clientConnection |
AnyP::ProtocolVersion | transferProtocol |
SBuf | inBuf |
read I/O buffer for the client connection More... | |
bool | receivedFirstByte_ |
true if at least one byte received on this connection More... | |
Pipeline | pipeline |
set of requests waiting to be serviced More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
AsyncCall::Pointer | cbControlMsgSent |
Call to schedule when the control msg has been sent. More... | |
Protected Member Functions | |
void | startDechunkingRequest () |
initialize dechunking state More... | |
void | finishDechunkingRequest (bool withSuccess) |
put parsed content into input buffer and clean up More... | |
void | abortChunkedRequestBody (const err_type error) |
quit on errors related to chunked request body handling More... | |
err_type | handleChunkedRequestBody () |
parses available chunked encoded body bytes, checks size, returns errors More... | |
Comm::ConnectionPointer | borrowPinnedConnection (HttpRequest *, const AccessLogEntryPointer &) |
ConnStateData-specific part of BorrowPinnedConnection() More... | |
void | startPinnedConnectionMonitoring () |
void | clientPinnedConnectionRead (const CommIoCbParams &io) |
bool | handleIdleClientPinnedTlsRead () |
Http::Stream * | parseHttpRequest (const Http1::RequestParserPointer &) |
virtual Http::Stream * | parseOneRequest ()=0 |
virtual void | processParsedRequest (Http::StreamPointer &)=0 |
start processing a freshly parsed request More... | |
virtual int | pipelinePrefetchMax () const |
returning N allows a pipeline of 1+N requests (see pipeline_prefetch) More... | |
virtual time_t | idleTimeout () const =0 |
timeout to use when waiting for the next request More... | |
void | whenClientIpKnown () |
bool | tunnelOnError (const err_type) |
initiate tunneling if possible or return false otherwise More... | |
void | doClientRead (const CommIoCbParams &io) |
void | clientWriteDone (const CommIoCbParams &io) |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
BodyPipe::Pointer | bodyPipe |
set when we are reading request body More... | |
bool | preservingClientData_ = false |
whether preservedClientData is valid and should be kept up to date More... | |
AsyncCall::Pointer | reader |
set when we are reading More... | |
AsyncCall::Pointer | writer |
set when we are writing More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Types | |
typedef void(RegisteredRunner::* | Method) () |
a pointer to one of the above notification methods More... | |
Private Member Functions | |
void | terminateAll (const Error &, const LogTagsErrors &) override |
abort any pending transactions and prevent new ones (by closing) More... | |
bool | shouldCloseOnEof () const override |
whether to stop serving our client after reading EOF on its connection More... | |
void | checkLogging () |
log the last (attempt at) transaction if nobody else did More... | |
void | parseRequests () |
void | clientAfterReadingRequests () |
bool | concurrentRequestQueueFilled () const |
void | pinConnection (const Comm::ConnectionPointer &pinServerConn, const HttpRequest &request) |
Forward future client requests using the given server connection. More... | |
bool | proxyProtocolValidateClient () |
bool | parseProxyProtocolHeader () |
bool | proxyProtocolError (const char *reason) |
Security::ContextPointer | getTlsContextFromCache (const SBuf &cacheKey, const Ssl::CertificateProperties &certProperties) |
void | storeTlsContextToCache (const SBuf &cacheKey, Security::ContextPointer &ctx) |
void | handleSslBumpHandshakeError (const Security::IoResult &) |
process a problematic Security::Accept() result on the SslBump code path More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
void | registerRunner () |
void | unregisterRunner () |
unregisters self; safe to call multiple times More... | |
virtual void | bootstrapConfig () |
virtual void | finalizeConfig () |
virtual void | claimMemoryNeeds () |
virtual void | useConfig () |
virtual void | startReconfigure () |
virtual void | syncConfig () |
virtual void | finishShutdown () |
Meant for cleanup of services needed by the already destroyed objects. More... | |
Private Attributes | |
bool | needProxyProtocolHeader_ = false |
whether PROXY protocol header is still expected More... | |
ProxyProtocol::HeaderPointer | proxyProtocolHeader_ |
the parsed PROXY protocol header More... | |
Auth::UserRequest::Pointer | auth_ |
some user details that can be used to perform authentication on this connection More... | |
bool | switchedToHttps_ = false |
bool | parsingTlsHandshake = false |
uint64_t | parsedBumpedRequestCount = 0 |
The number of parsed HTTP requests headers on a bumped client connection. More... | |
SBuf | tlsConnectHostOrIp |
The TLS server host name appears in CONNECT request or the server ip address for the intercepted requests. More... | |
AnyP::Port | tlsConnectPort |
The TLS server port number as passed in the CONNECT request. More... | |
SBuf | sslCommonName_ |
CN name for SSL certificate generation. More... | |
SBuf | tlsClientSni_ |
TLS client delivered SNI value. Empty string if none has been received. More... | |
SBuf | sslBumpCertKey |
Key to use to store/retrieve generated certificate. More... | |
Ssl::ServerBump * | sslServerBump = nullptr |
HTTPS server cert. fetching state for bump-ssl-server-first. More... | |
Ssl::CertSignAlgorithm | signAlgorithm = Ssl::algSignTrusted |
The signing algorithm to use. More... | |
const char * | stoppedSending_ = nullptr |
the reason why we no longer write the response or nil More... | |
const char * | stoppedReceiving_ = nullptr |
the reason why we no longer read the request or nil More... | |
NotePairs::Pointer | theNotes |
Detailed Description
Legacy Server code managing a connection to a client.
NP: presents AsyncJob API but does not operate autonomously as a Job. So Must() is not safe to use.
Multiple requests (up to pipeline_prefetch) can be pipelined. This object is responsible for managing which one is currently being fulfilled and what happens to the queue if the current one causes the client connection to be closed early.
Act as a manager for the client connection and passes data in buffer to a Parser relevant to the state (message headers vs body) that is being processed.
Performs HTTP message processing to kick off the actual HTTP request handling objects (Http::Stream, ClientHttpRequest, HttpRequest).
Performs SSL-Bump processing for switching between HTTP and HTTPS protocols.
To terminate a ConnStateData close() the client Comm::Connection it is managing, or for graceful half-close use the stopReceiving() or stopSending() methods.
Definition at line 79 of file client_side.h.
Member Typedef Documentation
◆ Method
|
inherited |
Definition at line 94 of file RunnersRegistry.h.
◆ Pointer [1/2]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [2/2]
|
inherited |
Definition at line 25 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ ConnStateData()
|
explicit |
Definition at line 2131 of file client_side.cc.
References SquidConfig::Addrs, Ip::Address::applyClientMask(), SquidConfig::client_netmask, Config, log_addr, IndependentRunner::registerRunner(), Comm::Connection::remote, and MasterXaction::tcpClient.
◆ ~ConnStateData()
|
override |
Definition at line 671 of file client_side.cc.
References bodyParser, bodyPipe, Server::clientConnection, DBG_IMPORTANT, debugs, flags, isOpen(), sslServerBump, and BodyProducer::stopProducingFor().
Member Function Documentation
◆ abortChunkedRequestBody()
|
protected |
Definition at line 2054 of file client_side.cc.
References assert, Server::clientConnection, comm_reset_close(), debugs, ERR_TOO_BIG, error(), finishDechunkingRequest(), flags, Pipeline::front(), clientReplyContext::http, Server::inBuf, NULL, Server::pipeline, ClientHttpRequest::request, Http::scContentTooLarge, clientReplyContext::setReplyToError(), and ClientHttpRequest::uri.
Referenced by handleRequestBodyData().
◆ abortRequestParsing()
Http::Stream * ConnStateData::abortRequestParsing | ( | const char *const | errUri | ) |
Definition at line 1022 of file client_side.cc.
References ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), StoreIOBuffer::data, HTTP_REQBUF_SZ, Server::inBuf, SBuf::length(), StoreIOBuffer::length, ClientHttpRequest::req_sz, and ClientHttpRequest::setErrorUri().
Referenced by parseHttpRequest().
◆ acceptTls()
Security::IoResult ConnStateData::acceptTls | ( | ) |
Starts or resumes accepting a TLS connection. TODO: Make this helper method protected after converting clientNegotiateSSL() into a method.
Definition at line 2249 of file client_side.cc.
References Security::Accept(), assert, Security::KeyLogger::checkpoint(), Server::clientConnection, Comm::Connection::fd, fd_table, and keyLogger.
Referenced by startPeekAndSplice().
◆ add()
void ConnStateData::add | ( | const Http::StreamPointer & | context | ) |
Definition at line 1722 of file client_side.cc.
References Pipeline::add(), assert, bareError, Error::clear(), Pipeline::count(), debugs, Pipeline::nrequests, and Server::pipeline.
◆ afterClientRead()
|
overridevirtual |
Implements Server.
Definition at line 1930 of file client_side.cc.
References clientAfterReadingRequests(), Server::clientConnection, Pipeline::empty(), Comm::Connection::fd, fd_note(), isOpen(), parseRequests(), parseTlsHandshake(), parsingTlsHandshake, and Server::pipeline.
◆ afterClientWrite()
|
overridevirtual |
Reimplemented from Server.
Definition at line 1007 of file client_side.cc.
References StatCounters::client_http, Pipeline::empty(), Pipeline::front(), StatCounters::hit_kbytes_out, StatCounters::kbytes_out, Server::pipeline, size, and statCounter.
◆ bootstrapConfig()
|
inlinevirtualinherited |
Called right before parsing squid.conf. Meant for initializing/preparing configuration parsing facilities.
Reimplemented in NtlmAuthRr.
Definition at line 46 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ BorrowPinnedConnection()
|
static |
- Returns
- validated pinned to-server connection, stopping its monitoring
- Exceptions
-
a newly allocated ErrorState if validation fails
Definition at line 3905 of file client_side.cc.
References ERR_CANNOT_FORWARD, ErrorState::NewForwarding(), and HttpRequest::pinnedConnection().
Referenced by FwdState::usePinned(), and TunnelStateData::usePinned().
◆ borrowPinnedConnection()
|
protected |
Definition at line 3874 of file client_side.cc.
References cbdataReferenceValid(), debugs, ERR_CANNOT_FORWARD, ERR_ZERO_SIZE_OBJECT, AnyP::Uri::host(), Comm::IsConnOpen(), Must, ErrorState::NewForwarding(), pinning, AnyP::Uri::port(), stopPinnedConnectionMonitoring(), unpinConnection(), and HttpRequest::url.
◆ buildFakeRequest()
ClientHttpRequest * ConnStateData::buildFakeRequest | ( | SBuf & | useHost, |
AnyP::KnownPort | usePort, | ||
const SBuf & | payload | ||
) |
Definition at line 3194 of file client_side.cc.
References ClientHttpRequest::al, HttpRequest::auth_user_request, SBuf::c_str(), ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), StoreIOBuffer::data, HttpRequest::effectiveRequestUri(), extendLifetime(), flags, Http::Stream::flags, getAuth(), RefCount< C >::getRaw(), Http::Message::header, AnyP::Uri::host(), Http::HOST, HTTP_REQBUF_SZ, Server::inBuf, ClientHttpRequest::initRequest(), StoreIOBuffer::length, MasterXaction::MakePortful(), HttpRequest::manager(), Http::Stream::mayUseConnection(), HttpRequest::method, Http::METHOD_CONNECT, Http::Stream::parsed_ok, AnyP::Uri::port(), port, AnyP::PROTO_AUTHORITY_FORM, AnyP::PROTO_HTTPS, HttpHeader::putStr(), Http::Stream::registerWithConn(), Http::Stream::reqbuf, SBufToCstring(), AnyP::Uri::setScheme(), Http::Message::sources, Http::Message::srcHttp, Http::Message::srcHttps, switchedToHttps(), ClientHttpRequest::uri, and HttpRequest::url.
Referenced by fakeAConnectRequest(), and initiateTunneledRequest().
◆ buildSslCertGenerationParams()
void ConnStateData::buildSslCertGenerationParams | ( | Ssl::CertificateProperties & | certProperties | ) |
Fill the certAdaptParams with the required data for certificate adaptation and create the key for storing/retrieve the certificate to/from the cache
Definition at line 2600 of file client_side.cc.
References Ssl::algSetCommonName, Ssl::algSetValidAfter, Ssl::algSetValidBefore, Ssl::algSignEnd, Ssl::algSignTrusted, Ssl::algSignUntrusted, Acl::Answer::allowed(), assert, SBuf::c_str(), SquidConfig::cert_adapt, SquidConfig::cert_sign, Ssl::CertAdaptAlgorithmStr, Ssl::CertificateProperties::commonName, Config, Ssl::ServerBump::connectedOk(), debugs, Ssl::DefaultSignHash, ACLChecklist::fastCheck(), fillChecklist(), Security::LockingPointer< T, UnLocker, Locker >::get(), RefCount< C >::getRaw(), SBuf::isEmpty(), Ssl::CertificateProperties::mimicCert, sslproxy_cert_sign::next, sslproxy_cert_adapt::next, port, Ssl::ServerBump::request, Security::LockingPointer< T, UnLocker, Locker >::resetAndLock(), Ssl::ServerBump::serverCert, Ssl::CertificateProperties::setCommonName, Ssl::CertificateProperties::setValidAfter, Ssl::CertificateProperties::setValidBefore, signAlgorithm, Ssl::CertificateProperties::signAlgorithm, Ssl::CertificateProperties::signHash, Ssl::CertificateProperties::signWithPkey, Ssl::CertificateProperties::signWithX509, SquidConfig::ssl_client, sslCommonName_, sslServerBump, and tlsConnectHostOrIp.
Referenced by getSslContextStart().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtual |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Server.
Definition at line 639 of file client_side.cc.
References AsyncJob::callException(), ERR_GATEWAY_FAILURE, Here, and updateError().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ checkLogging()
|
private |
Definition at line 3991 of file client_side.cc.
References assert, bareError, Pipeline::empty(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), Pipeline::nrequests, Server::pipeline, ClientHttpRequest::req_sz, ClientHttpRequest::setErrorUri(), and ClientHttpRequest::updateError().
Referenced by swanSong().
◆ claimMemoryNeeds()
|
inlinevirtualinherited |
Called after finalizeConfig(). Meant for announcing memory reservations before memory is allocated.
Reimplemented in IpcIoRr, and MemStoreRr.
Definition at line 55 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
◆ clientAfterReadingRequests()
|
private |
Definition at line 1441 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), commIsHalfClosed(), debugs, Comm::Connection::fd, flags, mayNeedToReadMoreBody(), and Server::readSomeData().
Referenced by afterClientRead().
◆ clientPinnedConnectionClosed()
|
virtual |
Reimplemented in Ftp::Server.
Definition at line 3697 of file client_side.cc.
References assert, Server::clientConnection, Comm::Connection::close(), CommCommonCbParams::conn, debugs, pinning, and unpinConnection().
Referenced by Ftp::Server::clientPinnedConnectionClosed(), and pinConnection().
◆ clientPinnedConnectionRead()
|
protected |
Our read handler called by Comm when the server either closes an idle pinned connection or perhaps unexpectedly sends something on that idle (from Squid p.o.v.) connection.
Definition at line 3845 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), CommCommonCbParams::conn, debugs, Pipeline::empty(), Comm::ERR_CLOSING, CommCommonCbParams::flag, handleIdleClientPinnedTlsRead(), Must, pinning, Server::pipeline, and CommIoCbParams::size.
Referenced by startPinnedConnectionMonitoring().
◆ clientReadFtpData()
void ConnStateData::clientReadFtpData | ( | const CommIoCbParams & | io | ) |
◆ clientWriteDone()
|
protectedinherited |
callback handling the Comm::Write completion
Will call afterClientWrite(size_t) to sync the I/O state. Then writeSomeData() to initiate any followup writes that could be immediately done.
Definition at line 193 of file Server.cc.
References Server::afterClientWrite(), Server::clientConnection, CommCommonCbParams::conn, debugs, Comm::ERR_CLOSING, Comm::Connection::fd, CommCommonCbParams::flag, Pipeline::front(), Comm::IsConnOpen(), Must, Server::pipeline, CommIoCbParams::size, Server::writer, and Server::writeSomeData().
Referenced by Server::write().
◆ concurrentRequestQueueFilled()
|
private |
Limit the number of concurrent requests.
- Returns
- true when there are available position(s) in the pipeline queue for another request.
- false when the pipeline queue is full or disabled.
Definition at line 1750 of file client_side.cc.
References Ssl::bumpSplice, Server::clientConnection, Pipeline::count(), debugs, Server::pipeline, pipelinePrefetchMax(), sslBumpMode, and transparent().
Referenced by parseRequests().
◆ connStateClosed()
void ConnStateData::connStateClosed | ( | const CommCloseCbParams & | io | ) |
Definition at line 507 of file client_side.cc.
References Server::clientConnection, AsyncJob::deleteThis(), and Comm::Connection::noteClosure().
Referenced by start().
◆ consumeInput()
void ConnStateData::consumeInput | ( | const size_t | byteCount | ) |
Definition at line 1433 of file client_side.cc.
References assert, SBuf::consume(), debugs, Server::inBuf, and SBuf::length().
Referenced by handleRequestBodyData(), and parseHttpRequest().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by connStateClosed(), and AsyncJob::deleteThis().
◆ doClientRead()
|
protectedinherited |
Definition at line 106 of file Server.cc.
References LogTagsErrors::aborted, Server::afterClientRead(), assert, StatCounters::client_http, Server::clientConnection, commMarkHalfClosed(), CommCommonCbParams::conn, debugs, Comm::ENDFILE, ERR_CLIENT_GONE, Comm::ERR_CLOSING, Helper::Error, Comm::Connection::fd, fd_note(), fd_table, CommCommonCbParams::flag, Server::handleReadData(), Server::inBuf, Comm::INPROGRESS, Comm::IsConnOpen(), SBuf::isEmpty(), StatCounters::kbytes_in, Server::maybeMakeSpaceAvailable(), Must, SysErrorDetail::NewIfAny(), Comm::OK, Server::reader, Server::reading(), Comm::ReadNow(), Server::readSomeData(), Server::receivedFirstByte(), Server::receivedFirstByte_, Server::shouldCloseOnEof(), CommIoCbParams::size, statCounter, Server::terminateAll(), LogTagsErrors::timedout, CommCommonCbParams::xerrno, and xstrerr().
Referenced by Server::readSomeData().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
inlineoverridevirtual |
Reimplemented from AsyncJob.
Definition at line 239 of file client_side.h.
References AsyncJob::doneAll().
◆ doneWithControlMsg()
|
overridevirtual |
Reimplemented from HttpControlMsgSink.
Definition at line 3685 of file client_side.cc.
References Server::clientConnection, ClientSocketContextPushDeferredIfNeeded(), debugs, HttpControlMsgSink::doneWithControlMsg(), Pipeline::front(), and Server::pipeline.
Referenced by sendControlMsg().
◆ doPeekAndSpliceStep()
void ConnStateData::doPeekAndSpliceStep | ( | ) |
Called when a peek-and-splice step finished. For example after server SSL certificates received and fake server SSL certificates generated
Definition at line 3085 of file client_side.cc.
References assert, BIO_get_data(), Server::clientConnection, clientNegotiateSSL(), COMM_SELECT_WRITE, debugs, Comm::Connection::fd, fd_table, Ssl::ClientBio::hold(), Comm::SetSelect(), and switchedToHttps_.
Referenced by getSslContextStart(), and sslCrtdHandleReply().
◆ endingShutdown()
|
overridevirtual |
Called after shutdown_lifetime grace period ends and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented from RegisteredRunner.
Definition at line 1049 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), and Comm::IsConnOpen().
Referenced by startShutdown().
◆ expectNoForwarding()
void ConnStateData::expectNoForwarding | ( | ) |
Definition at line 3608 of file client_side.cc.
References bodyPipe, debugs, BodyPipe::expectNoConsumption(), and BodyPipe::status().
Referenced by ClientHttpRequest::calloutsError().
◆ expectRequestBody()
BodyPipe::Pointer ConnStateData::expectRequestBody | ( | int64_t | size | ) |
Definition at line 3559 of file client_side.cc.
References bodyPipe, BodyPipe::setBodySize(), size, and startDechunkingRequest().
◆ extendLifetime()
void ConnStateData::extendLifetime | ( | ) |
Definition at line 606 of file client_side.cc.
References Server::clientConnection, commSetConnTimeout(), Config, JobCallback, SquidConfig::lifetime, lifetimeTimeout(), and SquidConfig::Timeout.
Referenced by buildFakeRequest(), and parseRequests().
◆ fakeAConnectRequest()
bool ConnStateData::fakeAConnectRequest | ( | const char * | reason, |
const SBuf & | payload | ||
) |
generate a fake CONNECT request with the given payload at the beginning of the client I/O buffer
Definition at line 3165 of file client_side.cc.
References assert, SBuf::assign(), buildFakeRequest(), ClientHttpRequest::calloutContext, Server::clientConnection, clientProcessRequestFinished(), debugs, ClientHttpRequest::doCallouts(), SBuf::isEmpty(), Comm::Connection::local, MAX_IPSTRLEN, Ip::Address::port(), ClientHttpRequest::request, tlsClientSni_, Ip::Address::toHostStr(), and transparent().
Referenced by httpsSslBumpAccessCheckDone(), and splice().
◆ fillChecklist()
|
overridevirtual |
Implements Acl::ChecklistFiller.
Definition at line 3515 of file client_side.cc.
References clientAclChecklistFill(), Pipeline::front(), Server::pipeline, and ACLFilledChecklist::setConn().
Referenced by buildSslCertGenerationParams(), postHttpsAccept(), proxyProtocolValidateClient(), startPeekAndSplice(), tunnelOnError(), and whenClientIpKnown().
◆ fillConnectionLevelDetails()
void ConnStateData::fillConnectionLevelDetails | ( | ACLFilledChecklist & | checklist | ) | const |
fillChecklist() obligations not fulfilled by the front request TODO: This is a temporary ACLFilledChecklist::setConn() callback to allow filling checklist using our non-public information sources. It should be removed as unnecessary by making ACLs extract the information they need from the ACLFilledChecklist::conn() without filling/copying.
Definition at line 3532 of file client_side.cc.
References assert, Server::clientConnection, ACLFilledChecklist::conn(), Comm::Connection::local, ACLFilledChecklist::my_addr, Comm::Connection::remote, ACLFilledChecklist::request, ACLFilledChecklist::rfc931, Comm::Connection::rfc931, ACLFilledChecklist::setIdent(), ACLFilledChecklist::src_addr, ACLFilledChecklist::sslErrors, Ssl::ServerBump::sslErrors(), and sslServerBump.
Referenced by ACLFilledChecklist::setConn().
◆ finalizeConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf. Meant for setting configuration options that depend on other configuration options and were not explicitly configured.
Reimplemented in sslBumpCfgRr, and MemStoreRr.
Definition at line 51 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finishDechunkingRequest()
|
protected |
Definition at line 3628 of file client_side.cc.
References bodyParser, bodyPipe, BodyPipe::bodySize(), BodyPipe::bodySizeKnown(), debugs, Pipeline::front(), Must, Server::pipeline, BodyPipe::status(), and BodyProducer::stopProducingFor().
Referenced by abortChunkedRequestBody(), and handleChunkedRequestBody().
◆ finishShutdown()
|
inlinevirtualinherited |
Definition at line 91 of file RunnersRegistry.h.
Referenced by RunRegistered(), SquidShutdown(), TestRock::tearDown(), and watch_child().
◆ getAuth()
|
inline |
Fetch the user details for connection based authentication NOTE: this is ONLY connection based because NTLM and Negotiate is against HTTP spec.
Definition at line 123 of file client_side.h.
References auth_.
Referenced by AuthenticateAcl(), buildFakeRequest(), ClientHttpRequest::calloutsError(), ClientRequestContext::clientAccessCheckDone(), constructHelperQuery(), and ClientRequestContext::hostHeaderVerifyFailed().
◆ getSslContextDone()
void ConnStateData::getSslContextDone | ( | Security::ContextPointer & | ctx | ) |
Definition at line 2764 of file client_side.cc.
References BIO_get_data(), SBuf::clear(), Server::clientConnection, clientNegotiateSSL(), Comm::Connection::close(), Config, DBG_IMPORTANT, debugs, Comm::Connection::fd, fd_table, httpsCreate(), Server::inBuf, port, Comm::Connection::remote, SquidConfig::request, resetReadTimeout(), Ssl::ClientBio::setReadBufData(), switchedToHttps_, SquidConfig::Timeout, and tlsConnectHostOrIp.
Referenced by getSslContextStart(), and sslCrtdHandleReply().
◆ getSslContextStart()
void ConnStateData::getSslContextStart | ( | ) |
Definition at line 2704 of file client_side.cc.
References Ssl::ServerBump::act, Ssl::algSignTrusted, assert, buildSslCertGenerationParams(), Ssl::bumpPeek, Ssl::bumpStare, SBuf::clear(), Server::clientConnection, Ssl::CrtdMessage::code_new_certificate, Ssl::CertificateProperties::commonName, Ssl::CrtdMessage::compose(), Ssl::CrtdMessage::composeRequest(), Ssl::configureSSL(), Ssl::configureUnconfiguredSslContext(), DBG_IMPORTANT, debugs, doPeekAndSpliceStep(), Comm::Connection::fd, fd_table, Ssl::GenerateSslContext(), Security::GetFrom(), getSslContextDone(), getTlsContextFromCache(), Ssl::InRamCertificateDbKey(), SBuf::isEmpty(), Store::nil, port, Ssl::CrtdMessage::REQUEST, Ssl::CrtdMessage::setCode(), signAlgorithm, Ssl::CertificateProperties::signAlgorithm, sslBumpCertKey, sslCrtdHandleReplyWrapper(), sslServerBump, Ssl::ServerBump::step1, storeTlsContextToCache(), and Ssl::Helper::Submit().
Referenced by httpsPeeked(), and parseTlsHandshake().
◆ getTlsContextFromCache()
|
private |
- Returns
- a pointer to the matching cached TLS context or nil
Definition at line 2676 of file client_side.cc.
References Ssl::CertificateProperties::commonName, debugs, ClpMap< Key, Value, MemoryUsedBy >::del(), ClpMap< Key, Value, MemoryUsedBy >::get(), Ssl::GlobalContextStorage::getLocalStorage(), port, Ssl::TheGlobalContextStorage, and Ssl::verifySslCertificate().
Referenced by getSslContextStart().
◆ handleChunkedRequestBody()
|
protected |
Definition at line 2013 of file client_side.cc.
References bodyParser, bodyPipe, BodyPipeCheckout::buf, BodyPipe::buf(), BodyPipeCheckout::checkIn(), Server::clientConnection, clientIsRequestBodyTooLargeForPolicy(), debugs, ERR_INVALID_REQ, ERR_NONE, ERR_TOO_BIG, finishDechunkingRequest(), MemBuf::hasContent(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), BodyPipe::mayNeedMoreData(), Must, Http::One::Parser::needsMoreData(), Http::One::TeChunkedParser::needsMoreSpace(), Http::One::TeChunkedParser::parse(), BodyPipe::producedSize(), Http::One::Parser::remaining(), Http::One::TeChunkedParser::setPayloadBuffer(), and BodyPipe::status().
Referenced by handleRequestBodyData().
◆ handleIdleClientPinnedTlsRead()
|
protected |
Handles a ready-for-reading TLS squid-to-server connection that we thought was idle.
- Returns
- false if and only if the connection should be closed.
Definition at line 3803 of file client_side.cc.
References DBG_IMPORTANT, debugs, error(), fd_table, Must, pinning, and startPinnedConnectionMonitoring().
Referenced by clientPinnedConnectionRead().
◆ handleReadData()
|
overridevirtual |
called when new request data has been read from the socket
- Return values
-
false called comm_close or setReplyToError (the caller should bail) true we did not call comm_close or setReplyToError
Implements Server.
Definition at line 1958 of file client_side.cc.
References bodyPipe, and handleRequestBodyData().
◆ handleReply()
|
pure virtual |
ClientStream calls this to supply response header (once) and data for the current Http::Stream.
Implemented in Ftp::Server, and Http::One::Server.
Referenced by clientSocketRecipient().
◆ handleRequestBodyData()
bool ConnStateData::handleRequestBodyData | ( | ) |
called when new request body data has been buffered in inBuf may close the connection if we were closing and piped everything out
- Return values
-
false called comm_close or setReplyToError (the caller should bail) true we did not call comm_close or setReplyToError
Definition at line 1974 of file client_side.cc.
References abortChunkedRequestBody(), assert, bodyParser, bodyPipe, SBuf::c_str(), Server::clientConnection, Comm::Connection::close(), consumeInput(), debugs, error(), handleChunkedRequestBody(), Server::inBuf, SBuf::length(), BodyPipe::mayNeedMoreData(), BodyPipe::putMoreData(), and stoppedSending().
Referenced by handleReadData().
◆ handleSslBumpHandshakeError()
|
private |
Definition at line 3049 of file client_side.cc.
References Security::IoResult::category, Server::clientConnection, Comm::Connection::close(), DBG_IMPORTANT, debugs, ERR_GATEWAY_FAILURE, ERR_NONE, ERR_SECURE_ACCEPT_FAIL, Security::IoResult::errorDescription, Security::IoResult::errorDetail, Security::IoResult::important, Security::IoResult::ioError, Security::IoResult::ioSuccess, Security::IoResult::ioWantRead, Security::IoResult::ioWantWrite, MakeNamedErrorDetail(), tunnelOnError(), and updateError().
Referenced by startPeekAndSplice().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ hasNotes()
|
inline |
Definition at line 358 of file client_side.h.
References NotePairs::empty(), and theNotes.
◆ httpsPeeked()
void ConnStateData::httpsPeeked | ( | PinnedIdleContext | pic | ) |
Definition at line 3100 of file client_side.cc.
References ConnStateData::PinnedIdleContext::connection, debugs, Pipeline::empty(), Pipeline::front(), RefCount< C >::getRaw(), getSslContextStart(), Comm::IsConnOpen(), Must, notePinnedConnectionBecameIdle(), Server::pipeline, ConnStateData::PinnedIdleContext::request, Ssl::ServerBump::request, sslServerBump, and tlsConnectHostOrIp.
Referenced by FwdState::completed(), and FwdState::dispatch().
◆ idleTimeout()
|
protectedpure virtual |
Implemented in Ftp::Server, and Http::One::Server.
Referenced by readNextRequest().
◆ initiateTunneledRequest()
bool ConnStateData::initiateTunneledRequest | ( | HttpRequest::Pointer const & | cause, |
const char * | reason, | ||
const SBuf & | payload | ||
) |
Definition at line 3118 of file client_side.cc.
References buildFakeRequest(), Server::clientConnection, clientProcessRequestFinished(), debugs, ERR_INVALID_REQ, HttpRequest::flags, RequestFlags::forceTunnel, AnyP::Uri::hostOrIp(), SBuf::isEmpty(), Comm::Connection::local, MakeNamedErrorDetail(), MAX_IPSTRLEN, pinning, Ip::Address::port(), AnyP::Uri::port(), tlsConnectHostOrIp, tlsConnectPort, Ip::Address::toStr(), transparent(), updateError(), and HttpRequest::url.
Referenced by splice(), and tunnelOnError().
◆ isOpen()
bool ConnStateData::isOpen | ( | ) | const |
Definition at line 664 of file client_side.cc.
References cbdataReferenceValid(), Server::clientConnection, Comm::Connection::fd, fd_table, and Comm::IsConnOpen().
Referenced by ~ConnStateData(), afterClientRead(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), kick(), ClientHttpRequest::noteAdaptationAclCheckDone(), parseRequests(), sendControlMsg(), and sslCrtdHandleReply().
◆ kick()
void ConnStateData::kick | ( | ) |
- We are done with the response, and we are either still receiving request body (early response!) or have already stopped receiving anything.
If we are still receiving, then parseRequests() below will fail. (XXX: but then we will call readNextRequest() which may succeed and execute a smuggled request as we are not done with the current request).
If we stopped because we got everything, then try the next request.
If we stopped receiving because of an error, then close now to avoid getting stuck and to prevent accidental request smuggling.
- Attempt to parse a request from the request buffer. If we've been fed a pipelined request it may already be in our read buffer.
- At this point we either have a parsed request (which we've kicked off the processing for) or not. If we have a deferred request (parsed but deferred for pipeling processing reasons) then look at processing it. If not, simply kickstart another read.
Definition at line 918 of file client_side.cc.
References Server::clientConnection, ClientSocketContextPushDeferredIfNeeded(), Comm::Connection::close(), DBG_IMPORTANT, debugs, flags, Pipeline::front(), Comm::IsConnOpen(), isOpen(), MYNAME, parseRequests(), pinning, Server::pipeline, readNextRequest(), and stoppedReceiving().
Referenced by notePinnedConnectionBecameIdle(), and Http::Stream::writeComplete().
◆ lifetimeTimeout()
void ConnStateData::lifetimeTimeout | ( | const CommTimeoutCbParams & | params | ) |
Definition at line 2121 of file client_side.cc.
References CommCommonCbParams::conn, DBG_IMPORTANT, debugs, ERR_LIFETIME_EXP, Debug::Extra(), terminateAll(), and LogTagsErrors::timedout.
Referenced by extendLifetime().
◆ maybeMakeSpaceAvailable()
|
inherited |
Prepare inBuf for I/O. This method balances several conflicting desires:
- Do not read too few bytes at a time.
- Do not waste too much buffer space.
- Do not [re]allocate or memmove the buffer too much.
- Obey Config.maxRequestBufferSize limit.
Definition at line 74 of file Server.cc.
References SBufReservationRequirements::allowShared, CLIENT_REQ_BUF_SZ, Config, debugs, SBufReservationRequirements::idealSpace, Server::inBuf, SBufReservationRequirements::maxCapacity, SquidConfig::maxRequestBufferSize, SBufReservationRequirements::minSpace, SBuf::reserve(), and SBuf::spaceSize().
Referenced by Server::doClientRead().
◆ mayNeedToReadMoreBody()
int64_t ConnStateData::mayNeedToReadMoreBody | ( | ) | const |
number of body bytes we need to comm_read for the "current" request
- Return values
-
0 We do not need to read any [more] body bytes negative May need more but do not know how many; could be zero! positive Need to read exactly that many more body bytes
Definition at line 3570 of file client_side.cc.
References bodyPipe, BodyPipe::bodySizeKnown(), Server::inBuf, SBuf::length(), and BodyPipe::unproducedSize().
Referenced by clientAfterReadingRequests(), and stopSending().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteBodyConsumerAborted()
|
overridepure virtual |
Implements BodyProducer.
Implemented in Ftp::Server, and Http::One::Server.
Definition at line 2087 of file client_side.cc.
References bodyPipe, and BodyPipe::enableAutoConsumption().
Referenced by Ftp::Server::noteBodyConsumerAborted(), and Http::One::Server::noteBodyConsumerAborted().
◆ noteMoreBodySpaceAvailable()
|
overridepure virtual |
Implements BodyProducer.
Implemented in Ftp::Server, and Http::One::Server.
◆ notePeerConnection()
|
inlinevirtual |
Reimplemented in Ftp::Server.
Definition at line 207 of file client_side.h.
Referenced by FwdState::successfullyConnectedToPeer().
◆ notePinnedConnectionBecameIdle()
void ConnStateData::notePinnedConnectionBecameIdle | ( | PinnedIdleContext | pic | ) |
Definition at line 3721 of file client_side.cc.
References ConnStateData::PinnedIdleContext::connection, Pipeline::empty(), kick(), Must, pinConnection(), Server::pipeline, ConnStateData::PinnedIdleContext::request, and startPinnedConnectionMonitoring().
Referenced by httpsPeeked().
◆ notes()
NotePairs::Pointer ConnStateData::notes | ( | ) |
- Returns
- existing non-empty connection annotations, creates and returns empty annotations otherwise
Definition at line 4042 of file client_side.cc.
References theNotes.
Referenced by UpdateRequestNotes().
◆ noteTakeServerConnectionControl()
|
inlinevirtual |
Gives us the control of the Squid-to-server connection. Used, for example, to initiate a TCP tunnel after protocol switching.
Reimplemented in Http::One::Server.
Definition at line 229 of file client_side.h.
Referenced by HttpStateData::proceedAfter1xx().
◆ parseHttpRequest()
|
protected |
Parse an HTTP request
- Note
- Sets result->flags.parsed_ok to 0 if failed to parse the request, to 1 if the request was correctly parsed
- Parameters
-
[in] hp an Http1::RequestParser
- Returns
- NULL on incomplete requests, a Http::Stream on success or failure. TODO: Move to HttpServer. Warning: Move requires large code nonchanges!
Definition at line 1279 of file client_side.cc.
References abortRequestParsing(), ClientHttpRequest::Flags::accel, SquidConfig::appendDomainLen, ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), Config, consumeInput(), StoreIOBuffer::data, DBG_IMPORTANT, debugs, ClientHttpRequest::flags, Http::Stream::flags, HTTP_REQBUF_SZ, Server::inBuf, internalCheck(), internalLocalUri(), SBuf::isEmpty(), SBuf::length(), StoreIOBuffer::length, Http::METHOD_CONNECT, Http::METHOD_NONE, Http::METHOD_PRI, Must, Http::Stream::parsed_ok, port, prepareAcceleratedURL(), prepareTlsSwitchingURL(), prepareTransparentURL(), preservedClientData, preservingClientData_, Http::ProtocolVersion(), ClientHttpRequest::req_sz, Http::Stream::reqbuf, SBufToCstring(), Http::scMethodNotAllowed, Http::scRequestHeaderFieldsTooLarge, Http::scUriTooLong, switchedToHttps(), Server::transferProtocol, transparent(), ClientHttpRequest::uri, xcalloc(), and xstrdup.
◆ parseOneRequest()
|
protectedpure virtual |
parse input buffer prefix into a single transfer protocol request return NULL to request more header bytes (after checking any limits) use abortRequestParsing() to handle parsing errors w/o creating request
Implemented in Ftp::Server, and Http::One::Server.
Referenced by parseRequests().
◆ parseProxyProtocolHeader()
|
private |
Attempts to extract a PROXY protocol header from the input buffer and, upon success, stores the parsed header in proxyProtocolHeader_.
- Returns
- true if the header was successfully parsed
- false if more data is needed to parse the header or on error
Definition at line 1819 of file client_side.cc.
References assert, Server::clientConnection, COMM_TRANSPARENT, SBuf::consume(), debugs, Comm::Connection::flags, Server::inBuf, SBuf::length(), Comm::Connection::local, needProxyProtocolHeader_, ProxyProtocol::Parse(), proxyProtocolError(), proxyProtocolHeader_, and Comm::Connection::remote.
Referenced by parseRequests().
◆ parseRequests()
|
private |
Attempt to parse one or more requests from the input buffer. May close the connection.
Definition at line 1856 of file client_side.cc.
References assert, bodyPipe, Server::clientConnection, Comm::Connection::close(), commIsHalfClosed(), concurrentRequestQueueFilled(), Config, debugs, Pipeline::empty(), extendLifetime(), Comm::Connection::fd, flags, Server::inBuf, SBuf::isEmpty(), isOpen(), SBuf::length(), SquidConfig::maxRequestHeaderSize, Must, needProxyProtocolHeader_, parsedBumpedRequestCount, parseOneRequest(), parseProxyProtocolHeader(), pinning, Server::pipeline, preservingClientData_, processParsedRequest(), switchedToHttps(), and whenClientIpKnown().
Referenced by afterClientRead(), and kick().
◆ parseTlsHandshake()
void ConnStateData::parseTlsHandshake | ( | ) |
Definition at line 2854 of file client_side.cc.
References Ssl::ServerBump::act, ClientHttpRequest::al, assert, Ssl::bumpClientFirst, Ssl::bumpPeek, Ssl::bumpServerFirst, Ssl::bumpStare, Server::clientConnection, Comm::Connection::close(), CurrentException(), debugs, Security::HandshakeParser::details, Ssl::ServerBump::entry, ERR_PROTOCOL_UNKNOWN, Comm::Connection::fd, fd_note(), Pipeline::front(), RefCount< C >::getRaw(), getSslContextStart(), TextException::id(), Server::inBuf, SBuf::isEmpty(), MakeNamedErrorDetail(), Must, Security::HandshakeParser::parseHello(), parsingTlsHandshake, Server::pipeline, preservedClientData, Server::readSomeData(), receivedFirstByte(), Ssl::ServerBump::request, Comm::ResetSelect(), resetSslCommonName(), Security::NegotiationHistory::retrieveParsedInfo(), sslServerBump, FwdState::Start(), startPeekAndSplice(), Ssl::ServerBump::step, Ssl::ServerBump::step1, tlsBump3, tlsClientSni_, Comm::Connection::tlsNegotiations(), tlsParser, tunnelOnError(), and updateError().
Referenced by afterClientRead().
◆ pinBusyConnection()
void ConnStateData::pinBusyConnection | ( | const Comm::ConnectionPointer & | pinServerConn, |
const HttpRequest::Pointer & | request | ||
) |
Forward future client requests using the given to-server connection. The connection is still being used by the current client request.
Definition at line 3715 of file client_side.cc.
References pinConnection().
◆ pinConnection()
|
private |
Definition at line 3736 of file client_side.cc.
References cbdataReference, Server::clientConnection, clientPinnedConnectionClosed(), comm_add_close_handler(), RequestFlags::connectionAuth, debugs, Comm::Connection::fd, FD_DESC_SZ, fd_note(), HttpRequest::flags, Comm::Connection::getPeer(), AnyP::Uri::host(), Comm::IsConnOpen(), JobCallback, MAX_IPSTRLEN, Must, pinning, AnyP::Uri::port(), Comm::Connection::remote, Ip::Address::toUrl(), unpinConnection(), HttpRequest::url, and xstrdup.
Referenced by notePinnedConnectionBecameIdle(), and pinBusyConnection().
◆ pinnedAuth()
|
inline |
Definition at line 204 of file client_side.h.
References pinning.
◆ pinnedPeer()
|
inline |
- Returns
- the pinned CachePeer if one exists, nil otherwise
Definition at line 203 of file client_side.h.
References pinning.
Referenced by PeerSelector::selectPinned().
◆ pipelinePrefetchMax()
|
protectedvirtual |
Reimplemented in Ftp::Server, and Http::One::Server.
Definition at line 1736 of file client_side.cc.
References Config, pinning, and SquidConfig::pipeline_max_prefetch.
Referenced by concurrentRequestQueueFilled(), and Http::One::Server::pipelinePrefetchMax().
◆ postHttpsAccept()
void ConnStateData::postHttpsAccept | ( | ) |
Definition at line 2494 of file client_side.cc.
References ACCESS_DENIED, SquidConfig::accessList, ACLFilledChecklist::al, assert, bareError, AccessLogEntry::cache, AccessLogEntry::CacheDetails::caddr, Server::clientConnection, COMM_INTERCEPTION, COMM_TRANSPARENT, Config, current_time, debugs, fatal(), fillChecklist(), Comm::Connection::flags, Pipeline::front(), AnyP::Uri::host(), HTTPMSGLOCK(), HTTPMSGUNLOCK(), httpsEstablish(), httpsSslBumpAccessCheckDone(), Comm::Connection::local, log_addr, ClientHttpRequest::log_uri, MasterXaction::MakePortful(), MAX_IPSTRLEN, HttpRequest::myportname, ACLChecklist::nonBlockingCheck(), Server::pipeline, AccessLogEntry::CacheDetails::port, port, Ip::Address::port(), AnyP::Uri::port(), AccessLogEntry::proxyProtocolHeader, proxyProtocolHeader_, AccessLogEntry::request, CodeContext::Reset(), SquidConfig::ssl_bump, AccessLogEntry::CacheDetails::start_time, ACLFilledChecklist::syncAle(), AccessLogEntry::tcpClient, Ip::Address::toStr(), AccessLogEntry::updateError(), and HttpRequest::url.
◆ prepareTlsSwitchingURL()
char * ConnStateData::prepareTlsSwitchingURL | ( | const Http1::RequestParserPointer & | hp | ) |
Definition at line 1220 of file client_side.cc.
References buildUrlFromHost(), debugs, AnyP::UriScheme::image(), SBuf::isEmpty(), SBuf::length(), Must, AnyP::ProtocolVersion::protocol, SQUIDSBUFPH, SQUIDSBUFPRINT, switchedToHttps(), tlsClientSni(), tlsConnectHostOrIp, tlsConnectPort, Server::transferProtocol, and xcalloc().
Referenced by parseHttpRequest().
◆ processParsedRequest()
|
protectedpure virtual |
Implemented in Ftp::Server, and Http::One::Server.
Referenced by parseRequests().
◆ proxyProtocolError()
|
private |
Perform cleanup on PROXY protocol errors. If header parsing hits a fatal error terminate the connection, otherwise wait for more data.
Definition at line 1798 of file client_side.cc.
References Server::clientConnection, DBG_IMPORTANT, debugs, and AsyncJob::mustStop().
Referenced by parseProxyProtocolHeader(), and proxyProtocolValidateClient().
◆ proxyProtocolHeader()
|
inline |
Definition at line 360 of file client_side.h.
References proxyProtocolHeader_.
Referenced by ClientHttpRequest::ClientHttpRequest().
◆ proxyProtocolValidateClient()
|
private |
Perform proxy_protocol_access ACL tests on the client which connected to PROXY protocol port to see if we trust the sender enough to accept their PROXY header claim.
Definition at line 1779 of file client_side.cc.
References SquidConfig::accessList, Acl::Answer::allowed(), Config, ACLChecklist::fastCheck(), fillChecklist(), SquidConfig::proxyProtocol, and proxyProtocolError().
Referenced by start().
◆ quitAfterError()
void ConnStateData::quitAfterError | ( | HttpRequest * | request | ) |
Changes state so that we close the connection and quit after serving the client-side-detected error response instead of getting stuck.
Definition at line 1455 of file client_side.cc.
References Server::clientConnection, debugs, flags, HttpRequest::flags, and RequestFlags::proxyKeepalive.
Referenced by serveDelayedError().
◆ reading()
|
inlineinherited |
Definition at line 60 of file Server.h.
References Server::reader.
Referenced by Server::doClientRead(), Server::readSomeData(), and Server::stopReading().
◆ readNextRequest()
void ConnStateData::readNextRequest | ( | ) |
Set the timeout BEFORE calling readSomeData().
Please don't do anything with the FD past here!
Definition at line 881 of file client_side.cc.
References Server::clientConnection, debugs, Comm::Connection::fd, fd_note(), idleTimeout(), Server::readSomeData(), resetReadTimeout(), and Comm::Connection::timeLeft().
Referenced by kick().
◆ readSomeData()
|
inherited |
Definition at line 89 of file Server.cc.
References Server::clientConnection, Config, debugs, Server::doClientRead(), Server::inBuf, JobCallback, SBuf::length(), SquidConfig::maxRequestBufferSize, Comm::Read(), Server::reader, and Server::reading().
Referenced by clientAfterReadingRequests(), Server::doClientRead(), parseTlsHandshake(), readNextRequest(), and switchToHttps().
◆ receivedFirstByte()
|
overridevirtual |
Implements Server.
Definition at line 1844 of file client_side.cc.
References Config, Server::receivedFirstByte_, SquidConfig::request, resetReadTimeout(), and SquidConfig::Timeout.
Referenced by parseTlsHandshake().
◆ registerRunner()
|
protectedinherited |
Definition at line 104 of file RunnersRegistry.cc.
References FindRunners(), and RegisterRunner_().
Referenced by ConnStateData(), IdleConnList::IdleConnList(), and Rock::Rebuild::Rebuild().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestTimeout()
void ConnStateData::requestTimeout | ( | const CommTimeoutCbParams & | io | ) |
general lifetime handler for HTTP requests
Definition at line 2098 of file client_side.cc.
References Comm::Connection::close(), CommCommonCbParams::conn, debugs, ERR_REQUEST_PARSE_TIMEOUT, ERR_REQUEST_START_TIMEOUT, error(), CommCommonCbParams::fd, Comm::IsConnOpen(), Server::receivedFirstByte_, tunnelOnError(), and updateError().
Referenced by resetReadTimeout(), and Http::One::Server::start().
◆ resetReadTimeout()
void ConnStateData::resetReadTimeout | ( | time_t | timeout | ) |
Definition at line 598 of file client_side.cc.
References Server::clientConnection, commSetConnTimeout(), JobCallback, and requestTimeout().
Referenced by getSslContextDone(), httpsEstablish(), readNextRequest(), receivedFirstByte(), and switchToHttps().
◆ resetSslCommonName()
|
inline |
Definition at line 293 of file client_side.h.
References sslCommonName_.
Referenced by parseTlsHandshake(), and switchToHttps().
◆ sendControlMsg()
|
overridevirtual |
Implements HttpControlMsgSink.
Definition at line 3651 of file client_side.cc.
References HttpControlMsgSink::cbControlMsgSent, HttpControlMsg::cbSuccess, Server::clientConnection, Comm::Connection::close(), debugs, doneWithControlMsg(), Pipeline::empty(), Pipeline::front(), RefCount< C >::getRaw(), isOpen(), JobCallback, Must, Server::pipeline, HttpControlMsg::reply, writeControlMsgAndCall(), and HttpControlMsgSink::wroteControlMsg().
Referenced by Ftp::Relay::forwardPreliminaryReply(), and HttpStateData::handle1xx().
◆ serveDelayedError()
bool ConnStateData::serveDelayedError | ( | Http::Stream * | context | ) |
Called when the client sends the first request on a bumped connection. Returns false if no [delayed] error should be written to the client. Otherwise, writes the error to the client and returns true. Also checks for SQUID_X509_V_ERR_DOMAIN_MISMATCH on bumped requests.
Definition at line 1467 of file client_side.cc.
References ClientHttpRequest::al, Acl::Answer::allowed(), assert, SquidConfig::cert_error, Ssl::checkX509ServerValidity(), clientAclChecklistFill(), Server::clientConnection, Config, debugs, Ssl::ServerBump::entry, ERR_SECURE_CONNECT_FAIL, HttpRequest::error, ACLChecklist::fastCheck(), Http::Stream::getClientReplyContext(), HttpRequest::hier, AnyP::Uri::host(), Http::Stream::http, StoreEntry::isEmpty(), HttpRequest::method, ClientHttpRequest::Out::offset, ClientHttpRequest::out, Http::Stream::pullData(), quitAfterError(), Comm::Connection::remote, ClientHttpRequest::request, Ssl::ServerBump::request, Http::scServiceUnavailable, Ssl::ServerBump::serverCert, clientReplyContext::setReplyToError(), clientReplyContext::setReplyToStoreEntry(), SQUID_X509_V_ERR_DOMAIN_MISMATCH, SquidConfig::ssl_client, ACLFilledChecklist::sslErrors, sslServerBump, Error::update(), updateError(), ClientHttpRequest::uri, and HttpRequest::url.
◆ serverBump()
|
inline |
Definition at line 285 of file client_side.h.
References sslServerBump.
Referenced by Format::Format::assemble(), TunnelStateData::clientExpectsConnectResponse(), httpsSslBumpStep2AccessCheckDone(), and ClientRequestContext::sslBumpAccessCheck().
◆ setAuth()
void ConnStateData::setAuth | ( | const Auth::UserRequest::Pointer & | aur, |
const char * | cause | ||
) |
Set the user details for connection-based authentication to use from now until connection closure.
Any change to existing credentials shows that something invalid has happened. Such as:
- NTLM/Negotiate auth was violated by the per-request headers missing a revalidation token
- NTLM/Negotiate auth was violated by the per-request headers being for another user
- SSL-Bump CONNECT tunnel with persistent credentials has ended
Definition at line 518 of file client_side.cc.
References auth_, Server::clientConnection, comm_reset_close(), debugs, Auth::UserRequest::releaseAuthServer(), and stopReceiving().
Referenced by ProxyAuthLookup::LookupDone(), ClientHttpRequest::sslBumpEstablish(), and swanSong().
◆ setServerBump()
|
inline |
Definition at line 286 of file client_side.h.
References assert, and sslServerBump.
Referenced by ClientHttpRequest::doCallouts().
◆ shouldCloseOnEof()
|
overrideprivatevirtual |
Implements Server.
Definition at line 1413 of file client_side.cc.
References Config, debugs, Pipeline::empty(), SquidConfig::half_closed_clients, Server::inBuf, SBuf::isEmpty(), SquidConfig::onoff, and Server::pipeline.
◆ shouldPreserveClientData()
bool ConnStateData::shouldPreserveClientData | ( | ) | const |
whether we should start saving inBuf client bytes in anticipation of tunneling them to the server later (on_unsupported_protocol)
Definition at line 4010 of file client_side.cc.
References SquidConfig::accessList, Config, needProxyProtocolHeader_, Pipeline::nrequests, SquidConfig::on_unsupported_protocol, parsedBumpedRequestCount, parsingTlsHandshake, Server::pipeline, port, AnyP::PROTO_FTP, switchedToHttps(), and transparent().
Referenced by start(), and switchToHttps().
◆ splice()
bool ConnStateData::splice | ( | ) |
Definition at line 2959 of file client_side.cc.
References assert, Server::clientConnection, Pipeline::empty(), fakeAConnectRequest(), Comm::Connection::fd, fd_table, Pipeline::front(), initiateTunneledRequest(), Must, Server::pipeline, preservedClientData, Http::ProtocolVersion(), ClientHttpRequest::request, Server::transferProtocol, and transparent().
Referenced by httpsSslBumpStep2AccessCheckDone().
◆ sslCommonName()
|
inline |
Definition at line 292 of file client_side.h.
References sslCommonName_.
◆ sslCrtdHandleReply()
void ConnStateData::sslCrtdHandleReply | ( | const Helper::Reply & | reply | ) |
Definition at line 2557 of file client_side.cc.
References Ssl::ServerBump::act, Ssl::algSignTrusted, Helper::BrokenHelper, Ssl::bumpPeek, Ssl::bumpStare, Server::clientConnection, Ssl::configureSSLUsingPkeyAndCertFromMemory(), Ssl::configureUnconfiguredSslContext(), MemBuf::content(), MemBuf::contentSize(), DBG_IMPORTANT, debugs, doPeekAndSpliceStep(), Comm::Connection::fd, fd_table, Ssl::GenerateSslContextUsingPkeyAndCertFromMemory(), Ssl::CrtdMessage::getBody(), Security::GetFrom(), getSslContextDone(), MemBuf::hasContent(), SBuf::isEmpty(), isOpen(), Store::nil, Ssl::CrtdMessage::OK, Helper::Okay, Helper::Reply::other(), Ssl::CrtdMessage::parse(), port, Ssl::CrtdMessage::REPLY, Helper::Reply::result, signAlgorithm, sslBumpCertKey, sslServerBump, Ssl::ServerBump::step1, storeTlsContextToCache(), and tlsConnectHostOrIp.
Referenced by sslCrtdHandleReplyWrapper().
◆ sslCrtdHandleReplyWrapper()
|
static |
Definition at line 2550 of file client_side.cc.
References sslCrtdHandleReply().
Referenced by getSslContextStart().
◆ start()
|
overridevirtual |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Server, and Http::One::Server.
Definition at line 2148 of file client_side.cc.
References Server::clientConnection, comm_add_close_handler(), connStateClosed(), DBG_IMPORTANT, debugs, DISABLE_PMTU_ALWAYS, DISABLE_PMTU_OFF, Comm::Connection::fd, JobCallback, needProxyProtocolHeader_, port, preservingClientData_, proxyProtocolValidateClient(), shouldPreserveClientData(), AsyncJob::start(), transparent(), whenClientIpKnown(), and xstrerr().
Referenced by Ftp::Server::start(), and Http::One::Server::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ startDechunkingRequest()
|
protected |
Definition at line 3618 of file client_side.cc.
References assert, bodyParser, bodyPipe, debugs, Must, and BodyPipe::status().
Referenced by expectRequestBody().
◆ startPeekAndSplice()
void ConnStateData::startPeekAndSplice | ( | ) |
Definition at line 2993 of file client_side.cc.
References acceptTls(), ACCESS_ALLOWED, SquidConfig::accessList, ClientHttpRequest::al, assert, Ssl::ServerBump::at(), ACLChecklist::banAction(), BIO_get_data(), Ssl::bumpClientFirst, Ssl::bumpNone, Ssl::bumpServerFirst, SBuf::clear(), Server::clientConnection, Config, Ssl::createSSLContext(), debugs, Ssl::ServerBump::entry, Comm::Connection::fd, fd_table, fillChecklist(), Pipeline::front(), RefCount< C >::getRaw(), handleSslBumpHandshakeError(), Ssl::ClientBio::hold(), httpsCreate(), httpsSslBumpStep2AccessCheckDone(), Server::inBuf, ACLChecklist::nonBlockingCheck(), Server::pipeline, port, Ssl::ServerBump::request, Ssl::ClientBio::setReadBufData(), SquidConfig::ssl_bump, sslServerBump, FwdState::Start(), Ssl::ServerBump::step, switchedToHttps_, tlsBump1, tlsBump2, and tlsBump3.
Referenced by httpsSslBumpStep2AccessCheckDone(), and parseTlsHandshake().
◆ startPinnedConnectionMonitoring()
|
protected |
[re]start monitoring pinned connection for peer closures so that we can propagate them to an idle client pinned to that peer
Definition at line 3781 of file client_side.cc.
References clientPinnedConnectionRead(), JobCallback, pinning, and Comm::Read().
Referenced by handleIdleClientPinnedTlsRead(), and notePinnedConnectionBecameIdle().
◆ startReconfigure()
|
inlinevirtualinherited |
Called after receiving a reconfigure request and before parsing squid.conf. Meant for modules that need to prepare for their configuration being changed [outside their control]. The changes end with the syncConfig() event.
Reimplemented in Dns::ConfigRr.
Definition at line 67 of file RunnersRegistry.h.
Referenced by mainReconfigureStart().
◆ startShutdown()
|
overridevirtual |
Called after receiving a shutdown request and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented from RegisteredRunner.
Definition at line 1038 of file client_side.cc.
References Pipeline::empty(), endingShutdown(), and Server::pipeline.
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Ecap::XactionRep, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Adaptation::Initiate, Http::Tunneler, Comm::TcpAcceptor, HappyConnOpener, Ipc::Inquirer, and Security::PeerConnector.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Adaptation::Initiate::status(), and Comm::TcpAcceptor::status().
◆ stoppedReceiving()
|
inline |
Definition at line 159 of file client_side.h.
References stoppedReceiving_.
Referenced by kick(), stopReceiving(), and stopSending().
◆ stoppedSending()
|
inline |
Definition at line 161 of file client_side.h.
References stoppedSending_.
Referenced by handleRequestBodyData(), stopReceiving(), and stopSending().
◆ stopPinnedConnectionMonitoring()
void ConnStateData::stopPinnedConnectionMonitoring | ( | ) |
Definition at line 3793 of file client_side.cc.
References pinning, and Comm::ReadCancel().
Referenced by borrowPinnedConnection(), and unpinConnection().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by ~ConnStateData(), Client::cleanAdaptation(), finishDechunkingRequest(), Client::noteBodyConsumerAborted(), and Client::serverComplete2().
◆ stopReading()
|
inherited |
Definition at line 60 of file Server.cc.
References Server::clientConnection, Comm::Connection::fd, Comm::ReadCancel(), Server::reader, and Server::reading().
Referenced by ClientHttpRequest::processRequest().
◆ stopReceiving()
void ConnStateData::stopReceiving | ( | const char * | error | ) |
Definition at line 3588 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), debugs, error(), stoppedReceiving(), stoppedReceiving_, stoppedSending(), and stoppedSending_.
Referenced by setAuth().
◆ stopSending()
void ConnStateData::stopSending | ( | const char * | error | ) |
Definition at line 983 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), debugs, error(), Server::inBuf, SBuf::length(), mayNeedToReadMoreBody(), stoppedReceiving(), stoppedReceiving_, stoppedSending(), and stoppedSending_.
◆ storeTlsContextToCache()
|
private |
Attempts to add a given TLS context to the cache, replacing the old same-key context, if any
Definition at line 2694 of file client_side.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), Server::clientConnection, Comm::Connection::fd, fd_table, Ssl::GlobalContextStorage::getLocalStorage(), port, and Ssl::TheGlobalContextStorage.
Referenced by getSslContextStart(), and sslCrtdHandleReply().
◆ swanSong()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 615 of file client_side.cc.
References checkLogging(), Server::clientConnection, clientdbEstablished(), debugs, ERR_NONE, flags, Comm::Connection::remote, setAuth(), Server::swanSong(), terminateAll(), and unpinConnection().
◆ switchedToHttps()
|
inline |
Definition at line 284 of file client_side.h.
References switchedToHttps_.
Referenced by buildFakeRequest(), parseHttpRequest(), parseRequests(), prepareTlsSwitchingURL(), shouldPreserveClientData(), and ClientRequestContext::sslBumpAccessCheck().
◆ switchToHttps()
void ConnStateData::switchToHttps | ( | ClientHttpRequest * | http, |
Ssl::BumpMode | bumpServerMode | ||
) |
Definition at line 2801 of file client_side.cc.
References assert, Ssl::bumpPeek, Ssl::bumpServerFirst, Ssl::bumpStare, Server::clientConnection, Config, debugs, flags, Must, parsingTlsHandshake, preservingClientData_, AnyP::PROTO_HTTPS, AnyP::ProtocolVersion::protocol, Server::readSomeData(), Server::receivedFirstByte_, ClientHttpRequest::request, SquidConfig::request_start_timeout, resetReadTimeout(), resetSslCommonName(), shouldPreserveClientData(), sslServerBump, switchedToHttps_, SquidConfig::Timeout, tlsConnectHostOrIp, tlsConnectPort, and Server::transferProtocol.
Referenced by ClientHttpRequest::sslBumpEstablish().
◆ syncConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf during reconfiguration. Meant for adjusting the module state based on configuration changes.
Reimplemented in Auth::CredentialCacheRr, and PeerPoolMgrsRr.
Definition at line 71 of file RunnersRegistry.h.
Referenced by mainReconfigureFinish().
◆ terminateAll()
|
overrideprivatevirtual |
Implements Server.
Definition at line 3948 of file client_side.cc.
References assert, Pipeline::back(), bareError, bodyPipe, SBuf::clear(), Server::clientConnection, Comm::Connection::close(), Pipeline::count(), debugs, Pipeline::empty(), error(), Pipeline::front(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), MakeNamedErrorDetail(), Pipeline::nrequests, parsingTlsHandshake, Server::pipeline, and Error::update().
Referenced by lifetimeTimeout(), and swanSong().
◆ tlsClientSni()
|
inline |
Definition at line 294 of file client_side.h.
References tlsClientSni_.
Referenced by prepareTlsSwitchingURL().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ transparent()
bool ConnStateData::transparent | ( | ) | const |
Definition at line 3553 of file client_side.cc.
References Server::clientConnection, COMM_INTERCEPTION, COMM_TRANSPARENT, and Comm::Connection::flags.
Referenced by concurrentRequestQueueFilled(), fakeAConnectRequest(), initiateTunneledRequest(), parseHttpRequest(), shouldPreserveClientData(), splice(), and start().
◆ tunnelOnError()
|
protected |
Definition at line 1541 of file client_side.cc.
References SquidConfig::accessList, Server::clientConnection, COMM_SELECT_READ, Config, debugs, ACLChecklist::fastCheck(), Comm::Connection::fd, fillChecklist(), Pipeline::front(), initiateTunneledRequest(), SquidConfig::on_unsupported_protocol, Server::pipeline, preservedClientData, preservingClientData_, ACLFilledChecklist::requestErrorType, and Comm::SetSelect().
Referenced by handleSslBumpHandshakeError(), parseTlsHandshake(), and requestTimeout().
◆ unpinConnection()
void ConnStateData::unpinConnection | ( | const bool | andClose | ) |
Definition at line 3917 of file client_side.cc.
References cbdataReferenceDone, comm_remove_close_handler(), debugs, Comm::IsConnOpen(), pinning, safe_free, and stopPinnedConnectionMonitoring().
Referenced by borrowPinnedConnection(), clientPinnedConnectionClosed(), pinConnection(), Ftp::Relay::serverComplete(), and swanSong().
◆ unregisterRunner()
|
protectedinherited |
Definition at line 96 of file RunnersRegistry.cc.
References FindRunners().
Referenced by IndependentRunner::~IndependentRunner().
◆ updateError() [1/2]
|
inline |
Definition at line 366 of file client_side.h.
References Helper::Error, and updateError().
Referenced by updateError().
◆ updateError() [2/2]
void ConnStateData::updateError | ( | const Error & | error | ) |
Definition at line 652 of file client_side.cc.
References assert, bareError, error(), Pipeline::front(), Server::pipeline, and Error::update().
Referenced by callException(), handleSslBumpHandshakeError(), initiateTunneledRequest(), parseTlsHandshake(), requestTimeout(), and serveDelayedError().
◆ useConfig()
|
inlinevirtualinherited |
Called after claimMemoryNeeds(). Meant for activating modules and features using a finalized configuration with known memory requirements.
Reimplemented in ClientDbRr, SharedMemPagesRr, Ipc::Mem::RegisteredRunner, MemStoreRr, PeerPoolMgrsRr, SharedSessionCacheRr, and TransientsRr.
Definition at line 60 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
◆ whenClientIpKnown()
|
protected |
Perform client data lookups that depend on client src-IP. The PROXY protocol may require some data input first.
Definition at line 2187 of file client_side.cc.
References Acl::Answer::allowed(), assert, ACLChecklist::changeAcl(), SquidConfig::client_db, Server::clientConnection, clientdbEstablished(), clientdbGetInfo(), SquidConfig::ClientDelay, clientIdentDone, Config, debugs, ACLChecklist::fastCheck(), Comm::Connection::fd, fd_table, fillChecklist(), FQDN_LOOKUP_IF_MISS, fqdncache_gethostbyaddr(), ClientDelayConfig::initial, ClientDelayPools::Instance(), SquidConfig::onoff, ClientDelayPools::pools, Comm::Connection::remote, Dns::ResolveClientAddressesAsap, ClientInfo::setWriteLimiter(), Ident::Start(), and Ident::TheConfig.
Referenced by parseRequests(), and start().
◆ write() [1/2]
|
inlineinherited |
Definition at line 79 of file Server.h.
References Server::clientConnection, Server::clientWriteDone(), JobCallback, Comm::Write(), and Server::writer.
◆ write() [2/2]
|
inlineinherited |
Definition at line 72 of file Server.h.
References Server::clientConnection, Server::clientWriteDone(), JobCallback, Comm::Write(), and Server::writer.
◆ writeControlMsgAndCall()
|
pure virtual |
Implemented in Ftp::Server, and Http::One::Server.
Referenced by sendControlMsg().
◆ writeSomeData()
|
inlinevirtualinherited |
Definition at line 69 of file Server.h.
Referenced by Server::clientWriteDone().
◆ writing()
|
inlineinherited |
Definition at line 89 of file Server.h.
References Server::writer.
◆ wroteControlMsg()
|
inherited |
called when we wrote the 1xx response
Definition at line 25 of file HttpControlMsg.cc.
References Comm::Connection::close(), CommCommonCbParams::conn, debugs, HttpControlMsgSink::doneWithControlMsg(), Comm::ERR_CLOSING, CommCommonCbParams::flag, Comm::OK, CommCommonCbParams::xerrno, and xstrerr().
Referenced by sendControlMsg().
Member Data Documentation
◆ auth
bool ConnStateData::auth = false |
Definition at line 147 of file client_side.h.
Referenced by clientCheckPinning().
◆ auth_
|
private |
Definition at line 474 of file client_side.h.
◆ bareError
Error ConnStateData::bareError |
Definition at line 381 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), add(), checkLogging(), postHttpsAccept(), terminateAll(), and updateError().
◆ bodyParser
Http1::TeChunkedParser* ConnStateData::bodyParser = nullptr |
Definition at line 108 of file client_side.h.
Referenced by ~ConnStateData(), finishDechunkingRequest(), handleChunkedRequestBody(), handleRequestBodyData(), and startDechunkingRequest().
◆ bodyPipe
|
protected |
Definition at line 431 of file client_side.h.
Referenced by ~ConnStateData(), expectNoForwarding(), expectRequestBody(), finishDechunkingRequest(), handleChunkedRequestBody(), handleReadData(), handleRequestBodyData(), mayNeedToReadMoreBody(), noteBodyConsumerAborted(), parseRequests(), startDechunkingRequest(), and terminateAll().
◆ cbControlMsgSent
|
inherited |
Definition at line 42 of file HttpControlMsg.h.
Referenced by clientSocketRecipient(), HttpControlMsgSink::doneWithControlMsg(), and sendControlMsg().
◆ clientConnection
|
inherited |
Definition at line 100 of file Server.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), Server::Server(), TunnelStateData::TunnelStateData(), ~ConnStateData(), abortChunkedRequestBody(), abortRequestParsing(), acceptTls(), afterClientRead(), Format::Format::assemble(), buildFakeRequest(), IdentLookup::checkForAsync(), clientAfterReadingRequests(), clientPinnedConnectionClosed(), clientPinnedConnectionRead(), ClientRequestContext::clientRedirectDone(), clientSocketRecipient(), Server::clientWriteDone(), concurrentRequestQueueFilled(), connStateClosed(), Server::doClientRead(), Server::doneAll(), doneWithControlMsg(), doPeekAndSpliceStep(), endingShutdown(), extendLifetime(), fakeAConnectRequest(), fillConnectionLevelDetails(), getSslContextDone(), getSslContextStart(), handleChunkedRequestBody(), handleRequestBodyData(), handleSslBumpHandshakeError(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), httpsCreate(), httpsEstablish(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), initiateTunneledRequest(), isOpen(), kick(), IdentLookup::LookupDone(), HttpRequest::manager(), ACLIdent::match(), ClientHttpRequest::noteAdaptationAclCheckDone(), ClientHttpRequest::noteBodyProducerAborted(), parseHttpRequest(), parseProxyProtocolHeader(), parseRequests(), parseTlsHandshake(), pinConnection(), postHttpsAccept(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), proxyProtocolError(), quitAfterError(), readNextRequest(), Server::readSomeData(), resetReadTimeout(), PeerSelector::resolveSelected(), sendControlMsg(), serveDelayedError(), setAuth(), splice(), ClientRequestContext::sslBumpAccessCheckDone(), ClientHttpRequest::sslBumpEstablish(), ClientHttpRequest::sslBumpStart(), sslCrtdHandleReply(), start(), startPeekAndSplice(), Server::stopReading(), stopReceiving(), stopSending(), storeTlsContextToCache(), swanSong(), Server::swanSong(), switchToHttps(), terminateAll(), transparent(), tunnelOnError(), tunnelStart(), whenClientIpKnown(), and Server::write().
◆ closeHandler
AsyncCall::Pointer ConnStateData::closeHandler |
Definition at line 153 of file client_side.h.
◆
struct { ... } ConnStateData::flags |
◆ host
char* ConnStateData::host = nullptr |
Definition at line 144 of file client_side.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inBuf
|
inherited |
Definition at line 113 of file Server.h.
Referenced by abortChunkedRequestBody(), abortRequestParsing(), buildFakeRequest(), checkLogging(), consumeInput(), Server::doClientRead(), getSslContextDone(), handleChunkedRequestBody(), handleRequestBodyData(), httpsSslBumpAccessCheckDone(), Server::maybeMakeSpaceAvailable(), mayNeedToReadMoreBody(), parseHttpRequest(), parseProxyProtocolHeader(), parseRequests(), parseTlsHandshake(), Server::readSomeData(), shouldCloseOnEof(), startPeekAndSplice(), stopSending(), terminateAll(), and tunnelStartShoveling().
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ keyLogger
Security::KeyLogger ConnStateData::keyLogger |
Definition at line 384 of file client_side.h.
Referenced by acceptTls().
◆ log_addr
Ip::Address ConnStateData::log_addr |
Definition at line 136 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), ConnStateData(), and postHttpsAccept().
◆ needProxyProtocolHeader_
|
private |
Definition at line 467 of file client_side.h.
Referenced by parseProxyProtocolHeader(), parseRequests(), shouldPreserveClientData(), and start().
◆ parsedBumpedRequestCount
|
private |
Definition at line 481 of file client_side.h.
Referenced by parseRequests(), and shouldPreserveClientData().
◆ parsingTlsHandshake
|
private |
whether we are getting/parsing TLS Hello bytes
Definition at line 479 of file client_side.h.
Referenced by afterClientRead(), parseTlsHandshake(), shouldPreserveClientData(), switchToHttps(), and terminateAll().
◆ peer
CachePeer* ConnStateData::peer = nullptr |
Definition at line 151 of file client_side.h.
◆ peerAccessDenied
bool ConnStateData::peerAccessDenied = false |
Definition at line 150 of file client_side.h.
Referenced by PeerSelector::selectPinned().
◆ pinned
bool ConnStateData::pinned = false |
Definition at line 146 of file client_side.h.
Referenced by HttpRequest::pinnedConnection().
◆
struct { ... } ConnStateData::pinning |
Referenced by borrowPinnedConnection(), clientCheckPinning(), clientPinnedConnectionClosed(), clientPinnedConnectionRead(), handleIdleClientPinnedTlsRead(), initiateTunneledRequest(), kick(), parseRequests(), pinConnection(), pinnedAuth(), HttpRequest::pinnedConnection(), pinnedPeer(), pipelinePrefetchMax(), PeerSelector::selectPinned(), startPinnedConnectionMonitoring(), stopPinnedConnectionMonitoring(), and unpinConnection().
◆ pipeline
|
inherited |
Definition at line 118 of file Server.h.
Referenced by abortChunkedRequestBody(), add(), afterClientRead(), afterClientWrite(), checkLogging(), clientPinnedConnectionRead(), clientSocketRecipient(), Server::clientWriteDone(), concurrentRequestQueueFilled(), doneWithControlMsg(), fillChecklist(), finishDechunkingRequest(), httpsPeeked(), httpsSslBumpStep2AccessCheckDone(), kick(), notePinnedConnectionBecameIdle(), parseRequests(), parseTlsHandshake(), postHttpsAccept(), sendControlMsg(), shouldCloseOnEof(), shouldPreserveClientData(), splice(), startPeekAndSplice(), startShutdown(), terminateAll(), tunnelOnError(), and updateError().
◆ port
AnyP::Port ConnStateData::port |
Definition at line 145 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), buildFakeRequest(), clientReplyContext::buildReplyHeader(), buildSslCertGenerationParams(), clientCheckPinning(), getSslContextDone(), getSslContextStart(), getTlsContextFromCache(), httpsCreate(), HttpRequest::manager(), parseHttpRequest(), postHttpsAccept(), shouldPreserveClientData(), ClientRequestContext::sslBumpAccessCheck(), sslCrtdHandleReply(), start(), startPeekAndSplice(), storeTlsContextToCache(), and ACLFilledChecklist::verifyAle().
◆ preservedClientData
SBuf ConnStateData::preservedClientData |
From-client handshake bytes (including bytes at the beginning of a CONNECT tunnel) which we may need to forward as-is if their syntax does not match the expected TLS or HTTP protocol (on_unsupported_protocol).
Definition at line 349 of file client_side.h.
Referenced by Format::Format::assemble(), parseHttpRequest(), parseTlsHandshake(), splice(), and tunnelOnError().
◆ preservingClientData_
|
protected |
Definition at line 434 of file client_side.h.
Referenced by parseHttpRequest(), parseRequests(), start(), switchToHttps(), and tunnelOnError().
◆ proxyProtocolHeader_
|
private |
Definition at line 470 of file client_side.h.
Referenced by parseProxyProtocolHeader(), postHttpsAccept(), and proxyProtocolHeader().
◆ reader
|
protectedinherited |
Definition at line 127 of file Server.h.
Referenced by Server::doClientRead(), Server::reading(), Server::readSomeData(), and Server::stopReading().
◆ readHandler
AsyncCall::Pointer ConnStateData::readHandler |
Definition at line 152 of file client_side.h.
◆ reading
bool ConnStateData::reading = false |
Definition at line 148 of file client_side.h.
◆ readMore
bool ConnStateData::readMore = true |
Definition at line 139 of file client_side.h.
Referenced by ClientHttpRequest::doCallouts().
◆ receivedFirstByte_
|
inherited |
Definition at line 115 of file Server.h.
Referenced by Server::doClientRead(), receivedFirstByte(), requestTimeout(), and switchToHttps().
◆ serverConnection
Comm::ConnectionPointer ConnStateData::serverConnection |
Definition at line 143 of file client_side.h.
Referenced by clientCheckPinning().
◆ signAlgorithm
|
private |
Definition at line 495 of file client_side.h.
Referenced by buildSslCertGenerationParams(), getSslContextStart(), and sslCrtdHandleReply().
◆ sslBumpCertKey
|
private |
Definition at line 491 of file client_side.h.
Referenced by getSslContextStart(), and sslCrtdHandleReply().
◆ sslBumpMode
Ssl::BumpMode ConnStateData::sslBumpMode = Ssl::bumpEnd |
Definition at line 304 of file client_side.h.
Referenced by concurrentRequestQueueFilled(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), ClientRequestContext::sslBumpAccessCheck(), and ClientHttpRequest::sslBumpStart().
◆ sslCommonName_
|
private |
Definition at line 487 of file client_side.h.
Referenced by buildSslCertGenerationParams(), resetSslCommonName(), and sslCommonName().
◆ sslServerBump
|
private |
Definition at line 494 of file client_side.h.
Referenced by ~ConnStateData(), buildSslCertGenerationParams(), fillConnectionLevelDetails(), getSslContextStart(), httpsPeeked(), parseTlsHandshake(), serveDelayedError(), serverBump(), setServerBump(), sslCrtdHandleReply(), startPeekAndSplice(), and switchToHttps().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ stoppedReceiving_
|
private |
Definition at line 501 of file client_side.h.
Referenced by stoppedReceiving(), stopReceiving(), and stopSending().
◆ stoppedSending_
|
private |
Definition at line 499 of file client_side.h.
Referenced by stoppedSending(), stopReceiving(), and stopSending().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang
bool ConnStateData::swanSang = false |
Definition at line 140 of file client_side.h.
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ switchedToHttps_
|
private |
Definition at line 478 of file client_side.h.
Referenced by doPeekAndSpliceStep(), getSslContextDone(), startPeekAndSplice(), switchedToHttps(), and switchToHttps().
◆ theNotes
|
private |
Connection annotations, clt_conn_tag and other tags are stored here. If set, are propagated to the current and all future master transactions on the connection.
Definition at line 505 of file client_side.h.
Referenced by hasNotes(), and notes().
◆ tlsClientSni_
|
private |
Definition at line 490 of file client_side.h.
Referenced by fakeAConnectRequest(), parseTlsHandshake(), and tlsClientSni().
◆ tlsConnectHostOrIp
|
private |
The TLS server host name as passed in the CONNECT request
Definition at line 485 of file client_side.h.
Referenced by buildSslCertGenerationParams(), getSslContextDone(), httpsPeeked(), initiateTunneledRequest(), prepareTlsSwitchingURL(), sslCrtdHandleReply(), and switchToHttps().
◆ tlsConnectPort
|
private |
Definition at line 486 of file client_side.h.
Referenced by initiateTunneledRequest(), prepareTlsSwitchingURL(), and switchToHttps().
◆ tlsParser
Security::HandshakeParser ConnStateData::tlsParser |
Tls parser to use for client HELLO messages parsing on bumped connections.
Definition at line 308 of file client_side.h.
Referenced by parseTlsHandshake().
◆ transferProtocol
|
inherited |
The transfer protocol currently being spoken on this connection. HTTP/1.x CONNECT, HTTP/1.1 Upgrade and HTTP/2 SETTINGS offer the ability to change protocols on the fly.
Definition at line 107 of file Server.h.
Referenced by parseHttpRequest(), prepareTlsSwitchingURL(), splice(), and switchToHttps().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ writer
|
protectedinherited |
Definition at line 128 of file Server.h.
Referenced by Server::clientWriteDone(), Server::write(), and Server::writing().
◆ zeroReply
bool ConnStateData::zeroReply = false |
Definition at line 149 of file client_side.h.
The documentation for this class was generated from the following files:
- src/client_side.h
- src/client_side.cc