#include <Address.h>
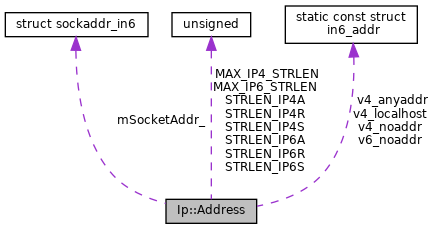
Public Member Functions | |
Constructors | |
Address () | |
Address (const struct in_addr &) | |
Address (const struct sockaddr_in &) | |
Address (const struct in6_addr &) | |
Address (const struct sockaddr_in6 &) | |
Address (const struct hostent &) | |
Address (const struct addrinfo &) | |
Address (const char *) | |
Assignment Operators | |
Address & | operator= (struct sockaddr_in const &s) |
Address & | operator= (struct sockaddr_storage const &s) |
Address & | operator= (struct in_addr const &s) |
Address & | operator= (struct in6_addr const &s) |
Address & | operator= (struct sockaddr_in6 const &s) |
bool | operator= (const struct hostent &s) |
bool | operator= (const struct addrinfo &s) |
bool | operator= (const char *s) |
Static Public Member Functions | |
static std::optional< Address > | Parse (const char *) |
Boolean Operators | |
struct sockaddr_in6 | mSocketAddr_ |
static const unsigned int | STRLEN_IP4A = 16 |
static const unsigned int | STRLEN_IP4R = 28 |
static const unsigned int | STRLEN_IP4S = 21 |
static const unsigned int | MAX_IP4_STRLEN = STRLEN_IP4R |
static const unsigned int | STRLEN_IP6A = 42 |
static const unsigned int | STRLEN_IP6R = 75 |
static const unsigned int | STRLEN_IP6S = 48 |
static const unsigned int | MAX_IP6_STRLEN = STRLEN_IP6R |
static const struct in6_addr | v4_localhost |
static const struct in6_addr | v4_anyaddr |
static const struct in6_addr | v4_noaddr |
static const struct in6_addr | v6_noaddr |
static void | FreeAddr (struct addrinfo *&ai) |
static void | InitAddr (struct addrinfo *&ai) |
static const Address & | NoAddr () |
bool | operator== (Address const &s) const |
bool | operator!= (Address const &s) const |
bool | operator>= (Address const &rhs) const |
bool | operator<= (Address const &rhs) const |
bool | operator> (Address const &rhs) const |
bool | operator< (Address const &rhs) const |
bool | isIPv4 () const |
bool | isIPv6 () const |
bool | isSockAddr () const |
bool | isAnyAddr () const |
bool | isNoAddr () const |
bool | isLocalhost () const |
bool | isSiteLocal6 () const |
bool | isSiteLocalAuto () const |
unsigned short | port () const |
unsigned short | port (unsigned short port) |
void | setAnyAddr () |
NOTE: Does NOT clear the Port stored. Only the Address and Type. More... | |
void | setNoAddr () |
void | setLocalhost () |
void | setEmpty () |
Fast reset of the stored content to what would be after default constructor. More... | |
bool | setIPv4 () |
int | cidr () const |
int | applyMask (const Address &mask) |
bool | applyMask (const unsigned int cidr, int mtype) |
void | applyClientMask (const Address &mask) |
void | turnMaskedBitsOn (const Address &mask) |
char * | toStr (char *buf, const unsigned int blen, int force=AF_UNSPEC) const |
char * | toUrl (char *buf, unsigned int len) const |
unsigned int | toHostStr (char *buf, const unsigned int len) const |
bool | fromHost (const char *hostWithoutPort) |
bool | getReverseString (char buf[MAX_IPSTRLEN], int show_type=AF_UNSPEC) const |
int | matchIPAddr (const Address &rhs) const |
int | compareWhole (const Ip::Address &rhs) const |
void | getAddrInfo (struct addrinfo *&ai, int force=AF_UNSPEC) const |
bool | GetHostByName (const char *s) |
void | getSockAddr (struct sockaddr_storage &addr, const int family) const |
void | getSockAddr (struct sockaddr_in &) const |
bool | getInAddr (struct in_addr &) const |
void | getSockAddr (struct sockaddr_in6 &) const |
void | getInAddr (struct in6_addr &) const |
bool | getReverseString4 (char buf[MAX_IPSTRLEN], const struct in_addr &dat) const |
bool | getReverseString6 (char buf[MAX_IPSTRLEN], const struct in6_addr &dat) const |
void | map4to6 (const struct in_addr &src, struct in6_addr &dest) const |
void | map6to4 (const struct in6_addr &src, struct in_addr &dest) const |
bool | lookupHostIP (const char *s, bool nodns) |
Detailed Description
Constructor & Destructor Documentation
◆ Address() [1/8]
|
inline |
Definition at line 56 of file Address.h.
References setEmpty().
◆ Address() [2/8]
Ip::Address::Address | ( | const struct in_addr & | s | ) |
Definition at line 485 of file Address.cc.
◆ Address() [3/8]
Ip::Address::Address | ( | const struct sockaddr_in & | s | ) |
Definition at line 443 of file Address.cc.
◆ Address() [4/8]
Ip::Address::Address | ( | const struct in6_addr & | s | ) |
Definition at line 499 of file Address.cc.
◆ Address() [5/8]
Ip::Address::Address | ( | const struct sockaddr_in6 & | s | ) |
Definition at line 472 of file Address.cc.
◆ Address() [6/8]
Ip::Address::Address | ( | const struct hostent & | s | ) |
Definition at line 514 of file Address.cc.
◆ Address() [7/8]
Ip::Address::Address | ( | const struct addrinfo & | s | ) |
Definition at line 558 of file Address.cc.
◆ Address() [8/8]
Ip::Address::Address | ( | const char * | s | ) |
Definition at line 379 of file Address.cc.
Member Function Documentation
◆ applyClientMask()
void Ip::Address::applyClientMask | ( | const Address & | mask | ) |
Apply so-called 'privacy masking' to IPv4 addresses, except localhost IP. IPv6 clients use 'privacy addressing' instead.
Definition at line 125 of file Address.cc.
Referenced by ConnStateData::ConnStateData(), and AccessLogEntry::getLogClientIp().
◆ applyMask() [1/2]
Apply a mask to the stored address.
- Parameters
-
mask Netmask format to be bit-mask-AND'd over the stored address.
Definition at line 97 of file Address.cc.
References mSocketAddr_.
Referenced by asnAddNet(), acl_ip_data::DecodeMask(), acl_ip_data::FactoryParse(), acl_ip_data::firstAddress(), networkFromInaddr(), and TestIpAddress::testMasking().
◆ applyMask() [2/2]
Apply a mask to the stored address. CIDR will be converted appropriate to map the stored content.
- Parameters
-
cidr CIDR Mask being applied. As an integer in host format. mtype Type of CIDR mask being applied (AF_INET or AF_INET6)
Definition at line 132 of file Address.cc.
◆ cidr()
int Ip::Address::cidr | ( | ) | const |
Valid results IF and only IF the stored IP address is actually a network bitmask
- Return values
-
N number of bits which are set in the bitmask stored.
Definition at line 54 of file Address.cc.
Referenced by acl_ip_data::DecodeMask(), acl_ip_data::FactoryParse(), printRadixNode(), TestIpAddress::testMasking(), and acl_ip_data::toStr().
◆ compareWhole()
int Ip::Address::compareWhole | ( | const Ip::Address & | rhs | ) | const |
Compare taking IP, port, protocol, etc. into account. Returns an integer less than, equal to, or greater than zero if the object is found, respectively, to be less than, to match, or to be greater than rhs. The exact ordering algorithm is not specified and may change.
Definition at line 744 of file Address.cc.
Referenced by Ipc::OpenListenerParams::operator<().
◆ FreeAddr()
|
static |
Equivalent to the sysem call freeaddrinfo() but for Ip::Address allocated data
Definition at line 706 of file Address.cc.
Referenced by comm_connect_addr(), comm_local_port(), comm_openex(), comm_udp_recvfrom(), comm_udp_sendto(), ftpSendPORT(), getMyHostname(), Ipc::ImportFdIntoComm(), ipc_thread_1(), ipcCreate(), Icmp6::Recv(), Icmp4::Recv(), Icmp6::SendEcho(), Icmp4::SendEcho(), Ipc::SharedListenJoined(), and TestIpAddress::testAddrInfo().
◆ fromHost()
bool Ip::Address::fromHost | ( | const char * | hostWithoutPort | ) |
Empties the address and then slowly imports the IP from a possibly [bracketed] portless host. For the reverse operation, see toHostStr().
- Returns
- whether the conversion was successful
Definition at line 918 of file Address.cc.
References xfree, and xstrdup.
Referenced by ipcacheCheckNumeric(), and AnyP::Uri::parseHost().
◆ getAddrInfo()
void Ip::Address::getAddrInfo | ( | struct addrinfo *& | ai, |
int | force = AF_UNSPEC |
||
) | const |
Get RFC 3493 addrinfo structure from the Ip::Address data for protocol-neutral socket operations. Should be passed a NULL pointer of type struct addrinfo* it will allocate memory for the structures involved. (see FreeAddr() to clear). Defaults to a TCP streaming socket, if other values (such as UDP) are needed the caller MUST override these default settings. Some situations may also require an actual call to the system getaddrinfo() to pull relevant OS details for the socket.
- Ip::Address allocated objects MUST be destructed by Ip::Address::FreeAddr System getaddrinfo() allocated objects MUST be freed with system freeaddrinfo()
- Parameters
-
ai structure to be filled out. force a specific sockaddr type is needed. default: don't care.
Definition at line 619 of file Address.cc.
References IASSERT.
Referenced by comm_connect_addr(), comm_openex(), comm_udp_sendto(), ftpSendPORT(), getMyHostname(), Ipc::ImportFdIntoComm(), Icmp6::SendEcho(), Icmp4::SendEcho(), Ipc::SharedListenJoined(), and TestIpAddress::testAddrInfo().
◆ GetHostByName()
bool Ip::Address::GetHostByName | ( | const char * | s | ) |
Lookup a Host by Name. Equivalent to system call gethostbyname(char*)
- Parameters
-
s The textual FQDN of the host being located.
- Return values
-
true lookup was successful and an IPA was located. false lookup failed or FQDN has no IP associated.
Definition at line 392 of file Address.cc.
Referenced by ProxyProtocol::One::ExtractIp(), GetHostWithPort(), and parse_address().
◆ getInAddr() [1/2]
void Ip::Address::getInAddr | ( | struct in6_addr & | buf | ) | const |
Definition at line 1034 of file Address.cc.
◆ getInAddr() [2/2]
bool Ip::Address::getInAddr | ( | struct in_addr & | buf | ) | const |
Definition at line 1040 of file Address.cc.
References assert, DBG_IMPORTANT, and debugs.
Referenced by addr2oid(), idnsPTRLookup(), Ip::Intercept::IpfInterception(), Eui::Eui64::lookupSlaac(), ClassCHostPool::makeHostKey(), IndividualPool::makeKey(), ClassCNetPool::makeKey(), ClassCHostPool::makeKey(), netdbBinaryExchange(), Ip::Intercept::PfInterception(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testMasking(), TestIpAddress::testStringConstructor(), and wccp2Init().
◆ getReverseString()
bool Ip::Address::getReverseString | ( | char | buf[MAX_IPSTRLEN], |
int | show_type = AF_UNSPEC |
||
) | const |
Convert the content into a Reverse-DNS string. The buffer sent MUST be allocated large enough to hold the resulting string. Name truncation will occur if buf does not have enough space. The constant MAX_IPSTRLEN is defined to provide for sizing arrays correctly.
- Parameters
-
show_type may be one of: AF_INET, AF_INET6 for the format of rDNS string wanted. AF_UNSPEC the default displays the IP in its most advanced native form. buf buffer to receive the text string output.
Definition at line 358 of file Address.cc.
References DBG_CRITICAL, debugs, and MAX_IPSTRLEN.
Referenced by TestIpAddress::testgetReverseString().
◆ getReverseString4()
|
private |
Definition at line 346 of file Address.cc.
References int.
◆ getReverseString6()
|
private |
Definition at line 319 of file Address.cc.
◆ getSockAddr() [1/3]
void Ip::Address::getSockAddr | ( | struct sockaddr_in & | buf | ) | const |
Definition at line 966 of file Address.cc.
References assert, DBG_CRITICAL, and debugs.
◆ getSockAddr() [2/3]
void Ip::Address::getSockAddr | ( | struct sockaddr_in6 & | buf | ) | const |
Definition at line 986 of file Address.cc.
◆ getSockAddr() [3/3]
void Ip::Address::getSockAddr | ( | struct sockaddr_storage & | addr, |
const int | family | ||
) | const |
Definition at line 944 of file Address.cc.
References assert, DBG_CRITICAL, debugs, and IASSERT.
Referenced by Eui::Eui48::lookup(), Ip::Intercept::NetfilterInterception(), TestIpAddress::testCopyConstructor(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), wccp2HandleUdp(), and wccpConnectionOpen().
◆ InitAddr()
|
static |
Initializes an empty addrinfo properly for use. It is intended for use in cases such as getsockopt() where the addrinfo is about to be changed and the stored details may not match the new ones coming.
- Parameters
-
ai addrinfo struct to be initialized as AF_UNSPEC with large address buffer
Definition at line 688 of file Address.cc.
Referenced by comm_local_port(), comm_udp_recvfrom(), ipc_thread_1(), ipcCreate(), Icmp6::Recv(), and Icmp4::Recv().
◆ isAnyAddr()
bool Ip::Address::isAnyAddr | ( | ) | const |
Content-neutral test for whether the specific IP case ANY_ADDR is stored. This is the default content of a new undefined Ip::Address object.
- Return values
-
true IPv4 0.0.0.0 true IPv6 :: false anything else.
Definition at line 190 of file Address.cc.
Referenced by aclIpAddrNetworkCompare(), asnMatchIp(), client_Inst(), Adaptation::Ecap::XactionRep::clientIpValue(), comm_apply_flags(), comm_open(), FindListeningPortAddress(), IdleConnList::findUseable(), fqdncache_gethostbyaddr(), getMyHostname(), getOutgoingAddress(), PeerSelector::handleHtcpParentMiss(), PeerSelector::handleIcpParentMiss(), Ftp::Server::handleUserRequest(), htcpOpenPorts(), icpOpenPorts(), internalRemoteUri(), acl_ip_data::lastAddress(), Adaptation::Icap::ModXact::makeRequestHeaders(), netdbExchangeHandleReply(), operator<(), operator<=(), Ftp::ParseIpPort(), Ftp::ParseProtoIpPort(), PeerSelector::selectSomeNeighborReplies(), Ssl::setClientSNI(), snmpOpenPorts(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testMasking(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), TestIpAddress::testStringConstructor(), acl_ip_data::toStr(), wccp2Init(), wccpConnectionOpen(), and wccpInit().
◆ isIPv4()
bool Ip::Address::isIPv4 | ( | ) | const |
Test whether content can be used as an IPv4 address
- Return values
-
true if content was received as an IPv4-Mapped address false if content was received as a non-mapped IPv6 native address.
Definition at line 178 of file Address.cc.
Referenced by Comm::TcpAcceptor::acceptInto(), Format::Format::assemble(), client_Inst(), comm_connect_addr(), ResolvedPeers::ConnectionFamily(), Ftp::Server::createDataConnection(), acl_ip_data::DecodeMask(), Log::TcpLogger::doConnect(), ftpSendPORT(), getOutgoingAddress(), VectorPool::id(), ClassCHostPool::id(), idnsInitVC(), Dns::Init(), logfile_mod_udp_open(), ClassCHostPool::makeHostKey(), IndividualPool::makeKey(), ClassCNetPool::makeKey(), ClassCHostPool::makeKey(), ACLARP::match(), ACLIP::match(), netdbBinaryExchange(), netdbExchangeUpdatePeer(), PeerSelector::noteIp(), Ip::Intercept::ProbeForTproxy(), IcmpPinger::Recv(), Ip::Qos::setSockTos(), snmp_meshCtblFn(), snmp_meshPtblFn(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testMasking(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), TestIpAddress::testStringConstructor(), and acl_ip_data::toStr().
◆ isIPv6()
bool Ip::Address::isIPv6 | ( | ) | const |
Test whether content can be used as an IPv6 address.
- Return values
-
true if content is a non IPv4-mapped address. false if content is IPv4-mapped.
Definition at line 184 of file Address.cc.
Referenced by Comm::TcpAcceptor::acceptInto(), addr2oid(), comm_openex(), idnsPTRLookup(), Dns::Init(), ipcacheAddEntryFromHosts(), Ip::Intercept::IpfInterception(), ACLEui64::match(), ACLIP::match(), Ip::Intercept::NetfilterInterception(), networkFromInaddr(), Ftp::ParseProtoIpPort(), Ip::Intercept::PfInterception(), Ip::Intercept::ProbeForTproxy(), IcmpPinger::Recv(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testMasking(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), and TestIpAddress::testStringConstructor().
◆ isLocalhost()
bool Ip::Address::isLocalhost | ( | ) | const |
Content-neutral test for whether the specific IP case LOCALHOST is stored. This is the default content of a new undefined Ip::Address object.
- Return values
-
true IPv4 127.0.0.1 true IPv6 ::1 false anything else.
Definition at line 269 of file Address.cc.
◆ isNoAddr()
bool Ip::Address::isNoAddr | ( | ) | const |
Content-neutral test for whether the specific IP case NO_ADDR is stored.
- Return values
-
true IPv4 255.255.255.255 true IPv6 ffff:ffff:ffff:ffff:ffff:ffff:ffff:ffff false anything else.
Definition at line 304 of file Address.cc.
Referenced by asnMatchIp(), Adaptation::Ecap::XactionRep::clientIpValue(), comm_apply_flags(), DelayId::DelayClient(), AccessLogEntry::detailCodeContext(), acl_ip_data::firstAddress(), fqdncache_gethostbyaddr(), AccessLogEntry::getLogClientIp(), htcpIncomingConnectionOpened(), htcpOpenPorts(), HttpStateData::httpBuildRequestHeader(), icpIncomingConnectionOpened(), icpOpenPorts(), idnsInitVC(), Dns::Init(), acl_ip_data::lastAddress(), Adaptation::Icap::ModXact::makeRequestHeaders(), Comm::operator<<(), operator>(), operator>=(), snmpOpenPorts(), FwdState::Start(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testMasking(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), TestIpAddress::testStringConstructor(), acl_ip_data::toStr(), and tunnelStart().
◆ isSiteLocal6()
bool Ip::Address::isSiteLocal6 | ( | ) | const |
Test whether content is an IPv6 Site-Local address.
- Return values
-
true if address begins with fd00::/8. false if –disable-ipv6 has been compiled. false if address does not match fd00::/8
Definition at line 287 of file Address.cc.
Referenced by Eui::Eui64::lookupSlaac().
◆ isSiteLocalAuto()
bool Ip::Address::isSiteLocalAuto | ( | ) | const |
Test whether content is an IPv6 address with SLAAC EUI-64 embedded.
- Return values
-
true if address matches ::ff:fe00:0 false if –disable-ipv6 has been compiled. false if address does not match ::ff:fe00:0
Definition at line 297 of file Address.cc.
Referenced by Eui::Eui64::lookupSlaac().
◆ isSockAddr()
bool Ip::Address::isSockAddr | ( | ) | const |
Test whether content can be used as a Socket address.
- Return values
-
true if address AND port are both set true if content was received as a Socket address with port false if port in unset (zero)
Definition at line 172 of file Address.cc.
Referenced by TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), and TestIpAddress::testStringConstructor().
◆ lookupHostIP()
|
private |
Definition at line 398 of file Address.cc.
References debugs, Ip::EnableIpv6, and port.
Referenced by Parse().
◆ map4to6()
|
private |
Definition at line 999 of file Address.cc.
◆ map6to4()
|
private |
Definition at line 1020 of file Address.cc.
◆ matchIPAddr()
Test how two IP relate to each other.
- Return values
-
0 IP are equal 1 IP rhs is greater (numerically) than that stored. -1 IP rhs is less (numerically) than that stored.
Definition at line 723 of file Address.cc.
References mSocketAddr_.
Referenced by IdleConnList::findUseable(), and TestIpAddress::testBooleans().
◆ NoAddr()
|
inlinestatic |
- Returns
- an Address with true isNoAddr()
- See also
- isNoAddr() for more details
Definition at line 321 of file Address.h.
References v6_noaddr.
Referenced by clientBuildError(), and Acl::SplayInserter< DataValue >::MakeCombinedValue().
◆ operator!=()
bool Ip::Address::operator!= | ( | Address const & | s | ) | const |
Definition at line 756 of file Address.cc.
References operator==().
◆ operator<()
bool Ip::Address::operator< | ( | Address const & | rhs | ) | const |
Definition at line 789 of file Address.cc.
References isAnyAddr().
◆ operator<=()
bool Ip::Address::operator<= | ( | Address const & | rhs | ) | const |
Definition at line 762 of file Address.cc.
References isAnyAddr().
◆ operator=() [1/8]
bool Ip::Address::operator= | ( | const char * | s | ) |
Interprets the given c-string as an IP address and, upon success, assigns that address. Does nothing if that interpretation fails.
- Returns
- whether the assignment was performed
- Deprecated:
- Use Parse() instead.
Definition at line 386 of file Address.cc.
◆ operator=() [2/8]
bool Ip::Address::operator= | ( | const struct addrinfo & | s | ) |
Definition at line 565 of file Address.cc.
References assert.
◆ operator=() [3/8]
bool Ip::Address::operator= | ( | const struct hostent & | s | ) |
Definition at line 521 of file Address.cc.
References IASSERT.
◆ operator=() [4/8]
Ip::Address & Ip::Address::operator= | ( | struct in6_addr const & | s | ) |
Definition at line 506 of file Address.cc.
◆ operator=() [5/8]
Ip::Address & Ip::Address::operator= | ( | struct in_addr const & | s | ) |
Definition at line 492 of file Address.cc.
◆ operator=() [6/8]
Ip::Address & Ip::Address::operator= | ( | struct sockaddr_in const & | s | ) |
Definition at line 450 of file Address.cc.
◆ operator=() [7/8]
Ip::Address & Ip::Address::operator= | ( | struct sockaddr_in6 const & | s | ) |
Definition at line 479 of file Address.cc.
◆ operator=() [8/8]
Ip::Address & Ip::Address::operator= | ( | struct sockaddr_storage const & | s | ) |
Definition at line 459 of file Address.cc.
◆ operator==()
bool Ip::Address::operator== | ( | Address const & | s | ) | const |
Definition at line 750 of file Address.cc.
◆ operator>()
bool Ip::Address::operator> | ( | Address const & | rhs | ) | const |
Definition at line 780 of file Address.cc.
References isNoAddr().
◆ operator>=()
bool Ip::Address::operator>= | ( | Address const & | rhs | ) | const |
Definition at line 771 of file Address.cc.
References isNoAddr().
◆ Parse()
|
static |
Creates an IP address object by parsing a given c-string. Accepts all three forms of IPv6 addresses from RFC 4291 section 2.2. Examples of valid input: 0, 1.0, 1.2.3.4, ff01::101, and ::FFFF:129.144.52.38. Fails if input contains characters before or after a valid IP address. For example, fails if given a bracketed IPv6 address (e.g., [::1]).
- Returns
- std::nullopt if parsing fails
Definition at line 44 of file Address.cc.
References lookupHostIP().
Referenced by Ssl::ParseAsSimpleDomainNameOrIp().
◆ port() [1/2]
unsigned short Ip::Address::port | ( | ) | const |
Retrieve the Port if stored.
- Return values
-
0 Port is unset or an error occurred. n Port associated with this address in host native -endian.
Definition at line 798 of file Address.cc.
Referenced by Comm::TcpAcceptor::acceptInto(), Format::Format::assemble(), ClientRequestContext::clientAccessCheck(), comm_apply_flags(), comm_connect_addr(), comm_local_port(), comm_open(), Ftp::Client::connectDataChannel(), Ftp::Server::createDataConnection(), Adaptation::Icap::Xaction::dnsLookupDone(), ProxyProtocol::One::ExtractPort(), ConnStateData::fakeAConnectRequest(), FindListeningPortNumber(), IdleConnList::findUseable(), ftpOpenListenSocket(), GetHostWithPort(), getOutgoingAddress(), Ftp::Client::handleEpsvReply(), ClientRequestContext::hostHeaderVerify(), htcpHandleTstResponse(), htcpOpenPorts(), icpOpenPorts(), idnsFromKnownNameserver(), ConnStateData::initiateTunneledRequest(), Ftp::Server::listenForDataConnection(), Eui::Eui48::lookup(), PeerSelector::noteIp(), PeerPoolMgr::openNewConnection(), Comm::operator<<(), Ftp::ParseIpPort(), Ftp::ParseProtoIpPort(), peerDNSConfigure(), peerProbeConnect(), Ip::Intercept::PfInterception(), ConnStateData::postHttpsAccept(), prepareAcceleratedURL(), prepareTransparentURL(), Ip::Intercept::ProbeForTproxy(), snmpOpenPorts(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testCopyConstructor(), TestIpAddress::testDefaults(), TestIpAddress::testHostentConstructor(), TestIpAddress::testInAddr6Constructor(), TestIpAddress::testInAddrConstructor(), TestIpAddress::testsetEmpty(), TestIpAddress::testSockAddr6Constructor(), TestIpAddress::testSockAddrConstructor(), TestIpAddress::testStringConstructor(), wccp2ConnectionOpen(), wccp2HereIam(), wccpConnectionOpen(), and whichPeer().
◆ port() [2/2]
unsigned short Ip::Address::port | ( | unsigned short | port | ) |
Set the Port value for an address. Replaces any previously existing Port value.
- Parameters
-
port Port being assigned in host native -endian.
- Return values
-
0 Port is unset or an error occurred. n Port associated with this address in host native -endian.
Definition at line 804 of file Address.cc.
◆ setAnyAddr()
void Ip::Address::setAnyAddr | ( | ) |
Set object to contain the specific IP case ANY_ADDR (format-neutral). see isAnyAddr() for more detail.
Definition at line 197 of file Address.cc.
Referenced by client_Inst(), Ftp::Server::createDataConnection(), Log::TcpLogger::doConnect(), acl_ip_data::FactoryParse(), GetHostWithPort(), logfile_mod_udp_open(), netdbExchangeHandleReply(), parse_address(), TestIpAddress::testBooleans(), TestIpAddress::testMasking(), and TestIpAddress::testtoStr().
◆ setEmpty()
void Ip::Address::setEmpty | ( | ) |
NOTE: completely empties the Ip::Address structure. Address, Port, Type, everything.
Definition at line 204 of file Address.cc.
Referenced by ACLFilledChecklist::ACLFilledChecklist(), Address(), AnyP::Uri::clear(), free_address(), HttpRequest::init(), ipcCreate(), ACLIP::match(), peerDNSConfigure(), and TestIpAddress::testsetEmpty().
◆ setIPv4()
bool Ip::Address::setIPv4 | ( | ) |
Require an IPv4-only address for this usage. Converts the object to prefer only IPv4 output.
- Return values
-
true Content can be IPv4 false Content CANNOT be IPv4
Definition at line 244 of file Address.cc.
Referenced by comm_local_port(), comm_openex(), Ftp::Server::createDataConnection(), Log::TcpLogger::doConnect(), getOutgoingAddress(), htcpOpenPorts(), icpOpenPorts(), idnsInitVC(), Dns::Init(), logfile_mod_udp_open(), IcmpSquid::Open(), parse_externalAclHelper(), Ip::Intercept::ProbeForTproxy(), snmpOpenPorts(), wccp2ConnectionOpen(), wccp2HandleUdp(), and wccpConnectionOpen().
◆ setLocalhost()
void Ip::Address::setLocalhost | ( | ) |
Set object to contain the specific IP case LOCALHOST (format-neutral). see isLocalhost() for more detail.
Definition at line 275 of file Address.cc.
References Ip::EnableIpv6.
Referenced by external_acl::external_acl(), DiskdIOStrategy::init(), logfile_mod_daemon_open(), IcmpSquid::Open(), and unlinkdInit().
◆ setNoAddr()
void Ip::Address::setNoAddr | ( | ) |
Set object to contain the specific IP case NO_ADDR (format-neutral). see isNoAddr() for more detail.
Definition at line 312 of file Address.cc.
Referenced by asnAddNet(), AccessLogEntry::CacheDetails::CacheDetails(), acl_ip_data::DecodeMask(), acl_ip_data::FactoryParse(), htcpAccessAllowed(), icpAccessAllowed(), Acl::DestinationAsnCheck::match(), parse_address(), TestIpAddress::testBooleans(), TestIpAddress::testMasking(), and TestIpAddress::testtoStr().
◆ toHostStr()
Return a properly hostname formatted copy of the address Provides a URL formatted version of the content. If buffer is not large enough the data is truncated silently. eg. 127.0.0.1 (IPv4) or [::1] (IPv6)
- Parameters
-
buf Allocated buffer to write address to len byte length of buffer available for writing.
- Returns
- amount of buffer filled.
Definition at line 862 of file Address.cc.
Referenced by ConnStateData::fakeAConnectRequest(), Ftp::Server::handleUserRequest(), internalRemoteUri(), prepareAcceleratedURL(), prepareTransparentURL(), and TestIpAddress::testBugNullingDisplay().
◆ toStr()
Return the ASCII equivalent of the address Semantically equivalent to the IPv4 inet_ntoa() eg. 127.0.0.1 (IPv4) or ::1 (IPv6) But for memory safety it requires a buffer as input instead of producing one magically. If buffer is not large enough the data is truncated silently.
- Parameters
-
buf Allocated buffer to write address to len byte length of buffer available for writing. force (optional) require the IPA in a specific format.
- Returns
- pointer to buffer received.
Definition at line 812 of file Address.cc.
References assert, DBG_CRITICAL, debugs, and min().
Referenced by Comm::TcpAcceptor::acceptInto(), Format::Format::assemble(), client_entry(), clientdbCutoffDenied(), clientdbEstablished(), clientdbGetInfo(), clientdbUpdate(), ClientInfo::ClientInfo(), Adaptation::Ecap::XactionRep::clientIpValue(), comm_connect_addr(), ErrorState::compileLegacyCode(), dump_address(), dump_IpAddress_list(), fqdncache_gethostbyaddr(), fqdncache_nbgethostbyaddr(), AccessLogEntry::getLogClientIp(), HttpStateData::httpBuildRequestHeader(), idnsPTRLookup(), ConnStateData::initiateTunneledRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), Acl::ServerNameMatcher::matchIp(), CommonNamesPrinter::matchIp(), netdbHashInsert(), netdbLookupAddr(), peerSourceHashSelectParent(), ConnStateData::postHttpsAccept(), printRadixNode(), snmp_meshCtblFn(), snmp_meshPtblFn(), Log::Format::SquidIcap(), Log::Format::SquidNative(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testMasking(), TestIpAddress::testtoStr(), and acl_ip_data::toStr().
◆ toUrl()
char * Ip::Address::toUrl | ( | char * | buf, |
unsigned int | len | ||
) | const |
Return the ASCII equivalent of the address:port combination Provides a URL formatted version of the content. If buffer is not large enough the data is truncated silently. eg. 127.0.0.1:80 (IPv4) or [::1]:80 (IPv6)
- Parameters
-
buf Allocated buffer to write address:port to len byte length of buffer available for writing.
- Returns
- pointer to buffer received.
Definition at line 894 of file Address.cc.
References port.
Referenced by PconnPool::key(), peerCountMcastPeersCreateAndSend(), ConnStateData::pinConnection(), fde::remoteAddr(), statClientRequests(), TestIpAddress::testBugNullingDisplay(), TestIpAddress::testtoUrl_fromInAddr(), and TestIpAddress::testtoUrl_fromSockAddr().
◆ turnMaskedBitsOn()
void Ip::Address::turnMaskedBitsOn | ( | const Address & | mask | ) |
Set bits of the stored IP address on if they are on in the given mask. For example, supplying a /24 mask turns 127.0.0.1 into 127.0.0.255.
- See also
- applyMask(const Address &)
Definition at line 115 of file Address.cc.
References mSocketAddr_.
Referenced by acl_ip_data::lastAddress().
Member Data Documentation
◆ MAX_IP4_STRLEN
|
staticprivate |
◆ MAX_IP6_STRLEN
|
staticprivate |
◆ mSocketAddr_
|
private |
Definition at line 355 of file Address.h.
Referenced by applyMask(), matchIPAddr(), and turnMaskedBitsOn().
◆ STRLEN_IP4A
|
staticprivate |
◆ STRLEN_IP4R
|
staticprivate |
◆ STRLEN_IP4S
|
staticprivate |
◆ STRLEN_IP6A
|
staticprivate |
◆ STRLEN_IP6R
|
staticprivate |
◆ STRLEN_IP6S
|
staticprivate |
◆ v4_anyaddr
|
staticprivate |
◆ v4_localhost
|
staticprivate |
◆ v4_noaddr
|
staticprivate |
◆ v6_noaddr
|
staticprivate |
The documentation for this class was generated from the following files:
- src/ip/Address.h
- src/ip/Address.cc
- src/tests/stub_libip.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products