#include <ServiceRep.h>
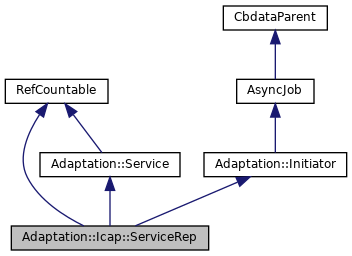
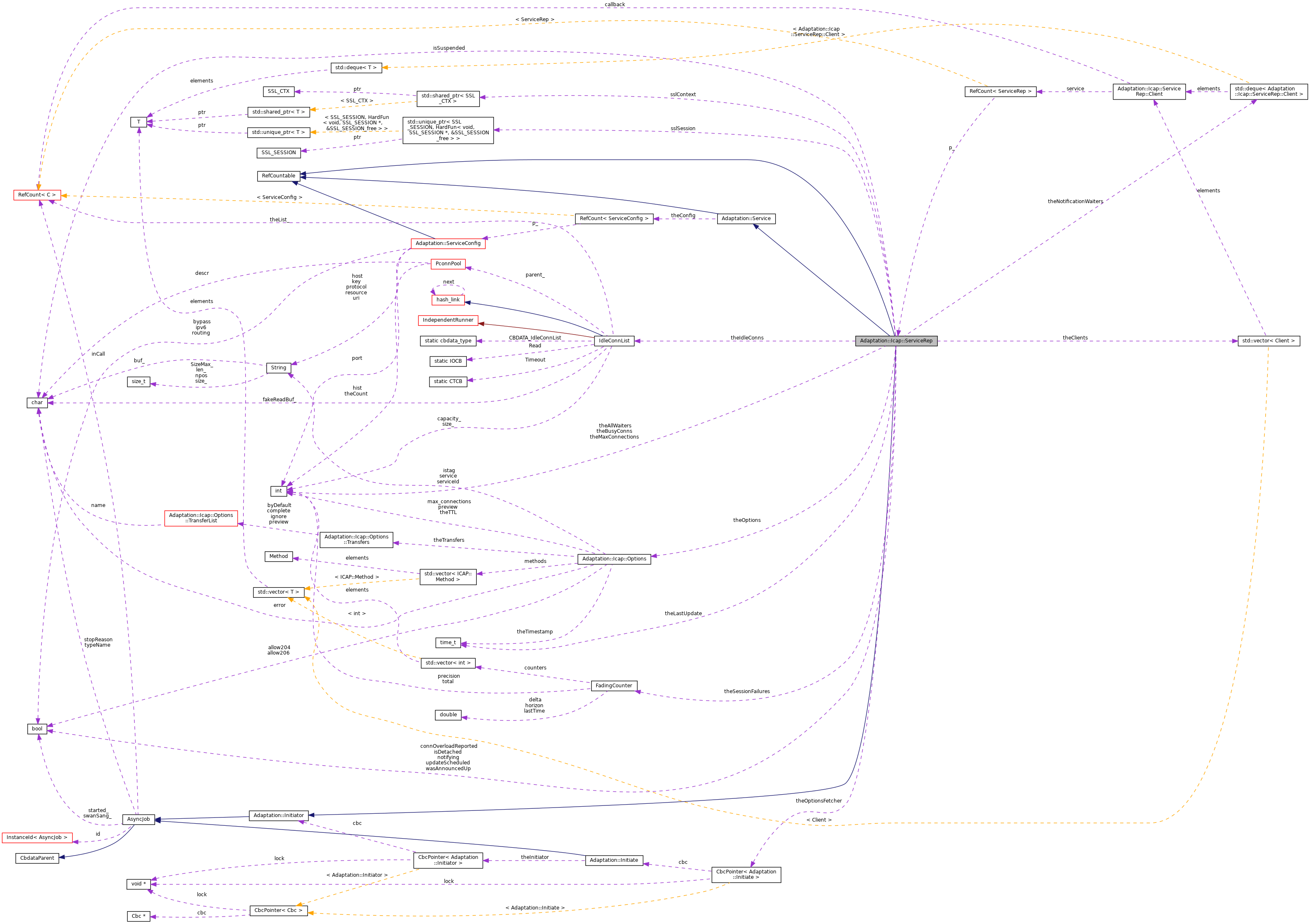
Classes | |
struct | Client |
Public Types | |
typedef RefCount< ServiceRep > | Pointer |
typedef String | Id |
Public Member Functions | |
ServiceRep (const ServiceConfigPointer &aConfig) | |
~ServiceRep () override | |
void | finalize () override |
bool | probed () const override |
bool | up () const override |
bool | availableForNew () const |
a new transaction may start communicating with the service More... | |
bool | availableForOld () const |
a transaction notified about connection slot availability may start communicating with the service More... | |
Initiate * | makeXactLauncher (Http::Message *virginHeader, HttpRequest *virginCause, AccessLogEntry::Pointer &alp) override |
void | callWhenAvailable (AsyncCall::Pointer &cb, bool priority=false) |
void | callWhenReady (AsyncCall::Pointer &cb) |
bool | wantsUrl (const SBuf &urlPath) const override |
bool | wantsPreview (const SBuf &urlPath, size_t &wantedSize) const |
bool | allows204 () const |
bool | allows206 () const |
Comm::ConnectionPointer | getIdleConnection (bool isRetriable) |
void | putConnection (const Comm::ConnectionPointer &conn, bool isReusable, bool sendReset, const char *comment) |
void | noteConnectionUse (const Comm::ConnectionPointer &conn) |
void | noteConnectionFailed (const char *comment) |
void | noteFailure () override |
void | noteGoneWaiter () |
An xaction is not waiting any more for service to be available. More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
void | detach () override |
bool | detached () const override |
whether detached() was called More... | |
void | noteTimeToUpdate () |
void | noteTimeToNotify () |
void | noteAdaptationAnswer (const Answer &answer) override |
virtual bool | broken () const |
bool | wants (const ServiceFilter &filter) const |
const ServiceConfig & | cfg () const |
virtual void | noteAdaptationAclCheckDone (Adaptation::ServiceGroupPointer group) |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
Security::ContextPointer | sslContext |
Security::FuturePeerContext | tlsContext |
Security::SessionStatePointer | sslSession |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
ServiceConfig & | writeableCfg () |
CbcPointer< Initiate > | initiateAdaptation (Initiate *x) |
< starts freshly created initiate and returns a safe pointer to it More... | |
void | clearAdaptation (CbcPointer< Initiate > &x) |
clears the pointer (does not call announceInitiatorAbort) More... | |
void | announceInitiatorAbort (CbcPointer< Initiate > &x) |
inform the transaction about abnormal termination and clear the pointer More... | |
bool | initiated (const CbcPointer< AsyncJob > &job) const |
Must(initiated(initiate)) instead of Must(initiate.set()), for clarity. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual void | start () |
called by AsyncStart; do not call directly More... | |
virtual void | swanSong () |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Types | |
typedef std::vector< Client > | Clients |
Private Member Functions | |
CBDATA_CHILD (ServiceRep) | |
ICAP::Method | parseMethod (const char *) const |
ICAP::VectPoint | parseVectPoint (const char *) const |
void | suspend (const char *reason) |
bool | hasOptions () const |
bool | needNewOptions () const |
time_t | optionsFetchTime () const |
void | scheduleUpdate (time_t when) |
void | scheduleNotification () |
void | startGettingOptions () |
void | handleNewOptions (Options *newOptions) |
void | changeOptions (Options *newOptions) |
void | checkOptions () |
void | announceStatusChange (const char *downPhrase, bool important) const |
void | setMaxConnections () |
Set the maximum allowed connections for the service. More... | |
int | excessConnections () const |
The number of connections which excess the Max-Connections limit. More... | |
int | availableConnections () const |
void | busyCheckpoint () |
const char * | status () const override |
internal cleanup; do not call directly More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
Clients | theClients |
Options * | theOptions |
CbcPointer< Adaptation::Initiate > | theOptionsFetcher |
time_t | theLastUpdate |
std::deque< Client > | theNotificationWaiters |
int | theBusyConns |
int | theAllWaiters |
int | theMaxConnections |
the maximum allowed connections to the service More... | |
bool | connOverloadReported |
whether we reported exceeding theMaxConnections More... | |
IdleConnList * | theIdleConns |
idle persistent connection pool More... | |
FadingCounter | theSessionFailures |
const char * | isSuspended |
bool | notifying |
bool | updateScheduled |
bool | wasAnnouncedUp |
bool | isDetached |
ServiceConfigPointer | theConfig |
Detailed Description
Definition at line 59 of file ServiceRep.h.
Member Typedef Documentation
◆ Clients
|
private |
Definition at line 127 of file ServiceRep.h.
◆ Id
|
inherited |
◆ Pointer
Definition at line 65 of file ServiceRep.h.
Constructor & Destructor Documentation
◆ ServiceRep()
|
explicit |
Definition at line 33 of file ServiceRep.cc.
References setMaxConnections(), and theIdleConns.
◆ ~ServiceRep()
|
override |
Definition at line 50 of file ServiceRep.cc.
References Must, and SWALLOW_EXCEPTIONS.
Member Function Documentation
◆ allows204()
bool Adaptation::Icap::ServiceRep::allows204 | ( | ) | const |
Definition at line 345 of file ServiceRep.cc.
References Must.
◆ allows206()
bool Adaptation::Icap::ServiceRep::allows206 | ( | ) | const |
Definition at line 351 of file ServiceRep.cc.
References Must.
◆ announceInitiatorAbort()
|
protectedinherited |
Definition at line 38 of file Initiator.cc.
References CallJobHere.
Referenced by Client::cleanAdaptation(), and ClientHttpRequest::~ClientHttpRequest().
◆ announceStatusChange()
|
private |
Definition at line 522 of file ServiceRep.cc.
References debugs.
◆ availableConnections()
|
private |
The available connections slots to the ICAP server
- Returns
- the available slots, or -1 if there is no limit on allowed connections
Definition at line 199 of file ServiceRep.cc.
References DBG_IMPORTANT, debugs, max(), and Adaptation::srvForce.
◆ availableForNew()
bool Adaptation::Icap::ServiceRep::availableForNew | ( | ) | const |
Definition at line 306 of file ServiceRep.cc.
References Must.
◆ availableForOld()
bool Adaptation::Icap::ServiceRep::availableForOld | ( | ) | const |
Definition at line 316 of file ServiceRep.cc.
References Must.
◆ broken()
|
virtualinherited |
Definition at line 30 of file Service.cc.
◆ busyCheckpoint()
|
private |
If there are xactions waiting for the service to be available, notify as many xactions as the available connections slots.
Definition at line 247 of file ServiceRep.cc.
References Adaptation::Icap::ServiceRep::Client::callback, debugs, and ScheduleCallHere.
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtual |
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ callWhenAvailable()
void Adaptation::Icap::ServiceRep::callWhenAvailable | ( | AsyncCall::Pointer & | cb, |
bool | priority = false |
||
) |
Definition at line 402 of file ServiceRep.cc.
References Adaptation::Icap::ServiceRep::Client::callback, debugs, Must, and Adaptation::Icap::ServiceRep::Client::service.
◆ callWhenReady()
void Adaptation::Icap::ServiceRep::callWhenReady | ( | AsyncCall::Pointer & | cb | ) |
Definition at line 420 of file ServiceRep.cc.
References Adaptation::Icap::ServiceRep::Client::callback, debugs, Must, and Adaptation::Icap::ServiceRep::Client::service.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ cfg()
|
inlineinherited |
Definition at line 51 of file Service.h.
References Adaptation::Service::theConfig.
Referenced by Adaptation::ServiceGroup::checkUniqueness(), Adaptation::Icap::Xaction::dnsLookupDone(), Adaptation::ServiceGroup::finalize(), Adaptation::Icap::Xaction::finalizeLogInfo(), Adaptation::Icap::ModXact::finalizeLogInfo(), Adaptation::ServiceGroup::findService(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::Xaction::openConnection(), Adaptation::Service::Service(), Adaptation::DynamicServiceChain::Split(), and Adaptation::Iterator::step().
◆ changeOptions()
|
private |
Definition at line 454 of file ServiceRep.cc.
References debugs, and squid_curtime.
◆ checkOptions()
|
private |
Definition at line 469 of file ServiceRep.cc.
References String::append(), DBG_IMPORTANT, debugs, int, Adaptation::methodStr(), and squid_curtime.
◆ clearAdaptation()
|
protectedinherited |
Definition at line 32 of file Initiator.cc.
References CbcPointer< Cbc >::clear().
Referenced by ClientHttpRequest::handleAdaptedHeader(), Client::noteAdaptationAnswer(), and ClientHttpRequest::noteAdaptationAnswer().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ detach()
|
overridevirtual |
called when removed from the config; the service will be auto-destroyed when the last refcounting user leaves
Implements Adaptation::Service.
Definition at line 718 of file ServiceRep.cc.
References debugs.
◆ detached()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 725 of file ServiceRep.cc.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
inlineoverridevirtual |
Reimplemented from AsyncJob.
Definition at line 101 of file ServiceRep.h.
References AsyncJob::doneAll().
◆ excessConnections()
|
private |
Definition at line 223 of file ServiceRep.cc.
◆ finalize()
|
overridevirtual |
Reimplemented from Adaptation::Service.
Definition at line 60 of file ServiceRep.cc.
References debugs, DEFAULT_ICAP_PORT, DEFAULT_ICAPS_PORT, Adaptation::Service::finalize(), Adaptation::Config::oldest_service_failure, and Adaptation::Icap::TheConfig.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ getIdleConnection()
Comm::ConnectionPointer Adaptation::Icap::ServiceRep::getIdleConnection | ( | bool | isRetriable | ) |
- Returns
- an idle persistent ICAP connection or nil
Definition at line 118 of file ServiceRep.cc.
References debugs.
Referenced by Adaptation::Icap::Xaction::openConnection().
◆ handleNewOptions()
|
private |
Definition at line 575 of file ServiceRep.cc.
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ hasOptions()
|
private |
Definition at line 296 of file ServiceRep.cc.
◆ initiateAdaptation()
|
protectedinherited |
Definition at line 23 of file Initiator.cc.
References Adaptation::Initiate::initiator().
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ initiated()
|
inlineprotectedinherited |
Definition at line 52 of file Initiator.h.
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ makeXactLauncher()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 671 of file ServiceRep.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ needNewOptions()
|
private |
Definition at line 449 of file ServiceRep.cc.
◆ noteAdaptationAclCheckDone()
|
virtualinherited |
AccessCheck calls this back with a possibly nil service group to signal whether adaptation is needed and where it should start.
Reimplemented in ClientHttpRequest, and Client.
Definition at line 17 of file Initiator.cc.
References Must.
◆ noteAdaptationAnswer()
|
overridevirtual |
called with the initial adaptation decision (adapt, block, error); virgin and/or adapted body transmission may continue after this
Implements Adaptation::Initiator.
Definition at line 537 of file ServiceRep.cc.
References Adaptation::Answer::akError, Adaptation::Answer::akForward, Adaptation::Icap::Options::configure(), DBG_IMPORTANT, debugs, RefCount< C >::getRaw(), Adaptation::Answer::kind, Adaptation::Answer::message, and Must.
◆ noteConnectionFailed()
void Adaptation::Icap::ServiceRep::noteConnectionFailed | ( | const char * | comment | ) |
Definition at line 178 of file ServiceRep.cc.
References debugs.
◆ noteConnectionUse()
void Adaptation::Icap::ServiceRep::noteConnectionUse | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 172 of file ServiceRep.cc.
References Comm::Connection::fd, fd_table, Comm::IsConnOpen(), and Must.
◆ noteFailure()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 95 of file ServiceRep.cc.
References debugs, Adaptation::Config::oldest_service_failure, Adaptation::Config::service_failure_limit, and Adaptation::Icap::TheConfig.
◆ noteGoneWaiter()
void Adaptation::Icap::ServiceRep::noteGoneWaiter | ( | ) |
Definition at line 238 of file ServiceRep.cc.
◆ noteTimeToNotify()
void Adaptation::Icap::ServiceRep::noteTimeToNotify | ( | ) |
Definition at line 381 of file ServiceRep.cc.
References Adaptation::Icap::ServiceRep::Client::callback, debugs, Must, and ScheduleCallHere.
◆ noteTimeToUpdate()
void Adaptation::Icap::ServiceRep::noteTimeToUpdate | ( | ) |
Definition at line 367 of file ServiceRep.cc.
References debugs.
Referenced by ServiceRep_noteTimeToUpdate().
◆ optionsFetchTime()
|
private |
Definition at line 647 of file ServiceRep.cc.
References debugs, Adaptation::Config::service_revival_delay, squid_curtime, and Adaptation::Icap::TheConfig.
◆ parseMethod()
|
private |
◆ parseVectPoint()
|
private |
◆ probed()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 291 of file ServiceRep.cc.
◆ putConnection()
void Adaptation::Icap::ServiceRep::putConnection | ( | const Comm::ConnectionPointer & | conn, |
bool | isReusable, | ||
bool | sendReset, | ||
const char * | comment | ||
) |
Definition at line 147 of file ServiceRep.cc.
References Comm::Connection::close(), comm_reset_close(), debugs, Comm::IsConnOpen(), and Must.
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ scheduleNotification()
|
private |
Definition at line 443 of file ServiceRep.cc.
References CallJobHere, and debugs.
◆ scheduleUpdate()
|
private |
Definition at line 609 of file ServiceRep.cc.
References DBG_IMPORTANT, debugs, eventAdd(), eventDelete(), eventFind(), ServiceRep_noteTimeToUpdate(), and squid_curtime.
◆ setMaxConnections()
|
private |
Definition at line 184 of file ServiceRep.cc.
Referenced by ServiceRep().
◆ start()
|
protectedvirtualinherited |
Reimplemented in Ipc::Port, ConnStateData, Adaptation::Icap::ModXact, Ftp::Client, HappyConnOpener, Ftp::Gateway, Ftp::Server, HttpStateData, Adaptation::Ecap::XactionRep, Ipc::UdsSender, Adaptation::Icap::Xaction, Rock::Rebuild, Downloader, Adaptation::AccessCheck, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Adaptation::Iterator, Http::One::Server, Ipc::Forwarder, Comm::TcpAcceptor, Ipc::Coordinator, Ipc::Inquirer, Rock::HeaderUpdater, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Mgr::Inquirer, Snmp::Inquirer, Adaptation::Icap::OptXact, Ipc::Strand, Mgr::Filler, and Mgr::ActionWriter.
Definition at line 59 of file AsyncJob.cc.
Referenced by Ipc::Port::start(), PeerPoolMgr::start(), AsyncJob::Start(), Adaptation::Iterator::start(), Http::Tunneler::start(), Adaptation::AccessCheck::start(), Adaptation::Icap::Launcher::start(), Security::PeerConnector::start(), Adaptation::Icap::Xaction::start(), Ipc::UdsSender::start(), and ConnStateData::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startGettingOptions()
|
private |
Definition at line 597 of file ServiceRep.cc.
◆ status()
|
overrideprivatevirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 678 of file ServiceRep.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), and MemBuf::terminate().
◆ suspend()
|
private |
Definition at line 279 of file ServiceRep.cc.
References DBG_IMPORTANT, debugs, Adaptation::Config::service_revival_delay, squid_curtime, and Adaptation::Icap::TheConfig.
◆ swanSong()
|
inlineprotectedvirtualinherited |
Reimplemented in Adaptation::Icap::ModXactLauncher, Adaptation::Icap::ModXact, ConnStateData, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Client, Ipc::UdsSender, Rock::Rebuild, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Downloader, Adaptation::Iterator, Comm::TcpAcceptor, Ipc::Forwarder, Adaptation::Initiate, Ipc::Inquirer, Adaptation::Icap::OptXact, Rock::HeaderUpdater, Mgr::Forwarder, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Snmp::Forwarder, and Mgr::Filler.
Definition at line 61 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), Server::swanSong(), Comm::ConnOpener::swanSong(), PeerPoolMgr::swanSong(), Rock::HeaderUpdater::swanSong(), Comm::TcpAcceptor::swanSong(), Http::Tunneler::swanSong(), Log::TcpLogger::swanSong(), Security::PeerConnector::swanSong(), Ipc::UdsSender::swanSong(), Client::swanSong(), and HappyConnOpener::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ up()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 301 of file ServiceRep.cc.
◆ wants()
|
inherited |
Definition at line 36 of file Service.cc.
References Adaptation::ServiceFilter::method, AnyP::Uri::path(), Adaptation::ServiceFilter::point, Adaptation::ServiceFilter::request, and HttpRequest::url.
Referenced by Adaptation::ServiceGroup::findService().
◆ wantsPreview()
Definition at line 330 of file ServiceRep.cc.
References Must, and Adaptation::Icap::Options::xferPreview.
◆ wantsUrl()
|
overridevirtual |
Implements Adaptation::Service.
Definition at line 324 of file ServiceRep.cc.
References Must, and Adaptation::Icap::Options::xferIgnore.
◆ writeableCfg()
|
inlineprotectedinherited |
Definition at line 62 of file Service.h.
References Adaptation::Service::theConfig.
Member Data Documentation
◆ connOverloadReported
|
mutableprivate |
Definition at line 144 of file ServiceRep.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ isDetached
|
private |
Definition at line 191 of file ServiceRep.h.
◆ isSuspended
|
private |
Definition at line 148 of file ServiceRep.h.
◆ notifying
|
private |
Definition at line 150 of file ServiceRep.h.
◆ sslContext
Security::ContextPointer Adaptation::Icap::ServiceRep::sslContext |
Definition at line 114 of file ServiceRep.h.
◆ sslSession
Security::SessionStatePointer Adaptation::Icap::ServiceRep::sslSession |
Definition at line 117 of file ServiceRep.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ theAllWaiters
|
private |
number of xactions waiting for a connection slot (notified and not) the number is decreased after the xaction receives notification
Definition at line 141 of file ServiceRep.h.
◆ theBusyConns
|
private |
number of connections given to active transactions
Definition at line 138 of file ServiceRep.h.
◆ theClients
|
private |
Definition at line 129 of file ServiceRep.h.
◆ theConfig
|
privateinherited |
Definition at line 65 of file Service.h.
Referenced by Adaptation::Service::cfg(), Adaptation::Service::Service(), and Adaptation::Service::writeableCfg().
◆ theIdleConns
|
private |
Definition at line 145 of file ServiceRep.h.
Referenced by ServiceRep().
◆ theLastUpdate
|
private |
Definition at line 133 of file ServiceRep.h.
◆ theMaxConnections
|
private |
Definition at line 142 of file ServiceRep.h.
◆ theNotificationWaiters
|
private |
FIFO queue of xactions waiting for a connection slot and not yet notified about it; xaction is removed when notification is scheduled
Definition at line 137 of file ServiceRep.h.
◆ theOptions
|
private |
Definition at line 131 of file ServiceRep.h.
◆ theOptionsFetcher
|
private |
Definition at line 132 of file ServiceRep.h.
◆ theSessionFailures
|
private |
Definition at line 147 of file ServiceRep.h.
◆ tlsContext
Security::FuturePeerContext Adaptation::Icap::ServiceRep::tlsContext |
Definition at line 116 of file ServiceRep.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ updateScheduled
|
private |
Definition at line 151 of file ServiceRep.h.
◆ wasAnnouncedUp
|
mutableprivate |
Definition at line 190 of file ServiceRep.h.
The documentation for this class was generated from the following files:
- src/adaptation/icap/ServiceRep.h
- src/adaptation/icap/ServiceRep.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products