#include <client_side_request.h>
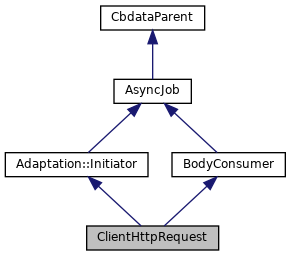
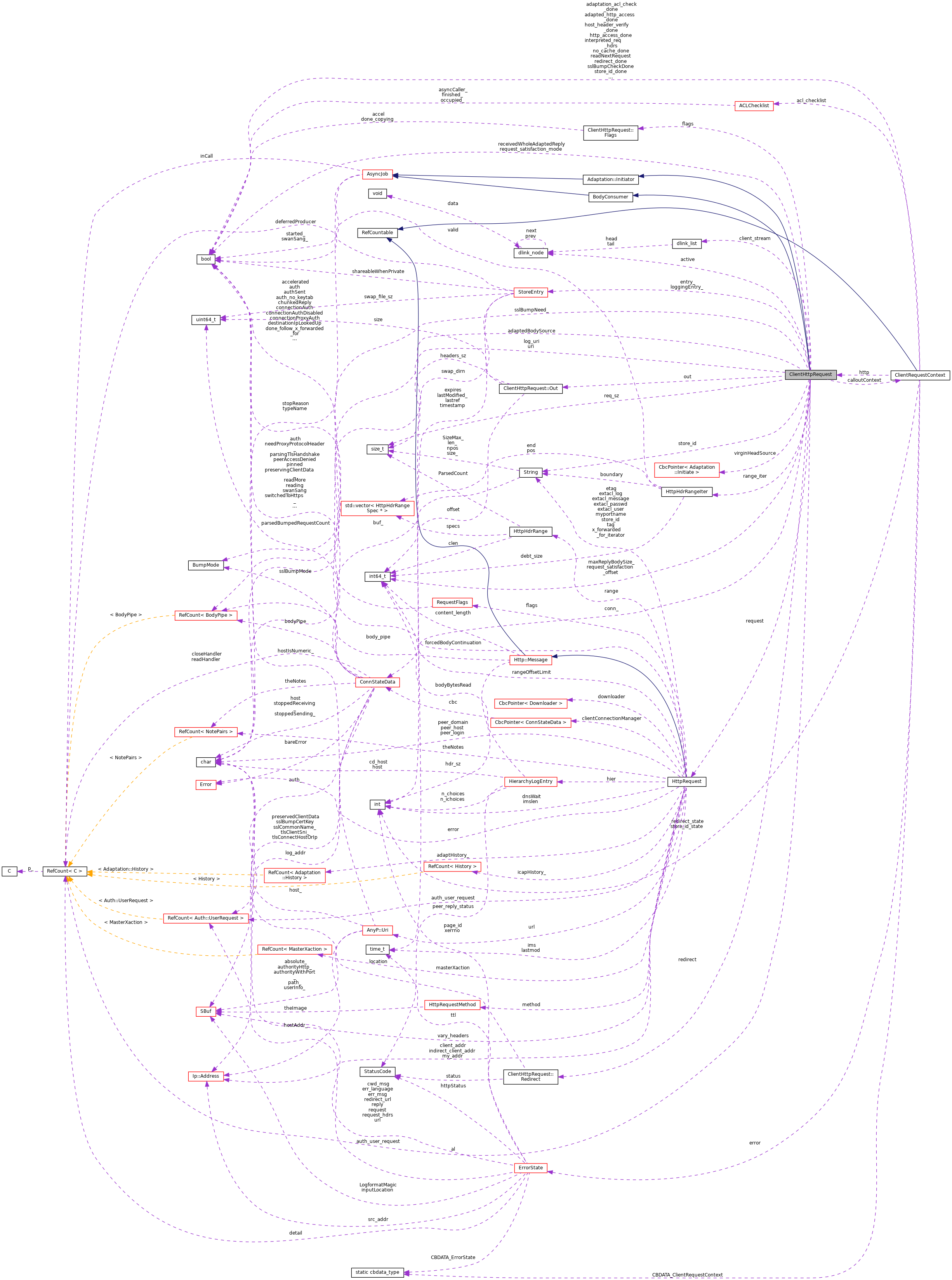
Classes | |
struct | Flags |
struct | Out |
struct | Redirect |
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
Public Member Functions | |
ClientHttpRequest (ConnStateData *) | |
ClientHttpRequest (ClientHttpRequest &&)=delete | |
~ClientHttpRequest () override | |
String | rangeBoundaryStr () const |
void | freeResources () |
void | updateCounters () |
void | logRequest () |
MemObject * | memObject () const |
bool | multipartRangeRequest () const |
void | processRequest () |
void | httpStart () |
bool | onlyIfCached () const |
bool | gotEnough () const |
StoreEntry * | storeEntry () const |
void | storeEntry (StoreEntry *) |
StoreEntry * | loggingEntry () const |
void | loggingEntry (StoreEntry *) |
ConnStateData * | getConn () const |
void | initRequest (HttpRequest *) |
void | resetRequest (HttpRequest *) |
void | resetRequestXXX (HttpRequest *, bool uriChanged) |
void | checkForInternalAccess () |
Checks whether the current request is internal and adjusts it accordingly. More... | |
void | updateLoggingTags (const LogTags_ot code) |
update the code in the transaction processing tags More... | |
const LogTags & | loggingTags () const |
the processing tags associated with this request transaction. More... | |
int64_t | mRangeCLen () const |
void | doCallouts () |
void | setLogUriToRequestUri () |
sets log_uri when we know the current request More... | |
void | setLogUriToRawUri (const char *, const HttpRequestMethod &) |
void | setErrorUri (const char *) |
int64_t | prepPartialResponseGeneration () |
void | calloutsError (const err_type, const ErrorDetail::Pointer &) |
Build an error reply. For use with the callouts. More... | |
void | updateError (const Error &) |
if necessary, stores new error information (if any) More... | |
Ssl::BumpMode | sslBumpNeed () const |
returns raw sslBump mode value More... | |
bool | sslBumpNeeded () const |
returns true if and only if the request needs to be bumped More... | |
void | sslBumpNeed (Ssl::BumpMode) |
set the sslBumpNeeded state More... | |
void | sslBumpStart () |
void | sslBumpEstablish (Comm::Flag) |
void | startAdaptation (const Adaptation::ServiceGroupPointer &) |
Initiate an asynchronous adaptation transaction which will call us back. More... | |
bool | requestSatisfactionMode () const |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | callException (const std::exception &) override |
called when the job throws during an async call More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
HttpRequest *const | request = nullptr |
char * | uri = nullptr |
char *const | log_uri = nullptr |
String | store_id |
struct ClientHttpRequest::Out | out |
HttpHdrRangeIter | range_iter |
size_t | req_sz = 0 |
raw request size on input, not current request size More... | |
const AccessLogEntry::Pointer | al |
access.log entry More... | |
struct ClientHttpRequest::Flags | flags |
struct ClientHttpRequest::Redirect | redirect |
dlink_node | active |
dlink_list | client_stream |
ClientRequestContext * | calloutContext = nullptr |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
CbcPointer< Initiate > | initiateAdaptation (Initiate *x) |
< starts freshly created initiate and returns a safe pointer to it More... | |
void | clearAdaptation (CbcPointer< Initiate > &x) |
clears the pointer (does not call announceInitiatorAbort) More... | |
void | announceInitiatorAbort (CbcPointer< Initiate > &x) |
inform the transaction about abnormal termination and clear the pointer More... | |
bool | initiated (const CbcPointer< AsyncJob > &job) const |
Must(initiated(initiate)) instead of Must(initiate.set()), for clarity. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual void | start () |
called by AsyncStart; do not call directly More... | |
virtual void | swanSong () |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Attributes | |
int64_t | maxReplyBodySize_ = 0 |
StoreEntry * | entry_ = nullptr |
StoreEntry * | loggingEntry_ = nullptr |
ConnStateData * | conn_ = nullptr |
Ssl::BumpMode | sslBumpNeed_ = Ssl::bumpEnd |
whether (and how) the request needs to be bumped More... | |
CbcPointer< Adaptation::Initiate > | virginHeadSource |
BodyPipe::Pointer | adaptedBodySource |
bool | receivedWholeAdaptedReply = false |
noteBodyProductionEnded() was called More... | |
bool | request_satisfaction_mode = false |
int64_t | request_satisfaction_offset = 0 |
Detailed Description
Definition at line 30 of file client_side_request.h.
Member Typedef Documentation
◆ Pointer [1/2]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [2/2]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ ClientHttpRequest() [1/2]
ClientHttpRequest::ClientHttpRequest | ( | ConnStateData * | aConn | ) |
Definition at line 128 of file client_side_request.cc.
References active, al, ConnStateData::bareError, ClientActiveRequests, Server::clientConnection, current_time, dlinkAdd(), Comm::Connection::fd, fd_table, Comm::Connection::isOpen(), ConnStateData::log_addr, ConnStateData::port, ConnStateData::proxyProtocolHeader(), and CodeContext::Reset().
◆ ClientHttpRequest() [2/2]
|
delete |
◆ ~ClientHttpRequest()
|
override |
Definition at line 235 of file client_side_request.cc.
References active, adaptedBodySource, al, Adaptation::Initiator::announceInitiatorAbort(), calloutContext, Error::category, cbdataReferenceDone, checkFailureRatio(), ClientActiveRequests, conn_, debugs, dlinkDelete(), HttpRequest::error, freeResources(), loggingEntry(), logRequest(), request, BodyConsumer::stopConsumingFrom(), uri, and virginHeadSource.
Member Function Documentation
◆ absorbLogUri()
|
private |
Definition at line 1810 of file client_side_request.cc.
References log_uri, and xfree.
Referenced by clearRequest(), setErrorUri(), setLogUriToRawUri(), and setLogUriToRequestUri().
◆ announceInitiatorAbort()
|
protectedinherited |
Definition at line 38 of file Initiator.cc.
References CallJobHere.
Referenced by Client::cleanAdaptation(), and ~ClientHttpRequest().
◆ assignRequest()
|
private |
initializes the current unassigned request to the virgin request sets the current request, asserting that it was unset
Definition at line 1574 of file client_side_request.cc.
References assert, HTTPMSGLOCK(), request, and setLogUriToRequestUri().
Referenced by initRequest(), and resetRequestXXX().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 2078 of file client_side_request.cc.
References DBG_IMPORTANT, debugs, getConn(), and Comm::IsConnOpen().
◆ calloutsError()
void ClientHttpRequest::calloutsError | ( | const err_type | error, |
const ErrorDetail::Pointer & | errDetail | ||
) |
Definition at line 2096 of file client_side_request.cc.
References al, HttpRequest::auth_user_request, ErrorState::auth_user_request, calloutContext, clientBuildError(), ErrorState::detailError(), ClientRequestContext::error, error(), ConnStateData::expectNoForwarding(), ConnStateData::getAuth(), getConn(), ClientRequestContext::readNextRequest, request, and Http::scInternalServerError.
Referenced by ClientRequestContext::clientRedirectDone(), and handleAdaptationFailure().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ checkForInternalAccess()
void ClientHttpRequest::checkForInternalAccess | ( | ) |
Definition at line 1550 of file client_side_request.cc.
References AnyP::Uri::authority(), Config, debugs, RequestFlags::disableCacheUse(), HttpRequest::flags, ForSomeCacheManager(), getMyPort(), AnyP::Uri::getScheme(), SquidConfig::global_internal_static, AnyP::Uri::host(), RequestFlags::internal, internalCheck(), internalHostname(), internalHostnameIs(), internalStaticCheck(), SquidConfig::onoff, AnyP::Uri::path(), AnyP::Uri::port(), AnyP::PROTO_HTTP, request, setLogUriToRequestUri(), AnyP::Uri::setScheme(), and HttpRequest::url.
Referenced by clientProcessRequest(), and resetRequestXXX().
◆ clearAdaptation()
|
protectedinherited |
Definition at line 32 of file Initiator.cc.
References CbcPointer< Cbc >::clear().
Referenced by handleAdaptedHeader(), Client::noteAdaptationAnswer(), and noteAdaptationAnswer().
◆ clearRequest()
|
private |
Definition at line 1584 of file client_side_request.cc.
References absorbLogUri(), HTTPMSGUNLOCK(), and request.
Referenced by freeResources(), and resetRequestXXX().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ doCallouts()
void ClientHttpRequest::doCallouts | ( | ) |
Definition at line 1625 of file client_side_request.cc.
References SquidConfig::accessList, aclFindNfMarkConfig(), aclMapTOS(), ClientRequestContext::adaptation_acl_check_done, ClientRequestContext::adapted_http_access_done, ACLFilledChecklist::al, al, assert, Ssl::bumpClientFirst, SBuf::c_str(), RequestFlags::cachable, calloutContext, cbdataReferenceDone, ClientRequestContext::checkNoCache(), HttpRequest::client_addr, client_stream, ClientRequestContext::clientAccessCheck(), ClientRequestContext::clientAccessCheck2(), clientInterpretRequestHeaders(), ClientRequestContext::clientRedirectStart(), ClientRequestContext::clientStoreIdStart(), clientStreamRead(), Config, dlink_node::data, debugs, Ip::Qos::dirAccepted, ClientRequestContext::error, errorAppendEntry(), ConnStateData::flags, HttpRequest::flags, getConn(), ClientRequestContext::host_header_verify_done, ClientRequestContext::hostHeaderVerify(), ClientRequestContext::http, ClientRequestContext::http_access_done, HttpRequest::icapHistory(), ClientRequestContext::interpreted_req_hdrs, Comm::IsConnOpen(), log_uri, loggingTags(), HttpRequest::method, Adaptation::methodReqmod, ACLFilledChecklist::my_addr, HttpRequest::my_addr, ClientRequestContext::no_cache_done, SquidConfig::noCache, Adaptation::pointPreCache, dlink_node::prev, processRequest(), SquidConfig::Program, ConnStateData::readMore, ClientRequestContext::readNextRequest, SquidConfig::redirect, ClientRequestContext::redirect_done, REDIRECT_PENDING, ClientRequestContext::redirect_state, request, Ip::Qos::setNfConnmark(), ConnStateData::setServerBump(), Ip::Qos::setSockNfmark(), Ip::Qos::setSockTos(), ACLFilledChecklist::src_addr, ClientRequestContext::sslBumpAccessCheck(), ClientRequestContext::sslBumpCheckDone, sslBumpNeed(), sslBumpNeeded(), Adaptation::AccessCheck::Start(), SquidConfig::store_id, ClientRequestContext::store_id_done, ClientRequestContext::store_id_state, storeCreateEntry(), HttpRequest::storeId(), ACLFilledChecklist::syncAle(), dlink_list::tail, Ip::Qos::TheConfig, ClientRequestContext::toClientMarkingDone, and StoreEntry::unlock().
Referenced by Downloader::buildRequest(), ClientRequestContext::checkNoCacheDone(), ClientRequestContext::clientAccessCheckDone(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientStoreIdDone(), ConnStateData::fakeAConnectRequest(), handleAdaptationFailure(), handleAdaptedHeader(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), noteAdaptationAclCheckDone(), and ClientRequestContext::sslBumpAccessCheckDone().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
inlineoverridevirtual |
Reimplemented from AsyncJob.
Definition at line 214 of file client_side_request.h.
References AsyncJob::doneAll().
◆ endRequestSatisfaction()
|
private |
Definition at line 2013 of file client_side_request.cc.
References adaptedBodySource, assert, StoreEntry::completeSuccessfully(), StoreEntry::completeTruncated(), debugs, receivedWholeAdaptedReply, request_satisfaction_mode, BodyConsumer::stopConsumingFrom(), and storeEntry().
Referenced by noteBodyProductionEnded(), and noteMoreBodyDataAvailable().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ freeResources()
void ClientHttpRequest::freeResources | ( | ) |
Definition at line 463 of file client_side.cc.
References HttpHdrRangeIter::boundary, String::clean(), clearRequest(), client_stream, clientStreamAbort(), dlink_node::data, ClientHttpRequest::Redirect::location, range_iter, redirect, safe_free, dlink_list::tail, and uri.
Referenced by ~ClientHttpRequest().
◆ getConn()
|
inline |
Definition at line 68 of file client_side_request.h.
References cbdataReferenceValid(), and conn_.
Referenced by clientReplyContext::buildReplyHeader(), callException(), calloutsError(), ClientRequestContext::clientAccessCheckDone(), clientAclChecklistFill(), clientCheckPinning(), TunnelStateData::clientExpectsConnectResponse(), clientFollowXForwardedForCheck(), ClientRequestContext::clientRedirectDone(), clientSocketRecipient(), ClientRequestContext::clientStoreIdDone(), constructHelperQuery(), clientReplyContext::createStoreEntry(), doCallouts(), clientReplyContext::doGetMoreData(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), initRequest(), logRequest(), noteAdaptationAclCheckDone(), noteBodyProducerAborted(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), clientReplyContext::processReplyAccessResult(), processRequest(), clientReplyContext::purgeDoPurge(), clientReplyContext::purgeRequest(), clientReplyContext::sendBodyTooLargeError(), clientReplyContext::sendMoreData(), clientReplyContext::sendPreconditionFailedError(), ClientRequestContext::sslBumpAccessCheck(), ClientRequestContext::sslBumpAccessCheckDone(), sslBumpEstablish(), sslBumpStart(), statClientRequests(), tunnelStart(), tunnelStartShoveling(), and TunnelStateData::TunnelStateData().
◆ gotEnough()
bool ClientHttpRequest::gotEnough | ( | ) | const |
Definition at line 1479 of file client_side_request.cc.
References assert, MemObject::baseReply(), HttpReply::bodySize(), memObject(), HttpRequest::method, ClientHttpRequest::Out::offset, out, and request.
Referenced by clientReplyContext::replyStatus().
◆ handleAdaptationBlock()
|
private |
Definition at line 1939 of file client_side_request.cc.
References assert, Adaptation::Answer::blockedToChecklistAnswer(), calloutContext, ClientRequestContext::clientAccessCheckDone(), HttpRequest::detailError(), ERR_ACCESS_DENIED, MakeNamedErrorDetail(), and request.
Referenced by noteAdaptationAnswer().
◆ handleAdaptationFailure()
|
private |
Handles an adaptation client request failure. Bypasses the error if possible, or build an error reply.
Definition at line 2050 of file client_side_request.cc.
References assert, Http::Message::body_pipe, calloutContext, calloutsError(), client_stream, BodyPipe::consumedSize(), dlink_node::data, debugs, doCallouts(), ERR_ICAP_FAILURE, StoreEntry::isEmpty(), dlink_node::prev, request, storeEntry(), and dlink_list::tail.
Referenced by noteAdaptationAnswer(), and noteBodyProducerAborted().
◆ handleAdaptedHeader()
|
private |
Definition at line 1897 of file client_side_request.cc.
References adaptedBodySource, al, assert, Adaptation::Initiator::clearAdaptation(), client_stream, clientGetMoreData, StoreEntry::complete(), dlink_node::data, debugs, doCallouts(), HttpRequest::flags, HttpRequestMethod::id(), HttpRequest::method, dlink_node::prev, StoreEntry::replaceHttpReply(), request, request_satisfaction_mode, request_satisfaction_offset, resetRequest(), BodyPipe::setConsumerIfNotLate(), storeEntry(), dlink_list::tail, StoreEntry::timestampsSet(), and virginHeadSource.
Referenced by noteAdaptationAnswer().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ httpStart()
void ClientHttpRequest::httpStart | ( | ) |
Definition at line 1384 of file client_side_request.cc.
References assert, client_stream, clientStreamRead(), dlink_node::data, debugs, LOG_TAG_NONE, loggingTags(), ClientHttpRequest::Out::offset, out, dlink_list::tail, updateLoggingTags(), and uri.
Referenced by processRequest().
◆ initiateAdaptation()
|
protectedinherited |
Definition at line 23 of file Initiator.cc.
References Adaptation::Initiate::initiator().
Referenced by Client::startAdaptation(), and startAdaptation().
◆ initiated()
|
inlineprotectedinherited |
Definition at line 52 of file Initiator.h.
Referenced by Client::startAdaptation(), and startAdaptation().
◆ initRequest()
void ClientHttpRequest::initRequest | ( | HttpRequest * | aRequest | ) |
Initializes the current request with the virgin request. Call this method when the virgin request becomes known. To update the current request later, use resetRequest().
Definition at line 1511 of file client_side_request.cc.
References al, NotePairs::appendNewOnly(), assert, assignRequest(), getConn(), HTTPMSGLOCK(), HttpRequest::notes(), and request.
Referenced by ConnStateData::buildFakeRequest(), Http::One::Server::buildHttpRequest(), Downloader::buildRequest(), and Ftp::Server::parseOneRequest().
◆ loggingEntry() [1/2]
|
inline |
Definition at line 65 of file client_side_request.h.
References loggingEntry_.
Referenced by logRequest(), clientReplyContext::processReplyAccessResult(), and ~ClientHttpRequest().
◆ loggingEntry() [2/2]
void ClientHttpRequest::loggingEntry | ( | StoreEntry * | newEntry | ) |
Definition at line 1499 of file client_side_request.cc.
References StoreEntry::lock(), loggingEntry_, and StoreEntry::unlock().
◆ loggingTags()
|
inline |
Definition at line 94 of file client_side_request.h.
References al.
Referenced by clientReplyContext::buildReplyHeader(), clientReplyContext::cacheHit(), doCallouts(), clientReplyContext::doGetMoreData(), httpStart(), logRequest(), clientReplyContext::processMiss(), clientReplyContext::processReplyAccess(), clientReplyContext::sendMoreData(), statClientRequests(), and updateCounters().
◆ logRequest()
void ClientHttpRequest::logRequest | ( | ) |
Definition at line 374 of file client_side.cc.
References SquidConfig::accessList, accessLogLog(), NotePairs::add(), al, Acl::Answer::allowed(), clientdbUpdate(), Config, StoreEntry::contentLen(), current_time, debugs, ACLChecklist::fastCheck(), fd_table, getConn(), RefCount< C >::getRaw(), ClientHttpRequest::Out::headers_sz, HTTPMSGLOCK(), HTTPMSGUNLOCK(), ICP_INVALID, LOG_TAG_NONE, log_uri, loggingEntry(), loggingTags(), HttpRequest::notes(), SquidConfig::notes, NULL, ClientHttpRequest::Out::offset, out, prepareLogWithRequestDetails(), AnyP::PROTO_HTTP, req_sz, request, ClientHttpRequest::Out::size, sslGetUserEmail(), SquidConfig::stats_collection, tvSub(), ACLFilledChecklist::updateAle(), and updateCounters().
Referenced by ~ClientHttpRequest().
◆ memObject()
|
inline |
Definition at line 55 of file client_side_request.h.
References storeEntry().
Referenced by gotEnough(), and mRangeCLen().
◆ mRangeCLen()
int64_t ClientHttpRequest::mRangeCLen | ( | ) | const |
returns expected content length for multi-range replies note: assumes that httpHdrRangeCanonize has already been called warning: assumes that HTTP headers for individual ranges at the time of the actuall assembly will be exactly the same as the headers when clientMRangeCLen() is called
Definition at line 740 of file client_side.cc.
References assert, HttpHdrRange::begin(), HttpHdrRangeIter::boundary, MemBuf::clean(), clientPackRangeHdr(), clientPackTermBound(), debugs, HttpHdrRange::end(), MemBuf::init(), memObject(), HttpRequest::range, range_iter, request, MemBuf::reset(), MemBuf::size, and storeEntry().
Referenced by prepPartialResponseGeneration().
◆ multipartRangeRequest()
bool ClientHttpRequest::multipartRangeRequest | ( | ) | const |
Definition at line 696 of file client_side.cc.
References HttpRequest::multipartRangeRequest(), and request.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteAdaptationAclCheckDone()
|
overrideprivatevirtual |
AccessCheck calls this back with a possibly nil service group to signal whether adaptation is needed and where it should start.
Reimplemented from Adaptation::Initiator.
Definition at line 693 of file client_side_request.cc.
References debugs, doCallouts(), fd_table, getConn(), HttpRequest::icapHistory(), ConnStateData::isOpen(), log_uri, req_sz, request, sslGetUserEmail(), and startAdaptation().
◆ noteAdaptationAnswer()
|
overrideprivatevirtual |
called with the initial adaptation decision (adapt, block, error); virgin and/or adapted body transmission may continue after this
Implements Adaptation::Initiator.
Definition at line 1873 of file client_side_request.cc.
References adaptedBodySource, Adaptation::Answer::akBlock, Adaptation::Answer::akError, Adaptation::Answer::akForward, assert, cbdataReferenceValid(), Adaptation::Initiator::clearAdaptation(), Adaptation::Answer::final, RefCount< C >::getRaw(), handleAdaptationBlock(), handleAdaptationFailure(), handleAdaptedHeader(), Adaptation::Answer::kind, MakeNamedErrorDetail(), Adaptation::Answer::message, and virginHeadSource.
◆ noteBodyProducerAborted()
|
overrideprivatevirtual |
Implements BodyConsumer.
Definition at line 2031 of file client_side_request.cc.
References adaptedBodySource, assert, Server::clientConnection, Comm::Connection::close(), debugs, HttpRequest::detailError(), ERR_ICAP_FAILURE, getConn(), handleAdaptationFailure(), Comm::IsConnOpen(), MakeNamedErrorDetail(), Must, request, request_satisfaction_mode, BodyConsumer::stopConsumingFrom(), and virginHeadSource.
◆ noteBodyProductionEnded()
|
overrideprivatevirtual |
Implements BodyConsumer.
Definition at line 1999 of file client_side_request.cc.
References adaptedBodySource, assert, endRequestSatisfaction(), BodyPipe::exhausted(), receivedWholeAdaptedReply, and virginHeadSource.
◆ noteMoreBodyDataAvailable()
|
overrideprivatevirtual |
Implements BodyConsumer.
Definition at line 1957 of file client_side_request.cc.
References adaptedBodySource, assert, asyncCall(), BodyPipeCheckout::buf, BodyPipe::buf(), StoreEntry::bytesWanted(), BodyPipeCheckout::checkIn(), MemBuf::consume(), MemBuf::contentSize(), debugs, StoreEntry::deferProducer(), endRequestSatisfaction(), BodyPipe::exhausted(), Important, StoreIOBuffer::length, receivedWholeAdaptedReply, request_satisfaction_mode, request_satisfaction_offset, resumeBodyStorage(), storeEntry(), and StoreEntry::write().
Referenced by resumeBodyStorage().
◆ onlyIfCached()
bool ClientHttpRequest::onlyIfCached | ( | ) | const |
Definition at line 159 of file client_side_request.cc.
References assert, Http::Message::cache_control, HttpHdrCc::hasOnlyIfCached(), and request.
Referenced by clientReplyContext::processExpired(), and clientReplyContext::processMiss().
◆ prepPartialResponseGeneration()
int64_t ClientHttpRequest::prepPartialResponseGeneration | ( | ) |
Prepares to satisfy a Range request with a generated HTTP 206 response. Initializes range_iter state to allow raw range_iter access.
- Returns
- Content-Length value for the future response; never negative
Definition at line 1834 of file client_side_request.cc.
References assert, HttpHdrRange::begin(), HttpHdrRangeIter::boundary, HttpHdrRangeIter::debt_size, HttpHdrRange::end(), HttpHdrRangeIter::end, mRangeCLen(), ClientHttpRequest::Out::offset, out, HttpHdrRangeIter::pos, HttpRequest::range, range_iter, rangeBoundaryStr(), request, HttpHdrRange::specs, HttpHdrRangeIter::updateSpec(), and HttpHdrRangeIter::valid.
◆ processRequest()
void ClientHttpRequest::processRequest | ( | ) |
Definition at line 1360 of file client_side_request.cc.
References assert, debugs, HttpRequest::flags, RequestFlags::forceTunnel, getConn(), httpStart(), HttpRequest::method, Http::METHOD_CONNECT, redirect, request, sslBumpNeeded(), sslBumpStart(), ClientHttpRequest::Redirect::status, Server::stopReading(), tunnelStart(), and uri.
Referenced by doCallouts().
◆ rangeBoundaryStr()
String ClientHttpRequest::rangeBoundaryStr | ( | ) | const |
generates a "unique" boundary string for multipart responses the caller is responsible for cleaning the string
Definition at line 780 of file client_side.cc.
References String::append(), StoreEntry::getMD5Text(), storeEntry(), and visible_appname_string.
Referenced by prepPartialResponseGeneration().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestSatisfactionMode()
|
inline |
Definition at line 211 of file client_side_request.h.
References request_satisfaction_mode.
Referenced by clientReplyContext::buildReplyHeader().
◆ resetRequest()
void ClientHttpRequest::resetRequest | ( | HttpRequest * | newRequest | ) |
Resets the current request to the latest adapted or redirected request. Call this every time adaptation or redirection changes the request. To set the virgin request, use initRequest().
Definition at line 1528 of file client_side_request.cc.
References HttpRequest::effectiveRequestUri(), request, and resetRequestXXX().
Referenced by handleAdaptedHeader().
◆ resetRequestXXX()
void ClientHttpRequest::resetRequestXXX | ( | HttpRequest * | newRequest, |
bool | uriChanged | ||
) |
resetRequest() variation for callers with custom URI change detection logic
- Parameters
-
uriChanged whether the new request URI differs from the current request URI
Definition at line 1535 of file client_side_request.cc.
References assert, assignRequest(), checkForInternalAccess(), clearRequest(), HttpRequest::effectiveRequestUri(), HttpRequest::flags, RequestFlags::redirected, request, SBufToCstring(), uri, and xfree.
Referenced by ClientRequestContext::clientRedirectDone(), and resetRequest().
◆ resumeBodyStorage()
|
private |
Definition at line 1948 of file client_side_request.cc.
References adaptedBodySource, and noteMoreBodyDataAvailable().
Referenced by noteMoreBodyDataAvailable().
◆ setErrorUri()
void ClientHttpRequest::setErrorUri | ( | const char * | aUri | ) |
sets log_uri and uri to an internally-generated "error:..." URI when neither the current request nor the parsed request URI are known
Definition at line 1817 of file client_side_request.cc.
References absorbLogUri(), al, assert, MAX_URL, uri, urlCanonicalCleanWithoutRequest(), xstrdup, and xstrndup().
Referenced by ConnStateData::abortRequestParsing(), and ConnStateData::checkLogging().
◆ setLogUriToRawUri()
void ClientHttpRequest::setLogUriToRawUri | ( | const char * | rawUri, |
const HttpRequestMethod & | method | ||
) |
sets log_uri to a parsed request URI when Squid fails to parse or validate other request components, yielding no current request
Definition at line 1794 of file client_side_request.cc.
References absorbLogUri(), al, assert, AnyP::Uri::cleanup(), urlCanonicalCleanWithoutRequest(), and xfree.
Referenced by Http::One::Server::buildHttpRequest().
◆ setLogUriToRequestUri()
void ClientHttpRequest::setLogUriToRequestUri | ( | ) |
Definition at line 1786 of file client_side_request.cc.
References absorbLogUri(), assert, HttpRequest::canonicalCleanUrl(), MAX_URL, request, and xstrndup().
Referenced by assignRequest(), and checkForInternalAccess().
◆ sslBumpEstablish()
void ClientHttpRequest::sslBumpEstablish | ( | Comm::Flag | errflag | ) |
Definition at line 1418 of file client_side_request.cc.
References assert, HttpRequest::auth_user_request, Server::clientConnection, Comm::Connection::close(), debugs, Comm::ERR_CLOSING, getConn(), request, ConnStateData::setAuth(), sslBumpNeed_, sslBumpNeeded(), and ConnStateData::switchToHttps().
Referenced by SslBumpEstablish().
◆ sslBumpNeed() [1/2]
|
inline |
Definition at line 195 of file client_side_request.h.
References sslBumpNeed_.
Referenced by doCallouts(), ClientRequestContext::sslBumpAccessCheck(), and ClientRequestContext::sslBumpAccessCheckDone().
◆ sslBumpNeed() [2/2]
void ClientHttpRequest::sslBumpNeed | ( | Ssl::BumpMode | mode | ) |
Definition at line 1400 of file client_side_request.cc.
References Ssl::bumpMode(), debugs, and sslBumpNeed_.
◆ sslBumpNeeded()
|
inline |
Definition at line 197 of file client_side_request.h.
References Ssl::bumpBump, Ssl::bumpClientFirst, Ssl::bumpPeek, Ssl::bumpServerFirst, Ssl::bumpStare, and sslBumpNeed_.
Referenced by doCallouts(), processRequest(), and sslBumpEstablish().
◆ sslBumpStart()
void ClientHttpRequest::sslBumpStart | ( | ) |
Definition at line 1441 of file client_side_request.cc.
References al, Ssl::bumpMode(), Server::clientConnection, commCbCall(), CommCommonCbParams::conn, debugs, CommCommonCbParams::flag, HttpRequest::flags, getConn(), RequestFlags::intercepted, RequestFlags::interceptTproxy, HttpReply::MakeConnectionEstablished(), Comm::OK, request, ScheduleCallHere, SslBumpEstablish(), ConnStateData::sslBumpMode, sslBumpNeed_, and Comm::Write().
Referenced by processRequest().
◆ start()
|
protectedvirtualinherited |
Reimplemented in Ipc::Port, ConnStateData, Adaptation::Icap::ModXact, Ftp::Client, HappyConnOpener, Ftp::Gateway, Ftp::Server, HttpStateData, Adaptation::Ecap::XactionRep, Ipc::UdsSender, Adaptation::Icap::Xaction, Rock::Rebuild, Downloader, Adaptation::AccessCheck, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Adaptation::Iterator, Http::One::Server, Ipc::Forwarder, Comm::TcpAcceptor, Ipc::Coordinator, Ipc::Inquirer, Rock::HeaderUpdater, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Mgr::Inquirer, Snmp::Inquirer, Adaptation::Icap::OptXact, Ipc::Strand, Mgr::Filler, and Mgr::ActionWriter.
Definition at line 59 of file AsyncJob.cc.
Referenced by Ipc::Port::start(), PeerPoolMgr::start(), AsyncJob::Start(), Adaptation::Iterator::start(), Http::Tunneler::start(), Security::PeerConnector::start(), Adaptation::AccessCheck::start(), Adaptation::Icap::Launcher::start(), Adaptation::Icap::Xaction::start(), Ipc::UdsSender::start(), and ConnStateData::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startAdaptation()
void ClientHttpRequest::startAdaptation | ( | const Adaptation::ServiceGroupPointer & | g | ) |
Definition at line 1859 of file client_side_request.cc.
References adaptedBodySource, al, assert, debugs, Adaptation::Initiator::initiateAdaptation(), Adaptation::Initiator::initiated(), Must, request, and virginHeadSource.
Referenced by noteAdaptationAclCheckDone().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by Client::cleanAdaptation(), Client::doneSendingRequestBody(), Client::endAdaptedBodyConsumption(), endRequestSatisfaction(), Client::handleAdaptedBodyProducerAborted(), Client::handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), Client::serverComplete(), Client::swanSong(), and ~ClientHttpRequest().
◆ storeEntry() [1/2]
|
inline |
Definition at line 63 of file client_side_request.h.
References entry_.
Referenced by clientReplyContext::blockedHit(), clientReplyContext::buildReplyHeader(), clientReplyContext::cacheHit(), clientReplyContext::checkTransferDone(), clientIfRangeMatch(), clientReplyContext::cloneReply(), clientReplyContext::createStoreEntry(), clientReplyContext::doGetMoreData(), Ftp::Server::doProcessRequest(), endRequestSatisfaction(), clientReplyContext::errorInStream(), clientReplyContext::forgetHit(), handleAdaptationFailure(), handleAdaptedHeader(), clientReplyContext::handleIMSReply(), Http::One::Server::handleReply(), clientReplyContext::identifyFoundObject(), memObject(), mRangeCLen(), noteMoreBodyDataAvailable(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processReplyAccessResult(), clientReplyContext::purgeDoPurge(), rangeBoundaryStr(), clientReplyContext::removeClientStoreReference(), clientReplyContext::replyStatus(), clientReplyContext::requestMoreBodyFromStore(), clientReplyContext::restoreState(), clientReplyContext::saveState(), clientReplyContext::sendClientOldEntry(), clientReplyContext::sendClientUpstreamResponse(), clientReplyContext::sendMoreData(), clientReplyContext::sendNotModified(), Ftp::Server::setReply(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), clientReplyContext::setReplyToStoreEntry(), clientReplyContext::startError(), statClientRequests(), clientReplyContext::storeNotOKTransferDone(), clientReplyContext::storeOKTransferDone(), clientReplyContext::traceReply(), and clientReplyContext::triggerInitialStoreRead().
◆ storeEntry() [2/2]
void ClientHttpRequest::storeEntry | ( | StoreEntry * | newEntry | ) |
Definition at line 1493 of file client_side_request.cc.
References entry_.
◆ swanSong()
|
inlineprotectedvirtualinherited |
Reimplemented in Adaptation::Icap::ModXactLauncher, Adaptation::Icap::ModXact, ConnStateData, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Client, Ipc::UdsSender, Rock::Rebuild, Adaptation::Icap::Launcher, Security::PeerConnector, Ftp::Relay, Log::TcpLogger, Http::Tunneler, Downloader, Adaptation::Iterator, Comm::TcpAcceptor, Ipc::Forwarder, Adaptation::Initiate, Ipc::Inquirer, Adaptation::Icap::OptXact, Rock::HeaderUpdater, Mgr::Forwarder, Comm::ConnOpener, PeerPoolMgr, Mgr::StoreToCommWriter, Server, Snmp::Forwarder, and Mgr::Filler.
Definition at line 61 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), Server::swanSong(), Comm::ConnOpener::swanSong(), PeerPoolMgr::swanSong(), Rock::HeaderUpdater::swanSong(), Comm::TcpAcceptor::swanSong(), Http::Tunneler::swanSong(), Log::TcpLogger::swanSong(), Security::PeerConnector::swanSong(), Ipc::UdsSender::swanSong(), Client::swanSong(), and HappyConnOpener::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ updateCounters()
void ClientHttpRequest::updateCounters | ( | ) |
Definition at line 308 of file client_side.cc.
References al, StatCounters::client_http, clientUpdateHierCounters(), clientUpdateStatCounters(), clientUpdateStatHistCounters(), current_time, HttpRequest::error, StatCounters::errors, HttpRequest::hier, loggingTags(), request, statCounter, and tvSubMsec().
Referenced by logRequest().
◆ updateError()
void ClientHttpRequest::updateError | ( | const Error & | error | ) |
Definition at line 1470 of file client_side_request.cc.
References al, error(), HttpRequest::error, request, and Error::update().
Referenced by ConnStateData::checkLogging().
◆ updateLoggingTags()
|
inline |
Definition at line 91 of file client_side_request.h.
Referenced by clientReplyContext::cacheHit(), ClientRequestContext::clientAccessCheckDone(), clientGetMoreData(), clientReplyContext::handleIMSReply(), httpStart(), clientReplyContext::identifyFoundObject(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processReplyAccessResult(), clientReplyContext::purgeDoPurge(), clientReplyContext::purgeRequest(), clientReplyContext::sendBodyTooLargeError(), clientReplyContext::sendClientOldEntry(), clientReplyContext::sendNotModified(), clientReplyContext::sendPreconditionFailedError(), and tunnelStart().
Member Data Documentation
◆ active
dlink_node ClientHttpRequest::active |
Definition at line 173 of file client_side_request.h.
Referenced by ClientHttpRequest(), clientReplyContext::makeThisHead(), and ~ClientHttpRequest().
◆ adaptedBodySource
|
private |
Definition at line 244 of file client_side_request.h.
Referenced by endRequestSatisfaction(), handleAdaptedHeader(), noteAdaptationAnswer(), noteBodyProducerAborted(), noteBodyProductionEnded(), noteMoreBodyDataAvailable(), resumeBodyStorage(), startAdaptation(), and ~ClientHttpRequest().
◆ al
const AccessLogEntry::Pointer ClientHttpRequest::al |
Definition at line 161 of file client_side_request.h.
Referenced by ConnStateData::buildFakeRequest(), clientReplyContext::buildReplyHeader(), calloutsError(), ClientRequestContext::clientAccessCheckDone(), clientAclChecklistFill(), clientFollowXForwardedForCheck(), ClientHttpRequest(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientRedirectStart(), ClientRequestContext::clientStoreIdDone(), clientReplyContext::cloneReply(), constructHelperQuery(), doCallouts(), handleAdaptedHeader(), clientReplyContext::handleIMSReply(), Ftp::Server::handleUploadRequest(), initRequest(), clientReplyContext::loggingTags(), loggingTags(), logRequest(), ConnStateData::parseTlsHandshake(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), Http::One::Server::processParsedRequest(), clientReplyContext::processReplyAccessResult(), clientReplyContext::purgeDoPurge(), clientReplyContext::purgeRequest(), clientReplyContext::sendBodyTooLargeError(), clientReplyContext::sendPreconditionFailedError(), ConnStateData::serveDelayedError(), setErrorUri(), setLogUriToRawUri(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), ClientRequestContext::sslBumpAccessCheck(), ClientRequestContext::sslBumpAccessCheckDone(), sslBumpStart(), startAdaptation(), ConnStateData::startPeekAndSplice(), statClientRequests(), tunnelStart(), TunnelStateData::TunnelStateData(), updateCounters(), updateError(), updateLoggingTags(), Http::One::Server::writeControlMsgAndCall(), and ~ClientHttpRequest().
◆ calloutContext
ClientRequestContext* ClientHttpRequest::calloutContext = nullptr |
Definition at line 176 of file client_side_request.h.
Referenced by Downloader::buildRequest(), calloutsError(), clientProcessRequest(), doCallouts(), ConnStateData::fakeAConnectRequest(), handleAdaptationBlock(), handleAdaptationFailure(), and ~ClientHttpRequest().
◆ client_stream
dlink_list ClientHttpRequest::client_stream |
Definition at line 174 of file client_side_request.h.
Referenced by ConnStateData::abortRequestParsing(), ConnStateData::buildFakeRequest(), Downloader::buildRequest(), clientInterpretRequestHeaders(), constructHelperQuery(), doCallouts(), freeResources(), handleAdaptationFailure(), handleAdaptedHeader(), ClientRequestContext::hostHeaderVerifyFailed(), httpStart(), clientReplyContext::next(), ConnStateData::parseHttpRequest(), Ftp::Server::parseOneRequest(), clientReplyContext::processReplyAccessResult(), clientReplyContext::pushStreamData(), clientReplyContext::sendMoreData(), and clientReplyContext::sendStreamError().
◆ conn_
|
private |
Definition at line 190 of file client_side_request.h.
Referenced by getConn(), and ~ClientHttpRequest().
◆ entry_
|
private |
Definition at line 188 of file client_side_request.h.
Referenced by storeEntry().
◆ flags
struct ClientHttpRequest::Flags ClientHttpRequest::flags |
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ log_uri
char* const ClientHttpRequest::log_uri = nullptr |
Cleaned up URI of the current (virgin or adapted/redirected) request, computed URI of an internally-generated requests, or one of the hard-coded "error:..." URIs.
Definition at line 141 of file client_side_request.h.
Referenced by absorbLogUri(), Http::One::Server::buildHttpRequest(), clientAclChecklistFill(), clientReplyContext::createStoreEntry(), doCallouts(), clientReplyContext::doGetMoreData(), Ftp::Server::handleUploadRequest(), logRequest(), noteAdaptationAclCheckDone(), ConnStateData::postHttpsAccept(), clientReplyContext::processExpired(), Http::One::Server::processParsedRequest(), and tunnelStart().
◆ loggingEntry_
|
private |
Definition at line 189 of file client_side_request.h.
Referenced by loggingEntry().
◆ maxReplyBodySize_
|
private |
Definition at line 187 of file client_side_request.h.
◆ out
struct ClientHttpRequest::Out ClientHttpRequest::out |
Referenced by clientProcessRequest(), clientReplyContext::doGetMoreData(), gotEnough(), Downloader::handleReply(), httpStart(), logRequest(), prepPartialResponseGeneration(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::replyStatus(), clientReplyContext::sendMoreData(), ConnStateData::serveDelayedError(), statClientRequests(), clientReplyContext::storeNotOKTransferDone(), clientReplyContext::storeOKTransferDone(), tunnelConnectedWriteDone(), and TunnelStateData::TunnelStateData().
◆ range_iter
HttpHdrRangeIter ClientHttpRequest::range_iter |
Definition at line 158 of file client_side_request.h.
Referenced by freeResources(), mRangeCLen(), and prepPartialResponseGeneration().
◆ receivedWholeAdaptedReply
|
private |
Definition at line 247 of file client_side_request.h.
Referenced by endRequestSatisfaction(), noteBodyProductionEnded(), and noteMoreBodyDataAvailable().
◆ redirect
struct ClientHttpRequest::Redirect ClientHttpRequest::redirect |
◆ req_sz
size_t ClientHttpRequest::req_sz = 0 |
Definition at line 159 of file client_side_request.h.
Referenced by ConnStateData::abortRequestParsing(), Downloader::buildRequest(), ConnStateData::checkLogging(), logRequest(), noteAdaptationAclCheckDone(), ConnStateData::parseHttpRequest(), Ftp::Server::parseOneRequest(), and statClientRequests().
◆ request
HttpRequest* const ClientHttpRequest::request = nullptr |
Request currently being handled by ClientHttpRequest. Usually remains nil until the virgin request header is parsed or faked. Starts as a virgin request; see initRequest(). Adaptation and redirections replace it; see resetRequest().
Definition at line 130 of file client_side_request.h.
Referenced by ConnStateData::abortChunkedRequestBody(), assignRequest(), clientReplyContext::blockedHit(), clientReplyContext::buildReplyHeader(), clientReplyContext::cacheHit(), calloutsError(), checkForInternalAccess(), ClientRequestContext::checkNoCacheDone(), clientReplyContext::checkTransferDone(), clearRequest(), ClientRequestContext::clientAccessCheck(), ClientRequestContext::clientAccessCheckDone(), clientAclChecklistFill(), clientCheckPinning(), clientFollowXForwardedForCheck(), clientGetMoreData(), clientHierarchical(), clientIfRangeMatch(), clientInterpretRequestHeaders(), clientProcessRequest(), ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientRedirectStart(), clientSetKeepaliveFlag(), ClientRequestContext::clientStoreIdDone(), constructHelperQuery(), clientReplyContext::createStoreEntry(), DelayId::DelayClient(), doCallouts(), clientReplyContext::doGetMoreData(), Ftp::Server::doProcessRequest(), ConnStateData::fakeAConnectRequest(), gotEnough(), guaranteedRequest(), handleAdaptationBlock(), handleAdaptationFailure(), handleAdaptedHeader(), clientReplyContext::handleIMSReply(), Http::One::Server::handleReply(), Ftp::Server::handleUploadRequest(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), clientReplyContext::identifyFoundObject(), clientReplyContext::identifyStoreObject(), initRequest(), logRequest(), mRangeCLen(), multipartRangeRequest(), noteAdaptationAclCheckDone(), noteBodyProducerAborted(), Ftp::Server::notePeerConnection(), onlyIfCached(), prepPartialResponseGeneration(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), Http::One::Server::processParsedRequest(), clientReplyContext::processReplyAccess(), clientReplyContext::processReplyAccessResult(), processRequest(), clientReplyContext::purgeAllCached(), clientReplyContext::purgeDoPurge(), clientReplyContext::purgeEntry(), clientReplyContext::purgeRequest(), clientReplyContext::replyStatus(), resetRequest(), resetRequestXXX(), clientReplyContext::restoreState(), clientReplyContext::saveState(), clientReplyContext::sendBodyTooLargeError(), clientReplyContext::sendMoreData(), clientReplyContext::sendNotModified(), clientReplyContext::sendNotModifiedOrPreconditionFailedError(), clientReplyContext::sendPreconditionFailedError(), clientReplyContext::sendStreamError(), ConnStateData::serveDelayedError(), Ssl::ServerBump::ServerBump(), Ftp::Server::setDataCommand(), setLogUriToRequestUri(), Ftp::Server::setReply(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), clientReplyContext::setReplyToStoreEntry(), ConnStateData::splice(), ClientRequestContext::sslBumpAccessCheck(), sslBumpEstablish(), sslBumpStart(), startAdaptation(), clientReplyContext::startError(), statClientRequests(), clientReplyContext::storeNotOKTransferDone(), ConnStateData::switchToHttps(), clientReplyContext::traceReply(), tunnelStart(), TunnelStateData::TunnelStateData(), updateCounters(), updateError(), Http::One::Server::writeControlMsgAndCall(), and ~ClientHttpRequest().
◆ request_satisfaction_mode
|
private |
Definition at line 249 of file client_side_request.h.
Referenced by endRequestSatisfaction(), handleAdaptedHeader(), noteBodyProducerAborted(), noteMoreBodyDataAvailable(), and requestSatisfactionMode().
◆ request_satisfaction_offset
|
private |
Definition at line 250 of file client_side_request.h.
Referenced by handleAdaptedHeader(), and noteMoreBodyDataAvailable().
◆ sslBumpNeed_
|
private |
Definition at line 205 of file client_side_request.h.
Referenced by sslBumpEstablish(), sslBumpNeed(), sslBumpNeeded(), and sslBumpStart().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ store_id
String ClientHttpRequest::store_id |
Definition at line 143 of file client_side_request.h.
Referenced by ClientRequestContext::clientStoreIdDone(), and clientReplyContext::storeId().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ uri
char* ClientHttpRequest::uri = nullptr |
Usually starts as a URI received from the client, with scheme and host added if needed. Is used to create the virgin request for initRequest(). URIs of adapted/redirected requests replace it via resetRequest().
Definition at line 135 of file client_side_request.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::buildFakeRequest(), Http::One::Server::buildHttpRequest(), Downloader::buildRequest(), clientReplyContext::cacheHit(), ClientRequestContext::clientAccessCheckDone(), ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientRedirectStart(), ClientRequestContext::clientStoreIdDone(), ClientRequestContext::clientStoreIdStart(), constructHelperQuery(), freeResources(), httpStart(), ConnStateData::parseHttpRequest(), Ftp::Server::parseOneRequest(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), Http::One::Server::processParsedRequest(), clientReplyContext::processReplyAccessResult(), processRequest(), redirectStart(), resetRequestXXX(), clientReplyContext::sendMoreData(), ConnStateData::serveDelayedError(), setErrorUri(), statClientRequests(), clientReplyContext::storeId(), storeIdStart(), tunnelStart(), TunnelStateData::TunnelStateData(), and ~ClientHttpRequest().
◆ virginHeadSource
|
private |
Definition at line 243 of file client_side_request.h.
Referenced by handleAdaptedHeader(), noteAdaptationAnswer(), noteBodyProducerAborted(), noteBodyProductionEnded(), startAdaptation(), and ~ClientHttpRequest().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products