#include <MemObject.h>
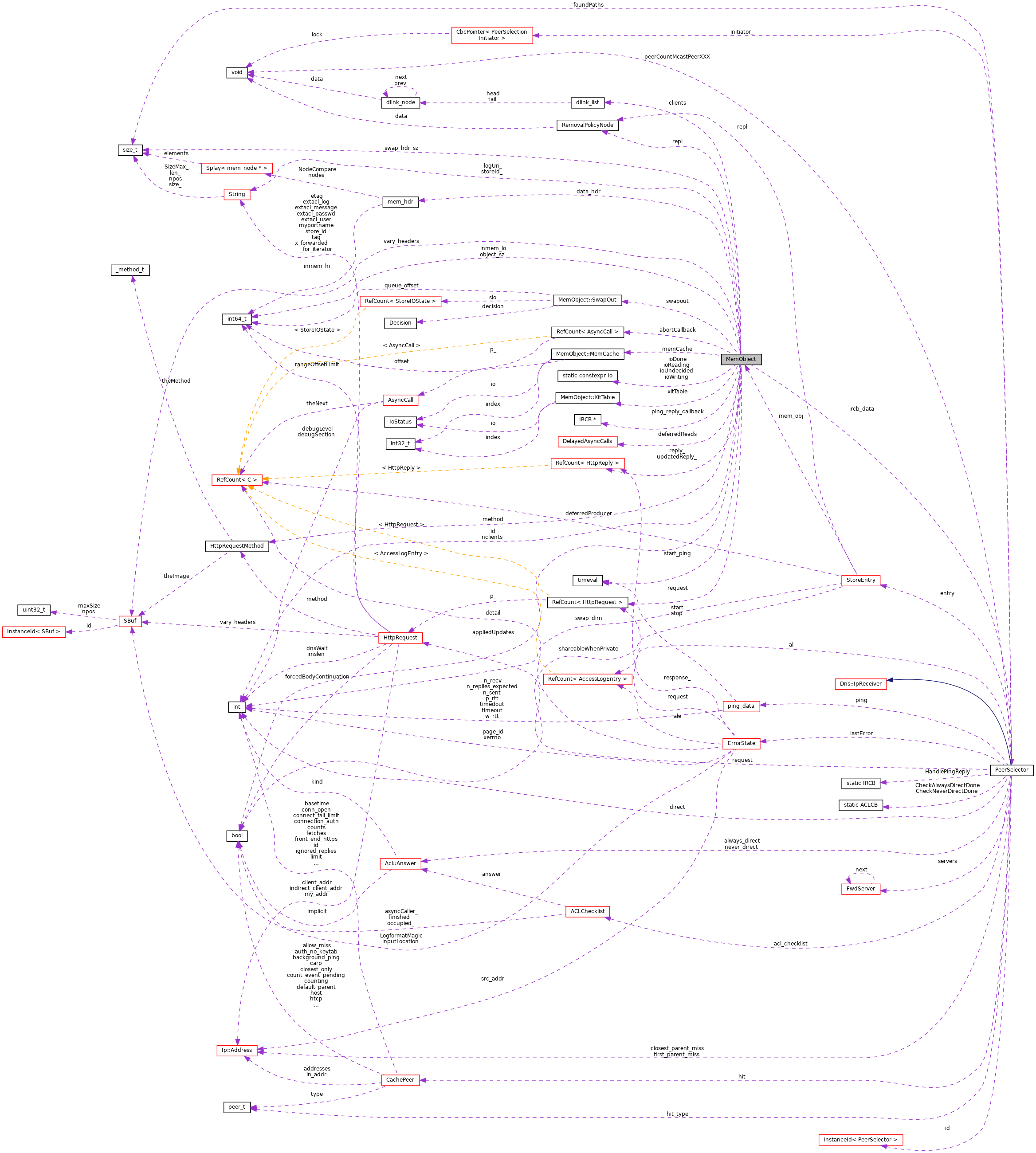
Classes | |
class | MemCache |
State of an entry with regards to the [shared] memory caching. More... | |
class | SwapOut |
class | XitTable |
State of an entry with regards to the [shared] in-transit table. More... | |
Public Member Functions | |
void | dump () const |
MemObject () | |
~MemObject () | |
void | setUris (char const *aStoreId, char const *aLogUri, const HttpRequestMethod &aMethod) |
bool | hasUris () const |
whether setUris() has been called More... | |
void | write (const StoreIOBuffer &buf) |
void | unlinkRequest () |
const HttpReply & | baseReply () const |
const HttpReplyPointer & | updatedReply () const |
const HttpReply & | freshestReply () const |
HttpReply & | adjustableBaseReply () |
void | replaceBaseReply (const HttpReplyPointer &r) |
void | updateReply (const HttpReply &r) |
(re)sets updated reply; More... | |
void | stat (MemBuf *mb) const |
int64_t | endOffset () const |
void | markEndOfReplyHeaders () |
sets baseReply().hdr_sz (i.e. written reply headers size) to endOffset() More... | |
int64_t | expectedReplySize () const |
int64_t | size () const |
void | reset () |
int64_t | lowestMemReaderOffset () const |
bool | readAheadPolicyCanRead () const |
void | addClient (store_client *) |
int64_t | objectBytesOnDisk () const |
int64_t | policyLowestOffsetToKeep (bool swap) const |
int64_t | availableForSwapOut () const |
buffered bytes we have not swapped out yet More... | |
void | trimSwappable () |
void | trimUnSwappable () |
bool | isContiguous () const |
int | mostBytesWanted (int max, bool ignoreDelayPools) const |
void | setNoDelay (bool const newValue) |
DelayId | mostBytesAllowed () const |
const char * | urlXXX () const |
const char * | storeId () const |
const char * | logUri () const |
client request URI used for logging; storeId() by default More... | |
size_t | clientCount () const |
bool | clientIsFirst (void *sc) const |
void | delayRead (const AsyncCallPointer &) |
void | kickReads () |
Static Public Member Functions | |
static size_t | inUseCount () |
Public Attributes | |
bool | appliedUpdates = false |
HttpRequestMethod | method |
mem_hdr | data_hdr |
int64_t | inmem_lo = 0 |
dlink_list | clients |
int | nclients = 0 |
SwapOut | swapout |
XitTable | xitTable |
current [shared] memory caching state for the entry More... | |
MemCache | memCache |
current [shared] memory caching state for the entry More... | |
HttpRequestPointer | request |
struct timeval | start_ping |
IRCB * | ping_reply_callback |
PeerSelector * | ircb_data = nullptr |
AsyncCallPointer | abortCallback |
used for notifying StoreEntry writers about 3rd-party initiated aborts More... | |
RemovalPolicyNode | repl |
int | id = 0 |
int64_t | object_sz = -1 |
size_t | swap_hdr_sz = 0 |
SBuf | vary_headers |
Private Member Functions | |
MEMPROXY_CLASS (MemObject) | |
Private Attributes | |
HttpReplyPointer | reply_ |
HttpReplyPointer | updatedReply_ |
SBuf | storeId_ |
StoreId for our entry (usually request URI) More... | |
SBuf | logUri_ |
URI used for logging (usually request URI) More... | |
DelayedAsyncCalls | deferredReads |
Detailed Description
Definition at line 33 of file MemObject.h.
Constructor & Destructor Documentation
◆ MemObject()
MemObject::MemObject | ( | ) |
Definition at line 97 of file MemObject.cc.
References debugs, ping_reply_callback, reply_, and start_ping.
◆ ~MemObject()
MemObject::~MemObject | ( | ) |
Definition at line 105 of file MemObject.cc.
References assert, data_hdr, debugs, mem_hdr::freeContent(), MemObject::XitTable::index, MemObject::MemCache::index, memCache, MemObject::SwapOut::sio, swapout, and xitTable.
Member Function Documentation
◆ addClient()
void MemObject::addClient | ( | store_client * | aClient | ) |
Definition at line 303 of file MemObject.cc.
References clients, dlinkAdd(), nclients, and store_client::node.
Referenced by storeClientListAdd().
◆ adjustableBaseReply()
HttpReply & MemObject::adjustableBaseReply | ( | ) |
- Returns
- writable base reply for parsing and other initial modifications Base modifications can only be done when forming/loading the entry. After that, use replaceBaseReply() to reset all of the replies.
Definition at line 121 of file MemObject.cc.
References assert, reply_, and updatedReply_.
Referenced by MemStore::copyFromShm(), TestRock::createEntry(), TestUfs::testUfsSearch(), and store_client::tryParsingHttpHeaders().
◆ availableForSwapOut()
int64_t MemObject::availableForSwapOut | ( | ) | const |
Definition at line 489 of file MemObject.cc.
References endOffset(), MemObject::SwapOut::queue_offset, and swapout.
Referenced by Store::Disks::accumulateMore(), and StoreEntry::swapOut().
◆ baseReply()
|
inline |
HTTP response before 304 (Not Modified) updates starts "empty"; modified via replaceBaseReply() or adjustableBaseReply()
Definition at line 60 of file MemObject.h.
References reply_.
Referenced by StoreEntry::append(), CheckQuickAbortIsReasonable(), StoreEntry::checkTooBig(), StoreEntry::checkTooSmall(), FwdState::complete(), StoreEntry::contentLen(), store_client::doCopy(), expectedReplySize(), store_client::finishCallback(), freshestReply(), ClientHttpRequest::gotEnough(), StoreEntry::hasParsedReplyHeader(), netdbExchangeHandleReply(), store_client::nextHttpReadOffset(), clientReplyContext::processConditional(), readAheadPolicyCanRead(), FwdState::reforward(), refreshIsCachable(), clientReplyContext::replyStatus(), store_client::sendingHttpHeaders(), store_client::skipHttpHeadersFromDisk(), StoreEntry::startWriting(), clientReplyContext::storeNotOKTransferDone(), clientReplyContext::storeOKTransferDone(), MemStore::updateHeadersOrThrow(), StoreEntry::updateOnNotModified(), urnHandleReply(), StoreEntry::validLength(), and StoreEntry::write().
◆ clientCount()
|
inline |
◆ clientIsFirst()
|
inline |
Definition at line 154 of file MemObject.h.
References clients, dlink_node::data, dlink_list::head, and sc.
◆ delayRead()
void MemObject::delayRead | ( | const AsyncCallPointer & | aRead | ) |
Definition at line 445 of file MemObject.cc.
References deferredReads, DelayedAsyncCalls::delay(), mostBytesAllowed(), and readAheadPolicyCanRead().
Referenced by Client::delayRead().
◆ dump()
void MemObject::dump | ( | ) | const |
Definition at line 149 of file MemObject.cc.
References appliedUpdates, data_hdr, DBG_IMPORTANT, debugs, mem_hdr::dump(), mem_hdr::endOffset(), inmem_lo, logUri_, nclients, reply_, request, start_ping, storeId_, and updatedReply_.
Referenced by storeId().
◆ endOffset()
int64_t MemObject::endOffset | ( | ) | const |
The offset of the last memory-stored HTTP response byte plus one.
- HTTP response headers (if any) are stored at offset zero.
- HTTP response body byte[n] usually has offset (hdr_sz + n), where hdr_sz is the size of stored HTTP response headers (zero if none); and n is the corresponding byte offset in the whole resource body. However, some 206 (Partial Content) response bodies are stored (and retrieved) as regular 200 response bodies, disregarding offsets of their body parts.
- See also
- HttpStateData::decideIfWeDoRanges().
Definition at line 214 of file MemObject.cc.
References data_hdr, and mem_hdr::endOffset().
Referenced by StoreEntry::append(), availableForSwapOut(), store_client::canReadFromMemory(), CheckQuickAbortIsReasonable(), StoreEntry::checkTooBig(), StoreEntry::complete(), MemStore::copyFromShm(), MemStore::copyFromShmSlice(), MemStore::copyToShm(), store_client::doCopy(), doPages(), store_client::finishCallback(), isContiguous(), StoreEntry::isEmpty(), Store::Controller::keepForLocalMemoryCache(), lowestMemReaderOffset(), markEndOfReplyHeaders(), store_client::maybeWriteFromDiskToMemory(), StoreEntry::mayStartSwapOut(), objectSizeForDirSelection(), policyLowestOffsetToKeep(), readAheadPolicyCanRead(), MemStore::shouldCache(), size(), StoreEntry::storeClientType(), and StoreEntry::swapOut().
◆ expectedReplySize()
int64_t MemObject::expectedReplySize | ( | ) | const |
negative if unknown; otherwise, expected object_sz, expected endOffset maximum, and stored reply headers+body size (all three are the same)
Definition at line 238 of file MemObject.cc.
References baseReply(), HttpReply::bodySize(), debugs, Http::Message::hdr_sz, method, and object_sz.
Referenced by Store::Controller::keepForLocalMemoryCache(), StoreEntry::mayStartSwapOut(), objectSizeForDirSelection(), Store::PackFields(), MemStore::shouldCache(), and MemStore::startCaching().
◆ freshestReply()
|
inline |
- Returns
- the updated-by-304(s) response (if it exists)
- baseReply() (otherwise)
Definition at line 68 of file MemObject.h.
References baseReply(), and updatedReply_.
Referenced by TestRock::addEntry(), StoreEntry::adjustVary(), Client::blockCaching(), clientReplyContext::blockedHit(), clientReplyContext::cloneReply(), clientReplyContext::handleIMSReply(), StoreEntry::hasFreshestReply(), StoreEntry::hasOneOfEtags(), peerDigestFetchReply(), HttpStateData::peerSupportsConnectionPinning(), clientReplyContext::sendNotModified(), storeLog(), TestUfs::testUfsSearch(), StoreEntry::timestampsSet(), MemStore::updateHeadersOrThrow(), StoreEntry::updateOnNotModified(), and varyEvaluateMatch().
◆ hasUris()
bool MemObject::hasUris | ( | ) | const |
Definition at line 70 of file MemObject.cc.
References SBuf::isEmpty(), and storeId_.
Referenced by StoreEntry::forcePublicKey(), StoreEntry::setPrivateKey(), setUris(), and storeLog().
◆ inUseCount()
|
static |
Definition at line 47 of file MemObject.cc.
Referenced by Store::Controller::getStats().
◆ isContiguous()
bool MemObject::isContiguous | ( | ) | const |
Definition at line 406 of file MemObject.cc.
References data_hdr, debugs, endOffset(), mem_hdr::hasContigousContentRange(), and inmem_lo.
Referenced by StoreEntry::mayStartSwapOut(), and MemStore::shouldCache().
◆ kickReads()
void MemObject::kickReads | ( | ) |
Definition at line 459 of file MemObject.cc.
References deferredReads, and DelayedAsyncCalls::schedule().
Referenced by store_client::copy(), and storeUnregister().
◆ logUri()
const char * MemObject::logUri | ( | ) | const |
Definition at line 64 of file MemObject.cc.
References SBuf::c_str(), SBuf::isEmpty(), logUri_, and storeId().
Referenced by StoreEntry::adjustVary(), stat(), and storeLog().
◆ lowestMemReaderOffset()
int64_t MemObject::lowestMemReaderOffset | ( | ) | const |
Definition at line 277 of file MemObject.cc.
References clients, LowestMemReader::current, and endOffset().
Referenced by policyLowestOffsetToKeep(), readAheadPolicyCanRead(), and StoreEntry::swapOut().
◆ markEndOfReplyHeaders()
void MemObject::markEndOfReplyHeaders | ( | ) |
Definition at line 220 of file MemObject.cc.
References assert, endOffset(), Http::Message::hdr_sz, and reply_.
Referenced by StoreEntry::startWriting().
◆ MEMPROXY_CLASS()
|
private |
◆ mostBytesAllowed()
DelayId MemObject::mostBytesAllowed | ( | ) | const |
Definition at line 466 of file MemObject.cc.
References clients, dlink_list::head, node::next, and sc.
Referenced by delayRead(), FwdState::establishTunnelThruProxy(), mostBytesWanted(), and HttpStateData::readReply().
◆ mostBytesWanted()
Definition at line 415 of file MemObject.cc.
References DelayId::bytesWanted(), max(), and mostBytesAllowed().
Referenced by StoreEntry::bytesWanted().
◆ objectBytesOnDisk()
int64_t MemObject::objectBytesOnDisk | ( | ) | const |
Definition at line 322 of file MemObject.cc.
References RefCount< C >::getRaw(), StoreIOState::offset(), MemObject::SwapOut::sio, swap_hdr_sz, and swapout.
Referenced by StoreEntry::swapOut(), and trimSwappable().
◆ policyLowestOffsetToKeep()
int64_t MemObject::policyLowestOffsetToKeep | ( | bool | swap | ) | const |
Definition at line 348 of file MemObject.cc.
References Config, endOffset(), inmem_lo, lowestMemReaderOffset(), SquidConfig::maxInMemObjSize, SquidConfig::memory_cache_first, SquidConfig::onoff, and SquidConfig::Store.
Referenced by trimSwappable(), and trimUnSwappable().
◆ readAheadPolicyCanRead()
bool MemObject::readAheadPolicyCanRead | ( | ) | const |
Definition at line 288 of file MemObject.cc.
References baseReply(), Config, debugs, endOffset(), Http::Message::hdr_sz, lowestMemReaderOffset(), and SquidConfig::readAheadGap.
Referenced by StoreEntry::bytesWanted(), delayRead(), and StoreEntry::write().
◆ replaceBaseReply()
void MemObject::replaceBaseReply | ( | const HttpReplyPointer & | r | ) |
(re)sets base reply, usually just replacing the initial/empty object also forgets the updated reply (if any)
Definition at line 128 of file MemObject.cc.
References assert, reply_, and updatedReply_.
Referenced by StoreEntry::replaceHttpReply().
◆ reset()
void MemObject::reset | ( | ) |
Definition at line 264 of file MemObject.cc.
References appliedUpdates, assert, data_hdr, mem_hdr::freeContent(), inmem_lo, reply_, HttpReply::reset(), MemObject::SwapOut::sio, swapout, and updatedReply_.
Referenced by StoreEntry::reset().
◆ setNoDelay()
void MemObject::setNoDelay | ( | bool const | newValue | ) |
Definition at line 431 of file MemObject.cc.
References clients, dlink_list::head, node::next, and sc.
Referenced by StoreEntry::setNoDelay().
◆ setUris()
void MemObject::setUris | ( | char const * | aStoreId, |
char const * | aLogUri, | ||
const HttpRequestMethod & | aMethod | ||
) |
Sets store ID, log URI, and request method (unless already set). Does not clobber the method so that, say, a HEAD hit for a GET entry keeps the GET method that matches the entry key. Same for the other parts of the trio because the entry filling code may expect them to be constant. XXX: Avoid this method. We plan to remove it and make the trio constant after addressing the XXX in MemStore::get().
Definition at line 76 of file MemObject.cc.
References SBuf::clear(), debugs, hasUris(), logUri_, method, storeId_, and urlXXX().
Referenced by StoreEntry::ensureMemObject().
◆ size()
int64_t MemObject::size | ( | ) | const |
Definition at line 229 of file MemObject.cc.
References endOffset(), and object_sz.
Referenced by peerDigestFetchSetStats().
◆ stat()
void MemObject::stat | ( | MemBuf * | mb | ) | const |
Definition at line 189 of file MemObject.cc.
References Packable::appendf(), clients, data_hdr, mem_hdr::endOffset(), RefCount< C >::getRaw(), HttpRequestMethod::image(), MemObject::XitTable::index, MemObject::MemCache::index, inmem_lo, MemObject::XitTable::io, MemObject::MemCache::io, SBuf::isEmpty(), logUri(), memCache, method, object_sz, StoreIOState::offset(), MemObject::MemCache::offset, PRId64, MemObject::SwapOut::queue_offset, MemObject::SwapOut::sio, SQUIDSBUFPH, SQUIDSBUFPRINT, swapout, vary_headers, and xitTable.
Referenced by statStoreEntry().
◆ storeId()
const char * MemObject::storeId | ( | ) | const |
Entry StoreID (usually just Request URI); if a buggy code requests this before the information is available, returns an "[unknown_URI]" string.
Definition at line 53 of file MemObject.cc.
References SBuf::c_str(), DBG_IMPORTANT, debugs, dump(), SBuf::isEmpty(), and storeId_.
Referenced by StoreEntry::adjustVary(), clientReplyContext::cacheHit(), StoreEntry::calcPublicKey(), findPreviouslyCachedEntry(), HeapKeyGen_StoreEntry_GDSF(), HeapKeyGen_StoreEntry_LFUDA(), HeapKeyGen_StoreEntry_LRU(), httpMaybeRemovePublic(), logUri(), refreshCheck(), StoreEntry::url(), and urlXXX().
◆ trimSwappable()
void MemObject::trimSwappable | ( | ) |
Definition at line 371 of file MemObject.cc.
References data_hdr, mem_hdr::freeDataUpto(), inmem_lo, objectBytesOnDisk(), and policyLowestOffsetToKeep().
Referenced by StoreEntry::trimMemory().
◆ trimUnSwappable()
void MemObject::trimUnSwappable | ( | ) |
Definition at line 396 of file MemObject.cc.
References assert, data_hdr, mem_hdr::freeDataUpto(), inmem_lo, and policyLowestOffsetToKeep().
Referenced by StoreEntry::trimMemory().
◆ unlinkRequest()
|
inline |
Definition at line 56 of file MemObject.h.
References request.
Referenced by Store::Controller::handleIdleEntry(), and storeDigestRewriteFinish().
◆ updatedReply()
|
inline |
- Returns
- nil – if no 304 updates since replaceBaseReply()
- combination of baseReply() and 304 updates – after updates
Definition at line 64 of file MemObject.h.
References updatedReply_.
Referenced by StoreEntry::startWriting().
◆ updateReply()
|
inline |
- See also
- updatedReply()
Definition at line 85 of file MemObject.h.
References updatedReply_.
Referenced by StoreEntry::updateOnNotModified().
◆ urlXXX()
|
inline |
Before StoreID, code assumed that MemObject stores Request URI. After StoreID, some old code still incorrectly assumes that. Use this method to mark that incorrect assumption.
Definition at line 138 of file MemObject.h.
References storeId().
Referenced by setUris(), and varyEvaluateMatch().
◆ write()
void MemObject::write | ( | const StoreIOBuffer & | buf | ) |
Definition at line 136 of file MemObject.cc.
References assert, data_hdr, debugs, mem_hdr::endOffset(), StoreIOBuffer::length, StoreIOBuffer::offset, and mem_hdr::write().
Referenced by store_client::maybeWriteFromDiskToMemory(), and StoreEntry::write().
Member Data Documentation
◆ abortCallback
AsyncCallPointer MemObject::abortCallback |
Definition at line 212 of file MemObject.h.
Referenced by StoreEntry::abort(), StoreEntry::registerAbortCallback(), and StoreEntry::unregisterAbortCallback().
◆ appliedUpdates
bool MemObject::appliedUpdates = false |
reflects past Controller::updateOnNotModified(old, e304) calls: for HTTP 304 entries: whether our entry was used as "e304" for other entries: whether our entry was updated as "old"
Definition at line 90 of file MemObject.h.
Referenced by dump(), reset(), Store::Controller::updateOnNotModified(), and StoreEntry::updateOnNotModified().
◆ clients
dlink_list MemObject::clients |
Definition at line 150 of file MemObject.h.
Referenced by addClient(), clientIsFirst(), StoreEntry::invokeHandlers(), lowestMemReaderOffset(), mostBytesAllowed(), setNoDelay(), stat(), and storeUnregister().
◆ data_hdr
mem_hdr MemObject::data_hdr |
Definition at line 148 of file MemObject.h.
Referenced by MemStore::copyFromShmSlice(), MemStore::copyToShmSlice(), doPages(), dump(), endOffset(), isContiguous(), StoreEntry::memoryCachable(), store_client::readFromMemory(), reset(), stat(), trimSwappable(), trimUnSwappable(), write(), and ~MemObject().
◆ deferredReads
|
private |
Definition at line 233 of file MemObject.h.
Referenced by delayRead(), and kickReads().
◆ id
int MemObject::id = 0 |
Definition at line 214 of file MemObject.h.
Referenced by peerCountMcastPeersCreateAndSend(), and StoreEntry::setPrivateKey().
◆ inmem_lo
int64_t MemObject::inmem_lo = 0 |
Definition at line 149 of file MemObject.h.
Referenced by store_client::canReadFromMemory(), dump(), isContiguous(), store_client::maybeWriteFromDiskToMemory(), StoreEntry::mayStartSwapOut(), StoreEntry::memoryCachable(), policyLowestOffsetToKeep(), reset(), StoreEntry::setMemStatus(), stat(), StoreEntry::storeClientType(), StoreEntry::swapOut(), StoreEntry::trimMemory(), trimSwappable(), trimUnSwappable(), and StoreEntry::validToSend().
◆ ircb_data
PeerSelector* MemObject::ircb_data = nullptr |
Definition at line 209 of file MemObject.h.
Referenced by neighborsHtcpReply(), neighborsUdpAck(), neighborsUdpPing(), and peerCountMcastPeersCreateAndSend().
◆ logUri_
|
mutableprivate |
Definition at line 231 of file MemObject.h.
◆ memCache
MemCache MemObject::memCache |
Definition at line 203 of file MemObject.h.
Referenced by MemStore::anchorEntry(), MemStore::anchorToCache(), Store::Controller::anchorToCache(), MemStore::completeWriting(), MemStore::copyToShm(), MemStore::copyToShmSlice(), MemStore::disconnect(), MemStore::evictCached(), StoreEntry::hasMemStore(), StoreEntry::memOutDecision(), operator<<(), MemStore::shouldCache(), MemStore::startCaching(), stat(), StoreEntry::storeWritingCheckpoint(), Store::Controller::syncCollapsed(), MemStore::updateAnchored(), MemStore::updateHeaders(), MemStore::write(), and ~MemObject().
◆ method
HttpRequestMethod MemObject::method |
Definition at line 147 of file MemObject.h.
Referenced by StoreEntry::adjustVary(), StoreEntry::calcPublicKey(), expectedReplySize(), findPreviouslyCachedEntry(), httpMaybeRemovePublic(), setUris(), stat(), storeLog(), and StoreEntry::validLength().
◆ nclients
int MemObject::nclients = 0 |
Definition at line 156 of file MemObject.h.
Referenced by addClient(), clientCount(), StoreEntry::complete(), dump(), errorAppendEntry(), StoreEntry::invokeHandlers(), StoreEntry::storeClientType(), storePendingNClients(), and storeUnregister().
◆ object_sz
int64_t MemObject::object_sz = -1 |
Definition at line 215 of file MemObject.h.
Referenced by MemStore::anchorEntry(), StoreEntry::checkTooSmall(), StoreEntry::complete(), MemStore::copyFromShm(), expectedReplySize(), StoreEntry::objectLen(), size(), stat(), StoreEntry::storeClientType(), and StoreEntry::swapOut().
◆ ping_reply_callback
IRCB* MemObject::ping_reply_callback |
Definition at line 208 of file MemObject.h.
Referenced by MemObject(), neighborsHtcpReply(), neighborsUdpAck(), neighborsUdpPing(), and peerCountMcastPeersCreateAndSend().
◆ repl
RemovalPolicyNode MemObject::repl |
Definition at line 213 of file MemObject.h.
Referenced by Store::Controller::dereferenceIdle(), heap_guessType(), Store::Controller::referenceBusy(), repl_guessType(), StoreEntry::setMemStatus(), LruPolicyData::setPolicyNode(), and HeapPolicyData::setPolicyNode().
◆ reply_
|
private |
- See also
- baseReply()
Definition at line 227 of file MemObject.h.
Referenced by adjustableBaseReply(), baseReply(), dump(), markEndOfReplyHeaders(), MemObject(), replaceBaseReply(), and reset().
◆ request
HttpRequestPointer MemObject::request |
Definition at line 205 of file MemObject.h.
Referenced by StoreEntry::adjustVary(), StoreEntry::calcPublicKey(), CheckQuickAbortIsReasonable(), StoreEntry::complete(), dump(), findPreviouslyCachedEntry(), httpMaybeRemovePublic(), MimeIcon::load(), neighborsHtcpReply(), neighborsUdpAck(), peerCountMcastPeersAbort(), peerCountMcastPeersCreateAndSend(), peerDigestFetchReply(), refreshCheckDigest(), FwdState::Start(), storeDigestRewriteStart(), StoreEntry::timestampsSet(), and unlinkRequest().
◆ start_ping
struct timeval MemObject::start_ping |
Definition at line 207 of file MemObject.h.
Referenced by dump(), MemObject(), neighborsUdpPing(), neighborUpdateRtt(), peerCountHandleIcpReply(), and peerCountMcastPeersCreateAndSend().
◆ storeId_
|
mutableprivate |
◆ swap_hdr_sz
size_t MemObject::swap_hdr_sz = 0 |
Definition at line 216 of file MemObject.h.
Referenced by store_client::fileRead(), store_client::handleBodyFromDisk(), objectBytesOnDisk(), objectSizeForDirSelection(), store_client::readBody(), store_client::readHeader(), storeSwapOutFileClosed(), storeSwapOutStart(), and Store::UnpackHitSwapMeta().
◆ swapout
SwapOut MemObject::swapout |
Definition at line 169 of file MemObject.h.
Referenced by availableForSwapOut(), Rock::SwapDir::disconnect(), doPages(), store_client::fileRead(), Rock::SwapDir::finalizeSwapoutSuccess(), StoreEntry::mayStartSwapOut(), objectBytesOnDisk(), reset(), stat(), statObjectsOpenfdFilter(), storeSwapOutFileClosed(), storeSwapOutStart(), StoreEntry::storeWritingCheckpoint(), StoreEntry::swapOut(), StoreEntry::swapOutDecision(), StoreEntry::swapOutFileClose(), TestRock::testRockSwapOut(), and ~MemObject().
◆ updatedReply_
|
private |
- See also
- updatedReply()
Definition at line 228 of file MemObject.h.
Referenced by adjustableBaseReply(), dump(), freshestReply(), replaceBaseReply(), reset(), updatedReply(), and updateReply().
◆ vary_headers
SBuf MemObject::vary_headers |
Definition at line 221 of file MemObject.h.
Referenced by StoreEntry::adjustVary(), HttpStateData::haveParsedReplyHeaders(), MemStore::shouldCache(), stat(), Store::UnpackNewSwapMetaVaryHeaders(), and varyEvaluateMatch().
◆ xitTable
XitTable MemObject::xitTable |
Definition at line 192 of file MemObject.h.
Referenced by Transients::addReaderEntry(), Transients::addWriterEntry(), CollapsedForwarding::Broadcast(), Transients::completeWriting(), Transients::disconnect(), Transients::evictCached(), Transients::get(), StoreEntry::hasTransients(), Transients::hasWriter(), Transients::isReader(), Transients::isWriter(), Transients::monitorIo(), operator<<(), Transients::readers(), stat(), Transients::status(), and ~MemObject().
The documentation for this class was generated from the following files:
- src/MemObject.h
- src/MemObject.cc
- src/tests/stub_MemObject.cc
- src/tests/testHttpReply.cc
- src/tests/testSBuf.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products