#include <stmem.h>
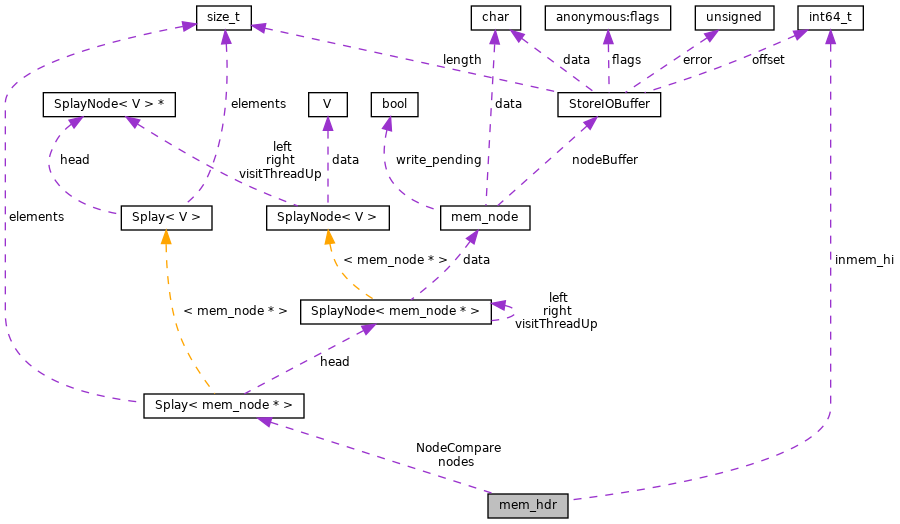
Public Member Functions | |
mem_hdr () | |
~mem_hdr () | |
void | freeContent () |
int64_t | lowestOffset () const |
int64_t | endOffset () const |
int64_t | freeDataUpto (int64_t) |
ssize_t | copy (StoreIOBuffer const &) const |
bool | hasContigousContentRange (Range< int64_t > const &range) const |
bool | write (StoreIOBuffer const &) |
void | dump () const |
size_t | size () const |
mem_node * | getBlockContainingLocation (int64_t location) const |
const Splay< mem_node * > & | getNodes () const |
char * | NodeGet (mem_node *aNode) |
Static Public Attributes | |
static Splay< mem_node * >::SPLAYCMP | NodeCompare |
Private Member Functions | |
void | debugDump () const |
bool | unlink (mem_node *aNode) |
void | appendNode (mem_node *aNode) |
size_t | copyAvailable (mem_node *aNode, int64_t location, size_t amount, char *target) const |
bool | unionNotEmpty (StoreIOBuffer const &) |
mem_node * | nodeToRecieve (int64_t offset) |
size_t | writeAvailable (mem_node *aNode, int64_t location, size_t amount, char const *source) |
Private Attributes | |
int64_t | inmem_hi |
Splay< mem_node * > | nodes |
Detailed Description
Constructor & Destructor Documentation
◆ mem_hdr()
◆ ~mem_hdr()
mem_hdr::~mem_hdr | ( | ) |
Definition at line 339 of file stmem.cc.
References freeContent().
Member Function Documentation
◆ appendNode()
|
private |
Definition at line 130 of file stmem.cc.
References Splay< V >::insert(), NodeCompare, and nodes.
Referenced by nodeToRecieve().
◆ copy()
ssize_t mem_hdr::copy | ( | StoreIOBuffer const & | target | ) | const |
Definition at line 189 of file stmem.cc.
References assert, copyAvailable(), StoreIOBuffer::data, DBG_IMPORTANT, debugDump(), debugs, fatal_dump(), getBlockContainingLocation(), StoreIOBuffer::length, nodes, StoreIOBuffer::offset, StoreIOBuffer::range(), and Splay< V >::size().
Referenced by MemStore::copyToShmSlice(), and store_client::readFromMemory().
◆ copyAvailable()
|
private |
Definition at line 153 of file stmem.cc.
References assert, StoreIOBuffer::data, mem_node::end(), StoreIOBuffer::length, min(), mem_node::nodeBuffer, and StoreIOBuffer::offset.
Referenced by copy().
◆ debugDump()
|
private |
Definition at line 172 of file stmem.cc.
References debugs, endOffset(), getNodes(), lowestOffset(), and Splay< V >::visit().
◆ dump()
void mem_hdr::dump | ( | ) | const |
Definition at line 361 of file stmem.cc.
References DBG_IMPORTANT, debugs, Splay< V >::finish(), nodes, and Splay< V >::start().
Referenced by MemObject::dump().
◆ endOffset()
int64_t mem_hdr::endOffset | ( | ) | const |
Definition at line 45 of file stmem.cc.
References assert, SplayNode< V >::data, mem_node::dataRange(), Splay< V >::finish(), inmem_hi, and nodes.
Referenced by debugDump(), MemObject::dump(), MemObject::endOffset(), MemObject::stat(), testLowAndHigh(), MemObject::write(), write(), and writeAvailable().
◆ freeContent()
void mem_hdr::freeContent | ( | ) |
Definition at line 59 of file stmem.cc.
References debugs, Splay< V >::destroy(), inmem_hi, and nodes.
Referenced by ~mem_hdr(), MemObject::~MemObject(), and MemObject::reset().
◆ freeDataUpto()
int64_t mem_hdr::freeDataUpto | ( | int64_t | target_offset | ) |
Definition at line 81 of file stmem.cc.
References assert, SplayNode< V >::data, debugs, mem_node::end(), Splay< V >::finish(), lowestOffset(), nodes, Splay< V >::start(), and unlink().
Referenced by MemObject::trimSwappable(), and MemObject::trimUnSwappable().
◆ getBlockContainingLocation()
mem_node * mem_hdr::getBlockContainingLocation | ( | int64_t | location | ) | const |
Definition at line 139 of file stmem.cc.
References Splay< V >::find(), StoreIOBuffer::length, mem_node::nodeBuffer, NodeCompare, and nodes.
Referenced by copy(), doPages(), and hasContigousContentRange().
◆ getNodes()
Definition at line 374 of file stmem.cc.
References nodes.
Referenced by debugDump(), and testHdrVisit().
◆ hasContigousContentRange()
bool mem_hdr::hasContigousContentRange | ( | Range< int64_t > const & | range | ) | const |
Definition at line 247 of file stmem.cc.
References Range< C, S >::end, getBlockContainingLocation(), Range< C, S >::size(), and Range< C, S >::start.
Referenced by MemObject::isContiguous(), and testLowAndHigh().
◆ lowestOffset()
int64_t mem_hdr::lowestOffset | ( | ) | const |
Definition at line 34 of file stmem.cc.
References SplayNode< V >::data, mem_node::nodeBuffer, nodes, StoreIOBuffer::offset, and Splay< V >::start().
Referenced by debugDump(), freeDataUpto(), and testLowAndHigh().
◆ NodeGet()
char * mem_hdr::NodeGet | ( | mem_node * | aNode | ) |
Definition at line 26 of file stmem.cc.
References assert, mem_node::data, and mem_node::write_pending.
Referenced by doPages().
◆ nodeToRecieve()
|
private |
Definition at line 271 of file stmem.cc.
References appendNode(), mem_node::canAccept(), SplayNode< V >::data, Splay< V >::find(), StoreIOBuffer::length, mem_node::nodeBuffer, NodeCompare, nodes, Splay< V >::size(), and Splay< V >::start().
Referenced by write().
◆ size()
size_t mem_hdr::size | ( | ) | const |
Definition at line 368 of file stmem.cc.
References nodes, and Splay< V >::size().
Referenced by StoreEntry::memoryCachable().
◆ unionNotEmpty()
|
private |
Definition at line 262 of file stmem.cc.
References assert, Splay< V >::find(), StoreIOBuffer::length, mem_node::nodeBuffer, NodeCompare, nodes, and StoreIOBuffer::offset.
Referenced by write().
◆ unlink()
|
private |
Definition at line 67 of file stmem.cc.
References DBG_CRITICAL, debugs, NodeCompare, nodes, Splay< V >::remove(), and mem_node::write_pending.
Referenced by freeDataUpto().
◆ write()
bool mem_hdr::write | ( | StoreIOBuffer const & | writeBuffer | ) |
Definition at line 305 of file stmem.cc.
References assert, StoreIOBuffer::data, DBG_CRITICAL, debugDump(), debugs, endOffset(), fatal_dump(), StoreIOBuffer::length, nodeToRecieve(), StoreIOBuffer::offset, StoreIOBuffer::range(), unionNotEmpty(), and writeAvailable().
Referenced by MemStore::copyFromShmSlice(), testHdrVisit(), testLowAndHigh(), and MemObject::write().
◆ writeAvailable()
|
private |
Definition at line 104 of file stmem.cc.
References assert, mem_node::canAccept(), StoreIOBuffer::data, debugs, endOffset(), inmem_hi, StoreIOBuffer::length, min(), mem_node::nodeBuffer, StoreIOBuffer::offset, and mem_node::space().
Referenced by write().
Member Data Documentation
◆ inmem_hi
|
private |
Definition at line 52 of file stmem.h.
Referenced by mem_hdr(), endOffset(), freeContent(), and writeAvailable().
◆ NodeCompare
|
static |
Definition at line 42 of file stmem.h.
Referenced by appendNode(), getBlockContainingLocation(), nodeToRecieve(), testSplayOfNodes(), unionNotEmpty(), and unlink().
◆ nodes
Definition at line 53 of file stmem.h.
Referenced by appendNode(), copy(), dump(), endOffset(), freeContent(), freeDataUpto(), getBlockContainingLocation(), getNodes(), lowestOffset(), nodeToRecieve(), size(), unionNotEmpty(), and unlink().
The documentation for this class was generated from the following files: