#include <Transients.h>
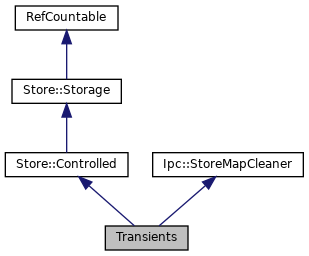
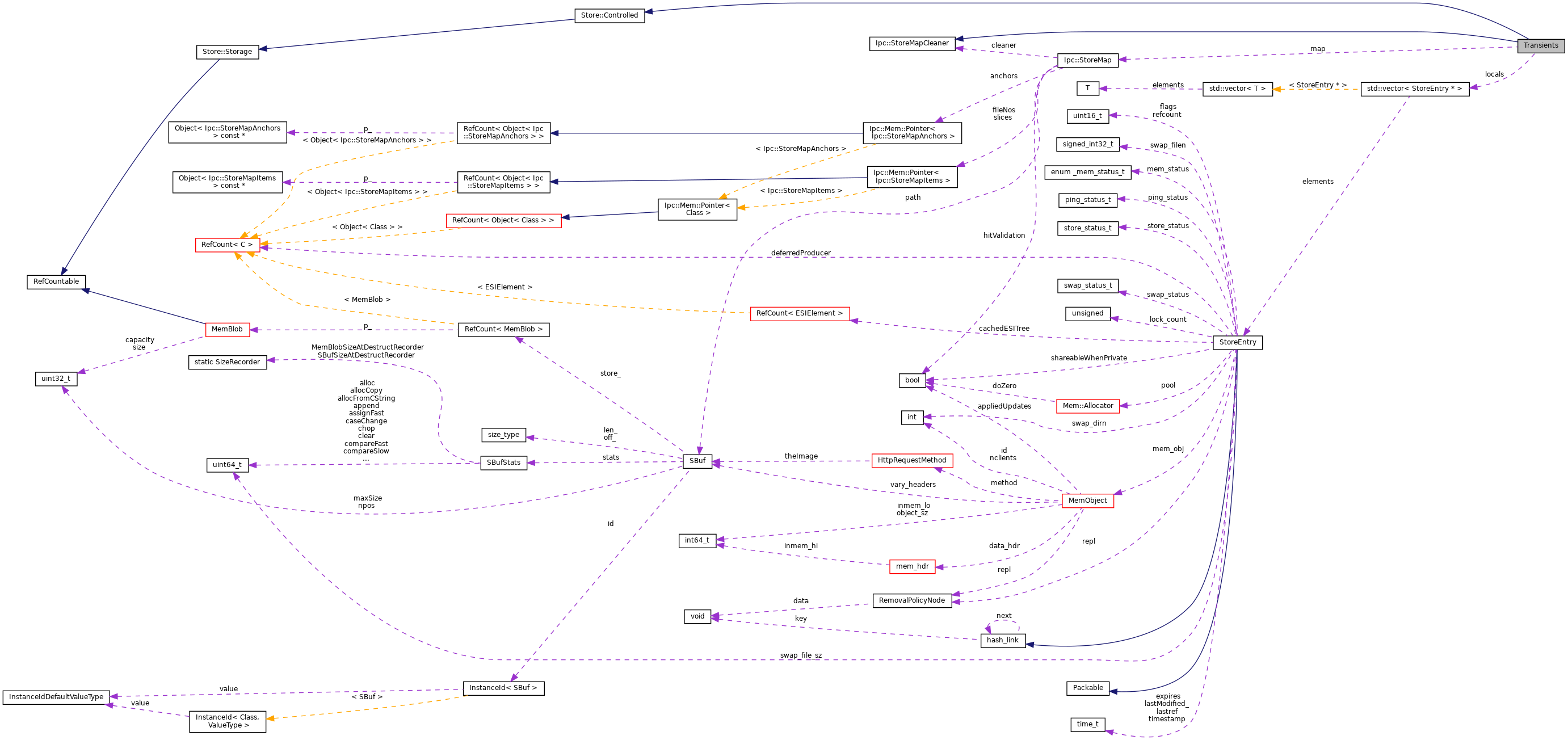
Classes | |
class | EntryStatus |
shared entry metadata, used for synchronization More... | |
Public Member Functions | |
Transients () | |
~Transients () override | |
StoreEntry * | findCollapsed (const sfileno xitIndex) |
return a local, previously collapsed entry More... | |
void | monitorIo (StoreEntry *, const cache_key *, const Store::IoStatus) |
void | completeWriting (const StoreEntry &e) |
called when the in-transit entry has been successfully cached More... | |
void | status (const StoreEntry &e, EntryStatus &entryStatus) const |
copies current shared entry metadata into entryStatus More... | |
int | readers (const StoreEntry &e) const |
number of entry readers some time ago More... | |
void | disconnect (StoreEntry &) |
the caller is done writing or reading the given entry More... | |
StoreEntry * | get (const cache_key *) override |
void | create () override |
create system resources needed for this store to operate in the future More... | |
void | init () override |
uint64_t | maxSize () const override |
uint64_t | minSize () const override |
the minimum size the store will shrink to via normal housekeeping More... | |
uint64_t | currentSize () const override |
current size More... | |
uint64_t | currentCount () const override |
the total number of objects stored right now More... | |
int64_t | maxObjectSize () const override |
the maximum size of a storable object; -1 if unlimited More... | |
void | getStats (StoreInfoStats &stats) const override |
collect statistics More... | |
void | stat (StoreEntry &e) const override |
void | reference (StoreEntry &e) override |
somebody needs this entry (many cache replacement policies need to know) More... | |
bool | dereference (StoreEntry &e) override |
void | evictCached (StoreEntry &) override |
void | evictIfFound (const cache_key *) override |
void | maintain () override |
perform regular periodic maintenance; TODO: move to UFSSwapDir::Maintain More... | |
bool | markedForDeletion (const cache_key *) const |
bool | isReader (const StoreEntry &) const |
whether the entry is in "reading from Transients" I/O state More... | |
bool | isWriter (const StoreEntry &) const |
whether the entry is in "writing to Transients" I/O state More... | |
bool | hasWriter (const StoreEntry &) |
whether we or somebody else is in the "writing to Transients" I/O state More... | |
virtual void | updateHeaders (StoreEntry *) |
make stored metadata and HTTP headers the same as in the given entry More... | |
virtual bool | anchorToCache (StoreEntry &) |
virtual bool | updateAnchored (StoreEntry &) |
virtual int | callback () |
called once every main loop iteration; TODO: Move to UFS code. More... | |
virtual void | sync () |
prepare for shutdown More... | |
Static Public Member Functions | |
static int64_t | EntryLimit () |
calculates maximum number of entries we need to store and map More... | |
static bool | Enabled () |
Can we create and initialize Transients? More... | |
Protected Member Functions | |
void | addEntry (StoreEntry *, const cache_key *, const Store::IoStatus) |
creates a new Transients entry More... | |
void | addWriterEntry (StoreEntry &, const cache_key *) |
addEntry() helper used for cache entry creators/writers More... | |
void | addReaderEntry (StoreEntry &, const cache_key *) |
void | noteFreeMapSlice (const Ipc::StoreMapSliceId sliceId) override |
adjust slice-linked state before a locked Readable slice is erased More... | |
Private Types | |
typedef std::vector< StoreEntry * > | Locals |
Private Attributes | |
TransientsMap * | map |
shared packed info indexed by Store keys, for creating new StoreEntries More... | |
Locals * | locals |
Detailed Description
A Transients entry allows workers to Broadcast() DELETE requests and swapout progress updates. In a collapsed forwarding context, it also represents a CF initiating worker promise to either cache the response or inform the waiting slaves (via false EntryStatus::hasWriter) that caching will not happen. A Transients entry itself does not carry response- or Store-specific metadata.
Definition at line 27 of file Transients.h.
Member Typedef Documentation
◆ Locals
|
private |
Definition at line 105 of file Transients.h.
Constructor & Destructor Documentation
◆ Transients()
Transients::Transients | ( | ) |
Definition at line 35 of file Transients.cc.
◆ ~Transients()
|
override |
Definition at line 39 of file Transients.cc.
Member Function Documentation
◆ addEntry()
|
protected |
Definition at line 216 of file Transients.cc.
References addReaderEntry(), addWriterEntry(), assert, StoreEntry::hasTransients(), Store::ioReading, Store::ioWriting, map, StoreEntry::mem_obj, and Must.
Referenced by monitorIo().
◆ addReaderEntry()
|
protected |
addEntry() helper used for cache readers readers do not modify the cache, but they must create a Transients entry
Definition at line 253 of file Transients.cc.
References Here, Store::ioReading, map, StoreEntry::mem_obj, MemObject::XitTable::open(), Ipc::StoreMap::openOrCreateForReading(), and MemObject::xitTable.
Referenced by addEntry().
◆ addWriterEntry()
|
protected |
Definition at line 233 of file Transients.cc.
References Here, Store::ioWriting, map, StoreEntry::mem_obj, MemObject::XitTable::open(), Ipc::StoreMap::openForWriting(), Ipc::StoreMap::startAppending(), and MemObject::xitTable.
Referenced by addEntry().
◆ anchorToCache()
|
inlinevirtualinherited |
tie StoreEntry to this storage if this storage has a matching entry
- Return values
-
true if this storage has a matching entry
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 39 of file Controlled.h.
Referenced by Store::Disks::anchorToCache().
◆ callback()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Disks, and Store::Controller.
◆ completeWriting()
void Transients::completeWriting | ( | const StoreEntry & | e | ) |
Definition at line 291 of file Transients.cc.
References assert, CollapsedForwarding::Broadcast(), debugs, StoreEntry::hasTransients(), MemObject::XitTable::index, MemObject::XitTable::io, Store::ioReading, isWriter(), map, StoreEntry::mem_obj, Ipc::StoreMap::switchWritingToReading(), and MemObject::xitTable.
◆ create()
|
inlineoverridevirtual |
Implements Store::Storage.
Definition at line 62 of file Transients.h.
◆ currentCount()
|
overridevirtual |
Implements Store::Storage.
Definition at line 125 of file Transients.cc.
References Ipc::StoreMap::entryCount(), and map.
Referenced by getStats(), and stat().
◆ currentSize()
|
overridevirtual |
◆ dereference()
|
overridevirtual |
somebody no longer needs this entry (usually after calling reference()) return false iff the idle entry should be destroyed
Implements Store::Controlled.
Definition at line 144 of file Transients.cc.
◆ disconnect()
void Transients::disconnect | ( | StoreEntry & | entry | ) |
Definition at line 337 of file Transients.cc.
References assert, CollapsedForwarding::Broadcast(), Ipc::StoreMap::closeForReadingAndFreeIdle(), Ipc::StoreMap::closeForWriting(), debugs, StoreEntry::hasTransients(), isReader(), isWriter(), locals, map, StoreEntry::mem_obj, and MemObject::xitTable.
◆ Enabled()
|
inlinestatic |
Definition at line 91 of file Transients.h.
References EntryLimit().
Referenced by Store::Controller::init(), and init().
◆ EntryLimit()
|
static |
Definition at line 361 of file Transients.cc.
References Config, SquidConfig::shared_transient_entries_limit, Store::Controller::SmpAware(), and UsingSmp().
Referenced by TransientsRr::create(), Enabled(), and init().
◆ evictCached()
|
overridevirtual |
Prevent new get() calls from returning the matching entry. If the matching entry is unused, it may be removed from the store now. The store entry is matched using either e
attachment info or e.key
.
Implements Store::Storage.
Definition at line 312 of file Transients.cc.
References CollapsedForwarding::Broadcast(), debugs, Ipc::StoreMap::freeEntry(), StoreEntry::hasTransients(), MemObject::XitTable::index, map, StoreEntry::mem_obj, and MemObject::xitTable.
◆ evictIfFound()
|
overridevirtual |
An evictCached() equivalent for callers that did not get() a StoreEntry. Callers with StoreEntry objects must use evictCached() instead.
Implements Store::Storage.
Definition at line 326 of file Transients.cc.
References CollapsedForwarding::Broadcast(), Ipc::StoreMap::fileNoByKey(), Ipc::StoreMap::freeEntry(), and map.
◆ findCollapsed()
StoreEntry * Transients::findCollapsed | ( | const sfileno | xitIndex | ) |
Definition at line 181 of file Transients.cc.
References assert, debugs, locals, map, and MapLabel().
◆ get()
|
overridevirtual |
- Returns
- a possibly unlocked/unregistered stored entry with key (or nil) The returned entry might not match the caller's Store ID or method. The caller must abandon()/release() the entry or register it with Root(). This method must not trigger slow I/O operations (e.g., disk swap in).
Implements Store::Controlled.
Definition at line 151 of file Transients.cc.
References assert, Ipc::StoreMap::closeForReadingAndFreeIdle(), StoreEntry::createMemObject(), debugs, EBIT_TEST, Store::ioReading, KEY_PRIVATE, locals, map, StoreEntry::mem_obj, MemObject::XitTable::open(), Ipc::StoreMap::openForReading(), and MemObject::xitTable.
◆ getStats()
|
overridevirtual |
Implements Store::Storage.
Definition at line 61 of file Transients.cc.
References Ipc::Mem::PageId::cachePage, StoreInfoStats::Part::capacity, StoreInfoStats::Part::count, currentCount(), StoreInfoStats::mem, Ipc::Mem::PageLevel(), Ipc::Mem::PageLimit(), Ipc::Mem::PageSize(), StoreInfoStats::Mem::shared, and StoreInfoStats::Part::size.
◆ hasWriter()
bool Transients::hasWriter | ( | const StoreEntry & | e | ) |
Definition at line 265 of file Transients.cc.
References StoreEntry::hasTransients(), MemObject::XitTable::index, map, StoreEntry::mem_obj, Ipc::StoreMap::peekAtWriter(), and MemObject::xitTable.
◆ init()
|
overridevirtual |
Start preparing the store for use. To check readiness, callers should use readable() and writable() methods.
Implements Store::Storage.
Definition at line 46 of file Transients.cc.
References assert, Ipc::StoreMap::cleaner, Ipc::StoreMap::disableHitValidation(), Enabled(), EntryLimit(), locals, map, MapLabel(), and Must.
Referenced by Store::Controller::init().
◆ isReader()
bool Transients::isReader | ( | const StoreEntry & | e | ) | const |
Definition at line 375 of file Transients.cc.
References MemObject::XitTable::io, Store::ioReading, StoreEntry::mem_obj, and MemObject::xitTable.
Referenced by disconnect().
◆ isWriter()
bool Transients::isWriter | ( | const StoreEntry & | e | ) | const |
Definition at line 381 of file Transients.cc.
References MemObject::XitTable::io, Store::ioWriting, StoreEntry::mem_obj, and MemObject::xitTable.
Referenced by completeWriting(), disconnect(), and status().
◆ maintain()
|
overridevirtual |
Implements Store::Storage.
Definition at line 98 of file Transients.cc.
◆ markedForDeletion()
bool Transients::markedForDeletion | ( | const cache_key * | key | ) | const |
Whether an entry with the given public key exists and (but) was marked for removal some time ago; get(key) returns nil in such cases.
Definition at line 368 of file Transients.cc.
References assert, map, and Ipc::StoreMap::markedForDeletion().
◆ maxObjectSize()
|
overridevirtual |
◆ maxSize()
|
overridevirtual |
The maximum size the store will support in normal use. Inaccuracy is permitted, but may throw estimates for memory etc out of whack.
Implements Store::Storage.
Definition at line 110 of file Transients.cc.
References max().
Referenced by stat().
◆ minSize()
|
overridevirtual |
Implements Store::Storage.
Definition at line 104 of file Transients.cc.
◆ monitorIo()
void Transients::monitorIo | ( | StoreEntry * | e, |
const cache_key * | key, | ||
const Store::IoStatus | direction | ||
) |
start listening for remote DELETE requests targeting either a complete StoreEntry (ioReading) or a being-formed miss StoreEntry (ioWriting)
Definition at line 197 of file Transients.cc.
References addEntry(), assert, StoreEntry::hasTransients(), MemObject::XitTable::index, locals, StoreEntry::mem_obj, and MemObject::xitTable.
◆ noteFreeMapSlice()
|
overrideprotectedvirtual |
Implements Ipc::StoreMapCleaner.
Definition at line 273 of file Transients.cc.
◆ readers()
int Transients::readers | ( | const StoreEntry & | e | ) | const |
Definition at line 302 of file Transients.cc.
References assert, StoreEntry::hasTransients(), MemObject::XitTable::index, Ipc::StoreMapAnchor::lock, map, StoreEntry::mem_obj, Ipc::StoreMap::peekAtEntry(), Ipc::ReadWriteLock::readers, and MemObject::xitTable.
◆ reference()
|
overridevirtual |
Implements Store::Controlled.
Definition at line 138 of file Transients.cc.
◆ stat()
|
overridevirtual |
Output stats to the provided store entry. TODO: make these calls asynchronous
Implements Store::Storage.
Definition at line 78 of file Transients.cc.
References currentCount(), currentSize(), Math::doublePercent(), Ipc::StoreMap::entryLimit(), map, maxSize(), PRId64, and storeAppendPrintf().
◆ status()
void Transients::status | ( | const StoreEntry & | e, |
Transients::EntryStatus & | entryStatus | ||
) | const |
Definition at line 279 of file Transients.cc.
References assert, StoreEntry::hasTransients(), Transients::EntryStatus::hasWriter, MemObject::XitTable::index, isWriter(), map, StoreEntry::mem_obj, Ipc::StoreMap::readableEntry(), Transients::EntryStatus::waitingToBeFreed, Ipc::StoreMap::writeableEntry(), and MemObject::xitTable.
◆ sync()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Controller, and Store::Disks.
◆ updateAnchored()
|
inlinevirtualinherited |
Update a local Transients entry with fresh info from this cache (if any). Return true iff the cache supports Transients entries and the given local Transients entry is now in sync with this storage.
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 44 of file Controlled.h.
Referenced by Store::Disks::updateAnchored().
◆ updateHeaders()
|
inlinevirtualinherited |
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 35 of file Controlled.h.
Referenced by Store::Disks::updateHeaders().
Member Data Documentation
◆ locals
|
private |
local collapsed reader and writer entries, indexed by transient ID, for syncing old StoreEntries
Definition at line 108 of file Transients.h.
Referenced by disconnect(), findCollapsed(), get(), init(), monitorIo(), and ~Transients().
◆ map
|
private |
Definition at line 103 of file Transients.h.
Referenced by addEntry(), addReaderEntry(), addWriterEntry(), completeWriting(), currentCount(), disconnect(), evictCached(), evictIfFound(), findCollapsed(), get(), hasWriter(), init(), markedForDeletion(), readers(), stat(), status(), and ~Transients().
The documentation for this class was generated from the following files:
- src/Transients.h
- src/Transients.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products