#include <ReadWriteLock.h>
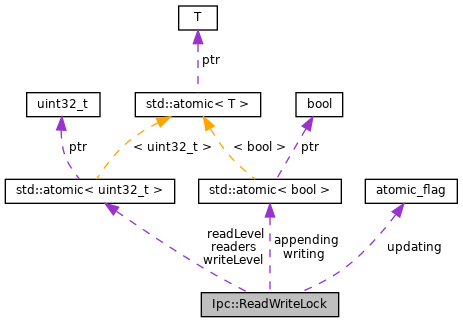
Public Member Functions | |
ReadWriteLock () | |
bool | lockShared () |
lock for reading or return false More... | |
bool | lockExclusive () |
lock for modification or return false More... | |
bool | lockHeaders () |
lock for [readable] metadata update or return false More... | |
void | unlockShared () |
undo successful sharedLock() More... | |
void | unlockExclusive () |
undo successful exclusiveLock() More... | |
void | unlockHeaders () |
undo successful lockHeaders() More... | |
void | switchExclusiveToShared () |
bool | unlockSharedAndSwitchToExclusive () |
void | startAppending () |
writer keeps its lock but also allows reading More... | |
bool | stopAppendingAndRestoreExclusive () |
void | updateStats (ReadWriteLockStats &stats) const |
adds approximate current stats to the supplied ones More... | |
Public Attributes | |
std::atomic< uint32_t > | readers |
number of reading users More... | |
std::atomic< bool > | writing |
there is a writing user (there can be at most 1) More... | |
std::atomic< bool > | appending |
the writer has promised to only append More... | |
std::atomic_flag | updating |
a reader is updating metadata/headers More... | |
Private Member Functions | |
bool | finalizeExclusive () |
Private Attributes | |
std::atomic< uint32_t > | readLevel |
number of users reading (or trying to) More... | |
std::atomic< uint32_t > | writeLevel |
number of users writing (or trying to write) More... | |
Detailed Description
an atomic readers-writer or shared-exclusive lock suitable for maps/tables Also supports reading-while-appending mode when readers and writer are allowed to access the same locked object because the writer promises to only append new data and all size-related object properties are atomic.
Definition at line 26 of file ReadWriteLock.h.
Constructor & Destructor Documentation
◆ ReadWriteLock()
|
inline |
Definition at line 29 of file ReadWriteLock.h.
Member Function Documentation
◆ finalizeExclusive()
|
private |
common lockExclusive() and unlockSharedAndSwitchToExclusive() logic: either finish exclusive locking or bail properly
- Precondition
- The caller must (be the first to) increment writeLevel.
- Returns
- whether we got the exclusive lock
Definition at line 28 of file ReadWriteLock.cc.
References appending, assert, readLevel, writeLevel, and writing.
◆ lockExclusive()
bool Ipc::ReadWriteLock::lockExclusive | ( | ) |
Definition at line 53 of file ReadWriteLock.cc.
Referenced by Ipc::MemMap::free(), Ipc::StoreMap::freeEntry(), Ipc::StoreMap::freeEntryByKey(), Ipc::MemMap::openForWritingAt(), Ipc::StoreMap::openForWritingAt(), and Ipc::StoreMap::purgeOne().
◆ lockHeaders()
bool Ipc::ReadWriteLock::lockHeaders | ( | ) |
Definition at line 63 of file ReadWriteLock.cc.
Referenced by Ipc::StoreMap::openForUpdating().
◆ lockShared()
bool Ipc::ReadWriteLock::lockShared | ( | ) |
Definition at line 41 of file ReadWriteLock.cc.
Referenced by Ipc::StoreMap::freeEntryByKey(), Ipc::MemMap::openForReadingAt(), and Ipc::StoreMap::openForReadingAt().
◆ startAppending()
void Ipc::ReadWriteLock::startAppending | ( | ) |
Definition at line 124 of file ReadWriteLock.cc.
References assert.
Referenced by Ipc::StoreMap::startAppending().
◆ stopAppendingAndRestoreExclusive()
bool Ipc::ReadWriteLock::stopAppendingAndRestoreExclusive | ( | ) |
writer keeps its lock and disallows future readers
- Returns
- whether access became exclusive (i.e. no readers) \prec appending is true
Definition at line 131 of file ReadWriteLock.cc.
References assert.
Referenced by Ipc::StoreMap::abortWriting().
◆ switchExclusiveToShared()
void Ipc::ReadWriteLock::switchExclusiveToShared | ( | ) |
stop writing, start reading
Definition at line 100 of file ReadWriteLock.cc.
References assert.
Referenced by Ipc::StoreMap::closeForUpdating(), Ipc::MemMap::switchWritingToReading(), and Ipc::StoreMap::switchWritingToReading().
◆ unlockExclusive()
void Ipc::ReadWriteLock::unlockExclusive | ( | ) |
Definition at line 83 of file ReadWriteLock.cc.
References assert.
Referenced by Ipc::StoreMap::abortWriting(), Ipc::MemMap::closeForWriting(), Ipc::StoreMap::closeForWriting(), Ipc::StoreMap::forgetWritingEntry(), Ipc::StoreMap::freeChain(), Ipc::StoreMap::freeEntryByKey(), Ipc::MemMap::freeLocked(), Ipc::MemMap::openForWritingAt(), Ipc::StoreMap::openForWritingAt(), and Ipc::StoreMap::purgeOne().
◆ unlockHeaders()
void Ipc::ReadWriteLock::unlockHeaders | ( | ) |
Definition at line 92 of file ReadWriteLock.cc.
References Ipc::AssertFlagIsSet().
Referenced by Ipc::StoreMap::abortUpdating(), and Ipc::StoreMap::closeForUpdating().
◆ unlockShared()
void Ipc::ReadWriteLock::unlockShared | ( | ) |
Definition at line 75 of file ReadWriteLock.cc.
References assert.
Referenced by Ipc::MemMap::closeForReading(), Ipc::StoreMap::closeForReading(), Ipc::StoreMap::freeEntryByKey(), Ipc::MemMap::openForReadingAt(), and Ipc::StoreMap::openForReadingAt().
◆ unlockSharedAndSwitchToExclusive()
bool Ipc::ReadWriteLock::unlockSharedAndSwitchToExclusive | ( | ) |
same as unlockShared() but also attempts to get a writer lock beforehand
- Returns
- whether the writer lock was acquired
Definition at line 109 of file ReadWriteLock.cc.
References assert.
◆ updateStats()
void Ipc::ReadWriteLock::updateStats | ( | ReadWriteLockStats & | stats | ) | const |
Definition at line 148 of file ReadWriteLock.cc.
References Ipc::ReadWriteLockStats::appenders, Ipc::ReadWriteLockStats::count, Ipc::ReadWriteLockStats::idle, Ipc::ReadWriteLockStats::readable, Ipc::ReadWriteLockStats::readers, Ipc::ReadWriteLockStats::writeable, and Ipc::ReadWriteLockStats::writers.
Member Data Documentation
◆ appending
std::atomic<bool> Ipc::ReadWriteLock::appending |
Definition at line 56 of file ReadWriteLock.h.
Referenced by Ipc::StoreMap::abortWriting(), finalizeExclusive(), and Ipc::operator<<().
◆ readers
|
mutable |
Definition at line 54 of file ReadWriteLock.h.
Referenced by Ipc::operator<<(), Transients::readers(), Ipc::MemMapSlot::reading(), and Ipc::StoreMapAnchor::reading().
◆ readLevel
|
mutableprivate |
Definition at line 62 of file ReadWriteLock.h.
Referenced by finalizeExclusive().
◆ updating
std::atomic_flag Ipc::ReadWriteLock::updating |
Definition at line 57 of file ReadWriteLock.h.
Referenced by Ipc::StoreMap::abortUpdating(), and Ipc::StoreMap::closeForUpdating().
◆ writeLevel
|
private |
Definition at line 63 of file ReadWriteLock.h.
Referenced by finalizeExclusive().
◆ writing
std::atomic<bool> Ipc::ReadWriteLock::writing |
Definition at line 55 of file ReadWriteLock.h.
Referenced by finalizeExclusive(), Ipc::operator<<(), Ipc::MemMapSlot::writing(), and Ipc::StoreMapAnchor::writing().
The documentation for this class was generated from the following files:
- src/ipc/ReadWriteLock.h
- src/ipc/ReadWriteLock.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products