#include <UFSSwapDir.h>
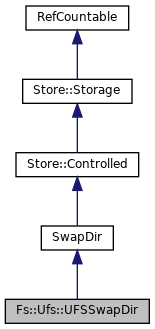

Public Types | |
typedef RefCount< Disk > | Pointer |
Public Member Functions | |
UFSSwapDir (char const *aType, const char *aModuleType) | |
~UFSSwapDir () override | |
void | create () override |
create system resources needed for this store to operate in the future More... | |
void | init () override |
void | dump (StoreEntry &) const override |
bool | doubleCheck (StoreEntry &) override |
bool | unlinkdUseful () const override |
whether SwapDir may benefit from unlinkd More... | |
void | statfs (StoreEntry &) const override |
void | maintain () override |
perform regular periodic maintenance; TODO: move to UFSSwapDir::Maintain More... | |
void | evictCached (StoreEntry &) override |
void | evictIfFound (const cache_key *) override |
bool | canStore (const StoreEntry &e, int64_t diskSpaceNeeded, int &load) const override |
check whether we can store the entry; if we can, report current load More... | |
void | reference (StoreEntry &) override |
somebody needs this entry (many cache replacement policies need to know) More... | |
bool | dereference (StoreEntry &) override |
StoreIOState::Pointer | createStoreIO (StoreEntry &, StoreIOState::STIOCB *, void *) override |
StoreIOState::Pointer | openStoreIO (StoreEntry &, StoreIOState::STIOCB *, void *) override |
void | openLog () override |
void | closeLog () override |
int | writeCleanStart () override |
void | writeCleanDone () override |
void | logEntry (const StoreEntry &e, int op) const override |
void | parse (int index, char *path) override |
void | reconfigure () override |
int | callback () override |
called once every main loop iteration; TODO: Move to UFS code. More... | |
void | sync () override |
prepare for shutdown More... | |
void | finalizeSwapoutSuccess (const StoreEntry &) override |
finalize the successful swapout that has been already noticed by Store More... | |
void | finalizeSwapoutFailure (StoreEntry &) override |
abort the failed swapout that has been already noticed by Store More... | |
uint64_t | currentSize () const override |
current size More... | |
uint64_t | currentCount () const override |
the total number of objects stored right now More... | |
ConfigOption * | getOptionTree () const override |
bool | smpAware () const override |
whether this disk storage is capable of serving multiple workers More... | |
bool | hasReadableEntry (const StoreEntry &) const override |
void | unlinkFile (sfileno f) |
char * | fullPath (sfileno, char *) const |
void | closeTmpSwapLog () |
FILE * | openTmpSwapLog (int *clean_flag, int *zero_flag) |
char * | swapSubDir (int subdirn) const |
int | mapBitTest (sfileno filn) |
void | mapBitReset (sfileno filn) |
void | mapBitSet (sfileno filn) |
StoreEntry * | addDiskRestore (const cache_key *key, sfileno file_number, uint64_t swap_file_sz, time_t expires, time_t timestamp, time_t lastref, time_t lastmod, uint32_t refcount, uint16_t flags, int clean) |
int | validFileno (sfileno filn, int flag) const |
int | mapBitAllocate () |
bool | validL2 (int) const |
bool | validL1 (int) const |
void | replacementAdd (StoreEntry *e) |
void | replacementRemove (StoreEntry *e) |
const char * | type () const |
virtual bool | needsDiskStrand () const |
needs a dedicated kid process More... | |
virtual bool | active () const |
virtual bool | doReportStat () const |
whether stat should be reported by this SwapDir More... | |
virtual void | diskFull () |
StoreEntry * | get (const cache_key *) override |
uint64_t | maxSize () const override |
uint64_t | minSize () const override |
the minimum size the store will shrink to via normal housekeeping More... | |
int64_t | maxObjectSize () const override |
the maximum size of a storable object; -1 if unlimited More... | |
void | maxObjectSize (int64_t newMax) |
void | getStats (StoreInfoStats &stats) const override |
collect statistics More... | |
void | stat (StoreEntry &) const override |
int64_t | minObjectSize () const |
the size of the smallest entry this cache_dir can store More... | |
bool | objectSizeIsAcceptable (int64_t objSize) const |
virtual void | disconnect (StoreEntry &) |
called when the entry is about to forget its association with cache_dir More... | |
bool | canLog (StoreEntry const &e) const |
virtual void | updateHeaders (StoreEntry *) |
make stored metadata and HTTP headers the same as in the given entry More... | |
virtual bool | anchorToCache (StoreEntry &) |
virtual bool | updateAnchored (StoreEntry &) |
Static Public Member Functions | |
static bool | IsUFSDir (SwapDir *sd) |
static int | DirClean (int swap_index) |
static bool | FilenoBelongsHere (int fn, int cachedir, int level1dir, int level2dir) |
Public Attributes | |
Fs::Ufs::UFSStrategy * | IO |
void * | fsdata |
char * | path |
int | index |
int | disker |
disker kid id dedicated to this SwapDir or -1 More... | |
RemovalPolicy * | repl |
int | removals |
int | scanned |
struct Store::Disk::Flags | flags |
CleanLog * | cleanLog |
struct { | |
int blksize | |
} | fs |
Protected Member Functions | |
void | parseOptions (int reconfiguring) |
void | dumpOptions (StoreEntry *e) const |
virtual bool | allowOptionReconfigure (const char *const) const |
int64_t | sizeInBlocks (const int64_t size) const |
Protected Attributes | |
FileMap * | map |
int | suggest |
int | l1 |
int | l2 |
uint64_t | max_size |
maximum allocatable size of the storage area More... | |
int64_t | min_objsize |
minimum size of any object stored here (-1 for no limit) More... | |
int64_t | max_objsize |
maximum size of any object stored here (-1 for no limit) More... | |
Private Member Functions | |
void | parseSizeL1L2 () |
bool | pathIsDirectory (const char *path) const |
bool | verifyCacheDirs () |
void | rebuild () |
int | createDirectory (const char *path, int) |
void | createSwapSubDirs () |
void | dumpEntry (StoreEntry &) const |
SBuf | logFile (char const *ext=nullptr) const |
void | changeIO (DiskIOModule *) |
bool | optionIOParse (char const *option, const char *value, int reconfiguring) |
void | optionIODump (StoreEntry *e) const |
bool | optionReadOnlyParse (char const *option, const char *value, int reconfiguring) |
void | optionReadOnlyDump (StoreEntry *e) const |
bool | optionObjectSizeParse (char const *option, const char *value, int reconfiguring) |
void | optionObjectSizeDump (StoreEntry *e) const |
Static Private Member Functions | |
static int | HandleCleanEvent () |
safely cleans a few unused files if possible More... | |
Private Attributes | |
int | swaplog_fd |
ConfigOptionVector * | currentIOOptions |
const char * | ioType |
uint64_t | cur_size |
currently used space in the storage area More... | |
uint64_t | n_disk_objects |
total number of objects stored More... | |
bool | rebuilding_ |
whether RebuildState is writing the new swap.state More... | |
const char * | theType |
Static Private Attributes | |
static size_t | NumberOfUFSDirs = 0 |
static int * | UFSDirToGlobalDirMapping = nullptr |
static EVH | CleanEvent |
Detailed Description
Definition at line 30 of file UFSSwapDir.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Constructor & Destructor Documentation
◆ UFSSwapDir()
Fs::Ufs::UFSSwapDir::UFSSwapDir | ( | char const * | aType, |
const char * | aModuleType | ||
) |
Definition at line 307 of file UFSSwapDir.cc.
References DiskIOModule::Find(), and IO.
◆ ~UFSSwapDir()
|
override |
Definition at line 328 of file UFSSwapDir.cc.
References file_close(), and xfree.
Member Function Documentation
◆ active()
|
virtualinherited |
may be used in this strand
Definition at line 236 of file Disk.cc.
References IamWorkerProcess(), and KidIdentifier.
Referenced by Store::Disks::anchorToCache().
◆ addDiskRestore()
StoreEntry * Fs::Ufs::UFSSwapDir::addDiskRestore | ( | const cache_key * | key, |
sfileno | file_number, | ||
uint64_t | swap_file_sz, | ||
time_t | expires, | ||
time_t | timestamp, | ||
time_t | lastref, | ||
time_t | lastmod, | ||
uint32_t | refcount, | ||
uint16_t | flags, | ||
int | clean | ||
) |
Add a new object to the cache with empty memory copy and pointer to disk
This method is used to rebuild a store from disk
Definition at line 788 of file UFSSwapDir.cc.
References asHex(), StoreEntry::attachToDisk(), debugs, EBIT_CLR, ENTRY_VALIDATED, StoreEntry::expires, StoreEntry::flags, StoreEntry::hashInsert(), StoreEntry::lastModified(), StoreEntry::lastref, n_disk_objects, NOT_IN_MEMORY, PING_NONE, StoreEntry::ping_status, StoreEntry::refcount, StoreEntry::setMemStatus(), STORE_OK, StoreEntry::store_status, storeKeyText(), StoreEntry::swap_file_sz, StoreEntry::swap_filen, SWAPOUT_DONE, and StoreEntry::timestamp.
◆ allowOptionReconfigure()
|
inlineprotectedvirtualinherited |
Reimplemented in Rock::SwapDir.
Definition at line 85 of file Disk.h.
Referenced by Rock::SwapDir::allowOptionReconfigure().
◆ anchorToCache()
|
inlinevirtualinherited |
tie StoreEntry to this storage if this storage has a matching entry
- Return values
-
true if this storage has a matching entry
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 39 of file Controlled.h.
Referenced by Store::Disks::anchorToCache().
◆ callback()
|
overridevirtual |
Reimplemented from Store::Storage.
Definition at line 1256 of file UFSSwapDir.cc.
◆ canLog()
|
inherited |
Definition at line 188 of file Disk.cc.
References EBIT_TEST, ENTRY_SPECIAL, StoreEntry::flags, StoreEntry::hasDisk(), KEY_PRIVATE, RELEASE_REQUEST, StoreEntry::swap_file_sz, and StoreEntry::swappedOut().
◆ canStore()
|
overridevirtual |
Implements Store::Disk.
Definition at line 115 of file UFSSwapDir.cc.
References Store::Disk::canStore(), IO, Fs::Ufs::UFSStrategy::load(), and Fs::Ufs::UFSStrategy::shedLoad().
◆ changeIO()
|
private |
Definition at line 194 of file UFSSwapDir.cc.
References DiskIOModule::createStrategy(), safe_free, DiskIOModule::type(), and xstrdup.
◆ closeLog()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 755 of file UFSSwapDir.cc.
References assert, debugs, file_close(), and safe_free.
◆ closeTmpSwapLog()
void Fs::Ufs::UFSSwapDir::closeTmpSwapLog | ( | ) |
Definition at line 831 of file UFSSwapDir.cc.
References assert, SBuf::c_str(), DBG_IMPORTANT, debugs, fatalf(), file_close(), file_open(), FileRename(), O_BINARY, SQUIDSBUFPH, SQUIDSBUFPRINT, and xstrerr().
◆ create()
|
overridevirtual |
Implements Store::Storage.
Definition at line 300 of file UFSSwapDir.cc.
References debugs.
Referenced by TestUfs::testUfsSearch().
◆ createDirectory()
Definition at line 604 of file UFSSwapDir.cc.
References DBG_IMPORTANT, debugs, fatalf(), getCurrentTime(), and xstrerr().
◆ createStoreIO()
|
overridevirtual |
Implements Store::Disk.
Definition at line 546 of file UFSSwapDir.cc.
◆ createSwapSubDirs()
|
private |
Definition at line 665 of file UFSSwapDir.cc.
References DBG_IMPORTANT, debugs, LOCAL_ARRAY, and MAXPATHLEN.
◆ currentCount()
|
inlineoverridevirtual |
◆ currentSize()
|
inlineoverridevirtual |
◆ dereference()
|
overridevirtual |
somebody no longer needs this entry (usually after calling reference()) return false iff the idle entry should be destroyed
Implements Store::Controlled.
Definition at line 534 of file UFSSwapDir.cc.
References debugs, StoreEntry::repl, StoreEntry::swap_dirn, and StoreEntry::swap_filen.
◆ DirClean()
Definition at line 1312 of file UFSSwapDir.cc.
References SBuf::appendf(), asHex(), assert, SBuf::c_str(), DBG_CRITICAL, debugs, FilenoBelongsHere(), StatCounters::files_cleaned, INDEXSD, l1, l2, mapBitTest(), MYNAME, Store::Disk::path, rev_int_sort(), safeunlink(), statCounter, StatCounters::swap, validFileno(), and xstrerr().
◆ disconnect()
|
inlinevirtualinherited |
Reimplemented in Rock::SwapDir.
Definition at line 71 of file Disk.h.
Referenced by destroyStoreEntry().
◆ diskFull()
|
virtualinherited |
Notify this disk that it is full. XXX move into a protected api call between store files and their stores, rather than a top level api call
Reimplemented in Rock::SwapDir.
Definition at line 145 of file Disk.cc.
References DBG_IMPORTANT, and debugs.
Referenced by storeSwapOutFileClosed().
◆ doReportStat()
|
inlinevirtualinherited |
Reimplemented in Rock::SwapDir.
◆ doubleCheck()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 349 of file UFSSwapDir.cc.
References DBG_CRITICAL, debugs, StoreEntry::swap_file_sz, and StoreEntry::swap_filen.
◆ dump()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 1228 of file UFSSwapDir.cc.
References PRIu64, and storeAppendPrintf().
◆ dumpEntry()
|
private |
Definition at line 341 of file UFSSwapDir.cc.
References asHex(), DBG_CRITICAL, debugs, StoreEntry::dump(), and StoreEntry::swap_filen.
◆ dumpOptions()
|
protectedinherited |
Definition at line 307 of file Disk.cc.
References ConfigOption::dump().
◆ evictCached()
|
overridevirtual |
Prevent new get() calls from returning the matching entry. If the matching entry is unused, it may be removed from the store now. The store entry is matched using either e
attachment info or e.key
.
Implements Store::Storage.
Definition at line 1178 of file UFSSwapDir.cc.
References debugs, StoreEntry::detachFromDisk(), StoreEntry::hasDisk(), StoreEntry::locked(), n_disk_objects, StoreEntry::swap_file_sz, StoreEntry::swap_filen, StoreEntry::swappedOut(), and unlinkFile().
◆ evictIfFound()
|
overridevirtual |
An evictCached() equivalent for callers that did not get() a StoreEntry. Callers with StoreEntry objects must use evictCached() instead.
Implements Store::Storage.
Definition at line 1200 of file UFSSwapDir.cc.
◆ FilenoBelongsHere()
|
static |
check whether swapfile belongs to the specified cachedir/l1dir/l2dir
- Parameters
-
cachedir the number of the cachedir which is being tested level1dir level-1 dir in the cachedir level2dir level-2 dir
Definition at line 1114 of file UFSSwapDir.cc.
References assert, SquidConfig::cacheSwap, Config, F1, F2, INDEXSD, IsUFSDir(), l1, l2, and Store::DiskConfig::n_configured.
Referenced by DirClean(), and Fs::Ufs::RebuildState::getNextFile().
◆ finalizeSwapoutFailure()
|
overridevirtual |
◆ finalizeSwapoutSuccess()
|
overridevirtual |
Implements Store::Disk.
Definition at line 1268 of file UFSSwapDir.cc.
References n_disk_objects, and StoreEntry::swap_file_sz.
◆ fullPath()
char * Fs::Ufs::UFSSwapDir::fullPath | ( | sfileno | filn, |
char * | fullpath | ||
) | const |
Definition at line 1235 of file UFSSwapDir.cc.
References LOCAL_ARRAY, and MAXPATHLEN.
◆ get()
|
overridevirtualinherited |
- Returns
- a possibly unlocked/unregistered stored entry with key (or nil) The returned entry might not match the caller's Store ID or method. The caller must abandon()/release() the entry or register it with Root(). This method must not trigger slow I/O operations (e.g., disk swap in).
Implements Store::Controlled.
◆ getOptionTree()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 250 of file UFSSwapDir.cc.
References Store::Disk::getOptionTree(), optionIODump(), and optionIOParse().
◆ getStats()
|
overridevirtualinherited |
Implements Store::Storage.
Definition at line 52 of file Disk.cc.
References StoreInfoStats::Part::capacity, StoreInfoStats::Part::count, StoreInfoStats::Part::size, and StoreInfoStats::swap.
◆ HandleCleanEvent()
|
staticprivate |
Definition at line 1039 of file UFSSwapDir.cc.
References assert, SquidConfig::cacheSwap, Config, INDEXSD, IsUFSDir(), l1, l2, Store::DiskConfig::n_configured, RandomSeed32(), Store::Controller::store_dirs_rebuilding, and xcalloc().
◆ hasReadableEntry()
|
inlineoverridevirtual |
as long as ufs relies on the global store_table to index entries, it is wrong to ask individual ufs cache_dirs whether they have an entry
Implements Store::Disk.
Definition at line 78 of file UFSSwapDir.h.
◆ init()
|
overridevirtual |
Start preparing the store for use. To check readiness, callers should use readable() and writable() methods.
Implements Store::Storage.
Definition at line 272 of file UFSSwapDir.cc.
References debugs, eventAdd(), fatal(), and fsBlockSize().
◆ IsUFSDir()
|
static |
Definition at line 1102 of file UFSSwapDir.cc.
Referenced by FilenoBelongsHere(), and HandleCleanEvent().
◆ logEntry()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 1283 of file UFSSwapDir.cc.
References debugs, StoreSwapLogData::expires, StoreEntry::expires, file_write(), StoreSwapLogData::finalize(), StoreSwapLogData::flags, StoreEntry::flags, FreeObject(), hash_link::key, StoreSwapLogData::key, StoreSwapLogData::lastmod, StoreEntry::lastModified(), StoreSwapLogData::lastref, StoreEntry::lastref, StoreSwapLogData::op, StoreSwapLogData::refcount, StoreEntry::refcount, SQUID_MD5_DIGEST_LENGTH, StoreSwapLogData::swap_file_sz, StoreEntry::swap_file_sz, StoreSwapLogData::swap_filen, StoreEntry::swap_filen, StoreSwapLogData::timestamp, and StoreEntry::timestamp.
◆ logFile()
|
private |
Definition at line 689 of file UFSSwapDir.cc.
References SBuf::append(), SBuf::appendf(), SBuf::cmp(), Config, SquidConfig::Log, MAXPATHLEN, SquidConfig::swap, and xstrncpy().
◆ maintain()
|
overridevirtual |
Implements Store::Storage.
Definition at line 414 of file UFSSwapDir.cc.
References Config, DBG_IMPORTANT, debugs, RemovalPurgeWalker::Done, double, SquidConfig::highWaterMark, int, RemovalPurgeWalker::Next, StoreEntry::release(), squid_curtime, Store::Controller::store_dirs_rebuilding, and SquidConfig::Swap.
◆ mapBitAllocate()
int Fs::Ufs::UFSSwapDir::mapBitAllocate | ( | ) |
Definition at line 585 of file UFSSwapDir.cc.
◆ mapBitReset()
void Fs::Ufs::UFSSwapDir::mapBitReset | ( | sfileno | filn | ) |
Definition at line 570 of file UFSSwapDir.cc.
◆ mapBitSet()
void Fs::Ufs::UFSSwapDir::mapBitSet | ( | sfileno | filn | ) |
Definition at line 564 of file UFSSwapDir.cc.
◆ mapBitTest()
Definition at line 558 of file UFSSwapDir.cc.
Referenced by DirClean().
◆ maxObjectSize() [1/2]
|
overridevirtualinherited |
Implements Store::Storage.
Definition at line 103 of file Disk.cc.
References Config, SquidConfig::maxObjectSize, min(), and SquidConfig::Store.
Referenced by Fs::Ufs::UFSStoreState::write().
◆ maxObjectSize() [2/2]
|
inherited |
configure the maximum object size for this storage area. May be any size up to the total storage area.
Definition at line 115 of file Disk.cc.
References DBG_PARSE_NOTE, and debugs.
◆ maxSize()
|
inlineoverridevirtualinherited |
The maximum size the store will support in normal use. Inaccuracy is permitted, but may throw estimates for memory etc out of whack.
Implements Store::Storage.
Reimplemented in TestSwapDir.
Definition at line 48 of file Disk.h.
References Store::Disk::max_size.
◆ minObjectSize()
|
inherited |
Definition at line 96 of file Disk.cc.
References Config, SquidConfig::minObjectSize, and SquidConfig::Store.
◆ minSize()
|
overridevirtualinherited |
Implements Store::Storage.
Definition at line 89 of file Disk.cc.
References Config, SquidConfig::lowWaterMark, and SquidConfig::Swap.
◆ needsDiskStrand()
|
virtualinherited |
Reimplemented in Rock::SwapDir.
◆ objectSizeIsAcceptable()
|
inherited |
◆ openLog()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 728 of file UFSSwapDir.cc.
References assert, SBuf::c_str(), SquidConfig::cacheSwap, Config, DBG_IMPORTANT, debugs, fatal(), file_open(), IamWorkerProcess(), Store::DiskConfig::n_configured, O_BINARY, and xstrerr().
◆ openStoreIO()
|
overridevirtual |
Implements Store::Disk.
Definition at line 552 of file UFSSwapDir.cc.
◆ openTmpSwapLog()
Definition at line 858 of file UFSSwapDir.cc.
References MemBuf::append(), MemBuf::appended(), assert, SBuf::c_str(), MemBuf::content(), MemBuf::contentSize(), DBG_CRITICAL, DBG_IMPORTANT, debugs, fatalf(), file_close(), file_open(), file_write(), MemBuf::freeFunc(), StoreSwapLogHeader::gapSize(), MemBuf::init(), O_BINARY, StoreSwapLogHeader::record_size, safeunlink(), MemBuf::space(), SQUIDSBUFPH, SQUIDSBUFPRINT, and xstrerr().
◆ optionIODump()
|
private |
Definition at line 244 of file UFSSwapDir.cc.
References storeAppendPrintf().
Referenced by getOptionTree().
◆ optionIOParse()
|
private |
Definition at line 217 of file UFSSwapDir.cc.
References DiskIOModule::Find(), and self_destruct().
Referenced by getOptionTree().
◆ optionObjectSizeDump()
|
privateinherited |
Definition at line 382 of file Disk.cc.
References PRId64, and storeAppendPrintf().
Referenced by Store::Disk::getOptionTree().
◆ optionObjectSizeParse()
|
privateinherited |
Definition at line 347 of file Disk.cc.
References DBG_IMPORTANT, debugs, self_destruct(), size, and strtoll().
Referenced by Store::Disk::getOptionTree().
◆ optionReadOnlyDump()
|
privateinherited |
Definition at line 340 of file Disk.cc.
References storeAppendPrintf().
Referenced by Store::Disk::getOptionTree().
◆ optionReadOnlyParse()
|
privateinherited |
Definition at line 318 of file Disk.cc.
References DBG_PARSE_NOTE, debugs, and xatoi().
Referenced by Store::Disk::getOptionTree().
◆ parse()
|
overridevirtual |
Implements Store::Disk.
Definition at line 180 of file UFSSwapDir.cc.
References Config, createRemovalPolicy(), SquidConfig::replPolicy, and xstrdup.
Referenced by TestUfs::testUfsDefaultEngine(), and TestUfs::testUfsSearch().
◆ parseOptions()
|
protectedinherited |
Definition at line 267 of file Disk.cc.
References DBG_IMPORTANT, debugs, ConfigParser::NextToken(), ConfigOption::parse(), and self_destruct().
◆ parseSizeL1L2()
|
private |
Definition at line 143 of file UFSSwapDir.cc.
References DBG_IMPORTANT, debugs, fatal(), GetInteger(), reconfiguring, and size.
◆ pathIsDirectory()
|
private |
Definition at line 629 of file UFSSwapDir.cc.
References DBG_CRITICAL, debugs, and xstrerr().
◆ rebuild()
|
private |
Definition at line 825 of file UFSSwapDir.cc.
References eventAdd(), and Fs::Ufs::RebuildState::RebuildStep.
◆ reconfigure()
|
overridevirtual |
Implements Store::Disk.
Definition at line 173 of file UFSSwapDir.cc.
◆ reference()
|
overridevirtual |
Implements Store::Controlled.
Definition at line 524 of file UFSSwapDir.cc.
References debugs, StoreEntry::repl, StoreEntry::swap_dirn, and StoreEntry::swap_filen.
◆ replacementAdd()
void Fs::Ufs::UFSSwapDir::replacementAdd | ( | StoreEntry * | e | ) |
Add and remove the given StoreEntry from the replacement policy in use
Definition at line 1207 of file UFSSwapDir.cc.
References debugs, and StoreEntry::repl.
◆ replacementRemove()
void Fs::Ufs::UFSSwapDir::replacementRemove | ( | StoreEntry * | e | ) |
Definition at line 1214 of file UFSSwapDir.cc.
References assert, debugs, RefCount< C >::getRaw(), StoreEntry::hasDisk(), INDEXSD, StoreEntry::repl, and StoreEntry::swap_dirn.
◆ sizeInBlocks()
|
inlineprotectedinherited |
Definition at line 87 of file Disk.h.
References Store::Disk::fs, and size.
◆ smpAware()
|
inlineoverridevirtual |
Implements Store::Disk.
Definition at line 75 of file UFSSwapDir.h.
◆ stat()
|
overridevirtualinherited |
Output stats to the provided store entry. TODO: make these calls asynchronous
Implements Store::Storage.
Reimplemented in TestSwapDir.
Definition at line 63 of file Disk.cc.
References storeAppendPrintf().
◆ statfs()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 371 of file UFSSwapDir.cc.
References Math::doublePercent(), fsStats(), Math::intPercent(), PRIu64, and storeAppendPrintf().
◆ swapSubDir()
char * Fs::Ufs::UFSSwapDir::swapSubDir | ( | int | subdirn | ) | const |
Definition at line 595 of file UFSSwapDir.cc.
References assert, LOCAL_ARRAY, and MAXPATHLEN.
◆ sync()
|
overridevirtual |
Reimplemented from Store::Storage.
Definition at line 1262 of file UFSSwapDir.cc.
◆ type()
◆ unlinkdUseful()
|
overridevirtual |
Implements Store::Disk.
Definition at line 1171 of file UFSSwapDir.cc.
References IamWorkerProcess().
◆ unlinkFile()
void Fs::Ufs::UFSSwapDir::unlinkFile | ( | sfileno | f | ) |
Definition at line 1162 of file UFSSwapDir.cc.
References asHex(), and debugs.
Referenced by evictCached().
◆ updateAnchored()
|
inlinevirtualinherited |
Update a local Transients entry with fresh info from this cache (if any). Return true iff the cache supports Transients entries and the given local Transients entry is now in sync with this storage.
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 44 of file Controlled.h.
Referenced by Store::Disks::updateAnchored().
◆ updateHeaders()
|
inlinevirtualinherited |
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 35 of file Controlled.h.
Referenced by Store::Disks::updateHeaders().
◆ validFileno()
Definition at line 1145 of file UFSSwapDir.cc.
Referenced by DirClean().
◆ validL1()
bool Fs::Ufs::UFSSwapDir::validL1 | ( | int | anInt | ) | const |
Definition at line 776 of file UFSSwapDir.cc.
◆ validL2()
bool Fs::Ufs::UFSSwapDir::validL2 | ( | int | anInt | ) | const |
Definition at line 782 of file UFSSwapDir.cc.
◆ verifyCacheDirs()
|
private |
Verify that the the CacheDir exists
If this returns < 0, then Squid exits, complains about swap directories not existing, and instructs the admin to run 'squid -z' Called by UFSSwapDir::init
Definition at line 649 of file UFSSwapDir.cc.
◆ writeCleanDone()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 976 of file UFSSwapDir.cc.
References SBuf::c_str(), UFSCleanLog::cln, UFSCleanLog::cur, DBG_CRITICAL, debugs, RemovalPolicyWalker::Done, UFSCleanLog::fd, FD_WRITE_METHOD(), file_close(), file_open(), FileRename(), MYNAME, UFSCleanLog::newLog, O_BINARY, UFSCleanLog::outbuf, UFSCleanLog::outbuf_offset, safe_free, Store::Controller::store_dirs_rebuilding, UFSCleanLog::walker, and xstrerr().
◆ writeCleanStart()
|
overridevirtual |
Reimplemented from Store::Disk.
Definition at line 932 of file UFSSwapDir.cc.
References SBuf::append(), SBuf::c_str(), CLEAN_BUF_SZ, UFSCleanLog::cln, UFSCleanLog::cur, debugs, UFSCleanLog::fd, file_open(), StoreSwapLogHeader::gapSize(), UFSCleanLog::newLog, O_BINARY, UFSCleanLog::outbuf, UFSCleanLog::outbuf_offset, StoreSwapLogHeader::record_size, UFSCleanLog::walker, and xcalloc().
Member Data Documentation
◆ blksize
◆ CleanEvent
|
staticprivate |
Definition at line 130 of file UFSSwapDir.h.
◆ cleanLog
|
inherited |
Definition at line 140 of file Disk.h.
Referenced by UFSCleanLog::write().
◆ cur_size
|
private |
Definition at line 149 of file UFSSwapDir.h.
Referenced by currentSize().
◆ currentIOOptions
|
mutableprivate |
Definition at line 147 of file UFSSwapDir.h.
◆ disker
◆ flags
|
inherited |
Referenced by storeDirSelectSwapDirLeastLoad().
◆ fs
struct { ... } Store::Disk::fs |
Referenced by Store::Disk::Disk(), and Store::Disk::sizeInBlocks().
◆ fsdata
void* Fs::Ufs::UFSSwapDir::fsdata |
Definition at line 109 of file UFSSwapDir.h.
◆ index
|
inherited |
Definition at line 103 of file Disk.h.
Referenced by Rock::Rebuild::Start(), and Fs::Ufs::UFSStoreState::UFSStoreState().
◆ IO
Fs::Ufs::UFSStrategy* Fs::Ufs::UFSSwapDir::IO |
Definition at line 83 of file UFSSwapDir.h.
Referenced by canStore(), TestUfs::testUfsDefaultEngine(), TestUfs::testUfsSearch(), and UFSSwapDir().
◆ ioType
|
private |
Definition at line 148 of file UFSSwapDir.h.
◆ l1
|
protected |
Definition at line 121 of file UFSSwapDir.h.
Referenced by DirClean(), FilenoBelongsHere(), and HandleCleanEvent().
◆ l2
|
protected |
Definition at line 122 of file UFSSwapDir.h.
Referenced by DirClean(), FilenoBelongsHere(), and HandleCleanEvent().
◆ map
|
protected |
Definition at line 119 of file UFSSwapDir.h.
◆ max_objsize
◆ max_size
|
protectedinherited |
Definition at line 97 of file Disk.h.
Referenced by Store::Disk::maxSize().
◆ min_objsize
◆ n_disk_objects
|
private |
Definition at line 150 of file UFSSwapDir.h.
Referenced by currentCount().
◆ NumberOfUFSDirs
|
staticprivate |
Definition at line 126 of file UFSSwapDir.h.
◆ path
|
inherited |
Definition at line 102 of file Disk.h.
Referenced by DirClean(), Rock::Rebuild::Stats::Init(), and Rock::Rebuild::Start().
◆ rebuilding_
|
private |
Definition at line 151 of file UFSSwapDir.h.
◆ removals
◆ repl
|
inherited |
◆ scanned
◆ suggest
|
protected |
Definition at line 120 of file UFSSwapDir.h.
◆ swaplog_fd
|
private |
Definition at line 129 of file UFSSwapDir.h.
◆ theType
◆ UFSDirToGlobalDirMapping
|
staticprivate |
Definition at line 127 of file UFSSwapDir.h.
The documentation for this class was generated from the following files:
- src/fs/ufs/UFSSwapDir.h
- src/fs/ufs/UFSSwapDir.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products