manages a single cache_dir More...
#include <Disk.h>
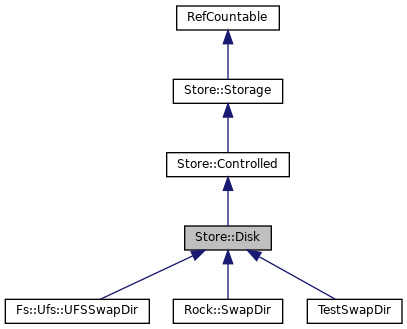
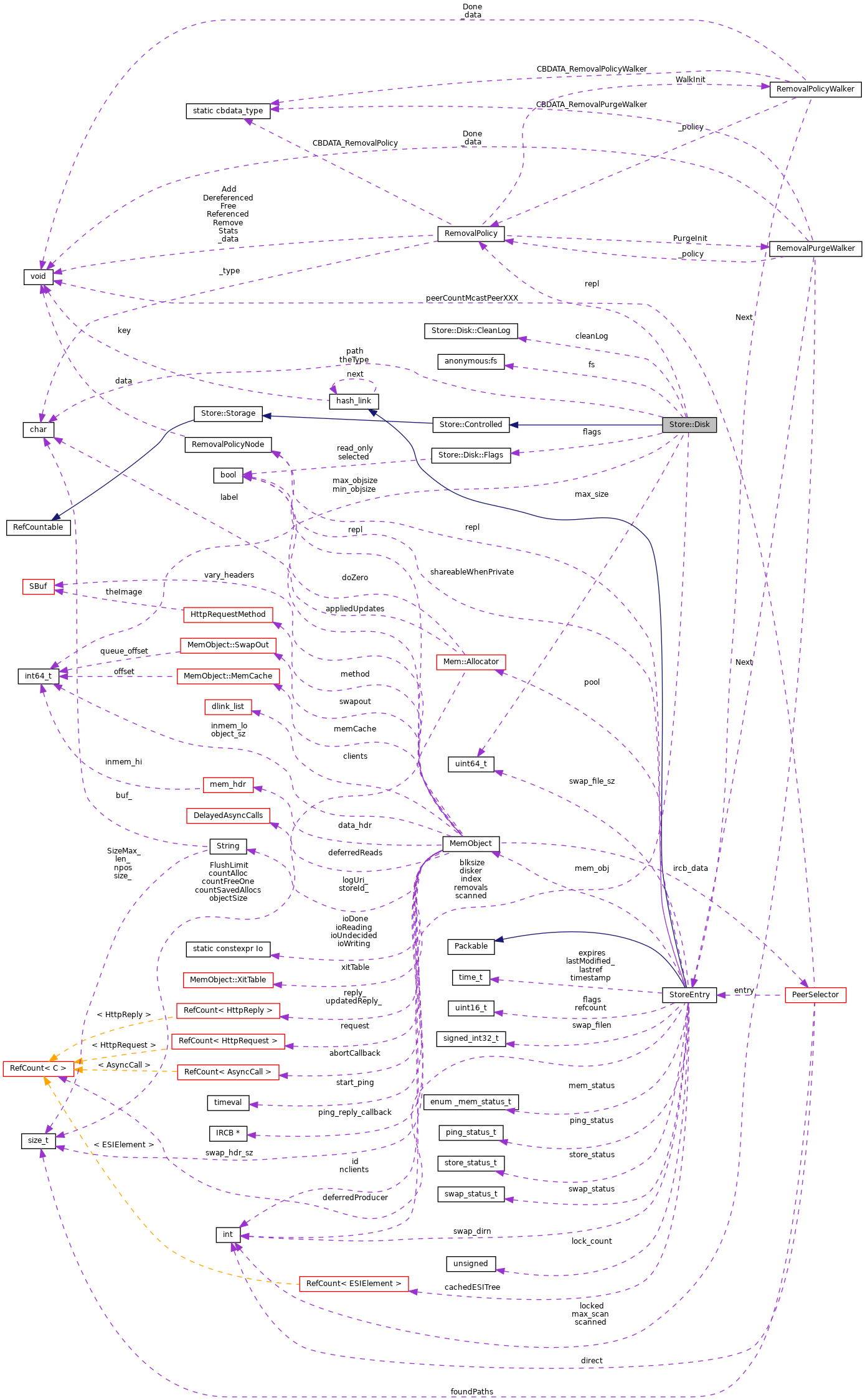
Classes | |
class | CleanLog |
struct | Flags |
Public Types | |
typedef RefCount< Disk > | Pointer |
Public Member Functions | |
Disk (char const *aType) | |
~Disk () override | |
virtual void | reconfigure ()=0 |
const char * | type () const |
virtual bool | needsDiskStrand () const |
needs a dedicated kid process More... | |
virtual bool | active () const |
virtual bool | doReportStat () const |
whether stat should be reported by this SwapDir More... | |
virtual bool | unlinkdUseful () const =0 |
whether SwapDir may benefit from unlinkd More... | |
virtual void | diskFull () |
void | create () override |
create system resources needed for this store to operate in the future More... | |
StoreEntry * | get (const cache_key *) override |
uint64_t | maxSize () const override |
uint64_t | minSize () const override |
the minimum size the store will shrink to via normal housekeeping More... | |
int64_t | maxObjectSize () const override |
the maximum size of a storable object; -1 if unlimited More... | |
void | getStats (StoreInfoStats &stats) const override |
collect statistics More... | |
void | stat (StoreEntry &) const override |
void | reference (StoreEntry &e) override |
somebody needs this entry (many cache replacement policies need to know) More... | |
bool | dereference (StoreEntry &e) override |
void | maintain () override |
perform regular periodic maintenance; TODO: move to UFSSwapDir::Maintain More... | |
virtual bool | smpAware () const =0 |
whether this disk storage is capable of serving multiple workers More... | |
int64_t | minObjectSize () const |
the size of the smallest entry this cache_dir can store More... | |
void | maxObjectSize (int64_t newMax) |
bool | objectSizeIsAcceptable (int64_t objSize) const |
virtual void | disconnect (StoreEntry &) |
called when the entry is about to forget its association with cache_dir More... | |
virtual void | finalizeSwapoutSuccess (const StoreEntry &)=0 |
finalize the successful swapout that has been already noticed by Store More... | |
virtual void | finalizeSwapoutFailure (StoreEntry &)=0 |
abort the failed swapout that has been already noticed by Store More... | |
virtual bool | hasReadableEntry (const StoreEntry &e) const =0 |
whether this cache dir has an entry with e.key More... | |
virtual void | dump (StoreEntry &) const |
virtual bool | doubleCheck (StoreEntry &) |
virtual void | statfs (StoreEntry &) const |
virtual bool | canStore (const StoreEntry &e, int64_t diskSpaceNeeded, int &load) const =0 |
check whether we can store the entry; if we can, report current load More... | |
virtual StoreIOState::Pointer | createStoreIO (StoreEntry &, StoreIOState::STIOCB *, void *)=0 |
virtual StoreIOState::Pointer | openStoreIO (StoreEntry &, StoreIOState::STIOCB *, void *)=0 |
bool | canLog (StoreEntry const &e) const |
virtual void | openLog () |
virtual void | closeLog () |
virtual void | logEntry (const StoreEntry &e, int op) const |
virtual int | writeCleanStart () |
virtual void | writeCleanDone () |
virtual void | parse (int index, char *path)=0 |
virtual void | updateHeaders (StoreEntry *) |
make stored metadata and HTTP headers the same as in the given entry More... | |
virtual bool | anchorToCache (StoreEntry &) |
virtual bool | updateAnchored (StoreEntry &) |
virtual void | init ()=0 |
virtual uint64_t | currentSize () const =0 |
current size More... | |
virtual uint64_t | currentCount () const =0 |
the total number of objects stored right now More... | |
virtual void | evictCached (StoreEntry &e)=0 |
virtual void | evictIfFound (const cache_key *)=0 |
virtual int | callback () |
called once every main loop iteration; TODO: Move to UFS code. More... | |
virtual void | sync () |
prepare for shutdown More... | |
Public Attributes | |
char * | path |
int | index |
int | disker |
disker kid id dedicated to this SwapDir or -1 More... | |
RemovalPolicy * | repl |
int | removals |
int | scanned |
struct Store::Disk::Flags | flags |
CleanLog * | cleanLog |
struct { | |
int blksize | |
} | fs |
Protected Member Functions | |
void | parseOptions (int reconfiguring) |
void | dumpOptions (StoreEntry *e) const |
virtual ConfigOption * | getOptionTree () const |
virtual bool | allowOptionReconfigure (const char *const) const |
int64_t | sizeInBlocks (const int64_t size) const |
Protected Attributes | |
uint64_t | max_size |
maximum allocatable size of the storage area More... | |
int64_t | min_objsize |
minimum size of any object stored here (-1 for no limit) More... | |
int64_t | max_objsize |
maximum size of any object stored here (-1 for no limit) More... | |
Private Member Functions | |
bool | optionReadOnlyParse (char const *option, const char *value, int reconfiguring) |
void | optionReadOnlyDump (StoreEntry *e) const |
bool | optionObjectSizeParse (char const *option, const char *value, int reconfiguring) |
void | optionObjectSizeDump (StoreEntry *e) const |
Private Attributes | |
const char * | theType |
Detailed Description
Member Typedef Documentation
◆ Pointer
typedef RefCount<Disk> Store::Disk::Pointer |
Constructor & Destructor Documentation
◆ Disk()
|
explicit |
◆ ~Disk()
Member Function Documentation
◆ active()
|
virtual |
may be used in this strand
Definition at line 236 of file Disk.cc.
References IamWorkerProcess(), and KidIdentifier.
Referenced by Store::Disks::anchorToCache().
◆ allowOptionReconfigure()
|
inlineprotectedvirtual |
Reimplemented in Rock::SwapDir.
Definition at line 85 of file Disk.h.
Referenced by Rock::SwapDir::allowOptionReconfigure().
◆ anchorToCache()
|
inlinevirtualinherited |
tie StoreEntry to this storage if this storage has a matching entry
- Return values
-
true if this storage has a matching entry
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 39 of file Controlled.h.
Referenced by Store::Disks::anchorToCache().
◆ callback()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Disks, and Store::Controller.
◆ canLog()
bool Store::Disk::canLog | ( | StoreEntry const & | e | ) | const |
Definition at line 188 of file Disk.cc.
References EBIT_TEST, ENTRY_SPECIAL, StoreEntry::flags, StoreEntry::hasDisk(), KEY_PRIVATE, RELEASE_REQUEST, StoreEntry::swap_file_sz, and StoreEntry::swappedOut().
◆ canStore()
|
pure virtual |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, and TestSwapDir.
Definition at line 164 of file Disk.cc.
References debugs, EBIT_TEST, ENTRY_SPECIAL, and StoreEntry::flags.
Referenced by Fs::Ufs::UFSSwapDir::canStore().
◆ closeLog()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Definition at line 215 of file Disk.cc.
Referenced by storeDirCloseSwapLogs().
◆ create()
|
overridevirtual |
Implements Store::Storage.
◆ createStoreIO()
|
pure virtual |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, and TestSwapDir.
◆ currentCount()
|
pure virtualinherited |
Implemented in Fs::Ufs::UFSSwapDir, Transients, MemStore, Rock::SwapDir, Store::Controller, Store::Disks, and TestSwapDir.
◆ currentSize()
|
pure virtualinherited |
Implemented in Fs::Ufs::UFSSwapDir, Transients, MemStore, Rock::SwapDir, Store::Controller, Store::Disks, and TestSwapDir.
◆ dereference()
|
overridevirtual |
somebody no longer needs this entry (usually after calling reference()) return false iff the idle entry should be destroyed
Implements Store::Controlled.
Definition at line 139 of file Disk.cc.
Referenced by Store::Disks::dereference().
◆ disconnect()
|
inlinevirtual |
Reimplemented in Rock::SwapDir.
Definition at line 71 of file Disk.h.
Referenced by destroyStoreEntry().
◆ diskFull()
|
virtual |
Notify this disk that it is full. XXX move into a protected api call between store files and their stores, rather than a top level api call
Reimplemented in Rock::SwapDir.
Definition at line 145 of file Disk.cc.
References DBG_IMPORTANT, and debugs.
Referenced by storeSwapOutFileClosed().
◆ doReportStat()
|
inlinevirtual |
Reimplemented in Rock::SwapDir.
◆ doubleCheck()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Definition at line 46 of file Disk.cc.
Referenced by storeCleanup().
◆ dump()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
◆ dumpOptions()
|
protected |
Definition at line 307 of file Disk.cc.
References ConfigOption::dump().
◆ evictCached()
|
pure virtualinherited |
Prevent new get() calls from returning the matching entry. If the matching entry is unused, it may be removed from the store now. The store entry is matched using either e
attachment info or e.key
.
Implemented in Transients, MemStore, Fs::Ufs::UFSSwapDir, Rock::SwapDir, Store::Disks, Store::Controller, and TestSwapDir.
Referenced by Store::Disks::evictCached().
◆ evictIfFound()
|
pure virtualinherited |
An evictCached() equivalent for callers that did not get() a StoreEntry. Callers with StoreEntry objects must use evictCached() instead.
Implemented in Transients, MemStore, Fs::Ufs::UFSSwapDir, Rock::SwapDir, Store::Disks, Store::Controller, and TestSwapDir.
◆ finalizeSwapoutFailure()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, and TestSwapDir.
Referenced by storeSwapOutFileClosed().
◆ finalizeSwapoutSuccess()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, and TestSwapDir.
Referenced by storeSwapOutFileClosed().
◆ get()
|
overridevirtual |
- Returns
- a possibly unlocked/unregistered stored entry with key (or nil) The returned entry might not match the caller's Store ID or method. The caller must abandon()/release() the entry or register it with Root(). This method must not trigger slow I/O operations (e.g., disk swap in).
Implements Store::Controlled.
◆ getOptionTree()
|
protectedvirtual |
Reimplemented in Rock::SwapDir, and Fs::Ufs::UFSSwapDir.
Definition at line 258 of file Disk.cc.
References optionObjectSizeDump(), optionObjectSizeParse(), optionReadOnlyDump(), optionReadOnlyParse(), and ConfigOptionVector::options.
Referenced by Fs::Ufs::UFSSwapDir::getOptionTree(), and Rock::SwapDir::getOptionTree().
◆ getStats()
|
overridevirtual |
Implements Store::Storage.
Definition at line 52 of file Disk.cc.
References StoreInfoStats::Part::capacity, StoreInfoStats::Part::count, StoreInfoStats::Part::size, and StoreInfoStats::swap.
◆ hasReadableEntry()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, and TestSwapDir.
◆ init()
|
pure virtualinherited |
Start preparing the store for use. To check readiness, callers should use readable() and writable() methods.
Implemented in Rock::SwapDir, Transients, MemStore, Fs::Ufs::UFSSwapDir, TestSwapDir, Store::Controller, and Store::Disks.
◆ logEntry()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Definition at line 227 of file Disk.cc.
Referenced by storeDirSwapLog().
◆ maintain()
|
overridevirtual |
Implements Store::Storage.
◆ maxObjectSize() [1/2]
|
overridevirtual |
Implements Store::Storage.
Definition at line 103 of file Disk.cc.
References Config, SquidConfig::maxObjectSize, min(), and SquidConfig::Store.
Referenced by Fs::Ufs::UFSStoreState::write().
◆ maxObjectSize() [2/2]
void Store::Disk::maxObjectSize | ( | int64_t | newMax | ) |
configure the maximum object size for this storage area. May be any size up to the total storage area.
Definition at line 115 of file Disk.cc.
References DBG_PARSE_NOTE, and debugs.
◆ maxSize()
|
inlineoverridevirtual |
The maximum size the store will support in normal use. Inaccuracy is permitted, but may throw estimates for memory etc out of whack.
Implements Store::Storage.
Reimplemented in TestSwapDir.
Definition at line 48 of file Disk.h.
References max_size.
◆ minObjectSize()
int64_t Store::Disk::minObjectSize | ( | ) | const |
Definition at line 96 of file Disk.cc.
References Config, SquidConfig::minObjectSize, and SquidConfig::Store.
◆ minSize()
|
overridevirtual |
Implements Store::Storage.
Definition at line 89 of file Disk.cc.
References Config, SquidConfig::lowWaterMark, and SquidConfig::Swap.
◆ needsDiskStrand()
|
virtual |
Reimplemented in Rock::SwapDir.
◆ objectSizeIsAcceptable()
bool Store::Disk::objectSizeIsAcceptable | ( | int64_t | objSize | ) | const |
◆ openLog()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Definition at line 212 of file Disk.cc.
Referenced by storeDirOpenSwapLogs().
◆ openStoreIO()
|
pure virtual |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, and TestSwapDir.
Referenced by storeOpen().
◆ optionObjectSizeDump()
|
private |
Definition at line 382 of file Disk.cc.
References PRId64, and storeAppendPrintf().
Referenced by getOptionTree().
◆ optionObjectSizeParse()
|
private |
Definition at line 347 of file Disk.cc.
References DBG_IMPORTANT, debugs, self_destruct(), size, and strtoll().
Referenced by getOptionTree().
◆ optionReadOnlyDump()
|
private |
Definition at line 340 of file Disk.cc.
References storeAppendPrintf().
Referenced by getOptionTree().
◆ optionReadOnlyParse()
|
private |
Definition at line 318 of file Disk.cc.
References DBG_PARSE_NOTE, debugs, and xatoi().
Referenced by getOptionTree().
◆ parse()
|
pure virtual |
Implemented in TestSwapDir, Fs::Ufs::UFSSwapDir, and Rock::SwapDir.
◆ parseOptions()
|
protected |
Definition at line 267 of file Disk.cc.
References DBG_IMPORTANT, debugs, ConfigParser::NextToken(), ConfigOption::parse(), and self_destruct().
◆ reconfigure()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, and TestSwapDir.
◆ reference()
|
overridevirtual |
Implements Store::Controlled.
Definition at line 136 of file Disk.cc.
Referenced by Store::Disks::reference().
◆ sizeInBlocks()
|
inlineprotected |
◆ smpAware()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, and TestSwapDir.
◆ stat()
|
overridevirtual |
Output stats to the provided store entry. TODO: make these calls asynchronous
Implements Store::Storage.
Reimplemented in TestSwapDir.
Definition at line 63 of file Disk.cc.
References storeAppendPrintf().
◆ statfs()
|
virtual |
Reimplemented in Rock::SwapDir, and Fs::Ufs::UFSSwapDir.
◆ sync()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Controller, and Store::Disks.
◆ type()
◆ unlinkdUseful()
|
pure virtual |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, and TestSwapDir.
Referenced by unlinkdNeeded().
◆ updateAnchored()
|
inlinevirtualinherited |
Update a local Transients entry with fresh info from this cache (if any). Return true iff the cache supports Transients entries and the given local Transients entry is now in sync with this storage.
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 44 of file Controlled.h.
Referenced by Store::Disks::updateAnchored().
◆ updateHeaders()
|
inlinevirtualinherited |
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 35 of file Controlled.h.
Referenced by Store::Disks::updateHeaders().
◆ writeCleanDone()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Definition at line 224 of file Disk.cc.
Referenced by storeDirWriteCleanLogs().
◆ writeCleanStart()
|
virtual |
Reimplemented in Fs::Ufs::UFSSwapDir.
Member Data Documentation
◆ blksize
◆ cleanLog
CleanLog* Store::Disk::cleanLog |
Definition at line 140 of file Disk.h.
Referenced by UFSCleanLog::write().
◆ disker
◆ flags
struct Store::Disk::Flags Store::Disk::flags |
Referenced by storeDirSelectSwapDirLeastLoad().
◆ fs
struct { ... } Store::Disk::fs |
Referenced by Disk(), and sizeInBlocks().
◆ index
int Store::Disk::index |
Definition at line 103 of file Disk.h.
Referenced by Rock::Rebuild::Start(), and Fs::Ufs::UFSStoreState::UFSStoreState().
◆ max_objsize
◆ max_size
|
protected |
◆ min_objsize
◆ path
char* Store::Disk::path |
Definition at line 102 of file Disk.h.
Referenced by Fs::Ufs::UFSSwapDir::DirClean(), Rock::Rebuild::Stats::Init(), and Rock::Rebuild::Start().
◆ removals
◆ repl
RemovalPolicy* Store::Disk::repl |
◆ scanned
◆ theType
The documentation for this class was generated from the following files:
- src/store/Disk.h
- src/store/Disk.cc
- src/tests/stub_libstore.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products