#include <RequestMethod.h>
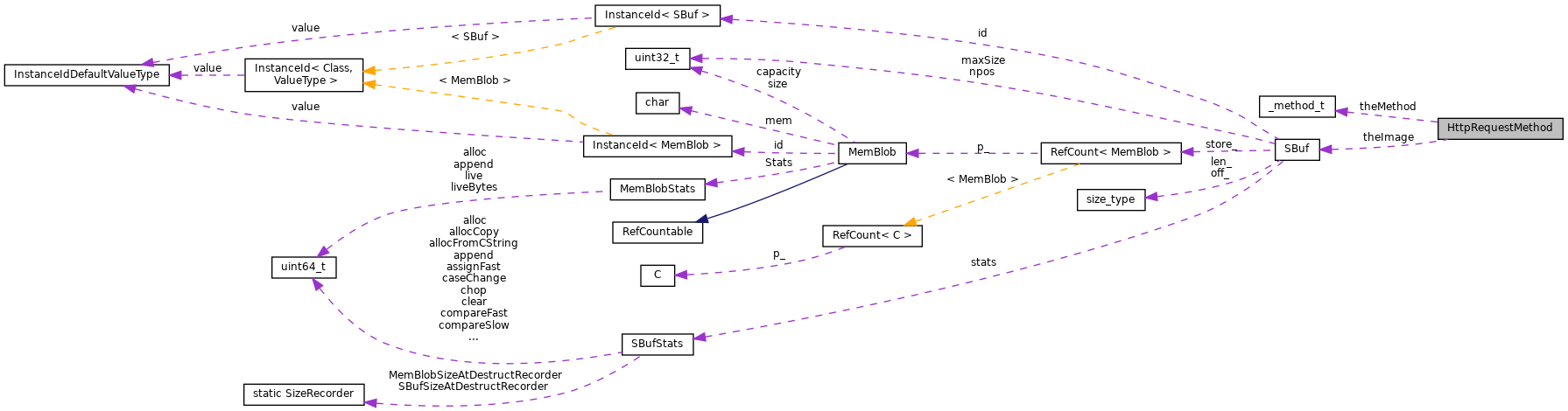
Public Member Functions | |
HttpRequestMethod () | |
HttpRequestMethod (Http::MethodType const aMethod) | |
HttpRequestMethod (const SBuf &) | |
void | HttpRequestMethodXXX (char const *) |
HttpRequestMethod & | operator= (Http::MethodType const aMethod) |
operator bool () const | |
whether the method is set/known More... | |
bool | operator== (Http::MethodType const &aMethod) const |
bool | operator== (HttpRequestMethod const &aMethod) const |
bool | operator!= (Http::MethodType const &aMethod) const |
bool | operator!= (HttpRequestMethod const &aMethod) const |
HttpRequestMethod & | operator++ () |
Http::MethodType | id () const |
const SBuf & | image () const |
bool | isHttpSafe () const |
bool | isIdempotent () const |
bool | respMaybeCacheable () const |
bool | shouldInvalidate () const |
bool | purgesOthers () const |
Private Attributes | |
Http::MethodType | theMethod |
Method type. More... | |
SBuf | theImage |
Used for storing the Http::METHOD_OTHER only. A copy of the parsed method text. More... | |
Detailed Description
This class represents an HTTP Request METHOD
- i.e. PUT, POST, GET etc. It has a runtime extension facility to allow it to efficiently support new methods
Definition at line 26 of file RequestMethod.h.
Constructor & Destructor Documentation
◆ HttpRequestMethod() [1/3]
|
inline |
Definition at line 29 of file RequestMethod.h.
◆ HttpRequestMethod() [2/3]
|
inline |
Definition at line 30 of file RequestMethod.h.
◆ HttpRequestMethod() [3/3]
|
explicit |
Construct a HttpRequestMethod from an SBuf string such as "GET" or from a range of chars such as "FOO" from buffer "GETFOOBARBAZ"
Assumes the s parameter contains only the characters representing the method name
Definition at line 73 of file RequestMethod.cc.
References Config, image(), SBuf::isEmpty(), Http::METHOD_ENUM_END, Http::METHOD_OTHER, SquidConfig::onoff, SquidConfig::relaxed_header_parser, theImage, and theMethod.
Member Function Documentation
◆ HttpRequestMethodXXX()
void HttpRequestMethod::HttpRequestMethodXXX | ( | char const * | begin | ) |
Construct a HttpRequestMethod from a C-string such as "GET" Assumes the string is either nul-terminated or contains whitespace
- Deprecated:
- use SBuf constructor instead
Definition at line 31 of file RequestMethod.cc.
References SBuf::assign(), SBuf::clear(), Config, image(), Http::METHOD_ENUM_END, Http::METHOD_NONE, Http::METHOD_OTHER, SquidConfig::onoff, SquidConfig::relaxed_header_parser, theImage, theMethod, and w_space.
Referenced by htcpUnpackSpecifier(), ACLMethodData::parse(), HttpRequest::parseFirstLine(), HttpRequest::sanityCheckStartLine(), and TestHttpRequestMethod::testConstructCharStart().
◆ id()
|
inline |
Get an ID representation of the method.
- Return values
-
Http::METHOD_NONE the method is unset Http::METHOD_OTHER the method is not recognized and has no unique ID * the method is on of the recognized HTTP methods.
Definition at line 70 of file RequestMethod.h.
References theMethod.
Referenced by HttpReply::bodySize(), HttpRequest::checkEntityFraming(), HttpRequest::effectiveRequestUri(), ClientHttpRequest::handleAdaptedHeader(), CacheManager::ParseHeaders(), purgeEntriesByHeader(), storeKeyPublic(), and storeKeyPublicByRequestMethod().
◆ image()
const SBuf & HttpRequestMethod::image | ( | ) | const |
Get a string representation of the method.
Definition at line 99 of file RequestMethod.cc.
References SBuf::isEmpty(), Http::METHOD_OTHER, Http::MethodType_sb, theImage, and theMethod.
Referenced by Format::Format::assemble(), HttpStateData::buildRequestPrefix(), ErrorState::compileLegacyCode(), Http::One::RequestParser::firstLineSize(), AccessLogEntry::getLogMethod(), htcpClear(), htcpQuery(), HttpRequestMethod(), HttpRequestMethodXXX(), operator<<(), HttpRequest::pack(), HttpRequest::packFirstLineInto(), HttpRequest::prefixLen(), MemObject::stat(), storeLog(), and TestHttpRequestMethod::testConstructCharStart().
◆ isHttpSafe()
bool HttpRequestMethod::isHttpSafe | ( | ) | const |
Whether this method is defined as a "safe" in HTTP/1.1 see RFC 2616 section 9.1.1
Definition at line 114 of file RequestMethod.cc.
References Http::METHOD_GET, Http::METHOD_HEAD, Http::METHOD_OPTIONS, Http::METHOD_PRI, Http::METHOD_PROPFIND, Http::METHOD_REPORT, Http::METHOD_SEARCH, and theMethod.
Referenced by FwdState::checkRetriable().
◆ isIdempotent()
bool HttpRequestMethod::isIdempotent | ( | ) | const |
Whether this method is defined as "idempotent" in HTTP/1.1 see RFC 2616 section 9.1.2
Definition at line 159 of file RequestMethod.cc.
References Http::METHOD_COPY, Http::METHOD_DELETE, Http::METHOD_GET, Http::METHOD_HEAD, Http::METHOD_MKCOL, Http::METHOD_MOVE, Http::METHOD_OPTIONS, Http::METHOD_PRI, Http::METHOD_PROPFIND, Http::METHOD_PROPPATCH, Http::METHOD_PUT, Http::METHOD_TRACE, Http::METHOD_UNLOCK, and theMethod.
Referenced by FwdState::checkRetriable().
◆ operator bool()
|
inlineexplicit |
Definition at line 42 of file RequestMethod.h.
References Http::METHOD_NONE, and theMethod.
◆ operator!=() [1/2]
|
inline |
Definition at line 50 of file RequestMethod.h.
References theMethod.
◆ operator!=() [2/2]
|
inline |
Definition at line 51 of file RequestMethod.h.
References operator==().
◆ operator++()
|
inline |
Iterate through all HTTP method IDs.
Definition at line 56 of file RequestMethod.h.
References assert, Http::METHOD_ENUM_END, and theMethod.
◆ operator=()
|
inline |
Definition at line 35 of file RequestMethod.h.
References SBuf::clear(), theImage, and theMethod.
◆ operator==() [1/2]
|
inline |
◆ operator==() [2/2]
|
inline |
Definition at line 45 of file RequestMethod.h.
References Http::METHOD_OTHER, theImage, and theMethod.
◆ purgesOthers()
bool HttpRequestMethod::purgesOthers | ( | ) | const |
Definition at line 292 of file RequestMethod.cc.
References Http::METHOD_CONNECT, Http::METHOD_COPY, Http::METHOD_GET, Http::METHOD_HEAD, Http::METHOD_LOCK, Http::METHOD_NONE, Http::METHOD_OPTIONS, Http::METHOD_PROPFIND, Http::METHOD_SEARCH, Http::METHOD_TRACE, Http::METHOD_UNLOCK, shouldInvalidate(), and theMethod.
Referenced by Client::maybePurgeOthers().
◆ respMaybeCacheable()
bool HttpRequestMethod::respMaybeCacheable | ( | ) | const |
Whether responses to this method MAY be cached.
- Return values
-
false Not cacheable. true Possibly cacheable. Other details will determine.
Definition at line 208 of file RequestMethod.cc.
References Http::METHOD_BASELINE_CONTROL, Http::METHOD_CHECKIN, Http::METHOD_CHECKOUT, Http::METHOD_GET, Http::METHOD_HEAD, Http::METHOD_LABEL, Http::METHOD_MERGE, Http::METHOD_MKACTIVITY, Http::METHOD_MKWORKSPACE, Http::METHOD_POST, Http::METHOD_PROPFIND, Http::METHOD_UNCHECKOUT, Http::METHOD_UPDATE, Http::METHOD_VERSION_CONTROL, and theMethod.
Referenced by clientHierarchical(), and HttpRequest::maybeCacheable().
◆ shouldInvalidate()
bool HttpRequestMethod::shouldInvalidate | ( | ) | const |
Whether this method SHOULD (or MUST) invalidate existing cached entries. Invalidation is always determined by the response
RFC 2616 defines invalidate as either immediate purge or delayed explicit revalidate all stored copies on next use.
- Return values
-
true SHOULD invalidate. Response details can raise this to a MUST. false Other details will determine. Method is not a factor.
Definition at line 263 of file RequestMethod.cc.
References Http::METHOD_DELETE, Http::METHOD_OTHER, Http::METHOD_POST, Http::METHOD_PURGE, Http::METHOD_PUT, and theMethod.
Referenced by purgesOthers().
Member Data Documentation
◆ theImage
|
private |
Definition at line 112 of file RequestMethod.h.
Referenced by HttpRequestMethod(), HttpRequestMethodXXX(), image(), operator=(), and operator==().
◆ theMethod
|
private |
Definition at line 111 of file RequestMethod.h.
Referenced by HttpRequestMethod(), HttpRequestMethodXXX(), id(), image(), isHttpSafe(), isIdempotent(), operator bool(), operator!=(), operator++(), operator=(), operator==(), purgesOthers(), respMaybeCacheable(), and shouldInvalidate().
The documentation for this class was generated from the following files:
- src/http/RequestMethod.h
- src/http/RequestMethod.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products