#include <BodyPipe.h>
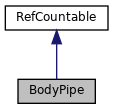
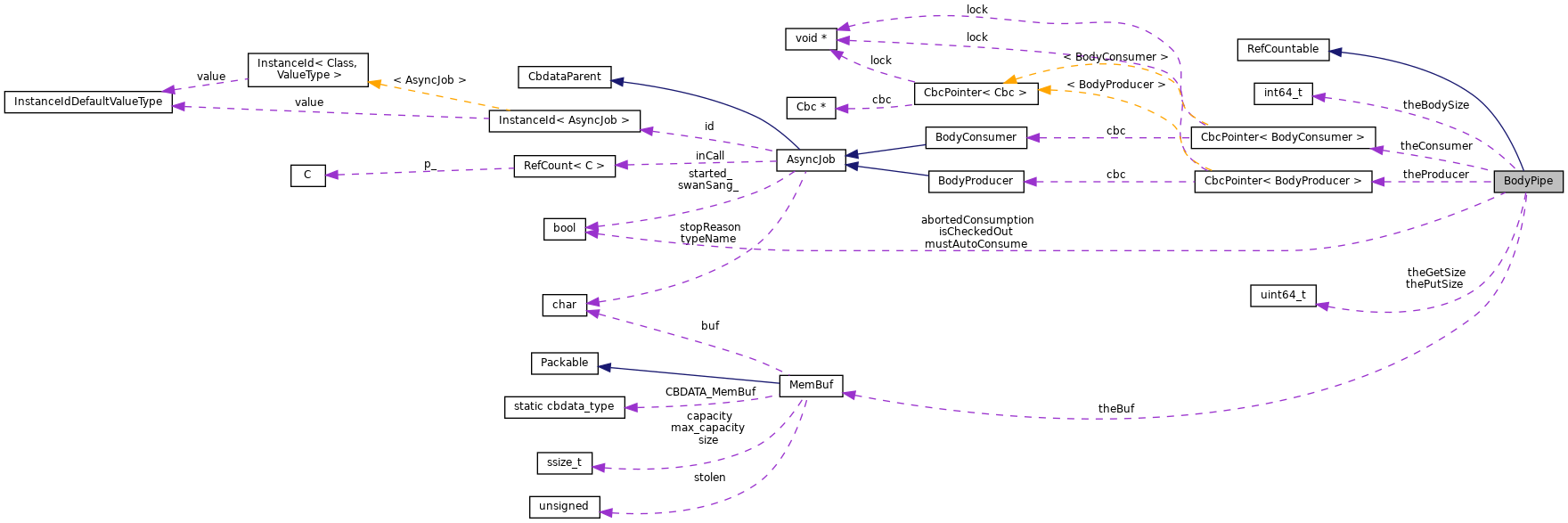
Public Types | |
typedef RefCount< BodyPipe > | Pointer |
typedef BodyProducer | Producer |
typedef BodyConsumer | Consumer |
typedef BodyPipeCheckout | Checkout |
Public Member Functions | |
BodyPipe (Producer *aProducer) | |
~BodyPipe () override | |
void | setBodySize (uint64_t aSize) |
bool | bodySizeKnown () const |
uint64_t | bodySize () const |
uint64_t | consumedSize () const |
uint64_t | producedSize () const |
bool | productionEnded () const |
void | clearProducer (bool atEof) |
size_t | putMoreData (const char *buf, size_t size) |
bool | mayNeedMoreData () const |
bool | needsMoreData () const |
uint64_t | unproducedSize () const |
bool | stillProducing (const Producer::Pointer &producer) const |
void | expectProductionEndAfter (uint64_t extraSize) |
sets or checks body size More... | |
bool | setConsumerIfNotLate (const Consumer::Pointer &aConsumer) |
void | clearConsumer () |
void | expectNoConsumption () |
there will be no more setConsumer() calls More... | |
size_t | getMoreData (MemBuf &buf) |
void | consume (size_t size) |
bool | expectMoreAfter (uint64_t offset) const |
bool | exhausted () const |
bool | stillConsuming (const Consumer::Pointer &consumer) const |
void | enableAutoConsumption () |
start or continue consuming when producing without consumer More... | |
const MemBuf & | buf () const |
const char * | status () const |
Static Public Attributes | |
static constexpr size_t | MaxCapacity = 64*1024 |
Protected Member Functions | |
MemBuf & | checkOut () |
void | checkIn (Checkout &checkout) |
void | undoCheckOut (Checkout &checkout) |
void | scheduleBodyDataNotification () |
void | scheduleBodyEndNotification () |
void | postConsume (size_t size) |
void | postAppend (size_t size) |
void | startAutoConsumptionIfNeeded () |
Private Member Functions | |
MEMPROXY_CLASS (BodyPipe) | |
Private Attributes | |
int64_t | theBodySize |
Producer::Pointer | theProducer |
Consumer::Pointer | theConsumer |
uint64_t | thePutSize |
uint64_t | theGetSize |
MemBuf | theBuf |
bool | mustAutoConsume |
keep theBuf empty when producing without consumer More... | |
bool | abortedConsumption |
called BodyProducer::noteBodyConsumerAborted More... | |
bool | isCheckedOut |
Friends | |
class | BodyPipeCheckout |
Detailed Description
Connects those who produces message body content with those who consume it. For example, connects ConnStateData with FtpStateData OR ICAPModXact with HttpStateData.
Definition at line 90 of file BodyPipe.h.
Member Typedef Documentation
◆ Checkout
typedef BodyPipeCheckout BodyPipe::Checkout |
Definition at line 98 of file BodyPipe.h.
◆ Consumer
typedef BodyConsumer BodyPipe::Consumer |
Definition at line 97 of file BodyPipe.h.
◆ Pointer
typedef RefCount<BodyPipe> BodyPipe::Pointer |
Definition at line 95 of file BodyPipe.h.
◆ Producer
typedef BodyProducer BodyPipe::Producer |
Definition at line 96 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ BodyPipe()
BodyPipe::BodyPipe | ( | Producer * | aProducer | ) |
Definition at line 128 of file BodyPipe.cc.
References debugs, MemBuf::init(), MaxCapacity, status(), and theBuf.
◆ ~BodyPipe()
|
override |
Definition at line 139 of file BodyPipe.cc.
References assert, MemBuf::clean(), debugs, status(), theBuf, theConsumer, and theProducer.
Member Function Documentation
◆ bodySize()
uint64_t BodyPipe::bodySize | ( | ) | const |
Definition at line 161 of file BodyPipe.cc.
References assert, bodySizeKnown(), and theBodySize.
Referenced by clearProducer(), expectProductionEndAfter(), ConnStateData::finishDechunkingRequest(), Adaptation::Icap::ModXact::prepEchoing(), scheduleBodyEndNotification(), and unproducedSize().
◆ bodySizeKnown()
|
inline |
Definition at line 109 of file BodyPipe.h.
References theBodySize.
Referenced by bodySize(), clearProducer(), expectProductionEndAfter(), ConnStateData::finishDechunkingRequest(), mayNeedMoreData(), ConnStateData::mayNeedToReadMoreBody(), needsMoreData(), Adaptation::Icap::ModXact::prepEchoing(), putMoreData(), scheduleBodyEndNotification(), and setBodySize().
◆ buf()
|
inline |
Definition at line 137 of file BodyPipe.h.
References theBuf.
Referenced by Client::calcBufferSpaceToReserve(), Adaptation::Icap::ModXact::estimateVirginBody(), ConnStateData::handleChunkedRequestBody(), Client::handleMoreAdaptedBodyAvailable(), HttpStateData::handleMoreRequestBodyAvailable(), BodySink::noteMoreBodyDataAvailable(), ClientHttpRequest::noteMoreBodyDataAvailable(), HttpStateData::readReply(), Client::replyBodySpace(), Adaptation::Ecap::XactionRep::vbContent(), Adaptation::Ecap::XactionRep::vbContentShift(), and Adaptation::Icap::ModXact::virginConsume().
◆ checkIn()
|
protected |
Definition at line 352 of file BodyPipe.cc.
References assert, BodyPipeCheckout::checkedOutSize, MemBuf::contentSize(), isCheckedOut, postAppend(), postConsume(), and theBuf.
Referenced by BodyPipeCheckout::checkIn(), and BodyPipeCheckout::~BodyPipeCheckout().
◆ checkOut()
|
protected |
Definition at line 344 of file BodyPipe.cc.
References assert, isCheckedOut, and theBuf.
◆ clearConsumer()
void BodyPipe::clearConsumer | ( | ) |
Definition at line 254 of file BodyPipe.cc.
References CbcPointer< Cbc >::clear(), consumedSize(), debugs, expectNoConsumption(), status(), and theConsumer.
Referenced by Adaptation::Message::ShortCircuit(), and BodyConsumer::stopConsumingFrom().
◆ clearProducer()
void BodyPipe::clearProducer | ( | bool | atEof | ) |
Definition at line 194 of file BodyPipe.cc.
References assert, bodySize(), bodySizeKnown(), CbcPointer< Cbc >::clear(), debugs, scheduleBodyEndNotification(), status(), theBodySize, theProducer, and thePutSize.
Referenced by postAppend(), and BodyProducer::stopProducingFor().
◆ consume()
void BodyPipe::consume | ( | size_t | size | ) |
Definition at line 309 of file BodyPipe.cc.
References MemBuf::consume(), postConsume(), size, and theBuf.
Referenced by BodySink::noteMoreBodyDataAvailable(), Adaptation::Ecap::XactionRep::vbContentShift(), and Adaptation::Icap::ModXact::virginConsume().
◆ consumedSize()
|
inline |
Definition at line 111 of file BodyPipe.h.
References theGetSize.
Referenced by HttpRequest::bodyNibbled(), clearConsumer(), ClientHttpRequest::handleAdaptationFailure(), Client::handleMoreAdaptedBodyAvailable(), Adaptation::Ecap::XactionRep::preserveVb(), and Adaptation::Message::ShortCircuit().
◆ enableAutoConsumption()
void BodyPipe::enableAutoConsumption | ( | ) |
Definition at line 316 of file BodyPipe.cc.
References debugs, mustAutoConsume, startAutoConsumptionIfNeeded(), and status().
Referenced by ConnStateData::noteBodyConsumerAborted(), and Adaptation::Ecap::XactionRep::sinkVb().
◆ exhausted()
bool BodyPipe::exhausted | ( | ) | const |
Definition at line 174 of file BodyPipe.cc.
References expectMoreAfter(), and theGetSize.
Referenced by expectNoConsumption(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), ClientHttpRequest::noteBodyProductionEnded(), ClientHttpRequest::noteMoreBodyDataAvailable(), Client::resumeBodyStorage(), and Client::sentRequestBody().
◆ expectMoreAfter()
bool BodyPipe::expectMoreAfter | ( | uint64_t | offset | ) | const |
Definition at line 167 of file BodyPipe.cc.
References assert, mayNeedMoreData(), productionEnded(), theGetSize, and thePutSize.
Referenced by exhausted().
◆ expectNoConsumption()
void BodyPipe::expectNoConsumption | ( | ) |
Definition at line 267 of file BodyPipe.cc.
References abortedConsumption, asyncCall(), debugs, exhausted(), BodyProducer::noteBodyConsumerAborted(), ScheduleCallHere, startAutoConsumptionIfNeeded(), status(), theConsumer, and theProducer.
Referenced by clearConsumer(), FwdState::doneWithRetries(), ConnStateData::expectNoForwarding(), and Client::handleAdaptedHeader().
◆ expectProductionEndAfter()
void BodyPipe::expectProductionEndAfter | ( | uint64_t | extraSize | ) |
Definition at line 184 of file BodyPipe.cc.
References bodySize(), bodySizeKnown(), Must, size, theBodySize, and thePutSize.
◆ getMoreData()
Definition at line 294 of file BodyPipe.cc.
References MemBuf::append(), MemBuf::consume(), MemBuf::content(), MemBuf::contentSize(), MemBuf::hasContent(), MemBuf::init(), MemBuf::isNull(), min(), postConsume(), MemBuf::potentialSpaceSize(), size, and theBuf.
Referenced by Client::getMoreRequestBody(), and HttpStateData::getMoreRequestBody().
◆ mayNeedMoreData()
|
inline |
Definition at line 118 of file BodyPipe.h.
References bodySizeKnown(), and needsMoreData().
Referenced by expectMoreAfter(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), postAppend(), postConsume(), and Adaptation::Ecap::XactionRep::vbMakeMore().
◆ MEMPROXY_CLASS()
|
private |
◆ needsMoreData()
|
inline |
Definition at line 119 of file BodyPipe.h.
References bodySizeKnown(), and unproducedSize().
Referenced by mayNeedMoreData().
◆ postAppend()
|
protected |
Definition at line 395 of file BodyPipe.cc.
References assert, clearProducer(), debugs, isCheckedOut, mayNeedMoreData(), scheduleBodyDataNotification(), size, startAutoConsumptionIfNeeded(), status(), and thePutSize.
Referenced by checkIn(), and putMoreData().
◆ postConsume()
|
protected |
Definition at line 380 of file BodyPipe.cc.
References assert, asyncCall(), debugs, isCheckedOut, mayNeedMoreData(), BodyProducer::noteMoreBodySpaceAvailable(), ScheduleCallHere, size, status(), theGetSize, and theProducer.
Referenced by checkIn(), consume(), and getMoreData().
◆ producedSize()
|
inline |
Definition at line 112 of file BodyPipe.h.
References thePutSize.
Referenced by Adaptation::Icap::ModXact::finalizeLogInfo(), and ConnStateData::handleChunkedRequestBody().
◆ productionEnded()
|
inline |
Definition at line 113 of file BodyPipe.h.
References theProducer.
Referenced by clientProcessRequest(), expectMoreAfter(), and Adaptation::Ecap::XactionRep::vbMakeMore().
◆ putMoreData()
Definition at line 213 of file BodyPipe.cc.
References MemBuf::append(), bodySizeKnown(), min(), postAppend(), MemBuf::potentialSpaceSize(), size, theBuf, and unproducedSize().
Referenced by Client::adaptVirginReplyBody(), and ConnStateData::handleRequestBodyData().
◆ scheduleBodyDataNotification()
|
protected |
Definition at line 414 of file BodyPipe.cc.
References asyncCall(), BodyConsumer::noteMoreBodyDataAvailable(), ScheduleCallHere, and theConsumer.
Referenced by postAppend(), setConsumerIfNotLate(), and startAutoConsumptionIfNeeded().
◆ scheduleBodyEndNotification()
|
protected |
Definition at line 426 of file BodyPipe.cc.
References asyncCall(), bodySize(), bodySizeKnown(), BodyConsumer::noteBodyProducerAborted(), BodyConsumer::noteBodyProductionEnded(), ScheduleCallHere, theConsumer, and thePutSize.
Referenced by clearProducer(), and setConsumerIfNotLate().
◆ setBodySize()
void BodyPipe::setBodySize | ( | uint64_t | aSize | ) |
Definition at line 147 of file BodyPipe.cc.
References assert, bodySizeKnown(), debugs, status(), theBodySize, theConsumer, and thePutSize.
Referenced by ConnStateData::expectRequestBody(), and Client::startAdaptation().
◆ setConsumerIfNotLate()
bool BodyPipe::setConsumerIfNotLate | ( | const Consumer::Pointer & | aConsumer | ) |
Definition at line 228 of file BodyPipe.cc.
References abortedConsumption, assert, debugs, MemBuf::hasContent(), Must, mustAutoConsume, scheduleBodyDataNotification(), scheduleBodyEndNotification(), status(), theBuf, theConsumer, theGetSize, and theProducer.
Referenced by Client::handleAdaptedHeader(), ClientHttpRequest::handleAdaptedHeader(), Client::startRequestBodyFlow(), and Adaptation::Ecap::XactionRep::vbMake().
◆ startAutoConsumptionIfNeeded()
|
protected |
Check the current need and, if needed, start auto consumption. In auto consumption mode, the consumer is gone, but the producer continues to produce data. We use a BodySink BodyConsumer to discard that data.
Definition at line 327 of file BodyPipe.cc.
References debugs, MemBuf::hasContent(), mustAutoConsume, scheduleBodyDataNotification(), AsyncJob::Start(), status(), theBuf, theConsumer, and theProducer.
Referenced by enableAutoConsumption(), expectNoConsumption(), and postAppend().
◆ status()
const char * BodyPipe::status | ( | ) | const |
Definition at line 446 of file BodyPipe.cc.
References abortedConsumption, MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::contentSize(), CbcPointer< Cbc >::get(), isCheckedOut, mustAutoConsume, PRId64, PRIu64, MemBuf::reset(), MemBuf::spaceSize(), MemBuf::terminate(), theBodySize, theBuf, theConsumer, theGetSize, theProducer, and thePutSize.
Referenced by BodyPipe(), BodyProducerDialer::canDial(), BodyConsumerDialer::canDial(), clearConsumer(), clearProducer(), enableAutoConsumption(), expectNoConsumption(), ConnStateData::expectNoForwarding(), ConnStateData::finishDechunkingRequest(), ConnStateData::handleChunkedRequestBody(), postAppend(), postConsume(), setBodySize(), setConsumerIfNotLate(), startAutoConsumptionIfNeeded(), ConnStateData::startDechunkingRequest(), Client::startRequestBodyFlow(), Adaptation::Icap::ModXact::virginConsume(), and ~BodyPipe().
◆ stillConsuming()
|
inline |
Definition at line 132 of file BodyPipe.h.
References theConsumer.
Referenced by BodyConsumerDialer::canDial(), Adaptation::Ecap::XactionRep::forgetVb(), Adaptation::Ecap::XactionRep::status(), Adaptation::Ecap::XactionRep::swanSong(), and Adaptation::Ecap::XactionRep::vbMakeMore().
◆ stillProducing()
|
inline |
Definition at line 121 of file BodyPipe.h.
References theProducer.
Referenced by BodyProducerDialer::canDial(), Adaptation::Ecap::XactionRep::status(), and Adaptation::Ecap::XactionRep::swanSong().
◆ undoCheckOut()
|
protected |
Definition at line 364 of file BodyPipe.cc.
References assert, BodyPipeCheckout::checkedOutSize, MemBuf::contentSize(), isCheckedOut, Must, and theBuf.
◆ unproducedSize()
uint64_t BodyPipe::unproducedSize | ( | ) | const |
Definition at line 179 of file BodyPipe.cc.
References bodySize(), and thePutSize.
Referenced by ConnStateData::mayNeedToReadMoreBody(), needsMoreData(), and putMoreData().
Friends And Related Function Documentation
◆ BodyPipeCheckout
|
friend |
Definition at line 102 of file BodyPipe.h.
Member Data Documentation
◆ abortedConsumption
|
private |
Definition at line 167 of file BodyPipe.h.
Referenced by expectNoConsumption(), setConsumerIfNotLate(), and status().
◆ isCheckedOut
|
private |
Definition at line 168 of file BodyPipe.h.
Referenced by checkIn(), checkOut(), postAppend(), postConsume(), status(), and undoCheckOut().
◆ MaxCapacity
|
staticconstexpr |
Definition at line 100 of file BodyPipe.h.
Referenced by BodyPipe().
◆ mustAutoConsume
|
private |
Definition at line 166 of file BodyPipe.h.
Referenced by enableAutoConsumption(), setConsumerIfNotLate(), startAutoConsumptionIfNeeded(), and status().
◆ theBodySize
|
private |
Definition at line 157 of file BodyPipe.h.
Referenced by bodySize(), bodySizeKnown(), clearProducer(), expectProductionEndAfter(), setBodySize(), and status().
◆ theBuf
|
private |
Definition at line 164 of file BodyPipe.h.
Referenced by BodyPipe(), buf(), checkIn(), checkOut(), consume(), getMoreData(), putMoreData(), setConsumerIfNotLate(), startAutoConsumptionIfNeeded(), status(), undoCheckOut(), and ~BodyPipe().
◆ theConsumer
|
private |
Definition at line 159 of file BodyPipe.h.
Referenced by clearConsumer(), expectNoConsumption(), scheduleBodyDataNotification(), scheduleBodyEndNotification(), setBodySize(), setConsumerIfNotLate(), startAutoConsumptionIfNeeded(), status(), stillConsuming(), and ~BodyPipe().
◆ theGetSize
|
private |
Definition at line 162 of file BodyPipe.h.
Referenced by consumedSize(), exhausted(), expectMoreAfter(), postConsume(), setConsumerIfNotLate(), and status().
◆ theProducer
|
private |
Definition at line 158 of file BodyPipe.h.
Referenced by clearProducer(), expectNoConsumption(), postConsume(), productionEnded(), setConsumerIfNotLate(), startAutoConsumptionIfNeeded(), status(), stillProducing(), and ~BodyPipe().
◆ thePutSize
|
private |
Definition at line 161 of file BodyPipe.h.
Referenced by clearProducer(), expectMoreAfter(), expectProductionEndAfter(), postAppend(), producedSize(), scheduleBodyEndNotification(), setBodySize(), status(), and unproducedSize().
The documentation for this class was generated from the following files:
- src/BodyPipe.h
- src/BodyPipe.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products