#include <crtd_message.h>
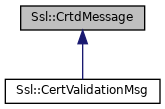
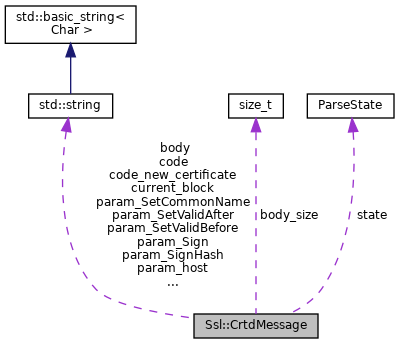
Public Types | |
enum | ParseResult { OK, INCOMPLETE, ERROR } |
Parse result codes. More... | |
enum | MessageKind { REPLY, REQUEST } |
typedef std::map< std::string, std::string > | BodyParams |
Public Member Functions | |
CrtdMessage (MessageKind kind) | |
ParseResult | parse (const char *buffer, size_t len) |
const std::string & | getBody () const |
Current body. If parsing is not finished the method returns incompleted body. More... | |
const std::string & | getCode () const |
Current response/request code. If parsing is not finished the method may return incompleted code. More... | |
void | setBody (std::string const &aBody) |
Set new body to encode. More... | |
void | setCode (std::string const &aCode) |
Set new request/reply code to compose. More... | |
std::string | compose () const |
void | clear () |
Reset the class. More... | |
void | parseBody (BodyParams &map, std::string &other_part) const |
void | composeBody (BodyParams const &map, std::string const &other_part) |
void | parseRequest (CertificateProperties &) |
orchestrates entire request parsing More... | |
void | composeRequest (Ssl::CertificateProperties const &) |
Static Public Attributes | |
static const std::string | code_new_certificate |
String code for "new_certificate" messages. More... | |
static const std::string | param_host |
Parameter name for passing hostname. More... | |
static const std::string | param_SetValidAfter |
Parameter name for passing SetValidAfter cert adaptation variable. More... | |
static const std::string | param_SetValidBefore |
Parameter name for passing SetValidBefore cert adaptation variable. More... | |
static const std::string | param_SetCommonName |
Parameter name for passing SetCommonName cert adaptation variable. More... | |
static const std::string | param_Sign |
Parameter name for passing signing algorithm. More... | |
static const std::string | param_SignHash |
The signing hash to use. More... | |
Protected Types | |
enum | ParseState { BEFORE_CODE, CODE, BEFORE_LENGTH, LENGTH, BEFORE_BODY, BODY, END } |
Protected Attributes | |
size_t | body_size |
The body size if exist or 0. More... | |
ParseState | state |
Parsing state. More... | |
std::string | body |
Current body. More... | |
std::string | code |
Current response/request code. More... | |
std::string | current_block |
Current block buffer. More... | |
Detailed Description
This class is responsible for composing and parsing messages destined to, or coming from an ssl_crtd server. Format of these messages is: response/request-code SP body length SP body
Definition at line 24 of file crtd_message.h.
Member Typedef Documentation
◆ BodyParams
typedef std::map<std::string, std::string> Ssl::CrtdMessage::BodyParams |
Definition at line 27 of file crtd_message.h.
Member Enumeration Documentation
◆ MessageKind
Enumerator | |
---|---|
REPLY | |
REQUEST |
Definition at line 34 of file crtd_message.h.
◆ ParseResult
Enumerator | |
---|---|
OK | |
INCOMPLETE | |
ERROR |
Definition at line 29 of file crtd_message.h.
◆ ParseState
|
protected |
Enumerator | |
---|---|
BEFORE_CODE | |
CODE | |
BEFORE_LENGTH | |
LENGTH | |
BEFORE_BODY | |
BODY | |
END |
Definition at line 90 of file crtd_message.h.
Constructor & Destructor Documentation
◆ CrtdMessage()
Ssl::CrtdMessage::CrtdMessage | ( | MessageKind | kind | ) |
Definition at line 19 of file crtd_message.cc.
Member Function Documentation
◆ clear()
void Ssl::CrtdMessage::clear | ( | ) |
Definition at line 134 of file crtd_message.cc.
References code.
◆ compose()
std::string Ssl::CrtdMessage::compose | ( | ) | const |
Compose current (request) code and body to string.
Definition at line 128 of file crtd_message.cc.
References code.
Referenced by ConnStateData::getSslContextStart(), processNewRequest(), Ssl::Helper::Submit(), and Ssl::CertValidationHelper::Submit().
◆ composeBody()
void Ssl::CrtdMessage::composeBody | ( | CrtdMessage::BodyParams const & | map, |
std::string const & | other_part | ||
) |
Compose parameters given by map with their values and the other part given by other_part to body data. The constructed body will have the form:
param1=value1 param2=value2 The other multistring part of body.
Definition at line 166 of file crtd_message.cc.
◆ composeRequest()
void Ssl::CrtdMessage::composeRequest | ( | Ssl::CertificateProperties const & | certProperties | ) |
Definition at line 233 of file crtd_message.cc.
References Ssl::algSignEnd, Ssl::appendCertToMemory(), Ssl::certSignAlgorithm(), Ssl::CertificateProperties::commonName, Security::LockingPointer< T, UnLocker, Locker >::get(), Here, Ssl::CertificateProperties::mimicCert, param_host, param_SetCommonName, param_SetValidAfter, param_SetValidBefore, param_Sign, param_SignHash, Ssl::CertificateProperties::setCommonName, Ssl::CertificateProperties::setValidAfter, Ssl::CertificateProperties::setValidBefore, Ssl::CertificateProperties::signAlgorithm, Ssl::CertificateProperties::signHash, Ssl::CertificateProperties::signWithPkey, Ssl::CertificateProperties::signWithX509, and Ssl::writeCertAndPrivateKeyToMemory().
Referenced by ConnStateData::getSslContextStart().
◆ getBody()
const std::string & Ssl::CrtdMessage::getBody | ( | ) | const |
Definition at line 120 of file crtd_message.cc.
Referenced by ConnStateData::sslCrtdHandleReply(), and sslCrtvdHandleReplyWrapper().
◆ getCode()
const std::string & Ssl::CrtdMessage::getCode | ( | ) | const |
◆ parse()
Ssl::CrtdMessage::ParseResult Ssl::CrtdMessage::parse | ( | const char * | buffer, |
size_t | len | ||
) |
Parse buffer of length len
- Return values
-
OK if parsing completes INCOMPLETE if more data required ERROR if there is an error.
Definition at line 23 of file crtd_message.cc.
References code, Comm::OK, xisalnum, xisalpha, xisdigit, and xisspace.
Referenced by main(), ConnStateData::sslCrtdHandleReply(), and sslCrtvdHandleReplyWrapper().
◆ parseBody()
void Ssl::CrtdMessage::parseBody | ( | CrtdMessage::BodyParams & | map, |
std::string & | other_part | ||
) | const |
Parse body data which has the form:
param1=value1 param2=value2 The other multistring part of body.
The parameters of the body stored to map and the remaining part to other_part
Definition at line 143 of file crtd_message.cc.
◆ parseRequest()
void Ssl::CrtdMessage::parseRequest | ( | CertificateProperties & | certProperties | ) |
Definition at line 179 of file crtd_message.cc.
References Ssl::algSignEnd, Ssl::algSignTrusted, Ssl::certSignAlgorithmId(), Ssl::CertificateProperties::commonName, Here, Ssl::CertificateProperties::mimicCert, param_host, param_SetCommonName, param_SetValidAfter, param_SetValidBefore, param_Sign, param_SignHash, Ssl::readCertAndPrivateKeyFromMemory(), Ssl::ReadCertificate(), Ssl::ReadOnlyBioTiedTo(), Ssl::CertificateProperties::setCommonName, Ssl::CertificateProperties::setValidAfter, Ssl::CertificateProperties::setValidBefore, Ssl::CertificateProperties::signAlgorithm, Ssl::CertificateProperties::signHash, Ssl::CertificateProperties::signWithPkey, Ssl::CertificateProperties::signWithX509, SQUID_SSL_SIGN_HASH_IF_NONE, and ToSBuf().
Referenced by processNewRequest().
◆ setBody()
void Ssl::CrtdMessage::setBody | ( | std::string const & | aBody | ) |
Definition at line 124 of file crtd_message.cc.
Referenced by processNewRequest().
◆ setCode()
void Ssl::CrtdMessage::setCode | ( | std::string const & | aCode | ) |
Definition at line 126 of file crtd_message.cc.
References code.
Referenced by ConnStateData::getSslContextStart(), processNewRequest(), and Ssl::CertValidationHelper::Submit().
Member Data Documentation
◆ body
|
protected |
Definition at line 101 of file crtd_message.h.
Referenced by Ssl::CertValidationMsg::composeRequest().
◆ body_size
|
protected |
Definition at line 99 of file crtd_message.h.
◆ code
|
protected |
Definition at line 102 of file crtd_message.h.
◆ code_new_certificate
|
static |
Definition at line 76 of file crtd_message.h.
Referenced by ConnStateData::getSslContextStart(), main(), and usage().
◆ current_block
|
protected |
Definition at line 103 of file crtd_message.h.
◆ param_host
|
static |
Definition at line 78 of file crtd_message.h.
Referenced by composeRequest(), Ssl::CertValidationMsg::composeRequest(), parseRequest(), and usage().
◆ param_SetCommonName
|
static |
Definition at line 84 of file crtd_message.h.
Referenced by composeRequest(), and parseRequest().
◆ param_SetValidAfter
|
static |
Definition at line 80 of file crtd_message.h.
Referenced by composeRequest(), and parseRequest().
◆ param_SetValidBefore
|
static |
Definition at line 82 of file crtd_message.h.
Referenced by composeRequest(), and parseRequest().
◆ param_Sign
|
static |
Definition at line 86 of file crtd_message.h.
Referenced by composeRequest(), and parseRequest().
◆ param_SignHash
|
static |
Definition at line 88 of file crtd_message.h.
Referenced by composeRequest(), and parseRequest().
◆ state
|
protected |
Definition at line 100 of file crtd_message.h.
The documentation for this class was generated from the following files:
- src/ssl/crtd_message.h
- src/ssl/crtd_message.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products