#include <Stream.h>
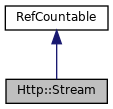
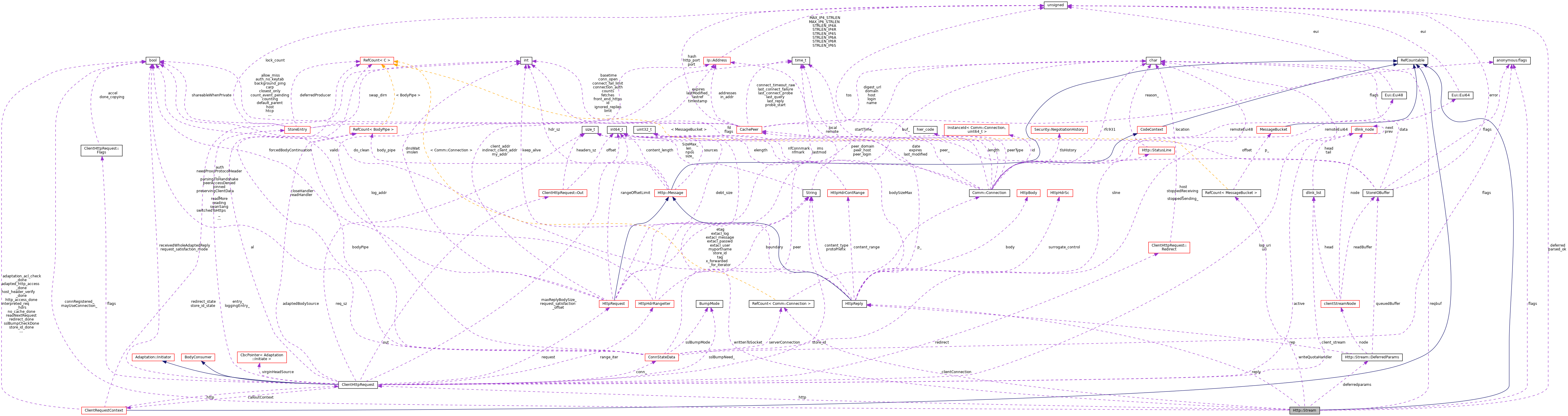
Classes | |
class | DeferredParams |
Public Member Functions | |
Stream (const Comm::ConnectionPointer &aConn, ClientHttpRequest *aReq) | |
construct with HTTP/1.x details More... | |
~Stream () override | |
void | registerWithConn () |
register this stream with the Server More... | |
bool | connRegistered () const |
whether it is registered with a Server More... | |
bool | startOfOutput () const |
whether the reply has started being sent More... | |
void | writeComplete (size_t size) |
update stream state after a write, may initiate more I/O More... | |
void | pullData () |
get more data to send More... | |
bool | multipartRangeRequest () const |
int64_t | getNextRangeOffset () const |
bool | canPackMoreRanges () const |
size_t | lengthToSend (Range< int64_t > const &available) const |
clientStream_status_t | socketState () |
Adapt stream status to account for Range cases. More... | |
void | sendStartOfMessage (HttpReply *, StoreIOBuffer bodyData) |
send an HTTP reply message headers and maybe some initial payload More... | |
void | sendBody (StoreIOBuffer bodyData) |
send some HTTP reply message payload More... | |
void | noteSentBodyBytes (size_t) |
void | buildRangeHeader (HttpReply *) |
add Range headers (if any) to the given HTTP reply message More... | |
clientStreamNode * | getTail () const |
clientStreamNode * | getClientReplyContext () const |
ConnStateData * | getConn () const |
void | noteIoError (const Error &, const LogTagsErrors &) |
update state to reflect I/O error More... | |
void | finished () |
cleanup when the transaction has finished. may destroy 'this' More... | |
void | initiateClose (const char *reason) |
terminate due to a send/write error (may continue reading) More... | |
void | deferRecipientForLater (clientStreamNode *, HttpReply *, StoreIOBuffer receivedData) |
bool | mayUseConnection () const |
void | mayUseConnection (bool aBool) |
Public Attributes | |
Comm::ConnectionPointer | clientConnection |
details about the client connection socket More... | |
ClientHttpRequest * | http |
HttpReply * | reply |
char | reqbuf [HTTP_REQBUF_SZ] |
struct { | |
unsigned deferred:1 | |
This is a pipelined request waiting for the current object to complete. More... | |
unsigned parsed_ok:1 | |
Was this parsed correctly? More... | |
} | flags |
DeferredParams | deferredparams |
int64_t | writtenToSocket |
Private Member Functions | |
MEMPROXY_CLASS (Stream) | |
void | prepareReply (HttpReply *) |
void | packChunk (const StoreIOBuffer &bodyData, MemBuf &) |
void | packRange (StoreIOBuffer const &, MemBuf *) |
void | doClose () |
Private Attributes | |
bool | mayUseConnection_ |
bool | connRegistered_ |
MessageBucket::Pointer | writeQuotaHandler |
response write limiter, if configured More... | |
Detailed Description
The processing context for a single HTTP transaction (stream).
A stream lifetime extends from directly after a request has been parsed off the client connection buffer, until the last byte of both request and reply payload (if any) have been written, or it is otherwise explicitly terminated.
Streams self-register with the Http::Server Pipeline being managed by the Server for the connection on which the request was received.
The socket level management and I/O is done by a Server which owns us. The scope of this objects control over a socket consists of the data buffer received from the Server with an initially unknown length. When that length is known it sets the end boundary of our access to the buffer.
The individual processing actions are done by other Jobs which we start.
When a stream is completed the finished() method needs to be called which will perform all cleanup and deregistration operations. If the reason for finishing is an error, then notifyIoError() needs to be called prior to the finished() method. The caller should follow finished() with a call to ConnStateData::kick() to resume processing of other transactions or I/O on the connection.
Alternatively the initiateClose() method can be called to terminate the whole client connection and all other pending streams.
HTTP/1.x:
When HTTP/1 pipeline is operating there may be multiple transactions using the client connection. Only the back() stream may read from the connection, and only the front() stream may write to it. A stream which needs to read or write to the connection but does not meet those criteria must be shifted to the deferred state.
XXX: If an async call ends the ClientHttpRequest job, Http::Stream (and ConnStateData) may not know about it, leading to segfaults and assertions. This is difficult to fix because ClientHttpRequest lacks a good way to communicate its ongoing destruction back to the Http::Stream which pretends to "own" *http.
Constructor & Destructor Documentation
◆ Stream()
Http::Stream::Stream | ( | const Comm::ConnectionPointer & | aConn, |
ClientHttpRequest * | aReq | ||
) |
Definition at line 24 of file Stream.cc.
References assert, deferredparams, flags, http, Http::Stream::DeferredParams::node, Http::Stream::DeferredParams::rep, and reqbuf.
◆ ~Stream()
|
override |
Definition at line 40 of file Stream.cc.
References assert, and httpRequestFree().
Member Function Documentation
◆ buildRangeHeader()
void Http::Stream::buildRangeHeader | ( | HttpReply * | rep | ) |
adds appropriate Range headers if needed
Definition at line 417 of file Stream.cc.
References assert, clientIfRangeMatch(), Http::CONTENT_LENGTH, Http::Message::content_length, Http::CONTENT_RANGE, Http::CONTENT_TYPE, HttpReply::contentRange(), debugs, HttpHeader::delById(), HttpRequest::getRangeOffsetLimit(), HttpHeader::has(), Http::Message::header, httpHeaderAddContRange(), httpHeaderPutStrf(), Http::IF_RANGE, Must, HttpHeader::putInt64(), HttpRequest::range, Http::scOkay, Http::scPartialContent, Http::StatusLine::set(), HttpReply::sline, SQUIDSTRINGPH, SQUIDSTRINGPRINT, Http::StatusLine::status(), and Http::StatusLine::version.
◆ canPackMoreRanges()
bool Http::Stream::canPackMoreRanges | ( | ) | const |
◆ connRegistered()
|
inline |
Definition at line 85 of file Stream.h.
References connRegistered_.
◆ deferRecipientForLater()
void Http::Stream::deferRecipientForLater | ( | clientStreamNode * | node, |
HttpReply * | rep, | ||
StoreIOBuffer | receivedData | ||
) |
◆ doClose()
◆ finished()
void Http::Stream::finished | ( | ) |
Definition at line 534 of file Stream.cc.
References assert, clientStreamDetach(), Server::pipeline, Pipeline::popMe(), and CodeContext::Reset().
◆ getClientReplyContext()
clientStreamNode * Http::Stream::getClientReplyContext | ( | ) | const |
Definition at line 511 of file Stream.cc.
Referenced by clientProcessRequest(), Ftp::Server::earlyError(), and ConnStateData::serveDelayedError().
◆ getConn()
ConnStateData * Http::Stream::getConn | ( | ) | const |
◆ getNextRangeOffset()
int64_t Http::Stream::getNextRangeOffset | ( | ) | const |
Definition at line 132 of file Stream.cc.
References assert, debugs, and HttpHdrRangeSpec::UnknownPosition.
◆ getTail()
clientStreamNode * Http::Stream::getTail | ( | ) | const |
◆ initiateClose()
void Http::Stream::initiateClose | ( | const char * | reason | ) |
◆ lengthToSend()
Definition at line 338 of file Stream.cc.
References assert, min(), Range< C, S >::size(), and Range< C, S >::start.
◆ mayUseConnection() [1/2]
|
inline |
Definition at line 143 of file Stream.h.
References mayUseConnection_.
Referenced by ConnStateData::buildFakeRequest(), and clientProcessRequest().
◆ mayUseConnection() [2/2]
|
inline |
Definition at line 145 of file Stream.h.
References debugs, and mayUseConnection_.
◆ MEMPROXY_CLASS()
|
private |
◆ multipartRangeRequest()
bool Http::Stream::multipartRangeRequest | ( | ) | const |
◆ noteIoError()
void Http::Stream::noteIoError | ( | const Error & | error, |
const LogTagsErrors & | lte | ||
) |
◆ noteSentBodyBytes()
void Http::Stream::noteSentBodyBytes | ( | size_t | bytes | ) |
◆ packChunk()
|
private |
Packs bodyData into mb using chunked encoding. Packs the last-chunk if bodyData is empty.
Definition at line 579 of file Stream.cc.
References MemBuf::append(), Packable::appendf(), StoreIOBuffer::data, PRIX64, and StoreIOBuffer::range().
◆ packRange()
|
private |
extracts a "range" from *buf and appends them to mb, updating all offsets and such.
Definition at line 595 of file Stream.cc.
References MemBuf::append(), assert, HttpHdrRangeIter::boundary, clientPackRangeHdr(), clientPackTermBound(), HttpHdrRangeIter::currentSpec(), StoreIOBuffer::data, HttpHdrRangeIter::debt(), debugs, HttpHdrRangeSpec::length, HttpHdrRangeSpec::offset, StoreIOBuffer::range(), Range< C, S >::size(), and Range< C, S >::start.
◆ prepareReply()
|
private |
◆ pullData()
void Http::Stream::pullData | ( | ) |
Definition at line 110 of file Stream.cc.
References clientStreamRead(), StoreIOBuffer::data, debugs, HTTP_REQBUF_SZ, StoreIOBuffer::length, and StoreIOBuffer::offset.
Referenced by clientProcessRequest(), and ConnStateData::serveDelayedError().
◆ registerWithConn()
void Http::Stream::registerWithConn | ( | ) |
Definition at line 53 of file Stream.cc.
References assert.
Referenced by ConnStateData::buildFakeRequest().
◆ sendBody()
void Http::Stream::sendBody | ( | StoreIOBuffer | bodyData | ) |
Definition at line 315 of file Stream.cc.
References MemBuf::contentSize(), StoreIOBuffer::data, MemBuf::init(), and StoreIOBuffer::range().
◆ sendStartOfMessage()
void Http::Stream::sendStartOfMessage | ( | HttpReply * | rep, |
StoreIOBuffer | bodyData | ||
) |
Definition at line 267 of file Stream.cc.
References MemBuf::append(), assert, MemBuf::buf, clientAclChecklistFill(), MemBuf::contentSize(), StoreIOBuffer::data, debugs, ACLChecklist::fastCheck(), fd_table, MessageDelayPools::Instance(), StoreIOBuffer::length, HttpReply::pack(), StoreIOBuffer::range(), and ACLFilledChecklist::updateReply().
◆ socketState()
clientStream_status_t Http::Stream::socketState | ( | ) |
Definition at line 211 of file Stream.cc.
References assert, clientStreamStatus(), debugs, fatal(), STREAM_COMPLETE, STREAM_FAILED, STREAM_NONE, STREAM_UNPLANNED_COMPLETE, and HttpHdrRangeSpec::UnknownPosition.
◆ startOfOutput()
◆ writeComplete()
void Http::Stream::writeComplete | ( | size_t | size | ) |
Definition at line 68 of file Stream.cc.
References debugs, fatal(), ConnStateData::kick(), StoreEntry::objectLen(), size, STREAM_COMPLETE, STREAM_FAILED, STREAM_NONE, and STREAM_UNPLANNED_COMPLETE.
Member Data Documentation
◆ clientConnection
Comm::ConnectionPointer Http::Stream::clientConnection |
◆ connRegistered_
|
private |
Definition at line 169 of file Stream.h.
Referenced by connRegistered().
◆ deferred
◆ deferredparams
DeferredParams Http::Stream::deferredparams |
◆ flags
struct { ... } Http::Stream::flags |
◆ http
ClientHttpRequest* Http::Stream::http |
Definition at line 135 of file Stream.h.
Referenced by clientProcessRequest(), ConnStateData::serveDelayedError(), and Stream().
◆ mayUseConnection_
|
private |
Definition at line 168 of file Stream.h.
Referenced by mayUseConnection().
◆ parsed_ok
unsigned Http::Stream::parsed_ok |
Definition at line 140 of file Stream.h.
Referenced by ConnStateData::buildFakeRequest(), ConnStateData::parseHttpRequest(), and Ftp::Server::parseOneRequest().
◆ reply
◆ reqbuf
char Http::Stream::reqbuf[HTTP_REQBUF_SZ] |
Definition at line 137 of file Stream.h.
Referenced by ConnStateData::buildFakeRequest(), ConnStateData::parseHttpRequest(), Ftp::Server::parseOneRequest(), and Stream().
◆ writeQuotaHandler
|
private |
◆ writtenToSocket
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products