#include <HttpHeader.h>
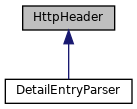
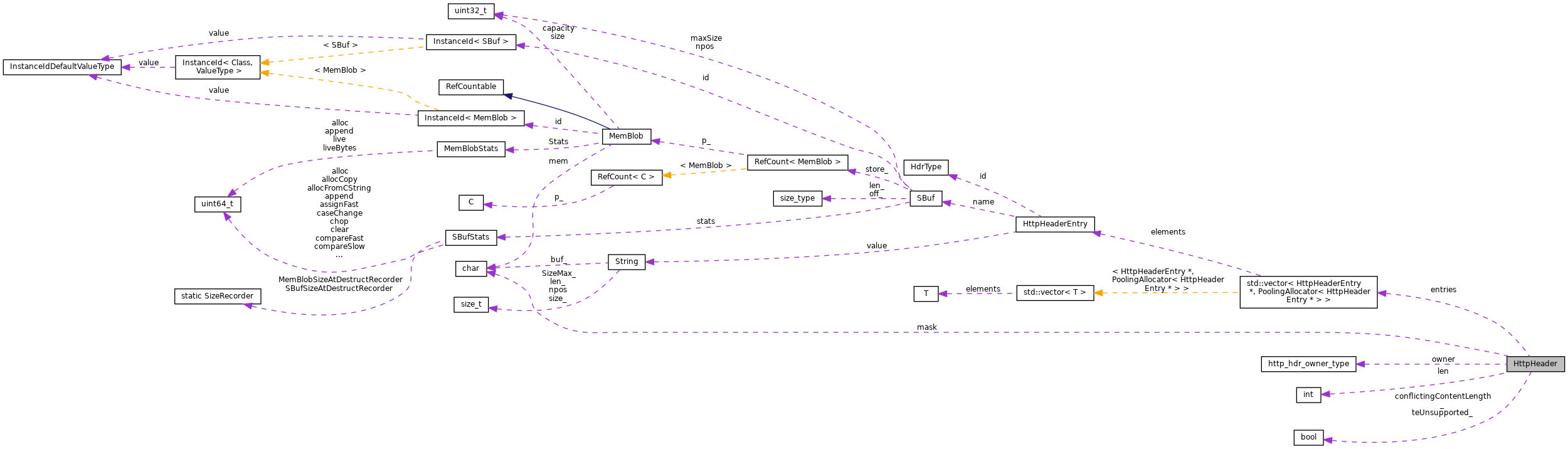
Public Member Functions | |
HttpHeader (const http_hdr_owner_type owner) | |
HttpHeader (const HttpHeader &other) | |
~HttpHeader () | |
HttpHeader & | operator= (const HttpHeader &other) |
void | clean () |
void | append (const HttpHeader *src) |
void | update (const HttpHeader *fresh) |
bool | needUpdate (const HttpHeader *fresh) const |
void | compact () |
int | parse (const char *header_start, size_t len, Http::ContentLengthInterpreter &interpreter) |
int | parse (const char *buf, size_t buf_len, bool atEnd, size_t &hdr_sz, Http::ContentLengthInterpreter &interpreter) |
void | packInto (Packable *p, bool mask_sensitive_info=false) const |
HttpHeaderEntry * | getEntry (HttpHeaderPos *pos) const |
HttpHeaderEntry * | findEntry (Http::HdrType id) const |
int | delByName (const SBuf &name) |
int | delByName (const char *name) |
int | delById (Http::HdrType id) |
void | delAt (HttpHeaderPos pos, int &headers_deleted) |
void | refreshMask () |
void | addEntry (HttpHeaderEntry *e) |
String | getList (Http::HdrType id) const |
bool | getList (Http::HdrType id, String *s) const |
bool | conflictingContentLength () const |
String | getStrOrList (Http::HdrType id) const |
String | getByName (const SBuf &name) const |
String | getByName (const char *name) const |
String | getById (Http::HdrType id) const |
bool | getByIdIfPresent (Http::HdrType id, String *result) const |
bool | hasNamed (const SBuf &s, String *value=nullptr) const |
bool | hasNamed (const char *name, unsigned int namelen, String *value=nullptr) const |
SBuf | getByNameListMember (const char *name, const char *member, const char separator) const |
SBuf | getListMember (Http::HdrType id, const char *member, const char separator) const |
int | has (Http::HdrType id) const |
void | addVia (const AnyP::ProtocolVersion &ver, const HttpHeader *from=nullptr) |
void | putInt (Http::HdrType id, int number) |
void | putInt64 (Http::HdrType id, int64_t number) |
void | putTime (Http::HdrType id, time_t htime) |
void | putStr (Http::HdrType id, const char *str) |
void | putAuth (const char *auth_scheme, const char *realm) |
void | putCc (const HttpHdrCc &cc) |
void | putContRange (const HttpHdrContRange *cr) |
void | putRange (const HttpHdrRange *range) |
void | putSc (HttpHdrSc *sc) |
void | putExt (const char *name, const char *value) |
void | updateOrAddStr (Http::HdrType, const SBuf &) |
int | getInt (Http::HdrType id) const |
int64_t | getInt64 (Http::HdrType id) const |
time_t | getTime (Http::HdrType id) const |
const char * | getStr (Http::HdrType id) const |
const char * | getLastStr (Http::HdrType id) const |
HttpHdrCc * | getCc () const |
HttpHdrRange * | getRange () const |
HttpHdrSc * | getSc () const |
HttpHdrContRange * | getContRange () const |
SBuf | getAuthToken (Http::HdrType id, const char *auth_scheme) const |
ETag | getETag (Http::HdrType id) const |
TimeOrTag | getTimeOrTag (Http::HdrType id) const |
int | hasListMember (Http::HdrType id, const char *member, const char separator) const |
int | hasByNameListMember (const char *name, const char *member, const char separator) const |
void | removeHopByHopEntries () |
bool | chunked () const |
bool | unsupportedTe () const |
whether message used an unsupported and/or invalid Transfer-Encoding More... | |
Public Attributes | |
std::vector< HttpHeaderEntry *, PoolingAllocator< HttpHeaderEntry * > > | entries |
HttpHeaderMask | mask |
http_hdr_owner_type | owner |
int | len |
Protected Member Functions | |
void | removeConnectionHeaderEntries () |
bool | skipUpdateHeader (const Http::HdrType id) const |
Static Protected Member Functions | |
static bool | Isolate (const char **parse_start, size_t l, const char **blk_start, const char **blk_end) |
Private Member Functions | |
HttpHeaderEntry * | findLastEntry (Http::HdrType id) const |
Private Attributes | |
bool | conflictingContentLength_ |
bool | teUnsupported_ = false |
Detailed Description
Definition at line 74 of file HttpHeader.h.
Constructor & Destructor Documentation
◆ HttpHeader() [1/2]
|
explicit |
Definition at line 147 of file HttpHeader.cc.
References assert, debugs, entries, hoEnd, hoNone, httpHeaderMaskInit(), mask, and owner.
◆ HttpHeader() [2/2]
HttpHeader::HttpHeader | ( | const HttpHeader & | other | ) |
Definition at line 156 of file HttpHeader.cc.
References entries, httpHeaderMaskInit(), mask, and update().
◆ ~HttpHeader()
HttpHeader::~HttpHeader | ( | ) |
Definition at line 163 of file HttpHeader.cc.
References clean().
Member Function Documentation
◆ addEntry()
void HttpHeader::addEntry | ( | HttpHeaderEntry * | e | ) |
Definition at line 736 of file HttpHeader.cc.
References Http::any_HdrType_enum_value(), assert, Http::BAD_HDR, CBIT_SET, CBIT_TEST, debugs, entries, headerStatsTable(), HttpHeaderEntry::id, len, HttpHeaderEntry::length(), SBuf::length(), mask, and HttpHeaderEntry::name.
Referenced by append(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), Adaptation::Icap::ModXact::encapsulateHead(), Ftp::Server::handleFeatReply(), HttpStateData::httpBuildRequestHeader(), httpHdrAdd(), HttpReply::make304(), parse(), putCc(), putContRange(), putExt(), putInt(), putInt64(), putRange(), putSc(), putStr(), putTime(), update(), and updateOrAddStr().
◆ addVia()
void HttpHeader::addVia | ( | const AnyP::ProtocolVersion & | ver, |
const HttpHeader * | from = nullptr |
||
) |
Appends "this cache" information to VIA header field. Takes the initial VIA value from "from" parameter, if provided.
Definition at line 945 of file HttpHeader.cc.
References SBuf::append(), SBuf::appendf(), Config, getList(), SBuf::isEmpty(), AnyP::ProtocolVersion::major, AnyP::ProtocolVersion::minor, SquidConfig::onoff, AnyP::PROTO_HTTP, AnyP::PROTO_HTTPS, AnyP::PROTO_NONE, AnyP::PROTO_UNKNOWN, AnyP::ProtocolVersion::protocol, AnyP::ProtocolType_str, StringToSBuf(), ThisCache, updateOrAddStr(), Http::VIA, and SquidConfig::via.
Referenced by clientReplyContext::buildReplyHeader(), and HttpStateData::httpBuildRequestHeader().
◆ append()
void HttpHeader::append | ( | const HttpHeader * | src | ) |
Definition at line 231 of file HttpHeader.cc.
References addEntry(), assert, debugs, and entries.
Referenced by HttpRequest::clone(), and HttpReply::clone().
◆ chunked()
|
inline |
whether the message uses chunked Transfer-Encoding optimized implementation relies on us rejecting/removing other codings
Definition at line 169 of file HttpHeader.h.
References has(), and Http::TRANSFER_ENCODING.
Referenced by HttpRequest::checkEntityFraming(), clientProcessRequest(), HttpReply::expectingBody(), and HttpRequest::expectingBody().
◆ clean()
void HttpHeader::clean | ( | ) |
Definition at line 185 of file HttpHeader.cc.
References Http::any_valid_header(), assert, conflictingContentLength_, DBG_CRITICAL, debugs, entries, hoEnd, hoNone, hoReply, httpHeaderMaskInit(), HttpHeaderStats, len, mask, owner, and teUnsupported_.
Referenced by HttpStateData::buildRequestPrefix(), HttpRequest::clean(), HttpReply::clean(), clientPackRangeHdr(), htcpClear(), htcpHandleTstResponse(), htcpQuery(), htcpTstReply(), operator=(), parse(), Http::Tunneler::writeRequest(), and ~HttpHeader().
◆ compact()
void HttpHeader::compact | ( | ) |
Definition at line 711 of file HttpHeader.cc.
References entries.
Referenced by HttpReply::recreateOnNotModified().
◆ conflictingContentLength()
|
inline |
Definition at line 113 of file HttpHeader.h.
References conflictingContentLength_.
Referenced by HttpRequest::checkEntityFraming().
◆ delAt()
void HttpHeader::delAt | ( | HttpHeaderPos | pos, |
int & | headers_deleted | ||
) |
Definition at line 694 of file HttpHeader.cc.
References assert, entries, HttpHeaderInitPos, len, SBuf::length(), HttpHeaderEntry::name, String::size(), and HttpHeaderEntry::value.
Referenced by clientReplyContext::buildReplyHeader(), delById(), delByName(), httpHdrMangleList(), removeConnectionHeaderEntries(), and removeHopByHopEntries().
◆ delById()
int HttpHeader::delById | ( | Http::HdrType | id | ) |
Definition at line 666 of file HttpHeader.cc.
References Http::any_registered_header(), assert, CBIT_CLR, CBIT_TEST, debugs, delAt(), getEntry(), HttpHeaderInitPos, and mask.
Referenced by Http::Stream::buildRangeHeader(), clientReplyContext::buildReplyHeader(), clientInterpretRequestHeaders(), Adaptation::Icap::ModXact::encapsulateHead(), HttpStateData::httpBuildRequestHeader(), parse(), clientReplyContext::processConditional(), putCc(), putContRange(), putRange(), putSc(), HttpReply::removeIrrelevantContentLength(), and update().
◆ delByName() [1/2]
|
inline |
- Deprecated:
- use SBuf method instead. performance regression: reallocates
Definition at line 106 of file HttpHeader.h.
References delByName().
Referenced by delByName().
◆ delByName() [2/2]
deletes all fields with a given name, if any.
- Returns
- #fields deleted
Definition at line 647 of file HttpHeader.cc.
References CBIT_SET, debugs, delAt(), getEntry(), HttpHeaderInitPos, httpHeaderMaskInit(), and mask.
Referenced by update().
◆ findEntry()
HttpHeaderEntry * HttpHeader::findEntry | ( | Http::HdrType | id | ) | const |
Definition at line 602 of file HttpHeader.cc.
References Http::any_registered_header(), assert, CBIT_TEST, entries, Http::HeaderLookupTable, and mask.
Referenced by clientReplyContext::buildReplyHeader(), getContRange(), getETag(), getInt(), getInt64(), getRange(), getStr(), getStrOrList(), getTime(), getTimeOrTag(), Ftp::Server::handleRequest(), HttpReply::make304(), packInto(), and Ftp::Relay::sendCommand().
◆ findLastEntry()
|
private |
Definition at line 627 of file HttpHeader.cc.
References Http::any_registered_header(), assert, CBIT_TEST, entries, Http::HeaderLookupTable, and mask.
Referenced by getLastStr().
◆ getAuthToken()
SBuf HttpHeader::getAuthToken | ( | Http::HdrType | id, |
const char * | auth_scheme | ||
) | const |
Definition at line 1275 of file HttpHeader.cc.
References assert, base64_decode_final(), base64_decode_init(), BASE64_DECODE_LENGTH, base64_decode_update(), getStr(), SBuf::rawAppendFinish(), SBuf::rawAppendStart(), and xisspace.
Referenced by Ftp::Gateway::checkAuth(), and CacheManager::ParseHeaders().
◆ getById()
String HttpHeader::getById | ( | Http::HdrType | id | ) | const |
Definition at line 857 of file HttpHeader.cc.
References getByIdIfPresent().
◆ getByIdIfPresent()
bool HttpHeader::getByIdIfPresent | ( | Http::HdrType | id, |
String * | result | ||
) | const |
returns true iff a [possibly empty] field identified by id is there when returning true, also sets the result
parameter (if it is not nil)
Definition at line 871 of file HttpHeader.cc.
References Http::BAD_HDR, getStrOrList(), and has().
Referenced by getById(), hasNamed(), and parse().
◆ getByName() [1/2]
String HttpHeader::getByName | ( | const char * | name | ) | const |
Definition at line 839 of file HttpHeader.cc.
References hasNamed().
◆ getByName() [2/2]
Definition at line 848 of file HttpHeader.cc.
References hasNamed().
Referenced by Format::Format::assemble(), assembleVaryKey(), Adaptation::Icap::Options::cfgIntHeader(), Adaptation::Icap::Options::cfgTransferList(), Adaptation::Icap::Options::configure(), getByNameListMember(), hasByNameListMember(), needUpdate(), Ssl::ErrorDetailFile::parse(), and Ftp::Server::writeErrorReply().
◆ getByNameListMember()
SBuf HttpHeader::getByNameListMember | ( | const char * | name, |
const char * | member, | ||
const char | separator | ||
) | const |
searches for the first matching key=value pair within the name-identified field
- Returns
- the value of the found pair or an empty string
Definition at line 917 of file HttpHeader.cc.
References assert, getByName(), and getListMember().
Referenced by Format::Format::assemble().
◆ getCc()
HttpHdrCc * HttpHeader::getCc | ( | ) | const |
Definition at line 1194 of file HttpHeader.cc.
References Http::CACHE_CONTROL, CBIT_TEST, getList(), httpHdrCcUpdateStats(), httpHeaderNoteParsedEntry(), HttpHeaderStats, mask, owner, and HttpHdrCc::parse().
Referenced by HttpStateData::httpBuildRequestHeader().
◆ getContRange()
HttpHdrContRange * HttpHeader::getContRange | ( | ) | const |
Definition at line 1261 of file HttpHeader.cc.
References Http::CONTENT_RANGE, findEntry(), httpHdrContRangeParseCreate(), httpHeaderNoteParsedEntry(), HttpHeaderEntry::id, String::termedBuf(), and HttpHeaderEntry::value.
Referenced by HttpReply::hdrCacheInit().
◆ getEntry()
HttpHeaderEntry * HttpHeader::getEntry | ( | HttpHeaderPos * | pos | ) | const |
Definition at line 583 of file HttpHeader.cc.
References assert, entries, and HttpHeaderInitPos.
Referenced by Format::Format::assemble(), clientReplyContext::buildReplyHeader(), clientCheckPinning(), delById(), delByName(), getList(), Ftp::Server::handleFeatReply(), hasNamed(), HttpStateData::httpBuildRequestHeader(), httpHdrMangleList(), packInto(), Ftp::PrintReply(), removeConnectionHeaderEntries(), removeHopByHopEntries(), and update().
◆ getETag()
ETag HttpHeader::getETag | ( | Http::HdrType | id | ) | const |
Definition at line 1317 of file HttpHeader.cc.
References assert, etagParseInit(), findEntry(), Http::ftETag, Http::HeaderLookupTable, String::termedBuf(), and HttpHeaderEntry::value.
Referenced by clientIfRangeMatch(), and StoreEntry::hasOneOfEtags().
◆ getInt()
int HttpHeader::getInt | ( | Http::HdrType | id | ) | const |
Definition at line 1120 of file HttpHeader.cc.
References Http::any_registered_header(), assert, findEntry(), Http::ftInt, HttpHeaderEntry::getInt(), and Http::HeaderLookupTable.
Referenced by Ftp::Server::handleFeatReply(), Ftp::PrintReply(), and Ftp::Server::writeForwardedReplyAndCall().
◆ getInt64()
int64_t HttpHeader::getInt64 | ( | Http::HdrType | id | ) | const |
Definition at line 1133 of file HttpHeader.cc.
References Http::any_registered_header(), assert, findEntry(), Http::ftInt64, HttpHeaderEntry::getInt64(), and Http::HeaderLookupTable.
Referenced by clientGetMoreData(), clientProcessRequest(), ftpSendStor(), HttpReply::hdrCacheInit(), and urlCheckRequest().
◆ getLastStr()
const char * HttpHeader::getLastStr | ( | Http::HdrType | id | ) | const |
Definition at line 1179 of file HttpHeader.cc.
References Http::any_registered_header(), assert, findLastEntry(), Http::ftStr, Http::HeaderLookupTable, httpHeaderNoteParsedEntry(), HttpHeaderEntry::id, String::termedBuf(), and HttpHeaderEntry::value.
◆ getList() [1/2]
String HttpHeader::getList | ( | Http::HdrType | id | ) | const |
Definition at line 788 of file HttpHeader.cc.
References assert, CBIT_TEST, debugs, getEntry(), Http::HeaderLookupTable, HttpHeaderInitPos, HttpHeaderEntry::id, mask, String::size(), strListAdd(), String::termedBuf(), and HttpHeaderEntry::value.
Referenced by addVia(), HttpStateData::blockSwitchingProtocols(), HttpStateData::buildRequestPrefix(), ClientRequestContext::clientAccessCheck(), clientInterpretRequestHeaders(), Ssl::ErrorDetailsManager::findDetail(), HttpStateData::forwardUpgrade(), getCc(), getSc(), getStrOrList(), StoreEntry::hasIfMatchEtag(), StoreEntry::hasIfNoneMatchEtag(), HttpStateData::httpBuildRequestHeader(), httpHeaderHasConnDir(), httpMakeVaryMark(), Auth::SchemeConfig::isCP1251EncodingAllowed(), TemplateFile::loadFor(), Http::One::Server::processParsedRequest(), removeConnectionHeaderEntries(), and Http::One::Server::writeControlMsgAndCall().
◆ getList() [2/2]
bool HttpHeader::getList | ( | Http::HdrType | id, |
String * | s | ||
) | const |
Definition at line 758 of file HttpHeader.cc.
References assert, CBIT_TEST, debugs, entries, Http::HeaderLookupTable, mask, String::size(), strListAdd(), and String::termedBuf().
◆ getListMember()
SBuf HttpHeader::getListMember | ( | Http::HdrType | id, |
const char * | member, | ||
const char | separator | ||
) | const |
searches for the first matching key=value pair within the field
- Returns
- the value of the found pair or an empty string
Definition at line 928 of file HttpHeader.cc.
References Http::any_registered_header(), assert, getListMember(), and getStrOrList().
◆ getRange()
HttpHdrRange * HttpHeader::getRange | ( | ) | const |
Definition at line 1220 of file HttpHeader.cc.
References findEntry(), httpHeaderNoteParsedEntry(), HttpHeaderEntry::id, HttpHdrRange::ParseCreate(), Http::RANGE, Http::REQUEST_RANGE, and HttpHeaderEntry::value.
Referenced by clientInterpretRequestHeaders(), and HttpRequest::hdrCacheInit().
◆ getSc()
HttpHdrSc * HttpHeader::getSc | ( | ) | const |
Definition at line 1239 of file HttpHeader.cc.
References CBIT_TEST, getList(), httpHdrScParseCreate(), httpHeaderNoteParsedEntry(), HttpHeaderStats, mask, owner, sc, and Http::SURROGATE_CONTROL.
Referenced by HttpReply::hdrCacheInit().
◆ getStr()
const char * HttpHeader::getStr | ( | Http::HdrType | id | ) | const |
Definition at line 1163 of file HttpHeader.cc.
References Http::any_registered_header(), assert, findEntry(), Http::ftStr, Http::HeaderLookupTable, httpHeaderNoteParsedEntry(), HttpHeaderEntry::id, String::termedBuf(), and HttpHeaderEntry::value.
Referenced by Auth::UserRequest::authenticate(), clientPackRangeHdr(), getAuthToken(), HttpReply::hdrCacheInit(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), Log::Format::HttpdCombined(), httpFixupAuthentication(), Ftp::PrintReply(), purgeEntriesByHeader(), HttpStateData::reusableReply(), Log::Format::SquidReferer(), Log::Format::SquidUserAgent(), CacheManager::start(), and Ftp::Server::writeErrorReply().
◆ getStrOrList()
String HttpHeader::getStrOrList | ( | Http::HdrType | id | ) | const |
Definition at line 822 of file HttpHeader.cc.
References findEntry(), getList(), Http::HeaderLookupTable, and HttpHeaderEntry::value.
Referenced by getByIdIfPresent(), getListMember(), hasListMember(), ACLHTTPHeaderData::match(), and HttpReply::validatorsMatch().
◆ getTime()
time_t HttpHeader::getTime | ( | Http::HdrType | id | ) | const |
Definition at line 1146 of file HttpHeader.cc.
References Http::any_registered_header(), assert, findEntry(), Http::ftDate_1123, Http::HeaderLookupTable, httpHeaderNoteParsedEntry(), HttpHeaderEntry::id, Time::ParseRfc1123(), String::termedBuf(), and HttpHeaderEntry::value.
Referenced by clientInterpretRequestHeaders(), Adaptation::Icap::Options::configure(), HttpReply::hdrCacheInit(), and HttpReply::hdrExpirationTime().
◆ getTimeOrTag()
TimeOrTag HttpHeader::getTimeOrTag | ( | Http::HdrType | id | ) | const |
Definition at line 1330 of file HttpHeader.cc.
References assert, etagParseInit(), findEntry(), Http::ftDate_1123_or_ETag, Http::HeaderLookupTable, Time::ParseRfc1123(), ETag::str, TimeOrTag::tag, String::termedBuf(), TimeOrTag::time, TimeOrTag::valid, and HttpHeaderEntry::value.
Referenced by clientIfRangeMatch().
◆ has()
int HttpHeader::has | ( | Http::HdrType | id | ) | const |
Definition at line 937 of file HttpHeader.cc.
References Http::any_registered_header(), assert, CBIT_TEST, debugs, and mask.
Referenced by HttpStateData::blockSwitchingProtocols(), Http::One::Server::buildHttpRequest(), Http::Stream::buildRangeHeader(), clientReplyContext::buildReplyHeader(), HttpStateData::buildRequestPrefix(), HttpRequest::canHandle1xx(), chunked(), ClientRequestContext::clientAccessCheck(), clientCheckPinning(), clientInterpretRequestHeaders(), clientPackRangeHdr(), HttpRequest::conditional(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), getByIdIfPresent(), Ftp::Server::handleRequest(), HttpStateData::haveParsedReplyHeaders(), HttpReply::hdrExpirationTime(), HttpStateData::httpBuildRequestHeader(), httpFixupAuthentication(), ACLHTTPHeaderData::match(), Ftp::PrintReply(), clientReplyContext::processConditional(), clientReplyContext::processExpired(), Http::One::Server::processParsedRequest(), removeConnectionHeaderEntries(), Ftp::Relay::sendCommand(), CacheManager::start(), varyEvaluateMatch(), Ftp::Server::writeCustomReply(), Ftp::Server::writeErrorReply(), Ftp::Server::writeForwardedReply(), and Ftp::Server::writeForwardedReplyAndCall().
◆ hasByNameListMember()
int HttpHeader::hasByNameListMember | ( | const char * | name, |
const char * | member, | ||
const char | separator | ||
) | const |
Definition at line 1686 of file HttpHeader.cc.
References assert, getByName(), and strListGetItem().
Referenced by Adaptation::Icap::Options::configure().
◆ hasListMember()
int HttpHeader::hasListMember | ( | Http::HdrType | id, |
const char * | member, | ||
const char | separator | ||
) | const |
Definition at line 1662 of file HttpHeader.cc.
References Http::any_registered_header(), assert, getStrOrList(), and strListGetItem().
Referenced by HttpStateData::blockSwitchingProtocols(), clientInterpretRequestHeaders(), Adaptation::Icap::Options::configure(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), and HttpStateData::haveParsedReplyHeaders().
◆ hasNamed() [1/2]
bool HttpHeader::hasNamed | ( | const char * | name, |
unsigned int | namelen, | ||
String * | value = nullptr |
||
) | const |
- Deprecated:
- use SBuf method instead.
Definition at line 883 of file HttpHeader.cc.
References assert, Http::BAD_HDR, SBuf::caseCmp(), getByIdIfPresent(), getEntry(), Http::HeaderLookupTable, HttpHeaderInitPos, HttpHeaderEntry::id, Http::HeaderTableRecord::id, SBuf::length(), Http::HeaderLookupTable_t::lookup(), HttpHeaderEntry::name, Http::OTHER, strListAdd(), String::termedBuf(), and HttpHeaderEntry::value.
◆ hasNamed() [2/2]
returns true iff a [possibly empty] named field is there when returning true, also sets the value
parameter (if it is not nil)
Definition at line 865 of file HttpHeader.cc.
References SBuf::length(), and SBuf::rawContent().
Referenced by getByName(), ACLHTTPHeaderData::match(), needUpdate(), and SlowlyParseQuotedField().
◆ Isolate()
|
staticprotected |
either finds the end of headers or returns false If the end was found: *parse_start points to the first character after the header delimiter *blk_start points to the first header character (i.e. old parse_start value) *blk_end points to the first header delimiter character (CR or LF in CR?LF). If block starts where it ends, then there are no fields in the header.
Definition at line 301 of file HttpHeader.cc.
References assert, and headersEnd().
Referenced by parse().
◆ needUpdate()
bool HttpHeader::needUpdate | ( | const HttpHeader * | fresh | ) | const |
- Returns
- whether calling update(fresh) would change our set of fields
Definition at line 244 of file HttpHeader.cc.
References entries, getByName(), hasNamed(), and skipUpdateHeader().
Referenced by HttpReply::recreateOnNotModified().
◆ operator=()
HttpHeader & HttpHeader::operator= | ( | const HttpHeader & | other | ) |
Definition at line 170 of file HttpHeader.cc.
References assert, clean(), conflictingContentLength_, len, owner, teUnsupported_, and update().
◆ packInto()
void HttpHeader::packInto | ( | Packable * | p, |
bool | mask_sensitive_info = false |
||
) | const |
Definition at line 539 of file HttpHeader.cc.
References Packable::append(), assert, Http::AUTHORIZATION, debugs, findEntry(), Http::FTP_ARGUMENTS, Http::FTP_COMMAND, getEntry(), HttpHeaderInitPos, HttpHeaderEntry::id, SBuf::length(), HttpHeaderEntry::name, HttpHeaderEntry::packInto(), Http::PROXY_AUTHORIZATION, and SBuf::rawContent().
Referenced by HttpStateData::buildRequestPrefix(), clientPackRangeHdr(), ErrorState::compileLegacyCode(), htcpClear(), htcpQuery(), htcpTstReply(), HttpRequest::pack(), HttpReply::packHeadersUsingFastPacker(), prepareLogWithRequestDetails(), and Http::Tunneler::writeRequest().
◆ parse() [1/2]
int HttpHeader::parse | ( | const char * | buf, |
size_t | buf_len, | ||
bool | atEnd, | ||
size_t & | hdr_sz, | ||
Http::ContentLengthInterpreter & | interpreter | ||
) |
Parses headers stored in a buffer.
- Returns
- 1 and sets hdr_sz on success
- 0 when needs more data
- -1 on error
Definition at line 323 of file HttpHeader.cc.
◆ parse() [2/2]
int HttpHeader::parse | ( | const char * | header_start, |
size_t | len, | ||
Http::ContentLengthInterpreter & | interpreter | ||
) |
Definition at line 349 of file HttpHeader.cc.
References addEntry(), assert, String::caseCmp(), Http::ContentLengthInterpreter::checkField(), clean(), Config, conflictingContentLength_, Http::CONTENT_LENGTH, DBG_IMPORTANT, debugs, delById(), getByIdIfPresent(), getStringPrefix(), Http::ContentLengthInterpreter::headerWideProblem, hoRequest, HttpHeaderStats, Http::ContentLengthInterpreter::needsSanitizing, SquidConfig::onoff, owner, HttpHeaderEntry::parse(), Http::ContentLengthInterpreter::prohibitedAndIgnored(), putInt64(), SquidConfig::relaxed_header_parser, Http::ContentLengthInterpreter::sawBad, Http::ContentLengthInterpreter::sawGood, teUnsupported_, Http::TRANSFER_ENCODING, and Http::ContentLengthInterpreter::value.
Referenced by Ssl::ErrorDetailFile::parse(), parse(), HtcpReplyData::parseHeader(), and HttpRequest::parseHeader().
◆ putAuth()
void HttpHeader::putAuth | ( | const char * | auth_scheme, |
const char * | realm | ||
) |
Definition at line 1004 of file HttpHeader.cc.
References assert, httpHeaderPutStrf(), and Http::WWW_AUTHENTICATE.
Referenced by Ftp::Gateway::ftpAuthRequired(), Ftp::Gateway::loginFailed(), and CacheManager::start().
◆ putCc()
void HttpHeader::putCc | ( | const HttpHdrCc & | cc | ) |
Definition at line 1011 of file HttpHeader.cc.
References addEntry(), MemBuf::buf, Http::CACHE_CONTROL, MemBuf::clean(), delById(), MemBuf::init(), and HttpHdrCc::packInto().
Referenced by HttpStateData::httpBuildRequestHeader(), Ftp::HttpReplyWrapper(), and MimeIcon::load().
◆ putContRange()
void HttpHeader::putContRange | ( | const HttpHdrContRange * | cr | ) |
Definition at line 1026 of file HttpHeader.cc.
References addEntry(), assert, MemBuf::buf, MemBuf::clean(), Http::CONTENT_RANGE, delById(), httpHdrContRangePackInto(), and MemBuf::init().
Referenced by httpHeaderAddContRange().
◆ putExt()
void HttpHeader::putExt | ( | const char * | name, |
const char * | value | ||
) |
Definition at line 1075 of file HttpHeader.cc.
References addEntry(), assert, debugs, and Http::OTHER.
Referenced by clientReplyContext::buildReplyHeader(), htcpTstReply(), CacheManager::PutCommonResponseHeaders(), and OptionsExtractor::visit().
◆ putInt()
void HttpHeader::putInt | ( | Http::HdrType | id, |
int | number | ||
) |
Definition at line 968 of file HttpHeader.cc.
References addEntry(), Http::any_registered_header(), assert, Http::ftInt, Http::HeaderLookupTable, and xitoa().
Referenced by clientReplyContext::buildReplyHeader(), htcpTstReply(), and Ftp::HttpReplyWrapper().
◆ putInt64()
void HttpHeader::putInt64 | ( | Http::HdrType | id, |
int64_t | number | ||
) |
Definition at line 977 of file HttpHeader.cc.
References addEntry(), Http::any_registered_header(), assert, Http::ftInt64, Http::HeaderLookupTable, and xint64toa().
Referenced by Http::Stream::buildRangeHeader(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), Ftp::HttpReplyWrapper(), parse(), HttpReply::redirect(), and HttpReply::setHeaders().
◆ putRange()
void HttpHeader::putRange | ( | const HttpHdrRange * | range | ) |
Definition at line 1042 of file HttpHeader.cc.
References addEntry(), assert, MemBuf::buf, MemBuf::clean(), delById(), MemBuf::init(), HttpHdrRange::packInto(), and Http::RANGE.
◆ putSc()
void HttpHeader::putSc | ( | HttpHdrSc * | sc | ) |
Definition at line 1058 of file HttpHeader.cc.
References addEntry(), assert, MemBuf::buf, MemBuf::clean(), delById(), MemBuf::init(), sc, and Http::SURROGATE_CONTROL.
◆ putStr()
void HttpHeader::putStr | ( | Http::HdrType | id, |
const char * | str | ||
) |
Definition at line 995 of file HttpHeader.cc.
References addEntry(), Http::any_registered_header(), assert, Http::ftStr, and Http::HeaderLookupTable.
Referenced by StoreEntry::adjustVary(), Ftp::Gateway::appendSuccessHeader(), ConnStateData::buildFakeRequest(), ErrorState::BuildHttpReply(), clientReplyContext::buildReplyHeader(), clientPackRangeHdr(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), Ftp::Relay::createHttpReply(), Adaptation::Icap::ModXact::encapsulateHead(), HttpStateData::forwardUpgrade(), Ftp::Server::handleFeatReply(), HttpStateData::httpBuildRequestHeader(), httpFixupAuthentication(), httpHeaderPutStrvf(), Ftp::HttpReplyWrapper(), peerDigestRequest(), HttpReply::redirect(), HttpReply::setHeaders(), UrnState::setUriResFromRequest(), Mgr::Inquirer::start(), and Http::One::Server::writeControlMsgAndCall().
◆ putTime()
void HttpHeader::putTime | ( | Http::HdrType | id, |
time_t | htime | ||
) |
Definition at line 986 of file HttpHeader.cc.
References addEntry(), Http::any_registered_header(), assert, Time::FormatRfc1123(), Http::ftDate_1123, and Http::HeaderLookupTable.
Referenced by clientReplyContext::buildReplyHeader(), htcpTstReply(), HttpStateData::httpBuildRequestHeader(), Ftp::HttpReplyWrapper(), HttpReply::redirect(), and HttpReply::setHeaders().
◆ refreshMask()
void HttpHeader::refreshMask | ( | ) |
Definition at line 722 of file HttpHeader.cc.
References CBIT_SET, debugs, entries, httpHeaderMaskInit(), and mask.
Referenced by clientReplyContext::buildReplyHeader(), Ftp::Server::handleFeatReply(), httpHdrMangleList(), and removeConnectionHeaderEntries().
◆ removeConnectionHeaderEntries()
|
protected |
- Deprecated:
- Public access replaced by removeHopByHopEntries()
Definition at line 1727 of file HttpHeader.cc.
References Http::CONNECTION, delAt(), getEntry(), getList(), has(), HttpHeaderInitPos, HttpHeaderEntry::name, refreshMask(), and strListIsMember().
Referenced by removeHopByHopEntries().
◆ removeHopByHopEntries()
void HttpHeader::removeHopByHopEntries | ( | ) |
Definition at line 1710 of file HttpHeader.cc.
References CBIT_CLR, delAt(), getEntry(), Http::HeaderLookupTable, HttpHeaderInitPos, HttpHeaderEntry::id, mask, and removeConnectionHeaderEntries().
Referenced by clientReplyContext::buildReplyHeader(), Adaptation::Icap::ModXact::encapsulateHead(), and Http::One::Server::writeControlMsgAndCall().
◆ skipUpdateHeader()
|
protected |
Definition at line 258 of file HttpHeader.cc.
References Http::VARY.
Referenced by needUpdate(), and update().
◆ unsupportedTe()
|
inline |
Definition at line 172 of file HttpHeader.h.
References teUnsupported_.
Referenced by HttpRequest::checkEntityFraming().
◆ update()
void HttpHeader::update | ( | const HttpHeader * | fresh | ) |
replaces fields with matching names and adds fresh fields with new names assuming fresh
is a 304 reply
Definition at line 267 of file HttpHeader.cc.
References addEntry(), assert, HttpHeaderEntry::clone(), debugs, delById(), delByName(), getEntry(), Http::HeaderLookupTable, HttpHeaderInitPos, HttpHeaderEntry::id, HttpHeaderEntry::name, Http::OTHER, and skipUpdateHeader().
Referenced by HttpHeader(), operator=(), and HttpReply::recreateOnNotModified().
◆ updateOrAddStr()
void HttpHeader::updateOrAddStr | ( | Http::HdrType | id, |
const SBuf & | newValue | ||
) |
Ensures that the header has the given field, removing or replacing any same-name fields with conflicting values as needed.
Definition at line 1083 of file HttpHeader.cc.
References addEntry(), Http::any_registered_header(), assert, Assure, SBuf::c_str(), CBIT_TEST, SBuf::cmp(), debugs, entries, Http::ftStr, Http::HeaderLookupTable, SBuf::length(), mask, SBuf::plength(), SBuf::rawContent(), and String::SizeMaxXXX().
Referenced by addVia(), ErrorState::BuildHttpReply(), Http::One::Server::buildHttpRequest(), and Ftp::Server::setDataCommand().
Member Data Documentation
◆ conflictingContentLength_
|
private |
found different Content-Length fields
Definition at line 194 of file HttpHeader.h.
Referenced by clean(), conflictingContentLength(), operator=(), and parse().
◆ entries
std::vector<HttpHeaderEntry*, PoolingAllocator<HttpHeaderEntry*> > HttpHeader::entries |
parsed fields in raw format
Definition at line 175 of file HttpHeader.h.
Referenced by addEntry(), append(), clean(), compact(), delAt(), findEntry(), findLastEntry(), getEntry(), getList(), HttpHeader(), needUpdate(), refreshMask(), and updateOrAddStr().
◆ len
int HttpHeader::len |
length when packed, not counting terminating null-byte
Definition at line 178 of file HttpHeader.h.
Referenced by addEntry(), clean(), delAt(), operator=(), peerDigestRequest(), HttpReply::prefixLen(), and HttpRequest::prefixLen().
◆ mask
HttpHeaderMask HttpHeader::mask |
bit set <=> entry present
Definition at line 176 of file HttpHeader.h.
Referenced by addEntry(), clean(), delById(), delByName(), findEntry(), findLastEntry(), getCc(), getList(), getSc(), has(), HttpHeader(), refreshMask(), removeHopByHopEntries(), and updateOrAddStr().
◆ owner
http_hdr_owner_type HttpHeader::owner |
request or reply
Definition at line 177 of file HttpHeader.h.
Referenced by clean(), getCc(), getSc(), HttpStateData::httpBuildRequestHeader(), HttpHeader(), operator=(), and parse().
◆ teUnsupported_
|
private |
unsupported encoding, unnecessary syntax characters, and/or invalid field-value found in Transfer-Encoding header
Definition at line 197 of file HttpHeader.h.
Referenced by clean(), operator=(), parse(), and unsupportedTe().
The documentation for this class was generated from the following files:
- src/HttpHeader.h
- src/HttpHeader.cc
- src/tests/stub_HttpHeader.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products