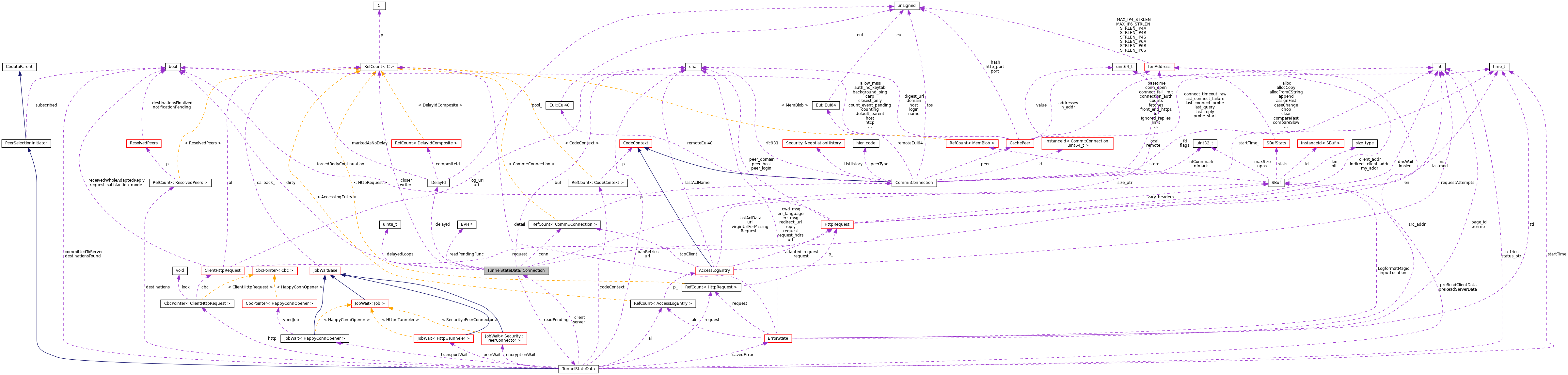
Public Member Functions | |
Connection () | |
~Connection () | |
template<typename Method > | |
void | initConnection (const Comm::ConnectionPointer &aConn, Method method, const char *name, TunnelStateData *tunnelState) |
initiates Comm::Connection ownership, including closure monitoring More... | |
void | noteClosure () |
reacts to the external closure of our connection More... | |
int | bytesWanted (int lower=0, int upper=INT_MAX) const |
void | bytesIn (int const &) |
void | setDelayId (DelayId const &) |
void | error (int const xerrno) |
int | debugLevelForError (int const xerrno) const |
void | dataSent (size_t amount) |
void | write (const char *b, int size, AsyncCall::Pointer &callback, FREE *free_func) |
writes 'b' buffer, setting the 'writer' member to 'callback'. More... | |
Public Attributes | |
int | len |
char * | buf |
AsyncCall::Pointer | writer |
pending Comm::Write callback More... | |
uint64_t * | size_ptr |
Comm::ConnectionPointer | conn |
The currently connected connection. More... | |
uint8_t | delayedLoops |
how many times a read on this connection has been postponed. More... | |
bool | dirty |
whether write() has been called (at least once) More... | |
TunnelStateData * | readPending |
EVH * | readPendingFunc |
DelayId | delayId |
Private Attributes | |
AsyncCall::Pointer | closer |
the registered close handler for the connection More... | |
Detailed Description
Constructor & Destructor Documentation
◆ Connection()
◆ ~Connection()
TunnelStateData::Connection::~Connection | ( | ) |
Definition at line 391 of file tunnel.cc.
References buf, eventDelete(), readPending, readPendingFunc, and safe_free.
Member Function Documentation
◆ bytesIn()
void TunnelStateData::Connection::bytesIn | ( | int const & | count | ) |
Definition at line 477 of file tunnel.cc.
References debugs.
Referenced by TunnelStateData::copyClientBytes(), TunnelStateData::copyServerBytes(), TunnelStateData::readClient(), and TunnelStateData::readServer().
◆ bytesWanted()
Definition at line 466 of file tunnel.cc.
Referenced by TunnelStateData::copyRead().
◆ dataSent()
void TunnelStateData::Connection::dataSent | ( | size_t | amount | ) |
Definition at line 724 of file tunnel.cc.
References assert, and debugs.
Referenced by TunnelStateData::writeClientDone(), and TunnelStateData::writeServerDone().
◆ debugLevelForError()
Definition at line 512 of file tunnel.cc.
References ignoreErrno().
◆ error()
void TunnelStateData::Connection::error | ( | int const | xerrno | ) |
Definition at line 564 of file tunnel.cc.
References conn, debugs, ignoreErrno(), and xstrerr().
Referenced by TunnelStateData::keepGoingAfterRead(), TunnelStateData::writeClientDone(), and TunnelStateData::writeServerDone().
◆ initConnection()
void TunnelStateData::Connection::initConnection | ( | const Comm::ConnectionPointer & | aConn, |
Method | method, | ||
const char * | name, | ||
TunnelStateData * | tunnelState | ||
) |
Definition at line 746 of file tunnel.cc.
References comm_add_close_handler(), commCbCall(), conn, Comm::IsConnOpen(), and Must.
Referenced by TunnelStateData::TunnelStateData(), and TunnelStateData::commitToServer().
◆ noteClosure()
void TunnelStateData::Connection::noteClosure | ( | ) |
Definition at line 757 of file tunnel.cc.
Referenced by TunnelStateData::clientClosed(), and TunnelStateData::serverClosed().
◆ setDelayId()
void TunnelStateData::Connection::setDelayId | ( | DelayId const & | newDelay | ) |
Definition at line 1461 of file tunnel.cc.
Referenced by TunnelStateData::connectDone(), switchToTunnel(), and tunnelStart().
◆ write()
void TunnelStateData::Connection::write | ( | const char * | b, |
int | size, | ||
AsyncCall::Pointer & | callback, | ||
FREE * | free_func | ||
) |
Definition at line 737 of file tunnel.cc.
References conn, size, and Comm::Write().
Referenced by TunnelStateData::copy(), and TunnelStateData::notePeerReadyToShovel().
Member Data Documentation
◆ buf
char* TunnelStateData::Connection::buf |
Definition at line 161 of file tunnel.cc.
Referenced by ~Connection(), TunnelStateData::copy(), TunnelStateData::copyClientBytes(), TunnelStateData::copyRead(), TunnelStateData::copyServerBytes(), and tunnelStartShoveling().
◆ closer
|
private |
◆ conn
Comm::ConnectionPointer TunnelStateData::Connection::conn |
Definition at line 165 of file tunnel.cc.
Referenced by TunnelStateData::TunnelStateData(), TunnelStateData::clientClosed(), TunnelStateData::closeConnections(), TunnelStateData::closePendingConnection(), TunnelStateData::copyRead(), TunnelStateData::getHost(), TunnelStateData::keepGoingAfterRead(), TunnelStateData::noConnections(), TunnelStateData::readClient(), TunnelStateData::readServer(), TunnelStateData::retryOrBail(), TunnelStateData::sendError(), tunnelErrorComplete(), tunnelStartShoveling(), TunnelStateData::writeClientDone(), and TunnelStateData::writeServerDone().
◆ delayedLoops
uint8_t TunnelStateData::Connection::delayedLoops |
Definition at line 166 of file tunnel.cc.
Referenced by TunnelStateData::copyRead(), TunnelStateData::readClient(), and TunnelStateData::readServer().
◆ delayId
DelayId TunnelStateData::Connection::delayId |
Definition at line 176 of file tunnel.cc.
Referenced by TunnelStateData::establishTunnelThruProxy().
◆ dirty
bool TunnelStateData::Connection::dirty |
Definition at line 168 of file tunnel.cc.
Referenced by TunnelStateData::notePeerReadyToShovel(), and TunnelStateData::retryOrBail().
◆ len
int TunnelStateData::Connection::len |
Definition at line 160 of file tunnel.cc.
Referenced by TunnelStateData::copyRead(), TunnelStateData::keepGoingAfterRead(), TunnelStateData::tunnelEstablishmentDone(), and tunnelStartShoveling().
◆ readPending
TunnelStateData* TunnelStateData::Connection::readPending |
Definition at line 171 of file tunnel.cc.
Referenced by ~Connection(), TunnelStateData::copyRead(), tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ readPendingFunc
EVH* TunnelStateData::Connection::readPendingFunc |
Definition at line 172 of file tunnel.cc.
Referenced by TunnelStateData::TunnelStateData(), ~Connection(), and TunnelStateData::copyRead().
◆ size_ptr
uint64_t* TunnelStateData::Connection::size_ptr |
Definition at line 163 of file tunnel.cc.
Referenced by TunnelStateData::TunnelStateData().
◆ writer
AsyncCall::Pointer TunnelStateData::Connection::writer |
Definition at line 162 of file tunnel.cc.
Referenced by TunnelStateData::clientClosed(), TunnelStateData::retryOrBail(), tunnelConnectedWriteDone(), TunnelStateData::WriteClientDone(), and TunnelStateData::WriteServerDone().
The documentation for this class was generated from the following file:
- src/tunnel.cc