#include <Allocator.h>
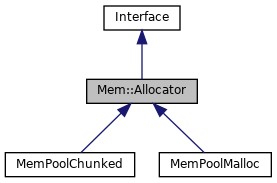
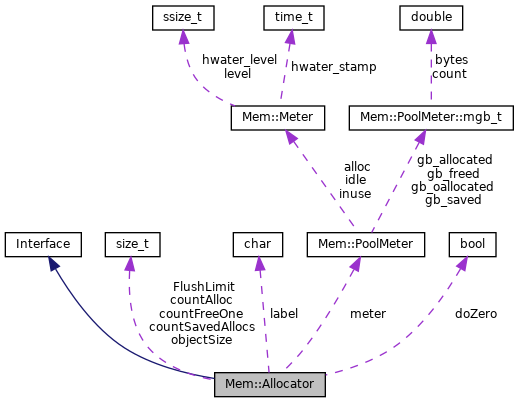
Public Member Functions | |
Allocator (const char *const aLabel, const size_t sz) | |
void | relabel (const char *const aLabel) |
virtual size_t | getStats (PoolStats &)=0 |
void * | alloc () |
provide (and reserve) memory suitable for storing one object More... | |
void | freeOne (void *obj) |
return memory reserved by alloc() More... | |
int | getInUseCount () const |
the difference between the number of alloc() and freeOne() calls More... | |
void | zeroBlocks (const bool doIt) |
virtual void | setChunkSize (size_t) |
XXX: Misplaced – not all allocators have a notion of a "chunk". See MemPoolChunked. More... | |
virtual bool | idleTrigger (int shift) const =0 |
virtual void | clean (time_t maxage)=0 |
void | flushCounters () |
Static Public Member Functions | |
static size_t | RoundedSize (const size_t minSize) |
Public Attributes | |
size_t | countAlloc = 0 |
the number of calls to Mem::Allocator::alloc() since last flush More... | |
size_t | countSavedAllocs = 0 |
the number of malloc()/calloc() calls avoided since last flush More... | |
size_t | countFreeOne = 0 |
the number of calls to Mem::Allocator::freeOne() since last flush More... | |
const char * | label |
brief description of objects returned by alloc() More... | |
const size_t | objectSize |
the size (in bytes) of objects managed by this allocator More... | |
PoolMeter | meter |
statistics tracked for this allocator More... | |
Static Public Attributes | |
static const size_t | FlushLimit = 1000 |
Flush counters to 'meter' after flush limit allocations. More... | |
Protected Member Functions | |
virtual void * | allocate ()=0 |
*alloc() More... | |
virtual void | deallocate (void *)=0 |
freeOne(void *) More... | |
Protected Attributes | |
bool | doZero = true |
Detailed Description
An interface for memory allocators that deal with fixed-size objects. Allocators may optimize repeated de/allocations using memory pools.
Definition at line 21 of file Allocator.h.
Constructor & Destructor Documentation
◆ Allocator()
|
inline |
Definition at line 27 of file Allocator.h.
Member Function Documentation
◆ alloc()
|
inline |
Definition at line 44 of file Allocator.h.
References allocate(), countAlloc, flushCounters(), and FlushLimit.
Referenced by Mem::AllocatorProxy::alloc(), authenticateDigestNonceNew(), cbdataInternalAlloc(), memAllocate(), squidaio_close(), squidaio_init(), squidaio_open(), squidaio_read(), squidaio_stat(), squidaio_unlink(), and squidaio_write().
◆ allocate()
|
protectedpure virtual |
◆ clean()
|
pure virtual |
Implemented in MemPoolMalloc, and MemPoolChunked.
◆ deallocate()
|
protectedpure virtual |
◆ flushCounters()
|
inline |
Flush temporary counter values into the statistics held in 'meter'.
Definition at line 74 of file Allocator.h.
References countAlloc, countFreeOne, countSavedAllocs, Mem::PoolMeter::gb_allocated, Mem::PoolMeter::gb_freed, Mem::PoolMeter::gb_saved, meter, objectSize, and Mem::PoolMeter::mgb_t::update().
Referenced by alloc(), MemPoolChunked::clean(), and MemPoolChunked::~MemPoolChunked().
◆ freeOne()
|
inline |
Definition at line 51 of file Allocator.h.
References assert, countFreeOne, deallocate(), objectSize, and VALGRIND_CHECK_MEM_IS_ADDRESSABLE.
Referenced by authenticateDigestNonceDelete(), memFree(), squidaio_cleanup_request(), and cbdata::~cbdata().
◆ getInUseCount()
|
inline |
Definition at line 59 of file Allocator.h.
References Mem::Meter::currentLevel(), Mem::PoolMeter::inuse, and meter.
Referenced by MemPoolChunked::getStats(), MemPoolMalloc::getStats(), StoreEntry::inUseCount(), memInUse(), MemPoolChunked::~MemPoolChunked(), and MemPoolMalloc::~MemPoolMalloc().
◆ getStats()
fill the given object with statistical data about pool
- Returns
- Number of objects in use, ie. allocated.
Implemented in MemPoolMalloc, and MemPoolChunked.
◆ idleTrigger()
|
pure virtual |
Implemented in MemPoolMalloc, and MemPoolChunked.
◆ relabel()
|
inline |
change the allocator description if we were only able to provide an approximate description at object construction time
Definition at line 34 of file Allocator.h.
References label.
◆ RoundedSize()
- Parameters
-
minSize Minimum size needed to be allocated.
- Return values
-
n Smallest size divisible by sizeof(void*)
Definition at line 93 of file Allocator.h.
◆ setChunkSize()
|
inlinevirtual |
Reimplemented in MemPoolChunked.
Definition at line 65 of file Allocator.h.
Referenced by Mem::Init().
◆ zeroBlocks()
|
inline |
- See also
- doZero
Definition at line 62 of file Allocator.h.
References doZero.
Referenced by Mem::AllocatorProxy::getAllocator(), and memDataInit().
Member Data Documentation
◆ countAlloc
size_t Mem::Allocator::countAlloc = 0 |
Definition at line 98 of file Allocator.h.
Referenced by alloc(), and flushCounters().
◆ countFreeOne
size_t Mem::Allocator::countFreeOne = 0 |
Definition at line 104 of file Allocator.h.
Referenced by flushCounters(), and freeOne().
◆ countSavedAllocs
size_t Mem::Allocator::countSavedAllocs = 0 |
Definition at line 101 of file Allocator.h.
Referenced by MemPoolMalloc::allocate(), flushCounters(), and MemPoolChunked::get().
◆ doZero
|
protected |
Whether to zero memory on initial allocation and on return to the pool.
We do this on some pools because many object constructors are/were incomplete and we are afraid some code may use the object after free. When possible, set this to false to avoid zeroing overheads.
Definition at line 130 of file Allocator.h.
Referenced by MemPoolMalloc::allocate(), MemPoolMalloc::deallocate(), MemChunk::MemChunk(), MemPoolChunked::push(), and zeroBlocks().
◆ FlushLimit
|
static |
Definition at line 25 of file Allocator.h.
Referenced by alloc().
◆ label
const char* Mem::Allocator::label |
Definition at line 109 of file Allocator.h.
Referenced by MemPoolChunked::getStats(), MemPoolMalloc::getStats(), and relabel().
◆ meter
PoolMeter Mem::Allocator::meter |
Definition at line 115 of file Allocator.h.
Referenced by MemPoolChunked::allocate(), MemPoolMalloc::allocate(), MemPoolMalloc::clean(), MemPoolChunked::deallocate(), MemPoolMalloc::deallocate(), flushCounters(), getInUseCount(), MemPoolChunked::getStats(), MemPoolMalloc::getStats(), MemPoolChunked::idleTrigger(), MemChunk::MemChunk(), Mem::Report(), and MemChunk::~MemChunk().
◆ objectSize
const size_t Mem::Allocator::objectSize |
Definition at line 112 of file Allocator.h.
Referenced by MemPoolMalloc::allocate(), MemPoolMalloc::deallocate(), flushCounters(), freeOne(), MemPoolChunked::get(), MemPoolChunked::getStats(), MemPoolMalloc::getStats(), MemChunk::MemChunk(), MemPoolChunked::push(), and MemPoolChunked::setChunkSize().
The documentation for this class was generated from the following file:
- src/mem/Allocator.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products