#include "squid.h"
#include "auth/CredentialsCache.h"
#include "auth/digest/Config.h"
#include "auth/digest/Scheme.h"
#include "auth/digest/User.h"
#include "auth/digest/UserRequest.h"
#include "auth/Gadgets.h"
#include "auth/State.h"
#include "auth/toUtf.h"
#include "base/LookupTable.h"
#include "base/Random.h"
#include "cache_cf.h"
#include "event.h"
#include "helper.h"
#include "HttpHeaderTools.h"
#include "HttpReply.h"
#include "HttpRequest.h"
#include "md5.h"
#include "mgr/Registration.h"
#include "rfc2617.h"
#include "sbuf/SBuf.h"
#include "sbuf/StringConvert.h"
#include "Store.h"
#include "StrList.h"
#include "wordlist.h"
#include "mem/Allocator.h"
#include "mem/Pool.h"
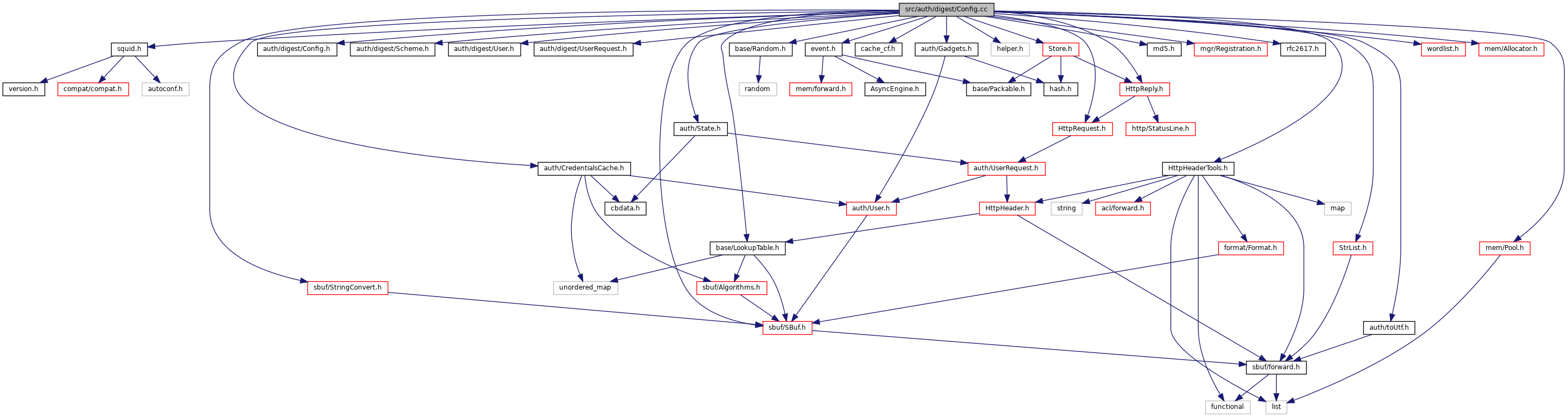
Go to the source code of this file.
Enumerations | |
enum | http_digest_attr_type { DIGEST_USERNAME, DIGEST_REALM, DIGEST_QOP, DIGEST_ALGORITHM, DIGEST_URI, DIGEST_NONCE, DIGEST_NC, DIGEST_CNONCE, DIGEST_RESPONSE, DIGEST_INVALID_ATTR } |
Functions | |
static const auto & | digestFieldsLookupTable () |
static void | authenticateDigestNonceCacheCleanup (void *data) |
static digest_nonce_h * | authenticateDigestNonceFindNonce (const char *noncehex) |
static void | authenticateDigestNonceDelete (digest_nonce_h *nonce) |
static void | authenticateDigestNonceSetup (void) |
static void | authDigestNonceEncode (digest_nonce_h *nonce) |
static void | authDigestNonceLink (digest_nonce_h *nonce) |
static void | authDigestNonceUserUnlink (digest_nonce_h *nonce) |
digest_nonce_h * | authenticateDigestNonceNew (void) |
void | authenticateDigestNonceShutdown (void) |
void | authDigestNonceUnlink (digest_nonce_h *nonce) |
const char * | authenticateDigestNonceNonceHex (const digest_nonce_h *nonce) |
int | authDigestNonceIsValid (digest_nonce_h *nonce, char nc[9]) |
int | authDigestNonceIsStale (digest_nonce_h *nonce) |
int | authDigestNonceLastRequest (digest_nonce_h *nonce) |
void | authDigestNoncePurge (digest_nonce_h *nonce) |
static void | authenticateDigestStats (StoreEntry *sentry) |
void | authDigestUserLinkNonce (Auth::Digest::User *user, digest_nonce_h *nonce) |
static Auth::UserRequest::Pointer | authDigestLogUsername (char *username, Auth::UserRequest::Pointer auth_user_request, const char *requestRealm) |
Variables | |
static AUTHSSTATS | authenticateDigestStats |
Helper::ClientPointer | digestauthenticators |
static hash_table * | digest_nonce_cache |
static int | authdigest_initialised = 0 |
static Mem::Allocator * | digest_nonce_pool = nullptr |
Enumeration Type Documentation
◆ http_digest_attr_type
Function Documentation
◆ authDigestLogUsername()
|
static |
Definition at line 686 of file Config.cc.
References assert, Auth::AUTH_BROKEN, Config, debugs, Auth::SchemeConfig::Find(), and Auth::UserRequest::user().
◆ authDigestNonceEncode()
|
static |
Definition at line 103 of file Config.cc.
References CvtHex(), H(), SquidMD5Final(), SquidMD5Init(), SquidMD5Update(), xcalloc(), and xfree.
Referenced by authenticateDigestNonceNew().
◆ authDigestNonceIsStale()
int authDigestNonceIsStale | ( | digest_nonce_h * | nonce | ) |
Definition at line 365 of file Config.cc.
References Config, current_time, debugs, and Auth::SchemeConfig::Find().
Referenced by authDigestNonceIsValid(), and authenticateDigestNonceCacheCleanup().
◆ authDigestNonceIsValid()
int authDigestNonceIsValid | ( | digest_nonce_h * | nonce, |
char | nc[9] | ||
) |
Definition at line 327 of file Config.cc.
References authDigestNonceIsStale(), Config, debugs, and Auth::SchemeConfig::Find().
◆ authDigestNonceLastRequest()
int authDigestNonceLastRequest | ( | digest_nonce_h * | nonce | ) |
- Return values
-
0 the digest is not stale yet -1 the digest will be stale on the next request
Definition at line 408 of file Config.cc.
References Config, debugs, and Auth::SchemeConfig::Find().
◆ authDigestNonceLink()
|
static |
Definition at line 272 of file Config.cc.
References assert, and debugs.
Referenced by authDigestUserLinkNonce(), and authenticateDigestNonceNew().
◆ authDigestNoncePurge()
void authDigestNoncePurge | ( | digest_nonce_h * | nonce | ) |
Definition at line 428 of file Config.cc.
References authDigestNonceUnlink(), digest_nonce_cache, and hash_remove_link().
Referenced by authenticateDigestNonceCacheCleanup(), and authenticateDigestNonceShutdown().
◆ authDigestNonceUnlink()
void authDigestNonceUnlink | ( | digest_nonce_h * | nonce | ) |
Definition at line 281 of file Config.cc.
References assert, authenticateDigestNonceDelete(), DBG_IMPORTANT, and debugs.
Referenced by authDigestNoncePurge(), and authDigestNonceUserUnlink().
◆ authDigestNonceUserUnlink()
|
static |
Definition at line 614 of file Config.cc.
References authDigestNonceUnlink(), dlink_node::data, dlinkDelete(), and dlink_node::next.
Referenced by authenticateDigestNonceCacheCleanup().
◆ authDigestUserLinkNonce()
void authDigestUserLinkNonce | ( | Auth::Digest::User * | user, |
digest_nonce_h * | nonce | ||
) |
Definition at line 652 of file Config.cc.
References assert, authDigestNonceLink(), dlinkAddTail(), and node::next.
◆ authenticateDigestNonceCacheCleanup()
|
static |
Definition at line 237 of file Config.cc.
References assert, authDigestNonceIsStale(), authDigestNoncePurge(), authDigestNonceUserUnlink(), Config, current_time, debugs, digest_nonce_cache, eventAdd(), Auth::SchemeConfig::Find(), hash_first(), and hash_next().
Referenced by authenticateDigestNonceSetup().
◆ authenticateDigestNonceDelete()
|
static |
Definition at line 190 of file Config.cc.
References assert, digest_nonce_pool, Mem::Allocator::freeOne(), and safe_free.
Referenced by authDigestNonceUnlink().
◆ authenticateDigestNonceFindNonce()
|
static |
Definition at line 307 of file Config.cc.
References authenticateDigestNonceNonceHex(), debugs, digest_nonce_cache, and hash_lookup().
Referenced by authenticateDigestNonceNew().
◆ authenticateDigestNonceNew()
digest_nonce_h* authenticateDigestNonceNew | ( | void | ) |
Definition at line 122 of file Config.cc.
References Mem::Allocator::alloc(), authDigestNonceEncode(), authDigestNonceLink(), authenticateDigestNonceFindNonce(), current_time, debugs, digest_nonce_cache, digest_nonce_pool, hash_join(), and RandomSeed32().
◆ authenticateDigestNonceNonceHex()
const char* authenticateDigestNonceNonceHex | ( | const digest_nonce_h * | nonce | ) |
Definition at line 298 of file Config.cc.
Referenced by authenticateDigestNonceFindNonce().
◆ authenticateDigestNonceSetup()
|
static |
Definition at line 203 of file Config.cc.
References assert, authenticateDigestNonceCacheCleanup(), Config, digest_nonce_cache, digest_nonce_pool, eventAdd(), Auth::SchemeConfig::Find(), hash_create(), hash_string, and memPoolCreate.
◆ authenticateDigestNonceShutdown()
void authenticateDigestNonceShutdown | ( | void | ) |
Definition at line 216 of file Config.cc.
References assert, authDigestNoncePurge(), debugs, digest_nonce_cache, hash_first(), and hash_next().
◆ authenticateDigestStats()
|
static |
Definition at line 605 of file Config.cc.
References digestauthenticators.
◆ digestFieldsLookupTable()
|
static |
Definition at line 70 of file Config.cc.
References DIGEST_ALGORITHM, DIGEST_CNONCE, DIGEST_INVALID_ATTR, DIGEST_NC, DIGEST_NONCE, DIGEST_QOP, DIGEST_REALM, DIGEST_RESPONSE, DIGEST_URI, DIGEST_USERNAME, and base64_decode_ctx::table.
Variable Documentation
◆ authdigest_initialised
◆ authenticateDigestStats
|
static |
◆ digest_nonce_cache
|
static |
Definition at line 51 of file Config.cc.
Referenced by authDigestNoncePurge(), authenticateDigestNonceCacheCleanup(), authenticateDigestNonceFindNonce(), authenticateDigestNonceNew(), authenticateDigestNonceSetup(), and authenticateDigestNonceShutdown().
◆ digest_nonce_pool
|
static |
Definition at line 54 of file Config.cc.
Referenced by authenticateDigestNonceDelete(), authenticateDigestNonceNew(), and authenticateDigestNonceSetup().
◆ digestauthenticators
Helper::ClientPointer digestauthenticators |
Definition at line 49 of file Config.cc.
Referenced by authenticateDigestStats().
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products