#include <RockIoState.h>
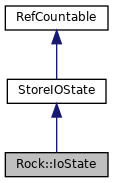
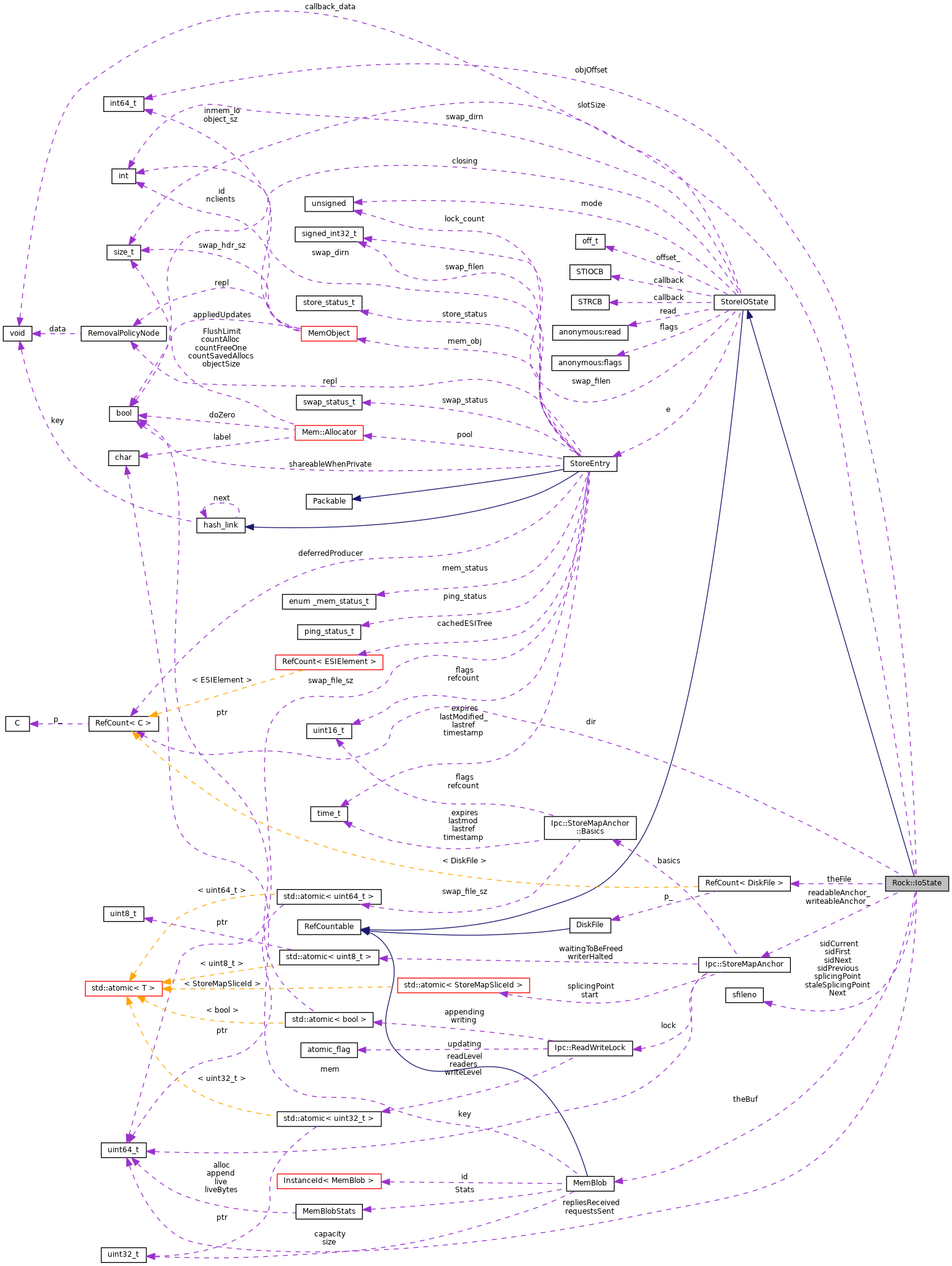
Public Types | |
typedef RefCount< IoState > | Pointer |
enum | CloseHow { wroteAll, writerGone, readerDone } |
typedef void | STRCB(void *their_data, const char *buf, ssize_t len, StoreIOState::Pointer self) |
typedef void | STIOCB(void *their_data, int errflag, StoreIOState::Pointer self) |
Public Member Functions | |
IoState (Rock::SwapDir::Pointer &, StoreEntry *, StoreIOState::STIOCB *, void *cbData) | |
~IoState () override | |
void | file (const RefCount< DiskFile > &aFile) |
void | read_ (char *buf, size_t size, off_t offset, STRCB *callback, void *callback_data) override |
bool | write (char const *buf, size_t size, off_t offset, FREE *free_func) override |
wraps tryWrite() to handle deep write failures centrally and safely More... | |
void | close (int how) override |
finish or abort swapping per CloseHow More... | |
bool | stillWaiting () const |
whether we are still waiting for the I/O results (i.e., not closed) More... | |
void | handleReadCompletion (Rock::ReadRequest &request, const int rlen, const int errFlag) |
forwards read data (or an error) to the reader that initiated this I/O More... | |
void | finishedWriting (const int errFlag) |
called by SwapDir::writeCompleted() after the last write and on error More... | |
bool | expectedReply (const IoXactionId receivedId) |
void * | operator new (size_t amount) |
void | operator delete (void *address) |
off_t | offset () const |
bool | touchingStoreEntry () const |
Public Attributes | |
const Ipc::StoreMapAnchor * | readableAnchor_ |
starting point for reading More... | |
Ipc::StoreMapAnchor * | writeableAnchor_ |
starting point for writing More... | |
SlotId | splicingPoint |
the last db slot successfully read or written More... | |
SlotId | staleSplicingPointNext |
sdirno | swap_dirn |
sfileno | swap_filen |
StoreEntry * | e |
mode_t | mode |
off_t | offset_ |
number of bytes written or read for this entry so far More... | |
STIOCB * | callback |
void * | callback_data |
struct { | |
STRCB * callback | |
void * callback_data | |
} | read |
struct { | |
bool closing | |
} | flags |
Private Member Functions | |
MEMPROXY_CLASS (IoState) | |
const Ipc::StoreMapAnchor & | readAnchor () const |
Ipc::StoreMapAnchor & | writeAnchor () |
const Ipc::StoreMapSlice & | currentReadableSlice () const |
convenience wrapper returning the map slot we are reading now More... | |
void | tryWrite (char const *buf, size_t size, off_t offset) |
size_t | writeToBuffer (char const *buf, size_t size) |
void | writeToDisk () |
write what was buffered during write() calls More... | |
void | callReaderBack (const char *buf, int rlen) |
report (already sanitized/checked) I/O results to the read initiator More... | |
void | callBack (int errflag) |
Private Attributes | |
Rock::SwapDir::Pointer | dir |
swap dir that initiated I/O More... | |
const size_t | slotSize |
db cell size More... | |
int64_t | objOffset |
object offset for current db slot More... | |
SlotId | sidFirst |
SlotId | sidPrevious |
SlotId | sidCurrent |
SlotId | sidNext |
uint64_t | requestsSent |
the number of read or write requests we sent to theFile More... | |
uint64_t | repliesReceived |
the number of successful responses we received from theFile More... | |
RefCount< DiskFile > | theFile |
MemBlob | theBuf |
Detailed Description
Definition at line 25 of file RockIoState.h.
Member Typedef Documentation
◆ Pointer
typedef RefCount<IoState> Rock::IoState::Pointer |
Definition at line 30 of file RockIoState.h.
◆ STIOCB
|
inherited |
Definition at line 39 of file StoreIOState.h.
◆ STRCB
|
inherited |
Definition at line 29 of file StoreIOState.h.
Member Enumeration Documentation
◆ CloseHow
|
inherited |
Enumerator | |
---|---|
wroteAll | success: caller supplied all data it wanted to swap out |
writerGone | failure: caller left before swapping out everything |
readerDone | success or failure: either way, stop swapping in |
Definition at line 57 of file StoreIOState.h.
Constructor & Destructor Documentation
◆ IoState()
Rock::IoState::IoState | ( | Rock::SwapDir::Pointer & | aDir, |
StoreEntry * | anEntry, | ||
StoreIOState::STIOCB * | cbIo, | ||
void * | cbData | ||
) |
Definition at line 25 of file RockIoState.cc.
References StoreIOState::e, StoreEntry::lock(), and store_open_disk_fd.
◆ ~IoState()
|
override |
Definition at line 52 of file RockIoState.cc.
References assert, cbdataReferenceDone, shutting_down, and store_open_disk_fd.
Member Function Documentation
◆ callBack()
|
private |
Definition at line 462 of file RockIoState.cc.
References asyncCall(), cbdataReferenceDone, debugs, and ScheduleCallHere.
◆ callReaderBack()
|
private |
Definition at line 167 of file RockIoState.cc.
References assert, and cbdataReferenceValidDone.
◆ close()
|
overridevirtual |
Implements StoreIOState.
Definition at line 368 of file RockIoState.cc.
References assert, CurrentException(), debugs, and DISK_ERROR.
◆ currentReadableSlice()
|
private |
Definition at line 91 of file RockIoState.cc.
◆ expectedReply()
bool Rock::IoState::expectedReply | ( | const IoXactionId | receivedId | ) |
notes that the disker has satisfied the given I/O request
- Returns
- whether all earlier I/O requests have been satisfied already
Definition at line 333 of file RockIoState.cc.
Referenced by Rock::SwapDir::writeCompleted().
◆ file()
Definition at line 68 of file RockIoState.cc.
References assert.
◆ finishedWriting()
void Rock::IoState::finishedWriting | ( | const int | errFlag | ) |
Definition at line 349 of file RockIoState.cc.
References CollapsedForwarding::Broadcast().
◆ handleReadCompletion()
void Rock::IoState::handleReadCompletion | ( | Rock::ReadRequest & | request, |
const int | rlen, | ||
const int | errFlag | ||
) |
Definition at line 150 of file RockIoState.cc.
References ReadRequest::buf, debugs, DISK_OK, and Rock::ReadRequest::id.
Referenced by Rock::SwapDir::readCompleted().
◆ MEMPROXY_CLASS()
|
private |
◆ offset()
|
inlineinherited |
Definition at line 48 of file StoreIOState.h.
References StoreIOState::offset_.
Referenced by store_client::fileRead(), MemObject::objectBytesOnDisk(), MemObject::stat(), and StoreEntry::swapOut().
◆ operator delete()
|
inherited |
Definition at line 25 of file StoreIOState.cc.
References assert.
◆ operator new()
|
inherited |
Definition at line 18 of file StoreIOState.cc.
References assert.
◆ read_()
|
overridevirtual |
Implements StoreIOState.
Definition at line 97 of file RockIoState.cc.
References assert, cbdataReference, debugs, min(), and size.
◆ readAnchor()
|
private |
Definition at line 76 of file RockIoState.cc.
References assert.
◆ stillWaiting()
|
inline |
Definition at line 43 of file RockIoState.h.
References theFile.
Referenced by Rock::SwapDir::writeCompleted().
◆ touchingStoreEntry()
|
inherited |
Definition at line 52 of file StoreIOState.cc.
References StoreIOState::e, StoreIOState::swap_filen, and StoreEntry::swap_filen.
Referenced by Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆ tryWrite()
|
private |
Possibly send data to be written to disk: We only write data when full slot is accumulated or when close() is called. We buffer, in part, to avoid forcing OS to read old unwritten portions of the slot when the write does not end at the page or sector boundary.
Definition at line 212 of file RockIoState.cc.
◆ write()
|
overridevirtual |
Implements StoreIOState.
Definition at line 184 of file RockIoState.cc.
References debugs, DISK_ERROR, and size.
◆ writeAnchor()
|
private |
Definition at line 83 of file RockIoState.cc.
References assert.
◆ writeToBuffer()
Buffers incoming data for the current slot.
- Returns
- the number of bytes buffered
Definition at line 247 of file RockIoState.cc.
◆ writeToDisk()
|
private |
Definition at line 266 of file RockIoState.cc.
References assert, debugs, Rock::DbCellHeader::entrySize, Rock::WriteRequest::eof, Rock::DbCellHeader::firstSlot, Rock::DbCellHeader::key, memAllocBuf(), memFreeBufFunc(), Rock::DbCellHeader::nextSlot, Rock::DbCellHeader::payloadSize, Rock::WriteRequest::sidCurrent, Rock::WriteRequest::sidPrevious, and Rock::DbCellHeader::version.
Member Data Documentation
◆ callback [1/2]
|
inherited |
Definition at line 76 of file StoreIOState.h.
◆ callback [2/2]
|
inherited |
Definition at line 80 of file StoreIOState.h.
◆ callback_data
|
inherited |
Definition at line 77 of file StoreIOState.h.
Referenced by StoreIOState::~StoreIOState().
◆ closing
|
inherited |
Definition at line 85 of file StoreIOState.h.
Referenced by storeClose().
◆ dir
|
private |
Definition at line 77 of file RockIoState.h.
◆ e
|
inherited |
Definition at line 73 of file StoreIOState.h.
Referenced by IoState(), StoreIOState::touchingStoreEntry(), Fs::Ufs::UFSStoreState::UFSStoreState(), Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆ flags
struct { ... } StoreIOState::flags |
Referenced by storeClose(), and StoreIOState::StoreIOState().
◆ mode
|
inherited |
Definition at line 74 of file StoreIOState.h.
Referenced by Fs::Ufs::UFSStrategy::create(), Fs::Ufs::UFSStoreState::ioCompletedNotification(), and Fs::Ufs::UFSStrategy::open().
◆ objOffset
|
private |
Definition at line 79 of file RockIoState.h.
◆ offset_
|
inherited |
Definition at line 75 of file StoreIOState.h.
Referenced by StoreIOState::offset().
◆ read
struct { ... } StoreIOState::read |
Referenced by StoreIOState::StoreIOState(), and StoreIOState::~StoreIOState().
◆ readableAnchor_
const Ipc::StoreMapAnchor* Rock::IoState::readableAnchor_ |
Definition at line 56 of file RockIoState.h.
◆ repliesReceived
|
private |
Definition at line 101 of file RockIoState.h.
◆ requestsSent
|
private |
Definition at line 98 of file RockIoState.h.
◆ sidCurrent
|
private |
For readers, the db slot currently being read from disk. For writers, the reserved db slot currently being filled (to be written).
Definition at line 91 of file RockIoState.h.
◆ sidFirst
|
private |
The very first entry slot. Usually the same as anchor.first, but writers set anchor.first only after the first write is done.
Definition at line 83 of file RockIoState.h.
◆ sidNext
|
private |
Unused by readers. For writers, the reserved db slot that sidCurrent.next will point to.
Definition at line 95 of file RockIoState.h.
◆ sidPrevious
|
private |
Unused by readers. For writers, the slot pointing (via .next) to sidCurrent.
Definition at line 87 of file RockIoState.h.
◆ slotSize
|
private |
Definition at line 78 of file RockIoState.h.
◆ splicingPoint
SlotId Rock::IoState::splicingPoint |
Definition at line 60 of file RockIoState.h.
Referenced by Rock::HeaderUpdater::noteDoneWriting(), and Rock::HeaderUpdater::stopReading().
◆ staleSplicingPointNext
SlotId Rock::IoState::staleSplicingPointNext |
when reading, this is the next slot we are going to read (if asked) when writing, this is the next slot to use after the last fresh slot
Definition at line 63 of file RockIoState.h.
Referenced by Rock::HeaderUpdater::startWriting(), and Rock::HeaderUpdater::stopReading().
◆ swap_dirn
|
inherited |
Definition at line 71 of file StoreIOState.h.
Referenced by Fs::Ufs::UFSStoreState::ioCompletedNotification(), storeSwapOutStart(), and Fs::Ufs::UFSStoreState::UFSStoreState().
◆ swap_filen
|
inherited |
Definition at line 72 of file StoreIOState.h.
Referenced by Fs::Ufs::UFSStrategy::create(), Fs::Ufs::UFSStoreState::ioCompletedNotification(), storeSwapOutStart(), StoreIOState::touchingStoreEntry(), Fs::Ufs::UFSStoreState::UFSStoreState(), Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆ theBuf
|
private |
Definition at line 104 of file RockIoState.h.
◆ theFile
Definition at line 103 of file RockIoState.h.
Referenced by stillWaiting().
◆ writeableAnchor_
Ipc::StoreMapAnchor* Rock::IoState::writeableAnchor_ |
Definition at line 57 of file RockIoState.h.
Referenced by Rock::SwapDir::disconnect(), Rock::SwapDir::finalizeSwapoutSuccess(), Rock::HeaderUpdater::noteDoneWriting(), and Rock::HeaderUpdater::swanSong().
The documentation for this class was generated from the following files:
- src/fs/rock/RockIoState.h
- src/fs/rock/RockIoState.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products