#include <helper.h>
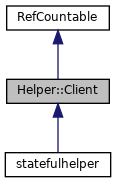
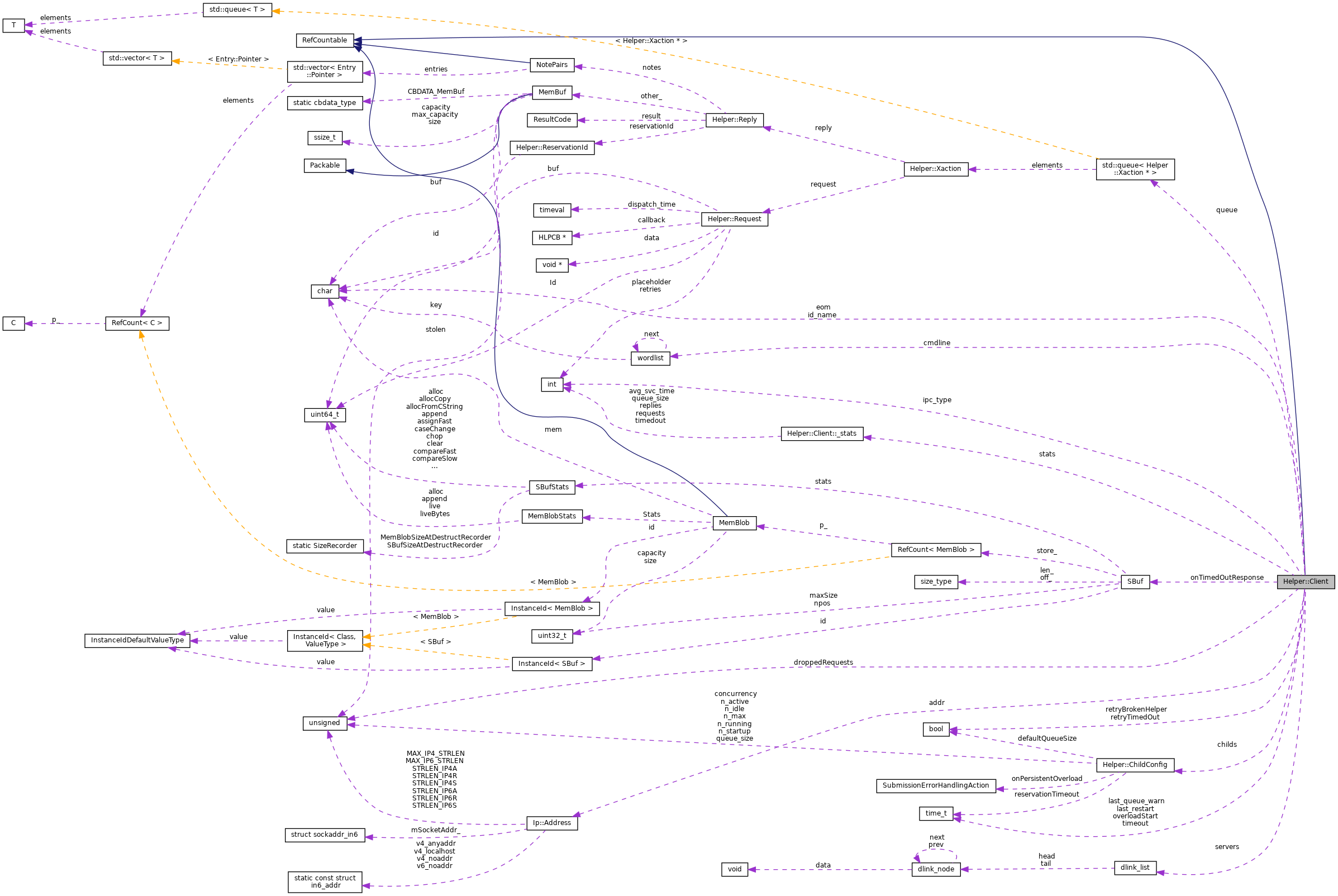
Classes | |
struct | _stats |
Public Types | |
using | Pointer = RefCount< Client > |
Public Member Functions | |
virtual | ~Client () |
Xaction * | nextRequest () |
bool | trySubmit (const char *buf, HLPCB *callback, void *data) |
If possible, submit request. Otherwise, either kill Squid or return false. More... | |
void | submitRequest (Xaction *) |
void | packStatsInto (Packable *p, const char *label=nullptr) const |
Dump some stats about the helper state to a Packable object. More... | |
bool | willOverload () const |
void | handleKilledServer (SessionBase *) |
void | handleFewerServers (bool madeProgress) |
void | dropQueued () |
void | callBack (Xaction &) |
sends transaction response to the transaction initiator More... | |
virtual void | openSessions () |
Static Public Member Functions | |
static Pointer | Make (const char *name) |
Public Attributes | |
wordlist * | cmdline = nullptr |
dlink_list | servers |
std::queue< Xaction * > | queue |
const char * | id_name = nullptr |
ChildConfig | childs |
Configuration settings for number running. More... | |
int | ipc_type = 0 |
Ip::Address | addr |
unsigned int | droppedRequests = 0 |
requests not sent during helper overload More... | |
time_t | overloadStart = 0 |
when the helper became overloaded (zero if it is not) More... | |
time_t | last_queue_warn = 0 |
time_t | last_restart = 0 |
time_t | timeout = 0 |
Requests timeout. More... | |
bool | retryTimedOut = false |
Whether the timed-out requests must retried. More... | |
bool | retryBrokenHelper = false |
Whether the requests must retried on BH replies. More... | |
SBuf | onTimedOutResponse |
The response to use when helper response timedout. More... | |
char | eom = '\n' |
The char which marks the end of (response) message, normally ' '. More... | |
struct Helper::Client::_stats | stats |
Protected Member Functions | |
Client (const char *const name) | |
bool | queueFull () const |
whether queuing an additional request would overload the helper More... | |
bool | overloaded () const |
void | syncQueueStats () |
synchronizes queue-dependent measurements with the current queue state More... | |
bool | prepSubmit () |
void | submit (const char *buf, HLPCB *callback, void *data) |
dispatches or enqueues a helper requests; does not enforce queue limits More... | |
Detailed Description
Managers a set of individual helper processes with a common queue of requests.
With respect to load, a helper goes through these states (roughly): idle: no processes are working on requests (and no requests are queued); normal: some, but not all processes are working (and no requests are queued); busy: all processes are working (and some requests are possibly queued); overloaded: a busy helper with more than queue-size requests in the queue.
A busy helper queues new requests and issues a WARNING every 10 minutes or so. An overloaded helper either drops new requests or keeps queuing them, depending on whether the caller can handle dropped requests (trySubmit vs helperSubmit APIs). If an overloaded helper has been overloaded for 3+ minutes, an attempt to use it results in on-persistent-overload action, which may kill worker.
Member Typedef Documentation
◆ Pointer
using Helper::Client::Pointer = RefCount<Client> |
Constructor & Destructor Documentation
◆ ~Client()
◆ Client()
|
inlineexplicitprotected |
Member Function Documentation
◆ callBack()
void Client::callBack | ( | Xaction & | r | ) |
Definition at line 572 of file helper.cc.
References Assure, Helper::Request::callback, cbdataReferenceValidDone, Helper::Request::data, Helper::Xaction::reply, and Helper::Xaction::request.
Referenced by helperReturnBuffer().
◆ dropQueued()
void Client::dropQueued | ( | ) |
satisfies all queued requests with a Helper::Unknown answer \prec no existing servers will be able to process queued requests
- See also
- SessionBase::dropQueued()
Definition at line 887 of file helper.cc.
References Assure, DBG_CRITICAL, debugs, GetFirstAvailable(), and Helper::Unknown.
Referenced by helperKickQueue(), helperShutdown(), and helperStatefulKickQueue().
◆ handleFewerServers()
void Client::handleFewerServers | ( | bool | madeProgress | ) |
Reacts to unexpected helper process death(s), including a failure to start helper(s) and an unexpected exit of a previously started helper.
- See also
- handleKilledServer()
- Parameters
-
madeProgress whether the died helper(s) responded to any requests
Definition at line 908 of file helper.cc.
References DBG_CRITICAL, DBG_IMPORTANT, debugs, Debug::Extra(), fatalf(), and squid_curtime.
◆ handleKilledServer()
void Client::handleKilledServer | ( | SessionBase * | srv | ) |
Updates internal statistics and starts new helper processes after an unexpected server exit
Definition at line 867 of file helper.cc.
References assert, DBG_CRITICAL, debugs, Helper::SessionBase::flags, Helper::SessionBase::index, Helper::SessionBase::replies, Helper::SessionBase::_helper_flags::shutdown, and Helper::SessionBase::stats.
Referenced by Helper::SessionBase::HelperServerClosed().
◆ Make()
|
static |
- Returns
- a newly created instance of the named helper client
- Parameters
-
name admin-visible helper category (with this process lifetime)
Definition at line 757 of file helper.cc.
References HappyOrderEnforcer::name.
Referenced by externalAclInit(), Ssl::Helper::Init(), Ssl::CertValidationHelper::Init(), and redirectInit().
◆ nextRequest()
Helper::Xaction * Client::nextRequest | ( | ) |
- Returns
- next request in the queue, or nil.
Definition at line 1302 of file helper.cc.
Referenced by helperKickQueue(), and helperStatefulKickQueue().
◆ openSessions()
|
virtual |
Starts required helper process(es). The caller is responsible for checking that new processes are needed.
Reimplemented in statefulhelper.
Definition at line 188 of file helper.cc.
References assert, asyncCall(), cbdataDialer(), comm_add_close_handler(), comm_read(), commCbCall(), commSetConnTimeout(), commSetNonBlocking(), DBG_IMPORTANT, debugs, dlinkAddTail(), FD_DESC_SZ, fd_note(), getCurrentTime(), HELPER_MAX_ARGS, helperHandleRead, helperKickQueue(), Helper::SessionBase::HelperServerClosed(), hIpc, Important, ipcCreate(), wordlist::key, memAllocBuf(), wordlist::next, pid, progname, ReadBufSize(), Helper::Session::requestTimeout(), safe_free, squid_curtime, xmalloc, and xstrdup.
Referenced by Enqueue().
◆ overloaded()
◆ packStatsInto()
void Client::packStatsInto | ( | Packable * | p, |
const char * | label = nullptr |
||
) | const |
Definition at line 694 of file helper.cc.
References Packable::append(), Packable::appendf(), assert, current_time, int, dlink_node::next, PRIu64, Format::QuoteMimeBlob(), and tvSubMsec().
Referenced by authenticateNegotiateStats(), and authenticateNTLMStats().
◆ prepSubmit()
|
protected |
prepares the helper for request submission returns true if and only if the submission should proceed may kill Squid if the helper remains overloaded for too long
Definition at line 526 of file helper.cc.
References Helper::ChildConfig::actDie, DBG_IMPORTANT, debugs, fatalf(), and squid_curtime.
◆ queueFull()
◆ submit()
|
protected |
◆ submitRequest()
void Client::submitRequest | ( | Xaction * | r | ) |
Submits a request to the helper or add it to the queue if none of the servers is available.
Definition at line 455 of file helper.cc.
References Enqueue(), GetFirstAvailable(), and helperDispatch().
Referenced by helperReturnBuffer().
◆ syncQueueStats()
|
protected |
Definition at line 499 of file helper.cc.
References DBG_IMPORTANT, debugs, and squid_curtime.
◆ trySubmit()
bool Client::trySubmit | ( | const char * | buf, |
HLPCB * | callback, | ||
void * | data | ||
) |
Definition at line 553 of file helper.cc.
Referenced by helperSubmit().
◆ willOverload()
bool Client::willOverload | ( | ) | const |
whether the helper will be in "overloaded" state after one more request already overloaded helpers return true
Definition at line 752 of file helper.cc.
References GetFirstAvailable().
Member Data Documentation
◆ addr
Ip::Address Helper::Client::addr |
◆ childs
ChildConfig Helper::Client::childs |
Definition at line 119 of file helper.h.
Referenced by Enqueue(), GetFirstAvailable(), helperKickQueue(), helperReturnBuffer(), helperShutdown(), helperStatefulKickQueue(), helperStatefulShutdown(), StatefulEnqueue(), and StatefulGetFirstAvailable().
◆ cmdline
◆ droppedRequests
◆ eom
◆ id_name
const char* Helper::Client::id_name = nullptr |
Definition at line 118 of file helper.h.
Referenced by Enqueue(), helperReturnBuffer(), helperShutdown(), helperStatefulShutdown(), and StatefulEnqueue().
◆ ipc_type
◆ last_queue_warn
time_t Helper::Client::last_queue_warn = 0 |
Definition at line 124 of file helper.h.
Referenced by Enqueue(), and StatefulEnqueue().
◆ last_restart
◆ onTimedOutResponse
◆ overloadStart
◆ queue
std::queue<Xaction *> Helper::Client::queue |
Definition at line 117 of file helper.h.
Referenced by Enqueue(), and StatefulEnqueue().
◆ retryBrokenHelper
◆ retryTimedOut
bool Helper::Client::retryTimedOut = false |
Definition at line 127 of file helper.h.
Referenced by helperReturnBuffer().
◆ servers
dlink_list Helper::Client::servers |
Definition at line 116 of file helper.h.
Referenced by GetFirstAvailable(), helperShutdown(), helperStatefulShutdown(), and StatefulGetFirstAvailable().
◆ stats
struct Helper::Client::_stats Helper::Client::stats |
Referenced by Enqueue(), helperReturnBuffer(), and StatefulEnqueue().
◆ timeout
time_t Helper::Client::timeout = 0 |
Definition at line 126 of file helper.h.
Referenced by helperReturnBuffer().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products