#include <helper.h>
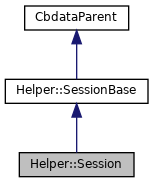
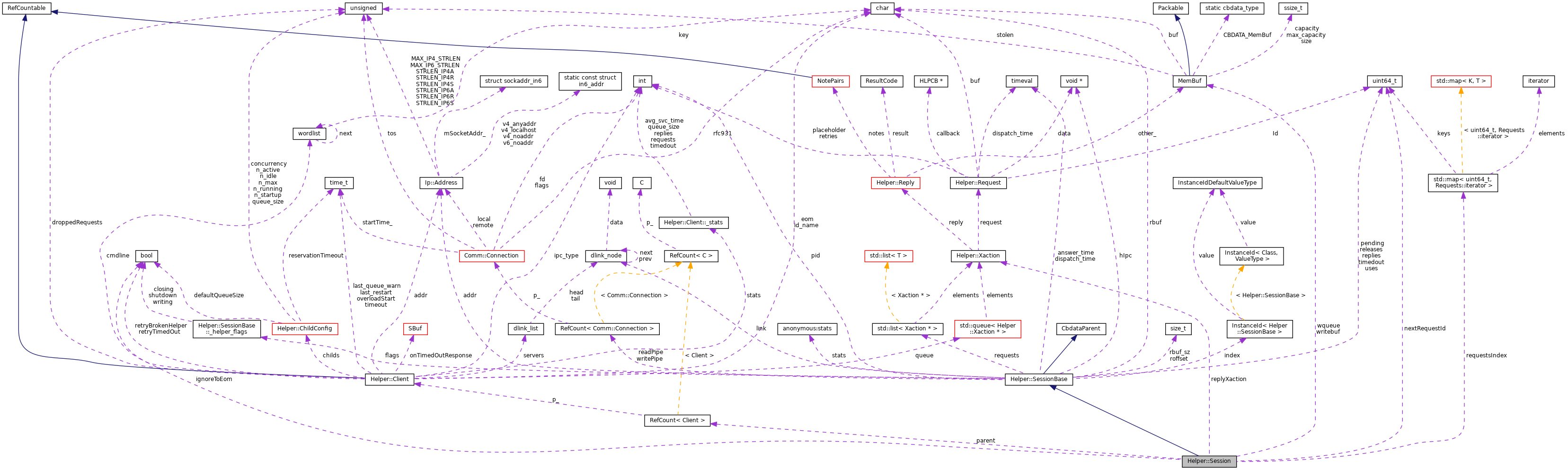
Public Types | |
typedef std::map< uint64_t, Requests::iterator > | RequestIndex |
using | Requests = std::list< Xaction * > |
Public Member Functions | |
~Session () override | |
Xaction * | popRequest (int requestId) |
void | checkForTimedOutRequests (bool const retry) |
bool | reserved () override |
whether the server is locked for exclusive use by a client More... | |
void | dropQueued () override |
dequeues and sends an Unknown answer to all queued requests More... | |
Client & | helper () const override |
our creator (parent) object More... | |
void | closePipesSafely () |
void | closeWritePipeSafely () |
void | initStats () |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | requestTimeout (const CommTimeoutCbParams &io) |
Read timeout handler. More... | |
static void | HelperServerClosed (SessionBase *) |
close handler to handle exited server processes More... | |
Public Attributes | |
uint64_t | nextRequestId |
MemBuf * | wqueue |
MemBuf * | writebuf |
Client::Pointer | parent |
Xaction * | replyXaction |
bool | ignoreToEom |
Whether to ignore current message, because it is timed-out or other reason. More... | |
RequestIndex | requestsIndex |
maps request IDs to requests More... | |
const InstanceId< SessionBase > | index |
int | pid |
Ip::Address | addr |
Comm::ConnectionPointer | readPipe |
Comm::ConnectionPointer | writePipe |
void * | hIpc |
char * | rbuf |
size_t | rbuf_sz |
size_t | roffset |
struct timeval | dispatch_time |
struct timeval | answer_time |
dlink_node | link |
struct Helper::SessionBase::_helper_flags | flags |
Requests | requests |
requests in order of submission/expiration More... | |
struct { | |
uint64_t uses | |
uint64_t replies | |
uint64_t pending | |
uint64_t releases | |
uint64_t timedout | |
} | stats |
Private Member Functions | |
CBDATA_CHILD (Session) | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Detailed Description
represents a single "stateless helper" process; supports concurrent helper requests
Member Typedef Documentation
◆ RequestIndex
typedef std::map<uint64_t, Requests::iterator> Helper::Session::RequestIndex |
◆ Requests
|
inherited |
Constructor & Destructor Documentation
◆ ~Session()
|
override |
Definition at line 142 of file helper.cc.
References assert, dlinkDelete(), and Comm::IsConnOpen().
Member Function Documentation
◆ CBDATA_CHILD()
|
private |
◆ checkForTimedOutRequests()
void Helper::Session::checkForTimedOutRequests | ( | bool const | retry | ) |
Run over the active requests lists and forces a retry, or timedout reply or the configured "on timeout response" for timedout requests.
Definition at line 1563 of file helper.cc.
References assert, cbdataReferenceValid(), debugs, MAX_RETRIES, and Helper::TimedOut.
Referenced by helperReturnBuffer(), and requestTimeout().
◆ closePipesSafely()
|
inherited |
Closes pipes to the helper safely. Handles the case where the read and write pipes are the same FD.
Definition at line 72 of file helper.cc.
References DBG_IMPORTANT, debugs, getCurrentTime(), hIpc, and pid.
Referenced by helperReturnBuffer(), helperStatefulHandleRead(), and helperStatefulShutdown().
◆ closeWritePipeSafely()
|
inherited |
Closes the reading pipe. If the read and write sockets are the same the write pipe will also be closed. Otherwise its left open for later handling.
Definition at line 98 of file helper.cc.
References DBG_IMPORTANT, debugs, getCurrentTime(), hIpc, and pid.
Referenced by helperReturnBuffer(), and helperStatefulServerDone().
◆ dropQueued()
|
overridevirtual |
Reimplemented from Helper::SessionBase.
Definition at line 165 of file helper.cc.
References Helper::SessionBase::dropQueued().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ helper()
|
inlineoverridevirtual |
◆ HelperServerClosed()
|
staticinherited |
Definition at line 928 of file helper.cc.
References Helper::SessionBase::dropQueued(), Helper::Client::handleKilledServer(), and Helper::SessionBase::helper().
Referenced by Helper::Client::openSessions(), and statefulhelper::openSessions().
◆ initStats()
|
inherited |
Definition at line 62 of file helper.cc.
References Helper::SessionBase::stats.
Referenced by statefulhelper::openSessions().
◆ popRequest()
Helper::Xaction * Helper::Session::popRequest | ( | int | requestId | ) |
◆ requestTimeout()
|
static |
Definition at line 1601 of file helper.cc.
References checkForTimedOutRequests(), commCbCall(), commSetConnTimeout(), CommCommonCbParams::conn, CommCommonCbParams::data, debugs, max(), and squid_curtime.
Referenced by Helper::Client::openSessions().
◆ reserved()
|
inlineoverridevirtual |
Implements Helper::SessionBase.
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
Member Data Documentation
◆ addr
|
inherited |
Definition at line 227 of file helper.h.
Referenced by statefulhelper::openSessions().
◆ answer_time
|
inherited |
Definition at line 237 of file helper.h.
Referenced by helperReturnBuffer(), and helperStatefulHandleRead().
◆ dispatch_time
|
inherited |
Definition at line 236 of file helper.h.
Referenced by helperReturnBuffer(), helperStatefulDispatch(), and helperStatefulHandleRead().
◆ flags
|
inherited |
◆ hIpc
|
inherited |
Definition at line 230 of file helper.h.
Referenced by statefulhelper::openSessions().
◆ ignoreToEom
◆ index
|
inherited |
Helper program identifier; does not change when contents do, including during assignment
Definition at line 224 of file helper.h.
Referenced by GetFirstAvailable(), Helper::Client::handleKilledServer(), helperDispatch(), helperReturnBuffer(), helperStatefulDispatch(), helperStatefulHandleRead(), helperStatefulKickQueue(), helperStatefulShutdown(), and StatefulGetFirstAvailable().
◆ link
|
inherited |
Definition at line 239 of file helper.h.
Referenced by statefulhelper::openSessions().
◆ nextRequestId
uint64_t Helper::Session::nextRequestId |
Definition at line 267 of file helper.h.
Referenced by helperDispatch().
◆ parent
Client::Pointer Helper::Session::parent |
Definition at line 272 of file helper.h.
Referenced by helper(), helperDispatch(), and helperHandleRead().
◆ pending
|
inherited |
Definition at line 253 of file helper.h.
Referenced by GetFirstAvailable(), helperDispatch(), helperReturnBuffer(), helperStatefulDispatch(), helperStatefulHandleRead(), helperStatefulServerDone(), helperStatefulShutdown(), and StatefulGetFirstAvailable().
◆ pid
|
inherited |
Definition at line 226 of file helper.h.
Referenced by statefulhelper::openSessions().
◆ rbuf
|
inherited |
Definition at line 232 of file helper.h.
Referenced by helperStatefulHandleRead(), and statefulhelper::openSessions().
◆ rbuf_sz
|
inherited |
Definition at line 233 of file helper.h.
Referenced by helperReturnBuffer(), helperStatefulHandleRead(), and statefulhelper::openSessions().
◆ readPipe
|
inherited |
Definition at line 228 of file helper.h.
Referenced by helperStatefulHandleRead(), and statefulhelper::openSessions().
◆ releases
◆ replies
|
inherited |
Definition at line 252 of file helper.h.
Referenced by Helper::Client::handleKilledServer(), helperReturnBuffer(), and helperStatefulHandleRead().
◆ replyXaction
Xaction* Helper::Session::replyXaction |
The helper request Xaction object for the current reply . A helper reply may be distributed to more than one of the retrieved packets from helper. This member stores the Xaction object as long as the end-of-message for current reply is not retrieved.
Definition at line 278 of file helper.h.
Referenced by helperReturnBuffer().
◆ requests
|
inherited |
Definition at line 248 of file helper.h.
Referenced by helperDispatch(), helperStatefulDispatch(), and helperStatefulHandleRead().
◆ requestsIndex
RequestIndex Helper::Session::requestsIndex |
Definition at line 285 of file helper.h.
Referenced by helperDispatch().
◆ roffset
|
inherited |
Definition at line 234 of file helper.h.
Referenced by helperStatefulHandleRead(), and statefulhelper::openSessions().
◆ stats
struct { ... } Helper::SessionBase::stats |
◆ timedout
◆ uses
|
inherited |
Definition at line 251 of file helper.h.
Referenced by helperDispatch(), and helperStatefulDispatch().
◆ wqueue
MemBuf* Helper::Session::wqueue |
Definition at line 269 of file helper.h.
Referenced by helperDispatch().
◆ writebuf
MemBuf* Helper::Session::writebuf |
Definition at line 270 of file helper.h.
Referenced by helperDispatch(), and helperDispatchWriteDone().
◆ writePipe
|
inherited |
Definition at line 229 of file helper.h.
Referenced by helperDispatch(), helperStatefulDispatch(), and statefulhelper::openSessions().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products