#include <EnumIterator.h>
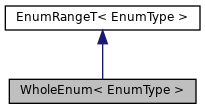
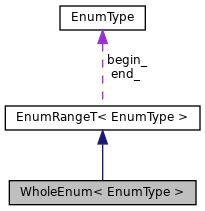
Public Types | |
typedef EnumIterator< EnumType > | iterator |
typedef ReverseEnumIterator< EnumType > | reverse_iterator |
Public Member Functions | |
WholeEnum () | |
iterator | begin () const |
iterator | end () const |
reverse_iterator | rbegin () const |
reverse_iterator | rend () const |
Private Attributes | |
EnumType | begin_ |
EnumType | end_ |
Detailed Description
class WholeEnum< EnumType >
Class expressing a continuous range of a whole enum for range-for expressions
Class for iterating all enum values, from EnumType::enumBegin_ up to, but not including, EnumType::enumEnd_.
This class requires that:
- the underlying enum values be represented by continuous values of an integral type.
- both enumBegin_ and enumEnd_ markers must be present as EnumType values;
- enumBegin_ must have the same representation as the first element of the enum
- enumEnd_ must have a representation that is one past the last user-accessible value of the enum.
Typical use:
Definition at line 221 of file EnumIterator.h.
Member Typedef Documentation
◆ iterator
|
inherited |
Definition at line 160 of file EnumIterator.h.
◆ reverse_iterator
|
inherited |
Definition at line 161 of file EnumIterator.h.
Constructor & Destructor Documentation
◆ WholeEnum()
|
inline |
Definition at line 224 of file EnumIterator.h.
Member Function Documentation
◆ begin()
|
inlineinherited |
Definition at line 163 of file EnumIterator.h.
References EnumRangeT< EnumType >::begin_.
Referenced by TestEnumIterator::testBidirectionalIter(), and TestEnumIterator::testForwardIter().
◆ end()
|
inlineinherited |
Definition at line 164 of file EnumIterator.h.
References EnumRangeT< EnumType >::end_.
Referenced by TestEnumIterator::testBidirectionalIter().
◆ rbegin()
|
inlineinherited |
Definition at line 165 of file EnumIterator.h.
References EnumRangeT< EnumType >::end_.
Referenced by TestEnumIterator::testReverseIter().
◆ rend()
|
inlineinherited |
Definition at line 166 of file EnumIterator.h.
References EnumRangeT< EnumType >::begin_.
Referenced by TestEnumIterator::testUnsignedEnum().
Member Data Documentation
◆ begin_
|
privateinherited |
Definition at line 168 of file EnumIterator.h.
Referenced by EnumRangeT< EnumType >::begin(), and EnumRangeT< EnumType >::rend().
◆ end_
|
privateinherited |
Definition at line 169 of file EnumIterator.h.
Referenced by EnumRangeT< EnumType >::end(), and EnumRangeT< EnumType >::rbegin().
The documentation for this class was generated from the following file:
- src/base/EnumIterator.h