#include <EnumIterator.h>

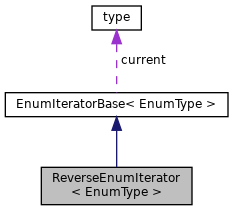
Public Types | |
using | iterator_category = std::bidirectional_iterator_tag |
using | value_type = EnumType |
using | difference_type = std::ptrdiff_t |
using | pointer = EnumType * |
using | reference = EnumType & |
Public Member Functions | |
ReverseEnumIterator (EnumType e) | |
ReverseEnumIterator & | operator++ () |
ReverseEnumIterator & | operator++ (int) |
ReverseEnumIterator & | operator-- () |
ReverseEnumIterator & | operator-- (int) |
bool | operator== (const EnumIteratorBase &i) const |
bool | operator!= (const EnumIteratorBase &i) const |
EnumType | operator* () const |
Protected Types | |
typedef std::underlying_type< EnumType >::type | iterator_type |
Protected Attributes | |
iterator_type | current |
Detailed Description
template<typename EnumType>
class ReverseEnumIterator< EnumType >
bidirectional reverse iterator over an enum type
It can be instantiated using any enum (or C++11 strongly-typed enum) value; the most common expected use scenario has iterators emitted by EnumRange and WholeEnum via standard rbegin() and rend() calls.
In order for the iterator to work, it is mandatory that the underlying enum type's representation values be sequential.
The iterator does not check for bounds; behavior is undefined if the iterator is incremented (or decremented) outside the range representing valid enum symbols (remember: an enum is not a data structure).
- See also
- EnumRange, WholeEnum, EnumIterator
Definition at line 111 of file EnumIterator.h.
Member Typedef Documentation
◆ difference_type
|
inherited |
Definition at line 31 of file EnumIterator.h.
◆ iterator_category
|
inherited |
Definition at line 29 of file EnumIterator.h.
◆ iterator_type
|
protectedinherited |
Definition at line 26 of file EnumIterator.h.
◆ pointer
|
inherited |
Definition at line 32 of file EnumIterator.h.
◆ reference
|
inherited |
Definition at line 33 of file EnumIterator.h.
◆ value_type
|
inherited |
Definition at line 30 of file EnumIterator.h.
Constructor & Destructor Documentation
◆ ReverseEnumIterator()
|
inlineexplicit |
Definition at line 114 of file EnumIterator.h.
Member Function Documentation
◆ operator!=()
|
inlineinherited |
Definition at line 41 of file EnumIterator.h.
References EnumIteratorBase< EnumType >::current.
◆ operator*()
|
inlineinherited |
Definition at line 45 of file EnumIterator.h.
References EnumIteratorBase< EnumType >::current.
◆ operator++() [1/2]
|
inline |
Definition at line 117 of file EnumIterator.h.
◆ operator++() [2/2]
|
inline |
Definition at line 123 of file EnumIterator.h.
◆ operator--() [1/2]
|
inline |
Definition at line 130 of file EnumIterator.h.
◆ operator--() [2/2]
|
inline |
Definition at line 136 of file EnumIterator.h.
◆ operator==()
|
inlineinherited |
Definition at line 37 of file EnumIterator.h.
References EnumIteratorBase< EnumType >::current.
Member Data Documentation
◆ current
|
protectedinherited |
Definition at line 49 of file EnumIterator.h.
Referenced by EnumIteratorBase< EnumType >::operator!=(), EnumIteratorBase< EnumType >::operator*(), and EnumIteratorBase< EnumType >::operator==().
The documentation for this class was generated from the following file:
- src/base/EnumIterator.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products