#include <EnumIterator.h>
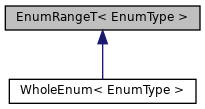
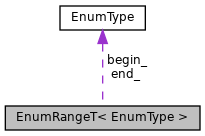
Public Types | |
typedef EnumIterator< EnumType > | iterator |
typedef ReverseEnumIterator< EnumType > | reverse_iterator |
Public Member Functions | |
EnumRangeT (EnumType first, EnumType one_past_last) | |
iterator | begin () const |
iterator | end () const |
reverse_iterator | rbegin () const |
reverse_iterator | rend () const |
Private Attributes | |
EnumType | begin_ |
EnumType | end_ |
Detailed Description
template<typename EnumType>
class EnumRangeT< EnumType >
Class expressing a continuous range of an enum for range-for expressions
This class requires that the underlying enum values be represented by continuous values of an integral type. Users will usually not rely on this class directly but on the more convenient EnumRange function
- Note
- EnumIterator<enum>(EnumType::firstmember,EnumType::lastmember) will miss EnumType::lastmember while iterating. If you need to iterate over all of EnumType, use class WholeEnum.
Definition at line 157 of file EnumIterator.h.
Member Typedef Documentation
◆ iterator
typedef EnumIterator<EnumType> EnumRangeT< EnumType >::iterator |
Definition at line 160 of file EnumIterator.h.
◆ reverse_iterator
typedef ReverseEnumIterator<EnumType> EnumRangeT< EnumType >::reverse_iterator |
Definition at line 161 of file EnumIterator.h.
Constructor & Destructor Documentation
◆ EnumRangeT()
|
inline |
Definition at line 162 of file EnumIterator.h.
Member Function Documentation
◆ begin()
|
inline |
Definition at line 163 of file EnumIterator.h.
References EnumRangeT< EnumType >::begin_.
Referenced by TestEnumIterator::testBidirectionalIter(), and TestEnumIterator::testForwardIter().
◆ end()
|
inline |
Definition at line 164 of file EnumIterator.h.
References EnumRangeT< EnumType >::end_.
Referenced by TestEnumIterator::testBidirectionalIter().
◆ rbegin()
|
inline |
Definition at line 165 of file EnumIterator.h.
References EnumRangeT< EnumType >::end_.
Referenced by TestEnumIterator::testReverseIter().
◆ rend()
|
inline |
Definition at line 166 of file EnumIterator.h.
References EnumRangeT< EnumType >::begin_.
Referenced by TestEnumIterator::testUnsignedEnum().
Member Data Documentation
◆ begin_
|
private |
Definition at line 168 of file EnumIterator.h.
Referenced by EnumRangeT< EnumType >::begin(), and EnumRangeT< EnumType >::rend().
◆ end_
|
private |
Definition at line 169 of file EnumIterator.h.
Referenced by EnumRangeT< EnumType >::end(), and EnumRangeT< EnumType >::rbegin().
The documentation for this class was generated from the following file:
- src/base/EnumIterator.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products