TLS squid.conf settings for a listening port. More...
#include <ServerOptions.h>
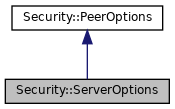
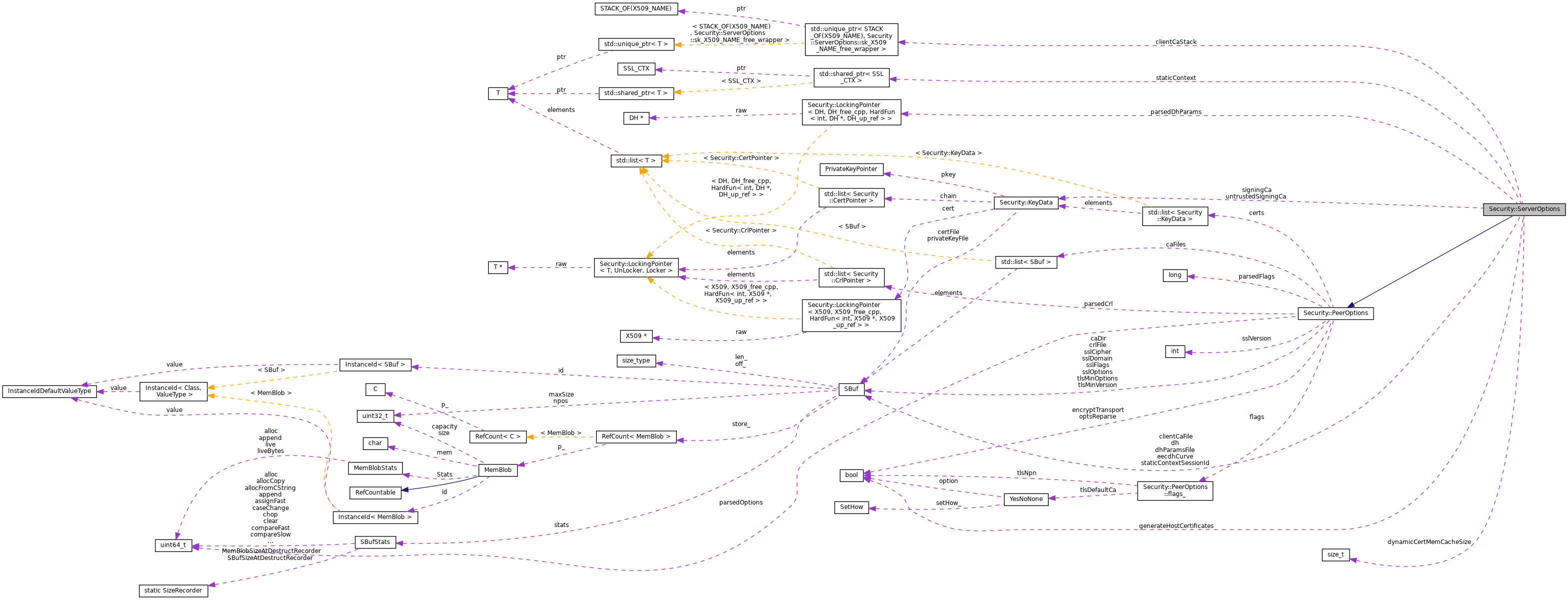
Public Types | |
typedef std::unique_ptr< STACK_OF(X509_NAME), Security::ServerOptions::sk_X509_NAME_free_wrapper > | X509_NAME_STACK_Pointer |
Public Member Functions | |
sk_dtor_wrapper (sk_X509_NAME, STACK_OF(X509_NAME) *, X509_NAME_free) | |
ServerOptions () | |
ServerOptions (const ServerOptions &o) | |
ServerOptions & | operator= (const ServerOptions &) |
ServerOptions (ServerOptions &&o) | |
ServerOptions & | operator= (ServerOptions &&o) |
~ServerOptions () override=default | |
void | parse (const char *) override |
parse a TLS squid.conf option More... | |
void | clear () override |
reset the configuration details to default More... | |
Security::ContextPointer | createBlankContext () const override |
generate an unset security context object More... | |
void | dumpCfg (std::ostream &, const char *pfx) const override |
output squid.conf syntax with 'pfx' prefix on parameters for the stored settings More... | |
void | initServerContexts (AnyP::PortCfg &) |
bool | updateContextConfig (Security::ContextPointer &) |
update the given TLS security context using squid.conf settings More... | |
void | updateContextEecdh (Security::ContextPointer &) |
update the context with DH, EDH, EECDH settings More... | |
void | updateContextClientCa (Security::ContextPointer &) |
update the context with CA details used to verify client certificates More... | |
void | updateContextSessionId (Security::ContextPointer &) |
update the context with a configured session ID (if any) More... | |
void | syncCaFiles () |
sync the various sources of CA files to be loaded More... | |
void | parseOptions () |
parse and verify the [tls-]options= string in sslOptions More... | |
Security::ContextPointer | createClientContext (bool setOptions) |
generate a security client-context from these configured options More... | |
void | updateTlsVersionLimits () |
sync the context options with tls-min-version=N configuration More... | |
void | updateContextOptions (Security::ContextPointer &) |
Setup the library specific 'options=' parameters for the given context. More... | |
void | updateContextNpn (Security::ContextPointer &) |
setup the NPN extension details for the given context More... | |
void | updateContextCa (Security::ContextPointer &) |
setup the CA details for the given context More... | |
void | updateContextCrl (Security::ContextPointer &) |
setup the CRL details for the given context More... | |
void | updateContextTrust (Security::ContextPointer &) |
decide which CAs to trust More... | |
void | updateSessionOptions (Security::SessionPointer &) |
setup any library-specific options that can be set for the given session More... | |
Public Attributes | |
Security::ContextPointer | staticContext |
TLS context to use for HTTPS accelerator or static SSL-Bump. More... | |
SBuf | staticContextSessionId |
"session id context" for staticContext More... | |
bool | generateHostCertificates = true |
dynamically make host cert More... | |
Security::KeyData | signingCa |
x509 certificate and key for signing generated certificates More... | |
Security::KeyData | untrustedSigningCa |
x509 certificate and key for signing untrusted generated certificates More... | |
size_t | dynamicCertMemCacheSize = 4*1024*1024 |
max size of generated certificates memory cache (4 MB default) More... | |
SBuf | sslOptions |
library-specific options string More... | |
SBuf | caDir |
path of directory containing a set of trusted Certificate Authorities More... | |
SBuf | crlFile |
path of file containing Certificate Revoke List More... | |
SBuf | sslCipher |
SBuf | sslFlags |
flags defining what TLS operations Squid performs More... | |
SBuf | sslDomain |
SBuf | tlsMinVersion |
version label for minimum TLS version to permit More... | |
ParsedPortFlags | parsedFlags = 0 |
parsed value of sslFlags More... | |
std::list< Security::KeyData > | certs |
details from the cert= and file= config parameters More... | |
std::list< SBuf > | caFiles |
paths of files containing trusted Certificate Authority More... | |
Security::CertRevokeList | parsedCrl |
CRL to use when verifying the remote end certificate. More... | |
bool | encryptTransport = false |
whether transport encryption (TLS/SSL) is to be used on connections to the peer More... | |
Protected Member Functions | |
template<typename T > | |
Security::ContextPointer | convertContextFromRawPtr (T ctx) const |
Protected Attributes | |
int | sslVersion = 0 |
struct Security::PeerOptions::flags_ | flags |
Private Member Functions | |
bool | loadClientCaFile () |
void | loadDhParams () |
bool | createStaticServerContext (AnyP::PortCfg &) |
void | createSigningContexts (const AnyP::PortCfg &) |
ParsedPortFlags | parseFlags () |
void | loadCrlFile () |
void | loadKeysFile () |
Private Attributes | |
SBuf | clientCaFile |
name of file to load client CAs from More... | |
X509_NAME_STACK_Pointer | clientCaStack |
CA certificate(s) to use when verifying client certificates. More... | |
SBuf | dh |
Diffi-Helman cipher config. More... | |
SBuf | dhParamsFile |
Diffi-Helman ciphers parameter file. More... | |
SBuf | eecdhCurve |
Elliptic curve for ephemeral EC-based DH key exchanges. More... | |
Security::DhePointer | parsedDhParams |
DH parameters for temporary/ephemeral DH key exchanges. More... | |
SBuf | tlsMinOptions |
Security::ParsedOptions | parsedOptions |
bool | optsReparse = true |
whether parsedOptions content needs to be regenerated More... | |
Detailed Description
Definition at line 25 of file ServerOptions.h.
Member Typedef Documentation
◆ X509_NAME_STACK_Pointer
typedef std::unique_ptr<STACK_OF(X509_NAME), Security::ServerOptions::sk_X509_NAME_free_wrapper> Security::ServerOptions::X509_NAME_STACK_Pointer |
Definition at line 30 of file ServerOptions.h.
Constructor & Destructor Documentation
◆ ServerOptions() [1/3]
|
inline |
Definition at line 33 of file ServerOptions.h.
References YesNoNone::defaultTo(), Security::PeerOptions::flags, and Security::PeerOptions::flags_::tlsDefaultCa.
Referenced by clear().
◆ ServerOptions() [2/3]
|
inline |
Definition at line 38 of file ServerOptions.h.
◆ ServerOptions() [3/3]
|
inline |
Definition at line 40 of file ServerOptions.h.
References operator=().
◆ ~ServerOptions()
|
overridedefault |
Member Function Documentation
◆ clear()
|
inlineoverridevirtual |
Reimplemented from Security::PeerOptions.
Definition at line 46 of file ServerOptions.h.
References ServerOptions().
◆ convertContextFromRawPtr()
|
inlineprotectedinherited |
Definition at line 111 of file PeerOptions.h.
◆ createBlankContext()
|
overridevirtual |
Reimplemented from Security::PeerOptions.
Definition at line 163 of file ServerOptions.cc.
References DBG_CRITICAL, debugs, Security::ErrorString(), Ssl::Initialize(), and TLS_server_method.
Referenced by Ssl::createSSLContext().
◆ createClientContext()
|
inherited |
Definition at line 271 of file PeerOptions.cc.
References Ssl::InitClientContext().
Referenced by configDoConfigure().
◆ createSigningContexts()
|
private |
initialize contexts for signing dynamic TLS certificates (if needed) the resulting keys are stored in signingCa and untrustedSigningCa
Definition at line 285 of file ServerOptions.cc.
References DBG_CRITICAL, DBG_IMPORTANT, debugs, fatalf(), Ssl::generateUntrustedCert(), port, and AnyP::ProtocolType_str.
◆ createStaticServerContext()
|
private |
generate a security server-context from these configured options the resulting context is stored in staticContext
- Returns
- true if a context could be created
Definition at line 214 of file ServerOptions.cc.
References SBuf::append(), SBuf::appendf(), DBG_CRITICAL, DBG_IMPORTANT, debugs, error(), Security::ErrorString(), and keys.
◆ dumpCfg()
|
overridevirtual |
Reimplemented from Security::PeerOptions.
Definition at line 140 of file ServerOptions.cc.
References Security::PeerOptions::dumpCfg().
◆ initServerContexts()
void Security::ServerOptions::initServerContexts | ( | AnyP::PortCfg & | port | ) |
initialize all server contexts as-needed and load PEM files. if none can be created this may do nothing.
Definition at line 193 of file ServerOptions.cc.
References fatalf(), port, and AnyP::ProtocolType_str.
◆ loadClientCaFile()
|
private |
load clientca= file (if any) into memory.
- Return values
-
true clientca is not set, or loaded successfully false unable to load the file, or not using OpenSSL
Definition at line 338 of file ServerOptions.cc.
References DBG_CRITICAL, and debugs.
◆ loadCrlFile()
|
privateinherited |
Load a CRLs list stored in the file whose /path/name is in crlFile replaces any CRL loaded previously
Definition at line 613 of file PeerOptions.cc.
References debugs.
◆ loadDhParams()
|
private |
Definition at line 355 of file ServerOptions.cc.
References asHex(), assert, DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, Ssl::ForgetErrors(), Security::LockingPointer< T, UnLocker, Locker >::get(), and Ssl::ReportAndForgetErrors().
◆ loadKeysFile()
|
privateinherited |
◆ operator=() [1/2]
Security::ServerOptions & Security::ServerOptions::operator= | ( | const ServerOptions & | old | ) |
Definition at line 35 of file ServerOptions.cc.
References clientCaFile, clientCaStack, dh, dhParamsFile, dynamicCertMemCacheSize, eecdhCurve, generateHostCertificates, Security::PeerOptions::operator=(), parsedDhParams, signingCa, staticContextSessionId, and untrustedSigningCa.
Referenced by operator=(), and ServerOptions().
◆ operator=() [2/2]
|
inline |
Definition at line 41 of file ServerOptions.h.
References operator=().
◆ parse()
|
overridevirtual |
Reimplemented from Security::PeerOptions.
Definition at line 60 of file ServerOptions.cc.
References DBG_CRITICAL, DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, max(), SBuf::npos, Security::PeerOptions::parse(), parseBytesOptionValue(), and self_destruct().
◆ parseFlags()
|
privateinherited |
Parses the TLS flags squid.conf parameter
Definition at line 549 of file PeerOptions.cc.
References DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, fatal(), fatalf(), Here, SQUIDSBUFPH, SQUIDSBUFPRINT, SSL_FLAG_CONDITIONAL_AUTH, SSL_FLAG_DELAYED_AUTH, SSL_FLAG_DONT_VERIFY_DOMAIN, SSL_FLAG_DONT_VERIFY_PEER, SSL_FLAG_NO_DEFAULT_CA, SSL_FLAG_NO_SESSION_REUSE, SSL_FLAG_VERIFY_CRL, and SSL_FLAG_VERIFY_CRL_ALL.
◆ parseOptions()
|
inherited |
Pre-parse TLS options= parameter to be applied when the TLS objects created. Options must not used in the case of peek or stare bump mode.
Definition at line 442 of file PeerOptions.cc.
References CharacterSet::ALPHA, SBuf::append(), Parser::Tokenizer::atEnd(), SBuf::c_str(), SBuf::cmp(), DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, CharacterSet::DIGIT, Security::ErrorString(), fatalf(), Parser::Tokenizer::int64(), SBuf::isEmpty(), ssl_option::name, SQUIDSBUFPH, SQUIDSBUFPRINT, and ssl_options.
Referenced by parse_securePeerOptions(), and Security::PeerOptions::PeerOptions().
◆ sk_dtor_wrapper()
Security::ServerOptions::sk_dtor_wrapper | ( | sk_X509_NAME | , |
STACK_OF(X509_NAME) * | , | ||
X509_NAME_free | |||
) |
◆ syncCaFiles()
void Security::ServerOptions::syncCaFiles | ( | ) |
Definition at line 323 of file ServerOptions.cc.
◆ updateContextCa()
|
inherited |
Definition at line 691 of file PeerOptions.cc.
References DBG_IMPORTANT, debugs, Security::ErrorString(), and loadSystemTrustedCa().
◆ updateContextClientCa()
void Security::ServerOptions::updateContextClientCa | ( | Security::ContextPointer & | ctx | ) |
Definition at line 495 of file ServerOptions.cc.
References Ssl::ConfigurePeerVerification(), DBG_CRITICAL, debugs, Ssl::DisablePeerVerification(), Security::ErrorString(), and STACK_OF().
◆ updateContextConfig()
bool Security::ServerOptions::updateContextConfig | ( | Security::ContextPointer & | ctx | ) |
Definition at line 453 of file ServerOptions.cc.
References Config, DBG_CRITICAL, debugs, Security::ErrorString(), Ssl::MaybeSetupRsaCallback(), Security::SetSessionCacheCallbacks(), SquidConfig::SSL, ssl_ctx_ex_index_dont_verify_domain, SSL_FLAG_DONT_VERIFY_DOMAIN, SSL_FLAG_NO_SESSION_REUSE, and SquidConfig::unclean_shutdown.
Referenced by Ssl::createSSLContext().
◆ updateContextCrl()
|
inherited |
Definition at line 727 of file PeerOptions.cc.
References debugs, SSL_FLAG_VERIFY_CRL, and SSL_FLAG_VERIFY_CRL_ALL.
◆ updateContextEecdh()
void Security::ServerOptions::updateContextEecdh | ( | Security::ContextPointer & | ctx | ) |
Definition at line 522 of file ServerOptions.cc.
References DBG_CRITICAL, debugs, Security::ErrorString(), Ssl::ForgetErrors(), and Ssl::ReportAndForgetErrors().
◆ updateContextNpn()
|
inherited |
Definition at line 659 of file PeerOptions.cc.
◆ updateContextOptions()
|
inherited |
Definition at line 634 of file PeerOptions.cc.
◆ updateContextSessionId()
void Security::ServerOptions::updateContextSessionId | ( | Security::ContextPointer & | ctx | ) |
Definition at line 575 of file ServerOptions.cc.
◆ updateContextTrust()
|
inherited |
Definition at line 754 of file PeerOptions.cc.
References assert, DBG_IMPORTANT, debugs, and Security::ErrorString().
◆ updateSessionOptions()
|
inherited |
Definition at line 774 of file PeerOptions.cc.
References DBG_IMPORTANT, debugs, and Security::ErrorString().
Referenced by CreateSession().
◆ updateTlsVersionLimits()
|
inherited |
Definition at line 153 of file PeerOptions.cc.
References SBuf::append(), SBuf::chop(), DBG_PARSE_NOTE, and debugs.
Member Data Documentation
◆ caDir
|
inherited |
Definition at line 81 of file PeerOptions.h.
◆ caFiles
|
inherited |
Definition at line 106 of file PeerOptions.h.
◆ certs
|
inherited |
Definition at line 105 of file PeerOptions.h.
Referenced by Ssl::InitClientContext().
◆ clientCaFile
|
private |
Definition at line 107 of file ServerOptions.h.
Referenced by operator=().
◆ clientCaStack
|
private |
Definition at line 110 of file ServerOptions.h.
Referenced by operator=().
◆ crlFile
|
inherited |
Definition at line 82 of file PeerOptions.h.
◆ dh
|
private |
Definition at line 115 of file ServerOptions.h.
Referenced by operator=().
◆ dhParamsFile
|
private |
Definition at line 116 of file ServerOptions.h.
Referenced by operator=().
◆ dynamicCertMemCacheSize
size_t Security::ServerOptions::dynamicCertMemCacheSize = 4*1024*1024 |
Definition at line 91 of file ServerOptions.h.
Referenced by operator=().
◆ eecdhCurve
|
private |
Definition at line 117 of file ServerOptions.h.
Referenced by operator=().
◆ encryptTransport
|
inherited |
Definition at line 147 of file PeerOptions.h.
Referenced by Adaptation::Config::dumpService(), PeerPoolMgr::handleOpenedConnection(), Security::BlindPeerConnector::initialize(), netdbExchangeStart(), FwdState::secureConnectionToPeerIfNeeded(), and CachePeer::securityContext().
◆ flags
|
protectedinherited |
Referenced by ServerOptions().
◆ generateHostCertificates
bool Security::ServerOptions::generateHostCertificates = true |
Definition at line 75 of file ServerOptions.h.
Referenced by operator=().
◆ optsReparse
|
privateinherited |
Definition at line 100 of file PeerOptions.h.
◆ parsedCrl
|
inherited |
Definition at line 107 of file PeerOptions.h.
◆ parsedDhParams
|
private |
Definition at line 119 of file ServerOptions.h.
Referenced by operator=().
◆ parsedFlags
|
inherited |
Definition at line 103 of file PeerOptions.h.
◆ parsedOptions
|
privateinherited |
Parsed value of sslOptions + tlsMinOptions settings. Set optsReparse=true to have this re-parsed before next use.
Definition at line 97 of file PeerOptions.h.
◆ signingCa
Security::KeyData Security::ServerOptions::signingCa |
Definition at line 87 of file ServerOptions.h.
Referenced by Ssl::chainCertificatesToSSLContext(), and operator=().
◆ sslCipher
|
inherited |
Definition at line 84 of file PeerOptions.h.
Referenced by Ssl::InitClientContext().
◆ sslDomain
|
inherited |
Definition at line 86 of file PeerOptions.h.
Referenced by Security::BlindPeerConnector::initialize().
◆ sslFlags
|
inherited |
Definition at line 85 of file PeerOptions.h.
◆ sslOptions
|
inherited |
Definition at line 80 of file PeerOptions.h.
◆ sslVersion
|
protectedinherited |
Definition at line 130 of file PeerOptions.h.
◆ staticContext
Security::ContextPointer Security::ServerOptions::staticContext |
Definition at line 71 of file ServerOptions.h.
◆ staticContextSessionId
SBuf Security::ServerOptions::staticContextSessionId |
Definition at line 72 of file ServerOptions.h.
Referenced by operator=().
◆ tlsMinOptions
|
privateinherited |
Library-specific options string generated from tlsMinVersion. Call updateTlsVersionLimits() to regenerate this string.
Definition at line 93 of file PeerOptions.h.
◆ tlsMinVersion
|
inherited |
Definition at line 88 of file PeerOptions.h.
◆ untrustedSigningCa
Security::KeyData Security::ServerOptions::untrustedSigningCa |
Definition at line 88 of file ServerOptions.h.
Referenced by operator=().
The documentation for this class was generated from the following files:
- src/security/ServerOptions.h
- src/security/ServerOptions.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products