#include <StatHist.h>
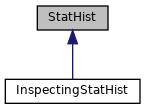
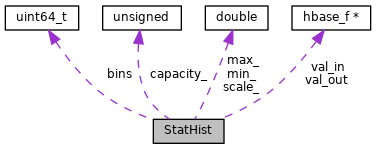
Public Types | |
typedef uint64_t | bins_type |
Public Member Functions | |
StatHist ()=default | |
StatHist (const StatHist &) | |
~StatHist () | |
StatHist & | operator= (const StatHist &) |
double | deltaPctile (const StatHist &B, double pctile) const |
double | val (unsigned int bin) const |
void | count (double val) |
void | dump (StoreEntry *sentry, StatHistBinDumper *bd) const |
void | logInit (unsigned int capacity, double min, double max) |
void | enumInit (unsigned int last_enum) |
StatHist & | operator+= (const StatHist &B) |
Protected Member Functions | |
void | init (unsigned int capacity, hbase_f *val_in, hbase_f *val_out, double min, double max) |
unsigned int | findBin (double v) |
Protected Attributes | |
bins_type * | bins = nullptr |
the histogram counters More... | |
unsigned int | capacity_ = 0 |
double | min_ = 0.0 |
minimum value to be stored, corresponding to the first bin More... | |
double | max_ = 0.0 |
value of the maximum counter in the histogram More... | |
double | scale_ = 1.0 |
scaling factor when looking for a bin More... | |
hbase_f * | val_in = nullptr |
hbase_f * | val_out = nullptr |
Detailed Description
Generic histogram class
see important comments on hbase_f restrictions in StatHist.cc
Definition at line 25 of file StatHist.h.
Member Typedef Documentation
◆ bins_type
typedef uint64_t StatHist::bins_type |
Definition at line 44 of file StatHist.h.
Constructor & Destructor Documentation
◆ StatHist() [1/2]
|
default |
- Note
- the default constructor doesn't fully initialize. you have to call one of the *init functions to specialize the histogram TODO: merge functionality from the *init functions to the constructor and drop these TODO: specialize the class in a small hierarchy so that all relevant initializations are done at build-time
◆ StatHist() [2/2]
StatHist::StatHist | ( | const StatHist & | src | ) |
Definition at line 39 of file StatHist.cc.
◆ ~StatHist()
|
inline |
Definition at line 39 of file StatHist.h.
Member Function Documentation
◆ count()
void StatHist::count | ( | double | val | ) |
increment the counter for the histogram entry associated to the supplied value
Definition at line 55 of file StatHist.cc.
References bins, and findBin().
Referenced by CacheDigest::add(), clientUpdateHierCounters(), clientUpdateStatHistCounters(), Comm::DoSelect(), Comm::Incoming::finishPolling(), fqdncacheHandleReply(), httpHdrCcUpdateStats(), icpCount(), ipcacheHandleReply(), recordMemBlobSizeAtDestruct(), recordSBufSizeAtDestruct(), TestStatHist::testStatHistBaseAssignment(), TestStatHist::testStatHistBaseEquality(), TestStatHist::testStatHistLog(), TestStatHist::testStatHistSum(), and HttpHdrScTarget::updateStats().
◆ deltaPctile()
◆ dump()
void StatHist::dump | ( | StoreEntry * | sentry, |
StatHistBinDumper * | bd | ||
) | const |
iterate the supplied bd function over the histogram values
Definition at line 171 of file StatHist.cc.
References assert, bins, capacity_, min_, statHistBinDumper, and val().
Referenced by commIncomingStats(), SBufStatsAction::dump(), httpHeaderStatDump(), and statCountersHistograms().
◆ enumInit()
void StatHist::enumInit | ( | unsigned int | last_enum | ) |
initialize the histogram to count occurrences in an enum-represented set
Definition at line 235 of file StatHist.cc.
References init(), and Math::Null.
Referenced by HttpHeaderStat::HttpHeaderStat(), Comm::Incoming::init(), TestStatHist::testStatHistBaseAssignment(), and TestStatHist::testStatHistBaseEquality().
◆ findBin()
find what entry in the histogram corresponds to v, by applying the preset input transformation function
Definition at line 64 of file StatHist.cc.
References capacity_, min_, scale_, and val_in.
Referenced by count(), and InspectingStatHist::counter().
◆ init()
|
protected |
low-level initialize function. called by *Init high-level functions
- Note
- Important restrictions on val_in and val_out functions:
- val_in: ascending, defined on [0, oo), val_in(0) == 0;
- val_out: x == val_out(val_in(x)) where val_in(x) is defined
In practice, the requirements are less strict, but then it gets hard to define them without math notation. val_in is applied after offsetting the value but before scaling See log and linear based histograms for examples
Definition at line 26 of file StatHist.cc.
References assert, bins, capacity_, max_, min_, scale_, val_in, val_out, and xcalloc().
Referenced by enumInit(), and logInit().
◆ logInit()
Initialize the Histogram using a logarithmic values distribution
Definition at line 221 of file StatHist.cc.
References Math::Exp, init(), Math::Log, max(), and min().
Referenced by makeDestructTimeHist(), TestStatHist::testStatHistLog(), and TestStatHist::testStatHistSum().
◆ operator+=()
◆ operator=()
◆ val()
obtain the output-transformed value from the specified bin
Definition at line 85 of file StatHist.cc.
References min_, scale_, and val_out.
Referenced by deltaPctile(), and dump().
Member Data Documentation
◆ bins
|
protected |
Definition at line 101 of file StatHist.h.
Referenced by count(), InspectingStatHist::counter(), deltaPctile(), dump(), init(), operator+=(), operator=(), InspectingStatHist::operator==(), StatHist(), and ~StatHist().
◆ capacity_
|
protected |
Definition at line 102 of file StatHist.h.
Referenced by deltaPctile(), dump(), findBin(), init(), operator+=(), operator=(), InspectingStatHist::operator==(), StatHist(), and ~StatHist().
◆ max_
|
protected |
Definition at line 108 of file StatHist.h.
Referenced by init(), operator+=(), operator=(), and InspectingStatHist::operator==().
◆ min_
|
protected |
Definition at line 105 of file StatHist.h.
Referenced by dump(), findBin(), init(), operator+=(), operator=(), InspectingStatHist::operator==(), and val().
◆ scale_
|
protected |
Definition at line 111 of file StatHist.h.
Referenced by findBin(), init(), operator=(), InspectingStatHist::operator==(), and val().
◆ val_in
|
protected |
Definition at line 112 of file StatHist.h.
Referenced by findBin(), init(), operator=(), and InspectingStatHist::operator==().
◆ val_out
|
protected |
Definition at line 113 of file StatHist.h.
Referenced by init(), operator=(), InspectingStatHist::operator==(), and val().
The documentation for this class was generated from the following files:
- src/StatHist.h
- src/StatHist.cc
- src/tests/stub_StatHist.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products