#include <ErrorDetail.h>
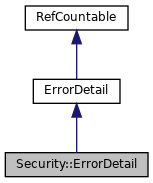
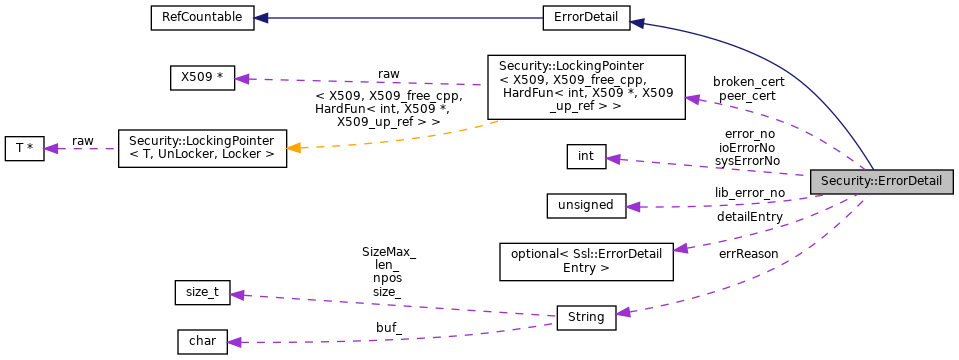
Public Types | |
typedef ErrorDetailPointer | Pointer |
Public Member Functions | |
ErrorDetail (ErrorCode err_no, const CertPointer &peer, const CertPointer &broken, const char *aReason=nullptr) | |
ErrorDetail (ErrorCode anErrorCode, int anIoErrorNo, int aSysErrorNo) | |
SBuf | brief () const override |
SBuf | verbose (const HttpRequestPointer &) const override |
ErrorCode | errorNo () const |
int | sysError () const |
Certificate * | peerCert () |
the peer certificate (or nil) More... | |
Certificate * | brokenCert () |
peer or intermediate certificate that failed validation (or nil) More... | |
void | setPeerCertificate (const CertPointer &) |
bool | equals (const ErrorDetail &other) const |
Private Types | |
using | ErrorDetailEntry = Ssl::ErrorDetailEntry |
Private Member Functions | |
MEMPROXY_CLASS (Security::ErrorDetail) | |
ErrorDetail (ErrorCode err, int aSysErrorNo) | |
helper constructor implementing the logic shared by the two public ones More... | |
void | printSubject (std::ostream &os) const |
textual representation of the subject of the broken certificate More... | |
void | printCaName (std::ostream &os) const |
the issuer of the broken certificate More... | |
void | printCommonName (std::ostream &os) const |
a list of the broken certificates CN and alternate names More... | |
void | printNotBefore (std::ostream &os) const |
textual representation of the "not before" field of the broken certificate More... | |
void | printNotAfter (std::ostream &os) const |
textual representation of the "not after" field of the broken certificate More... | |
void | printErrorCode (std::ostream &os) const |
textual representation of error_no More... | |
void | printErrorDescription (std::ostream &os) const |
short description of error_no More... | |
void | printErrorLibError (std::ostream &os) const |
textual representation of lib_error_no More... | |
size_t | convertErrorCodeToDescription (const char *code, std::ostream &os) const |
Private Attributes | |
CertPointer | peer_cert |
A pointer to the peer certificate. More... | |
CertPointer | broken_cert |
A pointer to the broken certificate (peer or intermediate) More... | |
ErrorCode | error_no = 0 |
Squid-discovered error, validation error, or zero;. More... | |
LibErrorCode | lib_error_no = 0 |
TLS library-reported non-validation error or zero;. More... | |
int | sysErrorNo = 0 |
errno(3); system call failure code or zero More... | |
int | ioErrorNo = 0 |
std::optional< ErrorDetailEntry > | detailEntry |
String | errReason |
a custom reason for the error More... | |
Detailed Description
Details a TLS-related error. Two kinds of errors can be detailed:
- certificate validation errors (including built-in and helper-driven) and
- TLS logic and I/O errors (detected by Squid or the TLS library).
The following details may be available (only the first one is required):
- for all errors: problem classification (
- See also
- ErrorCode)
- for all errors: peer certificate
- for certificate validation errors: the broken certificate
- for certificate validation errors: validation failure reason
- for non-validation errors: TLS library-reported error(s)
- for non-validation errors: system call errno(3)
Definition at line 39 of file ErrorDetail.h.
Member Typedef Documentation
◆ ErrorDetailEntry
|
private |
Definition at line 117 of file ErrorDetail.h.
◆ Pointer
Definition at line 44 of file ErrorDetail.h.
Constructor & Destructor Documentation
◆ ErrorDetail() [1/3]
ErrorDetail::ErrorDetail | ( | ErrorCode | err_no, |
const CertPointer & | peer, | ||
const CertPointer & | broken, | ||
const char * | aReason = nullptr |
||
) |
Details a server-side certificate verification failure. If broken
is nil, then the broken certificate is the peer certificate.
Definition at line 466 of file ErrorDetail.cc.
References broken_cert, errReason, and peer_cert.
◆ ErrorDetail() [2/3]
Details (or starts detailing) a non-validation failure.
- Parameters
-
anIoErrorNo TLS I/O function outcome;
- See also
- ErrorDetail::ioErrorNo
- Parameters
-
aSysErrorNo saved errno(3);
- See also
- ErrorDetail::sysErrorNo
Definition at line 475 of file ErrorDetail.cc.
References ioErrorNo.
◆ ErrorDetail() [3/3]
Extract and remember errors stored internally by the TLS library.
Definition at line 445 of file ErrorDetail.cc.
References asHex(), debugs, Security::ForgetErrors(), and lib_error_no.
Member Function Documentation
◆ brief()
|
overridevirtual |
- Returns
- a single "token" summarizing available details suitable as an access.log field and similar output processed by programs
Implements ErrorDetail.
Definition at line 500 of file ErrorDetail.cc.
References asHex(), SysErrorDetail::Brief(), and SBufStream::buf().
◆ brokenCert()
|
inline |
Definition at line 78 of file ErrorDetail.h.
References broken_cert, and Security::LockingPointer< T, UnLocker, Locker >::get().
◆ convertErrorCodeToDescription()
|
private |
Converts the code to a string value. Supported formatting codes are:
Error meta information: err_name: The name of a high-level SSL error (e.g., X509_V_ERR_*) ssl_error_descr: A short description of the SSL error ssl_lib_error: human-readable low-level error string by ErrorString()
Certificate information extracted from broken (not necessarily peer!) cert ssl_cn: The comma-separated list of common and alternate names ssl_subject: The certificate subject ssl_ca_name: The certificate issuer name ssl_notbefore: The certificate "not before" field ssl_notafter: The certificate "not after" field
- Returns
- the length of the code (the number of characters to be replaced by value)
- Return values
-
0 for unsupported codes
Definition at line 757 of file ErrorDetail.cc.
References code, printCaName(), printCommonName(), printErrorCode(), printErrorDescription(), printErrorLibError(), printNotAfter(), printNotBefore(), and printSubject().
◆ equals()
|
inlineinherited |
Definition at line 44 of file Detail.h.
Referenced by Error::update().
◆ errorNo()
|
inline |
- Returns
- error category;
- See also
- ErrorCode
Definition at line 67 of file ErrorDetail.h.
References error_no.
◆ MEMPROXY_CLASS()
|
private |
◆ peerCert()
|
inline |
Definition at line 75 of file ErrorDetail.h.
References Security::LockingPointer< T, UnLocker, Locker >::get(), and peer_cert.
◆ printCaName()
|
private |
Definition at line 643 of file ErrorDetail.cc.
References html_quote(), and Security::IssuerName().
Referenced by convertErrorCodeToDescription().
◆ printCommonName()
|
private |
Definition at line 628 of file ErrorDetail.cc.
References Ssl::HasMatchingSubjectName(), and CommonNamesPrinter::printed.
Referenced by convertErrorCodeToDescription().
◆ printErrorCode()
|
private |
Definition at line 695 of file ErrorDetail.cc.
References Security::ErrorNameFromCode().
Referenced by convertErrorCodeToDescription().
◆ printErrorDescription()
|
private |
Definition at line 709 of file ErrorDetail.cc.
Referenced by convertErrorCodeToDescription().
◆ printErrorLibError()
|
private |
Definition at line 728 of file ErrorDetail.cc.
References Security::ErrorString().
Referenced by convertErrorCodeToDescription().
◆ printNotAfter()
|
private |
Definition at line 677 of file ErrorDetail.cc.
References Ssl::asn1timeToString(), and X509_getm_notAfter.
Referenced by convertErrorCodeToDescription().
◆ printNotBefore()
|
private |
Definition at line 659 of file ErrorDetail.cc.
References Ssl::asn1timeToString(), and X509_getm_notBefore.
Referenced by convertErrorCodeToDescription().
◆ printSubject()
|
private |
Definition at line 561 of file ErrorDetail.cc.
References html_quote(), and Security::SubjectName().
Referenced by convertErrorCodeToDescription().
◆ setPeerCertificate()
void ErrorDetail::setPeerCertificate | ( | const CertPointer & | cert | ) |
remember the SSL certificate of our peer; requires nil peerCert() unlike the cert-setting constructor, does not assume the cert is bad
Definition at line 490 of file ErrorDetail.cc.
References assert.
◆ sysError()
|
inline |
- Returns
- the previously saved errno(3) or zero
Definition at line 70 of file ErrorDetail.h.
References sysErrorNo.
Referenced by Security::PeerConnector::noteNegotiationError().
◆ verbose()
|
overridevirtual |
- Returns
- all available details; may be customized for the given request suitable for error pages and other output meant for human consumption
Implements ErrorDetail.
Definition at line 534 of file ErrorDetail.cc.
References assert, SBufStream::buf(), and Ssl::ErrorDetailsManager::GetInstance().
Member Data Documentation
◆ broken_cert
|
private |
Definition at line 99 of file ErrorDetail.h.
Referenced by brokenCert(), and ErrorDetail().
◆ detailEntry
|
mutableprivate |
Definition at line 118 of file ErrorDetail.h.
◆ error_no
|
private |
◆ errReason
|
private |
Definition at line 123 of file ErrorDetail.h.
Referenced by ErrorDetail().
◆ ioErrorNo
|
private |
OpenSSL-specific (first-level or intermediate) TLS I/O operation result reported by SSL_get_error(3SSL) (e.g., SSL_ERROR_SYSCALL) or zero. Unlike lib_error_no, this error is mostly meant for I/O control and has no OpenSSL-provided human-friendly text representation.
Definition at line 115 of file ErrorDetail.h.
Referenced by ErrorDetail().
◆ lib_error_no
|
private |
◆ peer_cert
|
private |
Definition at line 98 of file ErrorDetail.h.
Referenced by ErrorDetail(), and peerCert().
◆ sysErrorNo
|
private |
Definition at line 108 of file ErrorDetail.h.
Referenced by sysError().
The documentation for this class was generated from the following files:
- src/security/ErrorDetail.h
- src/security/ErrorDetail.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products