#include <Pool.h>
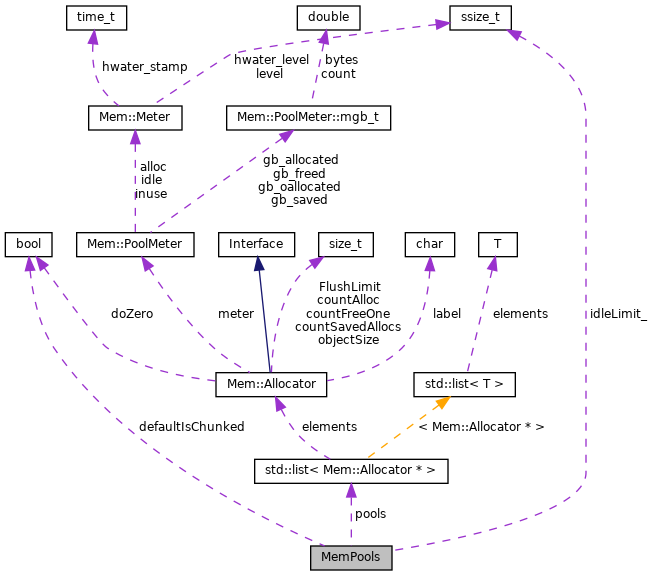
Public Member Functions | |
MemPools () | |
void | flushMeters () |
Mem::Allocator * | create (const char *, size_t) |
void | setIdleLimit (const ssize_t newLimit) |
ssize_t | idleLimit () const |
void | clean (time_t maxage) |
void | setDefaultPoolChunking (bool const &) |
Static Public Member Functions | |
static MemPools & | GetInstance () |
Public Attributes | |
std::list< Mem::Allocator * > | pools |
bool | defaultIsChunked = false |
Private Attributes | |
ssize_t | idleLimit_ = (2 << 20) |
Detailed Description
Constructor & Destructor Documentation
◆ MemPools()
MemPools::MemPools | ( | ) |
Member Function Documentation
◆ clean()
void MemPools::clean | ( | time_t | maxage | ) |
- Main cleanup handler. For MemPools to stay within upper idle limits, this function needs to be called periodically, preferably at some constant rate, eg. from Squid event. It looks through all pools and chunks, cleans up internal states and checks for releasable chunks.
- Between the calls to this function objects are placed onto internal cache instead of returning to their home chunks, mainly for speedup purpose. During that time state of chunk is not known, it is not known whether chunk is free or in use. This call returns all objects to their chunks and restores consistency.
- Should be called relatively often, as it sorts chunks in suitable order as to reduce free memory fragmentation and increase chunk utilisation. Suitable frequency for cleanup is in range of few tens of seconds to few minutes, depending of memory activity.
TODO: DOCS: Re-write this shorter!
- Parameters
-
maxage Release all totally idle chunks that have not been referenced for maxage seconds.
Definition at line 105 of file Pool.cc.
References Mem::Meter::currentLevel(), flushMeters(), Mem::PoolMeter::idle, idleLimit(), pools, and TheMeter.
Referenced by Mem::CleanIdlePools(), and memClean().
◆ create()
Mem::Allocator * MemPools::create | ( | const char * | label, |
size_t | obj_size | ||
) |
Create an allocator with given name to allocate fixed-size objects of the specified size.
Definition at line 47 of file Pool.cc.
References defaultIsChunked, and pools.
Referenced by Mem::AllocatorProxy::getAllocator().
◆ flushMeters()
void MemPools::flushMeters | ( | ) |
Definition at line 72 of file Pool.cc.
References Mem::PoolMeter::alloc, Mem::Meter::currentLevel(), Mem::PoolMeter::flush(), Mem::PoolMeter::gb_allocated, Mem::PoolMeter::gb_freed, Mem::PoolMeter::gb_saved, Mem::PoolMeter::idle, Mem::PoolMeter::inuse, pools, and TheMeter.
Referenced by clean(), and Mem::GlobalStats().
◆ GetInstance()
|
static |
Definition at line 27 of file Pool.cc.
References Instance(), and MemPools().
Referenced by Mem::CleanIdlePools(), MemPoolMalloc::deallocate(), Mem::AllocatorProxy::getAllocator(), Mem::GlobalStats(), memClean(), memConfigure(), and Mem::Report().
◆ idleLimit()
|
inline |
Limits the cumulative size of allocated (but unused) memory in all pools. Initial value is 2MB until first configuration, See squid.conf memory_pools_limit directive.
Definition at line 80 of file Pool.h.
References idleLimit_.
Referenced by clean().
◆ setDefaultPoolChunking()
void MemPools::setDefaultPoolChunking | ( | bool const & | aBool | ) |
Definition at line 63 of file Pool.cc.
References defaultIsChunked.
◆ setIdleLimit()
|
inline |
Sets upper limit in bytes to amount of free ram kept in pools. This is not strict upper limit, but a hint. When MemPools are over this limit, deallocate attempts to release memory to the system instead of pooling.
Definition at line 78 of file Pool.h.
References idleLimit_.
Referenced by memClean(), and memConfigure().
Member Data Documentation
◆ defaultIsChunked
bool MemPools::defaultIsChunked = false |
Definition at line 113 of file Pool.h.
Referenced by create(), MemPools(), and setDefaultPoolChunking().
◆ idleLimit_
|
private |
Limits the cumulative size of allocated (but unused) memory in all pools. Initial value is 2MB until first configuration, See squid.conf memory_pools_limit directive.
Definition at line 119 of file Pool.h.
Referenced by idleLimit(), and setIdleLimit().
◆ pools
std::list<Mem::Allocator *> MemPools::pools |
Definition at line 112 of file Pool.h.
Referenced by clean(), create(), flushMeters(), Mem::GlobalStats(), and Mem::Report().
The documentation for this class was generated from the following files:
- src/mem/Pool.h
- src/mem/Pool.cc
- src/tests/stub_libmem.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products