#include "asn1.h"
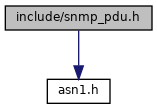

Go to the source code of this file.
Classes | |
struct | snmp_pdu |
Macros | |
#define | SNMP_PDU_GET (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x0) |
#define | SNMP_PDU_GETNEXT (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x1) |
#define | SNMP_PDU_RESPONSE (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x2) |
#define | SNMP_PDU_GETBULK (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x5) |
#define | MAX_BINDINGS 2147483647 /* PDU Defaults */ |
#define | SNMP_DEFAULT_ERRSTAT -1 |
#define | SNMP_DEFAULT_ERRINDEX -1 |
#define | SNMP_DEFAULT_ADDRESS 0 |
#define | SNMP_DEFAULT_REQID 0 |
Functions | |
struct snmp_pdu * | snmp_pdu_create (int) |
struct snmp_pdu * | snmp_pdu_clone (struct snmp_pdu *) |
struct snmp_pdu * | snmp_pdu_fix (struct snmp_pdu *, int) |
struct snmp_pdu * | snmp_fix_pdu (struct snmp_pdu *, int) |
void | snmp_free_pdu (struct snmp_pdu *) |
void | snmp_pdu_free (struct snmp_pdu *) |
u_char * | snmp_pdu_encode (u_char *, int *, struct snmp_pdu *) |
u_char * | snmp_pdu_decode (u_char *, int *, struct snmp_pdu *) |
void | snmp_add_null_var (struct snmp_pdu *, oid *, int) |
Macro Definition Documentation
◆ MAX_BINDINGS
#define MAX_BINDINGS 2147483647 /* PDU Defaults */ |
Definition at line 96 of file snmp_pdu.h.
◆ SNMP_DEFAULT_ADDRESS
#define SNMP_DEFAULT_ADDRESS 0 |
Definition at line 99 of file snmp_pdu.h.
◆ SNMP_DEFAULT_ERRINDEX
#define SNMP_DEFAULT_ERRINDEX -1 |
Definition at line 98 of file snmp_pdu.h.
◆ SNMP_DEFAULT_ERRSTAT
#define SNMP_DEFAULT_ERRSTAT -1 |
Definition at line 97 of file snmp_pdu.h.
◆ SNMP_DEFAULT_REQID
#define SNMP_DEFAULT_REQID 0 |
Definition at line 100 of file snmp_pdu.h.
◆ SNMP_PDU_GET
#define SNMP_PDU_GET (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x0) |
Definition at line 92 of file snmp_pdu.h.
◆ SNMP_PDU_GETBULK
#define SNMP_PDU_GETBULK (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x5) |
Definition at line 95 of file snmp_pdu.h.
◆ SNMP_PDU_GETNEXT
#define SNMP_PDU_GETNEXT (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x1) |
Definition at line 93 of file snmp_pdu.h.
◆ SNMP_PDU_RESPONSE
#define SNMP_PDU_RESPONSE (ASN_CONTEXT | ASN_CONSTRUCTOR | 0x2) |
Definition at line 94 of file snmp_pdu.h.
Function Documentation
◆ snmp_add_null_var()
Definition at line 637 of file snmp_pdu.c.
References variable_list::name, variable_list::name_length, variable_list::next_variable, NULL, snmp_var_new(), and snmp_pdu::variables.
◆ snmp_fix_pdu()
Definition at line 186 of file snmp_pdu.c.
References snmp_pdu::command, snmp_pdu::errindex, snmp_pdu::errstat, variable_list::next_variable, NULL, snmp_pdu::reqid, SNMP_DEFAULT_ERRINDEX, SNMP_DEFAULT_ERRSTAT, SNMP_DEFAULT_REQID, SNMP_ERR_NOERROR, snmp_free_pdu(), snmp_pdu_clone(), snmp_pdu_free(), SNMP_PDU_RESPONSE, snmp_set_api_error(), snmp_var_clone(), SNMPERR_UNABLE_TO_FIX, snmplib_debug(), and snmp_pdu::variables.
Referenced by snmp_pdu_fix().
◆ snmp_free_pdu()
void snmp_free_pdu | ( | struct snmp_pdu * | ) |
Definition at line 284 of file snmp_pdu.c.
References snmp_pdu::enterprise, variable_list::next_variable, snmp_var_free(), snmp_pdu::variables, and xfree.
Referenced by Snmp::SendResponse(), snmp_fix_pdu(), snmp_pdu_free(), snmpConstructReponse(), and snmpDecodePacket().
◆ snmp_pdu_clone()
Definition at line 147 of file snmp_pdu.c.
References NULL, snmp_set_api_error(), SNMPERR_OS_ERR, snmplib_debug(), and xmalloc.
Referenced by snmp_fix_pdu().
◆ snmp_pdu_create()
Definition at line 113 of file snmp_pdu.c.
References snmp_pdu::command, NULL, SNMP_DEFAULT_ADDRESS, SNMP_DEFAULT_ERRINDEX, SNMP_DEFAULT_ERRSTAT, snmp_set_api_error(), SNMPERR_OS_ERR, snmplib_debug(), and xmalloc.
Referenced by snmpAgentResponse(), and snmpDecodePacket().
◆ snmp_pdu_decode()
Definition at line 486 of file snmp_pdu.c.
References snmp_pdu::agent_addr, ASN_PARSE_ERROR, asn_parse_header(), asn_parse_int(), asn_parse_objid(), asn_parse_string(), asn_parse_unsigned_int(), snmp_pdu::command, snmp_pdu::enterprise, snmp_pdu::enterprise_length, snmp_pdu::errindex, snmp_pdu::errstat, four, MAX_NAME_LEN, snmp_pdu::max_repetitions, snmp_pdu::non_repeaters, NULL, variable_list::objid, snmp_pdu::reqid, SNMP_PDU_GETBULK, snmp_set_api_error(), SNMPERR_OS_ERR, snmplib_debug(), snmp_pdu::specific_type, snmp_pdu::time, snmp_pdu::trap_type, and xmalloc.
Referenced by snmp_msg_Decode().
◆ snmp_pdu_encode()
Definition at line 350 of file snmp_pdu.c.
References snmp_pdu::agent_addr, asn_build_int(), asn_build_objid(), asn_build_string(), asn_build_unsigned_int(), ASN_INTEGER, ASN_OBJECT_ID, ASN_PRIMITIVE, ASN_UNIVERSAL, snmp_pdu::command, snmp_pdu::enterprise, snmp_pdu::enterprise_length, snmp_pdu::errindex, snmp_pdu::errstat, snmp_pdu::max_repetitions, snmp_pdu::non_repeaters, NULL, snmp_pdu::reqid, SMI_IPADDRESS, SMI_TIMETICKS, SNMP_PDU_GETBULK, snmplib_debug(), snmp_pdu::specific_type, snmp_pdu::time, and snmp_pdu::trap_type.
Referenced by snmp_msg_Encode().
◆ snmp_pdu_fix()
Definition at line 181 of file snmp_pdu.c.
References snmp_pdu::command, and snmp_fix_pdu().
◆ snmp_pdu_free()
void snmp_pdu_free | ( | struct snmp_pdu * | ) |
Definition at line 275 of file snmp_pdu.c.
References snmp_free_pdu().
Referenced by snmp_fix_pdu().
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products