Manages arguments passed to a program (i.e., main(argc, argv) parameters). More...
#include <CommandLine.h>
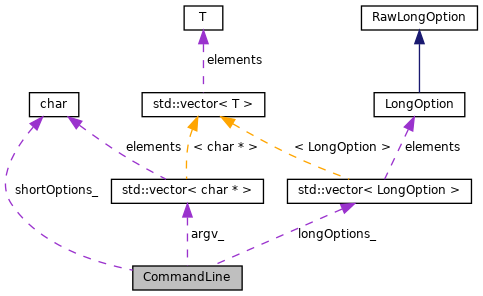
Public Types | |
typedef void | Visitor(const int optId, const char *optValue) |
Public Member Functions | |
CommandLine (int argc, char *argv[], const char *shortRules, const RawLongOption *longRules) | |
expects main() input plus getopt_long(3) grammar rules for parsing argv More... | |
CommandLine (const CommandLine &them) | |
~CommandLine () | |
CommandLine & | operator= (const CommandLine &) |
bool | hasOption (const int optId, const char **optValue=nullptr) const |
void | forEachOption (Visitor) const |
calls Visitor for each of the configured command line option More... | |
const char * | arg0 () const |
int | argc () const |
char ** | argv () const |
void | resetArg0 (const char *programName) |
replaces argv[0] with the new value More... | |
void | pushFrontOption (const char *name, const char *value=nullptr) |
inserts a (possibly duplicated) option at the beginning of options (just after argv[0]) More... | |
Private Member Functions | |
const RawLongOption * | longOptions () const |
bool | nextOption (int &optId) const |
Private Attributes | |
std::vector< char * > | argv_ |
raw main() parameters, including argv[0] and a nil argv[argc] More... | |
const char * | shortOptions_ |
single-dash, single-letter (-x) option rules More... | |
std::vector< LongOption > | longOptions_ |
long –option rules More... | |
Detailed Description
Definition at line 34 of file CommandLine.h.
Member Typedef Documentation
◆ Visitor
typedef void CommandLine::Visitor(const int optId, const char *optValue) |
A callback function for forEachOption(); receives parsed options. Must not call pushFrontOption(), hasOption() or forEachOption() – getopt(3) uses globals!
Definition at line 52 of file CommandLine.h.
Constructor & Destructor Documentation
◆ CommandLine() [1/2]
CommandLine::CommandLine | ( | int | argc, |
char * | argv[], | ||
const char * | shortRules, | ||
const RawLongOption * | longRules | ||
) |
Definition at line 24 of file CommandLine.cc.
References argv_, assert, longOptions_, and xstrdup.
◆ CommandLine() [2/2]
CommandLine::CommandLine | ( | const CommandLine & | them | ) |
Definition at line 46 of file CommandLine.cc.
◆ ~CommandLine()
CommandLine::~CommandLine | ( | ) |
Definition at line 62 of file CommandLine.cc.
References argv_, shortOptions_, and xfree.
Member Function Documentation
◆ arg0()
|
inline |
- Returns
- argv[0], which is usually a program "name"
Definition at line 58 of file CommandLine.h.
References argv_.
Referenced by watch_child().
◆ argc()
|
inline |
- Returns
- main()'s argc, which is traditionally missing the last/nil item
Definition at line 61 of file CommandLine.h.
References argv_.
Referenced by nextOption().
◆ argv()
|
inline |
- Returns
- main()'s argv[] which is traditionally const-wrong
Definition at line 64 of file CommandLine.h.
References argv_.
Referenced by nextOption().
◆ forEachOption()
void CommandLine::forEachOption | ( | Visitor | visitor | ) | const |
Definition at line 89 of file CommandLine.cc.
References nextOption(), optarg, and ResetGetopt().
Referenced by SquidMain().
◆ hasOption()
bool CommandLine::hasOption | ( | const int | optId, |
const char ** | optValue = nullptr |
||
) | const |
- Returns
- whether the option with optId identifier is present When returning true, sets non-nil optValue to the found option's value. For letter options (-x) and their –long synonyms, the letter is the ID. For long-only –options, the ID is the configured options::val value.
Definition at line 71 of file CommandLine.cc.
References nextOption(), optarg, and ResetGetopt().
Referenced by ConfigureCurrentKid().
◆ longOptions()
|
inlineprivate |
◆ nextOption()
|
private |
extracts the next option (if any)
- Returns
- whether the option was extracted throws on unknown option or missing required argument
Definition at line 101 of file CommandLine.cc.
References argc(), argv(), argv_, assert, longOptions(), optind, SBuf::Printf(), shortOptions_, and TexcHere.
Referenced by forEachOption(), and hasOption().
◆ operator=()
CommandLine & CommandLine::operator= | ( | const CommandLine & | them | ) |
Definition at line 52 of file CommandLine.cc.
References argv_, longOptions_, and shortOptions_.
◆ pushFrontOption()
void CommandLine::pushFrontOption | ( | const char * | name, |
const char * | value = nullptr |
||
) |
Definition at line 123 of file CommandLine.cc.
◆ resetArg0()
void CommandLine::resetArg0 | ( | const char * | programName | ) |
Definition at line 115 of file CommandLine.cc.
References argv_, assert, xfree, and xstrdup.
Referenced by watch_child().
Member Data Documentation
◆ argv_
|
private |
Definition at line 77 of file CommandLine.h.
Referenced by arg0(), argc(), argv(), CommandLine(), nextOption(), operator=(), pushFrontOption(), resetArg0(), and ~CommandLine().
◆ longOptions_
|
private |
Definition at line 81 of file CommandLine.h.
Referenced by CommandLine(), longOptions(), and operator=().
◆ shortOptions_
|
private |
Definition at line 80 of file CommandLine.h.
Referenced by nextOption(), operator=(), and ~CommandLine().
The documentation for this class was generated from the following files:
- src/CommandLine.h
- src/CommandLine.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products