#include <ClpMap.h>
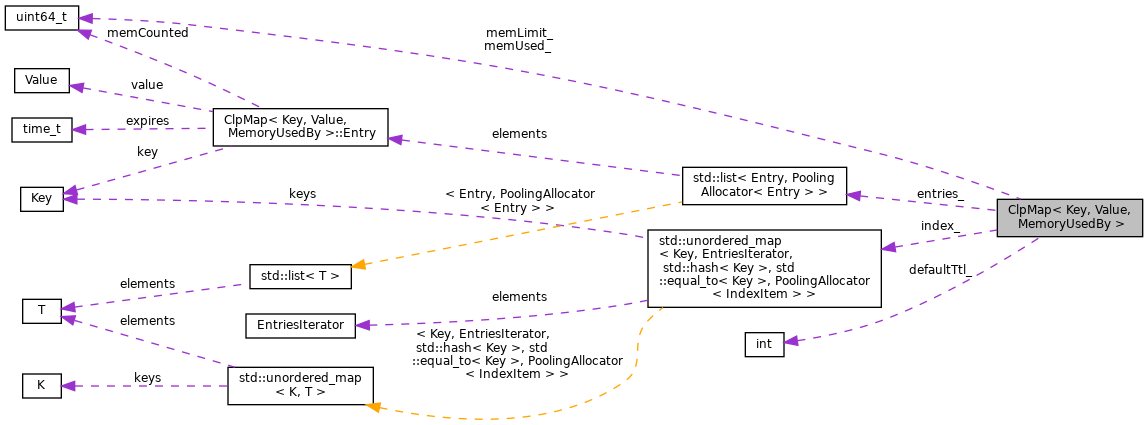
Classes | |
class | Entry |
the keeper of cache entry Key, Value, and caching-related entry metadata More... | |
Public Types | |
using | key_type = Key |
using | mapped_type = Value |
using | Ttl = int |
maximum desired entry caching duration (a.k.a. TTL), in seconds More... | |
using | Entries = std::list< Entry, PoolingAllocator< Entry > > |
Entries in LRU order. More... | |
using | EntriesIterator = typename Entries::iterator |
using | ConstEntriesIterator = typename Entries::const_iterator |
Public Member Functions | |
ClpMap (const uint64_t capacity) | |
ClpMap (uint64_t capacity, Ttl defaultTtl) | |
~ClpMap ()=default | |
ClpMap (const ClpMap &)=delete | |
ClpMap & | operator= (const ClpMap &)=delete |
const Value * | get (const Key &) |
bool | add (const Key &, const Value &, Ttl) |
bool | add (const Key &key, const Value &v) |
Copy the given value into the map (with the given key and default TTL) More... | |
void | del (const Key &) |
Remove the corresponding entry (if any) More... | |
void | setMemLimit (uint64_t newLimit) |
Reset the memory capacity for this map, purging if needed. More... | |
uint64_t | memLimit () const |
The memory capacity for the map. More... | |
uint64_t | freeMem () const |
The free space of the map. More... | |
uint64_t | memoryUsed () const |
The current (approximate) memory usage of the map. More... | |
size_t | entries () const |
The number of currently stored entries, including expired ones. More... | |
ConstEntriesIterator | cbegin () const |
ConstEntriesIterator | cend () const |
ConstEntriesIterator | begin () const |
range-based for loop support; More... | |
ConstEntriesIterator | end () const |
Private Types | |
using | IndexItem = std::pair< const Key, EntriesIterator > |
using | Index = std::unordered_map< Key, EntriesIterator, std::hash< Key >, std::equal_to< Key >, PoolingAllocator< IndexItem > > |
key:entry_position mapping for fast entry lookups by key More... | |
using | IndexIterator = typename Index::iterator |
Private Member Functions | |
void | trim (uint64_t wantSpace) |
purges entries to make free memory large enough to fit wantSpace bytes More... | |
void | erase (const IndexIterator &) |
removes the cached entry (identified by its index) from the map More... | |
IndexIterator | find (const Key &) |
Static Private Member Functions | |
static std::optional< uint64_t > | MemoryCountedFor (const Key &, const Value &) |
Private Attributes | |
Entries | entries_ |
cached entries, including expired ones, in LRU order More... | |
Index | index_ |
entries_ positions indexed by the entry key More... | |
Ttl | defaultTtl_ = std::numeric_limits<Ttl>::max() |
entry TTL to use if none provided to add() More... | |
uint64_t | memLimit_ = 0 |
the maximum memory we are allowed to use for all cached entries More... | |
uint64_t | memUsed_ = 0 |
the total amount of memory we currently use for all cached entries More... | |
Detailed Description
template<class Key, class Value, uint64_t MemoryUsedBy = DefaultMemoryUsage>
class ClpMap< Key, Value, MemoryUsedBy >
An in-memory associative container enforcing three primary caching policies:
- Capacity: The memory used by cached entries has a configurable limit;
- Lifetime: Entries are hidden (and may be deleted) after their TTL expires;
- Priority: Capacity victims are purged in LRU order. Individual cache entry operations have average constant-time complexity.
Value must meet STL requirements of Erasable and EmplaceConstructible. Key must come with std::hash<Key> and std::equal_to<Key> instantiations. Key::length() must return the number of memory bytes in use by the key. MemoryUsedBy() must return the number of memory bytes in use by the value.
Member Typedef Documentation
◆ ConstEntriesIterator
using ClpMap< Key, Value, MemoryUsedBy >::ConstEntriesIterator = typename Entries::const_iterator |
◆ Entries
using ClpMap< Key, Value, MemoryUsedBy >::Entries = std::list<Entry, PoolingAllocator<Entry> > |
◆ EntriesIterator
using ClpMap< Key, Value, MemoryUsedBy >::EntriesIterator = typename Entries::iterator |
◆ Index
|
private |
◆ IndexItem
|
private |
◆ IndexIterator
|
private |
◆ key_type
◆ mapped_type
using ClpMap< Key, Value, MemoryUsedBy >::mapped_type = Value |
◆ Ttl
Constructor & Destructor Documentation
◆ ClpMap() [1/3]
|
inlineexplicit |
Definition at line 70 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::setMemLimit().
◆ ClpMap() [2/3]
ClpMap< Key, Value, MemoryUsedBy >::ClpMap | ( | uint64_t | capacity, |
Ttl | defaultTtl | ||
) |
Definition at line 149 of file ClpMap.h.
References assert, and ClpMap< Key, Value, MemoryUsedBy >::setMemLimit().
◆ ~ClpMap()
|
default |
◆ ClpMap() [3/3]
|
delete |
Member Function Documentation
◆ add() [1/2]
bool ClpMap< Key, Value, MemoryUsedBy >::add | ( | const Key & | key, |
const Value & | v, | ||
Ttl | ttl | ||
) |
Copy the given value into the map (with the given key and TTL)
- Return values
-
true the value was successfully copied into the map false caching was rejected (the map remains unchanged)
Definition at line 220 of file ClpMap.h.
References addedEntry(), and assert.
Referenced by TestClpMap::addOneEntry(), TestClpMap::addSequenceOfEntriesToMap(), TestClpMap::testEntryCounter(), TestClpMap::testNegativeTtl(), and TestClpMap::testPutGetDelete().
◆ add() [2/2]
|
inline |
Definition at line 91 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::add(), and ClpMap< Key, Value, MemoryUsedBy >::defaultTtl_.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::add().
◆ begin()
|
inline |
- See also
- cbegin()
Definition at line 117 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::cbegin().
◆ cbegin()
|
inline |
Read-only traversal of all cached entries in LRU order, least recently used entry first. Stored expired entries (if any) are included. Any map modification may invalidate these iterators and their derivatives.
Definition at line 114 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::entries_.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::begin(), and TestClpMap::testClassicLoopTraversal().
◆ cend()
|
inline |
Definition at line 115 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::entries_.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::end(), and TestClpMap::testClassicLoopTraversal().
◆ del()
void ClpMap< Key, Value, MemoryUsedBy >::del | ( | const Key & | key | ) |
Definition at line 268 of file ClpMap.h.
Referenced by TestClpMap::testMisses(), and TestClpMap::testPutGetDelete().
◆ end()
|
inline |
Definition at line 118 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::cend().
◆ entries()
|
inline |
Definition at line 109 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::entries_.
Referenced by TestClpMap::testConstructor(), TestClpMap::testEntryCounter(), TestClpMap::testMemoryLimit(), and TestClpMap::testMisses().
◆ erase()
|
private |
◆ find()
|
private |
◆ freeMem()
|
inline |
Definition at line 103 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::memLimit(), and ClpMap< Key, Value, MemoryUsedBy >::memoryUsed().
Referenced by TestClpMap::testConstructor().
◆ get()
const Value * ClpMap< Key, Value, MemoryUsedBy >::get | ( | const Key & | key | ) |
- Returns
- a pointer to a fresh cached value (or nil) The underlying value is owned by the map, so the pointer may be invalidated by any non-constant method call, including another get(). Also moves the found entry to the end of the purging queue.
Definition at line 188 of file ClpMap.h.
Referenced by TestClpMap::addOneEntry(), TestClpMap::testMisses(), TestClpMap::testNegativeTtl(), TestClpMap::testPurgeIsLru(), TestClpMap::testPutGetDelete(), TestClpMap::testReplaceEntryWithShorterTtl(), TestClpMap::testTtlExpiration(), and TestClpMap::testZeroTtl().
◆ memLimit()
|
inline |
Definition at line 100 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::memLimit_.
Referenced by TestClpMap::fillMapWithEntries(), ClpMap< Key, Value, MemoryUsedBy >::freeMem(), and TestClpMap::testConstructor().
◆ MemoryCountedFor()
|
staticprivate |
◆ memoryUsed()
|
inline |
Definition at line 106 of file ClpMap.h.
References ClpMap< Key, Value, MemoryUsedBy >::memUsed_.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::freeMem(), TestClpMap::testConstructor(), and TestClpMap::testMemoryLimit().
◆ operator=()
|
delete |
◆ setMemLimit()
void ClpMap< Key, Value, MemoryUsedBy >::setMemLimit | ( | uint64_t | newLimit | ) |
Definition at line 158 of file ClpMap.h.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::ClpMap(), and TestClpMap::testMemoryLimit().
◆ trim()
|
private |
Member Data Documentation
◆ defaultTtl_
|
private |
Definition at line 139 of file ClpMap.h.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::add().
◆ entries_
|
private |
Definition at line 133 of file ClpMap.h.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::cbegin(), ClpMap< Key, Value, MemoryUsedBy >::cend(), and ClpMap< Key, Value, MemoryUsedBy >::entries().
◆ index_
◆ memLimit_
|
private |
Definition at line 142 of file ClpMap.h.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::memLimit().
◆ memUsed_
|
private |
Definition at line 145 of file ClpMap.h.
Referenced by ClpMap< Key, Value, MemoryUsedBy >::memoryUsed().
The documentation for this class was generated from the following file:
- src/base/ClpMap.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products